数学相关的类:
-Math类
1.是final的类
2.构造方法私有,不能创建对象
3.主要用法是Math类提供了大量的静态方法
4.计算小数的时候不够精确
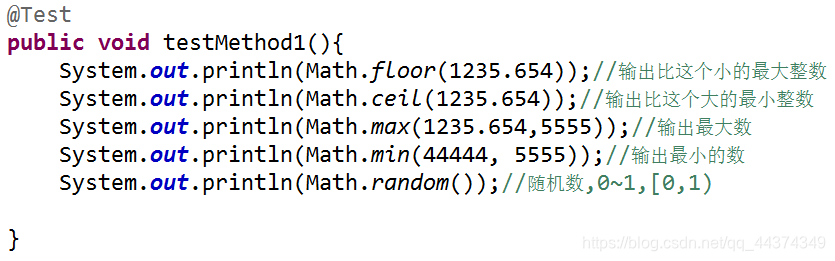
-BigDecimal类:
1.用于精确计算的类
2.在精确计算的时候,要求参数以字符串的方式传入此类的对象
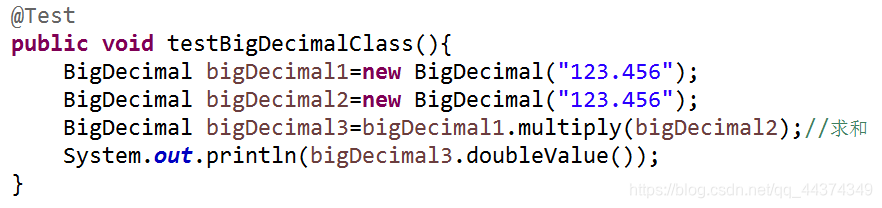
-BigInteger类:
1.用于存储任意大小的整数的类
2.存储数据最好用字符串传入对象
日期相关类:
-Date类:
1.表示日期的类
2.提供很多的操作日期的方法
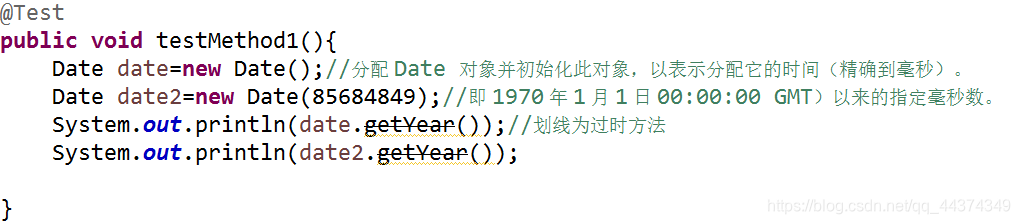
-SimpleDateFormat类:
1.日期的格式类
2.parse()方法将日期对象转换为日期格式的字符串
3.format()方法将日期对象转化为日期格式的字符串
4.在转换的时候,可以提供转换的格式
-Calendar类:
1.有关日历的区,控制时区
2.提供大量的方法来操作时间
3.Calendar类是一个抽象类,不能直接用new来实例化对象
用Calendar cal=Calendar.getInstance();
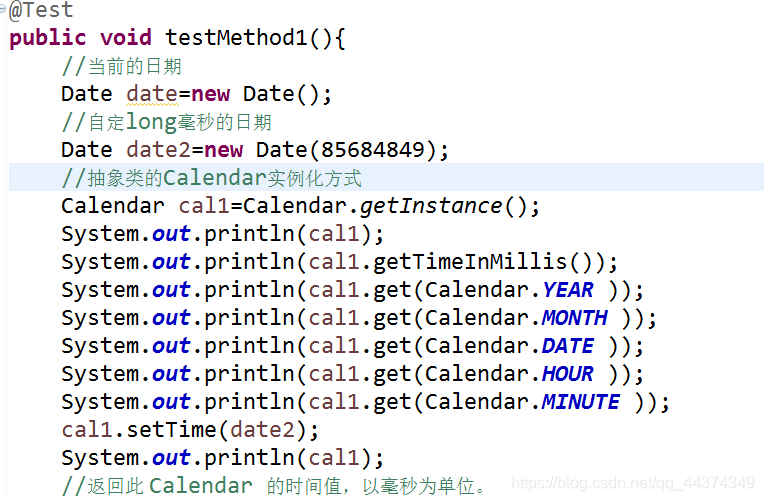
总结:
用Date对象存储日期的数据
用Calendar类的对象操作Date对象中的日期数据
用SimpleDateFormat类做Date对象和日期字符串的互相转换
java IO
input 输入:所有向内存中送数据都是输入
output 输出:所有的从内存取出数据的都是输出
能用java.io包中的api方法操作输入和输出
-内存-->外存(硬盘,光盘,U盘等) 本地流输出
-内存<--外存 本地流输入
结合socket网络编程
内存-->网络上 网络流输出
内存<--网络上 网络流输入
网络流案例:
计算机A和远程计算机B
从计算机B的计算机下载文件到计算机A:
-
先从B计算机的硬盘上本地流输入,把硬盘的文件读入到B的内存中
-
把内存中的文件数据以网络流输出,把内存数据输出到网络上
-
计算机A网络流输入,把网络中的文件数据读入到A的内存中
-
把A内存中的数据,本地流输出到A的硬盘上
上传文件的过程和下载是相反的
不能使用java.io包操作的流:
内存à显示器
内存àCPU
内存ßCPU
数据的持久化:
数据长时间存留在硬盘上
数据长时间保存在数据库中,其实数据库的本质是以数据文件的方式持久化到硬盘上
在硬盘中实际体现的是文件和目录
Java中提供了java.io.File类来操作文件的目录信息和文件信息,但是不能操作文件内容
根据对文件内容的操作分为:
字节流:对文件的内容的读写使用字节的方式操作
字符流:对文件内容的读写视同字符流(ascii)的方式操作
其本质底层还是字节流
java.io.File类:用户表示文件和目录的,与文件内容无关
注意不同操作系统上,对目录的间隔符的区分
Windows: C:\\aa\\bb\\cc.txt
Linux: /home/aa/bb/cc.txt
java中对路径的分隔符表示方式:
windows:
c:\\aa\\bb\\cc.txt
c:/aa/bb/cc.txt
Linux;
C:/aa/bb/cc.txt
如果想兼容Windows和Linux,选择:
"aa"+File.separator+"bb"+File.separator+"cc.txt"
/**
* 构建file对象
* File(String)
*/
@Test
public void testMethod1(){
File file1 = new File("d:\\aa\\aa.txt");
System.out.println(file1);
File file2= new File("d:/aa/aa.txt");
System.out.println(file2);
File file3= new File("d:"+File.separator+"aa"+File.separator+"aa.txt");
System.out.println(file3);
}
File类的api
-构建File类的对象:
File(String path);
File(File parent,String child);
/**
* 构建file对象
* File(File parent,String child)
*/
@Test
public void testMethod2(){
File parent = new File("d:\\aa");
File child =new File(parent,"aa.txt");
System.out.println(child);
}
File(String parentName,String child);
/**
* 构建file对象
* File(String parent,String child)
*/
@Test
public void testMethod3(){
String parent = "d:\\aa";
File child =new File(parent,"aa.txt");
System.out.println(child);
}
isFile():
判断是否是文件
/**
* isFile()
*/
@Test
public void testMethod4(){
File parent = new File("d:\\aa\\");
File child =new File(parent,"aa.txt");
if(child.isFile()){
System.out.println("是文件");
}else{
System.out.println("不是文件");
}
}
isDirectory()
判断文件是否是目录
/**
* isDirectory()
*/
@Test
public void testMethod5(){
String parent = "d:\\aa\\";
File file =new File(parent);
if(file.isDirectory()){
System.out.println("是目录");
}else{
System.out.println("不是目录");
}
}
length()
获取文件的长度
/**
* length
*/
@Test
public void testMethod7(){
String parent = "d:\\aa\\aa.txt";
File file =new File(parent);
System.out.println(file.length());
}
exists()
判断文件是否存在
/**
* exists() 判断文件或者目录是否存在
*/
@Test
public void testMethod8(){
String path = "d:\\aa\\bb\\aa.txt";
File file =new File(path);
System.out.println(file.exists());
String path1="d:/aa/cc";
File file1=new File(path1);
System.out.println(file1.exists());
}
createNewFile()
创建一个空文件,返回值是boolean类型
如果指定的文件不存在,创建文件并返回true
如果指定的文件存在,返回false
/**
* createNewFile() 创建文件
* @throws IOException
*/
@Test
public void testMethod9() throws IOException{
String path = "d:\\aa\\bb\\aa1.txt";
File file =new File(path);
if(!file.exists()){
boolean flag=file.createNewFile();
if(flag){
System.out.println();
System.out.println("文件创建成功");
}else{
System.out.println("文件创建失败");
}
}else{
System.out.println("文件已经存在");
}
}
delete()
删除文件
注意:如果file表示一个目录,删除的时候保证目录是空的
/**
* delete() 能删除目录和文件
*
*/
@Test
public void testMethod10() throws IOException{
String path = "d:\\aa\\bb\\aa1.txt";
File file =new File(path);
if(file.exists()){
boolean flag=file.delete();
if(flag){
System.out.println("删除文件成功");
}else{
System.out.println("删除文件失败");
}
}else{
System.out.println("文件不存在");
}
}
public void deleteFile(File file)throws Exception{
if(file==null){
System.out.println("file为null,不能删除");
}else{
throw new Exception("file不能为null,请指定具体的目录或者文件");
}
if(file.isDirectory()){
//指定的file为目录
File[] files=file.listFiles();//返回指定目录中的所有文件和目录
//遍历所有的fiies,有可能是目录,也可能是文件
for(File f:files){
//递归调用自己,如果是文件直接删除,目录也删除
deleteFile(f);
}
}
//不是目录是文件
file.delete();
}
mkdir()
创建目录
/**
* 创建文件夹
*/
@Test
public void testMethod12(){
File file=new File("d:/aa");
if(!file.exists()){
boolean flag=file.mkdir();
if(flag){
System.out.println("目录创建成功");
}else{
System.out.println("目录创建失败");
}
}else{
System.out.println("目录已经存在");
}
}
mkdirs()
创建多个目录
/**
* 创建多个文件夹
*/
@Test
public void testMethod13(){
File file=new File("d:/aa/bb/cc/dd/ee");
if(!file.exists()){
boolean flag=file.mkdirs();
if(flag){
System.out.println("目录创建成功");
}else{
System.out.println("目录创建失败");
}
}else{
System.out.println("目录已经存在");
}
}
listFile()
返回指定目录中的所有文件和目录
/**
* listFile()
*/
@Test
public void testMethod14(){
File file=new File("d:/aa");
File[] fs=file.listFiles();
for(File f:fs){
System.out.println(f);
}
}
lsitFile(FileFilter)
返回指定目录中的部分文件和目录,用FileFilter设定筛选条件
/**
* listFiles(FileFileter)
*/
@Test
public void testMethod16(){
File file=new File("d:/aa");
if(file.exists()){
File[] fs=file.listFiles(new FileFilter(){
//接口回调,子实现
@Override
public boolean accept(File pathname) {
// TODO Auto-generated method stub
return pathname.getName().endsWith(".txt");
}
});
for(File f:fs){
System.out.println(f);
}
}else{
System.out.println("目录不存在");
}
}
LsitFile(FilenameFilter)
返回指定目录中的部分文件和目录,用FileFilter设定筛选条件
/**
* listFiles(FilenameFileter)
*/
@Test
public void testMethod15(){
File file=new File("d:/aa");
if(file.exists()){
File[] fs=file.listFiles(new FilenameFilter(){
//接口回调,子实现
@Override
public boolean accept(File dir, String name) {
// TODO Auto-generated method stub
return name.endsWith(".txt");
}
});
for(File f:fs){
System.out.println(f);
}
}else{
System.out.println("目录不存在");
}
}
总结:
-
只能操作文件或者目录的信息
-
就是不能操作文件内容
RandomAccessFile类:
可以操作文件内容,按照字节流字符流
此类特殊,read读和write写都是此类中的api方法
能够通过seek方法随意改动/移动动文件指针
RandomAccessFile类对文件的随机访问分为两种模式;
只读模式
读写模式
创建对象:
RandomAccessFile(File file,String mode);
从文件中读取和向其中写入随机访问流(字节流)
文件通过File指定
模式通过String指定
RandomAccessFile(String name,String mode)
从文件中读取和写入随机访问流(字节流)\
文件通过String指定
模式通过String指定
mode取值:
“r”:只读模式 read
“rw”:读写模式 read write
/**
* RandomAccessFile(File file,String mode);
* RandomAccessFile(String name,String mode);
* @throws Exception
*/
@Test
public void testMethod() throws Exception{
RandomAccessFile raf1=new RandomAccessFile(new File("d:/aa/aa.txt"), "r");
RandomAccessFile raf2=new RandomAccessFile("d:/aa/aa.txt", "rw");
//读写文件的数据
System.out.println(raf1.getFilePointer());//获取当前指针位置
System.out.println(raf2.getFilePointer());
raf1.close();//开流记得关流
raf2.close();
}
写入操作:
void write(int d):
此方法根据当前指针所指的位置处写入一个字节
/**
* write (int )
* @throws Exception
*/
@Test
public void testMethod2() throws Exception{
RandomAccessFile raf1=new RandomAccessFile(new File("d:/aa/aa.txt"), "rw");
//一旦重新代开文件,指针就会从头开始
raf1.write(65);
System.out.println(raf1.getFilePointer());//获取当前指针位置
//读写文件的数据
raf1.close();
}
void write(byte[] b)
此方法根据当前指针所在的位置处写入一组字节
/**
* write (byte[] buf)
* @throws Exception
*/
@Test
public void testMethod4() throws Exception{
RandomAccessFile raf1=new RandomAccessFile(new File("d:/aa/aa.txt"), "rw");
raf1.write("hello world".getBytes());
System.out.println(raf1.getFilePointer());//获取当前指针位置
//读写文件的数据
raf1.close();
}
void write(byte[] b,int offset,int len);
此方法将len个字节从指定的byte数组写入文件,并且从偏移量offset开始
/**
* write (byte[] buf,int offset,int len)
* @throws Exception
*/
@Test
public void testMethod6() throws Exception{
RandomAccessFile raf1=new RandomAccessFile(new File("d:/aa/aa.txt"), "rw");
raf1.write("hello world".getBytes(),0,6);
System.out.println(raf1.getFilePointer());//获取当前指针位置
//读写文件的数据
raf1.close();
}
读取操作:
int read()
从文件中读取出一个byte字节,填充到整型的低八位中
如果返回-1,则表明读取到文件末尾EOF end of file
/**
* read (int )
* @throws Exception
*/
@Test
public void testMethod3() throws Exception{
RandomAccessFile raf1=new RandomAccessFile(new File("d:/aa/aa.txt"), "r");
int d=raf1.read();
System.out.println(raf1.getFilePointer());//获取当前指针位置
System.out.println(d);
//读写文件的数据
raf1.close();
}
int read(byte[] b)
从指针执行的位置开始读取若干个字节,存储到字节数组中
将读取到的字节按照顺序放置在字节数组对应的位置上(从0开始)
返回值为读取到的字节数,也可以说成读取到的长度
返回值为-1,则读取到文件的末尾
/**
* read (byte[] buf)
* @throws Exception
*/
@Test
public void testMethod5() throws Exception{
RandomAccessFile raf1=new RandomAccessFile(new File("d:/aa/aa.txt"), "r");
byte[] buf=new byte[3];
int len=raf1.read(buf);
System.out.println(raf1.getFilePointer()+" "+new String(buf)+len);
//获取当前指针位置
System.out.println();
len=raf1.read(buf);
System.out.println(raf1.getFilePointer()+" "+new String(buf)+len);
//获取当前指针位置
System.out.println();
len=raf1.read(buf);
System.out.println(raf1.getFilePointer()+" "+new String(buf)+len);
//获取当前指针位置
System.out.println();
len=raf1.read(buf);
System.out.println(raf1.getFilePointer()+" "+new String(buf)+len);
//获取当前指针位置
System.out.println();
//读写文件的数据
raf1.close();
}
int read(byte[] b,int offset,int len)
将最多len个数据读入到byte数组中,并从偏移量offset开始
/**
* read (byte[] buf,int offset,int len)
* @throws Exception
*/
@Test
public void testMethod7() throws Exception{
RandomAccessFile raf1=new RandomAccessFile(new File("d:/aa/aa.txt"), "r");
byte[] buf=new byte[5];
int len=raf1.read(buf,1,3);
System.out.println(raf1.getFilePointer()+" "+new String(buf)+len);//获取当前指针位置
System.out.println();
//读写文件的数据
raf1.close();
}
void getFilePointer()
返回此文件中当前的偏移量
/**
* getFilePointer()
* @throws Exception
*/
@Test
public void testMethod8() throws Exception{
RandomAccessFile raf1=new RandomAccessFile(new File("d:/aa/aa.txt"), "rw");
raf1.write('a');
System.out.println(raf1.getFilePointer());
raf1.write(1000);
System.out.println(raf1.getFilePointer());
//读写文件的数据
raf1.close();
}
void seek(long position)
设置到此文件开头文件指针的偏移量
在该位置发生下一个读取或者写入的操作
/**
* seek(long)
*/
@Test
public void testMethod9() throws Exception{
RandomAccessFile raf1=new RandomAccessFile(new File("d:/aa/aa.txt"), "rw");
raf1.seek(4);
int d=raf1.read();
System.out.println(d);
System.out.println(raf1.getFilePointer());
raf1.seek(4);
d=raf1.read();
System.out.println(d);
System.out.println(raf1.getFilePointer());
//读写文件的数据
raf1.close();
}
int skip(int n)
利用此方法可以跳过一些(n)字节,但是n只能是整数
/**
* skipBytes(int)
*/
@Test
public void testMethod10() throws Exception{
RandomAccessFile raf1=new RandomAccessFile(new File("d:/aa/aa.txt"), "rw");
raf1.seek(4);
int d=raf1.read();
System.out.println(d);
System.out.println(raf1.getFilePointer());
raf1.skipBytes(1);
d=raf1.read();
System.out.println(d);
System.out.println(raf1.getFilePointer());
//读写文件的数据
raf1.close();
}