数据结构》严奶奶版本—树(1)二叉树 完整源码
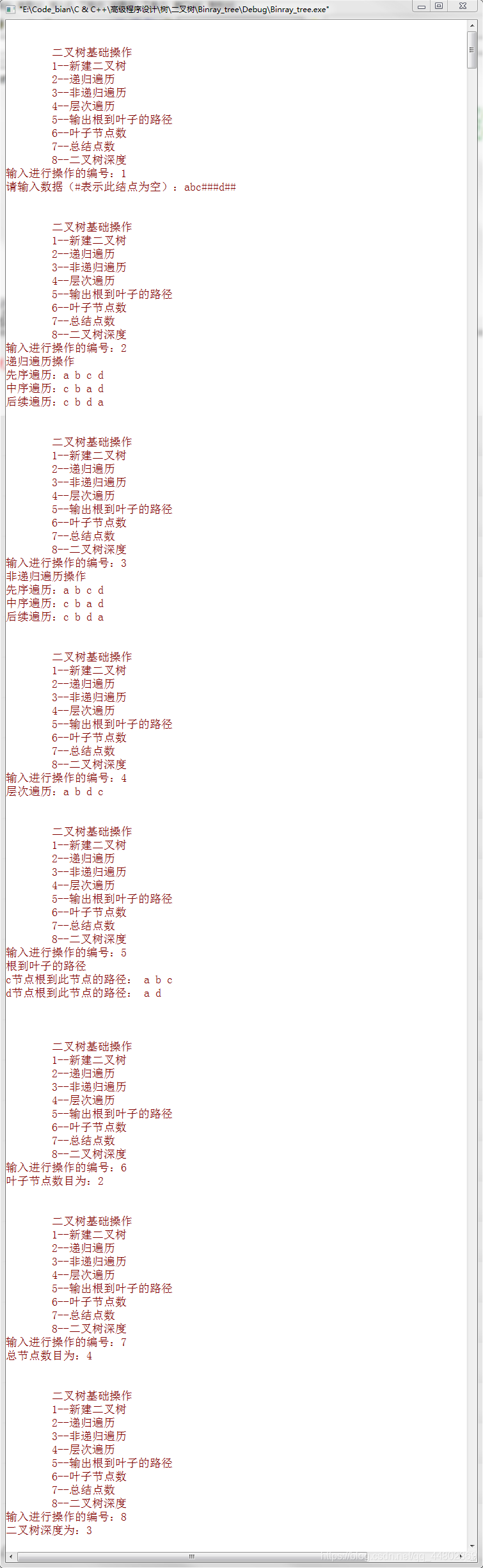
#include <iostream>
#include <windows.h>
#include <string>
using namespace std;
#define MAX_SIZE 100
typedef char ElemType;
typedef struct btnode{
ElemType data;
struct btnode *lchild;
struct btnode *rchild;
}BTNode,*BTree;
typedef struct sqstack{
BTree *top;
BTree *base;
int stack_size;
}SqStack;
bool init_stack(SqStack &sq);
bool push(SqStack &sq,BTree b);
bool pop(SqStack &sq,BTree &b);
bool judge_empty(SqStack sq);
bool get_top(SqStack sq,BTree &b);
typedef struct squeue{
BTree *front;
BTree *rail;
int queue_size;
}SQueue;
bool init_queue(SQueue &s);
bool en_queue(SQueue &s,BTree b);
bool de_queue(SQueue &s,BTree &b);
bool judge_empty(SQueue s);
bool get_front(SQueue s,BTree &b);
void create_btree(BTree &bt);
void dg_preorder(BTree bt);
void dg_inorder(BTree bt);
void dg_postorder(BTree bt);
void fdg_preorder(BTree bt);
void fdg_inorder(BTree bt);
void fdg_postorder(BTree bt);
void cxorder(BTree bt);
void print_path(BTree bt,char path[],int &path_length);
void counts_leaf(BTree bt,int &count);
void counts_nodes(BTree bt,int &count);
int tree_height(BTree bt);
void show();
void switch_channel(int channel);
BTree bt;
int count=0;
char path[100];
int path_length=0;
int main()
{
int channel;
do{
show();
cout<<"输入进行操作的编号:";
cin>>channel;
switch_channel(channel);
}while(1);
return 0;
}
void show()
{
cout<<endl<<endl<<"\t二叉树基础操作\n";
cout<<"\t1--新建二叉树\n";
cout<<"\t2--递归遍历\n";
cout<<"\t3--非递归遍历\n";
cout<<"\t4--层次遍历\n";
cout<<"\t5--输出根到叶子的路径\n";
cout<<"\t6--叶子节点数\n";
cout<<"\t7--总结点数\n";
cout<<"\t8--二叉树深度\n";
}
void switch_channel(int channel)
{
switch(channel)
{
case 1:
{
cout<<"请输入数据(#表示此结点为空):";
create_btree(bt);
}break;
case 2:
{ cout<<"递归遍历操作\n";
cout<<"先序遍历:";
dg_preorder(bt);
cout<<endl;
cout<<"中序遍历:";
dg_inorder(bt);
cout<<endl;
cout<<"后续遍历:";
dg_postorder(bt);
cout<<endl;
}break;
case 3:
{
cout<<"非递归遍历操作\n";
cout<<"先序遍历:";
fdg_preorder(bt);
cout<<endl;
cout<<"中序遍历:";
fdg_inorder(bt);
cout<<endl;
cout<<"后续遍历:";
fdg_postorder(bt);
cout<<endl;
}break;
case 4:
{
cout<<"层次遍历:";
cxorder(bt);
cout<<endl;
}break;
case 5:
{
cout<<"根到叶子的路径\n";
print_path(bt,path,path_length);
cout<<endl;
}break;
case 6:
{ count=0;
counts_leaf(bt,count);
cout<<"叶子节点数目为:"<<count<<endl;
}break;
case 7:
{ count=0;
counts_nodes(bt,count);
cout<<"总节点数目为:"<<count<<endl;
}break;
case 8:
{
count = tree_height(bt);
cout<<"二叉树深度为:"<<count<<endl;
}break;
default:exit(1);break;
}
}
bool init_stack(SqStack &sq)
{
sq.top = new BTree[MAX_SIZE];
if(!sq.top)
{
cout<<"初始化栈失败"<<endl;
return false;
}
sq.base = sq.top;
sq.stack_size = MAX_SIZE;
return true;
}
bool push(SqStack &sq,BTree b)
{
*sq.top = b;
sq.top++;
return true;
}
bool pop(SqStack &sq,BTree &b)
{
if(judge_empty(sq))
return false;
sq.top--;
b = *sq.top;
return true;
}
bool judge_empty(SqStack sq)
{
if(sq.base == sq.top)
return true;
return false;
}
bool get_top(SqStack sq,BTree &b)
{
if(judge_empty(sq))
return false;
b = *(sq.top-1);
return true;
}
bool init_queue(SQueue &s)
{
s.front = new BTree[MAX_SIZE];
if(!s.front)
{
cout<<"初始化队列失败"<<endl;
return false;
}
s.rail = s.front;
s.queue_size = MAX_SIZE;
return true;
}
bool en_queue(SQueue &s,BTree b)
{
s.rail++;
*(s.rail) = b;
return true;
}
bool de_queue(SQueue &s,BTree &b)
{
if(judge_empty(s))
return false;
s.front++;
b = *(s.front);
return true;
}
bool judge_empty(SQueue s)
{
if(s.front == s.rail)
return true;
return false;
}
bool get_front(SQueue s,BTree &b)
{
if(judge_empty(s))
return false;
b = *(s.front+1);
return true;
}
void create_btree(BTree &bt)
{
ElemType data;
cin>>data;
if(data == '#')
bt = NULL;
else
{
bt = new BTNode;
bt->data = data;
create_btree(bt->lchild);
create_btree(bt->rchild);
}
}
void dg_preorder(BTree bt)
{
if(bt)
{
if(bt->data != '#')
cout<<bt->data<<" ";
dg_preorder(bt->lchild);
dg_preorder(bt->rchild);
}
}
void dg_inorder(BTree bt)
{
if(bt)
{
dg_inorder(bt->lchild);
if(bt->data != '#')
cout<<bt->data<<" ";
dg_inorder(bt->rchild);
}
}
void dg_postorder(BTree bt)
{
if(bt)
{
dg_postorder(bt->lchild);
dg_postorder(bt->rchild);
if(bt->data != '#')
cout<<bt->data<<" ";
}
}
void fdg_preorder(BTree bt)
{
SqStack sq;
init_stack(sq);
BTree p = bt;
while(p || !judge_empty(sq))
{
if(p)
{
cout<<p->data<<" ";
push(sq,p);
p = p->lchild;
}
else
{
pop(sq,p);
p = p->rchild;
}
}
}
void fdg_inorder(BTree bt)
{
SqStack sq;
init_stack(sq);
BTree p = bt;
while(p || !judge_empty(sq))
{
if(p)
{
push(sq,p);
p = p->lchild;
}
else
{
pop(sq,p);
cout<<p->data<<" ";
p = p->rchild;
}
}
}
void fdg_postorder(BTree bt)
{
SqStack sq;
BTree p = bt;
BTree lv = NULL;
init_stack(sq);
while(p || !judge_empty(sq))
{
while(p)
{
push(sq,p);
p = p->lchild;
}
get_top(sq,p);
if(!p->rchild || lv == p->rchild)
{
cout<<p->data<<" ";
pop(sq,lv);
p = NULL;
}
else
p = p->rchild;
}
}
void counts_leaf(BTree bt,int &count)
{
if(bt == NULL)
return ;
if(bt->lchild == NULL && bt->rchild == NULL)
count++;
counts_leaf(bt->lchild,count);
counts_leaf(bt->rchild,count);
}
void counts_nodes(BTree bt,int &count)
{
if(bt == NULL)
return ;
else
{
count++;
counts_nodes(bt->lchild,count);
counts_nodes(bt->rchild,count);
}
}
int tree_height(BTree bt)
{
if(bt == NULL)
return 0;
int l_height = tree_height(bt->lchild);
int r_height = tree_height(bt->rchild);
return l_height > r_height ? l_height + 1 : r_height + 1;
}
void cxorder(BTree bt)
{
SQueue q;
init_queue(q);
if(bt!=NULL)
en_queue(q,bt);
while(!judge_empty(q))
{
de_queue(q,bt);
cout<<bt->data<<" ";
if(bt->lchild != NULL)1
en_queue(q,bt->lchild);
if(bt->rchild != NULL)
en_queue(q,bt->rchild);
}
}
void print_path(BTree bt,char path[],int &path_length)
{
if(bt)
{
if(bt->lchild == NULL && bt->rchild == NULL)
{
path[path_length] = bt->data;
cout<<bt->data<<"节点根到此节点的路径: ";
for(count = 0;count<=path_length; count++)
{
cout<<path[count]<<" ";
}
cout<<endl;
}
else
{
path[path_length++] = bt->data;
print_path(bt->lchild,path,path_length);
print_path(bt->rchild,path,path_length);
path_length--;
}
}
}