目录
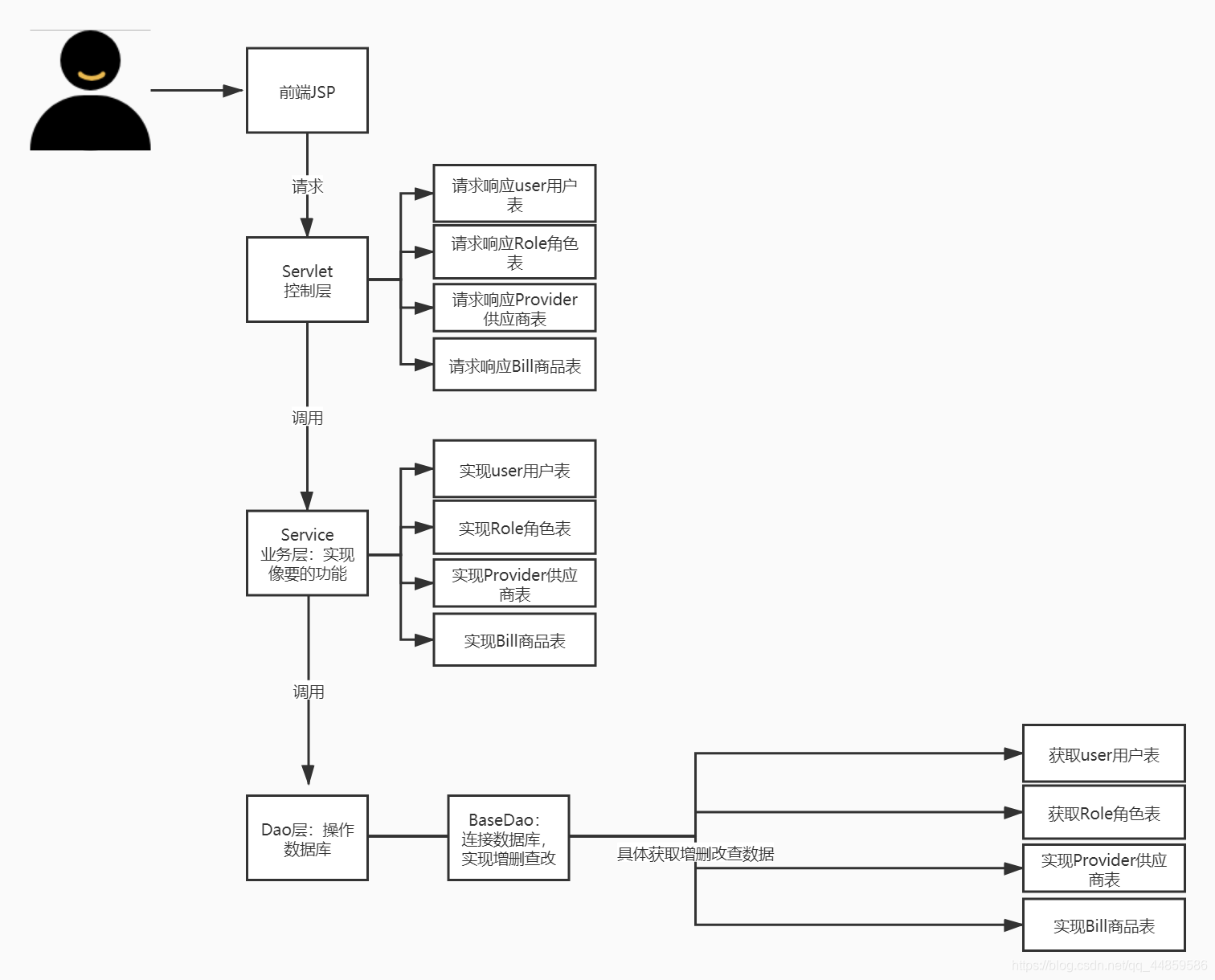
前端代码省略,之后补一个完整压缩包
1.项目搭建
1.1搭建一个maven web项目
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0"
metadata-complete="true">
</web-app>
1.2配置Tomcat
1.3测试项目是否能运行
1.4导入包(Servlet,javax-jsp,mysq,JSTLl,standard)
<dependencies>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>servlet-api</artifactId>
<version>2.5</version>
</dependency>
<dependency>
<groupId>javax.servlet.jsp</groupId>
<artifactId>javax.servlet.jsp-api</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.20</version>
</dependency>
<!--JSTL表达式的依赖-->
<dependency>
<groupId>javax.servlet.jsp.jstl</groupId>
<artifactId>jstl-api</artifactId>
<version>1.2</version>
</dependency>
<!--standard标签库-->
<dependency>
<groupId>taglibs</groupId>
<artifactId>standard</artifactId>
<version>1.1.2</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
</dependency>
1.5.创建项目包结构
1.6编写实体类
1.6.1 idea连接数据库!
1.6.2 ORM映射:表-类映射
1.7 编写基础公共类(Dao层)
1.7.1 数据库配置文件
driver=com.mysql.cj.jdbc.Driver
url=jdbc:mysql://192.168.43.88:3306/smbms?useSSL=false&useUnicode=true&serverTimezone=Asia/Shanghai
username=root
password=123456
1.7.2 编写数据库的公共类(获取+连接+增删改查+关闭)
package com.node.dao;
import java.io.IOException;
import java.io.InputStream;
import java.sql.*;
import java.util.Properties;
public class BaseDao {
private static String driver;
private static String url;
private static String username;
private static String password;
//静态代码块,类加载的时候就初始化了
static {
Properties properties = new Properties();
//通过类加载器读取对应的资源
String configFile = "db.properties";
InputStream resourceAsStream = BaseDao.class.getClassLoader().getResourceAsStream(configFile);
try {
properties.load(resourceAsStream);
} catch (IOException e) {
e.printStackTrace();
}
driver = properties.getProperty("driver");
url = properties.getProperty("url");
username = properties.getProperty("username");
password = properties.getProperty("password");
}
//获取数据库连接
public static Connection getConnection() {
Connection connection = null;
try {
Class.forName(driver);
connection = DriverManager.getConnection(url, username, password);
} catch (Exception e) {
e.printStackTrace();
}
return connection;
}
//编写查询公共方法
//ResultSet:查询结果集 connection:连接数据库 sql:具体值 params:具体值集合 PreparedStatement:代表sql语句,执行sql语句,且防止sql注入
public static ResultSet execute(Connection connection, PreparedStatement pstm, ResultSet rs, String sql, Object[] params) throws Exception {
//预编译的sql,在后面直接执行就可以了
pstm= connection.prepareStatement(sql);
for (int i = 0; i < params.length; i++) {
pstm.setObject(i + 1, params[i]);//setObject:占位符从1开始,数组从0开始,所以+1;
}
rs = pstm.executeQuery();
return rs;
}
//编写增删改公共方法
// connection:连接数据库 sql:具体值 params:具体值集合 PreparedStatement:代表sql语句,执行sql语句,且防止sql注入
public static int execute(Connection connection, PreparedStatement pstm, String sql, Object[] params) throws Exception {
pstm = connection.prepareStatement(sql);
int updateRows = 0;
for (int i = 0; i < params.length; i++) {
pstm.setObject(i + 1, params[i]);//setObject:占位符从1开始,数组从0开始,所以+1;
}
updateRows = pstm.executeUpdate();
return updateRows;
}
//释放资源
public static boolean closeResource(Connection connection, PreparedStatement pstm, ResultSet rs) {
boolean flag = true;
if (rs != null) {
try {
rs.close();
rs = null;//如果关闭成功了,把resultSet为空
} catch (SQLException throwables) {
throwables.printStackTrace();
flag = false;//如果关闭失败了,把flag为空
}
}
if (pstm != null) {
try {
pstm.close();
pstm = null;//如果关闭成功了,把resultSet为空
} catch (SQLException throwables) {
throwables.printStackTrace();
flag = false;//如果关闭失败了,把flag为空
}
}
if (connection != null) {
try {
connection.close();
connection = null;//如果关闭成功了,把resultSet为空
} catch (SQLException throwables) {
throwables.printStackTrace();
flag = false;//如果关闭失败了,把flag为空
}
}
return flag;
}
}
1.8编写过滤器
package com.node.fileter;
import javax.servlet.*;
import java.io.IOException;
public class CharacterEncodingFilter implements Filter {
public void init(FilterConfig filterConfig) throws ServletException {
}
public void doFilter(ServletRequest servletRequest, ServletResponse servletResponse, FilterChain filterChain) throws IOException, ServletException {
servletRequest.setCharacterEncoding("utf-8");
servletResponse.setCharacterEncoding("utf-8");
servletResponse.setContentType("text/html;charset=UTF-8");
filterChain.doFilter(servletRequest,servletResponse);
}
public void destroy() {
}
}
1.9导入静态资源包(hmtl+css+JavaScript)
2.登录界面
2.1编写前端页面(login.jsp)
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<html>
<head lang="en">
<meta charset="UTF-8">
<title>系统登录 - 超市订单管理系统</title>
<link type="text/css" rel="stylesheet" href="${pageContext.request.contextPath }/css/style.css" />
<script type="text/javascript">
/* if(top.location!=self.location){
top.location=self.location;
} */
</script>
</head>
<body class="login_bg">
<section class="loginBox">
<header class="loginHeader">
<h1>超市订单管理系统</h1>
</header>
<section class="loginCont">
<form class="loginForm" action="${pageContext.request.contextPath }/login.do" name="actionForm" id="actionForm" method="post" >
<div class="info">${error }</div>
<div class="inputbox">
<label for="userCode">用户名:</label>
<input type="text" class="input-text" id="userCode" name="userCode" placeholder="请输入用户名" required/>
</div>
<div class="inputbox">
<label for="userPassword">密码:</label>
<input type="password" id="userPassword" name="userPassword" placeholder="请输入密码" required/>
</div>
<div class="subBtn">
<input type="submit" value="登录"/>
<input type="reset" value="重置"/>
</div>
</form>
</section>
</section>
</body>
</html>
2.2在web.xml中配置首页
<welcome-file-list>
<welcome-file>login.jsp</welcome-file>
</welcome-file-list>
2.3编写dao层得到用户登陆的接口和实现类
package com.node.dao.user;
import com.node.pojo.User;
import java.sql.Connection;
import java.sql.SQLException;
public interface UserDao {
//得到要登陆的用户
//connection:需要连接
//userCode通过用户编码找到
public User getLoginUser(Connection connection,String userCode) throws SQLException;
}
package com.node.dao.user;
import com.node.dao.BaseDao;
import com.node.pojo.User;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class UserDaoImpl implements UserDao{
//得到要登陆的用户
public User getLoginUser(Connection connection, String userCode) throws Exception {
PreparedStatement pstm = null;
ResultSet rs = null;
User user = null;
//判断connection连接不为空
if (connection != null) {
String sql = "select * from smbms_user where userCode=?";
Object[] params = {userCode};
//调用BaseDao的查询方法
rs = BaseDao.execute(connection, pstm, rs, sql, params);
if(rs.next()){
user = new User();
user.setId(rs.getInt("id"));
user.setUserCode(rs.getString("userCode"));
user.setUserName(rs.getString("userName"));
user.setUserPassword(rs.getString("userPassword"));
user.setGender(rs.getInt("gender"));
user.setBirthday(rs.getDate("birthday"));
user.setPhone(rs.getString("phone"));
user.setAddress(rs.getString("address"));
user.setUserRole(rs.getInt("userRole"));
user.setCreatedBy(rs.getInt("createdBy"));
user.setCreationDate(rs.getTimestamp("creationDate"));
user.setModifyBy(rs.getInt("modifyBy"));
user.setModifyDate(rs.getTimestamp("modifyDate"));
}
//没有实例化connection,则为空
BaseDao.closeResource(null,pstm,rs);
}
return user;
}
}
2.4编写业务层,实现用户登陆
package com.node.service.User;
import com.node.pojo.User;
import java.sql.SQLException;
public interface UserService {
public User login(String userCode,String password) throws SQLException;
}
package com.node.service.User;
import com.node.dao.BaseDao;
import com.node.dao.user.UserDao;
import com.node.dao.user.UserDaoImpl;
import com.node.pojo.User;
import org.junit.Test;
import java.sql.Connection;
import java.sql.SQLException;
public class UserServiceImpl implements UserService{
//业务层都会调用Dao层(Dao层只做数据库操作,实现获取数据),业务层是完成实际操作
//引入Dao层
private UserDao userDao;
public UserServiceImpl(){
userDao = new UserDaoImpl();
}
public User login(String userCode, String userPassword) {
Connection connection=null;
User user=null;
try {
connection= BaseDao.getConnection();//连接数据库
user=userDao.getLoginUser(connection,userCode);//通过业务层调用对应的数据库操作(查询)
} catch (Exception throwables) {
throwables.printStackTrace();
} finally {
BaseDao.closeResource(connection,null,null);
}
return user;
}
@Test
public void test() { //测试是否能够连接上,测完删
UserServiceImpl userService = new UserServiceImpl();
User admin = userService.login("admin", "1234567");
System.out.println(admin.getUserPassword());
}
}
2.5编写Servlet,处理web登陆的请求
2.5.1编写主页(jsp)
frame.jsp
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%@include file="/jsp/common/head.jsp"%>
<div class="right">
<img class="wColck" src="${pageContext.request.contextPath }/images/clock.jpg" alt=""/>
<div class="wFont">
<h2>${userSession.userName }</h2>
<p>欢迎来到超市订单管理系统!</p>
</div>
</div>
</section>
<%@include file="/jsp/common/foot.jsp" %>
foot.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<footer class="footer">
超市订单管理系统
</footer>
<script type="text/javascript" src="${pageContext.request.contextPath }/js/time.js"></script>
<script type="text/javascript" src="${pageContext.request.contextPath }/js/jquery-1.8.3.min.js"></script>
<script type="text/javascript" src="${pageContext.request.contextPath }/js/common.js"></script>
<script type="text/javascript" src="${pageContext.request.contextPath }/calendar/WdatePicker.js"></script>
</body>
</html>
head.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/fmt" prefix="fmt" %>
<html>
<head lang="en">
<meta charset="UTF-8">
<title>超市订单管理系统</title>
<link type="text/css" rel="stylesheet" href="${pageContext.request.contextPath }/css/style.css" />
<link type="text/css" rel="stylesheet" href="${pageContext.request.contextPath }/css/public.css" />
</head>
<body>
<!--头部-->
<header class="publicHeader">
<h1>超市订单管理系统</h1>
<div class="publicHeaderR">
<p><span>下午好!</span><span style="color: #fff21b"> ${userSession.userName }</span> , 欢迎你!</p>
<a href="${pageContext.request.contextPath }/jsp/logout.do">退出</a>
</div>
</header>
<!--时间-->
<section class="publicTime">
<span id="time">2015年1月1日 11:11 星期一</span>
<a href="#">温馨提示:为了能正常浏览,请使用高版本浏览器!(IE10+)</a>
</section>
<!--主体内容-->
<section class="publicMian ">
<div class="left">
<h2 class="leftH2"><span class="span1"></span>功能列表 <span></span></h2>
<nav>
<ul class="list">
<li ><a href="${pageContext.request.contextPath }/jsp/bill.do?method=query">订单管理</a></li>
<li><a href="${pageContext.request.contextPath }/jsp/provider.do?method=query">供应商管理</a></li>
<li><a href="${pageContext.request.contextPath }/jsp/user.do?method=query">用户管理</a></li>
<li><a href="${pageContext.request.contextPath }/jsp/pwdmodify.jsp">密码修改</a></li>
<li><a href="${pageContext.request.contextPath }/jsp/logout.do">退出系统</a></li>
</ul>
</nav>
</div>
<input type="hidden" id="path" name="path" value="${pageContext.request.contextPath }"/>
<input type="hidden" id="referer" name="referer" value="<%=request.getHeader("Referer")%>"/>
2.5.2编写Servlet
package com.node.servlet.user;
import com.node.pojo.User;
import com.node.service.User.UserService;
import com.node.service.User.UserServiceImpl;
import com.node.util.Constants;
import org.omg.PortableInterceptor.USER_EXCEPTION;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class LoginServlet extends HttpServlet {
//Servlet:控制层调用业务层
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("LonginServlet--start...");
//获取用户名和密码,根据jsp前端和User实体类
String userCode=req.getParameter("userCode");
String userPassword=req.getParameter("userPassword");
//和数据库中的密码进行对比,调用业务层;
UserService userService = new UserServiceImpl();
User user = userService.login(userCode, userPassword);
if(user!=null){ //如果有这个人,则可以登陆
//将用户的信息放到Session中,键:Constants.USER_SESSION,值:user
req.getSession().setAttribute(Constants.USER_SESSION,user);
//跳转到主页,重定向
resp.sendRedirect("jsp/frame.jsp");
}else {
//如果找不到,则无法登陆,且转发回登陆页面,并提示用户名和密码错误
req.setAttribute("error","用户名或密码不正确");
req.getRequestDispatcher("login.jsp").forward(req,resp);
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
}
package com.node.util;
public class Constants {
//需要一个常量保存会话
public final static String USER_SESSION="userSession";
}
2.6注册Servlet
<servlet>
<servlet-name>LoginServlet</servlet-name>
<servlet-class>com.node.servlet.user.LoginServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>LoginServlet</servlet-name>
<url-pattern>/login.do</url-pattern>
</servlet-mapping>
2.7测试登陆
3.登陆功能优化
3.1主页注销按钮
package com.node.servlet.user;
import com.node.util.Constants;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class LoginoutServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//移除用户的Session
req.getSession().removeAttribute(Constants.USER_SESSION);
/* 返回登陆页面,重定向(req.getContextPath():为了拿到项目下的路径,以防找不到)
如果是jsp/frame.jsp 则不需要查询项目下的路径
*/
resp.sendRedirect(req.getContextPath()+"/login.jsp");
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
}
<servlet>
<servlet-name>LoginoutServlet</servlet-name>
<servlet-class>com.node.servlet.user.LoginoutServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>LoginoutServlet</servlet-name>
<url-pattern>/jsp/logout.do</url-pattern>
</servlet-mapping>
3.2 登陆拦截优化
防止退出后这次复制登陆主页连接会直接进入
error.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
你要访问的页面,已经飞往火星!
<a href="javascript:window.history.back(-1);">返回</a>
</body>
</html>
3.2.1实现
package com.node.fileter;
import com.node.pojo.User;
import com.node.util.Constants;
import javax.servlet.*;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class SysFilter implements Filter {//登陆拦截优化
public void init(FilterConfig filterConfig) throws ServletException {
}
public void doFilter(ServletRequest servletRequest, ServletResponse servletResponse, FilterChain filterChain) throws IOException, ServletException {
HttpServletRequest request=(HttpServletRequest) servletRequest;
HttpServletResponse response=(HttpServletResponse) servletResponse;
//从Session中获取用户
User user = (User) request.getSession().getAttribute(Constants.USER_SESSION);
if(user==null){
response.sendRedirect(request.getContextPath()+"/error.jsp");
}else {
filterChain.doFilter(request,response);
}
}
public void destroy() {
}
}
<filter>
<filter-name>SysFilter</filter-name>
<filter-class>com.node.fileter.SysFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>SysFilter</filter-name>
<url-pattern>/jsp/*</url-pattern>
</filter-mapping>
4.密码修改
存在的问题:无法把新修复的密码传入到数据库中
4.1修改新密码
pwdmodify.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@include file="/jsp/common/head.jsp"%>
<div class="right">
<div class="location">
<strong>你现在所在的位置是:</strong>
<span>密码修改页面</span>
</div>
<div class="providerAdd">
<form id="userForm" name="userForm" method="post" action="${pageContext.request.contextPath }/jsp/user.do">
<input type="hidden" name="method" value="savepwd">
<!--div的class 为error是验证错误,ok是验证成功-->
<div class="info">${message}</div>
<div class="">
<label for="oldPassword">旧密码:</label>
<input type="password" name="oldpassword" id="oldpassword" value="">
<font color="red"></font>
</div>
<div>
<label for="newPassword">新密码:</label>
<input type="password" name="newpassword" id="newpassword" value="">
<font color="red"></font>
</div>
<div>
<label for="rnewpassword">确认新密码:</label>
<input type="password" name="rnewpassword" id="rnewpassword" value="">
<font color="red"></font>
</div>
<div class="providerAddBtn">
<!--<a href="#">保存</a>-->
<input type="button" name="save" id="save" value="保存" class="input-button">
</div>
</form>
</div>
</div>
</section>
<%@include file="/jsp/common/foot.jsp" %>
<script type="text/javascript" src="${pageContext.request.contextPath }/js/pwdmodify.js"></script>
pwdmodify.js
var oldpassword = null;
var newpassword = null;
var rnewpassword = null;
var saveBtn = null;
$(function(){
oldpassword = $("#oldpassword");
newpassword = $("#newpassword");
rnewpassword = $("#rnewpassword");
saveBtn = $("#save");
oldpassword.next().html("*");
newpassword.next().html("*");
rnewpassword.next().html("*");
oldpassword.on("blur",function(){
$.ajax({
type:"GET",
url:path+"/jsp/user.do",
data:{method:"pwdmodify",oldpassword:oldpassword.val()},
dataType:"json",
success:function(data){
if(data.result == "true"){//旧密码正确
validateTip(oldpassword.next(),{"color":"green"},imgYes,true);
}else if(data.result == "false"){//旧密码输入不正确
validateTip(oldpassword.next(),{"color":"red"},imgNo + " 原密码输入不正确",false);
}else if(data.result == "sessionerror"){//当前用户session过期,请重新登录
validateTip(oldpassword.next(),{"color":"red"},imgNo + " 当前用户session过期,请重新登录",false);
}else if(data.result == "error"){//旧密码输入为空
validateTip(oldpassword.next(),{"color":"red"},imgNo + " 请输入旧密码",false);
}
},
error:function(data){
//请求出错
validateTip(oldpassword.next(),{"color":"red"},imgNo + " 请求错误",false);
}
});
}).on("focus",function(){
validateTip(oldpassword.next(),{"color":"#666666"},"* 请输入原密码",false);
});
newpassword.on("focus",function(){
validateTip(newpassword.next(),{"color":"#666666"},"* 密码长度必须是大于6小于20",false);
}).on("blur",function(){
if(newpassword.val() != null && newpassword.val().length > 6
&& newpassword.val().length < 20 ){
validateTip(newpassword.next(),{"color":"green"},imgYes,true);
}else{
validateTip(newpassword.next(),{"color":"red"},imgNo + " 密码输入不符合规范,请重新输入",false);
}
});
rnewpassword.on("focus",function(){
validateTip(rnewpassword.next(),{"color":"#666666"},"* 请输入与上面一致的密码",false);
}).on("blur",function(){
if(rnewpassword.val() != null && rnewpassword.val().length > 6
&& rnewpassword.val().length < 20 && newpassword.val() == rnewpassword.val()){
validateTip(rnewpassword.next(),{"color":"green"},imgYes,true);
}else{
validateTip(rnewpassword.next(),{"color":"red"},imgNo + " 两次密码输入不一致,请重新输入",false);
}
});
saveBtn.on("click",function(){
oldpassword.blur();
newpassword.blur();
rnewpassword.blur();
//oldpassword.attr("validateStatus") == "true" &&
if(newpassword.attr("validateStatus") == "true"
&& rnewpassword.attr("validateStatus") == "true"){
if(confirm("确定要修改密码?")){
$("#userForm").submit();
}
}
});
});
UserDao
//修改当前用户密码
public int updatePwd(Connection connection,int id,String password) throws Exception;
UserDaoImpl
//修改当前用户密码
public int updatePwd(Connection connection, int id, String password) throws Exception {
PreparedStatement pstm=null;
int flag=0;
if(connection!=null) {
String sql = "update smbms_user set userPassword= ? where id = ?";
Object params[] = {password, id};
flag=BaseDao.execute(connection, pstm, sql, params);
BaseDao.closeResource(null, pstm, null);
}
return flag;
}
UserService
//根据用户ID修改密码判断是否成功
public boolean updatePwd(int id,String pwd);
UserServiceImpl
public boolean updatePwd(int id, String pwd) {
Connection connection=null;
boolean flag=false;
//修改密码
try {
connection = BaseDao.getConnection();
if(userDao.updatePwd(connection,id,pwd)>0){
flag=true;
}
} catch (Exception throwables) {
throwables.printStackTrace();
} finally {
BaseDao.closeResource(connection,null,null);
}
return flag;
}
UserServlet
package com.node.servlet.user;
import com.mysql.cj.util.StringUtils;
import com.node.pojo.User;
import com.node.service.User.UserService;
import com.node.service.User.UserServiceImpl;
import com.node.util.Constants;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
//实现Servlet服用
public class UserServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//去pwdmodify.jsp里面取隐藏字段,实现复用(重复使用)
String method = req.getParameter("method");
if (method.equals("savepwd") && method != null) {
this.updatePwd(req, resp);
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
public void updatePwd(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//从Session里面拿ID
User user = (User) req.getSession().getAttribute(Constants.USER_SESSION);
System.out.println(user.getUserPassword());
//去前端拿新密码
String newPassword = req.getParameter("newPassword");
//判断ID不为空切新密码不为0
System.out.println(newPassword);
boolean flag=false;
//StringUtils.isNullOrEmpty(newPassword):用工具包判断newPassword是否为空。
//且返回值为false 所以需要添加 !
if(user!=null){
UserService userService = new UserServiceImpl();
flag = userService.updatePwd(user.getId(), newPassword);
if(flag){
req.setAttribute("message","修改密码成功,请退出使用新密码");
req.getSession().removeAttribute(Constants.USER_SESSION);
}else {
req.setAttribute("message","密码修改失败");
}
}else {
req.setAttribute("message","新密码有问题");
}
req.getRequestDispatcher("pwdmodify.jsp").forward(req,resp);
}
}
4.2修改旧密码使用Ajax
json:实现前后端的交互
4.3导入阿里巴巴的fastjson
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.73</version>
</dependency>
4.4代码实现
else if(method.equals("pwdmodify")&&method!=null){
this.pwdModify(req,resp);
}
public void pwdModify(HttpServletRequest req, HttpServletResponse resp){
//从Session里面拿ID
User user = (User) req.getSession().getAttribute(Constants.USER_SESSION);
System.out.println(user.getUserPassword());
//去前端输入旧密码
String oldPassword = req.getParameter("oldPassword");
//万能的Map:结果集,可以传递任何参数
Map<String,String> resultMap=new HashMap<String, String>();
if(user==null){ //Session失效了
resultMap.put("result","sessionerror");
}else if(StringUtils.isNullOrEmpty(oldPassword)){//密码为空
resultMap.put("result","error");
}else {
String userPassword=user.getUserPassword(); //取出Session中用户的密码
if(oldPassword.equals(userPassword)){ //判断是否和输入密码一样
resultMap.put("result","true");
}else {
resultMap.put("result","false");
}
}
try {
resp.setContentType("application/json");
PrintWriter writer = resp.getWriter();
//JSONArray 阿里巴巴的JSON工具类,转换格式
/*
resultMap=["result","sessionerror","result","error"]
Json格式={key:value}
*/
writer.write(JSONArray.toJSONString(resultMap));
writer.flush();
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
5.用户管理实现
5.1导入分页的工具类PageSupport.java
package cn.smbms.tools;
public class PageSupport {
//当前页码-来自于用户输入
private int currentPageNo = 1;
//总数量(表)
private int totalCount = 0;
//页面容量
private int pageSize = 0;
//总页数-totalCount/pageSize(+1)
private int totalPageCount = 1;
public int getCurrentPageNo() {
return currentPageNo;
}
public void setCurrentPageNo(int currentPageNo) {
if(currentPageNo > 0){
this.currentPageNo = currentPageNo;
}
}
public int getTotalCount() {
return totalCount;
}
public void setTotalCount(int totalCount) {
if(totalCount > 0){
this.totalCount = totalCount;
//设置总页数
this.setTotalPageCountByRs();
}
}
public int getPageSize() {
return pageSize;
}
public void setPageSize(int pageSize) {
if(pageSize > 0){
this.pageSize = pageSize;
}
}
public int getTotalPageCount() {
return totalPageCount;
}
public void setTotalPageCount(int totalPageCount) {
this.totalPageCount = totalPageCount;
}
public void setTotalPageCountByRs(){
if(this.totalCount % this.pageSize == 0){
this.totalPageCount = this.totalCount / this.pageSize;
}else if(this.totalCount % this.pageSize > 0){
this.totalPageCount = this.totalCount / this.pageSize + 1;
}else{
this.totalPageCount = 0;
}
}
}
5.2导入用户列表页userlist.jsp和转表页rollpage.jsp
5.3获取用户数量
5.3.1UserDao
//查询用户总数(用名字+角色)
public int getUserCount(Connection connection,String username,int userRole) throws Exception;
5.3.2UserDaoImpl
public int getUserCount(Connection connection, String username, int userRole) throws Exception {
PreparedStatement pstm=null;
ResultSet rs=null;
int count=0;
if(connection!=null){
StringBuffer sql=new StringBuffer();
//连表查询总人数
sql.append("select count(1) as count from smbms_user u,smbms_role r where u.userRole =r.id");
ArrayList<Object> list=new ArrayList<Object>();//传递参数,index:1
//String工具包:判断是username否为空
if(!StringUtils.isNullOrEmpty(username)){
//select count(1) as count from smbms_user u,smbms_role r where u.userRole =r.id and u.userName like="%李%"
//and 前记得打空格
sql.append(" and u.userName like=?");
list.add("%"+username+"%");
}
if(userRole>0){
sql.append(" and u.userRole=?");
list.add(userRole);
}
//把list转换为数组
Object[] params = list.toArray();
//输出最后完整的SQL语句
System.out.println("UserDaoImpl->getUserCount:"+sql.toString());
rs = BaseDao.execute(connection, pstm, rs, sql.toString(), params);
if(rs.next()){
count=rs.getInt("count"); //从结果集(sql语句里面的count)中获取最终的数量
}
BaseDao.closeResource(null,pstm,rs);
}
return count;
}
5.3.3UserService
//查询记录数
public int getUserCount(String username,int userRole);
5.3.4UserServiceImpl
//获取用户数量
public int getUserCount(String username, int userRole) {
Connection connection=null;
int count=0;
try {
connection=BaseDao.getConnection();
count=userDao.getUserCount(connection,username,userRole);
} catch (Exception e) {
e.printStackTrace();
}finally {
BaseDao.closeResource(connection,null,null);
}
return count;
}
5.4获取用户列表
5.4.1UserDao
//获取用户列表,currentPageNo:当前页 pageSize:页面总数
public List<User> getUserList(Connection connection, String userName, int userRole, int currentPageNo, int pageSize)throws Exception;
5.4.2UserDaoImpl
public List<User> getUserList(Connection connection, String userName, int userRole, int currentPageNo, int pageSize)
throws Exception {
PreparedStatement pstm = null;
ResultSet rs = null;
List<User> userList = new ArrayList<User>();
if(connection != null){
StringBuffer sql = new StringBuffer();
sql.append("select u.*,r.roleName as userRoleName from smbms_user u,smbms_role r where u.userRole = r.id");
List<Object> list = new ArrayList<Object>();
if(!StringUtils.isNullOrEmpty(userName)){
sql.append(" and u.userName like ?");
list.add("%"+userName+"%");
}
if(userRole > 0){
sql.append(" and u.userRole = ?");
list.add(userRole);
}
/*
* 在数据库中,分页使用 limi:startIndex,pageSize
* 当前页 ;(当前页-1)*页面大小
* 0,5 1 0 01234
* 5,5 2 5 26789
* 10,5 3 10
*/
sql.append(" order by creationDate DESC limit ?,?");
currentPageNo = (currentPageNo-1)*pageSize;
list.add(currentPageNo);
list.add(pageSize);
Object[] params = list.toArray();
System.out.println("sql ----> " + sql.toString());
rs = BaseDao.execute(connection, pstm, rs, sql.toString(), params);
while(rs.next()){
User _user = new User();
_user.setId(rs.getInt("id"));
_user.setUserCode(rs.getString("userCode"));
_user.setUserName(rs.getString("userName"));
_user.setGender(rs.getInt("gender"));
_user.setBirthday(rs.getDate("birthday"));
_user.setPhone(rs.getString("phone"));
_user.setUserRole(rs.getInt("userRole"));
_user.setUserRoleName(rs.getString("userRoleName"));
userList.add(_user);
}
BaseDao.closeResource(null, pstm, rs);
}
return userList;
}
5.4.3UserService
//根据条件查询用户列表
public List<User> getUserList(String queryUserName, int queryUserRole, int currentPageNo, int pageSize);
5.4.4UserServiceImpl
//获取用户列表
public List<User> getUserList(String queryUserName, int queryUserRole, int currentPageNo, int pageSize) {
// TODO Auto-generated method stub
Connection connection = null;
List<User> userList = null;
System.out.println("queryUserName ---- > " + queryUserName);
System.out.println("queryUserRole ---- > " + queryUserRole);
System.out.println("currentPageNo ---- > " + currentPageNo);
System.out.println("pageSize ---- > " + pageSize);
try {
connection = BaseDao.getConnection();
userList = userDao.getUserList(connection, queryUserName,queryUserRole,currentPageNo,pageSize);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
BaseDao.closeResource(connection, null, null);
}
return userList;
}
5.5获取角色列表
创建对应的包
5.5.1RoleDao
package com.node.dao.role;
import com.node.pojo.Role;
import java.sql.Connection;
import java.util.List;
public interface RoleDao {
//获取角色列表
public List<Role> getRoleList(Connection connection)throws Exception;
}
5.5.2RoleDaoImpl
package com.node.dao.role;
import com.node.dao.BaseDao;
import com.node.pojo.Role;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
public class RoleDaoImpl implements RoleDao{
//获取角色列表
public List<Role> getRoleList(Connection connection) throws Exception {
PreparedStatement pstm=null;
ResultSet rs=null;
ArrayList<Role> roleList=new ArrayList<Role>();
if(connection!=null){
String sql="select * from smbms_role";
Object[] params={};
rs = BaseDao.execute(connection, pstm, rs, sql, params);
while (rs.next()){
Role role = new Role();
role.setId(rs.getInt("id"));
role.setRoleCode(rs.getString("roleName"));
role.setRoleCode(rs.getString("roleName"));
roleList.add(role);
}
BaseDao.closeResource(null,pstm,rs);
}
return roleList;
}
}
5.5.3RoleService
package com.node.service.role;
import com.node.pojo.Role;
import java.util.List;
public interface RoleService {
public List<Role> getRoleList();
}
5.5.4RoleServiceImpl
package com.node.service.role;
import com.node.dao.BaseDao;
import com.node.dao.role.RoleDao;
import com.node.dao.role.RoleDaoImpl;
import com.node.pojo.Role;
import java.sql.Connection;
import java.util.List;
public class RoleServiceImpl implements RoleService{
private RoleDao roleDao;
public RoleServiceImpl(){
roleDao = new RoleDaoImpl();
}
public List<Role> getRoleList() {
Connection connection = null;
List<Role> roleList = null;
try {
connection = BaseDao.getConnection();
roleList = roleDao.getRoleList(connection);
} catch (Exception e) {
e.printStackTrace();
}finally{
BaseDao.closeResource(connection, null, null);
}
return roleList;
}
}
5.6编写Servlet
else if(method.equals("query")&&method!=null);{
this.query(req,resp);
}
//获取用户列表,角色列表,分页列表,对应userlist.jsp、rollpage.jsp里的数据
public void query(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//查询用户列表
String queryUserName = req.getParameter("queryname"); //对应jsp用户名称
String temp = req.getParameter("queryUserRole"); //对应jsp用户角色
String pageIndex = req.getParameter("pageIndex"); //对应jsp不同页面分页
int queryUserRole = 0; //当前页
UserService userService = new UserServiceImpl();
List<User> userList = null;
//设置页面容量
int pageSize = Constants.pageSize;
//当前页码
int currentPageNo = 1;
if(queryUserName == null){
queryUserName = "";
}
if(temp != null && !temp.equals("")){
queryUserRole = Integer.parseInt(temp);
}
if(pageIndex != null){
try{
currentPageNo = Integer.valueOf(pageIndex);
}catch(NumberFormatException e){
resp.sendRedirect("error.jsp");
}
}
//总数量(表)
int totalCount = userService.getUserCount(queryUserName,queryUserRole);
//总页数
PageSupport pages=new PageSupport();
pages.setCurrentPageNo(currentPageNo); // 当前页
pages.setPageSize(pageSize); //页面大小,一页有几条数据
pages.setTotalCount(totalCount); //页面总数,一共有几页
int totalPageCount = pages.getTotalPageCount();
//控制首页和尾页
if(currentPageNo < 1){
currentPageNo = 1;
}else if(currentPageNo > totalPageCount){
currentPageNo = totalPageCount;
}
/*
* 根据前端需要显示的数据然后传入
*/
userList = userService.getUserList(queryUserName,queryUserRole,currentPageNo, pageSize);
req.setAttribute("userList", userList); //传入到前端用户列表
List<Role> roleList = null;
RoleService roleService = new RoleServiceImpl();
roleList = roleService.getRoleList();
req.setAttribute("roleList", roleList); //传入到前端角色列表
req.setAttribute("queryUserName", queryUserName);//传入到前端用户名称
req.setAttribute("queryUserRole", queryUserRole);//传入到前端用户角色
req.setAttribute("totalPageCount", totalPageCount);//传入到前端总页数
req.setAttribute("totalCount", totalCount);//传入到前端数据总数(共12条记录=共12个用户数据)
req.setAttribute("currentPageNo", currentPageNo);//传入到前端页面总数
req.getRequestDispatcher("userlist.jsp").forward(req, resp);
}
5.7 增删改操作
5.7.1导入useradd.jsp、usermodify.jsp、userlist.jsp(已导入,里面有删除操作)
5.7.2UserDao
// 增加用户信息
public int add(Connection connection, User user)throws Exception;
//删除用户信息
public int deleteUserById(Connection connection, Integer delId)throws Exception;
//添加用户信息
public User getUserById(Connection connection, String id)throws Exception;
//修改用户信息
public int modify(Connection connection, User user)throws Exception;
5.7.3UserDaoImpl
public int add(Connection connection, User user) throws Exception {
PreparedStatement pstm = null;
int updateRows = 0;
if(null != connection){
String sql = "insert into smbms_user (userCode,userName,userPassword," +
"userRole,gender,birthday,phone,address,creationDate,createdBy) " +
"values(?,?,?,?,?,?,?,?,?,?)";
Object[] params = {user.getUserCode(),user.getUserName(),user.getUserPassword(),
user.getUserRole(),user.getGender(),user.getBirthday(),
user.getPhone(),user.getAddress(),user.getCreationDate(),user.getCreatedBy()};
updateRows = BaseDao.execute(connection, pstm, sql, params);
BaseDao.closeResource(null, pstm, null);
}
return updateRows;
}
public int deleteUserById(Connection connection,Integer delId) throws Exception {
PreparedStatement pstm = null;
int flag = 0;
if(null != connection){
String sql = "delete from smbms_user where id=?";
Object[] params = {delId};
flag = BaseDao.execute(connection, pstm, sql, params);
BaseDao.closeResource(null, pstm, null);
}
return flag;
}
public User getUserById(Connection connection, String id) throws Exception {
User user = null;
PreparedStatement pstm = null;
ResultSet rs = null;
if(null != connection){
String sql = "select u.*,r.roleName as userRoleName from smbms_user u,smbms_role r where u.id=? and u.userRole = r.id";
Object[] params = {id};
rs = BaseDao.execute(connection, pstm, rs, sql, params);
if(rs.next()){
user = new User();
user.setId(rs.getInt("id"));
user.setUserCode(rs.getString("userCode"));
user.setUserName(rs.getString("userName"));
user.setUserPassword(rs.getString("userPassword"));
user.setGender(rs.getInt("gender"));
user.setBirthday(rs.getDate("birthday"));
user.setPhone(rs.getString("phone"));
user.setAddress(rs.getString("address"));
user.setUserRole(rs.getInt("userRole"));
user.setCreatedBy(rs.getInt("createdBy"));
user.setCreationDate(rs.getTimestamp("creationDate"));
user.setModifyBy(rs.getInt("modifyBy"));
user.setModifyDate(rs.getTimestamp("modifyDate"));
user.setUserRoleName(rs.getString("userRoleName"));
}
BaseDao.closeResource(null, pstm, rs);
}
return user;
}
public int modify(Connection connection, User user) throws Exception {
int flag = 0;
PreparedStatement pstm = null;
if(null != connection){
String sql = "update smbms_user set userName=?,"+
"gender=?,birthday=?,phone=?,address=?,userRole=?,modifyBy=?,modifyDate=? where id = ? ";
Object[] params = {user.getUserName(),user.getGender(),user.getBirthday(),
user.getPhone(),user.getAddress(),user.getUserRole(),user.getModifyBy(),
user.getModifyDate(),user.getId()};
flag = BaseDao.execute(connection, pstm, sql, params);
BaseDao.closeResource(null, pstm, null);
}
return flag;
}
5.7.4UserService
//添加用户信息
public boolean add(User user);
//根据userCode查询用户信息
public User selectUserCodeExist(String userCode);
//删除用户信息
public boolean deleteUserById(Integer delId);
//根据ID查询用户信息
public User getUserById(String id);
//修改用户信息
public boolean modify(User user);
5.7.5UserServiceImop
public boolean add(User user) {
// TODO Auto-generated method stub
boolean flag = false;
Connection connection = null;
try {
connection = BaseDao.getConnection();
connection.setAutoCommit(false);//开启JDBC事务管理
int updateRows = userDao.add(connection,user);
connection.commit();
if(updateRows > 0){
flag = true;
System.out.println("add success!");
}else{
System.out.println("add failed!");
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
try {
System.out.println("rollback==================");
connection.rollback();
} catch (SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}finally{
//在service层进行connection连接的关闭
BaseDao.closeResource(connection, null, null);
}
return flag;
}
public User selectUserCodeExist(String userCode) {
// TODO Auto-generated method stub
Connection connection = null;
User user = null;
try {
connection = BaseDao.getConnection();
user = userDao.getLoginUser(connection, userCode);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
BaseDao.closeResource(connection, null, null);
}
return user;
}
public boolean deleteUserById(Integer delId) {
// TODO Auto-generated method stub
Connection connection = null;
boolean flag = false;
try {
connection = BaseDao.getConnection();
if(userDao.deleteUserById(connection,delId) > 0)
flag = true;
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
BaseDao.closeResource(connection, null, null);
}
return flag;
}
public User getUserById(String id) {
// TODO Auto-generated method stub
User user = null;
Connection connection = null;
try{
connection = BaseDao.getConnection();
user = userDao.getUserById(connection,id);
}catch (Exception e) {
// TODO: handle exception
e.printStackTrace();
user = null;
}finally{
BaseDao.closeResource(connection, null, null);
}
return user;
}
public boolean modify(User user) {
// TODO Auto-generated method stub
Connection connection = null;
boolean flag = false;
try {
connection = BaseDao.getConnection();
if(userDao.modify(connection,user) > 0)
flag = true;
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
BaseDao.closeResource(connection, null, null);
}
return flag;
}
5.7.6UserServlet
else if(method != null && method.equals("add")){
this.add(req, resp);//增加用户信息
}else if(method != null && method.equals("ucexist")){
this.userCodeExist(req, resp); //根据userCode查询用户信息
}else if(method != null && method.equals("deluser")){
this.delUser(req, resp);//删除用户信息
}else if(method != null && method.equals("view")){
this.getUserById(req, resp,"userview.jsp");//根据id查询用户信息
}else if(method != null && method.equals("modify")){
this.getUserById(req, resp,"usermodify.jsp");//根据id查询用户信息
}else if(method != null && method.equals("modifyexe")){
this.modify(req, resp);//修改用户信息
}else if(method != null && method.equals("savepwd")){
this.updatePwd(req, resp);//删除用户信息
}else if(method != null && method.equals("getrolelist")) {
this.getRoleList(req, resp);//把roleList转换成json对象输出
}
private void add(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
System.out.println("add()================");
String userCode = request.getParameter("userCode");
String userName = request.getParameter("userName");
String userPassword = request.getParameter("userPassword");
String gender = request.getParameter("gender");
String birthday = request.getParameter("birthday");
String phone = request.getParameter("phone");
String address = request.getParameter("address");
String userRole = request.getParameter("userRole");
User user = new User();
user.setUserCode(userCode);
user.setUserName(userName);
user.setUserPassword(userPassword);
user.setAddress(address);
try {
user.setBirthday(new SimpleDateFormat("yyyy-MM-dd").parse(birthday));
} catch (ParseException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
user.setGender(Integer.valueOf(gender));
user.setPhone(phone);
user.setUserRole(Integer.valueOf(userRole));
user.setCreationDate(new Date());
user.setCreatedBy(((User)request.getSession().getAttribute(Constants.USER_SESSION)).getId());
UserService userService = new UserServiceImpl();
if(userService.add(user)){
response.sendRedirect(request.getContextPath()+"/jsp/user.do?method=query");
}else{
request.getRequestDispatcher("useradd.jsp").forward(request, response);
}
}
private void userCodeExist(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
//判断用户账号是否可用
String userCode = request.getParameter("userCode");
HashMap<String, String> resultMap = new HashMap<String, String>();
if(StringUtils.isNullOrEmpty(userCode)){
//userCode == null || userCode.equals("")
resultMap.put("userCode", "exist");
}else{
UserService userService = new UserServiceImpl();
User user = userService.selectUserCodeExist(userCode);
if(null != user){
resultMap.put("userCode","exist");
}else{
resultMap.put("userCode", "notexist");
}
}
//把resultMap转为json字符串以json的形式输出
//配置上下文的输出类型
response.setContentType("application/json");
//从response对象中获取往外输出的writer对象
PrintWriter outPrintWriter = response.getWriter();
//把resultMap转为json字符串 输出
outPrintWriter.write(JSONArray.toJSONString(resultMap));
outPrintWriter.flush();//刷新
outPrintWriter.close();//关闭流
}
private void delUser(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String id = request.getParameter("uid");
Integer delId = 0;
try{
delId = Integer.parseInt(id);
}catch (Exception e) {
// TODO: handle exception
delId = 0;
}
HashMap<String, String> resultMap = new HashMap<String, String>();
if(delId <= 0){
resultMap.put("delResult", "notexist");
}else{
UserService userService = new UserServiceImpl();
if(userService.deleteUserById(delId)){
resultMap.put("delResult", "true");
}else{
resultMap.put("delResult", "false");
}
}
//把resultMap转换成json对象输出
response.setContentType("application/json");
PrintWriter outPrintWriter = response.getWriter();
outPrintWriter.write(JSONArray.toJSONString(resultMap));
outPrintWriter.flush();
outPrintWriter.close();
}
private void getUserById(HttpServletRequest request, HttpServletResponse response,String url)
throws ServletException, IOException {
String id = request.getParameter("uid");
if(!StringUtils.isNullOrEmpty(id)){
//调用后台方法得到user对象
UserService userService = new UserServiceImpl();
User user = userService.getUserById(id);
request.setAttribute("user", user);
request.getRequestDispatcher(url).forward(request, response);
}
}
private void modify(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String id = request.getParameter("uid");
String userName = request.getParameter("userName");
String gender = request.getParameter("gender");
String birthday = request.getParameter("birthday");
String phone = request.getParameter("phone");
String address = request.getParameter("address");
String userRole = request.getParameter("userRole");
User user = new User();
user.setId(Integer.valueOf(id));
user.setUserName(userName);
user.setGender(Integer.valueOf(gender));
try {
user.setBirthday(new SimpleDateFormat("yyyy-MM-dd").parse(birthday));
} catch (ParseException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
user.setPhone(phone);
user.setAddress(address);
user.setUserRole(Integer.valueOf(userRole));
user.setModifyBy(((User)request.getSession().getAttribute(Constants.USER_SESSION)).getId());
user.setModifyDate(new Date());
UserService userService = new UserServiceImpl();
if(userService.modify(user)){
response.sendRedirect(request.getContextPath()+"/jsp/user.do?method=query");
}else{
request.getRequestDispatcher("usermodify.jsp").forward(request, response);
}
}
private void getRoleList(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
List<Role> roleList = null;
RoleService roleService = new RoleServiceImpl();
roleList = roleService.getRoleList();
//把roleList转换成json对象输出
response.setContentType("application/json");
PrintWriter outPrintWriter = response.getWriter();
outPrintWriter.write(JSONArray.toJSONString(roleList));
outPrintWriter.flush();
outPrintWriter.close();
}
6.供应商管理实现
6.1增删改查
6.1.1导入provideradd.jsp、providerlist.jsp、providermodify.jsp、providerview.jsp
6.1.2providerDao
package com.node.dao.provider;
import com.node.pojo.Provider;
import java.sql.Connection;
import java.util.List;
public interface ProviderDao {
/**
* 增加供应商
* @param connection
* @param provider
* @return
* @throws Exception
*/
public int add(Connection connection, Provider provider)throws Exception;
/**
* 通过供应商名称、编码获取供应商列表-模糊查询-providerList
* @param connection
* @param proName
* @return
* @throws Exception
*/
public List<Provider> getProviderList(Connection connection, String proName, String proCode)throws Exception;
/**
* 通过proId删除Provider
* @param delId
* @return
* @throws Exception
*/
public int deleteProviderById(Connection connection, String delId)throws Exception;
/**
* 通过proId获取Provider
* @param connection
* @param id
* @return
* @throws Exception
*/
public Provider getProviderById(Connection connection, String id)throws Exception;
/**
* 修改用户信息
* @param connection
* @param user
* @return
* @throws Exception
*/
public int modify(Connection connection, Provider provider)throws Exception;
}
6.1.3providerDaoImpl
package com.node.dao.provider;
import com.mysql.cj.util.StringUtils;
import com.node.dao.BaseDao;
import com.node.pojo.Provider;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
public class ProviderDaoImpl implements ProviderDao{
public int add(Connection connection, Provider provider)
throws Exception {
// TODO Auto-generated method stub
PreparedStatement pstm = null;
int flag = 0;
if(null != connection){
String sql = "insert into smbms_provider (proCode,proName,proDesc," +
"proContact,proPhone,proAddress,proFax,createdBy,creationDate) " +
"values(?,?,?,?,?,?,?,?,?)";
Object[] params = {provider.getProCode(),provider.getProName(),provider.getProDesc(),
provider.getProContact(),provider.getProPhone(),provider.getProAddress(),
provider.getProFax(),provider.getCreatedBy(),provider.getCreationDate()};
flag = BaseDao.execute(connection, pstm, sql, params);
BaseDao.closeResource(null, pstm, null);
}
return flag;
}
public List<Provider> getProviderList(Connection connection, String proName, String proCode)
throws Exception {
// TODO Auto-generated method stub
PreparedStatement pstm = null;
ResultSet rs = null;
List<Provider> providerList = new ArrayList<Provider>();
if(connection != null){
StringBuffer sql = new StringBuffer();
sql.append("select * from smbms_provider where 1=1 ");
List<Object> list = new ArrayList<Object>();
if(!StringUtils.isNullOrEmpty(proName)){
sql.append(" and proName like ?");
list.add("%"+proName+"%");
}
if(!StringUtils.isNullOrEmpty(proCode)){
sql.append(" and proCode like ?");
list.add("%"+proCode+"%");
}
Object[] params = list.toArray();
System.out.println("sql ----> " + sql.toString());
rs = BaseDao.execute(connection, pstm, rs, sql.toString(), params);
while(rs.next()){
Provider _provider = new Provider();
_provider.setId(rs.getInt("id"));
_provider.setProCode(rs.getString("proCode"));
_provider.setProName(rs.getString("proName"));
_provider.setProDesc(rs.getString("proDesc"));
_provider.setProContact(rs.getString("proContact"));
_provider.setProPhone(rs.getString("proPhone"));
_provider.setProAddress(rs.getString("proAddress"));
_provider.setProFax(rs.getString("proFax"));
_provider.setCreationDate(rs.getTimestamp("creationDate"));
providerList.add(_provider);
}
BaseDao.closeResource(null, pstm, rs);
}
return providerList;
}
public int deleteProviderById(Connection connection, String delId)
throws Exception {
// TODO Auto-generated method stub
PreparedStatement pstm = null;
int flag = 0;
if(null != connection){
String sql = "delete from smbms_provider where id=?";
Object[] params = {delId};
flag = BaseDao.execute(connection, pstm, sql, params);
BaseDao.closeResource(null, pstm, null);
}
return flag;
}
public Provider getProviderById(Connection connection, String id)
throws Exception {
// TODO Auto-generated method stub
Provider provider = null;
PreparedStatement pstm = null;
ResultSet rs = null;
if(null != connection){
String sql = "select * from smbms_provider where id=?";
Object[] params = {id};
rs = BaseDao.execute(connection, pstm, rs, sql, params);
if(rs.next()){
provider = new Provider();
provider.setId(rs.getInt("id"));
provider.setProCode(rs.getString("proCode"));
provider.setProName(rs.getString("proName"));
provider.setProDesc(rs.getString("proDesc"));
provider.setProContact(rs.getString("proContact"));
provider.setProPhone(rs.getString("proPhone"));
provider.setProAddress(rs.getString("proAddress"));
provider.setProFax(rs.getString("proFax"));
provider.setCreatedBy(rs.getInt("createdBy"));
provider.setCreationDate(rs.getTimestamp("creationDate"));
provider.setModifyBy(rs.getInt("modifyBy"));
provider.setModifyDate(rs.getTimestamp("modifyDate"));
}
BaseDao.closeResource(null, pstm, rs);
}
return provider;
}
public int modify(Connection connection, Provider provider)
throws Exception {
// TODO Auto-generated method stub
int flag = 0;
PreparedStatement pstm = null;
if(null != connection){
String sql = "update smbms_provider set proName=?,proDesc=?,proContact=?," +
"proPhone=?,proAddress=?,proFax=?,modifyBy=?,modifyDate=? where id = ? ";
Object[] params = {provider.getProName(),provider.getProDesc(),provider.getProContact(),provider.getProPhone(),provider.getProAddress(),
provider.getProFax(),provider.getModifyBy(),provider.getModifyDate(),provider.getId()};
flag = BaseDao.execute(connection, pstm, sql, params);
BaseDao.closeResource(null, pstm, null);
}
return flag;
}
}
6.1.4ProviderService
package com.node.service.provide;
import com.node.pojo.Provider;
import java.util.List;
public interface ProviderService {
/**
* 增加供应商
* @param provider
* @return
*/
public boolean add(Provider provider);
/**
* 通过供应商名称、编码获取供应商列表-模糊查询-providerList
* @param proName
* @return
*/
public List<Provider> getProviderList(String proName, String proCode);
/**
* 通过proId删除Provider
* @param delId
* @return
*/
public int deleteProviderById(String delId);
/**
* 通过proId获取Provider
* @param id
* @return
*/
public Provider getProviderById(String id);
/**
* 修改用户信息
*/
public boolean modify(Provider provider);
}
6.1.5ProviderServiceImpl
package com.node.service.provide;
import com.node.dao.BaseDao;
import com.node.dao.bill.BillDao;
import com.node.dao.bill.BillDaoImpl;
import com.node.dao.provider.ProviderDao;
import com.node.dao.provider.ProviderDaoImpl;
import com.node.pojo.Provider;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.List;
public class ProviderServiceImpl implements ProviderService {
private ProviderDao providerDao;
private BillDao billDao;
public ProviderServiceImpl(){
providerDao = new ProviderDaoImpl();
billDao = new BillDaoImpl();
}
public boolean add(Provider provider) {
// TODO Auto-generated method stub
boolean flag = false;
Connection connection = null;
try {
connection = BaseDao.getConnection();
connection.setAutoCommit(false);//开启JDBC事务管理
if(providerDao.add(connection,provider) > 0)
flag = true;
connection.commit();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
try {
System.out.println("rollback==================");
connection.rollback();
} catch (SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}finally{
//在service层进行connection连接的关闭
BaseDao.closeResource(connection, null, null);
}
return flag;
}
public List<Provider> getProviderList(String proName, String proCode) {
// TODO Auto-generated method stub
Connection connection = null;
List<Provider> providerList = null;
System.out.println("query proName ---- > " + proName);
System.out.println("query proCode ---- > " + proCode);
try {
connection = BaseDao.getConnection();
providerList = providerDao.getProviderList(connection, proName,proCode);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
BaseDao.closeResource(connection, null, null);
}
return providerList;
}
/**
* 业务:根据ID删除供应商表的数据之前,需要先去订单表里进行查询操作
* 若订单表中无该供应商的订单数据,则可以删除
* 若有该供应商的订单数据,则不可以删除
* 返回值billCount
* 1> billCount == 0 删除---1 成功 (0) 2 不成功 (-1)
* 2> billCount > 0 不能删除 查询成功(0)查询不成功(-1)
*
* ---判断
* 如果billCount = -1 失败
* 若billCount >= 0 成功
*/
public int deleteProviderById(String delId) {
// TODO Auto-generated method stub
Connection connection = null;
int billCount = -1;
try {
connection = BaseDao.getConnection();
connection.setAutoCommit(false);
billCount = billDao.getBillCountByProviderId(connection,delId);
if(billCount == 0){
providerDao.deleteProviderById(connection, delId);
}
connection.commit();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
billCount = -1;
try {
connection.rollback();
} catch (SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}finally{
BaseDao.closeResource(connection, null, null);
}
return billCount;
}
public Provider getProviderById(String id) {
// TODO Auto-generated method stub
Provider provider = null;
Connection connection = null;
try{
connection = BaseDao.getConnection();
provider = providerDao.getProviderById(connection, id);
}catch (Exception e) {
// TODO: handle exception
e.printStackTrace();
provider = null;
}finally{
BaseDao.closeResource(connection, null, null);
}
return provider;
}
public boolean modify(Provider provider) {
// TODO Auto-generated method stub
Connection connection = null;
boolean flag = false;
try {
connection = BaseDao.getConnection();
if(providerDao.modify(connection,provider) > 0)
flag = true;
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
BaseDao.closeResource(connection, null, null);
}
return flag;
}
}
6.1.6ProviderServlet
package com.node.servlet.provide;
import com.alibaba.fastjson.JSONArray;
import com.mysql.cj.util.StringUtils;
import com.node.pojo.Provider;
import com.node.pojo.User;
import com.node.service.provide.ProviderService;
import com.node.service.provide.ProviderServiceImpl;
import com.node.util.Constants;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
public class ProvideServlet extends HttpServlet {
/**
* The doGet method of the servlet. <br>
*
* This method is called when a form has its tag value method equals to get.
*
* @param request the request send by the client to the server
* @param response the response send by the server to the client
* @throws ServletException if an error occurred
* @throws IOException if an error occurred
*/
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
/**
* The doPost method of the servlet. <br>
*
* This method is called when a form has its tag value method equals to post.
*
* @param request the request send by the client to the server
* @param response the response send by the server to the client
* @throws ServletException if an error occurred
* @throws IOException if an error occurred
*/
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String method = request.getParameter("method");
if(method != null && method.equals("query")){
this.query(request,response);
}else if(method != null && method.equals("add")){
this.add(request,response);
}else if(method != null && method.equals("view")){
this.getProviderById(request,response,"providerview.jsp");
}else if(method != null && method.equals("modify")){
this.getProviderById(request,response,"providermodify.jsp");
}else if(method != null && method.equals("modifysave")){
this.modify(request,response);
}else if(method != null && method.equals("delprovider")){
this.delProvider(request,response);
}
}
private void delProvider(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String id = request.getParameter("proid");
HashMap<String, String> resultMap = new HashMap<String, String>();
if(!StringUtils.isNullOrEmpty(id)){
ProviderService providerService = new ProviderServiceImpl();
int flag = providerService.deleteProviderById(id);
if(flag == 0){//删除成功
resultMap.put("delResult", "true");
}else if(flag == -1){//删除失败
resultMap.put("delResult", "false");
}else if(flag > 0){//该供应商下有订单,不能删除,返回订单数
resultMap.put("delResult", String.valueOf(flag));
}
}else{
resultMap.put("delResult", "notexit");
}
//把resultMap转换成json对象输出
response.setContentType("application/json");
PrintWriter outPrintWriter = response.getWriter();
outPrintWriter.write(JSONArray.toJSONString(resultMap));
outPrintWriter.flush();
outPrintWriter.close();
}
private void modify(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String proContact = request.getParameter("proContact");
String proPhone = request.getParameter("proPhone");
String proAddress = request.getParameter("proAddress");
String proFax = request.getParameter("proFax");
String proDesc = request.getParameter("proDesc");
String id = request.getParameter("id");
Provider provider = new Provider();
provider.setId(Integer.valueOf(id));
provider.setProContact(proContact);
provider.setProPhone(proPhone);
provider.setProFax(proFax);
provider.setProAddress(proAddress);
provider.setProDesc(proDesc);
provider.setModifyBy(((User)request.getSession().getAttribute(Constants.USER_SESSION)).getId());
provider.setModifyDate(new Date());
boolean flag = false;
ProviderService providerService = new ProviderServiceImpl();
flag = providerService.modify(provider);
if(flag){
response.sendRedirect(request.getContextPath()+"/jsp/provider.do?method=query");
}else{
request.getRequestDispatcher("providermodify.jsp").forward(request, response);
}
}
private void getProviderById(HttpServletRequest request, HttpServletResponse response,String url)
throws ServletException, IOException {
String id = request.getParameter("proid");
if(!StringUtils.isNullOrEmpty(id)){
ProviderService providerService = new ProviderServiceImpl();
Provider provider = null;
provider = providerService.getProviderById(id);
request.setAttribute("provider", provider);
request.getRequestDispatcher(url).forward(request, response);
}
}
private void add(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String proCode = request.getParameter("proCode");
String proName = request.getParameter("proName");
String proContact = request.getParameter("proContact");
String proPhone = request.getParameter("proPhone");
String proAddress = request.getParameter("proAddress");
String proFax = request.getParameter("proFax");
String proDesc = request.getParameter("proDesc");
Provider provider = new Provider();
provider.setProCode(proCode);
provider.setProName(proName);
provider.setProContact(proContact);
provider.setProPhone(proPhone);
provider.setProFax(proFax);
provider.setProAddress(proAddress);
provider.setProDesc(proDesc);
provider.setCreatedBy(((User)request.getSession().getAttribute(Constants.USER_SESSION)).getId());
provider.setCreationDate(new Date());
boolean flag = false;
ProviderService providerService = new ProviderServiceImpl();
flag = providerService.add(provider);
if(flag){
response.sendRedirect(request.getContextPath()+"/jsp/provider.do?method=query");
}else{
request.getRequestDispatcher("provideradd.jsp").forward(request, response);
}
}
private void query(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String queryProName = request.getParameter("queryProName");
String queryProCode = request.getParameter("queryProCode");
if(StringUtils.isNullOrEmpty(queryProName)){
queryProName = "";
}
if(StringUtils.isNullOrEmpty(queryProCode)){
queryProCode = "";
}
List<Provider> providerList = new ArrayList<Provider>();
ProviderService providerService = new ProviderServiceImpl();
providerList = providerService.getProviderList(queryProName,queryProCode);
request.setAttribute("providerList", providerList);
request.setAttribute("queryProName", queryProName);
request.setAttribute("queryProCode", queryProCode);
request.getRequestDispatcher("providerlist.jsp").forward(request, response);
}
/**
* Initialization of the servlet. <br>
*
* @throws ServletException if an error occurs
*/
public void init() throws ServletException {
// Put your code here
}
}
7.商品管理实现
7.1增删改查实现
7.1.1导入 billadd.jsp、billlist.jsp、billmodify.jsp、billview.jsp
7.1.2BillDao
package com.node.dao.bill;
import com.node.pojo.Bill;
import java.sql.Connection;
import java.util.List;
public interface BillDao {
/**
* 增加订单
* @param connection
* @param bill
* @return
* @throws Exception
*/
public int add(Connection connection, Bill bill)throws Exception;
/**
* 通过查询条件获取供应商列表-模糊查询-getBillList
* @param connection
* @param bill
* @return
* @throws Exception
*/
public List<Bill> getBillList(Connection connection, Bill bill)throws Exception;
/**
* 通过delId删除Bill
* @param connection
* @param delId
* @return
* @throws Exception
*/
public int deleteBillById(Connection connection, String delId)throws Exception;
/**
* 通过billId获取Bill
* @param connection
* @param id
* @return
* @throws Exception
*/
public Bill getBillById(Connection connection, String id)throws Exception;
/**
* 修改订单信息
* @param connection
* @param bill
* @return
* @throws Exception
*/
public int modify(Connection connection, Bill bill)throws Exception;
/**
* 根据供应商ID查询订单数量
* @param connection
* @param providerId
* @return
* @throws Exception
*/
public int getBillCountByProviderId(Connection connection, String providerId)throws Exception;
}
7.1.3BillDaoImpl
package com.node.dao.bill;
import com.mysql.cj.util.StringUtils;
import com.node.dao.BaseDao;
import com.node.pojo.Bill;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
public class BillDaoImpl implements BillDao{
public int add(Connection connection, Bill bill) throws Exception {
// TODO Auto-generated method stub
PreparedStatement pstm = null;
int flag = 0;
if(null != connection){
String sql = "insert into smbms_bill (billCode,productName,productDesc," +
"productUnit,productCount,totalPrice,isPayment,providerId,createdBy,creationDate) " +
"values(?,?,?,?,?,?,?,?,?,?)";
Object[] params = {bill.getBillCode(),bill.getProductName(),bill.getProductDesc(),
bill.getProductUnit(),bill.getProductCount(),bill.getTotalPrice(),bill.getIsPayment(),
bill.getProviderId(),bill.getCreatedBy(),bill.getCreationDate()};
flag = BaseDao.execute(connection, pstm, sql, params);
BaseDao.closeResource(null, pstm, null);
System.out.println("dao--------flag " + flag);
}
return flag;
}
public List<Bill> getBillList(Connection connection, Bill bill)
throws Exception {
// TODO Auto-generated method stub
PreparedStatement pstm = null;
ResultSet rs = null;
List<Bill> billList = new ArrayList<Bill>();
if(connection != null){
StringBuffer sql = new StringBuffer();
sql.append("select b.*,p.proName as providerName from smbms_bill b, smbms_provider p where b.providerId = p.id");
List<Object> list = new ArrayList<Object>();
if(!StringUtils.isNullOrEmpty(bill.getProductName())){
sql.append(" and productName like ?");
list.add("%"+bill.getProductName()+"%");
}
if(bill.getProviderId() > 0){
sql.append(" and providerId = ?");
list.add(bill.getProviderId());
}
if(bill.getIsPayment() > 0){
sql.append(" and isPayment = ?");
list.add(bill.getIsPayment());
}
Object[] params = list.toArray();
System.out.println("sql --------- > " + sql.toString());
rs = BaseDao.execute(connection, pstm, rs, sql.toString(), params);
while(rs.next()){
Bill _bill = new Bill();
_bill.setId(rs.getInt("id"));
_bill.setBillCode(rs.getString("billCode"));
_bill.setProductName(rs.getString("productName"));
_bill.setProductDesc(rs.getString("productDesc"));
_bill.setProductUnit(rs.getString("productUnit"));
_bill.setProductCount(rs.getBigDecimal("productCount"));
_bill.setTotalPrice(rs.getBigDecimal("totalPrice"));
_bill.setIsPayment(rs.getInt("isPayment"));
_bill.setProviderId(rs.getInt("providerId"));
_bill.setProviderName(rs.getString("providerName"));
_bill.setCreationDate(rs.getTimestamp("creationDate"));
_bill.setCreatedBy(rs.getInt("createdBy"));
billList.add(_bill);
}
BaseDao.closeResource(null, pstm, rs);
}
return billList;
}
public int deleteBillById(Connection connection, String delId)
throws Exception {
// TODO Auto-generated method stub
PreparedStatement pstm = null;
int flag = 0;
if(null != connection){
String sql = "delete from smbms_bill where id=?";
Object[] params = {delId};
flag = BaseDao.execute(connection, pstm, sql, params);
BaseDao.closeResource(null, pstm, null);
}
return flag;
}
public Bill getBillById(Connection connection, String id) throws Exception {
// TODO Auto-generated method stub
Bill bill = null;
PreparedStatement pstm = null;
ResultSet rs = null;
if(null != connection){
String sql = "select b.*,p.proName as providerName from smbms_bill b, smbms_provider p " +
"where b.providerId = p.id and b.id=?";
Object[] params = {id};
rs = BaseDao.execute(connection, pstm, rs, sql, params);
if(rs.next()){
bill = new Bill();
bill.setId(rs.getInt("id"));
bill.setBillCode(rs.getString("billCode"));
bill.setProductName(rs.getString("productName"));
bill.setProductDesc(rs.getString("productDesc"));
bill.setProductUnit(rs.getString("productUnit"));
bill.setProductCount(rs.getBigDecimal("productCount"));
bill.setTotalPrice(rs.getBigDecimal("totalPrice"));
bill.setIsPayment(rs.getInt("isPayment"));
bill.setProviderId(rs.getInt("providerId"));
bill.setProviderName(rs.getString("providerName"));
bill.setModifyBy(rs.getInt("modifyBy"));
bill.setModifyDate(rs.getTimestamp("modifyDate"));
}
BaseDao.closeResource(null, pstm, rs);
}
return bill;
}
public int modify(Connection connection, Bill bill) throws Exception {
// TODO Auto-generated method stub
int flag = 0;
PreparedStatement pstm = null;
if(null != connection){
String sql = "update smbms_bill set productName=?," +
"productDesc=?,productUnit=?,productCount=?,totalPrice=?," +
"isPayment=?,providerId=?,modifyBy=?,modifyDate=? where id = ? ";
Object[] params = {bill.getProductName(),bill.getProductDesc(),
bill.getProductUnit(),bill.getProductCount(),bill.getTotalPrice(),bill.getIsPayment(),
bill.getProviderId(),bill.getModifyBy(),bill.getModifyDate(),bill.getId()};
flag = BaseDao.execute(connection, pstm, sql, params);
BaseDao.closeResource(null, pstm, null);
}
return flag;
}
public int getBillCountByProviderId(Connection connection, String providerId)
throws Exception {
// TODO Auto-generated method stub
int count = 0;
PreparedStatement pstm = null;
ResultSet rs = null;
if(null != connection){
String sql = "select count(1) as billCount from smbms_bill where" +
" providerId = ?";
Object[] params = {providerId};
rs = BaseDao.execute(connection, pstm, rs, sql, params);
if(rs.next()){
count = rs.getInt("billCount");
}
BaseDao.closeResource(null, pstm, rs);
}
return count;
}
}
7.1.4BillService
package com.node.service.bill;
import com.node.pojo.Bill;
import java.util.List;
public interface BillService {
/**
* 增加订单
* @param bill
* @return
*/
public boolean add(Bill bill);
/**
* 通过条件获取订单列表-模糊查询-billList
* @param bill
* @return
*/
public List<Bill> getBillList(Bill bill);
/**
* 通过billId删除Bill
* @param delId
* @return
*/
public boolean deleteBillById(String delId);
/**
* 通过billId获取Bill
* @param id
* @return
*/
public Bill getBillById(String id);
/**
* 修改订单信息
* @param bill
* @return
*/
public boolean modify(Bill bill);
}
7.1.5BillServiceImpl
package com.node.service.bill;
import com.node.dao.BaseDao;
import com.node.dao.bill.BillDao;
import com.node.dao.bill.BillDaoImpl;
import com.node.pojo.Bill;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.List;
public class BillServiceImpl implements BillService{
private BillDao billDao;
public BillServiceImpl(){
billDao = new BillDaoImpl();
}
public boolean add(Bill bill) {
// TODO Auto-generated method stub
boolean flag = false;
Connection connection = null;
try {
connection = BaseDao.getConnection();
connection.setAutoCommit(false);//开启JDBC事务管理
if(billDao.add(connection,bill) > 0)
flag = true;
connection.commit();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
try {
System.out.println("rollback==================");
connection.rollback();
} catch (SQLException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}finally{
//在service层进行connection连接的关闭
BaseDao.closeResource(connection, null, null);
}
return flag;
}
public List<Bill> getBillList(Bill bill) {
// TODO Auto-generated method stub
Connection connection = null;
List<Bill> billList = null;
System.out.println("query productName ---- > " + bill.getProductName());
System.out.println("query providerId ---- > " + bill.getProviderId());
System.out.println("query isPayment ---- > " + bill.getIsPayment());
try {
connection = BaseDao.getConnection();
billList = billDao.getBillList(connection, bill);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
BaseDao.closeResource(connection, null, null);
}
return billList;
}
public boolean deleteBillById(String delId) {
// TODO Auto-generated method stub
Connection connection = null;
boolean flag = false;
try {
connection = BaseDao.getConnection();
if(billDao.deleteBillById(connection, delId) > 0)
flag = true;
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
BaseDao.closeResource(connection, null, null);
}
return flag;
}
public Bill getBillById(String id) {
// TODO Auto-generated method stub
Bill bill = null;
Connection connection = null;
try{
connection = BaseDao.getConnection();
bill = billDao.getBillById(connection, id);
}catch (Exception e) {
// TODO: handle exception
e.printStackTrace();
bill = null;
}finally{
BaseDao.closeResource(connection, null, null);
}
return bill;
}
public boolean modify(Bill bill) {
// TODO Auto-generated method stub
Connection connection = null;
boolean flag = false;
try {
connection = BaseDao.getConnection();
if(billDao.modify(connection,bill) > 0)
flag = true;
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}finally{
BaseDao.closeResource(connection, null, null);
}
return flag;
}
}
7.1.6BillServlet
package com.node.servlet.bill;
import com.alibaba.fastjson.JSONArray;
import com.mysql.cj.util.StringUtils;
import com.node.pojo.Bill;
import com.node.pojo.Provider;
import com.node.pojo.User;
import com.node.service.bill.BillService;
import com.node.service.bill.BillServiceImpl;
import com.node.service.provide.ProviderService;
import com.node.service.provide.ProviderServiceImpl;
import com.node.util.Constants;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
public class BillServlet extends HttpServlet {
/**
* The doGet method of the servlet. <br>
*
* This method is called when a form has its tag value method equals to get.
*
* @param request the request send by the client to the server
* @param response the response send by the server to the client
* @throws ServletException if an error occurred
* @throws IOException if an error occurred
*/
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doPost(request, response);
}
/**
* The doPost method of the servlet. <br>
*
* This method is called when a form has its tag value method equals to post.
*
* @param request the request send by the client to the server
* @param response the response send by the server to the client
* @throws ServletException if an error occurred
* @throws IOException if an error occurred
*/
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
/*String totalPrice = request.getParameter("totalPrice");
//23.234 45
BigDecimal totalPriceBigDecimal =
//设置规则,小数点保留两位,多出部分,ROUND_DOWN 舍弃
//ROUND_HALF_UP 四舍五入(5入) ROUND_UP 进位
//ROUND_HALF_DOWN 四舍五入(5不入)
new BigDecimal(totalPrice).setScale(2,BigDecimal.ROUND_DOWN);*/
String method = request.getParameter("method");
if(method != null && method.equals("query")){
this.query(request,response);
}else if(method != null && method.equals("add")){
this.add(request,response);
}else if(method != null && method.equals("view")){
this.getBillById(request,response,"billview.jsp");
}else if(method != null && method.equals("modify")){
this.getBillById(request,response,"billmodify.jsp");
}else if(method != null && method.equals("modifysave")){
this.modify(request,response);
}else if(method != null && method.equals("delbill")){
this.delBill(request,response);
}else if(method != null && method.equals("getproviderlist")){
this.getProviderlist(request,response);
}
}
private void getProviderlist(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
System.out.println("getproviderlist ========================= ");
List<Provider> providerList = new ArrayList<Provider>();
ProviderService providerService = new ProviderServiceImpl();
providerList = providerService.getProviderList("","");
//把providerList转换成json对象输出
response.setContentType("application/json");
PrintWriter outPrintWriter = response.getWriter();
outPrintWriter.write(JSONArray.toJSONString(providerList));
outPrintWriter.flush();
outPrintWriter.close();
}
private void getBillById(HttpServletRequest request, HttpServletResponse response,String url)
throws ServletException, IOException {
String id = request.getParameter("billid");
if(!StringUtils.isNullOrEmpty(id)){
BillService billService = new BillServiceImpl();
Bill bill = null;
bill = billService.getBillById(id);
request.setAttribute("bill", bill);
request.getRequestDispatcher(url).forward(request, response);
}
}
private void modify(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
System.out.println("modify===============");
String id = request.getParameter("id");
String productName = request.getParameter("productName");
String productDesc = request.getParameter("productDesc");
String productUnit = request.getParameter("productUnit");
String productCount = request.getParameter("productCount");
String totalPrice = request.getParameter("totalPrice");
String providerId = request.getParameter("providerId");
String isPayment = request.getParameter("isPayment");
Bill bill = new Bill();
bill.setId(Integer.valueOf(id));
bill.setProductName(productName);
bill.setProductDesc(productDesc);
bill.setProductUnit(productUnit);
bill.setProductCount(new BigDecimal(productCount).setScale(2,BigDecimal.ROUND_DOWN));
bill.setIsPayment(Integer.parseInt(isPayment));
bill.setTotalPrice(new BigDecimal(totalPrice).setScale(2,BigDecimal.ROUND_DOWN));
bill.setProviderId(Integer.parseInt(providerId));
bill.setModifyBy(((User)request.getSession().getAttribute(Constants.USER_SESSION)).getId());
bill.setModifyDate(new Date());
boolean flag = false;
BillService billService = new BillServiceImpl();
flag = billService.modify(bill);
if(flag){
response.sendRedirect(request.getContextPath()+"/jsp/bill.do?method=query");
}else{
request.getRequestDispatcher("billmodify.jsp").forward(request, response);
}
}
private void delBill(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String id = request.getParameter("billid");
HashMap<String, String> resultMap = new HashMap<String, String>();
if(!StringUtils.isNullOrEmpty(id)){
BillService billService = new BillServiceImpl();
boolean flag = billService.deleteBillById(id);
if(flag){//删除成功
resultMap.put("delResult", "true");
}else{//删除失败
resultMap.put("delResult", "false");
}
}else{
resultMap.put("delResult", "notexit");
}
//把resultMap转换成json对象输出
response.setContentType("application/json");
PrintWriter outPrintWriter = response.getWriter();
outPrintWriter.write(JSONArray.toJSONString(resultMap));
outPrintWriter.flush();
outPrintWriter.close();
}
private void add(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String billCode = request.getParameter("billCode");
String productName = request.getParameter("productName");
String productDesc = request.getParameter("productDesc");
String productUnit = request.getParameter("productUnit");
String productCount = request.getParameter("productCount");
String totalPrice = request.getParameter("totalPrice");
String providerId = request.getParameter("providerId");
String isPayment = request.getParameter("isPayment");
Bill bill = new Bill();
bill.setBillCode(billCode);
bill.setProductName(productName);
bill.setProductDesc(productDesc);
bill.setProductUnit(productUnit);
bill.setProductCount(new BigDecimal(productCount).setScale(2,BigDecimal.ROUND_DOWN));
bill.setIsPayment(Integer.parseInt(isPayment));
bill.setTotalPrice(new BigDecimal(totalPrice).setScale(2,BigDecimal.ROUND_DOWN));
bill.setProviderId(Integer.parseInt(providerId));
bill.setCreatedBy(((User)request.getSession().getAttribute(Constants.USER_SESSION)).getId());
bill.setCreationDate(new Date());
boolean flag = false;
BillService billService = new BillServiceImpl();
flag = billService.add(bill);
System.out.println("add flag -- > " + flag);
if(flag){
response.sendRedirect(request.getContextPath()+"/jsp/bill.do?method=query");
}else{
request.getRequestDispatcher("billadd.jsp").forward(request, response);
}
}
private void query(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
List<Provider> providerList = new ArrayList<Provider>();
ProviderService providerService = new ProviderServiceImpl();
providerList = providerService.getProviderList("","");
request.setAttribute("providerList", providerList);
String queryProductName = request.getParameter("queryProductName");
String queryProviderId = request.getParameter("queryProviderId");
String queryIsPayment = request.getParameter("queryIsPayment");
if(StringUtils.isNullOrEmpty(queryProductName)){
queryProductName = "";
}
List<Bill> billList = new ArrayList<Bill>();
BillService billService = new BillServiceImpl();
Bill bill = new Bill();
if(StringUtils.isNullOrEmpty(queryIsPayment)){
bill.setIsPayment(0);
}else{
bill.setIsPayment(Integer.parseInt(queryIsPayment));
}
if(StringUtils.isNullOrEmpty(queryProviderId)){
bill.setProviderId(0);
}else{
bill.setProviderId(Integer.parseInt(queryProviderId));
}
bill.setProductName(queryProductName);
billList = billService.getBillList(bill);
request.setAttribute("billList", billList);
request.setAttribute("queryProductName", queryProductName);
request.setAttribute("queryProviderId", queryProviderId);
request.setAttribute("queryIsPayment", queryIsPayment);
request.getRequestDispatcher("billlist.jsp").forward(request, response);
}
public static void main(String[] args) {
System.out.println(new BigDecimal("23.235").setScale(2,BigDecimal.ROUND_HALF_DOWN));
}
/**
* Initialization of the servlet. <br>
*
* @throws ServletException if an error occurs
*/
public void init() throws ServletException {
// Put your code here
}
}
下载链接: SMBMS-Java Web.