javaWeb项目介绍:
- 1、没有使用maven
- 2、使用注解加文件配置xml(只配置了错误页面)方式
- 3、只是用一个index.jsp页面配合js来完成整个项目,
- 4、使用原生js、axios方式请求数据
- 5、项目不完善,只适合javaWeb初级项目,可以练练手
- 6、…
项目gif图片
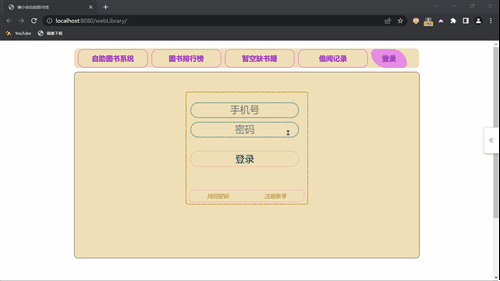
零、Tomcat服务器
1、下载和注意事项
- 可以在官网下载,但是速度太慢了👉官网
- 推荐使用镜像网站下载👉镜像网站
- 请注意各个版本对应的jdk版本
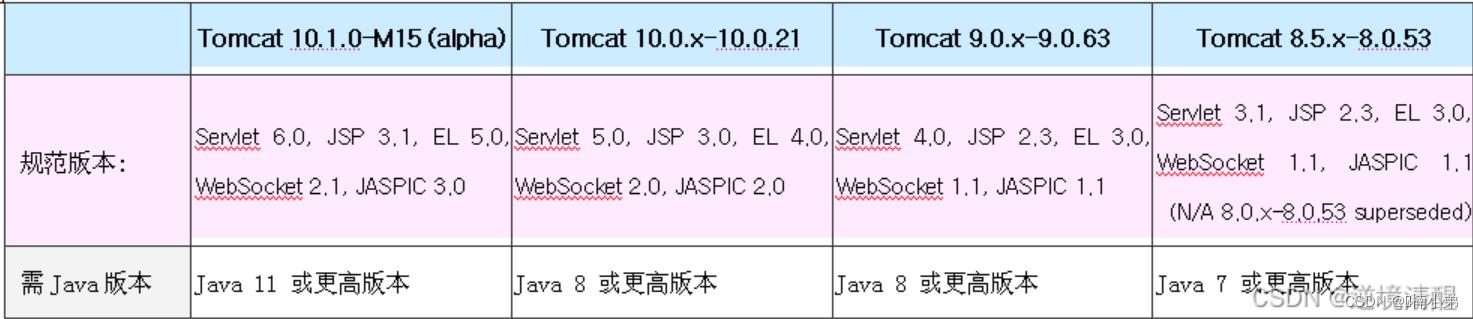
- 注意:
- 1 若版本为10.1.x则jdk最低为11,否则tomcat启动不了
- 2 要解压在没有中文字符的文件夹中
2、文件夹介绍
- bin文件夹 👉可执行文件
- conf文件夹👉全局配置文件
- lib文件夹👉依赖的jar包
- logs文件夹👉存放tomcat运行日志
- temp文件夹👉临时文件夹
- webapps文件夹👉放项目之地
- work 文件夹👉
一、 数据库
1、新建数据库weblibrary
create database weblibrary charset utf8;
use weblibrary;
2、创建用户表lbuser
create table if not exists lbUser(
id int primary key auto_increment,
uid varchar(30) unique not null default '',
uname varchar(50) default '',
upwd varchar(32) not null default '',
uage int default 18,
uphone char(11) default '',
uquestion varchar(50) default '',
uanwser varchar(50) default ''
) charset utf8;
3、创建图书表books
create table books(
id int primary key auto_increment,
bname varchar(50) not null default '',
bwriter varchar(30) not null default '',
btype varchar(30)not null default '',
bcomny varchar(30) not null default '',
bnum int default 0,
bstate int default 0,
bbnum int default 0
);
insert into weblibrary.books (id, bname, bwriter, btype, bcomny, bnum, bstate, bbnum)
values (1, '小王子', '埃克苏佩里', '童话', '光明出版社', 8, 0, 6),
(2, '天路历程', '约翰班杨', '文学', '光明出版社', 10, 0, 13),
(3, '活着', '余华', '文学', '光明出版社', 8, 0, 6),
(4, '岛上书店', '加泽文', '文学', '光明出版社', 10, 0, 0),
(5, '吞噬星空', '我吃西红柿', '小说', '阅文集团', 10, 0, 21),
(6, '平凡的世界', '路遥', '文学', '光明出版社', 10, 0, 0),
(7, '雨有时横着走', '郭怀宽', '诗歌', '光明出版社', 10, 0, 10),
(8, '百年孤独', '加西亚马尔科', '小说', '光明出版社', 10, 0, 0),
(9, '三体', '刘慈欣', '小说', '光明出版社', 1, 0, 54),
(10, '斗罗大陆', '唐家三少', '小说', '阅文集团', 0, 0, 79);
4、创建用户借阅表borrhistory
create table borrhistory(
id int primary key auto_increment,
uid varchar(11),
bid int not null ,
bname varchar(30) not null default '',
bwriter varchar(30) not null default '',
btype varchar(30) not null default '',
btime datetime,
rtime datetime,
bstate int default 0
);
二、开始建javaWeb项目
0、导入jar包:在WEB-INF下的lib包中导入相应的jar包
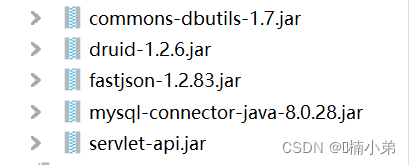
- 然后把他们添加到项目中
- 打开此项目设置,在设置中添加jar包
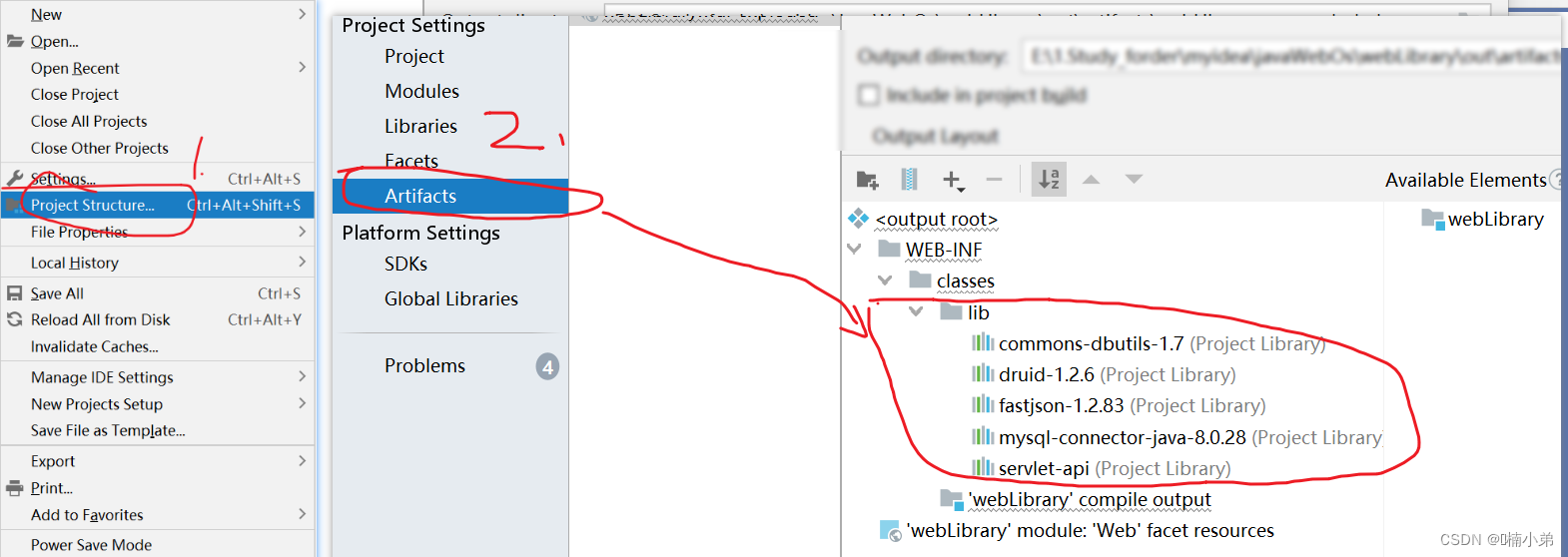
1、包的目录
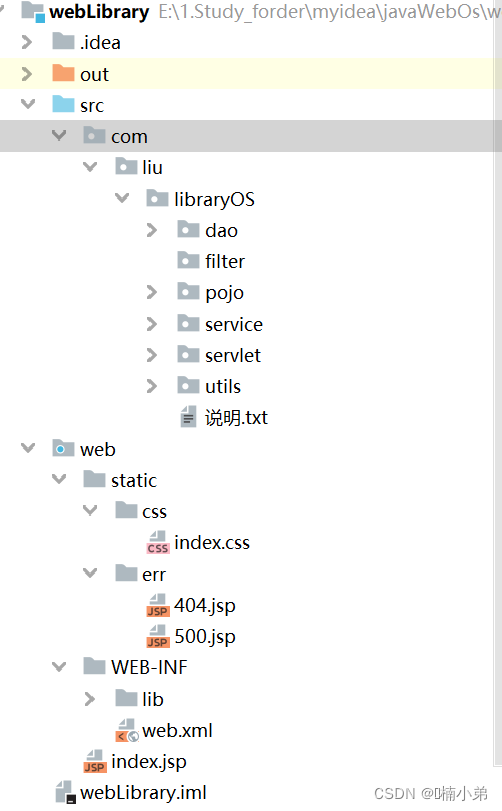
2、在utils包中创建
a、创建db.properties
driverClassName=com.mysql.cj.jdbc.Driver
url=jdbc:mysql://localhost:3306/weblibrary?serverTimeZone=Asia/ShangHai
#?????
username=root
#??
password=123456
#?????
initialSize=10
# ??????
maxActive=100
# ??????
maxWait=3000
b、创建JDBCDruid.java
public class JDBCDruid {
private static DataSource ds=null;
static {
try {
Properties p=new Properties();
p.load(new InputStreamReader(JDBCDruid.class.getClassLoader().getResourceAsStream("com/liu/libraryOS/utils/db.properties")));
ds= DruidDataSourceFactory.createDataSource(p);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public static Connection getConn(){
try {
return ds.getConnection();
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
public static void getClose(ResultSet rs, Statement st,Connection conn){
try {
if (rs!=null){
rs.close();
}
if(st!=null){
st.close();
}
if(conn!=null){
conn.close();
}
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
}
3、在pojo包中创建
a、创建Books.java类
public class Books implements Serializable {
private Integer id;
private String bname;
private String bwriter;
private String btype;
private String bcomny;
private Integer bnum;
private Integer bstate;
private Integer bbnum;
public Books() {
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getBname() {
return bname;
}
public void setBname(String bname) {
this.bname = bname;
}
public String getBwriter() {
return bwriter;
}
public void setBwriter(String bwriter) {
this.bwriter = bwriter;
}
public String getBtype() {
return btype;
}
public void setBtype(String btype) {
this.btype = btype;
}
public String getBcomny() {
return bcomny;
}
public void setBcomny(String bcomny) {
this.bcomny = bcomny;
}
public Integer getBnum() {
return bnum;
}
public void setBnum(Integer bnum) {
this.bnum = bnum;
}
public Integer getBstate() {
return bstate;
}
public void setBstate(Integer bstate) {
this.bstate = bstate;
}
public Integer getBbnum() {
return bbnum;
}
public void setBbnum(Integer bbnum) {
this.bbnum = bbnum;
}
}
b、创建借阅历史类BorrHistory.java
public class BorrHistory implements Serializable {
private Integer id;
private String uid;
private Integer bid;
private String bname;
private String bwriter;
private String btype;
private String btime;
private String rtime;
private String bstate;
public BorrHistory() {
}
public String getUid() {
return uid;
}
public void setUid(String uid) {
this.uid = uid;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public Integer getBid() {
return bid;
}
public void setBid(Integer bid) {
this.bid = bid;
}
public String getBname() {
return bname;
}
public void setBname(String bname) {
this.bname = bname;
}
public String getBwriter() {
return bwriter;
}
public void setBwriter(String bwriter) {
this.bwriter = bwriter;
}
public String getBtype() {
return btype;
}
public void setBtype(String btype) {
this.btype = btype;
}
public String getBtime() {
return btime;
}
public void setBtime(String btime) {
this.btime = btime;
}
public String getRtime() {
return rtime;
}
public void setRtime(String rtime) {
this.rtime = rtime;
}
public String getBstate() {
return bstate;
}
public void setBstate(String bstate) {
this.bstate = bstate;
}
}
c、创建用户类LbUser
public class LbUser implements Serializable {
private Integer id;
private String uid;
private String uname;
private int uage;
private String uquestion;
private String uanwser;
private String udate;
public LbUser() {
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getUid() {
return uid;
}
public void setUid(String uid) {
this.uid = uid;
}
public String getUname() {
return uname;
}
public void setUname(String uname) {
this.uname = uname;
}
public int getUage() {
return uage;
}
public void setUage(int uage) {
this.uage = uage;
}
public String getUquestion() {
return uquestion;
}
public void setUquestion(String uquestion) {
this.uquestion = uquestion;
}
public String getUanwser() {
return uanwser;
}
public void setUanwser(String uanwser) {
this.uanwser = uanwser;
}
public String getUdate() {
return udate;
}
public void setUdate(String udate) {
this.udate = udate;
}
}
4、在dao包中创建
a、创建父类BasicDao.java
public class BasicDao<T> {
private QueryRunner qr = new QueryRunner();
private Connection conn = null;
public List<T> getAllData(String sql, Class<T> clazz, Object... obj) {
try {
conn = JDBCDruid.getConn();
return qr.query(conn, sql, new BeanListHandler<>(clazz), obj);
} catch (SQLException e) {
throw new RuntimeException(e);
} finally {
JDBCDruid.getClose(null, null, conn);
}
}
public T getOneData(String sql, Class<T> clazz, Object... obj) {
try {
conn = JDBCDruid.getConn();
return qr.query(conn, sql, new BeanHandler<>(clazz), obj);
} catch (SQLException e) {
throw new RuntimeException(e);
} finally {
JDBCDruid.getClose(null, null, conn);
}
}
public boolean update(String sql, Object... obj) {
int num = 0;
try {
conn = JDBCDruid.getConn();
conn.setAutoCommit(false);
num = qr.update(conn, sql, obj);
conn.commit();
} catch (SQLException e) {
try {
conn.rollback();
} catch (SQLException ex) {
ex.printStackTrace();
}
throw new RuntimeException(e);
} finally {
JDBCDruid.getClose(null, null, conn);
}
return num>0;
}
public Connection startTransaction() {
try {
conn = JDBCDruid.getConn();
conn.setAutoCommit(false);
return conn;
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
public void rollBack(Connection conn) {
try {
conn.rollback();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
b、创建BooksDao.java
public class BooksDao extends BasicDao<Books>{
}
c、创建BorrHistory.java
public class BorrHistoryDao extends BasicDao<BorrHistory>{
}
d、创建LbUser.java
public class LbUserDao extends BasicDao<LbUser>{
}
5、在service包下创建
a、创建BookService.java
public class BooksService {
private BooksDao bd = new BooksDao();
private String sql = "";
public List<Books> getAllBook() {
sql = "select*from books where bnum>0";
return bd.getAllData(sql, Books.class);
}
public List<Books> paiHangBook() {
sql = "select*from books where bbnum>0 order by bbnum desc limit 0,5";
return bd.getAllData(sql, Books.class);
}
public List<Books> alreadyOverBook() {
sql = "select*from books where bnum<1 ";
return bd.getAllData(sql, Books.class);
}
public Books queryById(String bid) {
sql = "select*from books where id=?";
return bd.getOneData(sql, Books.class, bid);
}
}
b、创建BorrHistoryService
public class BorrHistoryService {
private BorrHistoryDao bd = new BorrHistoryDao();
private BooksService bs = new BooksService();
private String sql = "";
public List<BorrHistory> getAllData(String uid) {
sql = "select * from borrhistory where uid=?";
return bd.getAllData(sql, BorrHistory.class, uid);
}
public BorrHistory queryByBidCo(String count, String bid) {
sql = "select*from borrhistory where uid=? and bid=? and bstate=0";
return bd.getOneData(sql, BorrHistory.class, count, bid);
}
public boolean borrowBook(String count, String bid) {
Connection conn = bd.startTransaction();
QueryRunner qr = new QueryRunner();
int num = 0;
try {
sql = "update books set bnum=(bnum-1) where id=?";
num = qr