一、题目
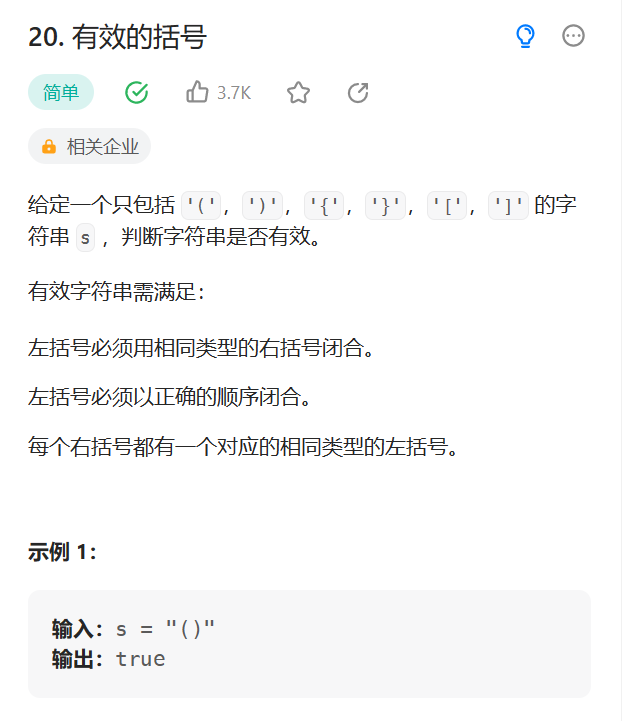
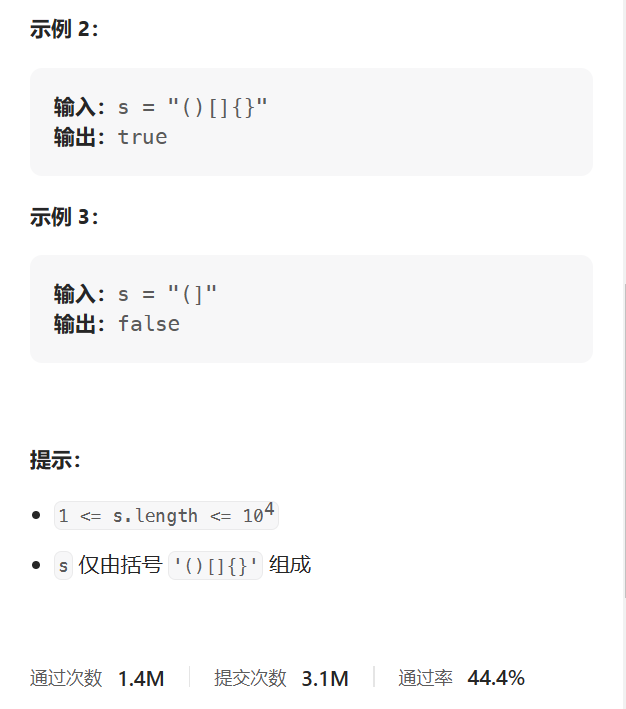
二、解题思路
这个题目我们可以使用数据结构与算法中顺序栈这个数据结构,栈长度为0时,我们进行入栈,之后的新字符入栈时开始比较是否匹配,对比准备入栈的字符和栈顶的字符,例如:左小括号是否对应右小括号,左中括号是否对应右中括号等。如果对应我们把栈顶的字符进行出栈,如果不对应我们把这个字符进行入栈。图解()[]{}:
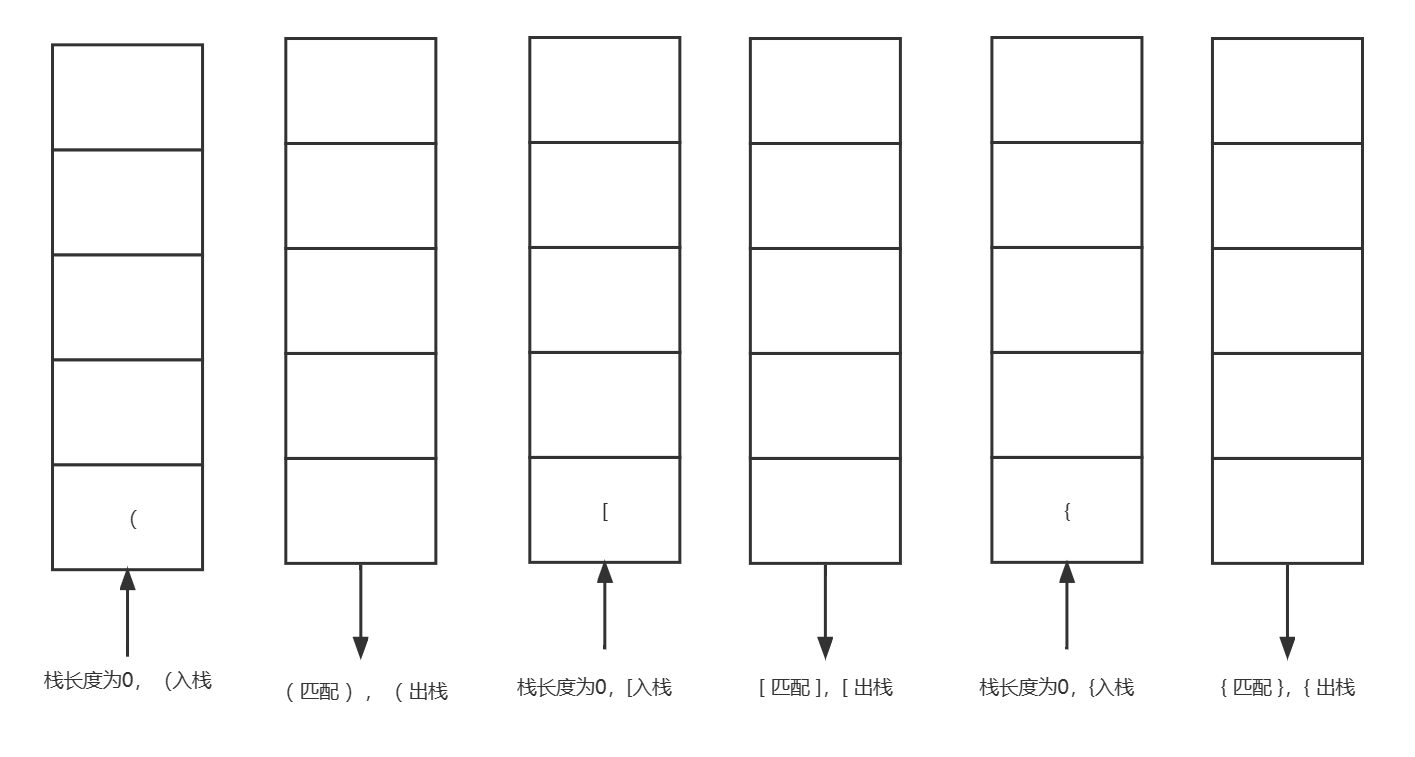
只需要遍历一遍所有数据,如果最后栈的长度为0,表示所有括号有效,反之无效,时间复杂度为O(n)。
三、虚机测试代码
1、lee_20_ValidBracket.c
#include <stdio.h>
#include <stdbool.h>
#include <stdlib.h>
#define ORDER_STACK_SIZE 10000
#define TEST_DATA_SIZE 3
typedef struct Stack
{
char* OrderStack;
int OrderStackLen;
}Stack;
bool isValid(char * s);
void PrintfStack(Stack* s);
bool JudgeBacketIsMatching(char c1, char c2);
int main()
{
char TestData[TEST_DATA_SIZE][10] = {"()", "()[]{}", "(]"};
int i;
for(i = 0; i < TEST_DATA_SIZE; i++)
{
printf("%s\n",TestData[i]);
printf("%d\n",isValid(TestData[i]));
}
return 1;
}
bool isValid(char * s){
if(s == NULL)
{
return false;
}
Stack* St = (Stack*)malloc(sizeof(Stack));
St->OrderStack = (char*)malloc(sizeof(char) * ORDER_STACK_SIZE);
St->OrderStackLen = 0;
int StringIndex = 0;
while(s[StringIndex] != '\0')
{
PrintfStack(St);
if(St->OrderStackLen == 0)
{
St->OrderStack[St->OrderStackLen] = s[StringIndex];
St->OrderStackLen++;
}
else if(JudgeBacketIsMatching(St->OrderStack[St->OrderStackLen - 1], s[StringIndex]))
{
St->OrderStackLen--;
}
else
{
St->OrderStack[St->OrderStackLen] = s[StringIndex];
St->OrderStackLen++;
}
StringIndex++;
}
if(St->OrderStackLen == 0)
{
free(St);
St = NULL;
return true;
}
free(St->OrderStack);
St->OrderStack = NULL;
free(St);
St = NULL;
return false;
}
bool JudgeBacketIsMatching(char c1, char c2)
{
if(c1 == '(' && c2 == ')')
{
return true;
}
else if(c1 == '[' && c2 == ']')
{
return true;
}
else if(c1 == '{' && c2 == '}')
{
return true;
}
return false;
}
void PrintfStack(Stack* s)
{
int i = 0;
printf("Stack : ");
while(i < s->OrderStackLen)
{
printf("%c ",s->OrderStack[i]);
i++;
}
printf("\nStack Len : %d\n",s->OrderStackLen);
}
四、虚机测试
[root@czg2 leecode_src]# gcc -Wall -O3 lee_20_ValidBracket.c -o ../exec/lee_20_ValidBracket
[root@czg2 leecode_src]# ../exec/lee_20_ValidBracket
()
Stack :
Stack Len : 0
Stack : (
Stack Len : 1
1
()[]{}
Stack :
Stack Len : 0
Stack : (
Stack Len : 1
Stack :
Stack Len : 0
Stack : [
Stack Len : 1
Stack :
Stack Len : 0
Stack : {
Stack Len : 1
1
(]
Stack :
Stack Len : 0
Stack : (
Stack Len : 1
0
[root@czg2 leecode_src]#
五、leetcode提交代码
#define ORDER_STACK_SIZE 10000
typedef struct Stack
{
char* OrderStack;
int OrderStackLen;
}Stack;
bool isValid(char * s);
void PrintfStack(Stack* s);
bool JudgeBacketIsMatching(char c1, char c2);
bool isValid(char * s){
if(s == NULL)
{
return false;
}
//printf("s : %s\n",s);
Stack* St = (Stack*)malloc(sizeof(Stack));
St->OrderStack = (char*)malloc(sizeof(char) * ORDER_STACK_SIZE);
St->OrderStackLen = 0;
int StringIndex = 0;
while(s[StringIndex] != '\0')
{
//PrintfStack(St);
if(St->OrderStackLen == 0)
{
St->OrderStack[St->OrderStackLen] = s[StringIndex];
St->OrderStackLen++;
}
else if(JudgeBacketIsMatching(St->OrderStack[St->OrderStackLen - 1], s[StringIndex]))
{
St->OrderStackLen--;
}
else
{
St->OrderStack[St->OrderStackLen] = s[StringIndex];
St->OrderStackLen++;
}
StringIndex++;
}
if(St->OrderStackLen == 0)
{
free(St);
St = NULL;
return true;
}
free(St->OrderStack);
St->OrderStack = NULL;
free(St);
St = NULL;
return false;
}
bool JudgeBacketIsMatching(char c1, char c2)
{
if(c1 == '(' && c2 == ')')
{
return true;
}
else if(c1 == '[' && c2 == ']')
{
return true;
}
else if(c1 == '{' && c2 == '}')
{
return true;
}
return false;
}
void PrintfStack(Stack* s)
{
int i = 0;
printf("Stack : ");
while(i < s->OrderStackLen)
{
printf("%c ",s->OrderStack[i]);
i++;
}
printf("\nStack Len : %d\n",s->OrderStackLen);
}
六、leetcode运行结果
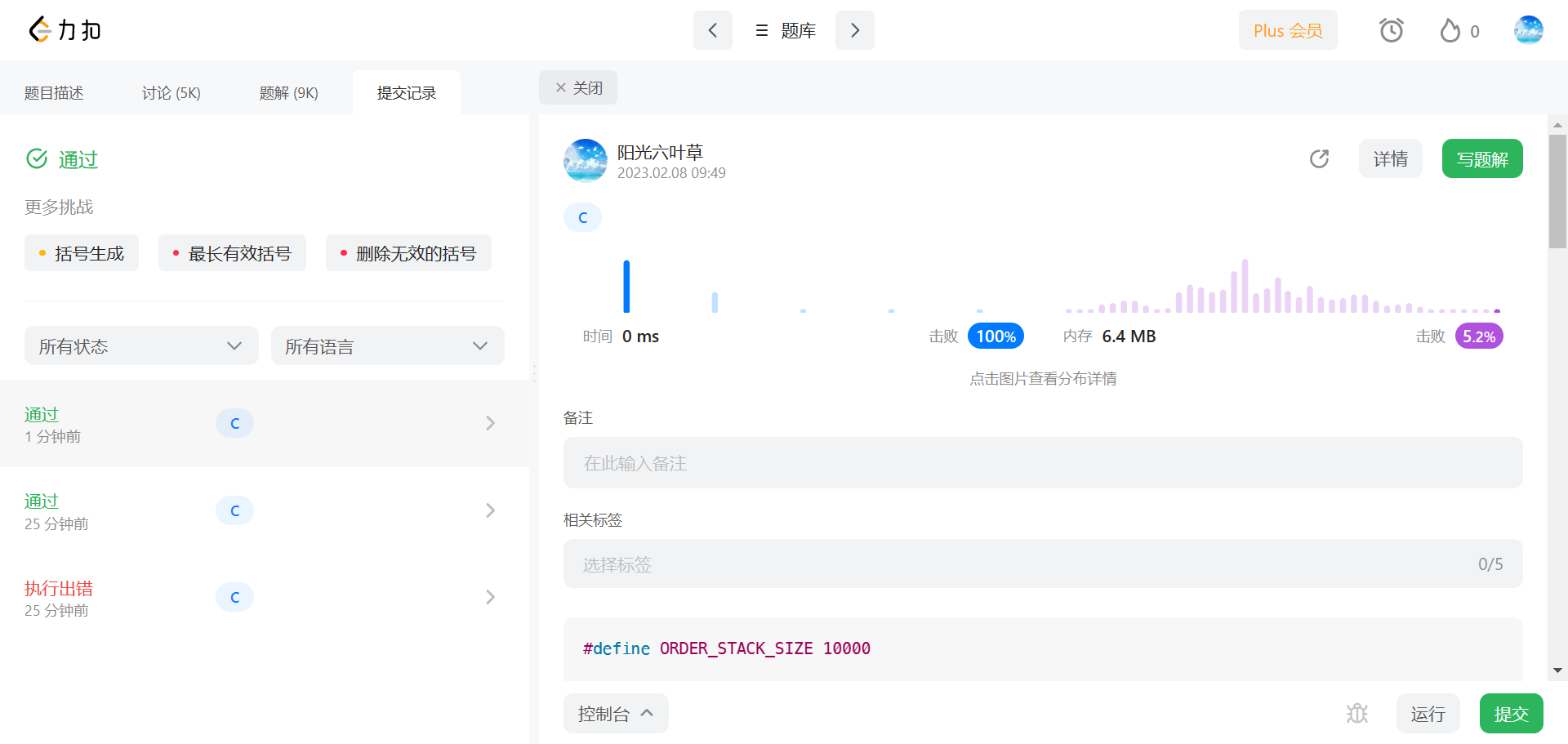