需求:vue-template-admin的项目开发后,需要添加隐私条款之类的在页面底部,点击后浏览器跳转一个新标签页并渲染页面。
方法一:
将一个简单的h5页面写好后部署到服务器去,然后获得链接,使用
window.open('链接')
这样就可以直接跳转。但这个方法太过繁琐,而且一般也没必要特意为了一个简单的文字展示页面部署环境。
方法二:
首先创建好terms.vue文件,再在router中注册一个路由
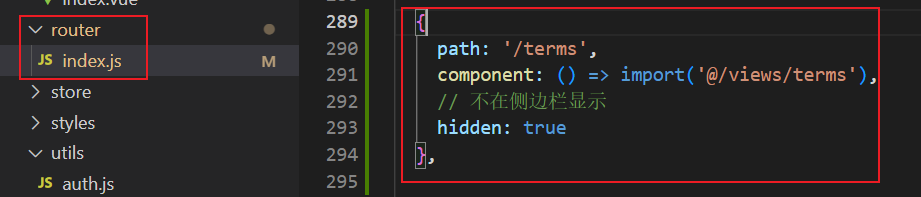
然后在你需要跳转的点击事件中写
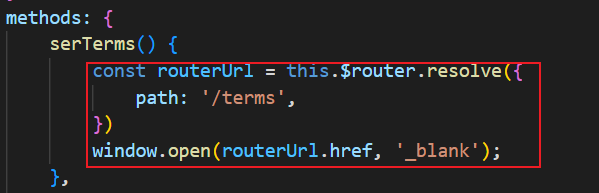
const routerUrl = this.$router.resolve({
path: '/terms',
})
window.open(routerUrl.href, '_blank')
这样就可以了,新标签页接的是原来系统的url,所以不需要另外部署,也很方便。
所需要的数据都可以在创建的terms.vue中通过接口获取,然后渲染就行。