C++语言实现
#include <bits/stdc++.h>
using namespace std;
struct Node {
int data;
Node* left;
Node* right;
};
struct Tree {
Node* root;
};
void Insert(Tree* tree, int value) {
Node* node = (Node*)malloc(sizeof(Node));
node->data = value;
node->left = NULL;
node->right = NULL;
if(tree->root == NULL)
tree->root = node;
else {
Node* temp = tree->root;
while(temp != NULL) {
if(value < temp->data) {
if(temp->left == NULL) {
temp->left = node;
return;
} else {
temp = temp->left;
}
} else {
if(temp->right == NULL) {
temp->right = node;
return;
} else
temp = temp->right;
}
}
}
}
void preorder(Node* node) {
if(node != NULL) {
cout << node->data << " ";
preorder(node->left);
preorder(node->right);
}
}
void inorder(Node* node) {
if(node != NULL) {
inorder(node->left);
cout << node->data << " ";
inorder(node->right);
}
}
void postorder(Node* node) {
if(node != NULL) {
postorder(node->left);
postorder(node->right);
cout << node->data << " ";
}
}
int findMaximum(Node* node) {
if(node == NULL)
return -1;
int value;
while(node != NULL) {
value = node->data;
node = node->right;
}
return value;
}
void bfs(Node* node) {
queue<Node*> q;
q.push(node);
while(!q.empty()) {
Node* temp = q.front();
cout << temp->data << " ";
q.pop();
if(temp->left != NULL)
q.push(temp->left);
if(temp->right != NULL)
q.push(temp->right);
}
}
int main() {
Tree tree;
tree.root = NULL;
int a[7] = {10, 2, 7, 13, 11, 18, 15};
for(int i = 0; i < 7; i++) {
Insert(&tree, a[i]);
}
cout << "层序遍历:" << endl;
bfs(tree.root);
cout << endl << endl << "先序遍历:" << endl;
preorder(tree.root);
cout << endl << endl << "中序遍历:" << endl;
inorder(tree.root);
cout << endl << endl << "后序遍历:" << endl;
postorder(tree.root);
cout << endl << endl << "二叉搜索树的最大值为:" << endl << findMaximum(tree.root) << endl;
return 0;
}
实现结果截图
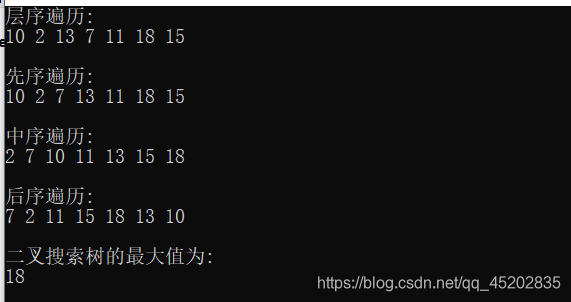