#pragma once
#define _CRT_SECURE_NO_WARNINGS
#include<stdio.h>
#include<stdlib.h>
#define QMAXSIZE 10
typedef int ElemType;
typedef struct SqQueue {
ElemType* base;
int front;
int rear;
}SqQueue,*pSqQueue;
int initQueue(pSqQueue psq)
{
psq->base = (ElemType*)malloc(sizeof(ElemType)*QMAXSIZE);
if (!psq)return 0;
psq->front = psq->rear = 0;
return 1;
}
void destroyQueue(pSqQueue psq)
{
if (!psq->base)free(psq->base);
psq->base = NULL;
psq->front = psq->rear = 0;
}
void clearQueue(pSqQueue psq)
{
psq->front = psq->rear = 0;
}
int isEmptyQueue(pSqQueue psq)
{
if (psq->front == psq->rear)return 1;
return 0;
}
int getHeadQueue(pSqQueue psq,ElemType& e)
{
if (psq->front == psq->rear)return 0;
e = psq->base[psq->front];
return 1;
}
int lengthQueue(pSqQueue psq)
{
return (psq->rear - psq->front);
}
int pushQueue(pSqQueue psq, ElemType e)
{
if (psq->rear >= QMAXSIZE)return 0;
psq->base[psq->rear] = e;
psq->rear += 1;
return 1;
}
int popQueue(pSqQueue psq,ElemType& e)
{
if (psq->front == psq->rear)return 0;
e = psq->base[psq->front];
psq->front += 1;
return 1;
}
void traverseQueue(pSqQueue psq, void(*callback)(ElemType))
{
int head = psq->front;
while (head != psq->rear)
(*callback)(psq->base[head++]);
printf("\n");
}
void qprint(ElemType e)
{
printf("%d ", e);
}
#include"sqqueue.h"
int main()
{
SqQueue psq;
int i=0, state;
ElemType e;
state = initQueue(&psq);
if (state)
printf("队列初始化成功!\n");
else
printf("队列初始化失败!\n");
printf("队列长度:%d\n", lengthQueue(&psq));
printf("队列头指针:%d\n", psq.front);
printf("队列尾指针:%d\n\n", psq.rear);
printf("向队列中尾插元素!\n");
while (1)
{
printf("尾插第%d个元素:", ++i);
scanf("%d", &e);
if (e == -999)break;
state=pushQueue(&psq, e);
if (!state) {
printf("队列已满!\n");
break;
}
}
printf("遍历队列中元素为:");
traverseQueue(&psq, qprint);
printf("队列长度:%d\n", lengthQueue(&psq));
printf("队列头指针:%d\n", psq.front);
printf("队列尾指针:%d\n\n", psq.rear);
getHeadQueue(&psq, e);
printf("队列头元素:%d\n\n", e);
printf("头删队列中一个元素!\n");
state = popQueue(&psq, e);
printf("删除成功!删除的元素为%d\n", e);
printf("遍历队列中元素为:");
traverseQueue(&psq, qprint);
printf("队列长度:%d\n", lengthQueue(&psq));
printf("队列头指针:%d\n", psq.front);
printf("队列尾指针:%d\n\n", psq.rear);
getHeadQueue(&psq, e);
printf("队列头元素:%d\n\n", e);
printf("清空队列!\n");
clearQueue(&psq);
printf("队列长度:%d\n", lengthQueue(&psq));
printf("队列头指针:%d\n", psq.front);
printf("队列尾指针:%d\n\n", psq.rear);
printf("队列是否为空[1-空,0-不空]:%d\n\n", isEmptyQueue(&psq));
printf("销毁队列!\n");
destroyQueue(&psq);
printf("队列长度:%d\n", lengthQueue(&psq));
printf("队列头指针:%d\n", psq.front);
printf("队列尾指针:%d\n", psq.rear);
printf("队列是否为空[1-空,0-不空]:%d\n\n", isEmptyQueue(&psq));
return 1;
}
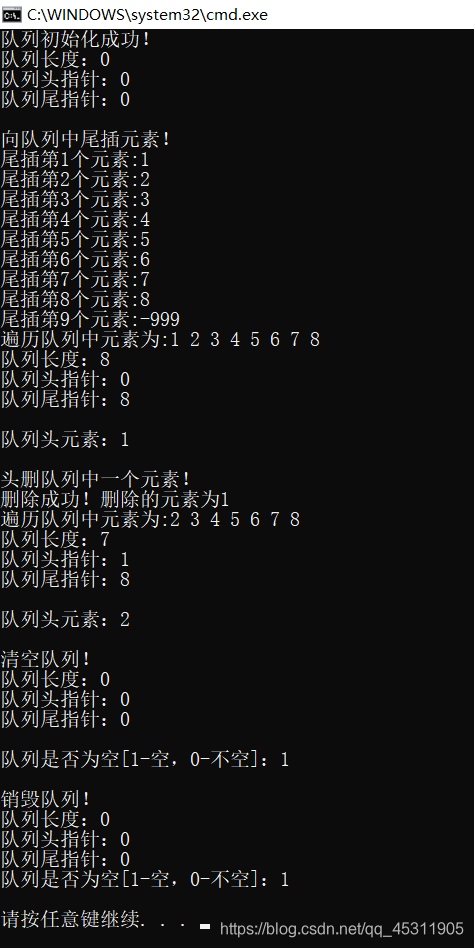