java代码, eclipse连接数据库
eclipse 连接 MySQL 数据库的基本操作
- 1.jar文件
java代码要想操作数据库需要引入jar文件
jar文件是eclipse连接数据库的驱动文件.
jar文件可在网上自行搜索下载.
jar包下载地址:https://mvnrepository.com
- 2 eclipse中导入jar包
eclipse中新建项目,直接把jar包复制到项目中
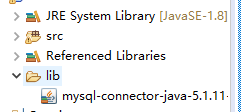
然后对jar包进行解压操作
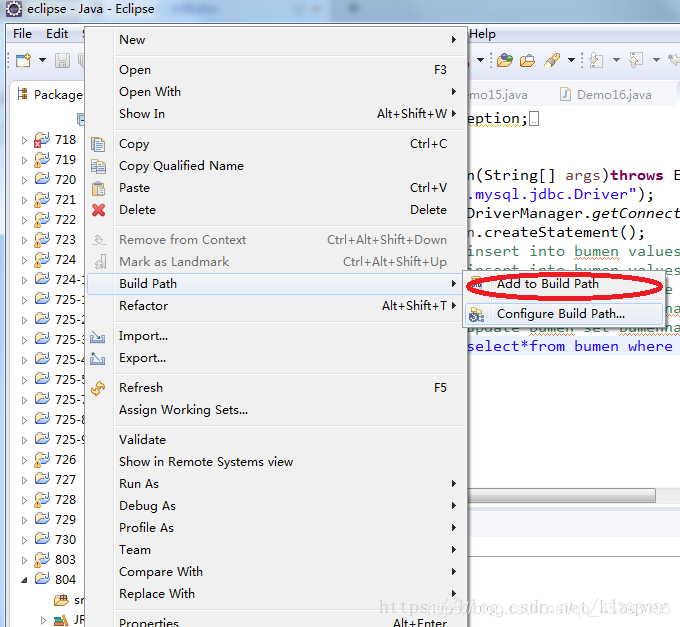
然后项目里新建class文件.
3.加载驱动
> 主函数里编写:Class.forName("com.mysql.jdbc.Driver");
4.建立连接
获取数据库连接对象
String url="jdbc:mysql://localhost:3306/ishopn?characterEncoding=utf8"; //url jdbc有自己的写法 jdbc:oracl
String user="root";
String password="1234";
conn = DriverManager.getConnection(url, user, password);
实现数据库的操作
1.编写sql语句
String query_sql="select*from commoditytype";
2.获取执行SQL语句的对象
Statement state = conn.createStatement();
3.执行SQL语句,实现查询
ResultSet rs=state.executeQuery(query_sql);
注意:要记得关闭连接
示例:
public static void main(String[] args) {
Connection conn = null;
try {
Class.forName("com.mysql.jdbc.Driver");
String url="jdbc:mysql://localhost:3306/ishopn?characterEncoding=utf8";
String user="root";
String password="1234";
conn = DriverManager.getConnection(url, user, password);
String query_sql="select*from commoditytype";
Statement state = conn.createStatement();
ResultSet rs=state.executeQuery(query_sql);
while(rs.next()) {
String cu_id=rs.getString(1);
String cu_name=rs.getString(2);
String cu_phone=rs.getString(3);
int cu_gender=rs.getInt(4);
String cu_address=rs.getString(5);
System.out.print(cu_id+":"+cu_name+"|"+cu_phone+"|"+cu_gender+"|"+cu_address);
System.out.println();
}
}catch(Exception e) {
e.printStackTrace();
}finally {
if(conn!=null) {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
-
除了查询语句之外,其他的修改,删除,添加语句返回值都为int类型,表示数据库中执行此命令后几行受到影响
-
连接MySQL数据库用到DriverManager类,通过DriverManager类中的getConnection()方法可以建立连接,则经连接返回,否则抛出SQLException异常。
模仿登录
public static void main(String[] args) {
Scanner scanner=new Scanner(System.in);
Connection conn = null;
try {
Class.forName("com.mysql.jdbc.Driver");
String url="jdbc:mysql://localhost:3306/ishopn?characterEncoding=utf8";
String user="root";
String password="1234";
conn = DriverManager.getConnection(url, user, password);
String cu_name="' or 1=1 #";
String cu_phone="dadadadad";
String sql = "select count(*) from customer where cu_name='"+cu_name+"' and cu_phone='"+cu_phone+"'";
Statement state = conn.createStatement();
ResultSet rs = state.executeQuery(sql);
rs.next();
int count=rs.getInt(1);
if(count>0) {
System.out.println("登录成功!");
}else {
System.out.println("用户名或手机号错误,登录失败!");
}
}catch(Exception e) {
e.printStackTrace();
}finally {
if(conn!=null) {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
如何实现查询 来解决注入攻击
public static void main(String[] args) {
Connection conn = null;
try {
Class.forName("com.mysql.jdbc.Driver");
String url="jdbc:mysql://localhost:3306/ishopn?characterEncoding=utf8";
String user="root";
String password="1234";
conn = DriverManager.getConnection(url, user, password);
String cu_id = "2a4e0cdd-380e-41bf-960f-f2f6d0a6ccae";
String cu_phone="9999";
String sql = "update customer set cu_phone=? where cu_id=?";
PreparedStatement ps = conn.prepareStatement(sql);
ps.setString(1, cu_phone);
ps.setString(2, cu_id);
int line = ps.executeUpdate();
System.out.println(line);
}catch(Exception e) {
e.printStackTrace();
}finally {
if(conn!=null) {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}