<template>
<div>
<a-tree :treeData="treeData">
<span slot="switcherIcon" class="icon-plus"></span>
<template slot="custom" slot-scope="item">
<!-- if -->
<span v-if="item.isNewItem">
<a-input placeholder="请输入内容" class="editInput" v-model="item.name" />
<span class="tree-cancle_icon edit-require_icon">
<a-icon type="close-circle" style="font-size:16px" @click="closecontent(item)" />
</span>
<span class="tree-save_icon edit-require_icon">
<a-icon type="check-circle" style="font-size:16px" @click="addinputcontent(item)" />
</span>
</span>
<!-- else -->
<div v-else>
<!-- 如果是修改 -->
<div v-if="item.isEdit">
<a-input v-model="item.title" class="editInput" />
<span class="tree-cancle_icon edit-require_icon" @click="editnocontent(item)">
<a-icon type="close-circle" />
</span>
<span class="tree-save_icon edit-require_icon" @click="edityescontent(item)">
<a-icon type="check-circle" />
</span>
</div>
<div v-else>
<span class="node-title">{{ item.title }}</span>
<span class="icon-wrap" @click="addNode(item)">
<a-icon type="plus" />
</span>
<span class="icon-wrap" @click="editNode(item)">
<a-icon type="form" />
</span>
<a-popconfirm title="确定删除吗?" ok-text="确定" cancel-text="取消" @confirm="confirm" @cancel="cancel">
<span class="icon-wrap" @click="delNode(item)">
<a-icon type="delete" />
</span>
</a-popconfirm>
</div>
</div>
</template>
</a-tree>
</div>
</template>
<script>
export default {
data() {
return {
codeArray: [],
treeData: [
{
id: 9,
key: 0,
isEdit: false,
isNewItem: false,
title: '七年级',
depth: 1,
scopedSlots: { title: 'custom' },
children: []
},
{
id: 10,
key: 1,
isEdit: false, // 是否处于编辑状态
isNewItem: false, // 该节点是否是新增节点
title: '八年级',
depth: 1, // 该节点的层级
scopedSlots: { title: 'custom' }, // 自定义组件需要绑定
children: [
{
id: 2,
key: 2,
isEdit: false, // 是否处于编辑状态
isNewItem: false, // 该节点是否是新增节点
title: '八(一)班',
depth: 2, // 该节点的层级
scopedSlots: { title: 'custom' }
}
]
},
{
id: 11,
key: 2,
isEdit: false,
isNewItem: false,
title: '九年级',
depth: 1,
scopedSlots: { title: 'custom' },
children: []
}
]
};
},
watch: {},
methods: {
confirm(e) {
console.log(e);
this.$message.success('Click on Yes');
},
cancel(e) {
this.$message.error('取消操作');
},
addNode(item) {
//点击新增
console.log(this.treeData,'aaaa', item);
let code = item.pos.replace(/-/g, '');
let codeArray = code.split('');
this.codeArray = codeArray;
let newNode = {
id: Math.ceil(Math.random() * 10000), //避免和已有的id冲突
key: Math.ceil(Math.random() * 10000), //避免和已有的key冲突
isEdit: false,
name: '',
isNewItem: true,
title: '',
depth: item.depth + 1, //如需要添加顶级节点,值为0
scopedSlots: { title: 'custom' },
children: []
};
let index = item.depth - 1;
console.log(codeArray)
this.treeData[codeArray[1]].children.push(newNode);
},
editnocontent(item){ //修改的时候的叉叉
let codeArray = this.codeArray;
let treeData = this.treeData
function queryindex(x){ //[1,2]
if(x.length == 1){
return treeData[codeArray[0]].isEdit = false;
}else{
return treeData[codeArray[0]].children[codeArray[1]].isEdit = false
}
}
queryindex(codeArray)
},
edityescontent(item){ //修改时候的对号
let codeArray = this.codeArray;
let treeData = this.treeData
function queryindex(x){ //[1,2]
if(x.length == 1){
treeData[codeArray[0]].isEdit = false
return treeData[codeArray[0]].title = item.title;
}else{
treeData[codeArray[0]].children[codeArray[1]].isEdit = false
return treeData[codeArray[0]].children[codeArray[1]].title = item.title
}
}
queryindex(codeArray)
},
addinputcontent(item) {
//点击确定
let newNode = {
id: item.id, //避免和已有的id冲突
key: item.key, //避免和已有的key冲突
isEdit: false,
isNewItem: false,
title: item.name,
depth: item.depth + 1, //如需要添加顶级节点,值为0
scopedSlots: { title: 'custom' },
children: []
};
let codeindex = this.codeArray[1];
this.treeData[codeindex].children.pop();
this.treeData[codeindex].children.push(newNode);
},
closecontent(item) {
//点击叉掉
console.log(item);
let code = item.pos.replace(/-/g, '');
let codeArray = code.split('');
codeArray.shift();
this.treeData[codeArray[0]].children.splice(codeArray[1],1);
},
editNode(item) {
//点击修改
let code = item.pos.replace(/-/g, '');
let codeArray = code.split('');
codeArray.shift();
this.codeArray = codeArray;
let treeData = this.treeData
function queryindex(x){ //[1,2]
if(x.length == 1){
return treeData[codeArray[0]].isEdit = true;
}else{
return treeData[codeArray[0]].children[codeArray[1]].isEdit = true
}
}
queryindex(codeArray)
},
delNode(item) {
//删除
let code = item.pos.replace(/-/g, '');
let codeArray = code.split('');
codeArray.shift();
let treeData = this.treeData
function queryindex(x){ //[1,2]
if(x.length == 1){
treeData.splice(codeArray[0],1);
}else{
treeData[codeArray[0]].children.splice(codeArray[1],1);
}
}
queryindex(codeArray)
}
}
};
</script>
<style lang="scss" scoped>
::v-deep .ant-tree-switcher.ant-tree-switcher_open {
.icon-plus {
background-image: url("./minus.png") ; // 展开节点时的icon
background-size: 24px;
width: 24px;
height: 24px;
}
}
::v-deep .ant-tree-switcher.ant-tree-switcher_close {
.icon-plus {
background-image: url("./add.png") ; // 收起节点时的icon
background-size: 24px;
width: 24px;
height: 24px;
}
}
.icon-wrap {
margin: 0 6px;
}
.node-title {
padding-right: 15px;
}
.tree-cancle_icon {
margin: 0 6px;
}
</style>
antdvue的tree组件增加增删改功能
最新推荐文章于 2024-09-19 16:49:00 发布
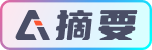