一、逻辑分析
汽车类(抽象父类)
含三个成员变量:品牌、车牌号、日租金;
含一个抽象方法用来计算租金;
轿车类(子类)
含一个特有属性,车型号;
重写父类抽象方法;
客车类(子类)
含一个特有属性,座位数;
重写父类抽象方法;
4.功能实现类(可以使用静态方法,方便调用)
初始化汽车仓库,使用Vehicle类对象数组;
主界面显示方法;
响应用户输入方法;
计算租金的方法;
5.主类main方法
由于静态方法,直接调用
二、上成品图
主界面
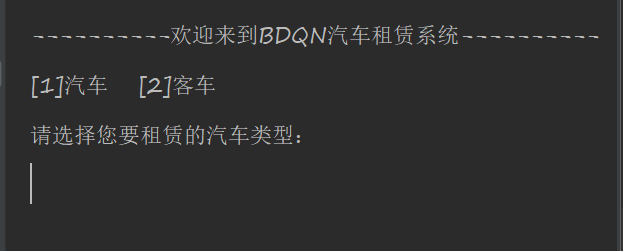
租汽车
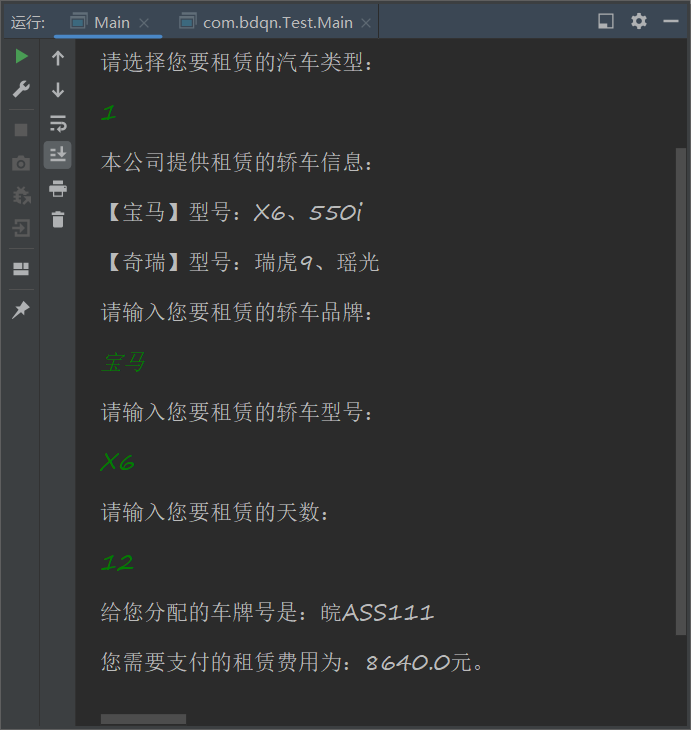
3.租客车
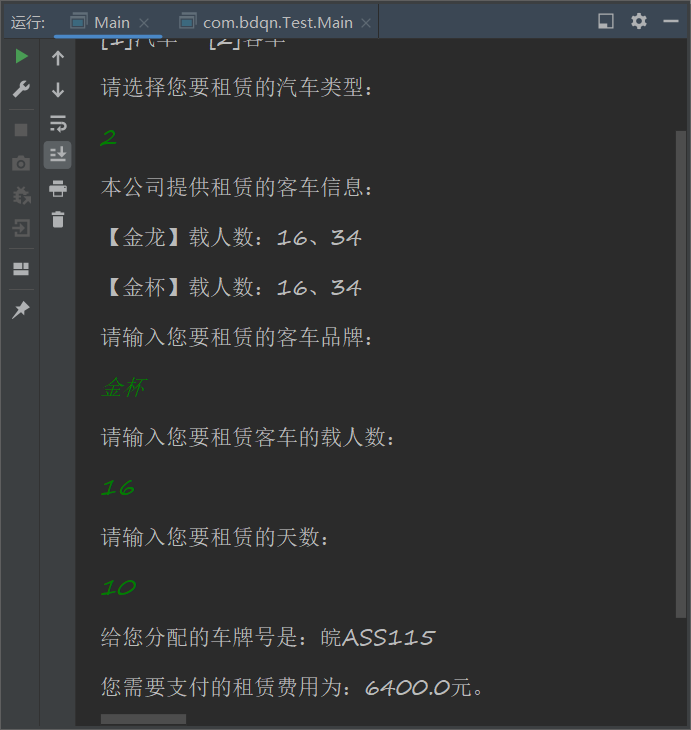
4.错误输入提醒,并重新输入
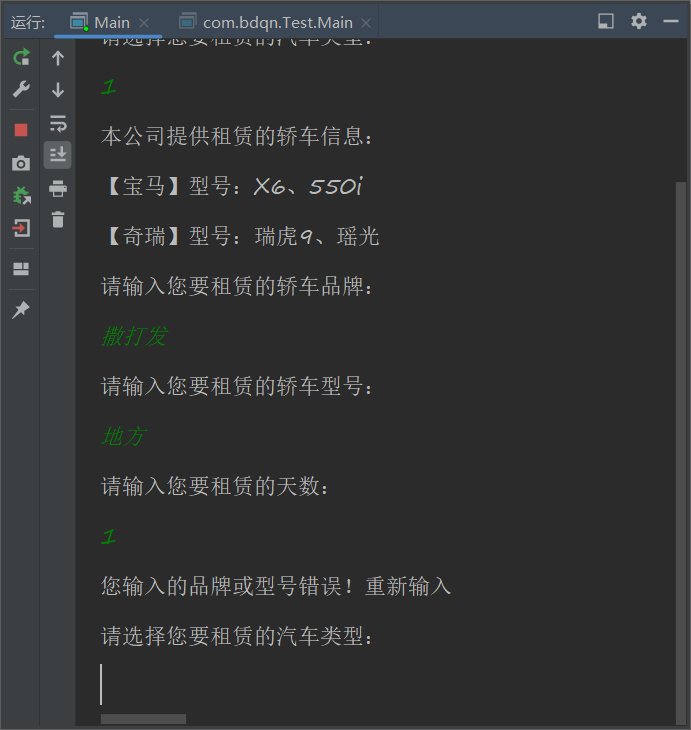
三、源码
Vehicle类:
public abstract class Vehicle {
//汽车车牌
private String numberplate;
//品牌
private String brand;
//租金
private double rent;
//构造
public Vehicle() {
}
public Vehicle(String numberplate, String brand, double rent) {
this.numberplate = numberplate;
this.brand = brand;
this.rent = rent;
}
//setter getter
public String getNumberplate() {
return numberplate;
}
public void setNumberplate(String numberplate) {
this.numberplate = numberplate;
}
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
public double getRent() {
return rent;
}
public void setRent(double rent) {
this.rent = rent;
}
//重写toString方法
@Override
public String toString() {
return "Vehicle{" +
"numberplate='" + numberplate + '\'' +
", brand='" + brand + '\'' +
", rent=" + rent +
'}';
}
//计算租金
public abstract double calculate(int days);
}
Car类:
public class Car extends Vehicle {
//型号
private String type;
//构造方法
public Car(String numberplate, String brand, double rent, String type) {
super(numberplate, brand, rent);
this.type = type;
}
public Car() {
}
//setter getter
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
//重写toString
@Override
public String toString() {
return "Car{" +
"type='" + type + '\'' +
'}';
}
//实现方法 计算租金
@Override
public double calculate(int days) {
if(days>150)
return getRent()*0.7*days;
if (days>30)
return getRent()*0.8*days;
if (days>7)
return getRent()*0.9*days;
return days*getRent();
}
}
Bus类:
public class Bus extends Vehicle {
//座位
private int seat;
//构造方法
public Bus() {
}
public Bus(String numberplate, String brand, double rent, int seat) {
super(numberplate, brand, rent);
this.seat = seat;
}
//setter getter
public int getSeat() {
return seat;
}
public void setSeat(int seat) {
this.seat = seat;
}
//重写toString
@Override
public String toString() {
return "Bus{" +
"seat=" + seat +
'}';
}
//实现方法 计算租金
@Override
public double calculate(int days) {
if (days >= 150)
return getRent() * 0.6 * days;
if (days >= 30)
return getRent() * 0.7 * days;
if (days >= 7)
return getRent() * 0.8 * days;
if (days >= 3)
return getRent() * 0.9 * days;
return getRent() * days;
}
}
Manage类:
import java.util.Scanner;
public class Manage {
//实例化输入对象
static Scanner sc = new Scanner(System.in);
//声明Vehicle对象数组
static Vehicle[] vehicles = new Vehicle[8];
//初始化类对象数组 静态方法
public static void init() {
//赋值
vehicles[0] = new Car("皖ASS111", "宝马", 800, "X6");
vehicles[1] = new Car("皖ASS112", "宝马", 600, "550i");
vehicles[2] = new Car("皖ASS113", "奇瑞", 200, "瑞虎9");
vehicles[3] = new Car("皖ASS114", "奇瑞", 400, "瑶光");
vehicles[4] = new Bus("皖ASS115", "金杯", 800, 16);
vehicles[5] = new Bus("皖ASS116", "金龙", 800, 16);
vehicles[6] = new Bus("皖ASS117", "金杯", 1500, 34);
vehicles[7] = new Bus("皖ASS118", "金龙", 1500, 34);
}
//判断哪个车的租金计算 静态方法
public static double judgeRentCalculate(Vehicle vehicle, String brand, String type, int seat, int days) {
if (vehicle instanceof Car car) {
if (type.equals("X6"))
return car.calculate(days);
if (type.equals("550i"))
return car.calculate(days);
if (type.equals("瑞虎9"))
return car.calculate(days);
if (type.equals("瑶光"))
return car.calculate(days);
} else if (vehicle instanceof Bus bus) {
if (seat == 16 && brand.equals("金杯"))
return bus.calculate(days);
if (seat == 16 && brand.equals("金龙"))
return bus.calculate(days);
if (seat == 34 && brand.equals("金杯"))
return bus.calculate(days);
if (seat == 34 && brand.equals("金龙"))
return bus.calculate(days);
}
return 0;
}
//主界面 静态方法
public static void mainInterface() {
System.out.println("----------欢迎来到BDQN汽车租赁系统----------");
System.out.println("[1]汽车\t[2]客车");
}
//已弃用 对用户的选择进行响应 静态方法 原因输入数字不人性化
public static void select() {
while (true) {
System.out.println("请选择您要租赁的汽车类型:");
int input1 = sc.nextInt();
//轿车
if (input1 == 1) {
System.out.println("[1]宝马\t[2]奇瑞");
int input2 = sc.nextInt();
//宝马
if (input2 == 1) {//X6 550i
System.out.println("请选择该品牌的型号:\n[1]X6\t[2]550i");
int input3 = sc.nextInt();
System.out.println("请输入您要租赁的天数:");//天数
int days = sc.nextInt();
if (input3 == 1) {//X6
System.out.println("给您分配的宝马X6车牌为:" + vehicles[0].getNumberplate());
System.out.println("您需要支付的租金为:" + judgeRentCalculate(vehicles[0], "宝马", "X6", 5, days) + "元。");
break;
} else if (input3 == 2) {//550i
System.out.println("给您分配的宝马550i车牌为:" + vehicles[1].getNumberplate());
System.out.println("您需要支付的租金为:" + vehicles[1].calculate(days) + "元。");
break;
}
} else if (input2 == 2) {//奇瑞
System.out.println("请选择该品牌的型号:\n[1]瑞虎9\t[2]瑶光");
int input3 = sc.nextInt();
System.out.println("请输入您要租赁的天数:");//天数
int days = sc.nextInt();
if (input3 == 1) {//瑞虎9
System.out.print("给您分配的奇瑞瑞虎9车牌为:" + vehicles[2].getNumberplate());
System.out.println("您需要支付的租金为:" + vehicles[2].calculate(days) + "元。");
break;
} else if (input3 == 2) {//瑶光
System.out.print("给您分配的奇瑞瑶光车牌为:" + vehicles[3].getNumberplate());
System.out.println("您需要支付的租金为:" + vehicles[3].calculate(days) + "元。");
break;
}
}
//客车
} else if (input1 == 2) {//客车
System.out.println("[1]金杯\t[2]金龙");
int input2 = sc.nextInt();
if (input2 == 1) {//金杯16 34
System.out.println("请选择该品牌的座位数:\n[1]16座\t[2]34座");
int input3 = sc.nextInt();
System.out.println("请输入您要租赁的天数:");//天数
int days = sc.nextInt();
if (input3 == 1) {
System.out.print("给您分配的金杯16座车牌为:" + vehicles[4].getNumberplate());
System.out.println("您需要支付的租金为:" + vehicles[4].calculate(days) + "元。");
break;
} else if (input3 == 2) {
System.out.print("给您分配的金杯34座车牌为:" + vehicles[6].getNumberplate());
System.out.println("您需要支付的租金为:" + vehicles[6].calculate(days) + "元。");
break;
}
} else if (input2 == 2) {//金龙 16 34
System.out.println("请选择该品牌的座位数:\n[1]16座\t[2]34座");
int input3 = sc.nextInt();
System.out.println("请输入您要租赁的天数:");//天数
int days = sc.nextInt();
if (input3 == 1) {
System.out.print("给您分配的金龙16座车牌为:" + vehicles[5].getNumberplate());
System.out.println("您需要支付的租金为:" + vehicles[5].calculate(days) + "元。");
break;
} else if (input3 == 2) {
System.out.print("给您分配的金龙34座车牌为:" + vehicles[7].getNumberplate());
System.out.println("您需要支付的租金为:" + vehicles[7].calculate(days) + "元。");
break;
}
}
} else {
System.out.println("-----您输入有误-----");
}
}
}
//对用户的选择进行响应 静态方法
public static void select1() {
While:while (true) {
System.out.println("请选择您要租赁的汽车类型:");//汽车 客车
int input1 = sc.nextInt();
if (input1 == 1) {
System.out.println("本公司提供租赁的轿车信息:\n【宝马】型号:X6、550i\n【奇瑞】型号:瑞虎9、瑶光");//宝马 奇瑞
System.out.println("请输入您要租赁的轿车品牌:");
String brand = sc.next();
System.out.println("请输入您要租赁的轿车型号:");
String type = sc.next();
System.out.println("请输入您要租赁的天数:");
int days = sc.nextInt();
for (Vehicle v : vehicles) {
if (judgeRentCalculate(v, brand, type, 5, days) == 0) {
System.out.println("您输入的品牌或型号错误!重新输入");
continue While;
}
if (((Car) v).getType().equals(type)) {
System.out.println("给您分配的车牌号是:" + v.getNumberplate());
System.out.println("您需要支付的租赁费用为:" + judgeRentCalculate(v, brand, type, 5, days) + "元。");
break While;
}
}
} else if (input1 == 2) {
System.out.println("本公司提供租赁的客车信息:\n【金龙】载人数:16、34\n【金杯】载人数:16、34");//宝马 奇瑞
System.out.println("请输入您要租赁的客车品牌:");
String brand = sc.next();
System.out.println("请输入您要租赁客车的载人数:");
int seat = sc.nextInt();
System.out.println("请输入您要租赁的天数:");
int days = sc.nextInt();
for(int i = 4;i<=7;i++) {
if (judgeRentCalculate(vehicles[i], brand, "type", seat, days) == 0) {
System.out.println("您输入的品牌或型号错误!重新输入");
continue While;
}
if (((Bus) vehicles[i]).getSeat() == seat && ((Bus) vehicles[i]).getBrand().equals(brand)) {
System.out.println("给您分配的车牌号是:" + vehicles[i].getNumberplate());
System.out.println("您需要支付的租赁费用为:" + judgeRentCalculate(vehicles[i], brand, "type", seat, days) + "元。");
break While;
}
}
} else {
System.out.println("您输入1或2");
}
}
}
}
Main类:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
//初始化
Manage.init();
//显示主界面
Manage.mainInterface();
//响应用户输入的操作
Manage.select1();
}
}
四、注意
如果对nextInt()等方法进行错误输入优化,则需要try{}catch(){},异常处理。