Json web token (jwt)的构成
- jwt 由三部分组成
- 头部分
{
'typ': 'JWT',
'alg': 'HS256'
}
-playload 负载部分 存放数据
-iss (issuer):签发人
- sub (subject):主题
- aud (audience):受众
- exp (expiration time):过期时间
- nbf (Not Before):生效时间,在此之前是无效的
- iat (Issued At):签发时间
- jti (JWT ID):编号
- 私钥 部分
- 使用私钥,为了保证token不被篡改
代码
引入Microsoft.AspNetCore.Authentication.JwtBearer
在appsetting.json 添加如下配置
"jwt": {
"Secret": "your-256-bit-secret-siyao", //私钥
"Iss": "https://localhost:44355", //签发
"Aud": "api" //受众端
},
public Startup(IConfiguration configuration)
{
_configuration = configuration;
}
public IConfiguration _configuration { get; set; }
public void ConfigureServices(IServiceCollection services)
{
var jwtConfig = _configuration.GetSection("jwt");
string scree = jwtConfig.GetValue<string>("Secret"); //读取配置
services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options =>
{
options.RequireHttpsMetadata = false;
options.SaveToken = true;
options.TokenValidationParameters = new TokenValidationParameters
{
ValidateIssuer = true, //是否验证Issuer
ValidateAudience = true, //是否验证Audience
ValidateLifetime = true, //是否验证失效时间
ClockSkew = TimeSpan.FromSeconds(30),
ValidateIssuerSigningKey = true, //是否验证SecurityKey
ValidAudience = "this", //Audience
ValidIssuer = "this", //Issuer,这两项和前面签发jwt的设置一致
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(scree)) //拿到SecurityKey
};
});
services.AddControllers();
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
///添加jwt验证
/// app.UseAuthorization
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseAuthentication();
app.UseRouting();
app.UseAuthorization();
app.UseEndpoints(p =>
{
p.MapControllers();
});
}
添加两个控制器
public class LoginController: ControllerBase
{
private IConfiguration _config;
public LoginController(IConfiguration config)
{
_config = config;
}
[AllowAnonymous]
[HttpGet]
[Route("/login")]
public IActionResult login(string name, string pwd)
{
var jwtConfig= _config.GetSection("jwt");
if (!string.IsNullOrEmpty(name) && !string.IsNullOrEmpty(pwd))
{
var claims = new[]
{
new Claim(JwtRegisteredClaimNames.Nbf,$"{new DateTimeOffset(DateTime.Now).ToUnixTimeSeconds()}") ,
new Claim (JwtRegisteredClaimNames.Exp,$"{new DateTimeOffset(DateTime.Now.AddMinutes(30)).ToUnixTimeSeconds()}"),
new Claim(ClaimTypes.Name, name)
};
var key = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(jwtConfig.GetValue<string>("Secret")));
var creds = new SigningCredentials(key, SecurityAlgorithms.HmacSha256);
var token = new JwtSecurityToken(
issuer: "this",
audience: "this",
claims: claims,
expires: DateTime.Now.AddMinutes(30),
signingCredentials: creds);
return Ok(new
{
token = new JwtSecurityTokenHandler().WriteToken(token)
});
}
else
{
return BadRequest(new { message = "username or password is incorrect." });
}
}
[HttpGet]
[Route("/getName")]
[Authorize]
public string GetName()
{
return "访问我需要admin权限";
}
[HttpGet]
[Route("/Homs")]
public string Homs()
{
return ".,..";
}
}
[Route("/api")]
public class ValueController: ControllerBase
{
static UseDao _useDao=new UseDao();
[HttpGet]
[Authorize]
[Route("/getUser")]
public User GetUser()
{
var auth = HttpContext.AuthenticateAsync();
string admin= auth.Result.Principal.Claims
.First(a => a.Type.Equals(ClaimTypes.Name))?.Value; //获取当前用户
return _useDao.GetUser(admin);
}
}
```
public class User
{
public int id { get; set; }
public string Name { get; set; }
public String admin
{
get;
set;
}
}
public class UseDao
{
static List<User> _list=new List<User>()
{
new User(){id=1,admin = "list",Name = "李四"},
new User(){id=2,admin = "zhangsan",Name = "法外狂徒张三"}
};
public User GetUser(String admin)
{
User use = _list.Find(a => a.admin == admin);
return use;
}
}
```
打开postman
- 获得token
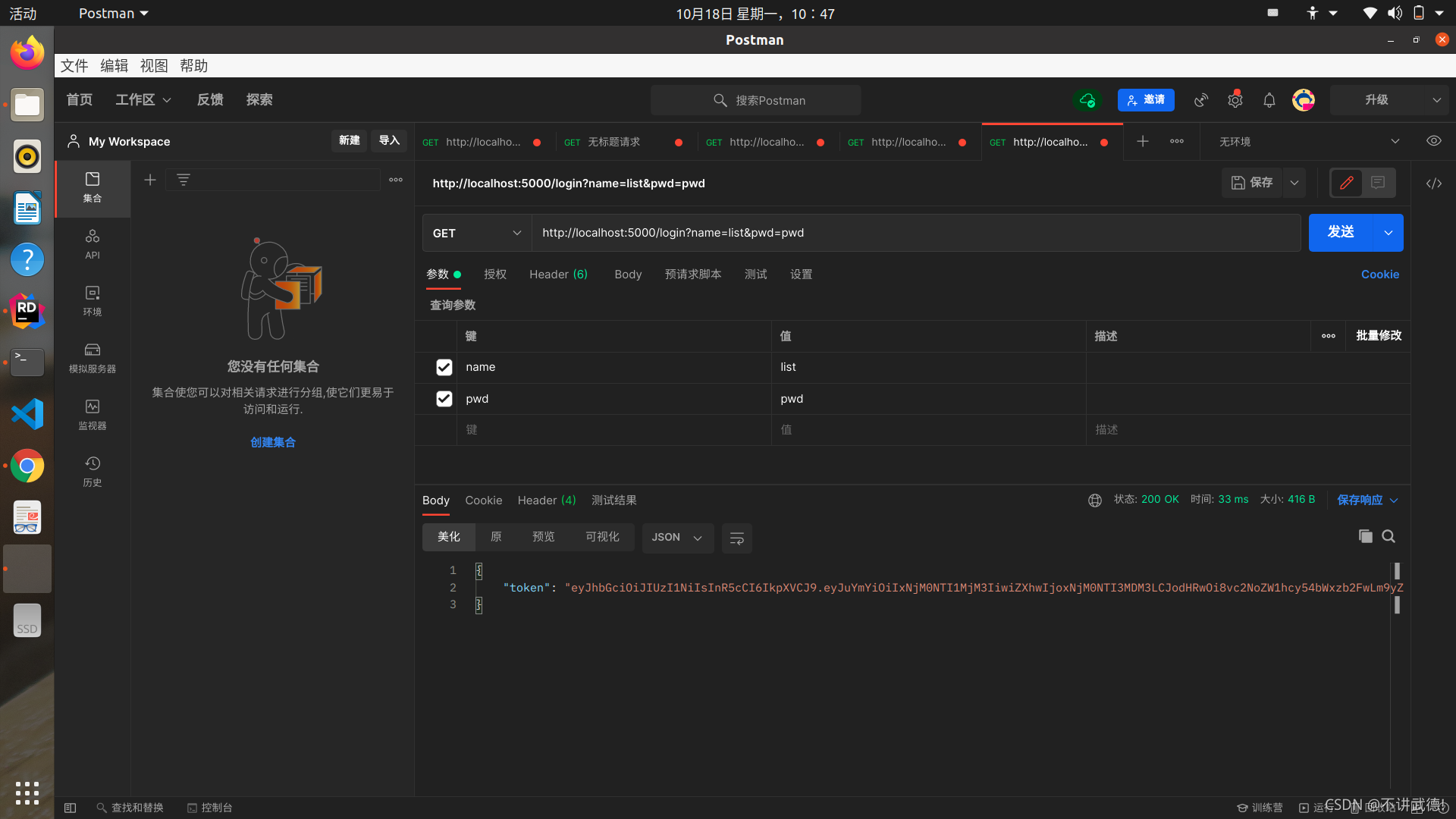
- 在授权中添加token 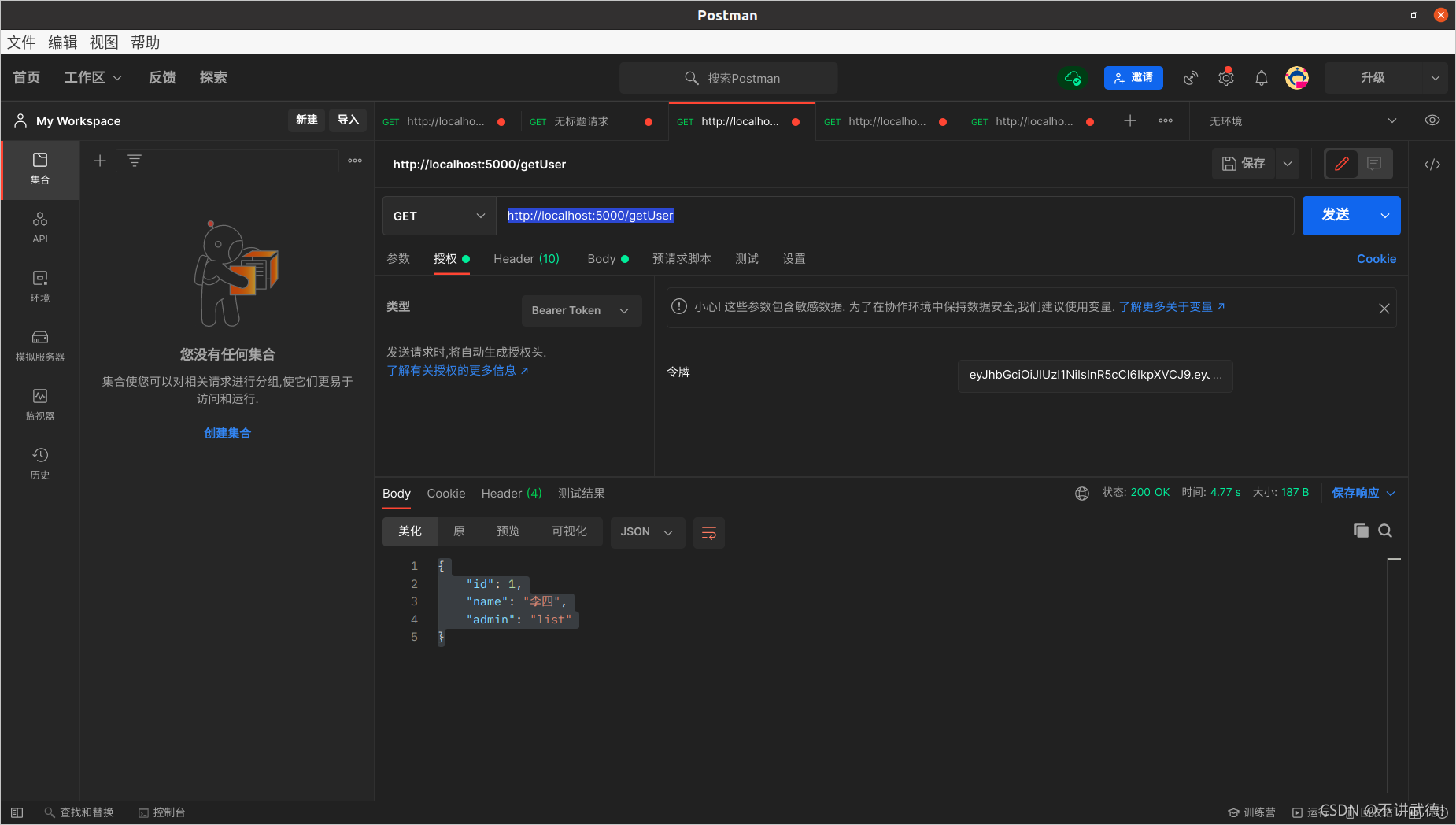