#include<iostream>
#include<string>
#include<windows.h>
#include<fstream>
#include<conio.h>
#include<cstring>
using namespace std;
int checkcin(int n)
{
if(cin.fail())
{
cin.clear();cin.sync();
}
cout<<"输入错误,请重新输入"<<endl;
cin>>n;
return n;
}
class W{
public:
int showmenu()
{
cout<<endl;
system("date/t");system("time/t");
cout<<endl;
cout<<endl<<"\t\t\t\t\t欢迎使用职工管理系统"<<endl<<endl<<endl;
cout<<"\t\t\t\t\t1.添加职工信息"<<endl<<endl;
cout<<"\t\t\t\t\t2.浏览职工信息"<<endl<<endl;
cout<<"\t\t\t\t\t3.删除职工信息"<<endl<<endl;
cout<<"\t\t\t\t\t4.修改职工信息"<<endl<<endl;
cout<<"\t\t\t\t\t5.查找职工信息"<<endl<<endl;
cout<<"\t\t\t\t\t6.按照职工号(从小到大)进行排序"<<endl<<endl;
cout<<"\t\t\t\t\t7.按照职工工资(从大到小)进行排序"<<endl<<endl;
cout<<"\t\t\t\t\t8.职工号排序后按照职工号进行插入"<<endl<<endl;
cout<<"\t\t\t\t\t9.对员工信息进行保存"<<endl<<endl;
cout<<"\t\t\t\t\t0.退出程序"<<endl<<endl;
}
int showmenu1()
{
system("color 07");
cout<<endl;
system("date/t");system("time/t");
cout<<endl;
cout<<endl<<"\t\t\t\t\t欢迎使用职工管理系统"<<endl<<endl<<endl;
cout<<"\t\t\t\t\t1.浏览职工信息"<<endl<<endl;
cout<<"\t\t\t\t\t2.查找职工信息"<<endl<<endl;
cout<<"\t\t\t\t\t3.按照职工号(从小到大)进行排序"<<endl<<endl;
cout<<"\t\t\t\t\t4.按照职工工资(从大到小)进行排序"<<endl<<endl;
cout<<"\t\t\t\t\t5.返回选择登录方式页面"<<endl<<endl;
cout<<"\t\t\t\t\t0.退出程序"<<endl<<endl;
}
int exit1()
{
cout<<"欢迎下次使用"<<endl;
system("pause");
exit(0);
}
};
class worker{
public:
int setnumber(int number_)
{
this->number=number_;}
int setname(string name_)
{
this->name=name_;}
int setsex(string sex_)
{
this->sex=sex_;}
int setbirthday(string birthday_)
{
this->birthday=birthday_;}
int setxl(string xl_)
{
this->xl=xl_;}
int setposition(string position_)
{
this->position=position_;}
int setmoney(int money_)
{
this->money=money_;}
int setadd(string add_)
{
this->add=add_;}
int settele(string tele_)
{
this->tele=tele_;}
int getnumber()
{
return this->number;}
string getname()
{
return this->name;}
string getsex()
{
return this->sex;}
string getbirthday()
{
return this->birthday;}
string getxl()
{
return this->xl;}
string getposition()
{
return this->position;}
int getmoney()
{
return this->money;}
string getadd()
{
return this->add;}
string gettele()
{
return this->tele;}
worker* setnext(worker *p)
{
this->next=p;}
worker* getnext()
{
return this->next;}
private:
int number;
string name;
string sex;
string birthday;
string xl;
string position;
int money;
string add;
string tele;
worker *next;
};
class functionwork:public worker{
public:
functionwork()
{
head=new worker();//建立头节点并将其初始化
head->setnext(NULL);}
int add();//添加职工信息
int liulan();//浏览职工信息
int del();//删除职工信息
int xiugai();//修改职工信息
int chaxun();//查询职工信息
int keep();//保存职工信息
int read(); //读取职工信息
int numpaixu();//按照职工号进行排序
int monpaixu();//按照工资进行排序
int charu();//插入职工信息
private:
worker *p1,*p2,*head;//建立头指针和备用指针
};
int functionwork::add()
{
int number1;
string name1;
string sex1;
string birthday1;
string xl1;
string position1;
int money1;
string add1;
string tele1;
p2=head;
worker *p3;
while(p2->getnext())
{
p2=p2->getnext();}
int n;
cout<<"请输入你想添加的职工数:";
cin>>n;
while(cin.fail())
n=checkcin(n);
for(int i=0;i<n;i++)
{
int cntt=0;
p1=new worker();
p1->setnext(NULL);
cout<<"请输入第"<<i+1<<"个职工信息"<<endl;
while(1)
{
cout<<"请输入职工号:";
cin>>number1;
while(cin.fail())
number1=checkcin(number1);
p3=head->getnext();
while(p3!=NULL)
{
if(p3->getnumber()==number1)
{
cout<<"职工号重复,请重新输入!";
break;}
p3=p3->getnext();
}
if(p3==NULL)
{
p1->setnumber(number1);
break;}
else
continue;
}
cout<<"请输入姓名:";
cin>>name1;
p1->setname(name1);
while(1)
{
if(cntt>0)
cout<<"你输入性别有误!请重新输入";
cout<<"请输入性别:";
cin>>sex1;
if(sex1=="男")
break;
else if(sex1=="女")
break;
cntt++;
}
p1->setsex(sex1);
cout<<"请输入出生年月:";
cin>>birthday1;
p1->setbirthday(birthday1);
cout<<"请输入学历:";
cin>>xl1;
p1->setxl(xl1);
cout<<"请输入职务:";
cin>>position1;
p1->setposition(position1);
while(1)
{
cout<<"请输入工资:";
cin>>money1;
if(cin.fail())
{
while(cin.fail())
money1=checkcin(money1) ;}
else
break;
}
p1->setmoney(money1);
cout<<"请输入地址:";
cin>>add1;
p1->setadd(add1);
cout<<"请输入电话:";
cin>>tele1;
p1->settele(tele1);
system("cls");
p2->setnext(p1);
p2=p1;
}
cout<<"添加完毕!谢谢使用";
system("pause");
}
int functionwork::liulan()
{
if(head->getnext()==NULL)
{
cout<<"您尚未添加任何职工信息!"<<endl;
system("pause");
return 0;
}
cout<<"职工号\t"<<"姓名\t"<<"性别\t"<<"出生年月 "<<"学历\t"<<"职务\t"<<"工资\t"<<"地址\t"<<"职工电话\t"<<endl;
p1=head->getnext();
while(p1!=NULL)
{
cout<<p1->getnumber()<<"\t"<<p1->getname()<<"\t"<<p1->getsex()<<"\t"<<p1->getbirthday()<<" \t"<<p1->getxl()<<"\t"<<p1->getposition()<<"\t"<<p1->getmoney()<<"\t"<<p1->getadd()<<"\t"<<p1->gettele()<<endl;
p1=p1->getnext();
}
system("pause");
return 0;
}
int functionwork::del()
{
int a;
int h=0;
cout<<"请输入你想删除的职工号:" ;
cin>>a;
if(cin.fail())
{
while(cin.fail())
a=checkcin(a) ;}
worker *p3;
p3=head->getnext();
while(p3!=NULL)
{
if(p3->getnumber()==a)
{
break;
}
p3=p3->getnext();
}
if(p3==NULL)
{
cout<<"没有此职工!"<<endl;
system("pause");
system("cls");
return 0;
}
else
{
cout<<"你想要删除职工号信息如下:"<<endl;
cout<<"姓名:"<<p3->getname()<<endl<<"性别:"<<p3->getsex()<<endl<<"出生年月:"<<p3->getbirthday()<<endl<<"学历:"<<p3->getxl()<<endl<<"职务:"<<p3->getposition()<<endl<<"工资:"<<p3->getmoney()<<endl<<"地址:"<<p3->getadd()<<endl<<"电话:"<<p3->gettele()<<endl<<endl;
string arr;
while(1)
{
cout<<"是否确认删除(是或否):";
cin>>arr;
if(arr=="是")
break;
else if(arr=="否")
{
cout<<"谢谢使用!"<<endl;
system("pause");
return 0;
}
else
{
cout<<"请输入是或否,其他无效!";
system("pause");
continue;
}
}
fstream fp;
fp.open("删除职工统计",ios::app);
fp<<"姓名:"<<p3->getname()<<endl<<"性别&#
C++职工信息管理系统
最新推荐文章于 2024-08-03 22:21:32 发布
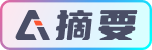