命名管道的特点
- 可以在不同的进程之间通信,而匿名管道只能在有亲缘关系的进程间通信;
- 命名管道创建时相当于在系统上生成了一个管道文件,并不会随系统的退出自动删除,在不再使用时需要手动删除文件。
创建命名管道
create.c
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/stat.h>
#define FIFO_NAME "./fifo"
int main(){
if(mkfifo(FIFO_NAME, 0666) < 0){
perror("mkfifo()");
exit(1);
}
puts("create OK!");
exit(0);
}
运行结果
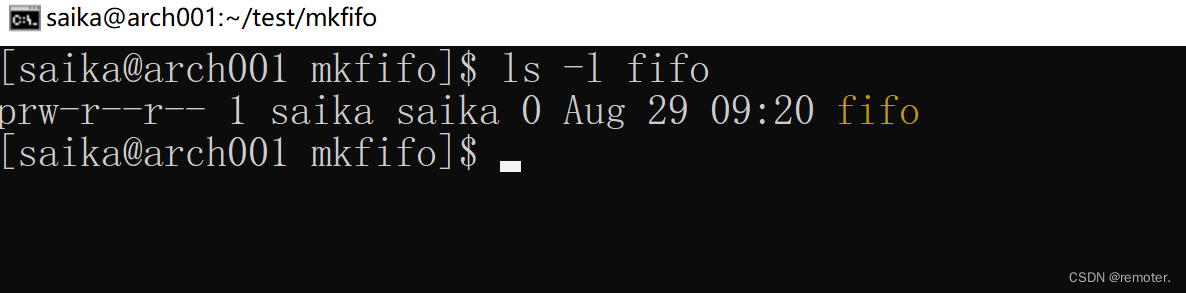
写端 / 发送端
write.c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <unistd.h>
#define FIFO_NAME "./fifo"
#define MSG_MAX 2048
int main(){
int fd = open(FIFO_NAME, O_WRONLY);
if(fd < 0){
perror("open()");
exit(1);
}
char msg[MSG_MAX];
int i = 0;
for(i = 0; i < 1024; i++){
sprintf(msg, "msg : %d\n", i);
size_t msg_len = strlen(msg);
write(fd, msg, msg_len);
puts("write OK!");
sleep(1);
}
exit(0);
}
运行结果
只打开写端
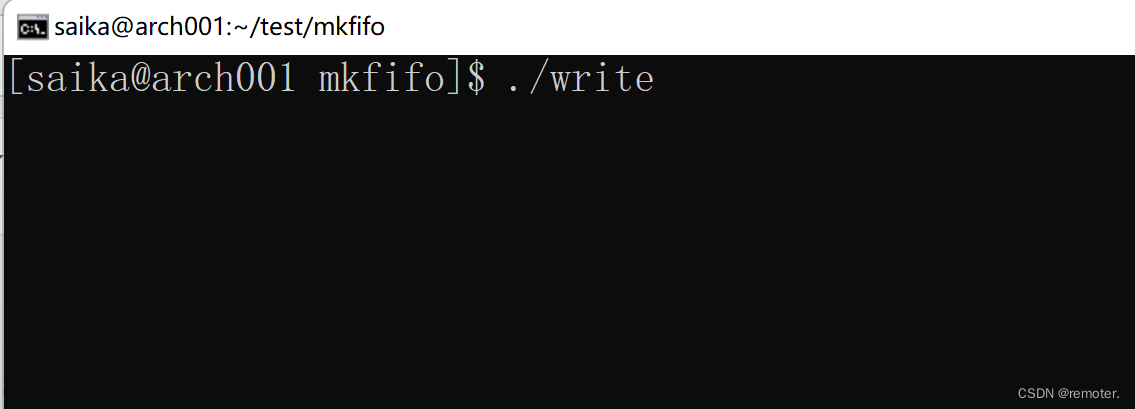
- 由于之打开了写端,管道内的数据没有程序取出,程序阻塞在 write();
同时运行读端和写端
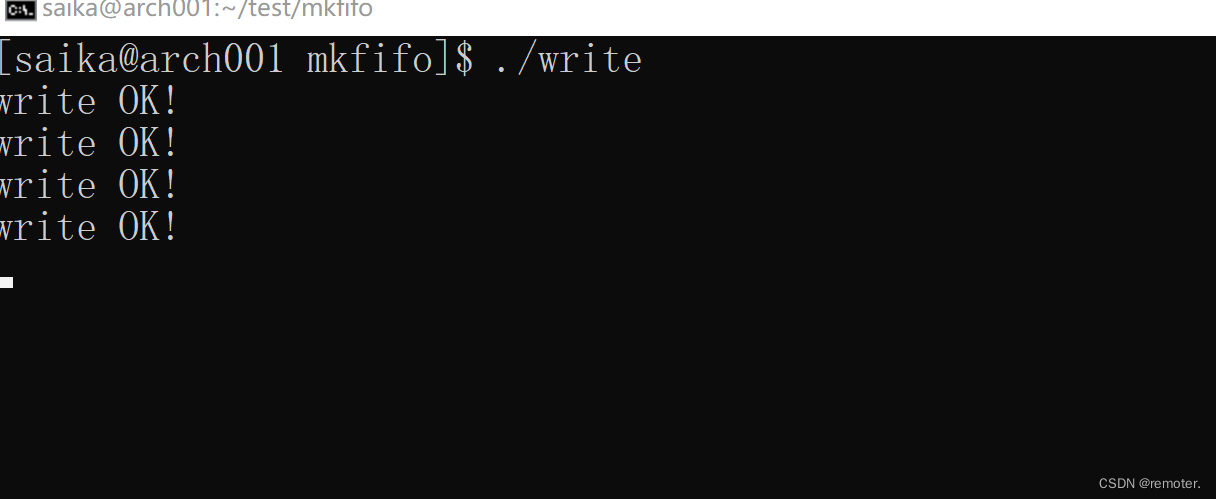
读端 / 接收端
read.c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/epoll.h>
#define FIFO_NAME "./fifo"
#define MSG_MAX 1024
int main(){
int fd = open(FIFO_NAME, O_RDONLY);
if(fd < 0){
perror("open()");
exit(1);
}
int epfd = epoll_create(1);
struct epoll_event ev;
memset(&ev, 0, sizeof(ev));
ev.events = EPOLLIN | EPOLLET;
ev.data.fd = fd;
if(epoll_ctl(epfd, EPOLL_CTL_ADD, fd, &ev) < 0){
perror("epoll_ctl()");
exit(1);
}
while(1){
struct epoll_event events[8];
int nready = epoll_wait(epfd, events, 8, -1);
if(nready < 0){
perror("epoll_wait()");
exit(1);
}
int i = 0;
for(i = 0; i < nready; i++){
if(events[i].events & EPOLLIN){
char msg[MSG_MAX];
read(fd, msg, MSG_MAX);
printf("read msg : %s\n", msg);
}
}
}
exit(0);
}
运行结果
只打开读端
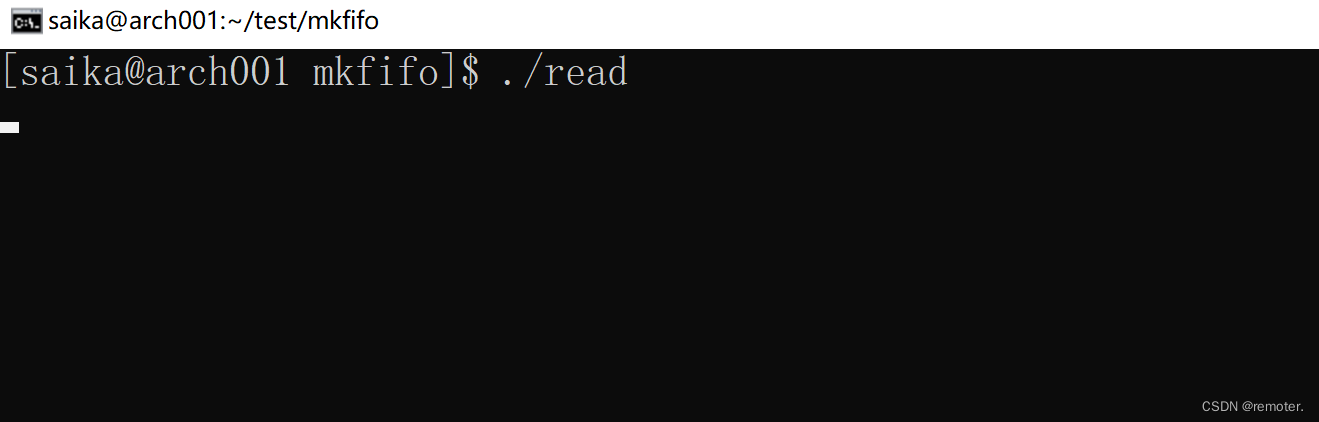
- 只打开读端,由于没有写事件的发生,程序阻塞在 epoll_wait(),并等待发送端程序运行
同时运行读端和写端
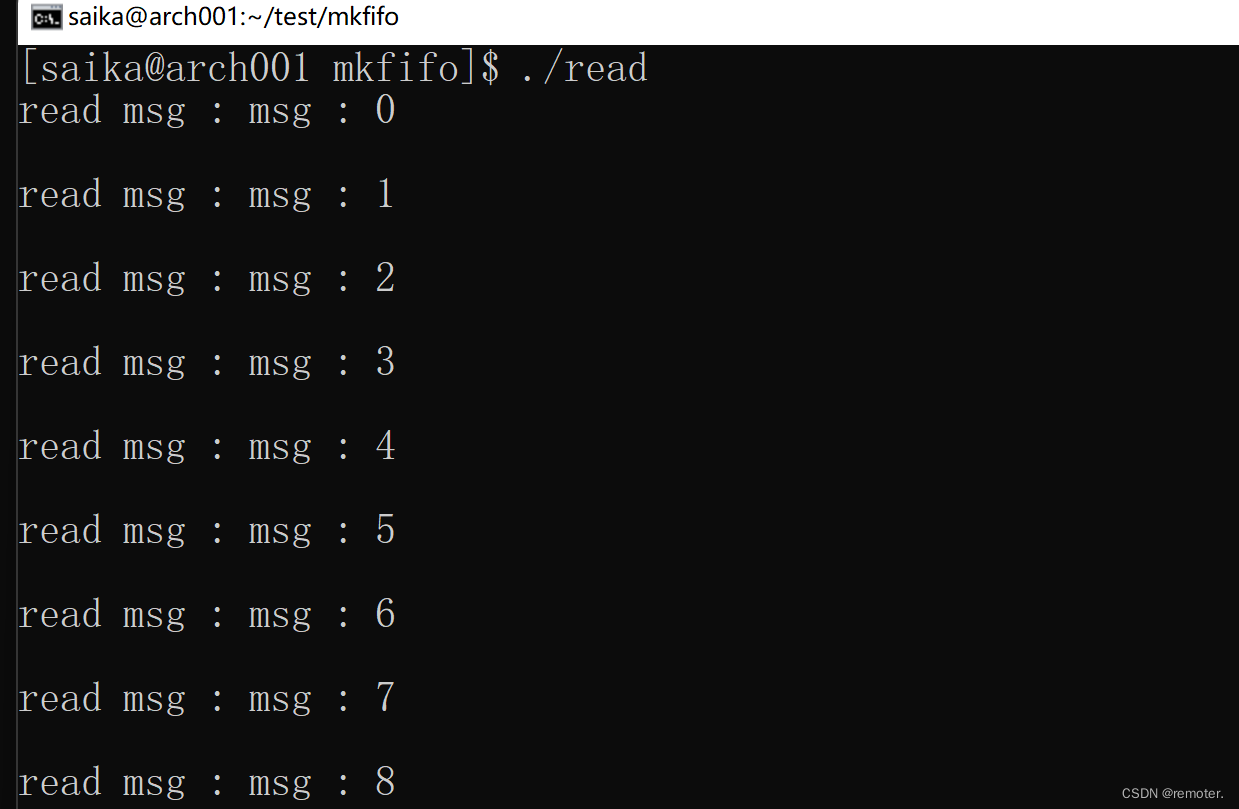