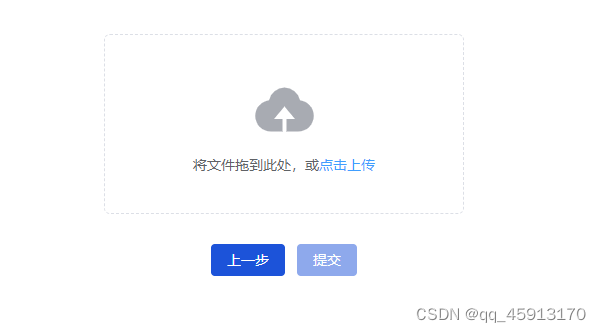
<el-upload
ref="uploadDom"
action=""
v-model:file-list="fileList"
:auto-upload="false"
multiple
drag
:on-preview="openFile"
:on-change="handleChange"
class="uploadElement"
>
<el-icon class="el-icon--upload"><upload-filled /></el-icon>
<div class="el-upload__text">将文件拖到此处,或<em>点击上传</em></div>
</el-upload>
import type { UploadProps, UploadRawFile } from "element-plus";
let fileList = ref([]);
const openFile = (e) => {
let a = document.createElement("a");
let event = new MouseEvent("click");
a.target = "_blank";
a.download = e.name;
a.href = e.url;
a.dispatchEvent(event);
};
const handleChange = (file:any, files:any) => {
console.log('file',file)
console.log('files',files)
fileList.value=files
};
let uploadDom = ref();
const handleExceed: UploadProps["onExceed"] = (files, uploadFiles) => {
uploadDom.value!.clearFiles();
const file = files[0] as UploadRawFile;
file.uid = genFileId();
uploadDom.value!.handleStart(file);
fileList.value = uploadFiles;
};
let classicCaseAttachmentFile = ref();
const handleChange = (file, files) => {
if (files) {
classicCaseAttachmentFile.value = files;
}
};
const handleSubmit = async () => {
// 创建一个FormData实例,用于存储要发送的文件数据
let formData = new FormData();
// 添加文件到FormData实例中
formData.append("id", route.query.id as string);
fileList.value.forEach((item, index) => {
formData.append(`checkAttachmentFile[${index}]`, item.raw);
});
let res: any = await updateCaseInfoAndStartProcess(formData);
if (res.code == 0) {
}
};
点击按钮下载
const handleDownload = async () => {
let res: any = await downloadClassicCase();
// 创建一个Blob对象URL
const url = window.URL.createObjectURL(new Blob([res]));
// 创建一个新的<a>标签
const link = document.createElement("a");
link.style.display = "none";
link.href = url;
// 设置下载的文件名(如果服务器没有提供)
link.setAttribute("download", "aaa.doc");
// 触发点击事件来下载文件
document.body.appendChild(link);
link.click();
// 清理(可选)
// 在下载完成后,你可以从DOM中移除这个<a>标签,并释放Blob URL
link.remove();
};
export const caseInfoUpdate = (data?: any) => {
return service({
url: "/bb/update",
method: "post",
data,
headers: { "Content-Type": "multipart/form-data" },//告知axios发送multipart/form-data类型的数据
});
};
export const downloadClassicCase = () => {
return service({
url: "/cc/Case",
method: "get",
responseType: "blob",
});
};
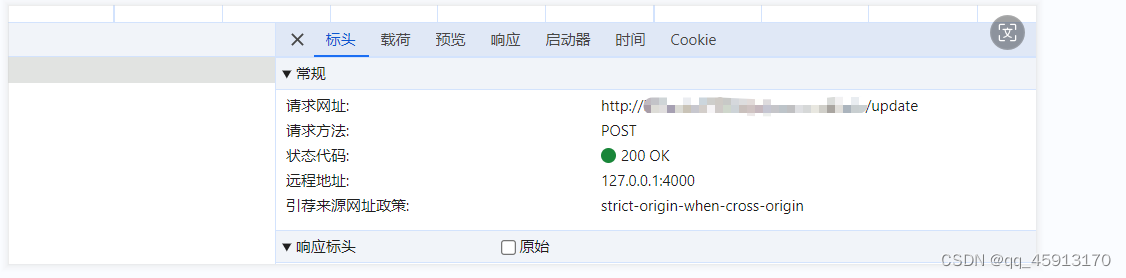
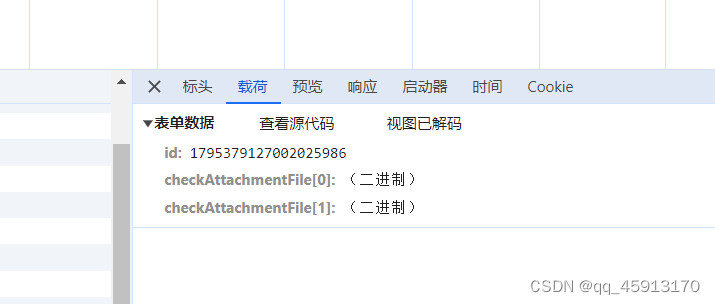
上传一个
<el-upload
ref="uploadDom"
action=""
v-model:file-list="fileList"
:auto-upload="false"
:limit="1"
drag
:on-exceed="handleExceed"
:on-change="handleChange"
:on-preview=" openFile"
class="uploadElement"
>
<el-icon class="el-icon--upload"><upload-filled /></el-icon>
<div class="el-upload__text">将文件拖到此处,或<em>点击上传</em></div>
</el-upload>
let classicCaseAttachmentFile = ref();
let fileList = ref([]);
const openFile = (e) => {
let a = document.createElement("a");
let event = new MouseEvent("click");
a.target = "_blank";
a.download = e.name;
a.href = e.url;
a.dispatchEvent(event);
};
let uploadDom = ref();
const handleExceed: UploadProps["onExceed"] = (files, uploadFiles) => {
uploadDom.value!.clearFiles();
const file = files[0] as UploadRawFile;
file.uid = genFileId();
uploadDom.value!.handleStart(file);
fileList.value = uploadFiles;
};
const handleChange = (file, files) => {
if (files) {
classicCaseAttachmentFile.value = files;
}
};
const handleDownload = async () => {
let res: any = await downloadClassicCase();
const url = window.URL.createObjectURL(new Blob([res]));
const link = document.createElement("a");
link.style.display = "none";
link.href = url;
link.setAttribute("download", "哈哈哈.doc");
document.body.appendChild(link);
link.click();
link.remove();
};
const handleSubmit = async () => {
// 新增/编辑
let formData = new FormData();
formData.append("id", route.query.id as string);
formData.append(
"classicCaseAttachmentFile",
classicCaseAttachmentFile.value[0].raw
);
let res: any = await caseInfoUpdate(formData);
if (res.code == 0) {
}
}
};