-
服务器端
//服务端接收文件 public class TcpServerDemo2 { public static void main(String[] args) throws Exception { //1.创建服务 ServerSocket serverSocket = new ServerSocket(9000); //2.监听客户端的连接 Socket socket = serverSocket.accept();//阻塞式监听,会一直等待客户端连接 //3.获取输入流 InputStream is = socket.getInputStream(); //4.文件输出 FileOutputStream fos = new FileOutputStream(new File("receive.png")); byte[] buffer = new byte[1024]; int len; while((len=is.read(buffer))!=-1){ fos.write(buffer,0,len); } //通知客户端我接收完毕了 OutputStream os = socket.getOutputStream(); os.write("我接收完毕了,你可以断开了".getBytes()); //5.关闭资源 fos.close(); is.close(); socket.close(); serverSocket.close(); } }
-
客户端
//客户端读取文件 public class TcpClientDemo2 { public static void main(String[] args) throws Exception { //1,创建一个Socket连接 Socket socket = new Socket(InetAddress.getByName("127.0.0.1"), 9000); //2.创建一个输出流 OutputStream os = socket.getOutputStream(); //3.文件流 FileInputStream fis = new FileInputStream(new File("javaio.png")); //4.写出文件 byte[] buffer = new byte[1024]; int len; while((len=fis.read(buffer))!=-1){ os.write(buffer, 0,len); } //通知服务器,我已经结束了 socket.shutdownOutput();//我已经传输完了 //确定服务器接收完毕,才能够断开连接 InputStream inputStream = socket.getInputStream(); //String byte[] ByteArrayOutputStream baos = new ByteArrayOutputStream(); byte[] buffer2 = new byte[1024]; int len2; while((len2=inputStream.read(buffer2))!=-1){ baos.write(buffer2, 0,len2); } System.out.println(baos.toString()); //5.关闭资源 fis.close(); os.close(); socket.close(); inputStream.close(); baos.close(); } }
javaTCP实现文件上传
最新推荐文章于 2022-10-23 15:38:49 发布
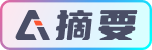