上篇总结
ionic基础组件的使用:
ionic 就是基于 Angular框架, 制作的一套组件库.
这套组件库中的组件 就是 HTML 添加了一些样式, 变成手机端组件的样子.
- 容器:
– ion-app: 根容器
– ion-header: 头部栏
– ion-content: 身体部分, 主体
– ion-footer: 脚部栏
- ion-button: 按钮
- ion-badge: 徽章
- ion-card: 卡片
- ion-slides: 横向滚动栏
- ion-icon: 系统图标
- ion-fab: 悬浮按钮
- ion-gird: 宫格布局, 做多列布局
- ion-infinite-scroll: 加载更多
- ion-refresher: 下拉刷新
-
ion-item: 栏目
路由系统_______________
Angular自带一套路由系统, 通过创建项目时, 可以直接生成默认模板:
ionic start 包名 tabs
这里的 tabs 就代表 标签栏导航模板
设置 标签栏的 标题和图标
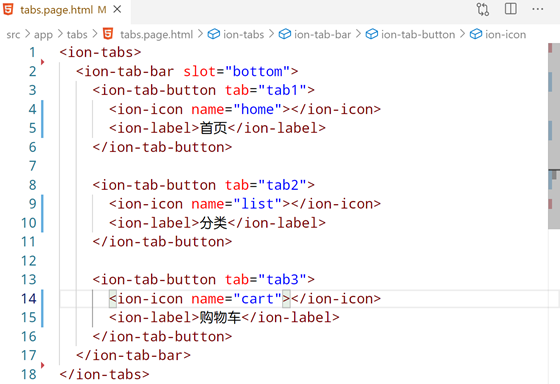
添加新的页面
新建页面命令
ng generate page 页面名
简写: ng g page 页面名
例如: ng g page tab4
相较于 组件, 页面会多出 相应的配置文件.
页面会自动更新注册到 全局路由中
由于 此页面需要出现在 tabs 中, 所以需要手动到 主路由文件中: app-routing.module.ts 删除 自动生成的注册操作
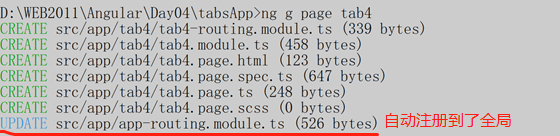
到 tabs-routing.module.ts 文件中,添加 tab4 页面的注入操作
到 tabs.page.html 文件中,添加新页面的标签按钮
栈式导航_____________________________
生成页面 ng g page detail
在tab1进行
基础跳转:
<ion-content>
<!-- vue 中 <router-link to='路径'> 实现跳转-->
<ion-item button detail routerLink='/detail'>
<ion-label>详情页</ion-label>
</ion-item>
<!-- 参数传递 -->
<!-- [routerLink]="[路径,参数]" -->
<ion-item button detaill [routerLink]="['/detail',{name:'天天',age:22}]">
<ion-label>天天</ion-label>
</ion-item>
<ion-item button detail [routerLink]="['/detail',{name:'弟弟',age:44}]">
<ion-label>弟弟</ion-label>
</ion-item>
编程跳转:
html
<ion-button (click)="goDetail()">
跳转到详情页
</ion-button>
ts:
import { NavController } from "@ionic/angular";
import { Component } from '@angular/core';
@Component({
selector: 'app-tab1',
templateUrl: 'tab1.page.html',
styleUrls: ['tab1.page.scss']
})
export class Tab1Page {
//路由导航服务:NavController;负责编程式跳转
constructor(public router: NavController) { }
goDetail() {
// this.router.navigateForward('/detail');
//带参数的跳转
this.router.navigateForward(['/detail', { name: '球球', age: 25 }])
}
}
在detail进行
参数的获取:
import { ActivatedRoute } from "@angular/router";
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-detail',
templateUrl: './detail.page.html',
styleUrls: ['./detail.page.scss'],
})
export class DetailPage implements OnInit {
//依赖注入方式,引入服务ActivateRoute
//此服务用于管理参数
constructor(public route: ActivatedRoute) { }
ngOnInit() {
console.log(this.route)
const { name, age } = this.route.snapshot.params;
console.log(name, age)
}
}
弹出提示框组件_____________
在tab2进行操作
html:
<ion-header [translucent]="true">
<ion-toolbar>
<ion-title>
分类
</ion-title>
</ion-toolbar>
</ion-header>
<ion-content [fullscreen]="true">
<!-- 需要点击事件触发弹出 -->
<ion-button (click)="alert()">弹出提示框</ion-button>
</ion-content>
ts:
import { AlertController } from "@ionic/angular";
import { Component } from '@angular/core';
@Component({
selector: 'app-tab2',
templateUrl: 'tab2.page.html',
styleUrls: ['tab2.page.scss']
})
export class Tab2Page {
//弹窗服务 AlertController
constructor(public alertC: AlertController) { }
alert() {
//create (配置项) :根据配置项创建弹窗
//then() 弹窗创建成功后 回调函数
this.alertC.create({
header: '我是标题',
subHeader: '我是子标题',
message: '内容部分',
buttons: [
{ text: '确定', handler: () => console.log('确定') },
{ text: '取消', handler: () => console.log('取消') },
],
}).then(res => res.present());
//res是创建的提示框 present()是弹出的意思
}
}
搜索组件___________
tab3中进行
html:
<!-- 搜索组件 -->
<ion-header>
<ion-toolbar>
<ion-searchbar placeholder='输入关键词' (ionChange)="onSearchChange($event)" [debounce]="250">
<!-- debounce自带节流防抖输入完0.25s后再执行操作 -->
</ion-searchbar>
</ion-toolbar>
</ion-header>
<ion-content>
<ion-content>
<!-- ion-list:专门显示列表内容的组件 配合item组件使用 -->
<ion-list>
<ion-item *ngFor="let item of result; let i=index">
<div style="display: flex;flex-direction: column;">
<h4>{{item.title}}</h4>
<div style="color:gray"> {{item.des}}</div>
</div>
</ion-item>
</ion-list>
</ion-content>
</ion-content>
ts:
import { HttpClient } from '@angular/common/http';
import { Component } from '@angular/core';
@Component({
selector: 'app-tab3',
templateUrl: 'tab3.page.html',
styleUrls: ['tab3.page.scss'],
})
export class Tab3Page {
constructor(public http: HttpClient) { }
result = [];
onSearchChange(e) {
console.log(e.detail.value);
const url = `/juhe/dream/query?q=${e.detail.value}&cid=&full=&key=0be022b622a8379bd7113b5aa54fbd19`;
this.http.get(url).subscribe((res: any) => {
console.log(res);
this.result = res.result;
});
}
}
这里发送了跨域请求 注意引入 网络模块 注意配置跨域