6.1 网络API
6.1.1 发起网络请求
6.1.1.1
属性
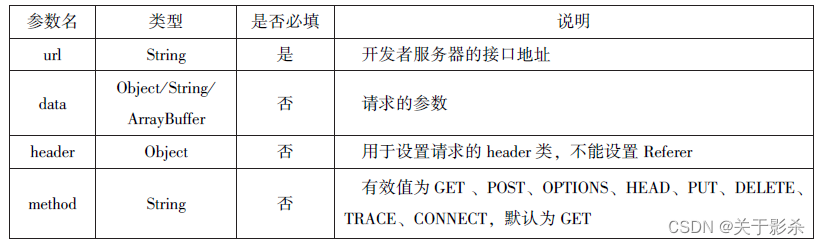
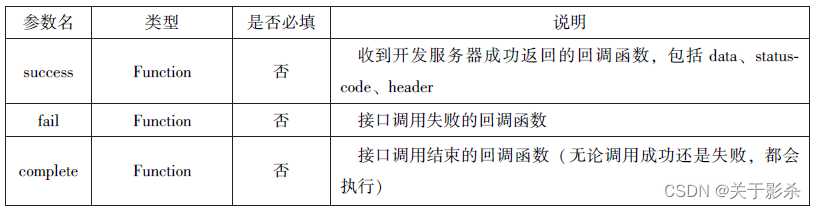
wxml代码
<button type="primary"bindtap="getbaidutap">获取HTML数据</button>
<textarea value='{{html}}'auto-height maxlength='0'></textarea>
js代码
getbaidutap:function(){
var that=this;
wx.request({
url:'https:www.baidu.com',
data:{},
header:{'Content-Type':'application/json'},
success:function(res){
console.log(res);
that.setData({
html:res.data
})
}
})
},
运行效果图
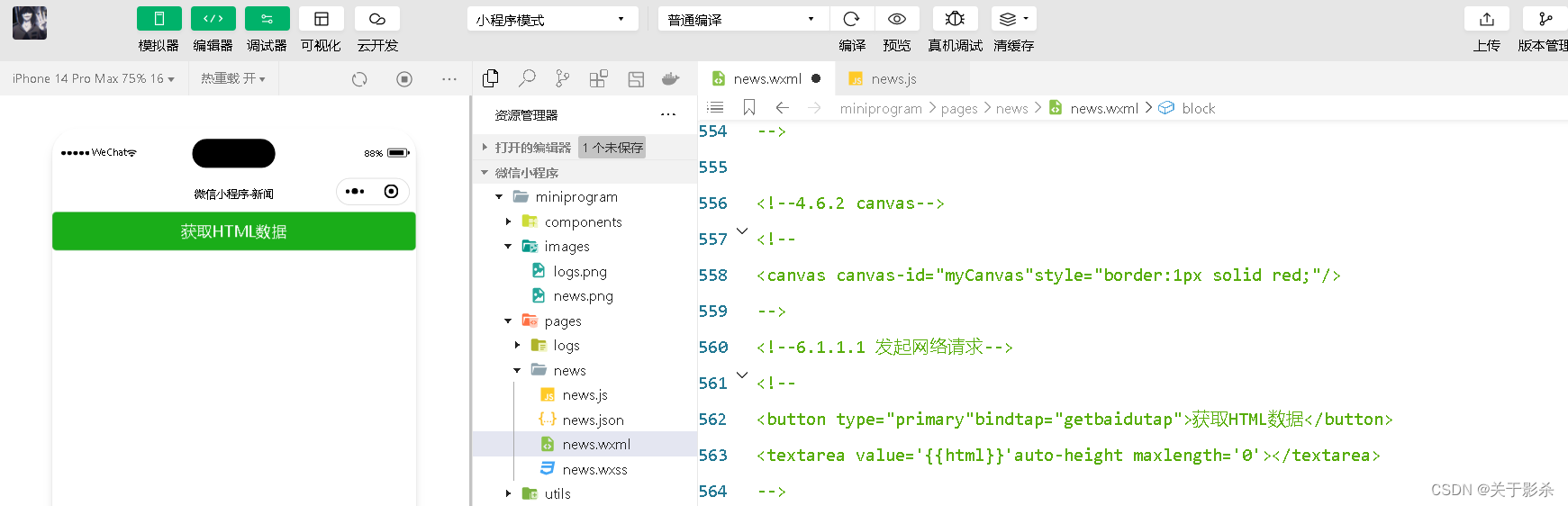
6.1.1.2
wxml代码
<view>邮政编码:</view>
<input type="text"bindinput="input"placeholder='6位邮政编码'/>
<button type="primary"bindtap="find">查询</button>
<block wx:for="{{address}}">
<block wx:for="{{item}}">
<text>{{item}}</text>
</block>
</block>
js代码
postcode:"",
address:[],
errMsg:"",
error_code:-1,
input:function(e){
this.setData({
postcode:e.detail.value,
})
console.log(e.detail.value)
},
find:function(){
var postcode=this.data.postcode;
if(postcode!=null&&postcode!=""){
var self=this;
wx.showToast({
title: '正在查询,请稍后....',
icon:'loading',
duration:10000
});
wx.request({
url:'https://v.juhe.cn/postcode/query',
data:{
'postcode':postcode,
'key':'0ff9bfccdf147476e067de994eb5496e'
},
header:{
'Content-Type':'application/json',
},
method:'GET',
success:function(res){
wx.hideToast();
if(res.data.error_code==0){
console.log(res);
self.setData({
errMsg:"",
error_code:res.data.error_code,
address:res.data.result.list
})
}
else{
self.setData({
errMsg:res.data.reason||res.data.reason,
error_code:res.data.error_code
})
}
}
})
}
},
运行结果图
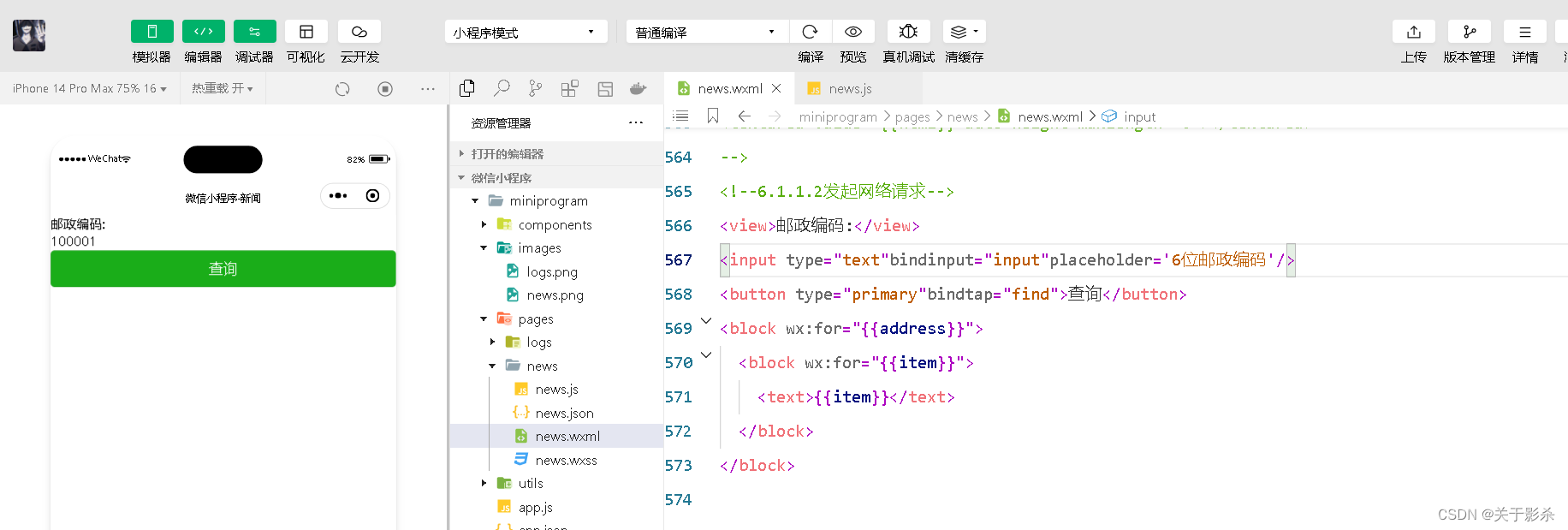
6.1.1.3
wxml代码
<view>邮政编码:</view>
<input type="text"bindinput="input"placeholder='6位邮政编码'/>
<button type="primary"bindtap="find">查询</button>
<block wx:for="{{address}}">
<block wx:for="{{item}}">
<text>{{item}}</text>
</block>
</block>
js代码
input:function(e){
this.setData({
postcode:e.detail.value,
})
console.log(e.detail.value)
},
find:function(){
var postcode=this.data.postcode;
if(postcode!=null&&postcode!=""){
var self=this;
wx.showToast({
title: '正在查询,请稍后....',
icon:'loading',
duration:10000
});
wx.request({
url:'https://v.juhe.cn/postcode/query',
data:{
'postcode':postcode,
'key':'0ff9bfccdf147476e067de994eb5496e'
},
header:{
'Content-Type':'application/json',
},
method:'GET',
success:function(res){
wx.hideToast();
if(res.data.error_code==0){
console.log(res);
self.setData({
errMsg:"",
error_code:res.data.error_code,
address:res.data.result.list
})
}
else{
self.setData({
errMsg:res.data.reason||res.data.reason,
error_code:res.data.error_code
})
}
}
})
}
},
运行结果图
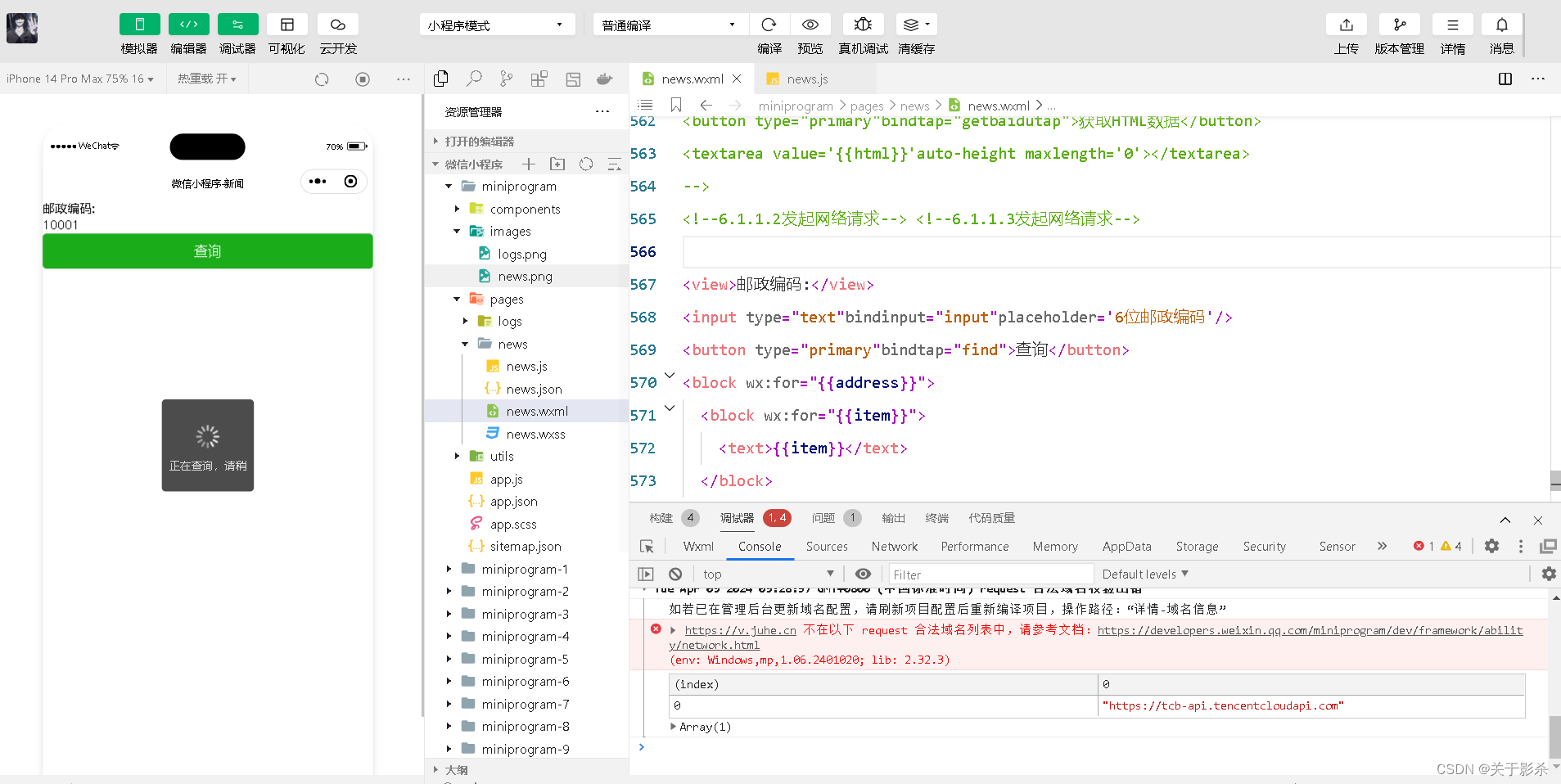
6.1.2 上传文件
属性
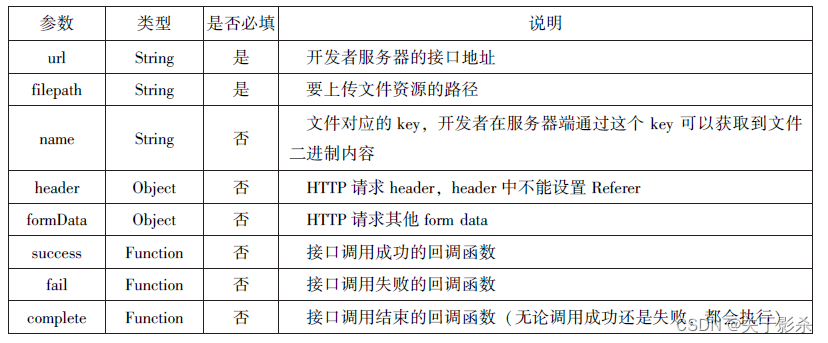
wxml代码
<button type="primary"bindtap="uploadimage">上传图片</button>
<image src="{{img}}"mode="widthFix"/>
js代码
data:{
img:null,
}
uploadimage:function(){
var that=this;
wx.chooseImage({
success:function(res){
var tempFilePaths=res.tempFilePaths
upload(that.tempFilePaths);
}
})
function upload(page,path){
wx.showToast({
icon:"loading",
title:"正在上传"
}),
wx.uploadFile({
url:"http://localhost/",
filePath:path[0],
name:'file',
success:function(res){
console.log(res);
if(res.statusCode!=200){
wx.showModal({
title: '提示',
content: '上传失败',
showCancel:false
})
return;
}
var data=res.data
page.setData({
img:path[0]
})
},
fail:function(e){
console.log(e);
wx.showModal({
title:'提示',
content:'上传失败',
showCancel:false
})
},
complete:function(){
wx.hideToast();
}
})
}
},
运行结果图
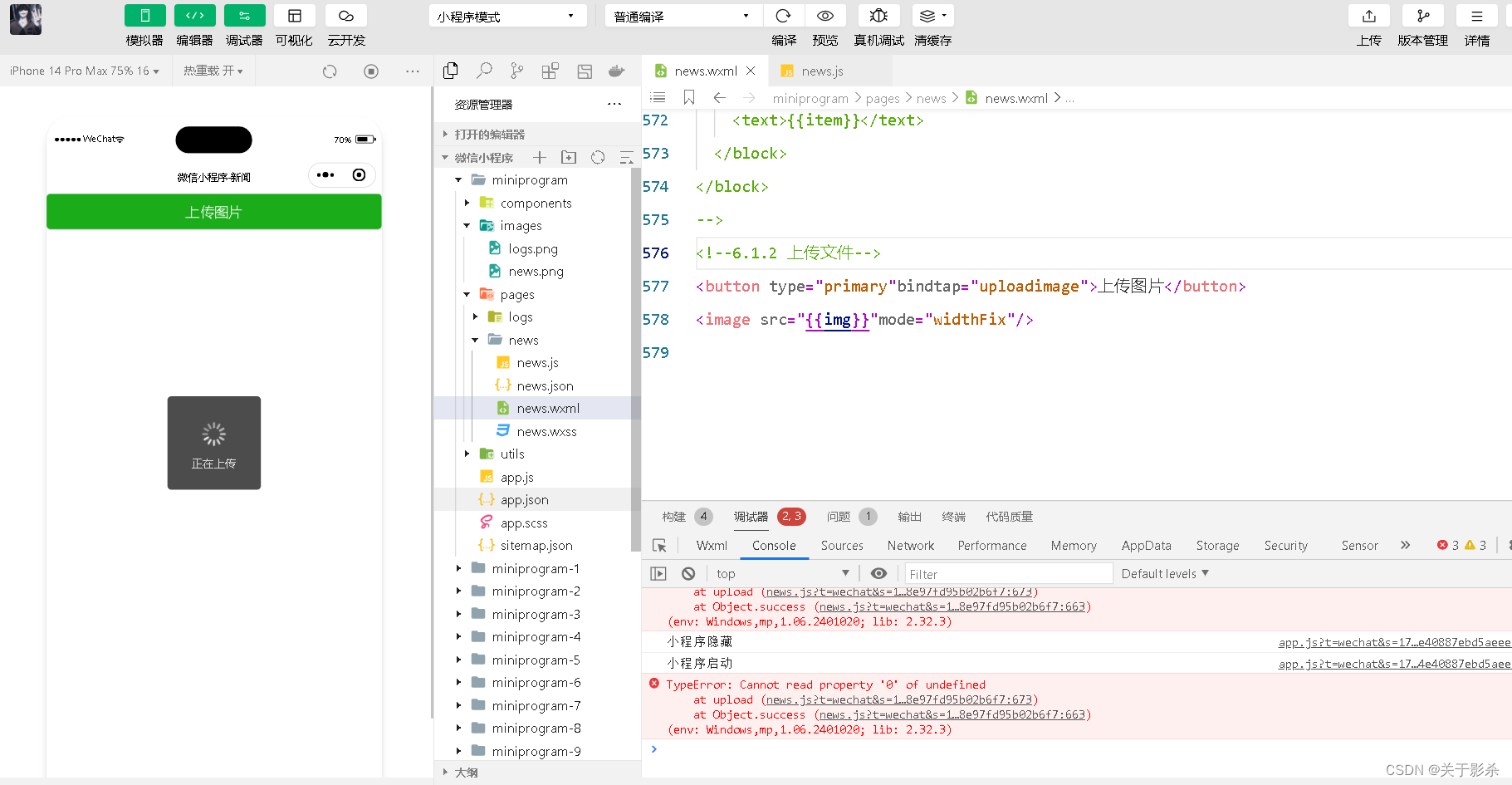
6.1.3 下载文件
属性
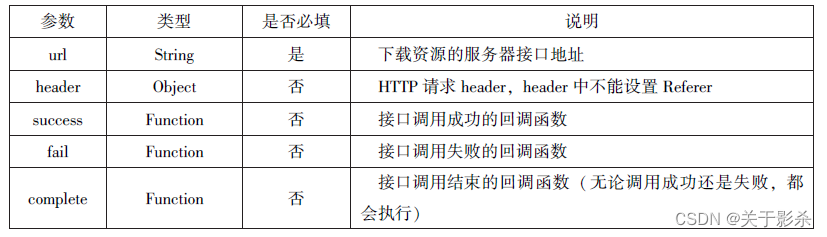
wxml代码
<button type="primary"bindtap='downloadimage'>下载图像</button>
<image src="{{img}}"mode='widthFix'style="width:90%;height:500px"></image>
js代码
Page({
data:{
img:null
},
downloadimage:function(){
var that=this;
wx.downloadFile({
url:"http://localhost/1.jpg",
success:function(res){
console.log(res)
that.setData({
img:res.tempFilePath
})
}
})
},
})
运行结果图
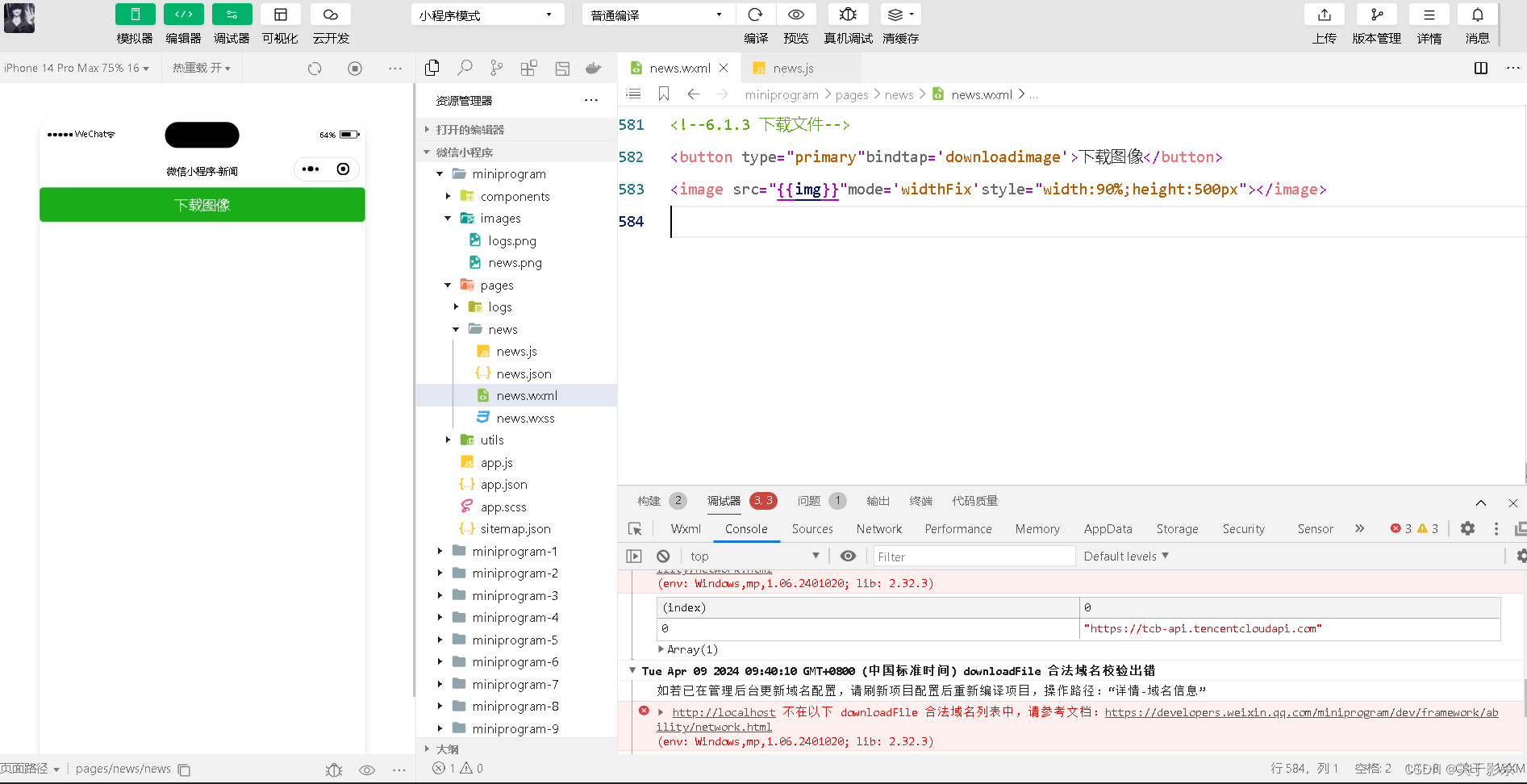
6.2 多媒体API
1.选择图片或拍照
属性


js代码
wx.chooseImage({
count:2,
sizeType:['original','compressed'],
sourceType:['album','camera'],
success:function(res){
var tempFilePaths=res.tempFilePaths
var tempFiles=res.tempFiles;
console.log(tempFilePaths)
console.log(tempFiles)
}
})
运行结果图
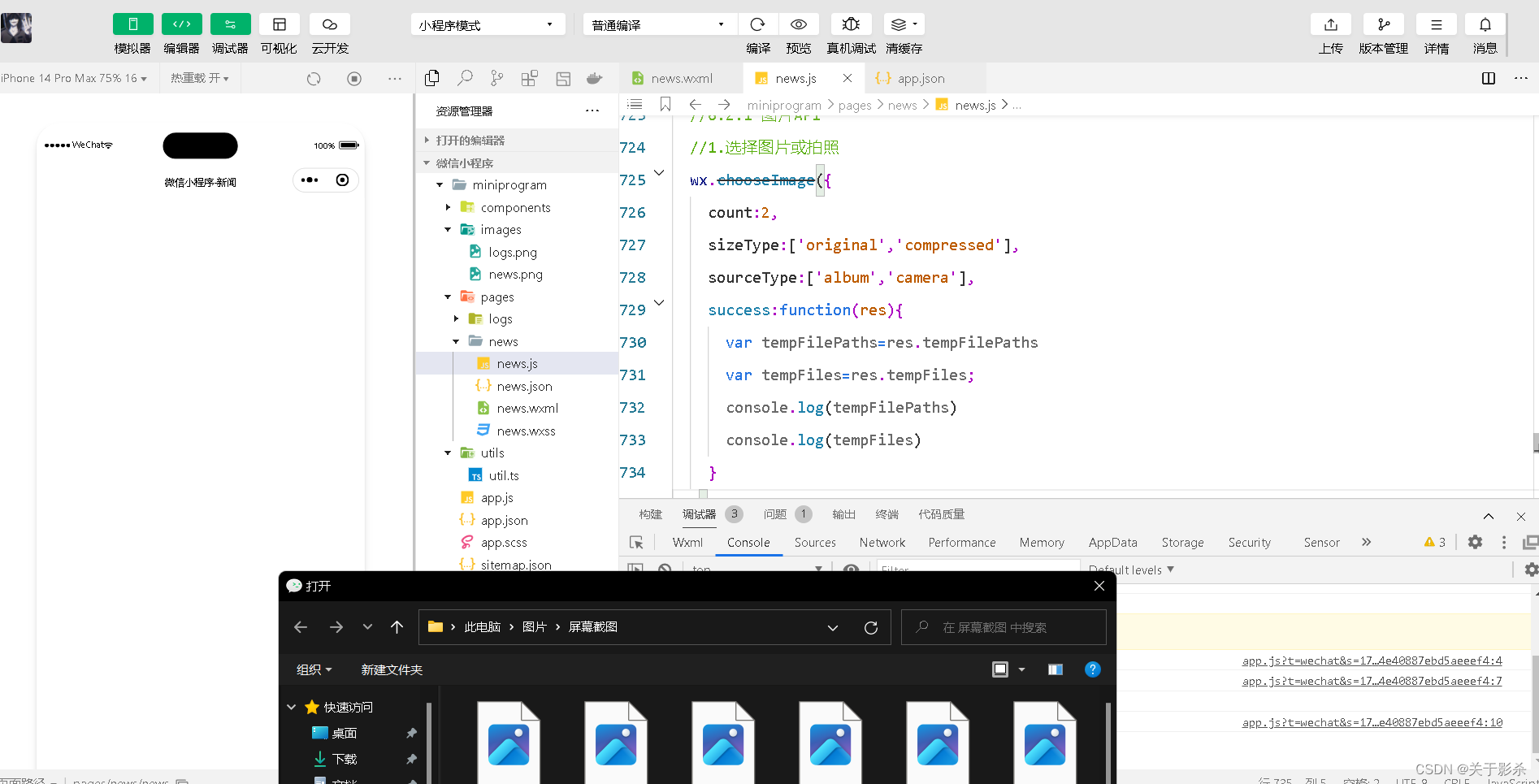
2.预览图片
属性
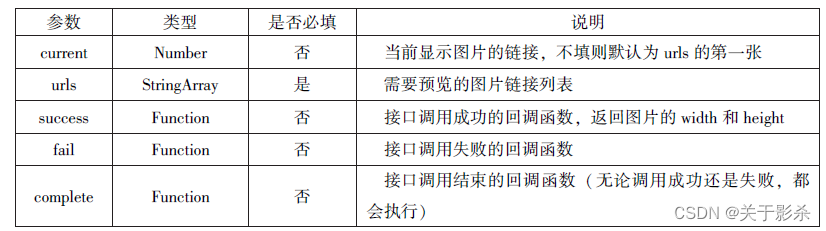
js代码
wx.previewImage({
current:"http://bmob-cdn-16488.b0.upaiyun.com/2018/02/05/2.png",
urls: ["http://bmob-cdn-16488.b0.upaiyun.com/2018/02/05/1.png",
"http://bmob-cdn-16488.b0.upaiyun.com/2018/02/05/2.png",
"http://bmob-cdn-16488.b0.upaiyun.com/2018/02/05/3.png"]
})
运行结果图
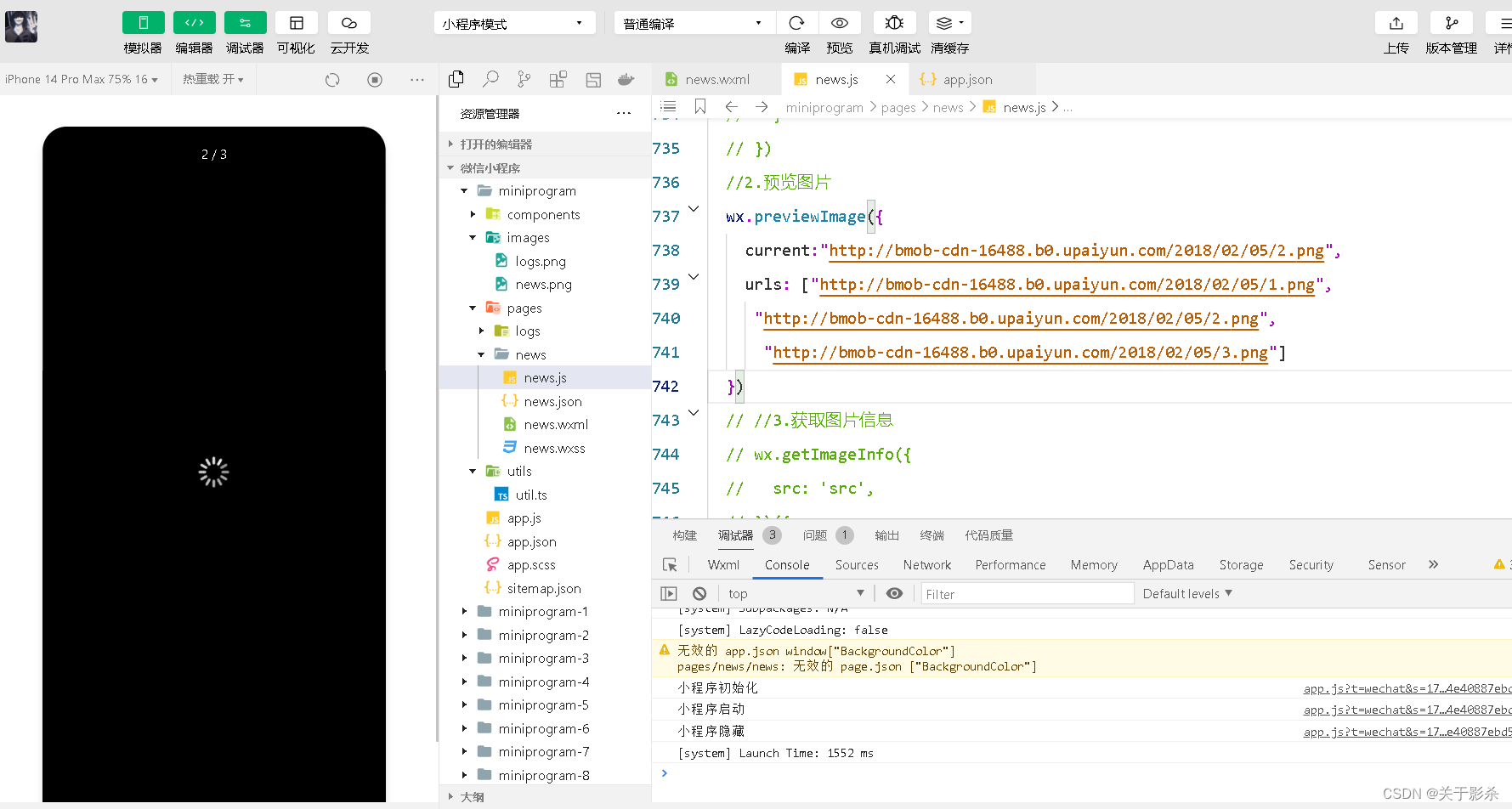
3.获取图片信息
属性
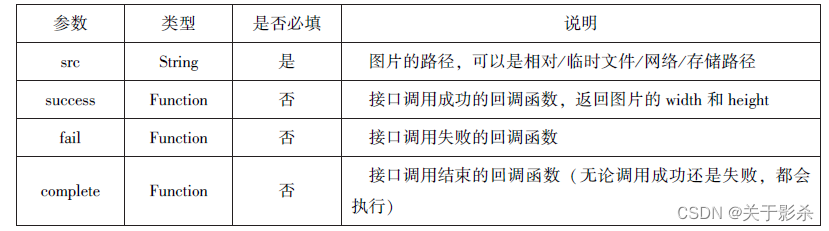
js代码
wx.getImageInfo({
src: 'src',
})({
success:function(res){
wx.getImageInfo({
src:res.tempFilePaths[0],
success:function(e){
console.log(e.width)
console.log(e.height)
}
})
}
})
运行结果图
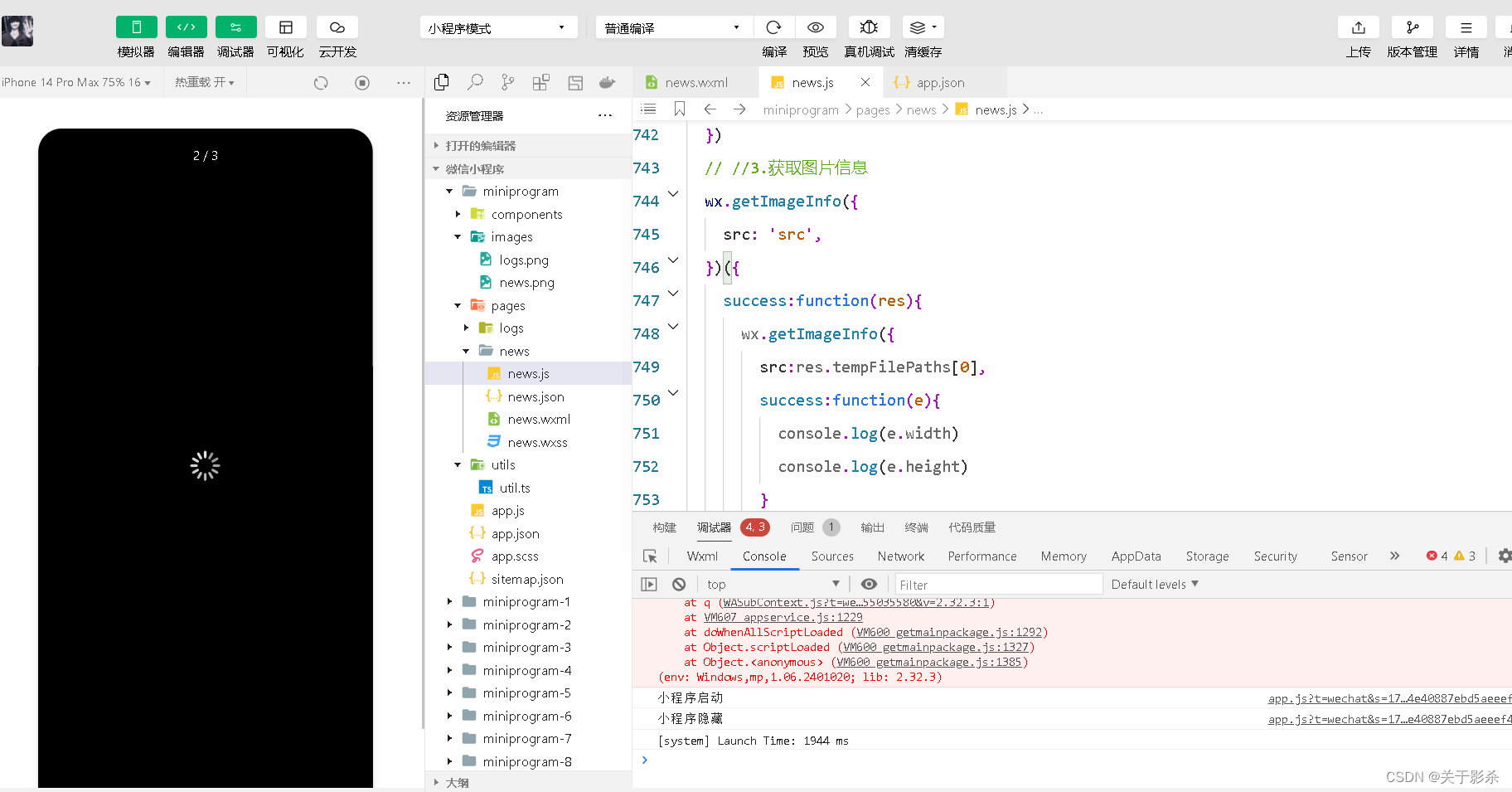
4.保存图片到系统相册
属性
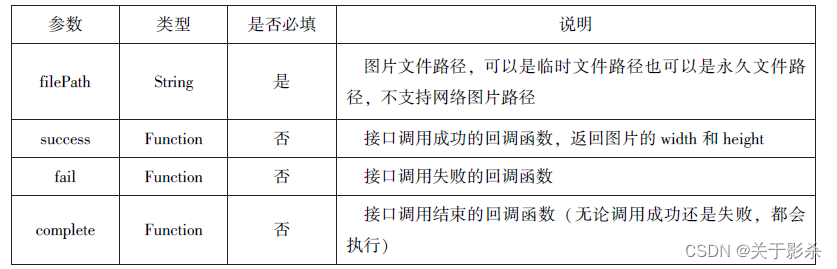
js代码
wx.saveImageToPhotosAlbum({
filePath: 'filePath',
})({
success:function(res){
wx.saveImageToPhotosAlbum({
filePath: res.tempFilePaths[0],
success:function(e){
console.log(e)
}
})
},
})
运行结果图
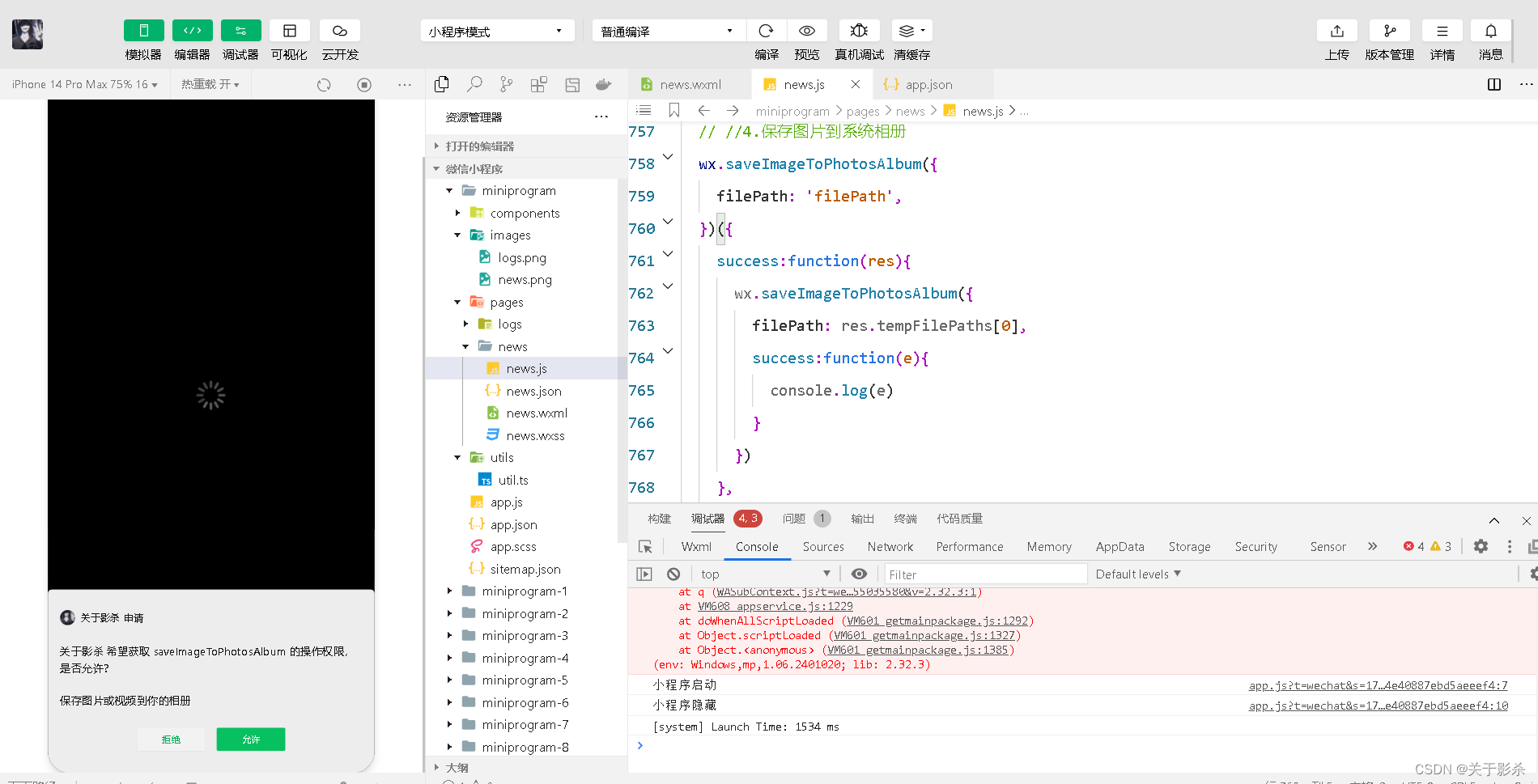
6.2.2 录音API
1.开始录音
属性
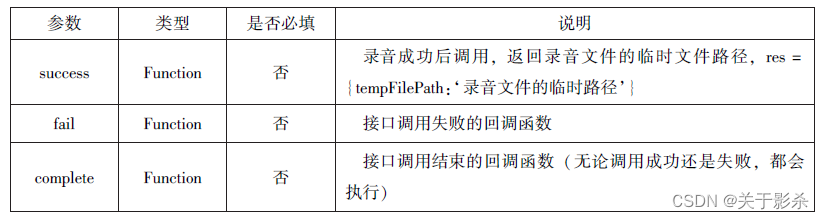
js代码
wx.startRecord({
success:function(res){
var tempFilePath=res.tempFilePath
},
fail:function(res){
}
}),
setTimeout(function() {
wx.stopRecord();
}, 10000)
运行结果图
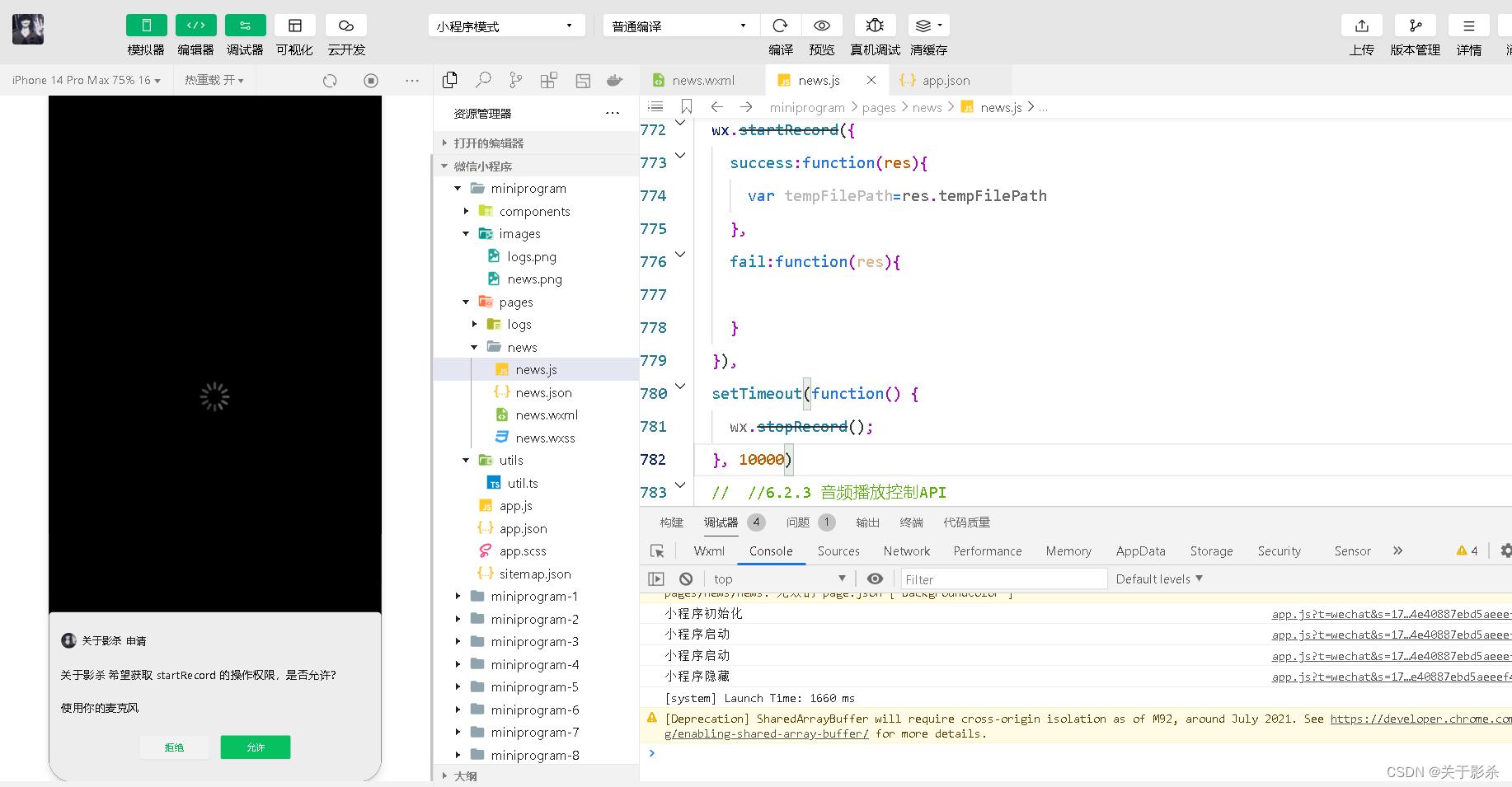
2.停止录音
属性
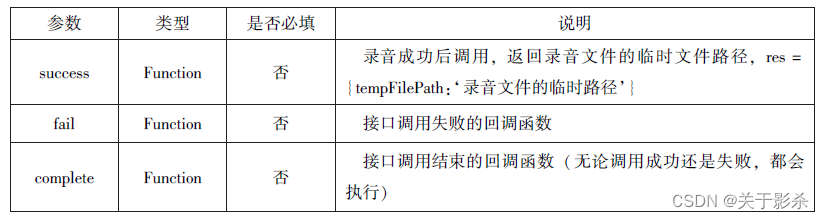
js代码
wx.startRecord({
success:function(res){
var tempFilePath=res.tempFilePath
},
fail:function(res){
}
}),
setTimeout(function() {
wx.stopRecord();
}, 10000)
运行结果图
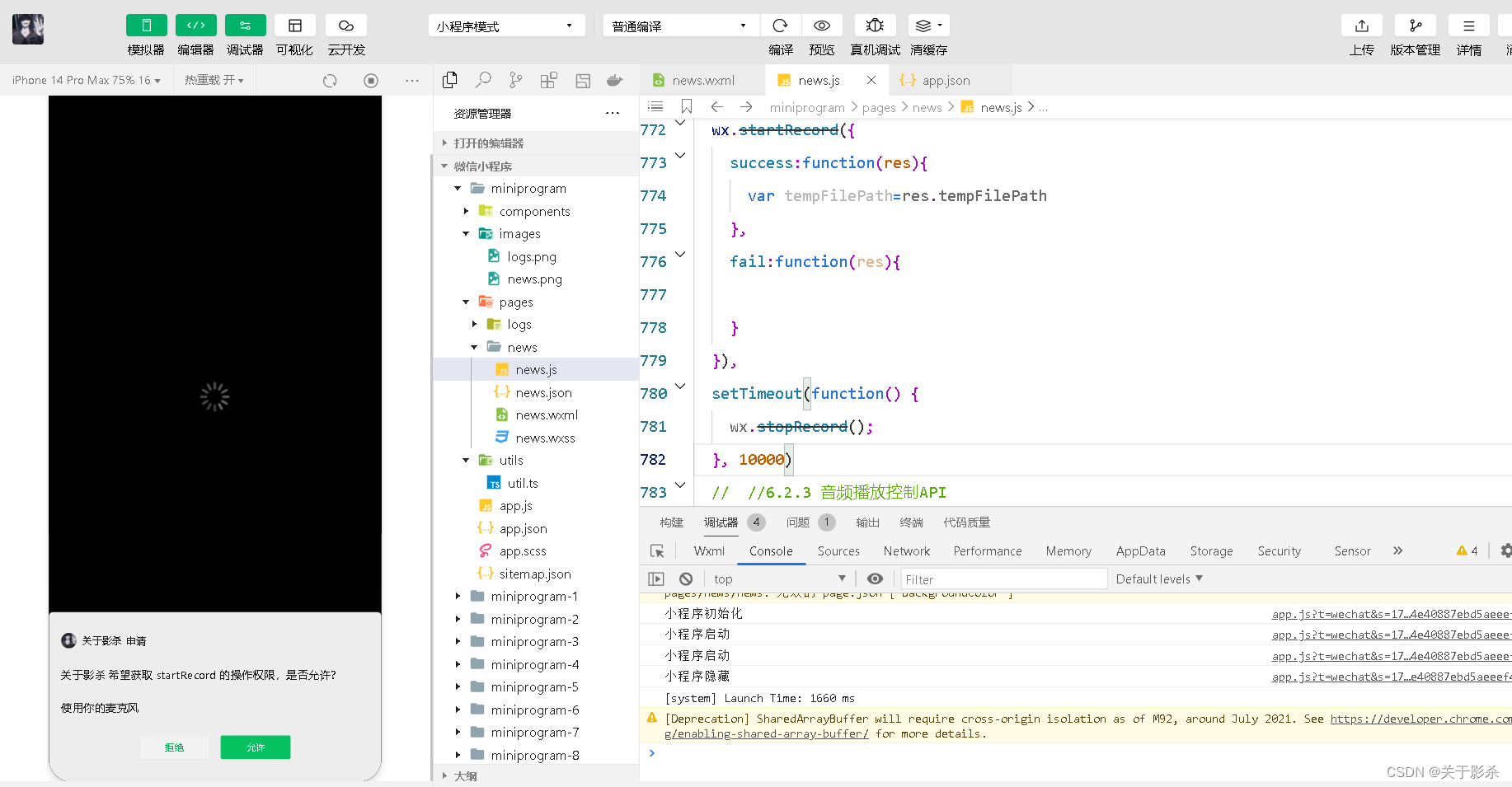
6.2.3 音频播放控制API
1.播放语音
属性
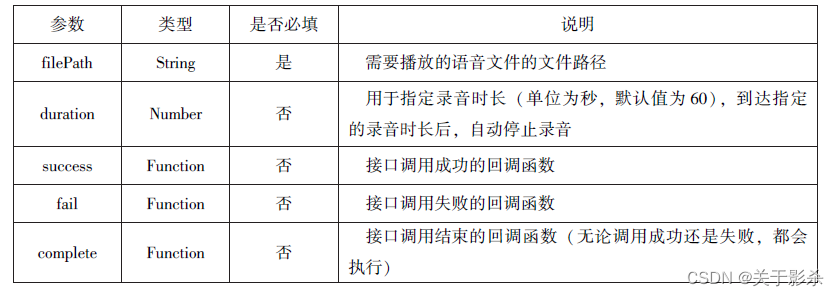
js代码
wx.startRecord({
success:function(res){
var tempFilePath=res.tempFilePath
wx.playVoice({
filePath:tempFilePath,
complete:function(){
}
})
}
})
运行结果图
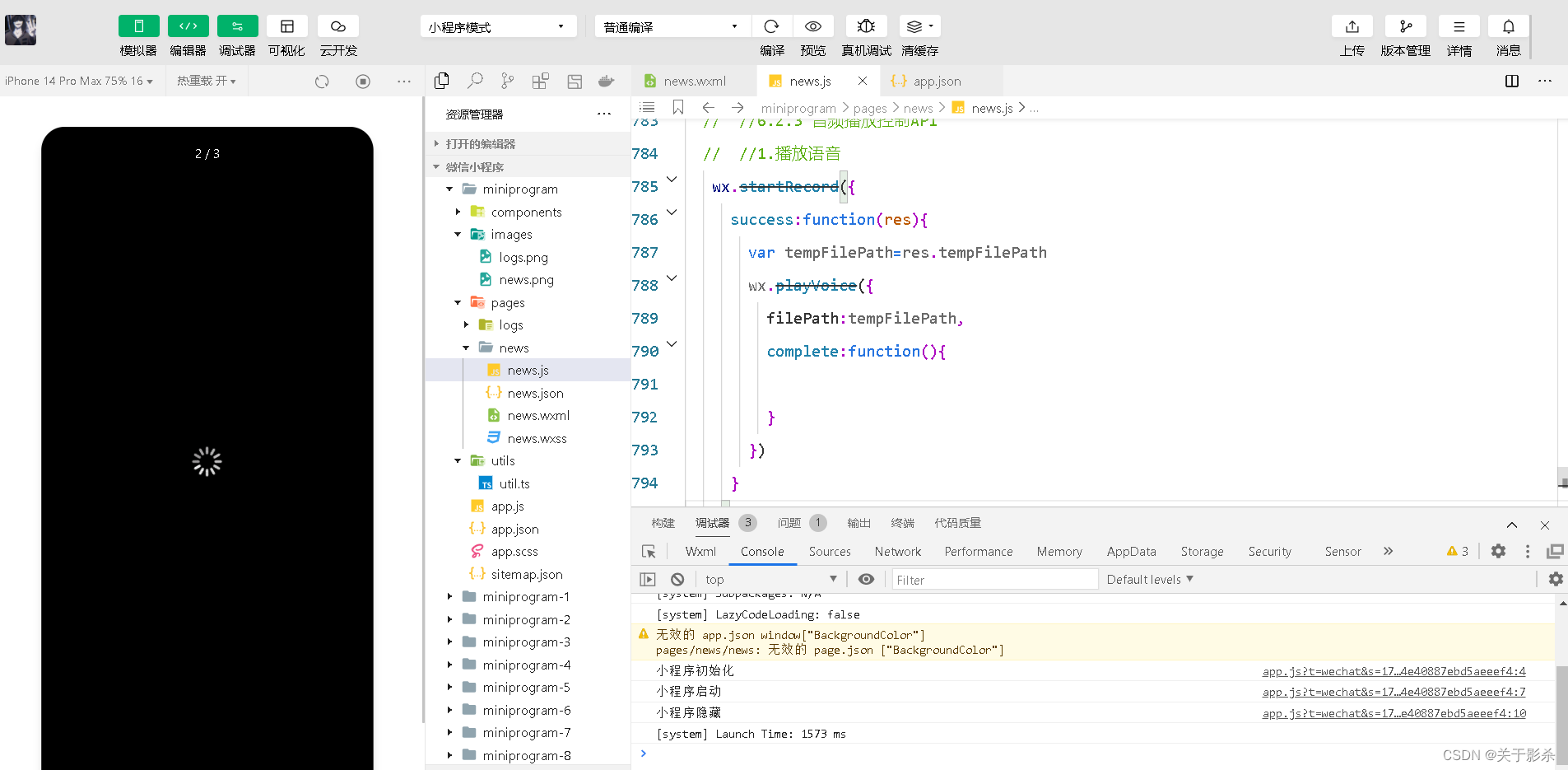
2.暂停播放
属性
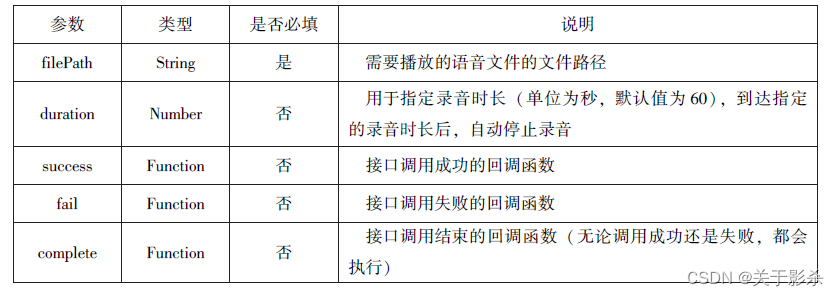
js代码
wx.startRecord({
success:function(res){
var tempFilePath=res.tempFilePath
wx.playVoice({
filePath:tempFilePath
})
setTimeout(function(){
wx.pauseVoice({
filePath:tempFilePath
})
setTimeout(function(){
wx.pauseVoice()
},5000)
})
}
})
运行结果图
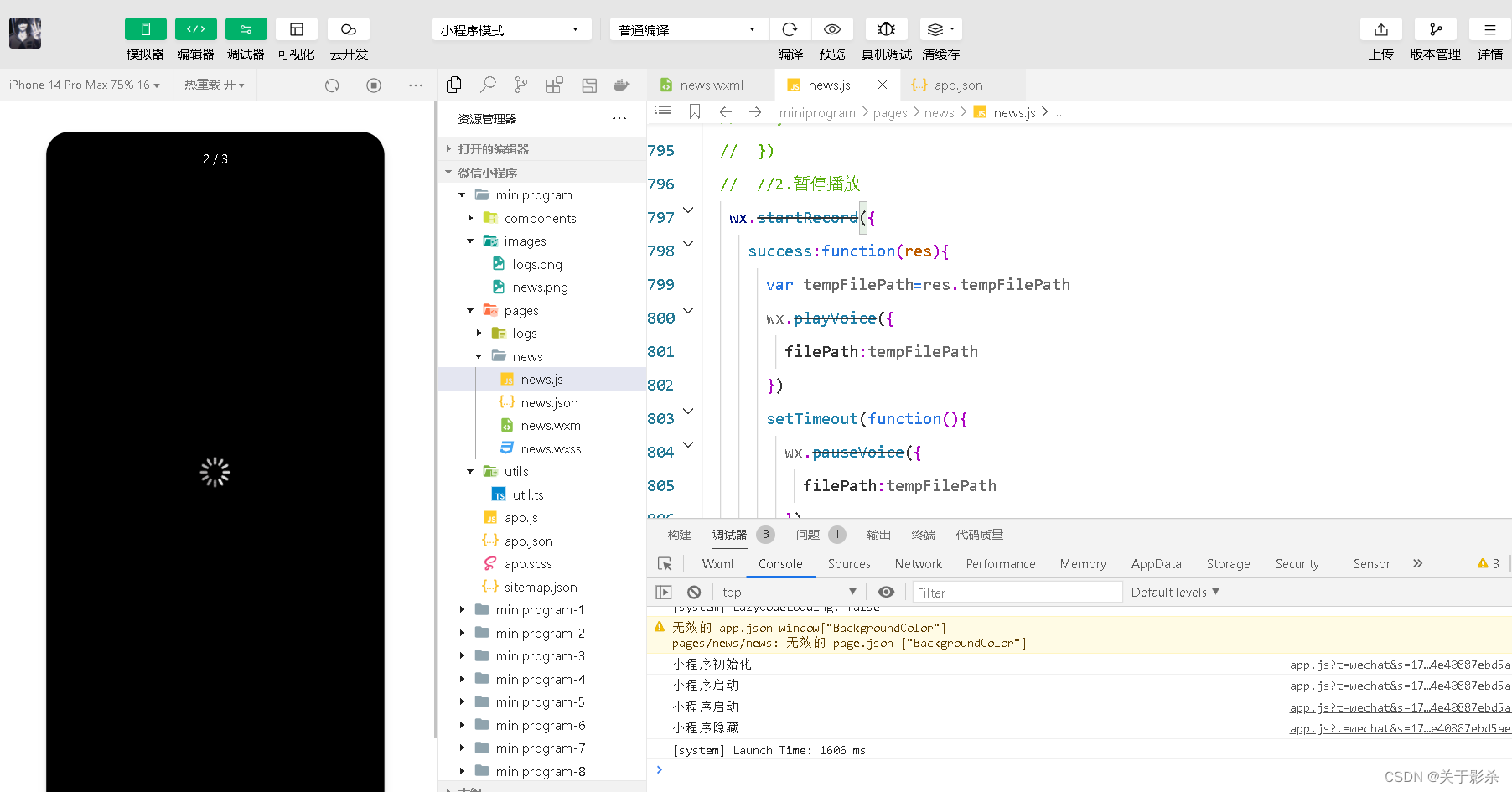
3.结束播放
属性
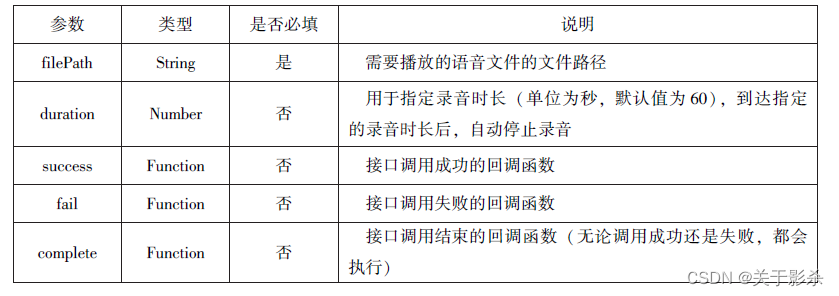
js代码
wx.startRecord({
success:function(res){
var tempFilePath=res.tempFilePath
wx.playVoice({
filePath:tempFilePath
})
setTimeout(function(){
wx.stopVoice()
},5000)
}
})
运行结果图
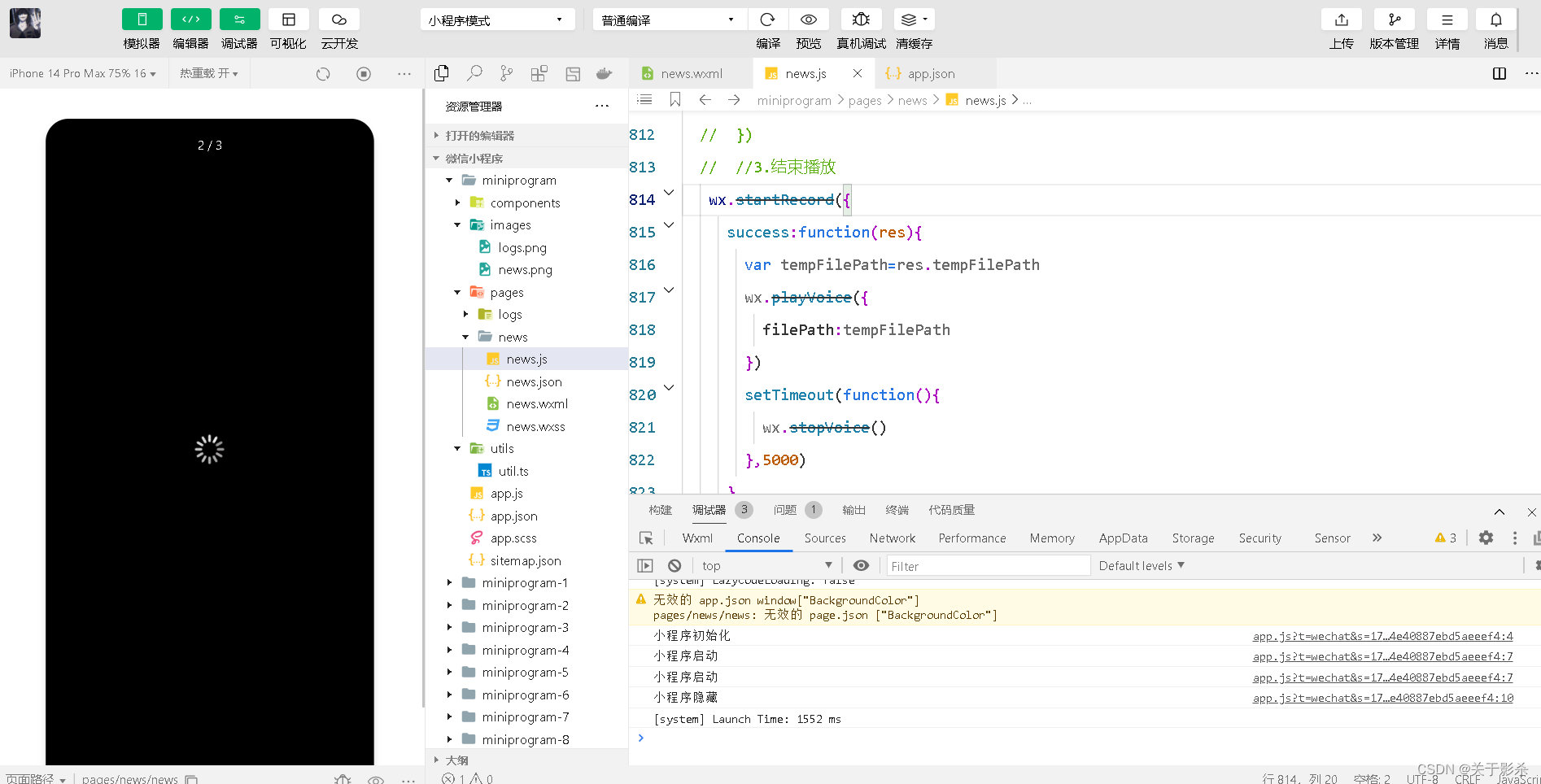
6.2.4 音乐播放控制API
1.播放音乐
属性
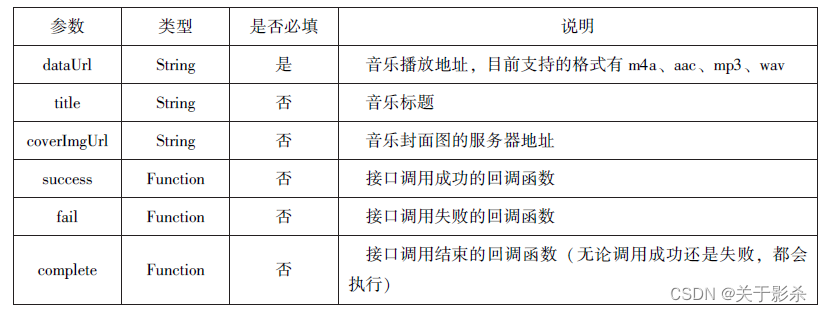
2.获取音乐播放状态
属性
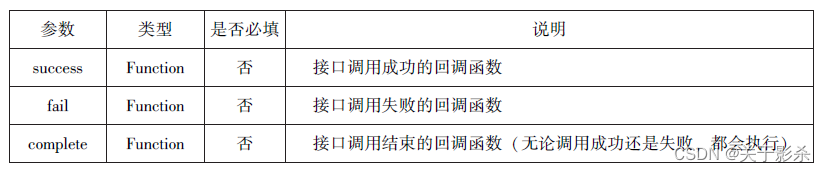
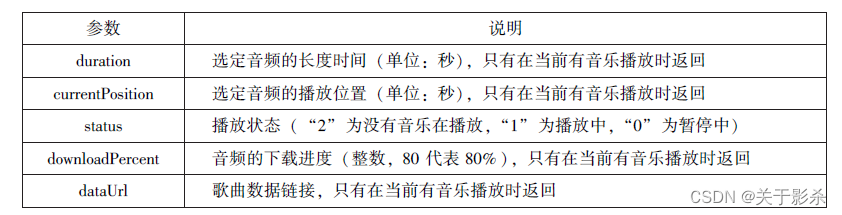
3.控制音乐播放进度
属性
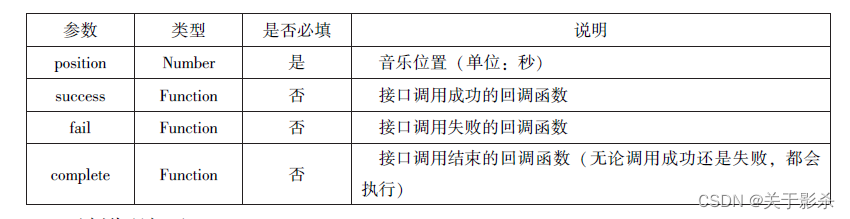
9.案例展示
wxml代码
<view class="container">
<image class="bgaudio" src="{{changedImg?music.coverImg:'/images/logs.png'}}"/>
<view class="control-view">
<image src="/images/logs.png"bindtap="onPositionTap" data-how="0"/>
<image src="../../images/{{isplaying?'pause':'play'}}.png" bindtap="onAudioTap"/>
<image src="/images/logs.png" bindtap="onStopTap"/>
<image src="/images/news.png" bindtap="onPositionTap" data-how="1"/>
</view>
</view>
wxss代码
.bgaudio{
height: 350rpx;
width: 350rpx;
margin-bottom: 100rpx;
}
.control-viewimage{
height: 64rpx;
width: 64rpx;
margin: 30rpx;
}
json代码
{}
js代码
Page({
data:{
isplaying:false,
coverImg:"",
changedImg:false,
music:{
"url":"http://bmob-cdn-16488.b0.upaiyun.com/2018/02/09/117e4a1b405195b18061299e2de89597.mp3",
"title":"盛晓玫-有一天",
"coverImg":"http://bmob-cdn-16488.b0.upaiyun.com/2018/02/09/f604297140c9681880cc3d3e581f7724.jpg"
},
},
onLoad:function(){
this.onAudioState();
},
onAudioTap:function(event){
if(this.data.isplaying){
wx.pauseBackgroundAudio();
}else{
let music=this.data.music;
wx.playBackgroundAudio({
dataUrl: music.url,
title:music.title,
coverImgUrl:music.coverImg
})
}
},
onStopTap:function(){
let that=this;
wx.stopBackgroundAudio({
success:function(){
that.setData({
isplaying:false,changedImg:false
});
}
})
},
onPositionTap:function(event){
let how=event.target.dataset.how;
wx.getBackgroundAudioPlayerState({
success:function(res){
let status=res.status;
if(status===1){
let duration=res.duration;
let currentPosition=res.currentPosition;
if(how==="0"){
let position=currentPosition-10;
if(position<0){
position=1;
}
wx.setBackgroundAudio({
position:position
});
wx.showToast({
title: '快退10s',
duration:500
});
}
if(how==="1"){
let position=currentPosition+10;
if(position>duration){
position=duration-1;
}
wx.seekBackgroundAudio({
position: position
});
wx.showToast({
title: '快进10s',
duration:500
});
}
}else{
wx.showToast({
title: '音乐未播放',
duration:800
});
}
}
})
},
onAudioState:function(){
let that=this;
wx.onBackgroundAudioPlay(function(){
that.setData({
isplaying:true,
changedImg:true
});
console.log("on play");
});
wx.onBackgroundAudioPause(function(){
that.setData({
isplaying:false
});
console.log("on pause");
});
wx.onBackgroundAudioStop(function(){
that.setData({
isplaying:false,
changedImg:false
});
console.log("on stop")
});
}
})
6.3 文件API
1.保存文件
属性
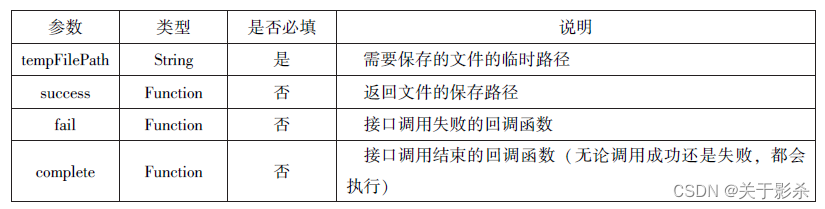
js代码
Page({
saveImg:function () {
wx.chooseImage({
count:1,
sizeType:['original','compressed'],
sourceType:['album','camera'],
success:function (res) {
var tempFilePaths=res.tempFilePaths[0]
wx.saveFile({
tempFilePath:tempFilePaths,
success:function (res) {
var saveFilePath=res.savedFilePath;
console.log(saveFilePath)
}
})
}
})
}
})
运行结果图
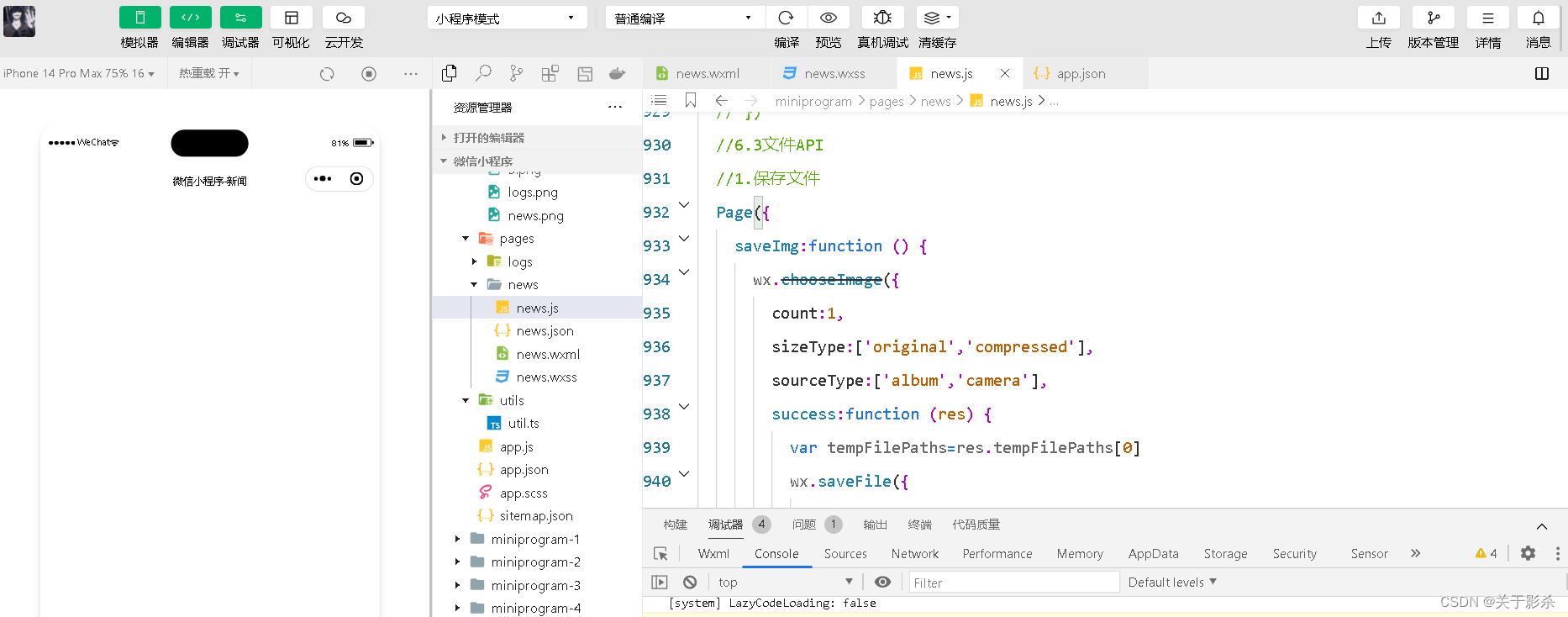
2.获取本地文件列表
属性

js代码
Page({})
wx.getFileSystemManager().getSavedFileList({
success:function (res) {
that.setData({
fileList:res.fileList
})
}
})
运行结果图
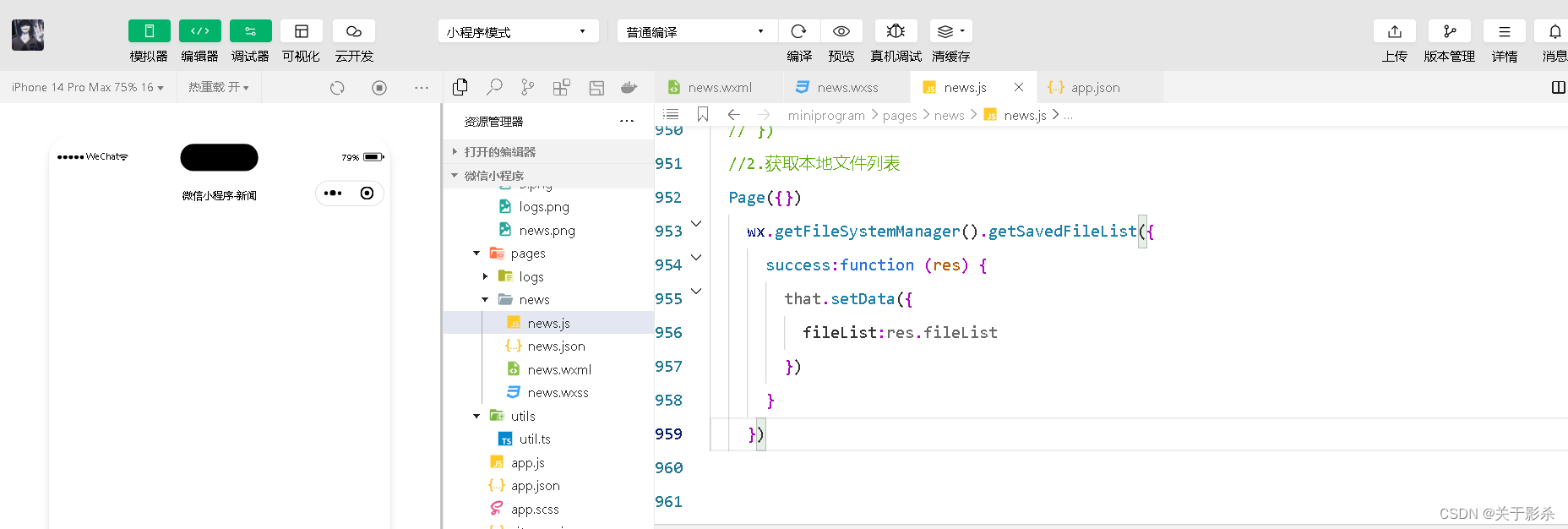
3.获取本地文件的文件信息
属性
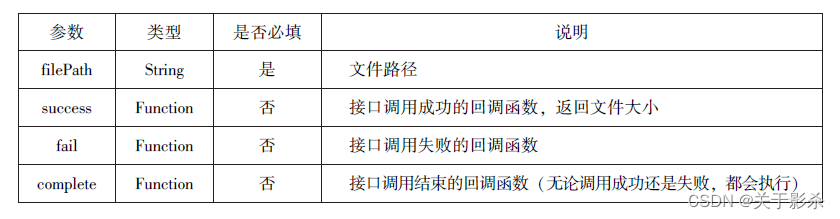
js代码
wx.chooseImage({
count:1,
sizeType:['original','compressed'],
sourceType:['album','camera'],
success:function (res) {
var tempFilePaths=res.tempFilePaths[0]
wx.saveFile({
tempFilePath:tempFilePaths,
success:function (res) {
var saveFilePath=res.savedFilePath;
wx.getSavedFileInfo({
filePath:saveFilePath,
success:function (res) {
console.log(res.size)
}
})
}
})
}
})
运行结果图
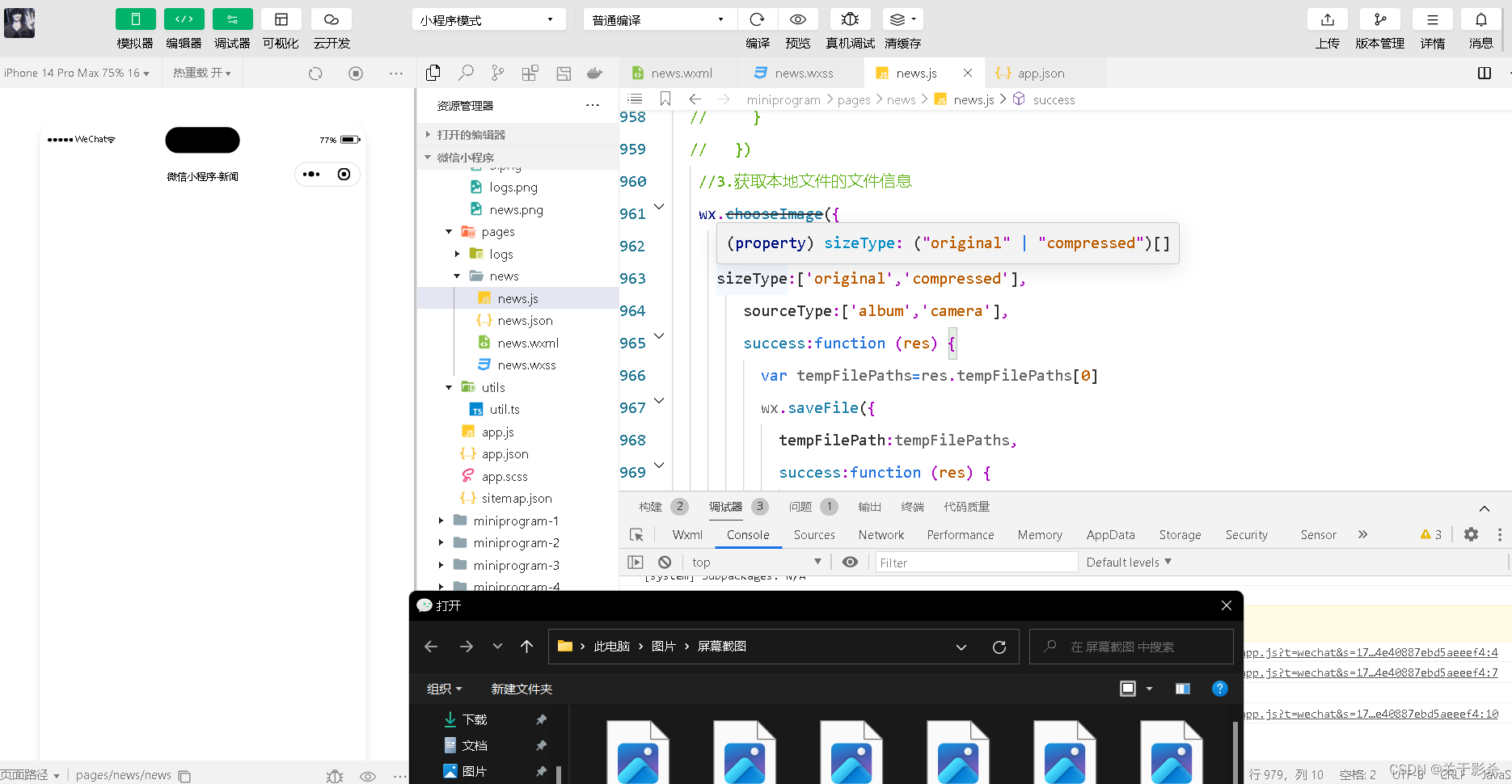
4.删除本地文件
属性
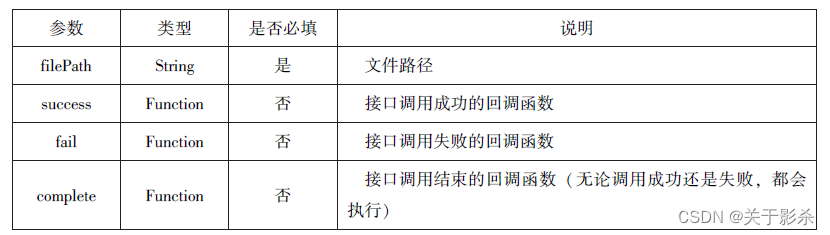
js代码
wx.getSavedFileList({
success:function (res) {
if(res.fileList.length>0){
wx.removeSavedFile({
filePath:res.fileList[0].filePath,
complete:function (res) {
console.log(res)
}
})
}
}
})
运行结果图
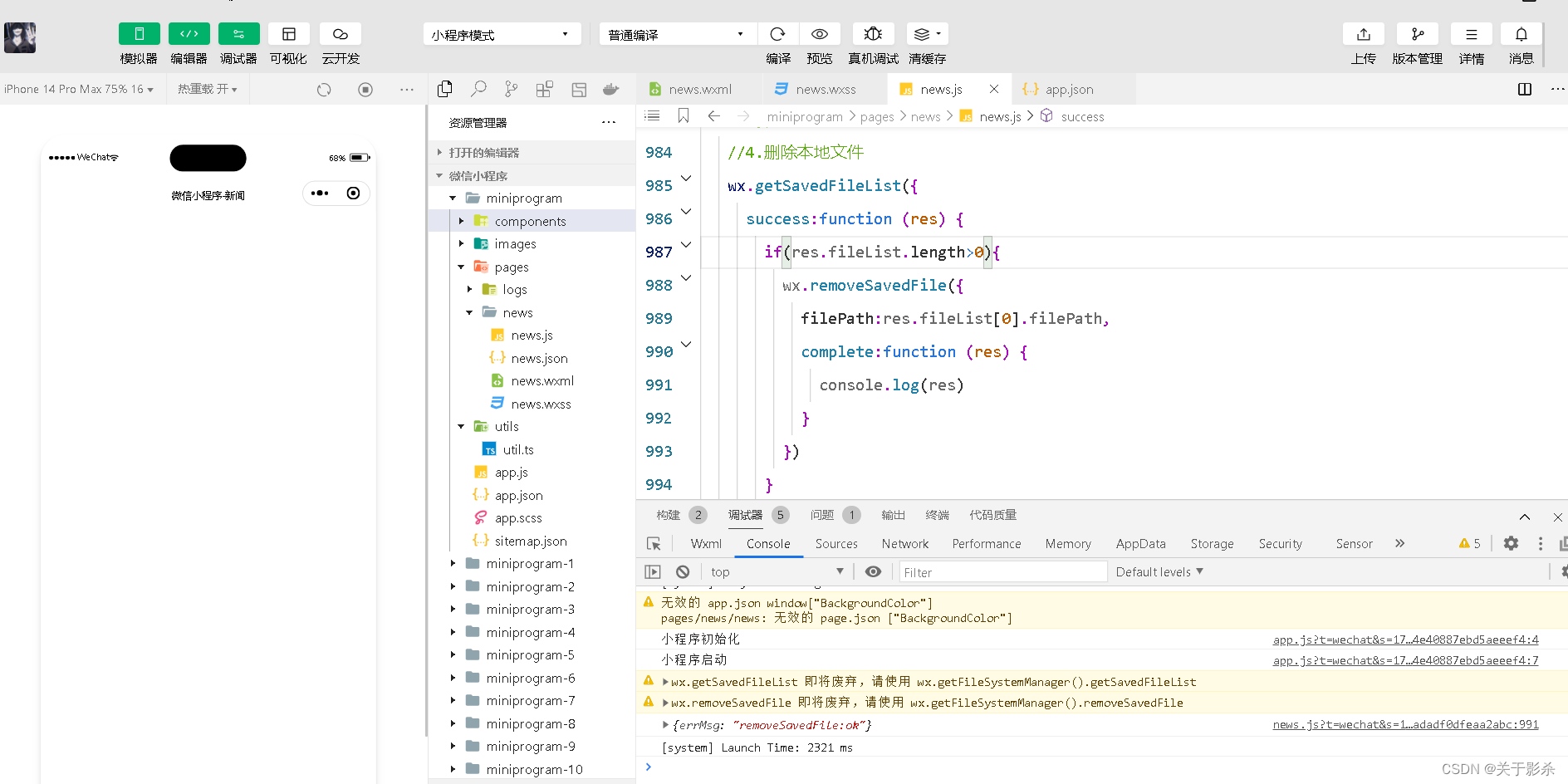
5.打开文档
属性
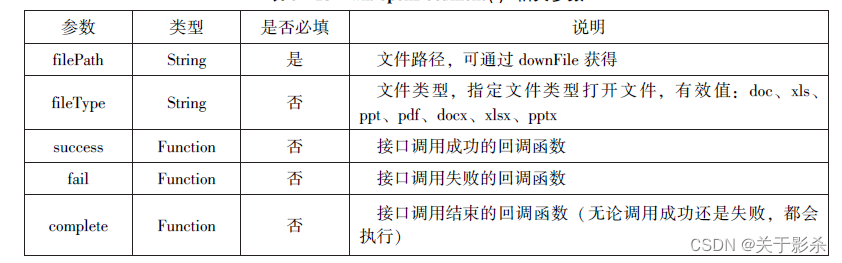
js代码
wx.downloadFile({
url:"http://localhost/fm2.pdf",
seccess:function (res) {
var tempFilePath=res.tempFilePath;
wx.openDocument({
filePath:tempFilePath,
success:function(res){
console.log("打开成功")
}
})
}
})
运行结果图
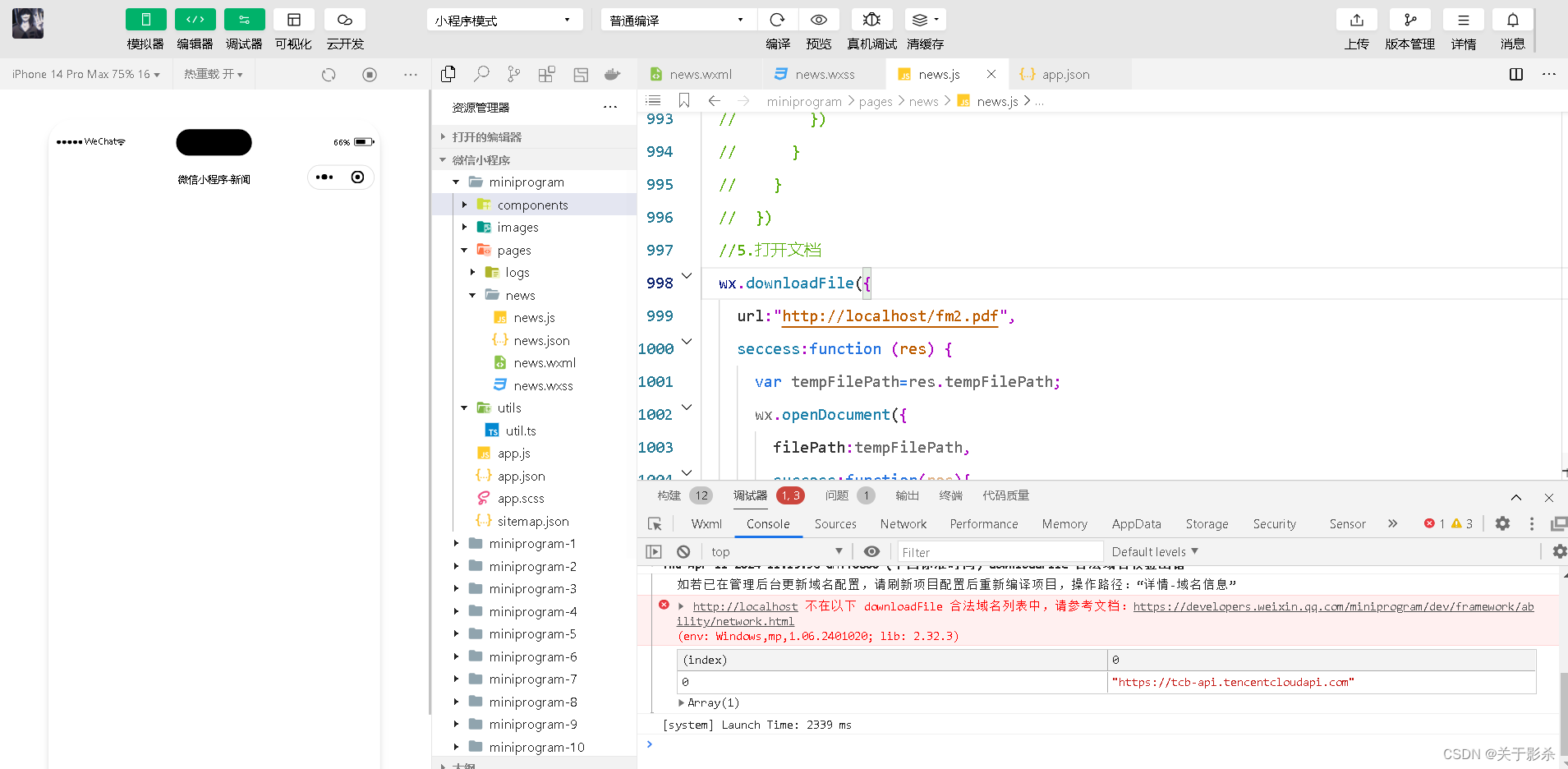
6.4 本地数据及缓存API
6.4.1 保存数据
属性
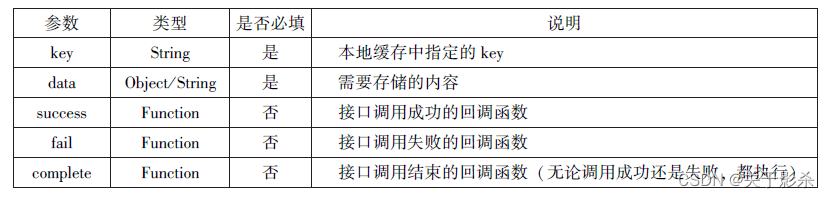
1.异步
js代码
wx.setStorage({
key:'name',
data:'sdy',
success:function (res) {
console.log(res)
}
})
运行结果图
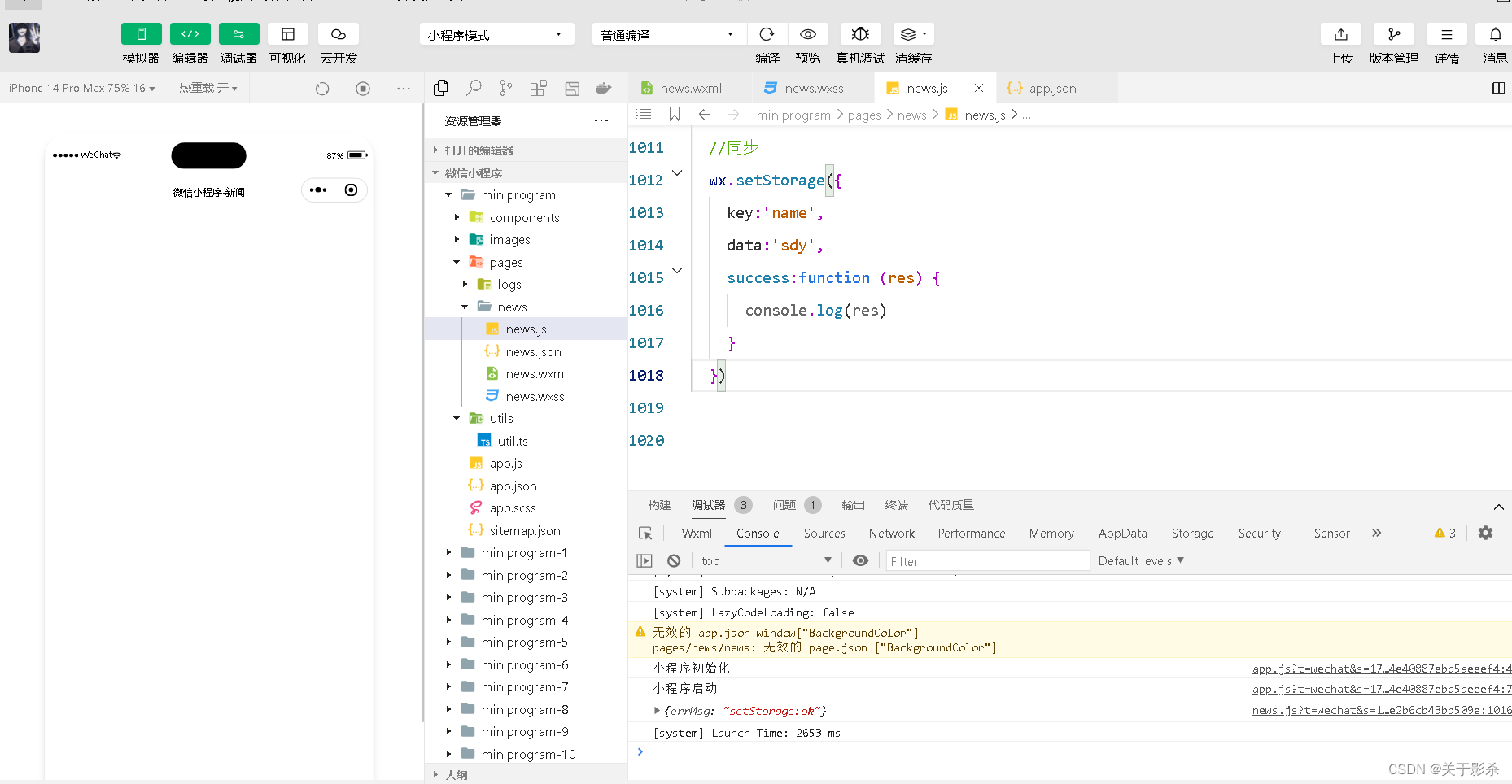
2.同步
js代码
wx.setStorageSync('age','25')
运行结果图
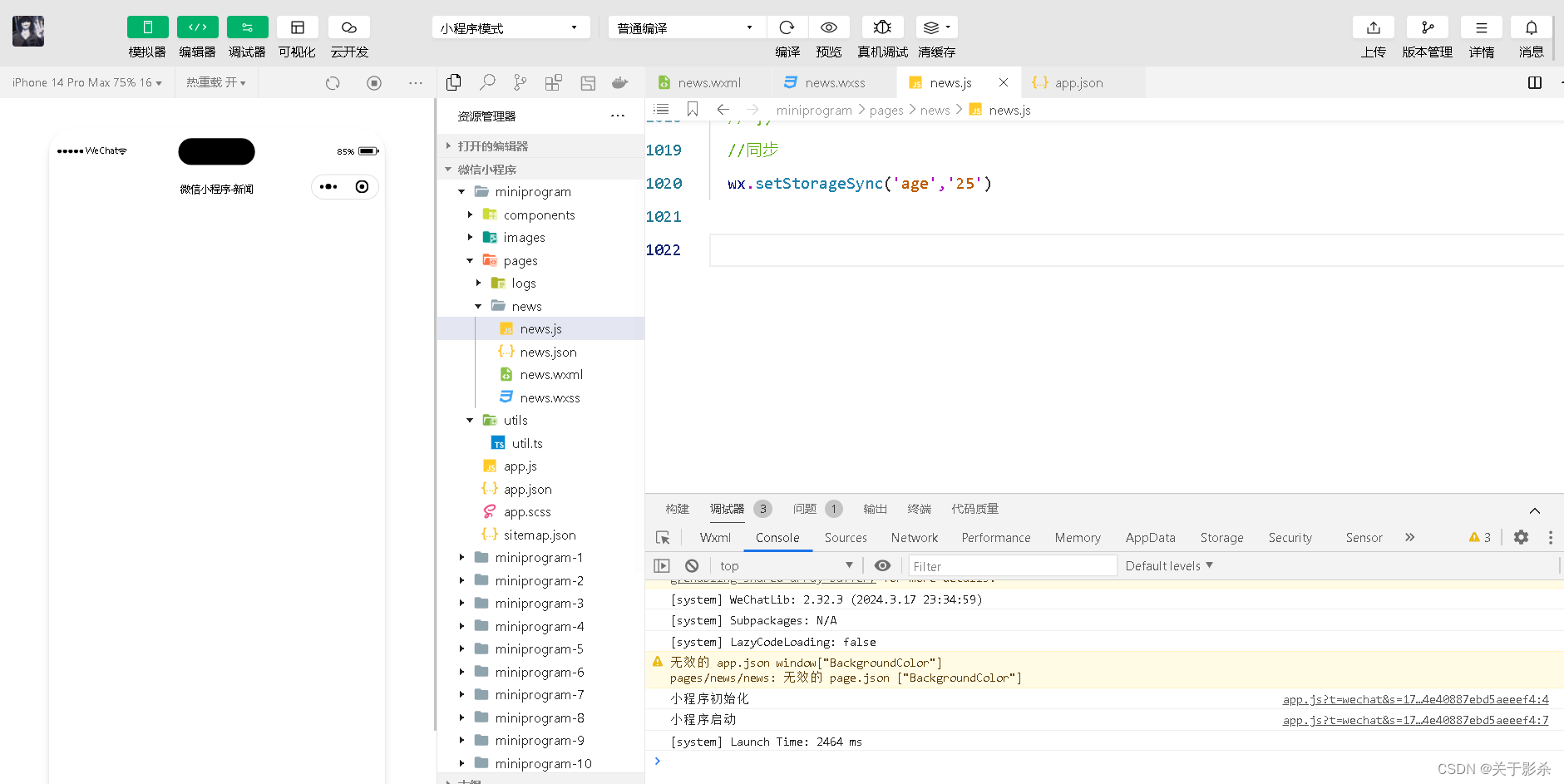
6.4.2 获取数据
属性
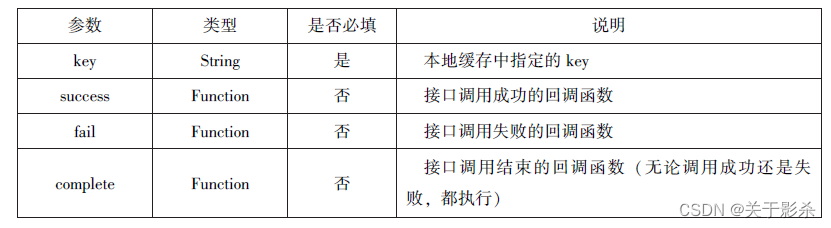
1.异步
js代码
wx.getStorage({
key:'name',
success:function(res){
console.log(res.data)
},
})
运行结果图
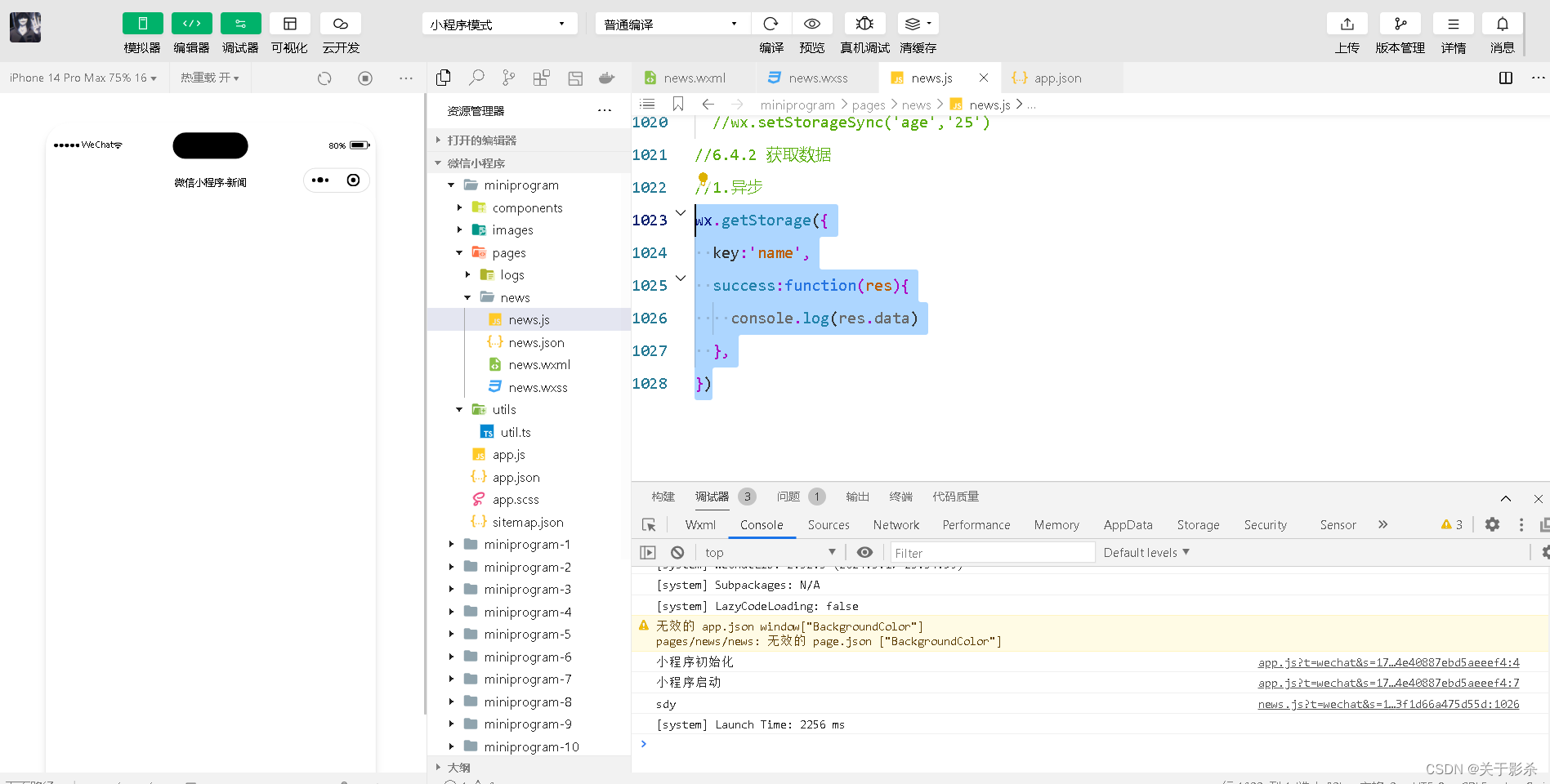
2.同步
js代码
try{
var value=wx.getStorageSync('age')
if(value){
console.log("获取成功"+value)
}
}catch(e){
console.log("获取失败")
}
运行结果图
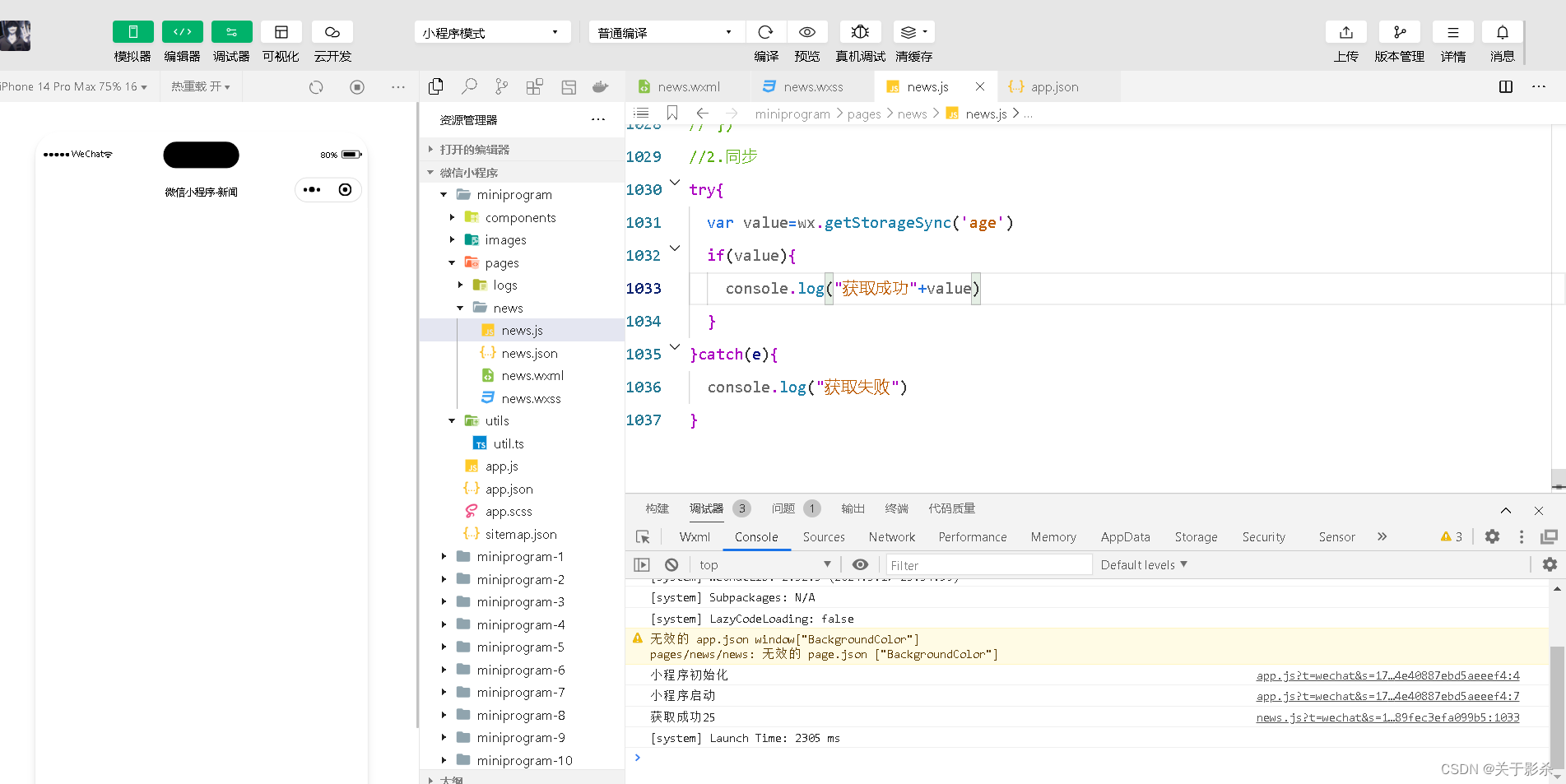
6.4.3 删除数据
属性
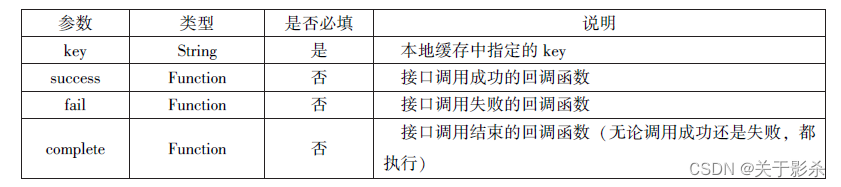
1.异步
js代码
wx.removeStorage({
key:'name',
success:function(res){
console.log("删除成功")
},
fail:function(){
console.log("删除失败")
}
})
运行结果图
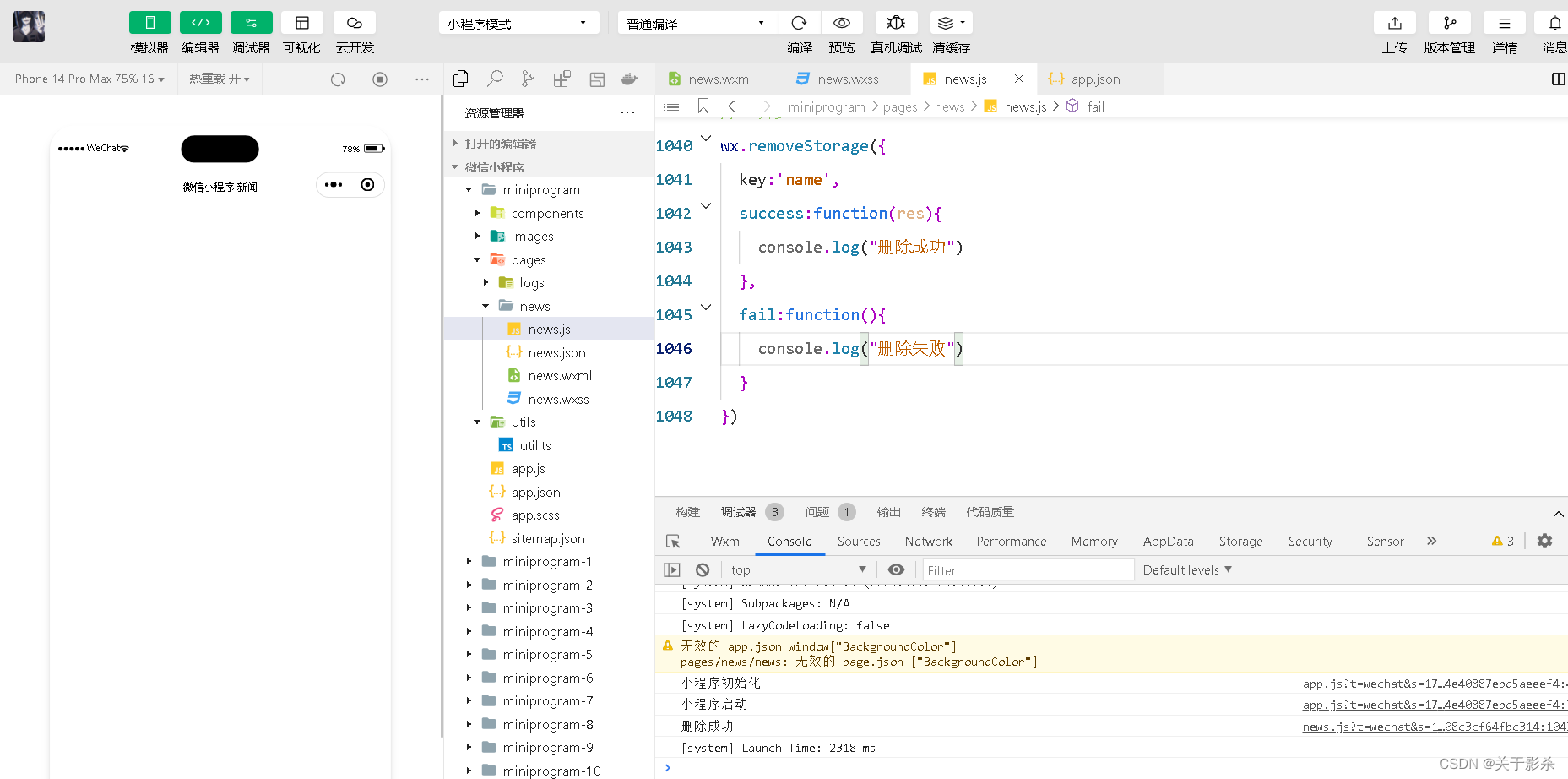
2.同步
js代码
try{
wx.removeStorageSync('name')
}catch(e){
}
运行结果图
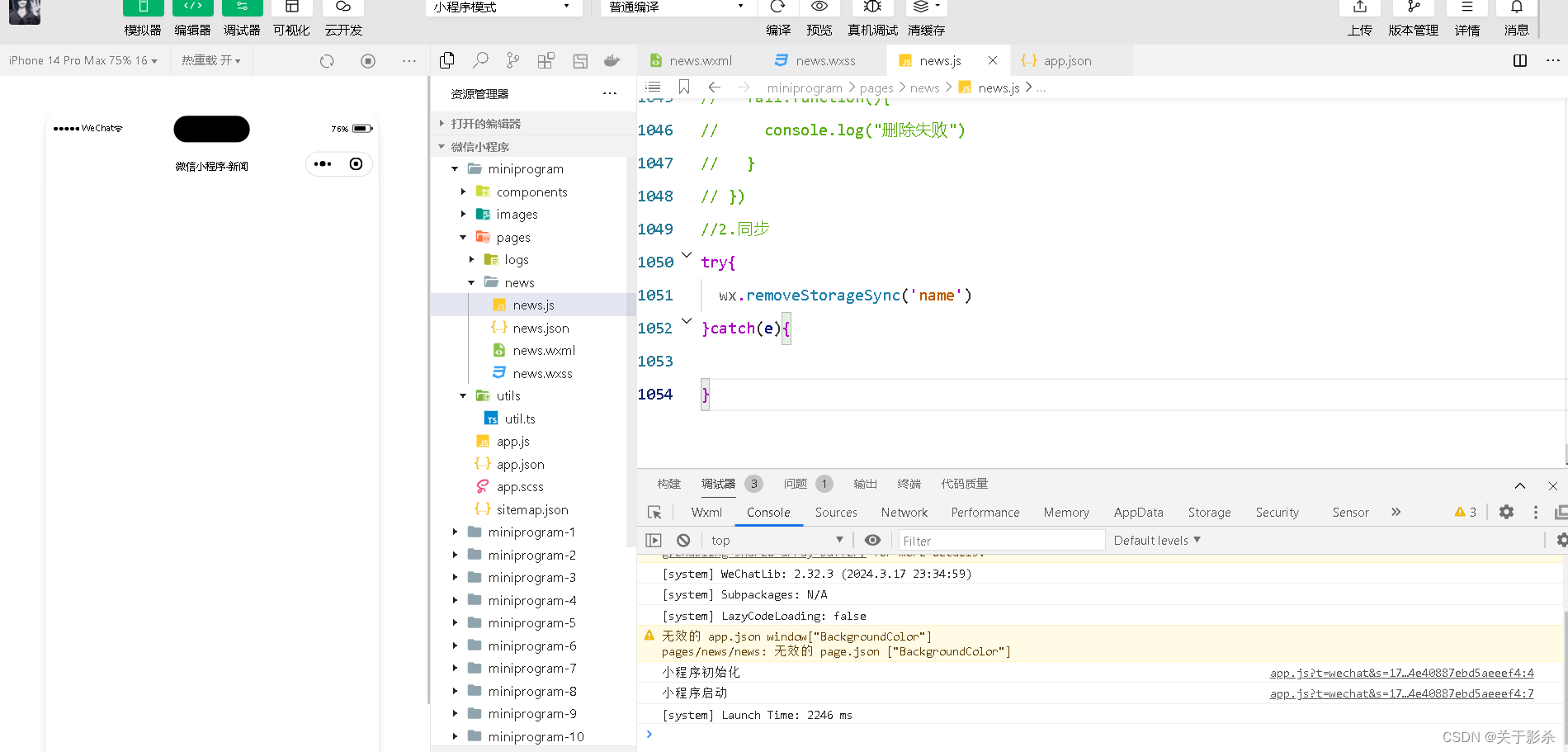
6.4.4 清空数据
1.异步
js代码
wx.getStorage({
key:'name',
success:function(res){
wx.clearStorage()
},
})
运行结果图
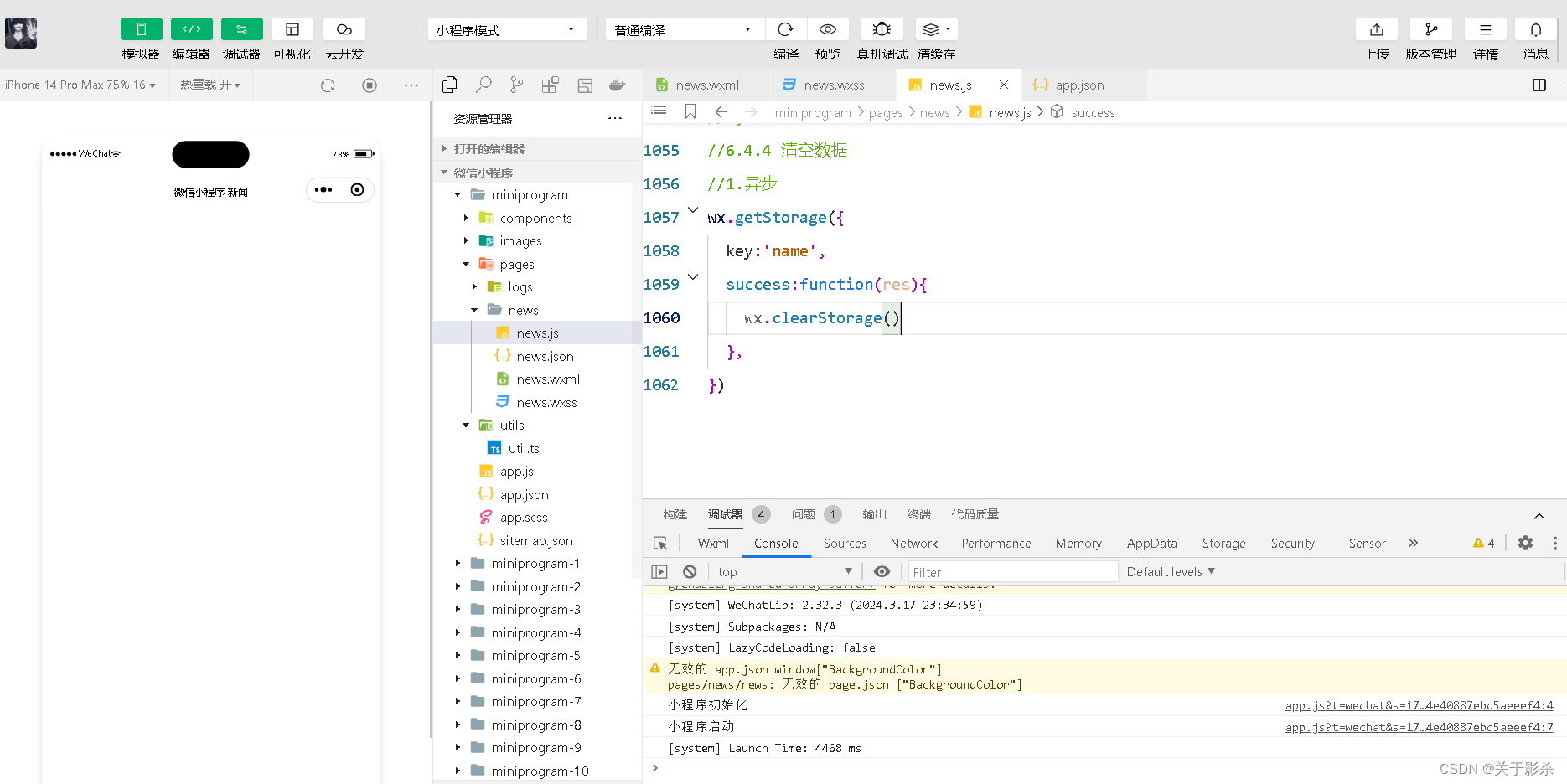
2.同步
js代码
try{
wx.clearStorageSync()
}catch(e){
}
运行结果图
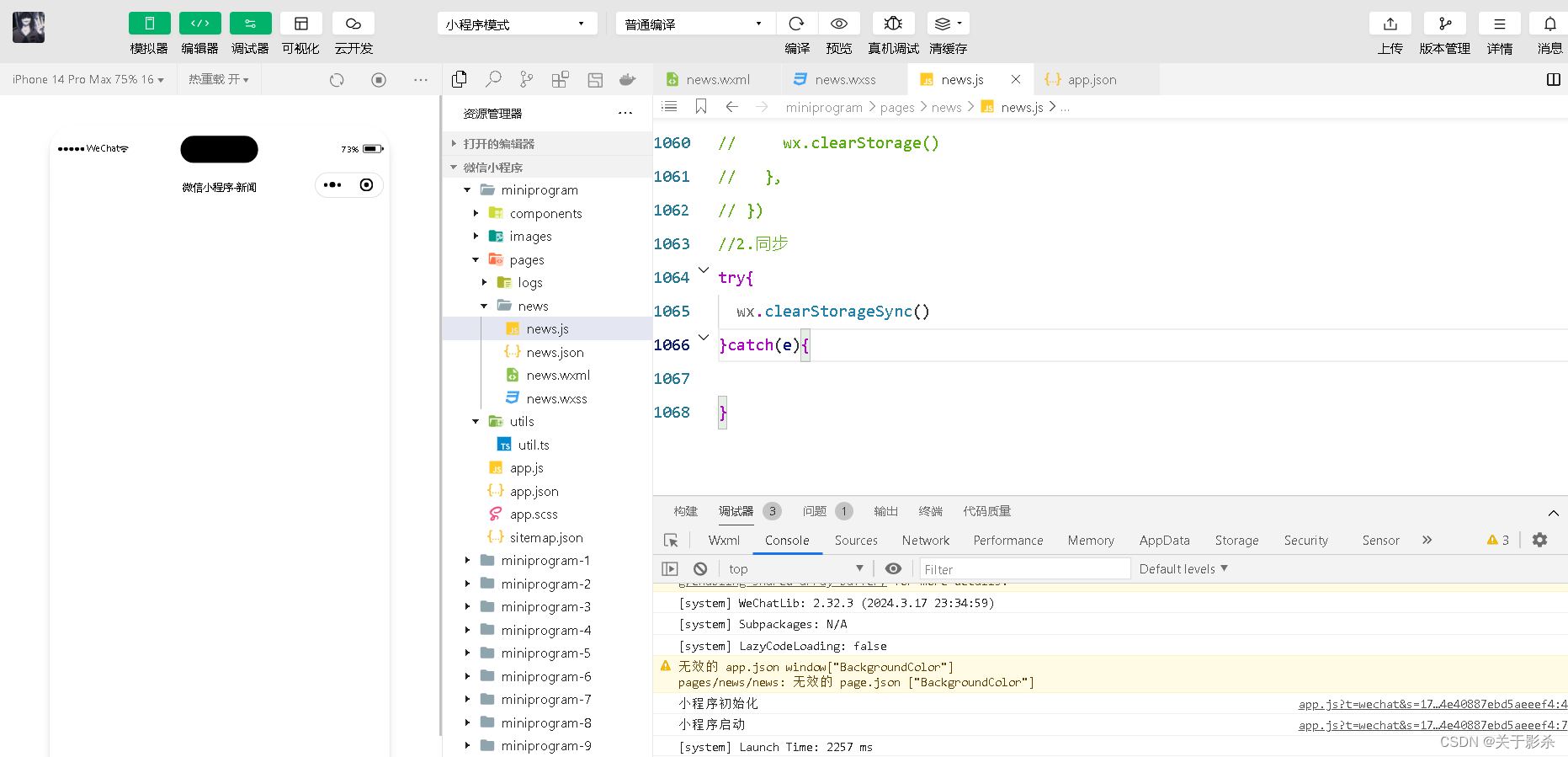
6.5 位置信息API
6.5.1 获取位置信息
属性
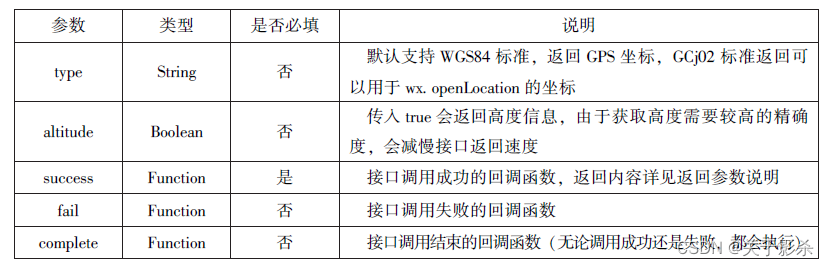
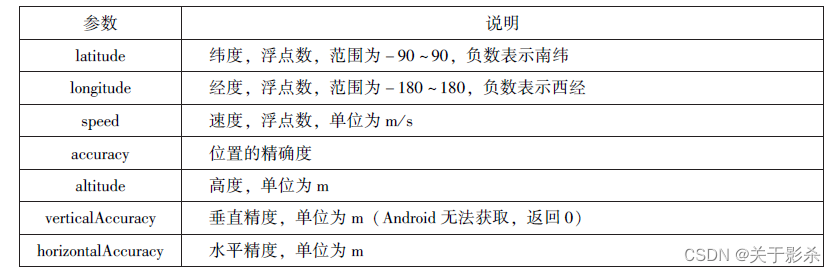
js代码
wx.getLocation({
type:'wgs84',
success:function(res){
console.log("经度:"+res.longitude);
console.log("纬度:"+res.latitude);
console.log("速度:"+res.speed);
console.log("位置的精确度:"+res.accuracy);
console.log("水平的精确度:"+res.horizontalAccuracy);
console.log("垂直的精确度:"+res.verticalAccuracy);
}
})
运行结果图
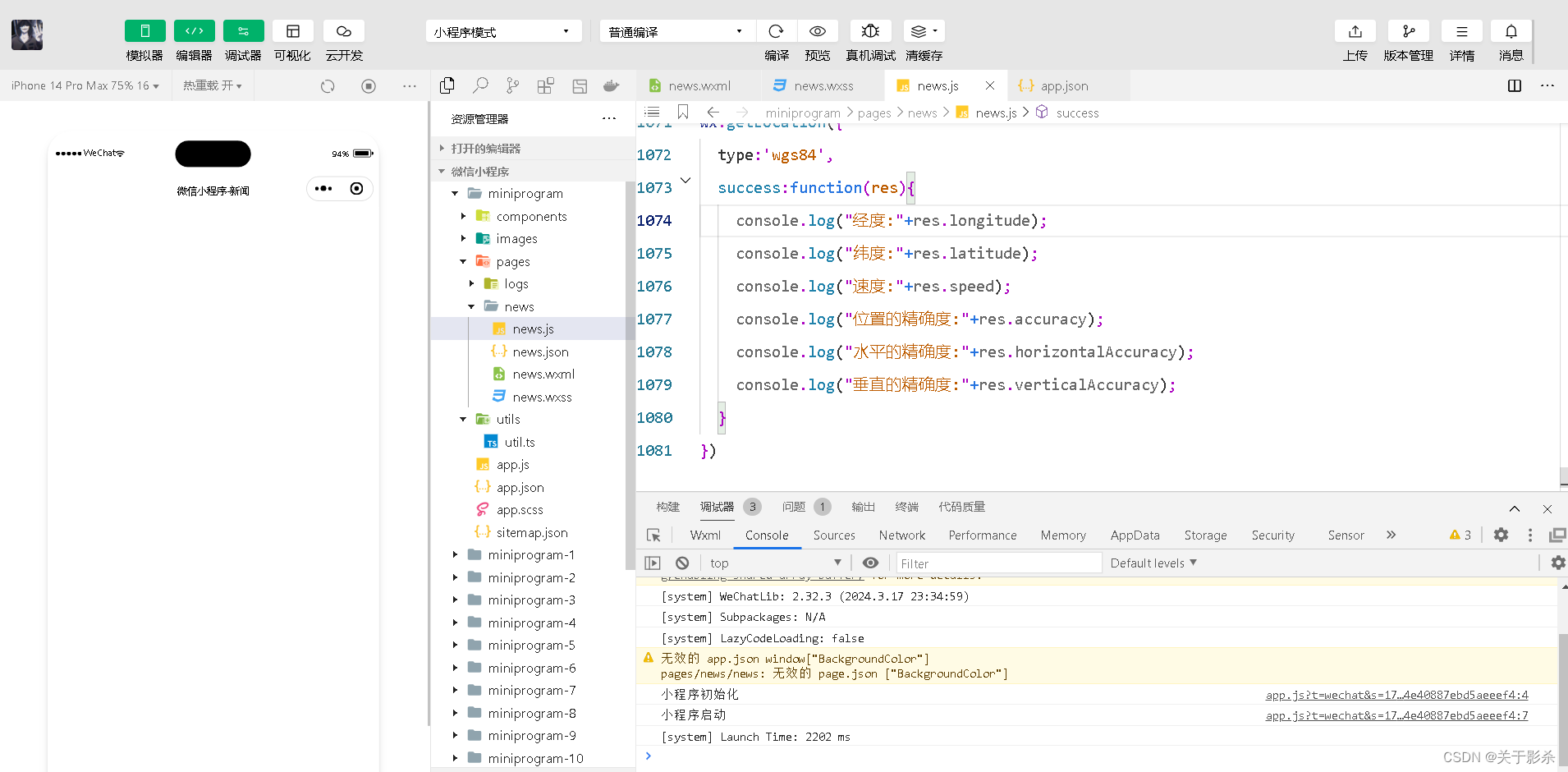
6.5.2 选择位置信息
属性

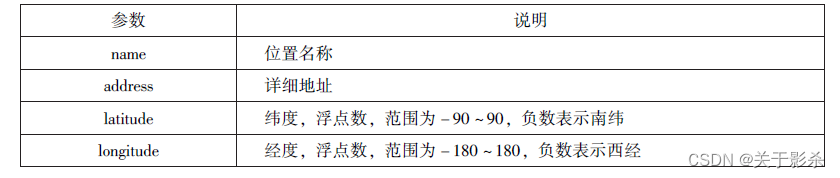
js代码
wx.chooseLocation({
success:function(res){
console.log("位置的名称:"+res.name);
console.log("位置的地址:"+res.address);
console.log("位置的经度:"+res.longitude);
console.log("位置的纬度:"+res.latitude);
}
})
运行结果图
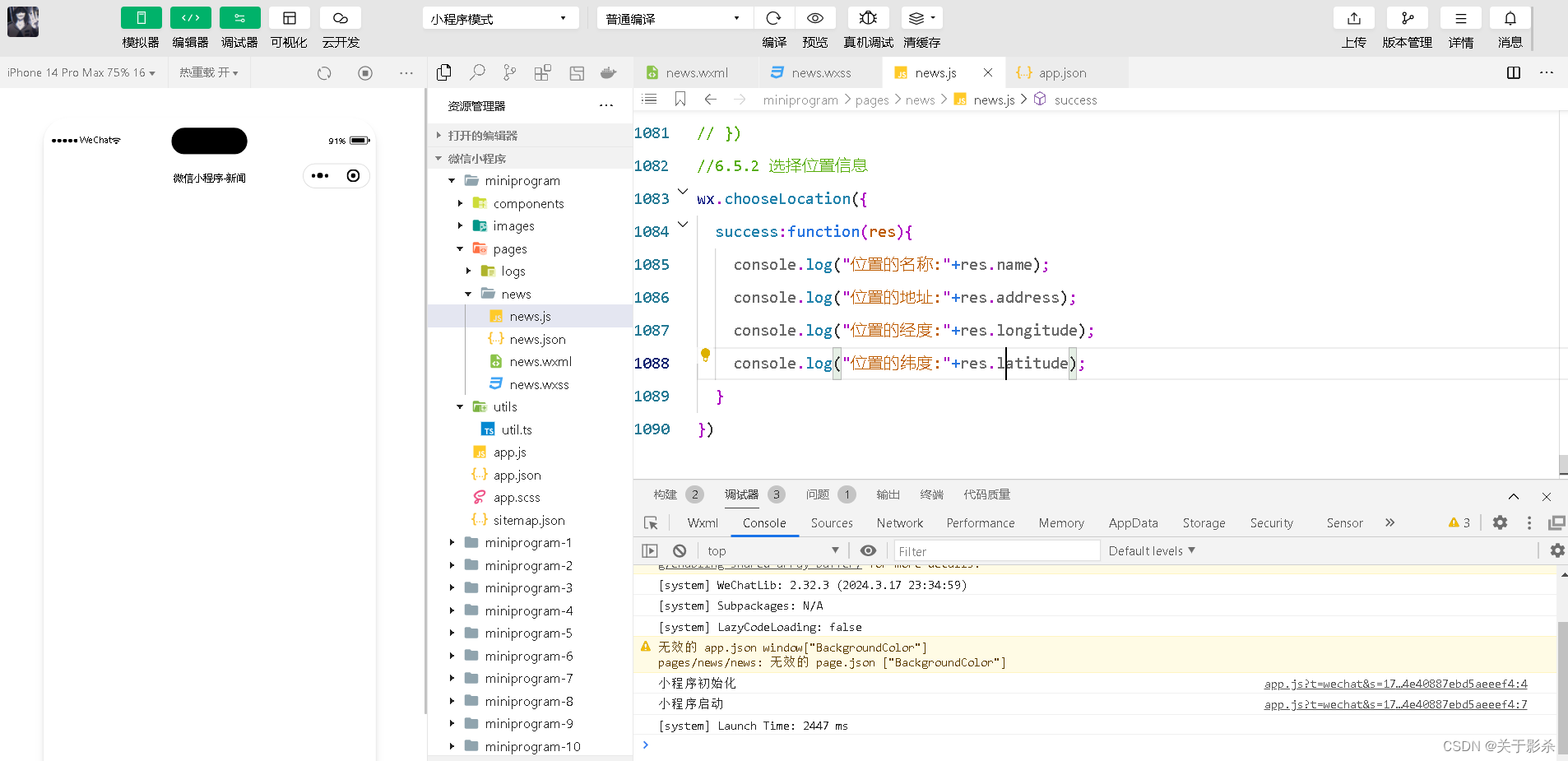
6.5.3 显示位置信息
属性
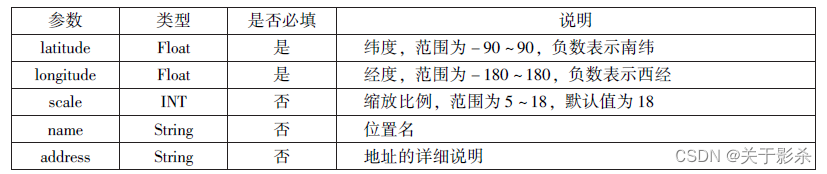

js代码
wx.getLocation({
type:'gcj02',
success:function(res){
var latitude=res.latitude
var longitude=res.longitude
wx.openLocation({
latitude:latitude,
longitude:longitude,
scale:10,
name:'智慧国际酒店',
address:'西安市长安区西长安区300号'
})
}
})
运行结果图
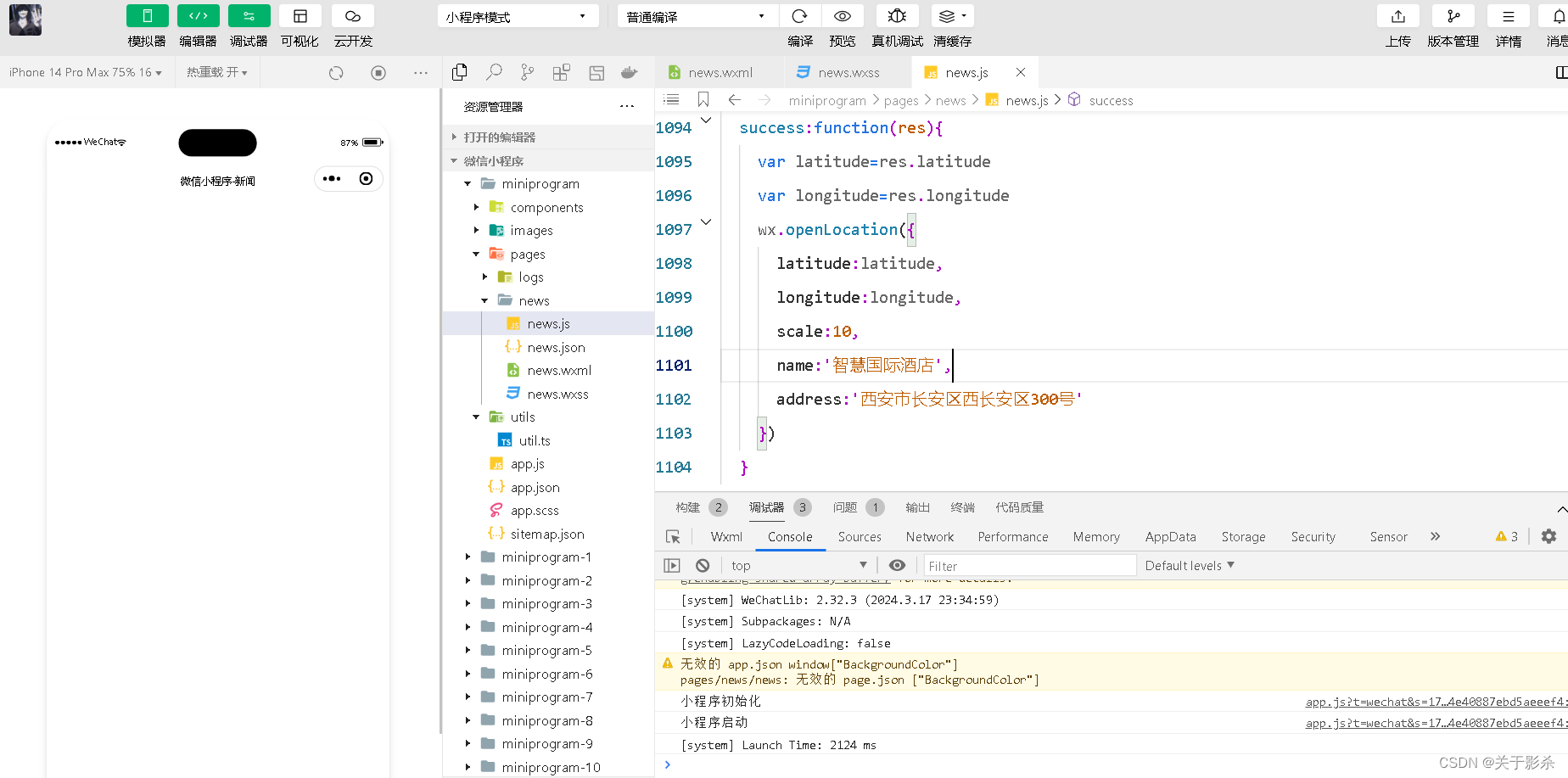
6.6 设备相关API
6.6.1 获取系统信息
属性
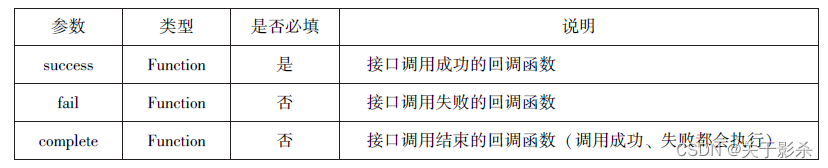
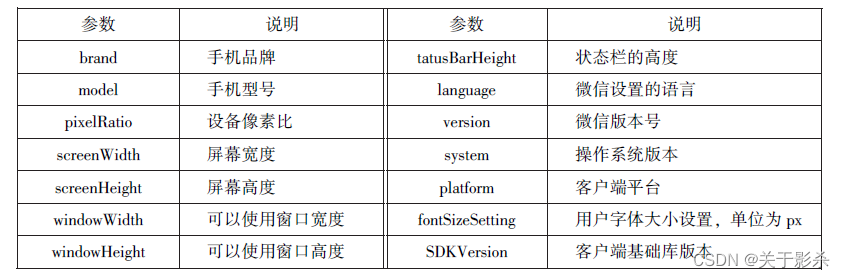
js代码
wx.getSystemInfo({
success:function(res){
console.log("手机型号:"+res.model)
console.log("设备像素比:"+res.pixelRatio)
console.log("窗口的宽度:"+res.windowWidth)
console.log("窗口的高度:"+res.windowHeight)
console.log("微信的版本号:"+res.version)
console.log("操作系统版本:"+res.system)
console.log("客户端平台:"+res.platform)
}
})
运行结果图
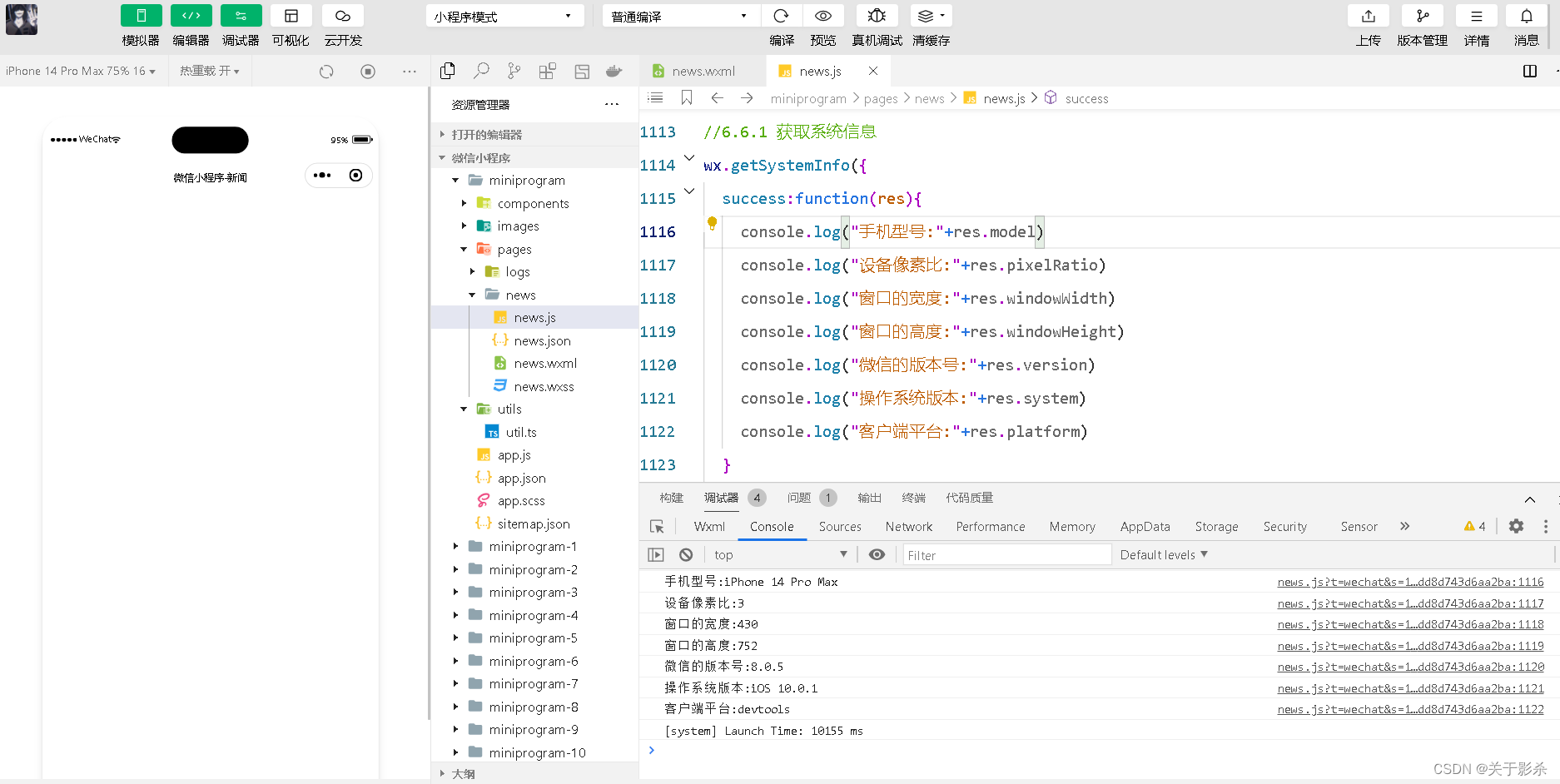
6.6.2 网络状态
属性

6.6.2.1 获取网络状态
js代码
wx.getNetworkType({
success:function (res) {
console.log(res.networkType)
},
})
运行结果图
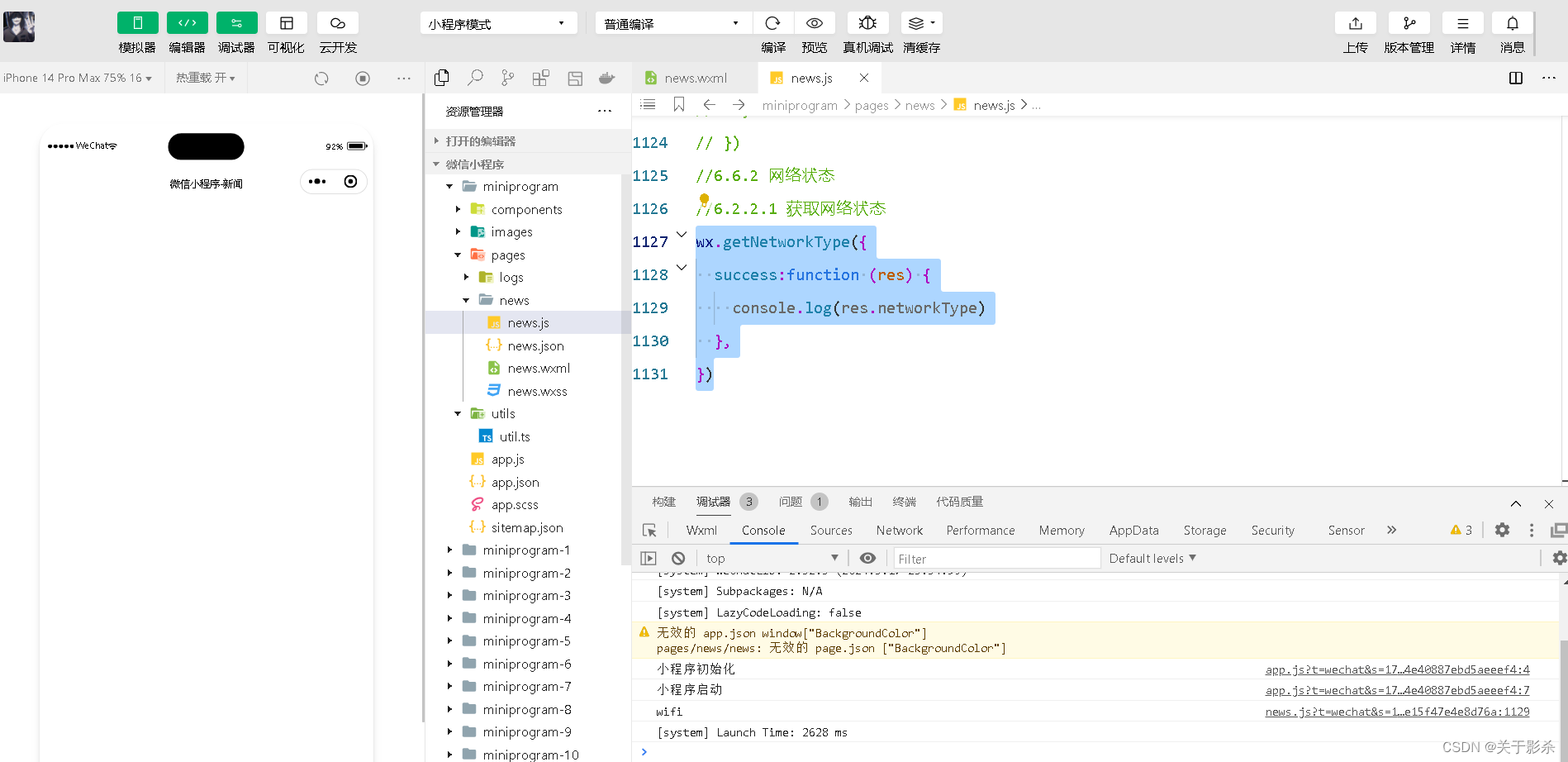
6.6.2.2 监听网络状态变化
js代码
wx.onNetworkStatusChange(function (res) {
console.log("网络是否连接:"+res.isConnected)
console.log("变化后的网络类型:"+res.networkType)
})
运行结果图
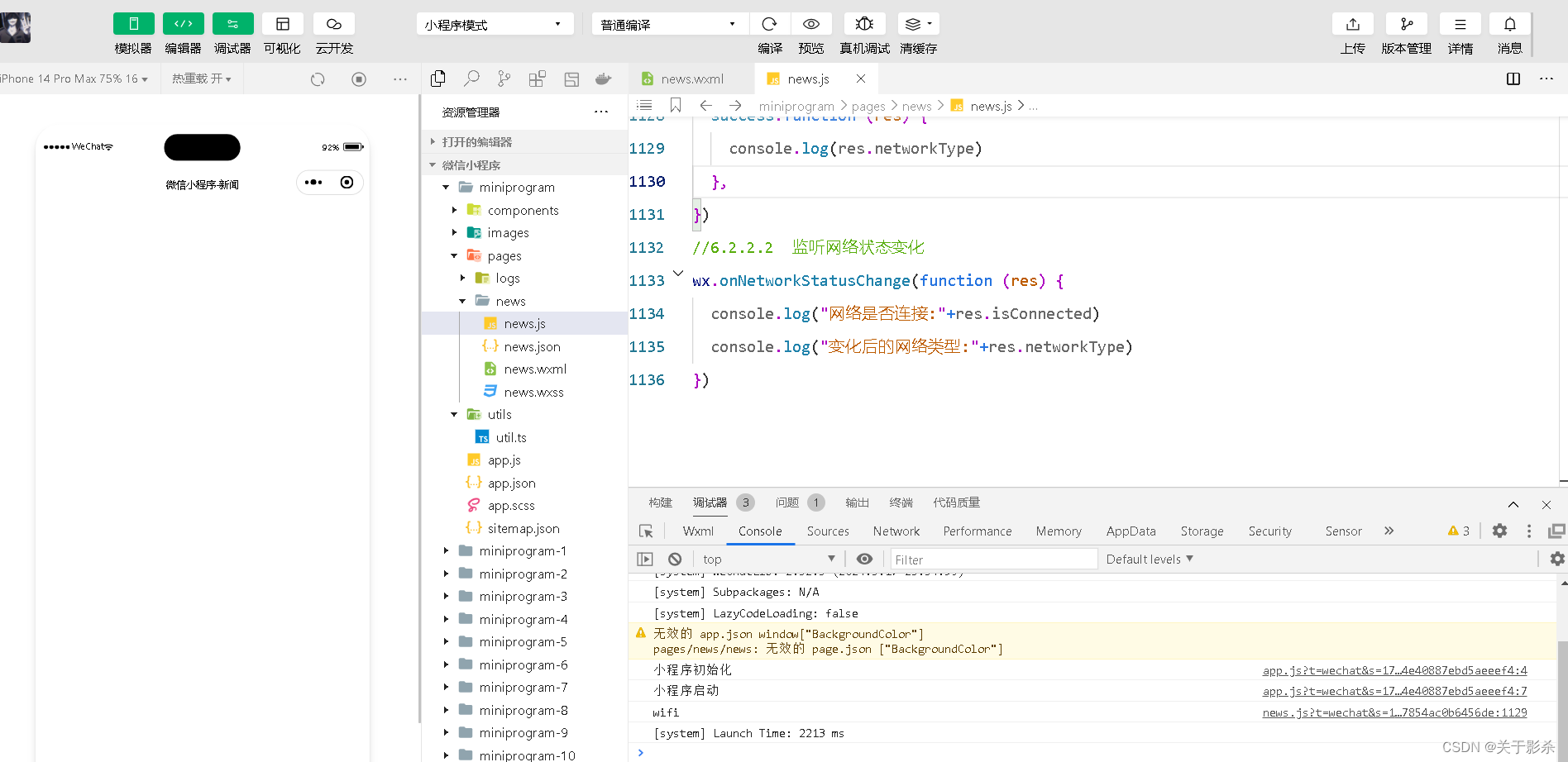
6.6.3 拨打电话
属性
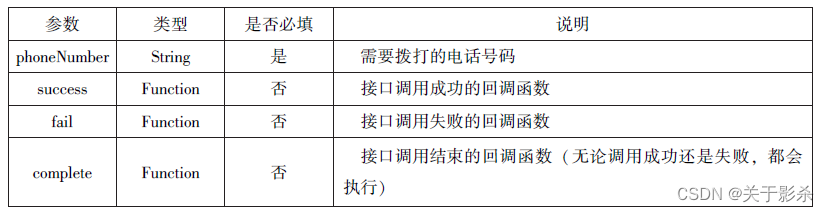
js代码
wx.makePhoneCall({
phoneNumber: '18092585093',
})
运行结果图
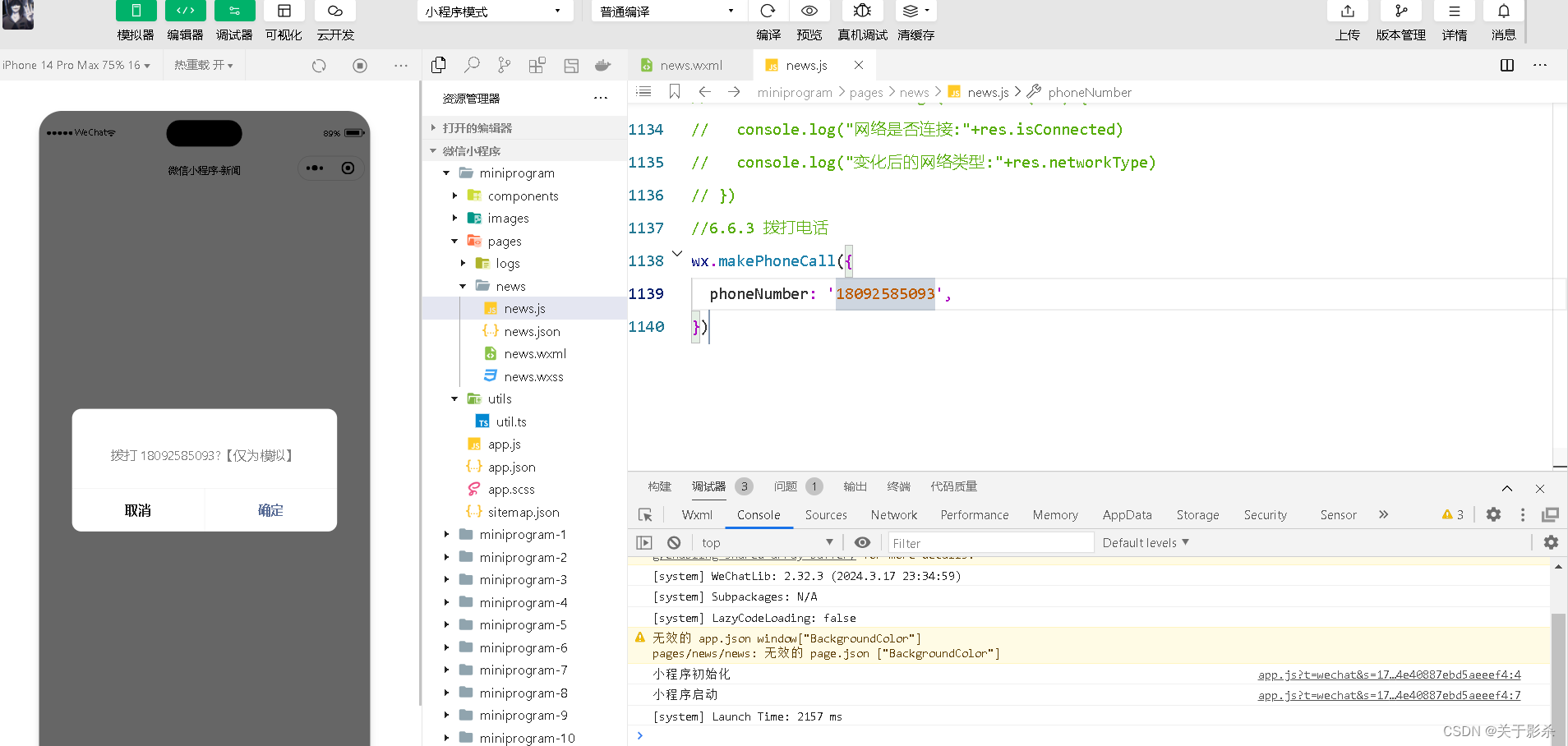
6.6.4 扫描二维码
属性
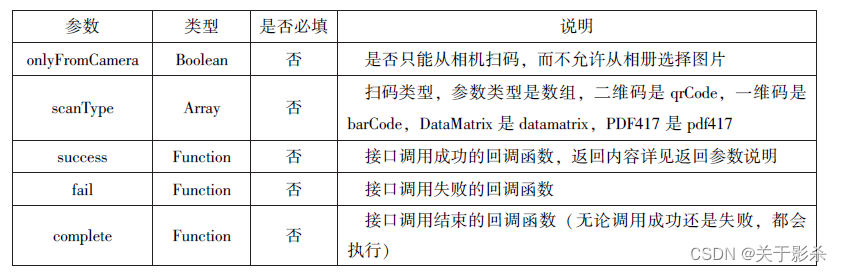
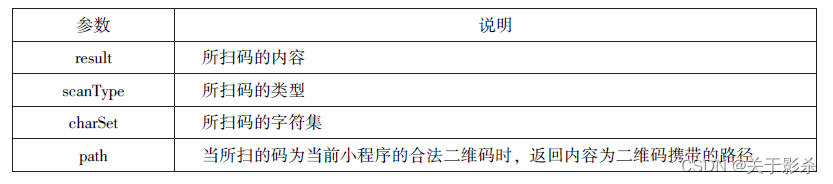
6.6.4.1 允许从相机和相册扫码
js代码
wx.scanCode({
success:(res)=> {
console.log(res.result)
console.log(res.scanType)
console.log(res.charSet)
console.log(res.path)
}
})
运行结果图
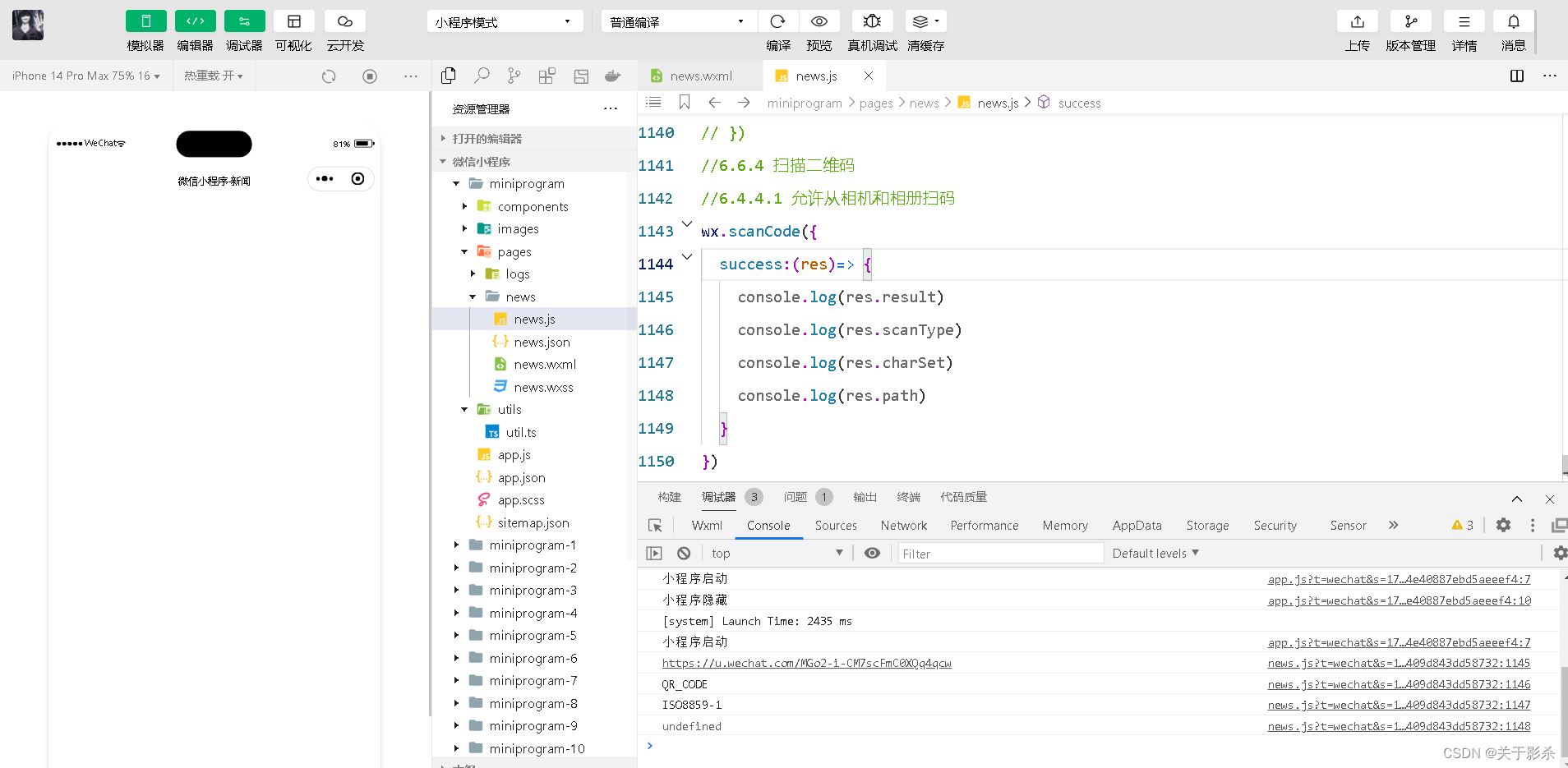
6.6.4.2 只允许从相机扫码
js代码
wx.scanCode({
onlyFromCamera:true,
success:(res)=>{
console.log(res)
}
})
运行结果图
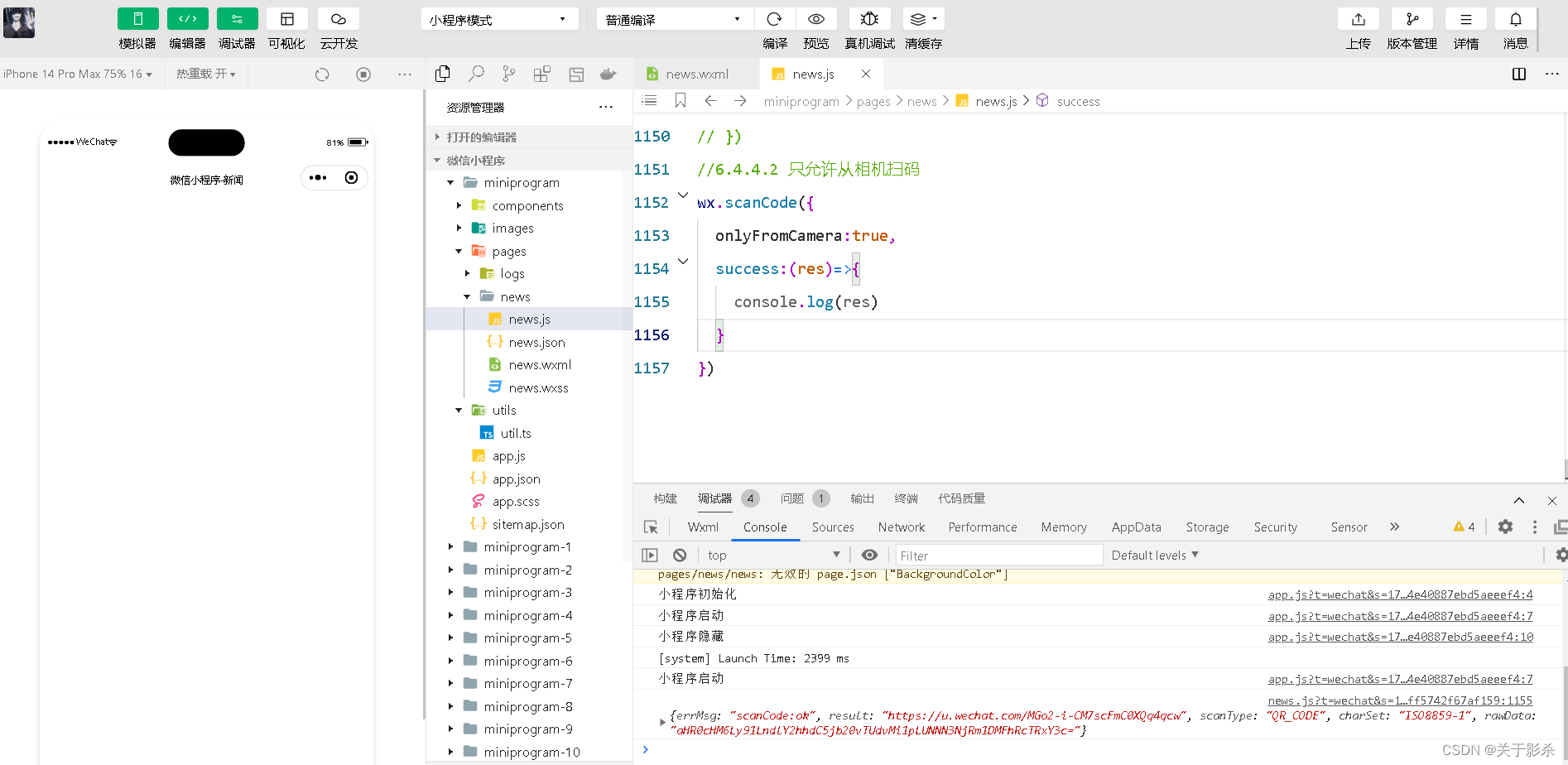