散知识
typeid() :看数据是什么类型
主要针对c++泛型编程和STL技术
模板
模板就是建立通用的模具,大大提高复用性
c++提供两种模板机制:函数模板和类模板
函数模板
作用:建立一个通用函数,其返回值类型和形参类型可以不具体制定,用一个虚拟的类型来代表
语法:
template
函数声明或定义
解释:
template—声明创建模板
typename----表明其后面的符号是一种数据类型,可以用class代替
T—通用的数据类型,名称可以替换,通常为大写字母
两种方式使用函数模板
1、自动类型推导
mySwap(a,b);
2、显示指定类型
mySwap<int>(a,b);
函数模板注意事项:
1、自动类型推导,必须推导出一致的数据类型T,才可以使用
2、模板必须要确定出T的数据类型,才可以使用
函数模板代码示例
#include<iostream>
using namespace std;
template<typename T>
void mySort(T array[], int len)
{
for (int i = 0; i < len; i++)
{
int min = i;
for (int j = i + 1; j < len; j++)
{
if (array[min] > array[j])
{
min = j;
}
}
if (min != i)
{
int temp;
temp = array[min];
array[min] = array[i];
array[i] = temp;
}
}
for (int i = 0; i < len; i++)
{
cout << array[i];
}
cout << endl;
}
void test01()
{
char p1[] = "acbfgsd";
int len = sizeof(p1) / sizeof(p1[0]);
mySort(p1, len);
}
void test02()
{
int p2[] = {3,6,9,5,7,1,2,4,8};
int len = sizeof(p2) / sizeof(p2[0]);
mySort(p2, len);
}
int main()
{
test01();
test02();
return 0;
}
普通函数和函数模板的区别
1、普通函数调用时可以发生自动类型转换(隐式类型转换)
2、函数模板调用时,如果利用自动类型推导,不会发生隐式类型转换
3、如果利用显示指定类型的方式,可以发生隐式类型转换
建议使用显示指定类型的方式,调用函数模板,因为可以自己确定通用类型T
普通函数和函数模板的调用规则
1、如果函数模板和普通函数都可以实现,优先调用普通函数
2、可以通过空模板参数列表来强制调用函数模板
myPrint<>(a,b);
3、函数模板也可以发生重载
4、如果函数模板可以产生更好的匹配,优先调用函数模板
模板的局限性
c++为了解决这种局限性,提供模板的重载,可以为特定的类型提供具体化的模板
代码示例
#include<iostream>
using namespace std;
#include<string>
class Person
{
public:
Person(int age, string name)
{
this->m_age = age;
this->m_name = name;
}
int m_age;
string m_name;
};
template<typename T>
bool MyCompare(T a, T b)
{
if (a == b)
return true;
else
return false;
}
template<>bool MyCompare(Person p1, Person p2)//具体化模板
{
if (p1.m_age == p2.m_age && p1.m_name == p2.m_name)
return true;
else
return false;
}
void test01()
{
int a = 8;
//int a=7;
int b = 8;
int ret = MyCompare(a, b);
if (ret)
cout << "a和b相等" << endl;
else
cout << "a和b不相等" << endl;
}
void test02()
{
//Person p1(33,"tom");
Person p1(23, "Tom");
Person p2(23, "Tom");
int ret = MyCompare(p1, p2);
if (ret)
cout << "p1和p2相等" << endl;
else
cout << "p1和p2不相等" << endl;
}
int main()
{
test01();
test02();
return 0;
}
1、利用具体化的模板,可以解决自定义类型的通用化
2、学习模板并不是为了写模板,而是在STL能够运用系统提供的模板
类模板
作用:建立一个通用类,类中的成员数据类型可以不具体制定,用一个虚拟的类型来代表
语法:
template<typename T>
类
类模板与函数模板的区别
1、类模板没有自动类型推导的使用方式
2、类模板在模板参数列表中可以有默认参数
代码示例
#include<iostream>
using namespace std;
#include<string>
template<typename T1,typename T2=int>
class Person
{
public:
Person(T1 name, T2 age)
{
this->m_age = age;
this->m_name = name;
}
public:
int m_age;
string m_name;
};
void test01()
{
Person<string, int> p2("Jim", 44);
Person<string> p1("Tom", 23);//使用了默认参数可以不用再写int
}
int main()
{
return 0;
}
类模板中成员函数创建时机
类模板中成员函数和普通类中成员函数创建时机是有区别的
1、普通类中的成员函数一开始就可以创建
2、类模板中的成员函数在调用时才创建
类模板对象做函数参数
目的:类模板实例化的对象,向函数传参的方式
三种传入方式:
1、指定传入的类型-----直接显示对象的数据类型
2、参数模板化------将对象中的参数变为模板进行传递
3、整个类模板化------将这个对象类型模板化进行传递
代码示例:
#include<iostream>
using namespace std;
#include<string>
template<class T1,class T2>
class Person
{
public:
Person(T1 name,T2 age)
{
this->m_name = name;
this->m_age = age;
}
T1 m_name;
T2 m_age;
};
void printPerson1(Person<string, int>&p1)//直接指定传入的类型
{
cout << "姓名:" << p1.m_name << "\t年龄:" << p1.m_age << endl;
}
template<typename T1,typename T2>//将参数变为模板进行传递
void printPerson2(Person<T1, T2>& p1)
{
cout << "姓名:" << p1.m_name << "\t年龄:" << p1.m_age << endl;
}
template<typename T>//将整个类模板化进行传递
void printPerson3(T& p1)
{
cout << "姓名:" << p1.m_name << "\t年龄:" << p1.m_age << endl;
cout << "T的类型是:" << typeid(T).name() << endl;//用typeid可以查看数据类型
}
void test01()
{
Person<string, int>p1("Tom", 34);
printPerson1(p1);
}
void test02()
{
Person<string, int>p1("Jim", 94);
printPerson2(p1);
}
void test03()
{
Person<string, int>p1("Stem", 76);
printPerson3(p1);
}
int main()
{
test01();
test02();
test03();
return 0;
}
类模板与继承
注意点:
1、当子类继承的父类是一个类模板时,子类在声明的时候,要指定出父类中T的类型
2、如果不指定,编译器无法给子类分配内存
3、如果想灵活指定出父类中T的类型,子类也需要变为类模板
代码示例
#include<iostream>
using namespace std;
template<typename T>
class Person
{
public:
T m_m;
};
class Son :public Person<int>
{
public:
int h;
};
template<typename T1,typename T2>
class Son2 :public Person<T2>
{
public:
T1 m_h;
};
void test01()
{
Son2<int, double>S1;
S1.m_h = 7;
S1.m_m = 4.6;
}
int main()
{
return 0;
}
类模板成员函数的类外实现
类模板分文件编写
问题:
类模板成员函数创建时机是在调用阶段,导致分文件编写时链接找不到
解决:
方法1、直接包含.cpp源文件
方法2、将声明和实现写在同一个文件中,并更改后缀名为.hpp,hpp是约定的名称,并不是强制
类模板和友元
全局函数类内实现:直接在类内声明友元即可
全局函数类外实现:需要提前让编译器知道全局函数的存在
用类模板实现数组类封装案例
MyArray.hpp
#pragma once
#include<iostream>
using namespace std;
#include<string>
template<class T>
class MyArray
{
public:
MyArray(int capacity)
{
//cout << "调用有参构造函数" << endl;
this->m_capacity = capacity;
this->pAddress = new T[m_capacity];
this->m_size = 0;
}
MyArray(const MyArray& arr)
{
//cout << "调用拷贝构造函数" << endl;
this->m_capacity = arr.m_capacity;
this->m_size = arr.m_size;
this->pAddress = new T[this->m_capacity];
for (int i = 0; i < this->m_capacity; i++)
{
this->pAddress[i] = arr.pAddress[i];
}
}
MyArray& operator=(const MyArray& arr)
{
if (this->pAddress != NULL)
{
// << "调用=运算符重载" << endl;
delete[] pAddress;
this->m_size = 0;
this->m_capacity = 0;
}
this->m_size = arr.m_size;
this->m_capacity = arr.m_capacity;
this->pAddress = new T[this.m_capacity];
for (int i = 0; i < this->m_size; i++)
{
this->pAddress[i] = arr[i];
}
return *this;
}
//尾插法插入数据
void Push_Back(const T& val)
{
if (this->m_capacity == this->m_size)
return;
this->pAddress[this->m_size] = val;//在数组尾部插入数据
this->m_size++;//更新数组大小
}
//尾删法
void Pop_Back()
{
if (this->m_size == 0)
return;
this->m_size--;
}
//通过下标获取数组中的元素
T& operator[](int index)
{
return this->pAddress[index];
}
//返回数组容量
int GetCapacity()
{
return this->m_capacity;
}
//返回数组大小
int GetSize()
{
return this->m_size;
}
~MyArray()
{
if (pAddress != NULL)
{
//cout << "调用析构函数" << endl;
delete[] pAddress;
pAddress = NULL;
this->m_size = 0;
this->m_capacity = 0;
}
}
private:
int m_size;//数组大小
int m_capacity;//数组容量
T* pAddress;//指针指向堆区开辟的真实数据
};
main.cpp
#include<iostream>
using namespace std;
#include"MyArray.hpp"
#include<string>
void test01()
{
MyArray<int> arr(10);
/*MyArray<int>Arr1(arr);
MyArray<int> arr2(100);
arr2 = arr;*/
for (int i = 0; i < 10; i++)
{
arr.Push_Back(i);
}
cout << arr.GetSize() << endl;
cout << arr.GetCapacity() << endl;
cout << arr[6] << endl;
arr.Pop_Back();
cout << arr.GetSize() << endl;
}
//测试自定义类型
class Person
{
public:
Person() :m_age(0),m_name(" "){}
Person(string name, int age)
{
this->m_age = age;
this->m_name = name;
}
string m_name;
int m_age;
};
void printPerson(MyArray<Person>& arr)
{
for (int i = 0; i < arr.GetSize(); i++)
{
cout << "姓名:" << arr[i].m_name << "\t年龄:" << arr[i].m_age << endl;
}
}
void test02()
{
MyArray<Person>arr(10);
Person p1("孙悟空", 33);
Person p2("李白", 45);
Person p3("安其拉", 67);
arr.Push_Back(p1);
arr.Push_Back(p2);
arr.Push_Back(p3);
printPerson(arr);
cout << "容量为:" << arr.GetCapacity() << endl;
cout << "大小为:" << arr.GetSize() << endl;
}
int main()
{
//test01();
test02();
return 0;
}
STL
为了提高代码的复用性而诞生
STL基本概念
1、STL(standard template library,标准模板库)
2、STL从广义上分:容器(container)算法(algorithm)迭代器(iterator)
3、容器和算法直接通过迭代器进行无缝连接
4、STL几乎所有的代码都采用了模板类或者模板函数
STL六大组件
常用的容器中迭代器种类为双向迭代器和随机访问迭代器
容器算法迭代器初识
vector存放内置数据类型
容器:vector
算法:for_each
迭代器:vector::iterator
#include<iostream>
using namespace std;
#include<vector>
#include<algorithm>
void Print(int cal)
{
cout << cal << endl;
}
void test01()
{
//创建vector类型的容器变量
vector<int> v;
v.push_back(10);
v.push_back(20);
v.push_back(30);
v.push_back(40);
//通过迭代器访问容器中的数据
vector<int>::iterator itBegin = v.begin();//起始迭代器,指向容器中第一个元素
vector<int>::iterator itEnd = v.end();//结束迭代器,指向容器中最后一个元素的下一个位置
//第一种遍历方式
/*while (itBegin != itEnd)
{
cout << *itBegin << endl;
itBegin++;
}*/
//第二种遍历方式
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
{
cout << *it << endl;
}
//第三种遍历方式
//for_each(v.begin(), v.end(),Print);
}
int main()
{
test01();
return 0;
}
vector存放自定义数据类型
#include<iostream>
using namespace std;
#include<string>
#include<vector>
class Person
{
public:
Person(string name, int age)
{
this->m_age = age;
this->m_name = name;
}
string m_name;
int m_age;
};
void test01()
{
vector<Person> v;
//存放自定义数据类型
Person p1("aaa", 33);
Person p2("aa4", 83);
Person p3("aa5", 73);
Person p4("aad", 53);
Person p5("aah", 39);
v.push_back(p1);
v.push_back(p2);
v.push_back(p3);
v.push_back(p4);
v.push_back(p5);
for (vector<Person>::iterator it = v.begin(); it != v.end(); it++)
{
cout << "姓名:" << (*it).m_name << "\t年龄:" << (*it).m_age << endl;
//cout << "姓名:" << it->m_name << "\t年龄:" << it->m_age << endl;
}
}
void test02()
{
vector<Person*> v;
//存放自定义数据类型 指针
Person p1("aaa", 33);
Person p2("aa4", 83);
Person p3("aa5", 73);
Person p4("aad", 53);
Person p5("aah", 39);
v.push_back(&p1);
v.push_back(&p2);
v.push_back(&p3);
v.push_back(&p4);
v.push_back(&p5);
for (vector<Person*>::iterator it = v.begin(); it != v.end(); it++)
{
cout << "姓名:" << (*it)->m_name << "\t年龄:" << (*it)->m_age << endl;
}
}
int main()
{
test01();
test02();
return 0;
}
vector容器嵌套容器
#include<iostream>
using namespace std;
#include<vector>
void test01()
{
vector<vector<int>> v;
vector<int> v1;
vector<int> v2;
vector<int> v3;
vector<int> v4;
vector<int> v5;
for (int i = 0; i < 5; i++)
{
v1.push_back(i + 1);
v2.push_back(i + 2);
v3.push_back(i + 3);
v4.push_back(i + 4);
v5.push_back(i + 5);
}
v.push_back(v1);
v.push_back(v2);
v.push_back(v3);
v.push_back(v4);
v.push_back(v5);
for (vector<vector<int>>::iterator it = v.begin(); it != v.end(); it++)
{
//(*it)----容器vector<int>----看尖括号是啥,解引用后就是啥
for (vector<int>::iterator vit = (*it).begin(); vit != (*it).end(); vit++)
{
cout << (*vit) << " ";
}
cout << endl;
}
}
int main()
{
test01();
return 0;
}
string容器
本质:
string 是c++风格的字符串,而string本质上是一个类
string和char区别
1、char是一个指针
2、string是一个类,内部封装了char*,管理这个字符串,是一个char*型的容器
特点:
1、string类内部封装了很多成员方法
2、string管理char*所分配的内存,不用担心复制越界和取值越界等,由类内部进行负责
string构造函数
string赋值操作
string字符串拼接
string查找和替换
string字符串比较
string字符串存取
string字符串插入和删除
string子串获取
string容器代码示例
#include<iostream>
using namespace std;
#include<string>
void test01()//构造,赋值
{
string str;
string str1("hello world");
string str2(str1);
string str3(10, 'c');
cout << str1 << endl;
cout << str2 << endl;
cout << str3 << endl;
const char* ch = "hello kitty";
str = ch;
string str4;
str4 = str1;
string str5;
str5 = 'v';
string str6;
str6.assign(str5);
string str7;
str7.assign(ch, 5);
string str8;
str8.assign(ch);
string str9;
str9.assign(6, 'p');
cout << str4 << endl;
cout << str5 << endl;
cout << str6 << endl;
cout << str7 << endl;
cout << str8 << endl;
cout << str9 << endl;
}
void test02()//拼接
{
string str = "hello world";
str += "!";
cout << str << endl;
string str1 = "girl";
str += str1;
cout << str << endl;
string str2;
const char* ch = "hello world";
str2.append(ch, 2);
cout << str2 << endl;
str2.append(str, 2, 3);
cout << str2 << endl;
}
void test03()//查找和替换
{
string str = "hello world hew";
int pos = str.find('w');
cout << pos << endl;
int rpos = str.rfind('w');
cout << rpos << endl;
string str2 = str.replace(2, 3, "999999");
cout << str2 << endl;
string str3 = "jjjjj";
str2 = str2.replace(2, 6, str3);
cout << str2 << endl;
}
void test04()//比较
{
string str("hello orld");
string str1("hello world");
int pos = str.compare(str1);
cout << "pos=" << pos << endl;
}
void test05()//获取单个字符
{
string str = "hello world";
str[1] = 'x';
str.at(2) = 'L';
cout << str << endl;
}
void test06()//插入和删除
{
string str = "hello world";
str.insert(2, "pptr");
str.insert(2, 6, 'm');
cout << str << endl;
str.erase(7, 9);
cout << str << endl;
}
void test07()//子串获取
{
string str = "xiaoming@qq.com";
int pos = str.find('@');
string str1 = str.substr(0, pos);
cout << str1 << endl;
}
int main()
{
test01();
test02();
test03();
test04();
test05();
test06();
test07();
return 0;
}
vector容器
功能:vector数据结构和数组非常相似,也称为单端数组
vector与普通数组区别:
不同之处在于数组是静态空间,而vector可以动态扩展
动态扩展:
不是在原空间之后继续接新空间,而是找更大的内存空间,然后将原数据拷贝新空间,释放原空间
vector构造函数
vector赋值操作
vector容量和大小
vector插入和删除
vector数据存取
vector互换容器
vector预留空间
vector容器代码
#include<iostream>
using namespace std;
#include<vector>
void printVector(vector<int>& v)
{
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
{
cout << (*it) << " ";
}
cout << endl;
}
void test01()//构造函数
{
vector<int>v1;
for (int i = 0; i < 10; i++)
{
v1.push_back(i);
}
printVector(v1);
vector<int>v2(v1.begin(), v1.end());
printVector(v2);
vector<int>v3(10, 100);
printVector(v3);
vector<int>v4(v3);
printVector(v4);
}
void test02()//赋值
{
vector<int>v1;
for (int i = 0; i < 10; i++)
{
v1.push_back(i);
}
printVector(v1);
vector<int>v2;
v2 = v1;
printVector(v2);
vector<int>v3;
v3.assign(v2.begin(), v2.end());
printVector(v3);
vector<int>v4;
v4.assign(10, 100);
printVector(v4);
}
void test03()//容量和大小
{
vector<int>v1;
for (int i = 0; i < 10; i++)
{
v1.push_back(i);
}
printVector(v1);
if (v1.empty())
{
cout << "v1为空!" << endl;
}
else
{
cout << "v1不为空!" << endl;
cout << "v1的容量为: " << v1.capacity() << endl;
cout << "v1的大小为: " << v1.size() << endl;
}
v1.resize(17, 78);//可以指定大了后所填充的数
printVector(v1);
v1.resize(19);//重新指定的大小大了会默认用0填充
printVector(v1);
v1.resize(5);//重新指定的大小小了会截取前几个
printVector(v1);
}
void test04()//插入和删除
{
vector<int>v1;
v1.push_back(10);
v1.push_back(20);
v1.push_back(30);
v1.push_back(40);
v1.push_back(50);
printVector(v1);
v1.pop_back();
printVector(v1);
v1.insert(v1.begin() + 1, 5);
printVector(v1);
v1.insert(v1.begin(), 2, 1000);
printVector(v1);
v1.erase(v1.begin()+3, v1.end());
printVector(v1);
v1.clear();
printVector(v1);
}
void test05()//数据存取
{
vector<int>v1;
for (int i = 0; i < 10; i++)
{
v1.push_back(i);
}
for (int i = 0; i < v1.size(); i++)
{
cout << v1[i] << " ";
}
cout << endl;
for (int i = 0; i < v1.size(); i++)
{
cout << v1.at(i) << " ";
}
cout << endl;
cout << "v1的第一个元素是:" << v1.front() << endl;
cout << "v1的最后一个元素是:" << v1.back() << endl;
}
void test06()//互换
{
vector<int> v1;
for (int i = 0; i < 10; i++)
{
v1.push_back(i);
}
printVector(v1);
vector<int> v2;
for (int i = 10; i > 0; i--)
{
v2.push_back(i);
}
printVector(v2);
cout << "交换后:" << endl;
v1.swap(v2);
printVector(v1);
printVector(v2);
vector<int>v3;
for (int i = 0; i < 100000; i++)
{
v3.push_back(i);
}
cout << "v3的容量是:" << v3.capacity() << endl;
cout << "v3的大小是:" << v3.size() << endl;
v3.resize(6);
cout << "v3的容量是:" << v3.capacity() << endl;
cout << "v3的大小是:" << v3.size() << endl;
vector<int>(v3).swap(v3);//巧用swap收缩内存
cout << "v3的容量是:" << v3.capacity() << endl;
cout << "v3的大小是:" << v3.size() << endl;
}
void test07()
{
vector<int>v1;
v1.reserve(10000);//加上这一句num从24变为1
int num = 0;
int* p = NULL;
for (int i = 0; i < 10000; i++)
{
v1.push_back(i);
if (p != &v1[0])
{
p = &v1[0];
num++;
}
}
cout << "num=" << num << endl;
}
int main()
{
test01();
test02();
test03();
test04();
test05();
test06();
test07();
return 0;
}
deque容器
功能:
双端数组,可以对头端进行插入删除操作
deque构造函数
deque赋值操作
deque大小操作
deque插入删除
插入和删除提供的位置是迭代器
deque数据存取
deque排序操作
对于支持随机访问的迭代器的容器,都可以使用sort算法直接对其进行排序
deque容器代码
#include<iostream>
using namespace std;
#include<deque>
#include<algorithm>
void printDeque(const deque<int>& d)//只读
{
for (deque<int>::const_iterator it = d.begin(); it != d.end(); it++)
{
cout << *it << " ";
}
cout << endl;
}
void test01()//构造
{
deque<int>d1;
for (int i = 0; i < 10; i++)
{
d1.push_back(i);
}
printDeque(d1);
deque<int>d2(d1.begin(), d1.end());
printDeque(d2);
deque<int>d3(10, 100);
printDeque(d3);
deque<int>d4(d3);
printDeque(d4);
}
void test02()//赋值
{
deque<int>d1;
for (int i = 0; i < 10; i++)
{
d1.push_back(i);
}
deque<int>d2;
d2 = d1;
printDeque(d2);
deque<int>d3;
d3.assign(d2.begin(), d2.end());
printDeque(d3);
deque<int>d4;
d4.assign(10, 100);
printDeque(d4);
}
void test03()//大小
{
deque<int>d1;
for (int i = 0; i < 10; i++)
{
d1.push_back(i);
}
printDeque(d1);
if (d1.empty())
{
cout << "d1为空!" << endl;
}
else
{
cout << "d1不为空!" << endl;
cout << "d1的大小为:" << d1.size() << endl;
}
//d1.resize(15);
d1.resize(15, 2);
printDeque(d1);
d1.resize(4);
printDeque(d1);
}
void test04()//插入和删除
{
deque<int>d1;
d1.push_back(100);
d1.push_back(30);
d1.push_front(58);
d1.push_front(68);
printDeque(d1);
d1.pop_back();
d1.pop_front();
printDeque(d1);
deque<int>d2;
d2.push_back(100);
d2.push_back(90);
d2.push_back(80);
d2.insert(d2.begin() + 1, 78);
d2.insert(d2.begin(), 2, 777);
printDeque(d2);
deque<int>d3;
d3.push_back(8);
d3.push_back(7);
d3.push_back(6);
d2.insert(d2.begin(), d3.begin(), d3.end());
printDeque(d2);
d3.erase(d3.begin());
printDeque(d3);
d3.erase(d3.begin(), d3.end() - 1);
printDeque(d3);
d3.clear();
printDeque(d3);
}
void test05()//数据存取
{
deque<int>d1;
for (int i = 0; i < 5; i++)
{
d1.push_back(i);
}
for (int i = 0; i < d1.size(); i++)
{
cout << d1[i] << " ";
}
cout << endl;
for (int i = d1.size() - 1; i >= 0; i--)
{
cout << d1.at(i) << " ";
}
cout << endl;
cout << "第一个元素是:" << d1.front() << endl;
cout << "最后一个元素是:" << d1.back() << endl;
}
void test06()//排序
{
deque<int>d1;
d1.push_back(100);
d1.push_back(90);
d1.push_back(80);
d1.push_front(70);
d1.push_front(60);
d1.push_front(50);
printDeque(d1);
sort(d1.begin(), d1.end());
printDeque(d1);
for (int i = d1.size() - 1; i >= 0; i--)
{
cout << d1.at(i) << " ";
}
cout << endl;
}
int main()
{
//test01();
//test02();
//test03();
//test04();
//test05();
test06();
return 0;
}
容器案例—评委打分
#include<iostream>
using namespace std;
#include<string>
#include<deque>
#include<vector>
#include<algorithm>
#include<ctime>
class Person
{
public:
Person(string name, int score)
{
this->m_name = name;
this->m_score = score;
}
string m_name;//姓名
int m_score;//平均分
};
void creatPerson(vector<Person>& v)
{
string nameSeed = "ABCDE";
for (int i = 0; i < 5; i++)
{
string name = "选手";
name += nameSeed[i];
int score = 0;
Person p(name, score);
v.push_back(p);
}
}
void setScore(vector<Person>& v)
{
for (vector<Person>::iterator it = v.begin(); it != v.end(); it++)
{
deque<int>d;
for (int i = 0; i < 10; i++)
{
int score = rand() % 41 + 60;
d.push_back(score);
}
sort(d.begin(), d.end());
d.pop_back();
d.pop_front();
int num = 0;
for (deque<int>::iterator dit = d.begin(); dit != d.end(); dit++)
{
num += *dit;
}
(*it).m_score = num / d.size();
}
}
void printScore(vector<Person>&v)
{
for (vector<Person>::iterator it = v.begin(); it != v.end(); it++)
{
cout << "姓名:" << (*it).m_name << "\t分数:" << (*it).m_score << endl;
}
}
int main()
{
srand((unsigned int)time(NULL));
vector<Person> v;
creatPerson(v);
setScore(v);
printScore(v);
return 0;
}
stack容器
栈不允许有遍历
stack常用接口
stack容器代码
#include<iostream>
using namespace std;
#include<stack>
void test01()
{
stack<int>s;
s.push(10);
s.push(20);
s.push(30);
s.push(40);
cout << "元素个数为:" << s.size() << endl;
while (!s.empty())
{
cout << "栈顶元素为:" << s.top() << endl;
s.pop();
}
cout << "元素个数为:" << s.size() << endl;
}
int main()
{
test01();
return 0;
}
queue容器
queue常用接口
queue容器代码
#include<queue>
#include<iostream>
#include<string>
using namespace std;
class Person
{
public:
Person(string name, int age)
{
this->m_age = age;
this->m_name = name;
}
string m_name;
int m_age;
};
void test01()
{
queue<Person>q;
Person p1("李白",33);
Person p2("张飞", 55);
Person p3("杜甫", 78);
Person p4("韩信", 50);
Person p5("成吉思汗", 95);
q.push(p1);
q.push(p2);
q.push(p3);
q.push(p4);
q.push(p5);
cout << "q的大小为:" << q.size() << endl;
while (!q.empty())
{
cout << "队头元素----姓名:" << q.front().m_name << "\t年龄:" << q.front().m_age << endl;
cout << "队尾元素----姓名:" << q.back().m_name << "\t年龄:" << q.back().m_age << endl;
q.pop();
}
cout << "q的大小为:" << q.size() << endl;
}
int main()
{
test01();
return 0;
}
list容器
优点:
可以对任意位置进行快速插入和删除元素
缺点:
1、容器遍历速度没有数组快
2、占用空间比数组大
属于双向迭代器
List构造函数
List赋值和交换
list大小操作
list插入和删除
list数据存取
list本质链表,不是用连续线性空间存储数据,迭代器也是不支持随机访问的
list容器反转和排序
所有不支持随机访问迭代器的容器,不可以用标准算法
不支持随机访问迭代器的容器,内部会提供对应一些算法
list容器代码
#include<iostream>
using namespace std;
#include<list>
void printList(const list<int>& L)
{
for (list<int>::const_iterator it = L.begin(); it != L.end(); it++)
{
cout << *it << " ";
}
cout << endl;
}
void test01()//构造
{
list<int>L1;
L1.push_back(10);
L1.push_back(20);
L1.push_back(30);
L1.push_back(40);
printList(L1);
list<int>L2(L1.begin(), L1.end());
printList(L2);
list<int>L3(L2);
printList(L3);
list<int>L4(10,99);
printList(L4);
}
void test02()//赋值和交换
{
list<int>L1;
L1.push_back(100);
L1.push_back(20);
L1.push_back(90);
L1.push_back(23);
list<int>L2;
L2 = L1;
printList(L2);
list<int>L3;
L3.assign(L2.begin(), L2.end());
printList(L3);
list<int>L4;
L4.push_back(8);
L4.push_back(6);
cout << "交换前:" << endl;
printList(L3);
printList(L4);
cout << "交换后:" << endl;
L3.swap(L4);
printList(L3);
printList(L4);
}
void test03()//大小
{
list<int>L1;
L1.push_back(100);
L1.push_back(20);
L1.push_back(90);
L1.push_back(23);
printList(L1);
if (L1.empty())
{
cout << "容器是空的!" << endl;
}
else
{
cout << "容器不为空!" << endl;
cout << "容器的大小为:" << L1.size() << endl;
}
L1.resize(6);
printList(L1);
L1.resize(10, 78);
printList(L1);
L1.resize(2);
printList(L1);
}
void test04()//插入和删除
{
list<int>L1;
L1.push_back(20);
L1.push_back(30);
L1.push_front(40);
L1.push_front(60);
printList(L1);
L1.pop_back();
L1.pop_front();
printList(L1);
L1.insert(L1.begin(), 20);
printList(L1);
list<int>::iterator it = L1.begin();
it++;
L1.insert(++it, 2, 99);
printList(L1);
L1.erase(L1.begin(), it);
printList(L1);
it = L1.begin();
L1.erase(it);
printList(L1);
L1.push_back(99);
L1.push_back(99);
L1.push_front(99);
printList(L1);
L1.remove(99);
printList(L1);
L1.clear();
printList(L1);
}
void test05()//数据存取
{
list<int>L1;
L1.push_back(20);
L1.push_back(30);
L1.push_front(40);
L1.push_front(60);
printList(L1);
cout << "L1的第一个元素是:" << L1.front() << endl;
cout << "L1的最后一个元素是:" << L1.back() << endl;
}
void test06()//反转
{
list<int>L1;
L1.push_back(20);
L1.push_back(30);
L1.push_front(40);
L1.push_front(60);
cout << "反转前:" << endl;
printList(L1);
cout << "反转后:" << endl;
L1.reverse();
printList(L1);
}
bool myCompare(int v1, int v2)//降序
{
return v1 > v2;
}
void test07()//排序
{
list<int>L1;
L1.push_back(20);
L1.push_back(30);
L1.push_front(40);
L1.push_front(60);
printList(L1);
L1.sort();//默认升序
printList(L1);
L1.sort(myCompare);
printList(L1);
}
int main()
{
test01();
test02();
test03();
test04();
test05();
test06();
test07();
return 0;
}
对于自定义数据类型,必须指定
list容器排序示例代码
#include<iostream>
using namespace std;
#include<string>
#include<list>
#include<algorithm>
//按年龄进行升序,若年龄相同,按身高进行降序
class Person
{
public:
Person(string name, int age, int height)
{
this->m_age = age;
this->m_height = height;
this->m_name = name;
}
string m_name;
int m_age;
int m_height;
};
void printList(const list < Person >&L)
{
for (list<Person>::const_iterator it = L.begin(); it != L.end(); it++)
{
cout << "姓名:" << (*it).m_name << "\t年龄:" << it->m_age << "\t身高:" << it->m_height << endl;
}
}
bool myCompare(Person& p1, Person& p2)
{
if (p1.m_age == p2.m_age)
{
return (p1.m_height > p2.m_height);
}
else
{
return (p1.m_age < p2.m_age);
}
}
void test01()
{
Person p1("张三",33,178);
Person p2("李四", 33, 156);
Person p3("王五", 56, 188);
Person p4("赵六", 55, 168);
Person p5("郑和", 33, 198);
Person p6("韩信", 55, 158);
Person p7("王维", 34, 190);
list<Person>L1;
L1.push_back(p1);
L1.push_back(p2);
L1.push_back(p3);
L1.push_back(p4);
L1.push_back(p5);
L1.push_back(p6);
L1.push_back(p7);
printList(L1);
cout << "排序后:" << endl;
L1.sort(myCompare);
printList(L1);
}
int main()
{
test01();
return 0;
}
set容器和multiset容器
set构造和赋值
set大小和交换
set插入和删除
set查找和统计
set和multiset区别
pair对组创建
set容器排序
在vs2019中编译未能通过,暂时未解决这个问题,可以用别的编译器编译通过
set容器代码
//排序部分代码在vs2019有误,不清楚原因,用其他编译器可以成功运行
#include<iostream>
using namespace std;
#include<set>
void printSet(set<int>& s)
{
for (set<int>::iterator it = s.begin(); it != s.end(); it++)
{
cout << *it << " ";
}
cout << endl;
}
void test01()//构造和赋值
{
set<int>s1;
s1.insert(30);
s1.insert(60);
s1.insert(50);
s1.insert(20);
printSet(s1);
set<int>s2(s1);
printSet(s2);
set<int>s3;
s3 = s2;
printSet(s3);
}
void test02()//大小和交换
{
set<int>s1;
s1.insert(30);
s1.insert(60);
s1.insert(50);
s1.insert(20);
printSet(s1);
if (s1.empty())
{
cout << "容器为空!" << endl;
}
else
{
cout << "容器不为空!" << endl;
cout << "容器的大小为:" << s1.size() << endl;
}
set<int>s2;
s2.insert(300);
s2.insert(600);
s2.insert(500);
s2.insert(200);
cout << "交换前:" << endl;
printSet(s1);
printSet(s2);
s1.swap(s2);
cout << "交换后:" << endl;
printSet(s1);
printSet(s2);
}
void test03()//插入和删除
{
set<int>s1;
s1.insert(30);
s1.insert(60);
s1.insert(50);
s1.insert(20);
s1.insert(300);
s1.insert(600);
s1.insert(500);
s1.insert(200);
printSet(s1);
s1.erase(s1.begin());
printSet(s1);
s1.erase(60);
printSet(s1);
//s1.erase(s1.begin(), s1.end());
s1.clear();
printSet(s1);
}
void test04()//查找和统计
{
set<int>s1;
s1.insert(30);
s1.insert(60);
s1.insert(50);
s1.insert(20);
printSet(s1);
set<int>::iterator pos=s1.find(30);
if (pos != s1.end())
{
cout << "查到了:" << *pos << endl;
}
else
cout << "未查到!" << endl;
int num = s1.count(30);
cout << "30个数为:" << num << endl;
}
void test05()//set\multiset区别
{
set<int>s1;
pair<set<int>::iterator,bool>it=s1.insert(10);
if (it.second)
{
cout << "第一次插入成功!" << endl;
}
else
{
cout << "第一次插入失败!" << endl;
}
it = s1.insert(10);
if (it.second)
{
cout << "第二次插入成功!" << endl;
}
else
{
cout << "第二次插入失败!" << endl;
}
it = s1.insert(20);
if (it.second)
{
cout << "第三次插入成功!" << endl;
}
else
{
cout << "第三次插入失败!" << endl;
}
multiset<int>ms1;
ms1.insert(10);
ms1.insert(10);
ms1.insert(10);
ms1.insert(10);
for (multiset<int>::iterator it = ms1.begin(); it != ms1.end(); it++)
{
cout << *it << " ";
}
cout << endl;
}
void test06()//对组的创建
{
pair<string, int>p1("Tom", 22);
cout << "name:" << p1.first << "\tage:" << p1.second << endl;
pair<string, int>p2 = make_pair("Jim", 44);
cout << "name:" << p2.first << "\tage:" << p2.second << endl;
}
class myCompare
{
public:
bool operator()(int s1,int s2)
{
return s1 > s2;
}
};
void test07()//排序内置数据类型
{
set<int>s1;
s1.insert(20);
s1.insert(30);
s1.insert(50);
s1.insert(10);
s1.insert(40);
printSet(s1);
set<int, myCompare>s2;
s2.insert(20);
s2.insert(30);
s2.insert(50);
s2.insert(10);
s2.insert(40);
for (set<int, myCompare>::const_iterator it = s2.begin(); it != s2.end(); it++)
{
cout << *it << " ";
}
cout << endl;
}
class Person
{
public:
Person(string name,int age)
{
this->m_age = age;
this->m_name = name;
}
int m_age;
string m_name;
};
class comparePerson
{
public:
bool operator()(const Person& p1, const Person& p2)
{
return p1.m_age > p2.m_age;
}
};
void test08()//排序自定义数据类型
{
Person p1("张飞", 33);
Person p2("赵云", 56);
Person p3("刘备", 26);
Person p4("关羽", 74);
set<Person, comparePerson>s1;
s1.insert(p1);
s1.insert(p2);
s1.insert(p3);
s1.insert(p4);
for (set<Person, comparePerson>::iterator it = s1.begin(); it != s1.end(); it++)
{
cout << "姓名:" << it->m_name << "\t年龄:" << (*it).m_age << endl;
}
}
int main()
{
//test01();
//test02();
//test03();
//test04();
//test05();
//test06();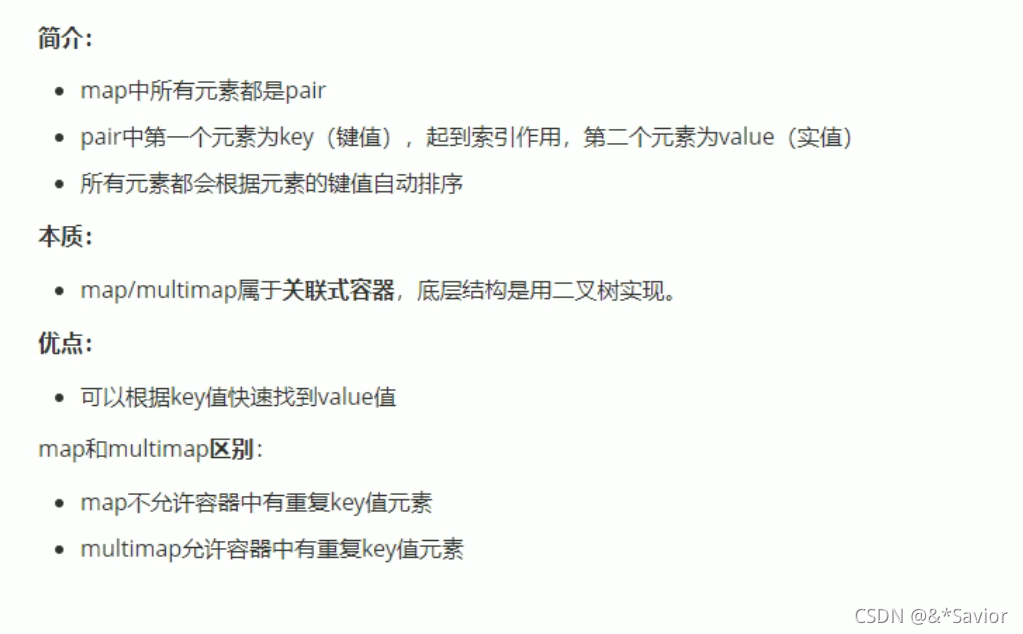
//test07();
test08();
return 0;
}
map/multimap容器
map构造和赋值
map大小和交换
map插入和删除
map查找和统计
map不允许插入重复key元素,所以count统计结果只能是0和1,multimap可能大于1
map排序
map容器代码
//vs2019排序部分无法编译,换个编译器可以
#include<iostream>
#include<map>
using namespace std;
void printMap(map<int, int>m)
{
for (map<int, int>::iterator it = m.begin(); it != m.end(); it++)
{
cout << "key=" << it->first << "\tvalue=" << (*it).second << endl;
}
cout << endl;
}
void test01()//map构造和赋值
{
map<int, int>m1;
m1.insert(pair<int, int>(1, 10));
m1.insert(pair<int, int>(3, 76));
m1.insert(pair<int, int>(4, 60));
m1.insert(pair<int, int>(2, 50));
printMap(m1);
map<int, int>m2(m1);
printMap(m2);
map<int, int>m3;
m3 = m2;
printMap(m3);
}
void test02()//大小和交换
{
map<int, int>m1;
m1.insert(pair<int, int>(1, 10));
m1.insert(pair<int, int>(3, 76));
m1.insert(pair<int, int>(4, 60));
m1.insert(pair<int, int>(2, 50));
printMap(m1);
if (m1.empty())
{
cout << "容器为空!" << endl;
}
else
{
cout << "容器不为空!" << endl;
cout << "大小为:" << m1.size() << endl;
}
map<int, int>m2;
m2.insert(pair<int, int>(6, 90));
m2.insert(pair<int, int>(7, 30));
m2.insert(pair<int, int>(8, 70));
cout << "交换前:" << endl;
printMap(m1);
printMap(m2);
m1.swap(m2);
cout << "交换后:" << endl;
printMap(m1);
printMap(m2);
}
void test03()//插入和清空
{
map<int, int>m1;
m1.insert(pair<int, int>(1, 10));//第一种插入方式
m1.insert(make_pair(2, 70));//第二种插入方式
m1.insert(map<int, int>::value_type(3, 80));//第三种插入方式
m1[4] = 90;//第四种插入方式-----不建议使用[]来进行插入,可以用来访问某个键的值
printMap(m1);
m1.erase(m1.begin());
printMap(m1);
m1.erase(2);//按键值来删
printMap(m1);
//m1.erase(m1.begin(), m1.end());//清空
m1.clear();
printMap(m1);
}
void test04()//查找和统计
{
map<int, int>m1;
m1.insert(pair<int, int>(1, 10));
m1.insert(pair<int, int>(3, 76));
m1.insert(pair<int, int>(4, 60));
m1.insert(pair<int, int>(2, 50));
printMap(m1);
map<int, int>::iterator pos = m1.find(2);
if (pos != m1.end())
{
cout << "查到了key=" << pos->first << "\tvalue=" << (*pos).second << endl;
}
else
{
cout << "未查到!" << endl;
}
int num = m1.count(3);
cout << "num=" << num << endl;
}
class compareMap
{
public:
bool operator()(int v1, int v2)
{
return v1 > v2;
}
};
void test05()//利用仿函数改变排序规则
{
map<int, int,compareMap>m1;
m1.insert(make_pair(1, 20));
m1.insert(make_pair(3, 29));
m1.insert(make_pair(8, 89));
m1.insert(make_pair(4, 50));
m1.insert(make_pair(6, 54));
for (map<int, int, compareMap>::iterator it = m1.begin(); it != m1.end(); it++)
{
cout << "key=" << (*it).first << "\tvalue=" << it->second << endl;
}
cout << endl;
}
int main()
{
test01();
test02();
test03();
test04();
test05();
return 0;
}
员工分组案例
#include<iostream>
using namespace std;
#include<vector>
#include<map>
#include<ctime>
#include<string>
#define YANFA 0
#define SHENGCHAN 1
#define XUANCHUAN 2
class Worker
{
public:
int m_wage;
string m_name;
};
void createWorker(vector<Worker>& v)
{
string nameSeed = "ABCDEFGHIJ";
for (int i = 0; i < 10; i++)
{
Worker w;
w.m_name = "员工";
w.m_name += nameSeed[i];
w.m_wage = rand() % 10000 + 10000;
v.push_back(w);
}
}
void setGroup(vector<Worker>& v, multimap<int, Worker>& m)
{
for (vector<Worker>::iterator it = v.begin(); it != v.end(); it++)
{
int key = rand() % 3;
m.insert(make_pair(key, (*it)));
}
}
void showWorker(multimap<int, Worker>& m)
{
cout << "研发组:" << endl;
multimap<int ,Worker>::iterator pos = m.find(YANFA);
int count = m.count(YANFA);
int num = 0;
for (; pos != m.end() && num < count; pos++, num++)
{
cout << "姓名:" << pos->second.m_name << "\t工资:" << pos->second.m_wage << endl;
}
cout << "------------------------------" << endl;
cout << "生产组:" << endl;
pos = m.find(SHENGCHAN);
count = m.count(SHENGCHAN);
num = 0;
for (; pos != m.end() && num < count; pos++, num++)
{
cout << "姓名:" << pos->second.m_name << "\t工资:" << pos->second.m_wage << endl;
}
cout << "------------------------------" << endl;
cout << "宣传组:" << endl;
pos = m.find(XUANCHUAN);
count = m.count(XUANCHUAN);
num = 0;
for (; pos != m.end() && num < count; pos++, num++)
{
cout << "姓名:" << pos->second.m_name << "\t工资:" << pos->second.m_wage << endl;
}
}
int main()
{
srand((unsigned int)time(NULL));
vector<Worker>vWorker;
createWorker(vWorker);
/*for (vector<Worker>::iterator it = vWorker.begin(); it != vWorker.end(); it++)
{
cout << "姓名:" << (*it).m_name << "\t工资:" << it->m_wage << endl;
}*/
multimap<int, Worker>mWorker;
setGroup(vWorker, mWorker);;
showWorker(mWorker);
return 0;
}
函数对象
概念:
重载函数调用操作符的类,其对象常称为函数对象
函数对象使用重载的()时,行为类似函数调用,也叫仿函数
本质:
函数对象(仿函数)本质是一个类,不是一个函数
特点:
代码示例
#include<iostream>
using namespace std;
#include<string>
class myAdd
{
public:
int operator()(int a, int b)
{
return a + b;
}
};
class myPrint
{
public:
myPrint()
{
m_count = 0;
}
void operator()(string test)
{
cout << test << endl;
m_count++;
}
int m_count;
};
void test01()
{
myAdd md;
cout << md(2, 3) << endl;
}
void test02()
{
myPrint md;
md("hello c++");
md("hello c++");
md("hello c++");
md("hello c++");
cout << "打印次数为:" << md.m_count << endl;
}
void secPrint(myPrint& m, string s)
{
m(s);
}
void test03()
{
myPrint mp;
secPrint(mp, "helloworld");
}
int main()
{
//test01();
//test02();
test03();
return 0;
}
谓词
一元谓词代码示例
#include<iostream>
using namespace std;
#include<vector>
#include<algorithm>
class greaterFive
{
public:
bool operator()(int val)
{
return val > 5;
}
};
int main()
{
vector<int>v;
for (int i = 0; i < 10; i++)
{
v.push_back(i);
}
vector<int>::iterator pos = find_if(v.begin(), v.end(), greaterFive());
if (pos == v.end())
{
cout << "未找到!" << endl;
}
else
{
cout << "找到位置为:" << *pos << endl;
}
return 0;
}
二元谓词代码示例
#include<iostream>
using namespace std;
#include<algorithm>
#include<vector>
class myCompare
{
public:
bool operator()(int val1, int val2)
{
return val1 > val2;
}
};
void printVector(vector<int>& v)
{
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
{
cout << *it << " ";
}
cout << endl;
}
int main()
{
vector<int>v;
v.push_back(30);
v.push_back(20);
v.push_back(80);
v.push_back(10);
v.push_back(70);
sort(v.begin(), v.end());
printVector(v);
cout << "重新排序后:" << endl;
sort(v.begin(), v.end(), myCompare());
printVector(v);
return 0;
}
内建函数对象
算术仿函数
代码示例
#include<iostream>
using namespace std;
#include<functional>
int main()
{
negate<int>n;//取反
cout << n(20) << endl;
plus<int>p;//相加
cout << p(10, 30) << endl;
return 0;
}
关系仿函数
代码示例
#include<iostream>
using namespace std;
#include<functional>
#include<vector>
#include<algorithm>
void printVector(vector<int>& v)
{
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
{
cout << *it << " ";
}
cout << endl;
}
void test01()
{
vector<int>v;
v.push_back(30);
v.push_back(20);
v.push_back(50);
v.push_back(10);
v.push_back(40);
printVector(v);
cout << "排序后:" << endl;
sort(v.begin(), v.end(), greater<int>());
printVector(v);
}
int main()
{
test01();
return 0;
}
逻辑仿函数
代码示例
#include<iostream>
using namespace std;
#include<functional>
#include<vector>
#include<algorithm>
void printVector(vector<bool>& v)
{
for (vector<bool>::iterator it = v.begin(); it != v.end(); it++)
{
cout << *it << " ";
}
cout << endl;
}
void test01()
{
vector<bool>v;
v.push_back(true);
v.push_back(false);
v.push_back(true);
v.push_back(false);
printVector(v);
vector<bool>v1;
v1.resize(v.size());
transform(v.begin(), v.end(), v1.begin(), logical_not<bool>());
printVector(v1);
}
int main()
{
test01();
return 0;
}
算法
遍历算法
示例代码
#include<iostream>
using namespace std;
#include<vector>
#include<algorithm>
void print(int val)
{
cout << val << " ";
}
class printV
{
public:
void operator()(int val)
{
cout << val << " ";
}
};
int main()
{
vector<int>v;
v.push_back(20);
v.push_back(40);
v.push_back(50);
v.push_back(10);
v.push_back(40);
for_each(v.begin(), v.end(), print);
cout << endl;
for_each(v.begin(), v.end(), printV());
return 0;
}
代码示例
#include<iostream>
using namespace std;
#include<vector>
#include<algorithm>
class Transform
{
public:
int operator()(int val)
{
return val + 100;
}
};
class printV
{
public:
void operator()(int val)
{
cout << val << " ";
}
};
int main()
{
vector<int>v;
for (int i = 0; i < 10; i++)
{
v.push_back(i);
}
vector<int>v1;
v1.resize(v.size());
transform(v.begin(), v.end(), v1.begin(), Transform());
for_each(v1.begin(), v1.end(), printV());
cout << endl;
return 0;
}
查找算法
返回值是一个迭代器
代码示例
#include<iostream>
using namespace std;
#include<string>
#include<vector>
#include<algorithm>
void test01()
{
vector<int>v;
for (int i = 0; i < 10; i++)
{
v.push_back(i);
}
vector<int>::iterator it = find(v.begin(), v.end(), 7);
if (it == v.end())
{
cout << "未找到!" << endl;
}
else
{
cout << "找到了" << *it << endl;
}
}
class Person
{
public:
Person(string name, int age)
{
this->m_name = name;
this->m_age = age;
}
bool operator==(const Person& p)
{
if (this->m_name == p.m_name && this->m_age == p.m_age)
{
return true;
}
else
{
return false;
}
}
int m_age;
string m_name;
};
void test02()
{
vector<Person>v;
Person p1("liyuli", 834);
Person p2("lilri", 934);
Person p3("liyli", 374);
Person p4("lilrti", 343);
v.push_back(p1);
v.push_back(p2);
v.push_back(p3);
v.push_back(p4);
Person p5("liyuli", 834);
vector<Person>::iterator it = find(v.begin(), v.end(), p5);
if (it == v.end())
{
cout << "未找到!" << endl;
}
else
{
cout << "找到姓名:" << it->m_name << "\t年龄:" << (*it).m_age << endl;
}
}
int main()
{
//test01();
test02();
return 0;
}
代码示例
#include<iostream>
using namespace std;
#include<string>
#include<vector>
#include<algorithm>
class greater7
{
public:
bool operator()(int val)
{
return val > 7;
}
};
void test01()
{
vector<int>v;
for (int i = 0; i < 10; i++)
{
v.push_back(i);
}
vector<int>::iterator it = find_if(v.begin(), v.end(), greater7());
if (it == v.end())
{
cout << "未找到!" << endl;
}
else
{
cout << "找到了" << *it << endl;
}
}
class Person
{
public:
Person(string name, int age)
{
this->m_name = name;
this->m_age = age;
}
int m_age;
string m_name;
};
class greater30
{
public:
bool operator()(Person& p)
{
if (p.m_age > 30)
{
return true;
}
else
{
return false;
}
}
};
void test02()
{
vector<Person>v;
Person p1("liyuli", 34);
Person p2("lilri", 24);
Person p3("liyli", 37);
Person p4("lilrti", 10);
v.push_back(p1);
v.push_back(p2);
v.push_back(p3);
v.push_back(p4);
Person p5("liyuli", 834);
vector<Person>::iterator it = find_if(v.begin(), v.end(), greater30());
if (it == v.end())
{
cout << "未找到!" << endl;
}
else
{
cout << "找到姓名:" << it->m_name << "\t年龄:" << (*it).m_age << endl;
}
}
int main()
{
//test01();
test02();
return 0;
}
代码示例
#include<iostream>
using namespace std;
#include<vector>
#include<algorithm>
void test01()
{
vector<int>v;
v.push_back(10);
v.push_back(1);
v.push_back(4);
v.push_back(3);
v.push_back(4);
v.push_back(6);
//v.push_back(6);
vector<int>::iterator it = adjacent_find(v.begin(), v.end());
if (it == v.end())
{
cout << "未找到!" << endl;
}
else
{
cout << "找到了" << *it << endl;
}
}
int main()
{
test01();
return 0;
}
注意:容器必须是有序的序列,如果无序,结果未知
代码示例
#include<iostream>
using namespace std;
#include<vector>
#include<algorithm>
void test01()
{
vector<int>v;
for (int i = 0; i < 10; i++)
{
v.push_back(i);
}
bool ret = binary_search(v.begin(), v.end(), 8);
if (ret)
{
cout << "找到了!" << endl;
}
else
{
cout << "未找到" << endl;
}
}
int main()
{
test01();
return 0;
}
代码示例
#include<iostream>
using namespace std;
#include<vector>
#include<algorithm>
#include<string>
void test01()
{
vector<int>v;
v.push_back(10);
v.push_back(3);
v.push_back(3);
v.push_back(4);
v.push_back(3);
v.push_back(7);
int num = count(v.begin(), v.end(), 3);
cout << "3的个数为:" << num << endl;
}
class Person
{
public:
Person(string name, int age)
{
this->m_age = age;
this->m_name = name;
}
bool operator==(const Person& p)
{
if (this->m_age == p.m_age)
{
return true;
}
else
{
return false;
}
}
string m_name;
int m_age;
};
void test02()
{
vector<Person>v;
Person p1("赵云", 34);
Person p2("关羽", 35);
Person p3("刘备", 34);
Person p4("张飞", 34);
v.push_back(p1);
v.push_back(p2);
v.push_back(p3);
v.push_back(p4);
Person p5("孙尚香", 34);
int num = count(v.begin(), v.end(), p5);
cout << "和孙尚香年龄相同的有:" << num << endl;
}
int main()
{
//test01();
test02();
return 0;
}
代码示例
#include<iostream>
using namespace std;
#include<vector>
#include<algorithm>
#include<string>
class greater3
{
public:
bool operator()(int val)
{
return val > 3;
}
};
void test01()
{
vector<int>v;
v.push_back(10);
v.push_back(3);
v.push_back(3);
v.push_back(4);
v.push_back(3);
v.push_back(7);
int num = count_if(v.begin(), v.end(), greater3());
cout << "大于3的个数为:" << num << endl;
}
class Person
{
public:
Person(string name, int age)
{
this->m_age = age;
this->m_name = name;
}
string m_name;
int m_age;
};
class greater40
{
public:
bool operator()(const Person& p)
{
return p.m_age > 40;
}
};
void test02()
{
vector<Person>v;
Person p1("赵云", 54);
Person p2("关羽", 35);
Person p3("刘备", 34);
Person p4("张飞", 44);
Person p5("孙尚香", 34);
v.push_back(p1);
v.push_back(p2);
v.push_back(p3);
v.push_back(p4);
v.push_back(p5);
int num = count_if(v.begin(), v.end(), greater40());
cout << "年龄大于40的有:" << num << endl;
}
int main()
{
//test01();
test02();
return 0;
}
排序算法
#include<iostream>
using namespace std;
#include<algorithm>
#include<vector>
#include<functional>
void printV(int val)
{
cout << val << " ";
}
class printVt
{
public:
bool operator()(int val,int val2)
{
return val > val2;
}
};
void test01()
{
vector<int>v;
v.push_back(10);
v.push_back(40);
v.push_back(20);
v.push_back(60);
sort(v.begin(), v.end());
for_each(v.begin(), v.end(), printV);
cout << endl;
sort(v.begin(), v.end(), greater<int>());
//sort(v.begin(), v.end(),printVt());
for_each(v.begin(), v.end(), printV);
cout << endl;
}
int main()
{
test01();
return 0;
}
#include<iostream>
using namespace std;
#include<algorithm>
#include<vector>
#include<ctime>
void printV(int val)
{
cout << val << " ";
}
void test01()
{
vector<int>v;
for (int i = 0; i < 10; i++)
{
v.push_back(i);
}
random_shuffle(v.begin(), v.end());
for_each(v.begin(), v.end(), printV);
cout << endl;
}
int main()
{
srand((unsigned int)time(NULL));
test01();
return 0;
}
代码示例
#include<iostream>
using namespace std;
#include<algorithm>
#include<vector>
void printV(int val)
{
cout << val << " ";
}
void test01()
{
vector<int>v;
vector<int>v1;
for (int i = 0; i < 10; i++)
{
v.push_back(i);
v1.push_back(i + 100);
}
vector<int>v2;
v2.resize(v.size() + v1.size());
merge(v.begin(), v.end(), v1.begin(), v1.end(), v2.begin());
for_each(v2.begin(), v2.end(), printV);
cout << endl;
}
int main()
{
test01();
return 0;
}
代码示例
#include<iostream>
using namespace std;
#include<algorithm>
#include<vector>
void printV(int val)
{
cout << val << " ";
}
void test01()
{
vector<int>v;
for (int i = 0; i < 10; i++)
{
v.push_back(i);
}
for_each(v.begin(), v.end(), printV);
cout << endl;
cout << "翻转后:" << endl;
reverse(v.begin(), v.end());
for_each(v.begin(), v.end(), printV);
cout << endl;
}
int main()
{
test01();
return 0;
}
拷贝和替换算法
代码示例
#include<iostream>
using namespace std;
#include<algorithm>
#include<vector>
void printV(int val)
{
cout << val << " ";
}
void test01()
{
vector<int>v;
for (int i = 0; i < 10; i++)
{
v.push_back(i);
}
for_each(v.begin(), v.end(), printV);
cout << endl;
vector<int>v2;
v2.resize(v.size());
copy(v.begin(), v.end(), v2.begin());
for_each(v2.begin(), v2.end(), printV);
cout << endl;
}
int main()
{
test01();
return 0;
}
#include<iostream>
using namespace std;
#include<algorithm>
#include<vector>
void printV(int val)
{
cout << val << " ";
}
void test01()
{
vector<int>v;
for (int i = 0; i < 10; i++)
{
v.push_back(i);
}
for_each(v.begin(), v.end(), printV);
cout << endl;
cout << "替换后:" << endl;
replace(v.begin(), v.end(), 8, 44);
for_each(v.begin(), v.end(), printV);
cout << endl;
}
int main()
{
test01();
return 0;
}
代码示例
#include<iostream>
using namespace std;
#include<algorithm>
#include<vector>
class greater6
{
public:
bool operator()(int val)
{
return val > 6;
}
};
void printV(int val)
{
cout << val << " ";
}
void test01()
{
vector<int>v;
for (int i = 0; i < 10; i++)
{
v.push_back(i);
}
for_each(v.begin(), v.end(), printV);
cout << endl;
cout << "替换后:" << endl;
replace_if(v.begin(), v.end(), greater6(), 44);
for_each(v.begin(), v.end(), printV);
cout << endl;
}
int main()
{
test01();
return 0;
}
代码示例
注意:交换容器必须是同种类型
#include<iostream>
using namespace std;
#include<algorithm>
#include<vector>
class greater6
{
public:
bool operator()(int val)
{
return val > 6;
}
};
void printV(int val)
{
cout << val << " ";
}
void test01()
{
vector<int>v;
vector<int>v1;
for (int i = 0; i < 10; i++)
{
v.push_back(i);
v1.push_back(100 + i);
}
for_each(v.begin(), v.end(), printV);
cout << endl;
for_each(v1.begin(), v1.end(), printV);
cout << endl;
cout << "交换后:" << endl;
swap(v, v1);
for_each(v.begin(), v.end(), printV);
cout << endl;
for_each(v1.begin(), v1.end(), printV);
cout << endl;
}
int main()
{
test01();
return 0;
}
算术生成算法
代码示例
注意算法头文件
#include<iostream>
using namespace std;
#include<numeric>
#include<vector>
void test01()
{
vector<int>v;
for (int i = 0; i <= 100; i++)
{
v.push_back(i);
}
int count = accumulate(v.begin(), v.end(), 0);
cout << "count=" << count << endl;
}
int main()
{
test01();
return 0;
}
代码示例
#include<iostream>
using namespace std;
#include<numeric>
#include<vector>
#include<algorithm>
void myprint(int val)
{
cout << val << " ";
}
void test01()
{
vector<int>v;
v.resize(10);
fill(v.begin(), v.end(), 100);
for_each(v.begin(), v.end(), myprint);
cout << endl;
}
int main()
{
test01();
return 0;
}
集合算法
代码示例
#include<iostream>
using namespace std;
#include<vector>
#include<algorithm>
void myprint(int val)
{
cout << val << " ";
}
void test01()
{
vector<int>v;
vector<int>v1;
for (int i = 0; i < 10; i++)
{
v.push_back(i);
v1.push_back(i + 4);
}
vector<int>vtarget;
vtarget.resize(min(v1.size(), v.size()));
vector<int>::iterator itEnd = set_intersection(v.begin(), v.end(), v1.begin(), v1.end(), vtarget.begin());
for_each(vtarget.begin(),itEnd, myprint);
cout << endl;
}
int main()
{
test01();
return 0;
}
代码示例
#include<iostream>
using namespace std;
#include<vector>
#include<algorithm>
void myprint(int val)
{
cout << val << " ";
}
void test01()
{
vector<int>v;
vector<int>v1;
for (int i = 0; i < 10; i++)
{
v.push_back(i);
v1.push_back(i + 4);
}
vector<int>vtarget;
vtarget.resize(v1.size() + v.size());
vector<int>::iterator itEnd = set_union(v.begin(), v.end(), v1.begin(), v1.end(), vtarget.begin());
for_each(vtarget.begin(),itEnd, myprint);
cout << endl;
}
int main()
{
test01();
return 0;
}
代码示例
#include<iostream>
using namespace std;
#include<vector>
#include<algorithm>
void myprint(int val)
{
cout << val << " ";
}
void test01()
{
vector<int>v;
vector<int>v1;
for (int i = 0; i < 10; i++)
{
v.push_back(i);
v1.push_back(i + 4);
}
vector<int>vtarget;
vtarget.resize(max(v1.size(), v.size()));
vector<int>::iterator itEnd = set_difference(v.begin(), v.end(), v1.begin(), v1.end(), vtarget.begin());
cout << "v和v1容器的差集为:" << endl;
for_each(vtarget.begin(),itEnd, myprint);
cout << endl;
vector<int>::iterator litEnd = set_difference(v1.begin(), v1.end(), v.begin(), v.end(), vtarget.begin());
cout << "v1和v容器的差集为:" << endl;
for_each(vtarget.begin(), litEnd, myprint);
cout << endl;
}
int main()
{
test01();
return 0;
}