类和对象
C++面对对象三大特点:封装、继承、多态
C++认为,万事万物皆为对象,对象上有其属性和行为。
人可以作为对象,属性有姓名、身高、体重……行为有走、跑、跳、吃饭……
车可以作为对象,属性有轮胎、方姓盘、车灯……行为有载人、放音乐、放空调……
具有相同性质的对象,可以抽象称为类,人属于人类,车属于车类。(物以类聚)
1.1.1封装的意义
属性和行为写在一起,表现事物。类中的属性和行为统一称为成员,属性又称成员属性、成员变量,行为称为成员函数、成员方法。
语法:class 类名{ 访问权限 : 属性 / 行为}
要通过类来创建一个具体的圆(对象)
封装的访问权限有3种:
public 公共权限 —— 成员 类内可以访问,类外可以访问
protected 保护权限 —— 成员 类内可以访问 类外不可以访问 儿子可以访问父亲中保护的内容
private 私有权限 —— 成员 类内可以访问 类外不可以访问 儿子不可以访问父亲中保护的内容
#include <iostream>
using namespace std;
const double PI = 3.14; //常量的double类型
class Circle //class代表设计一个类,紧随其后的是类名称
{
public: //访问权限
int m_r; //属性-半径 属性一般用变量
double calculateZC() //行为-获取圆的周长 行为一般用函数
{
return 2 * PI * m_r;
}
};
int main()
{
Circle c1; //通过圆类来创建一个具体的圆cl(对象)
c1.m_r = 10; //给圆对象cl 的属性进行赋值
cout << "圆的周长为: " << c1.calculateZC() << endl;
system("pause");
return 0;
}
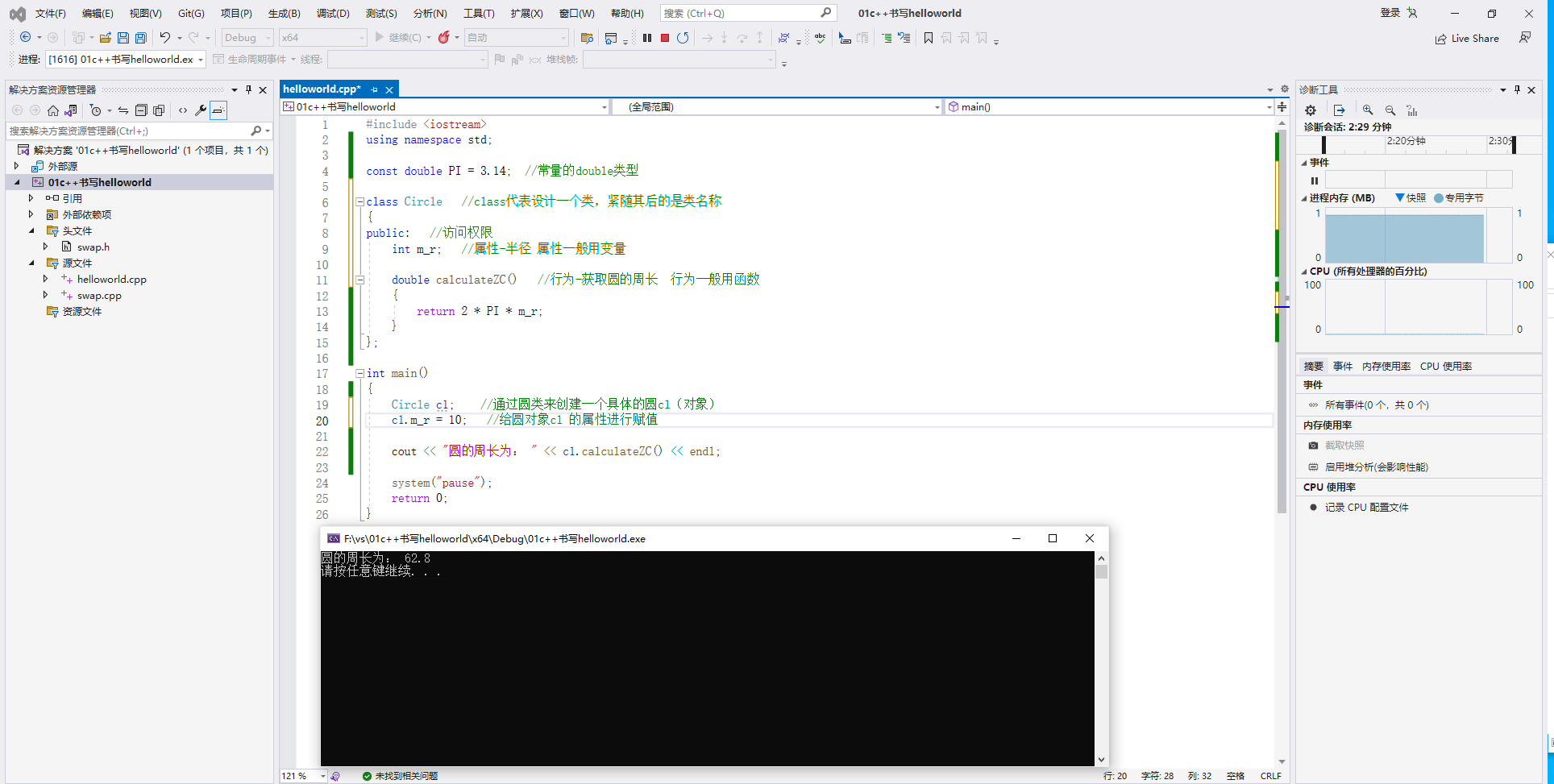
示例:设计学生类
属性有姓名和学号,可给姓名和学号赋值,可以显示学生的姓名和学号。
#include <iostream>
using namespace std;
#include <string>
class Student
{
public://权限
string m_Name;//属性
int m_Id;
void showStudent()//行为
{
cout << "姓名: " << m_Name << " 学号: " << m_Id << endl;
}
};
int main()
{
//创建一个具体的对象(学生)
Student s1;
s1.m_Name = "张三";
s1.m_Id = 1;
s1.showStudent();
Student s2;
s1.m_Name = "李四";
s1.m_Id = 2;
s1.showStudent();
system("pause");
return 0;
}
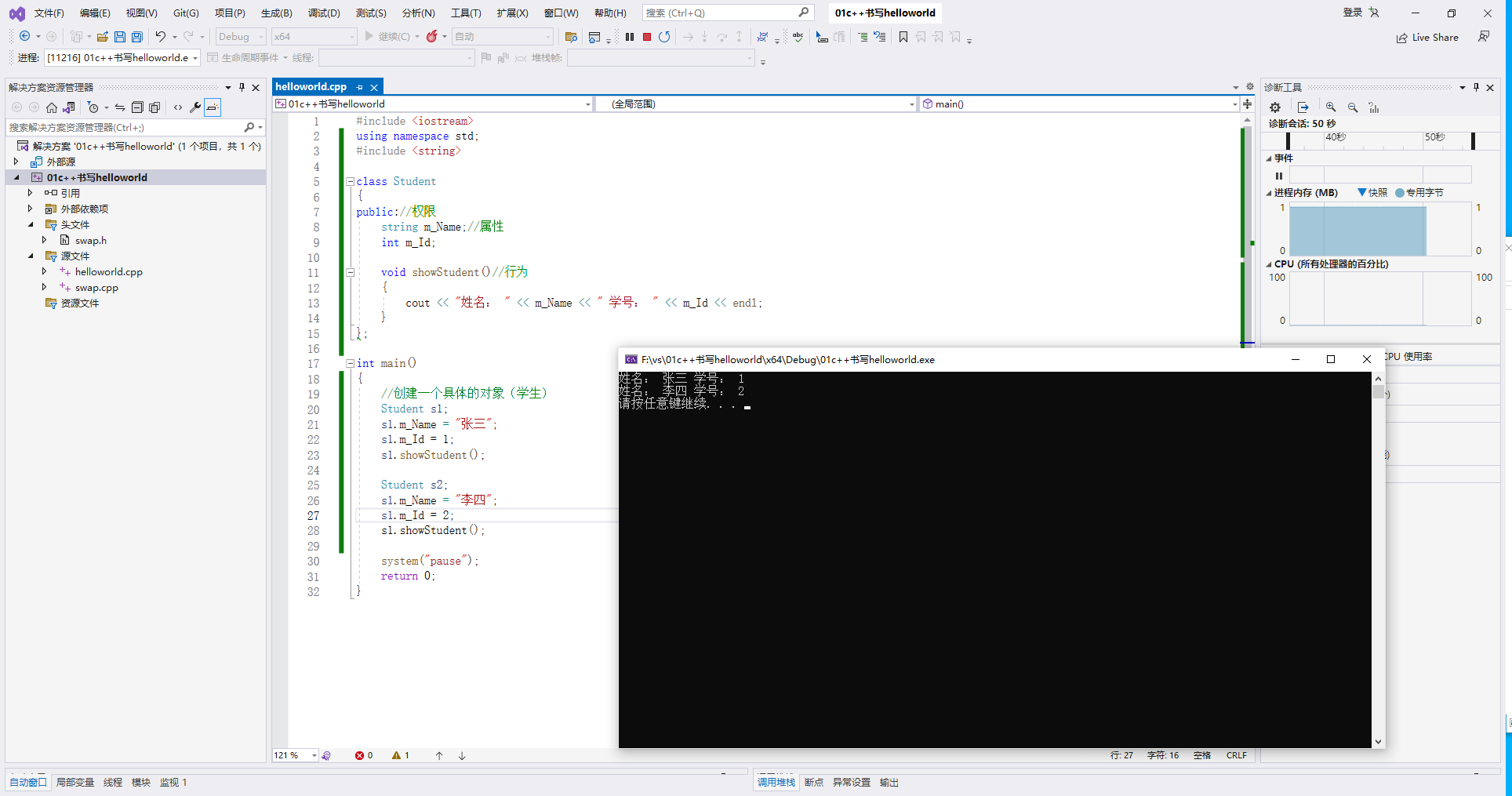
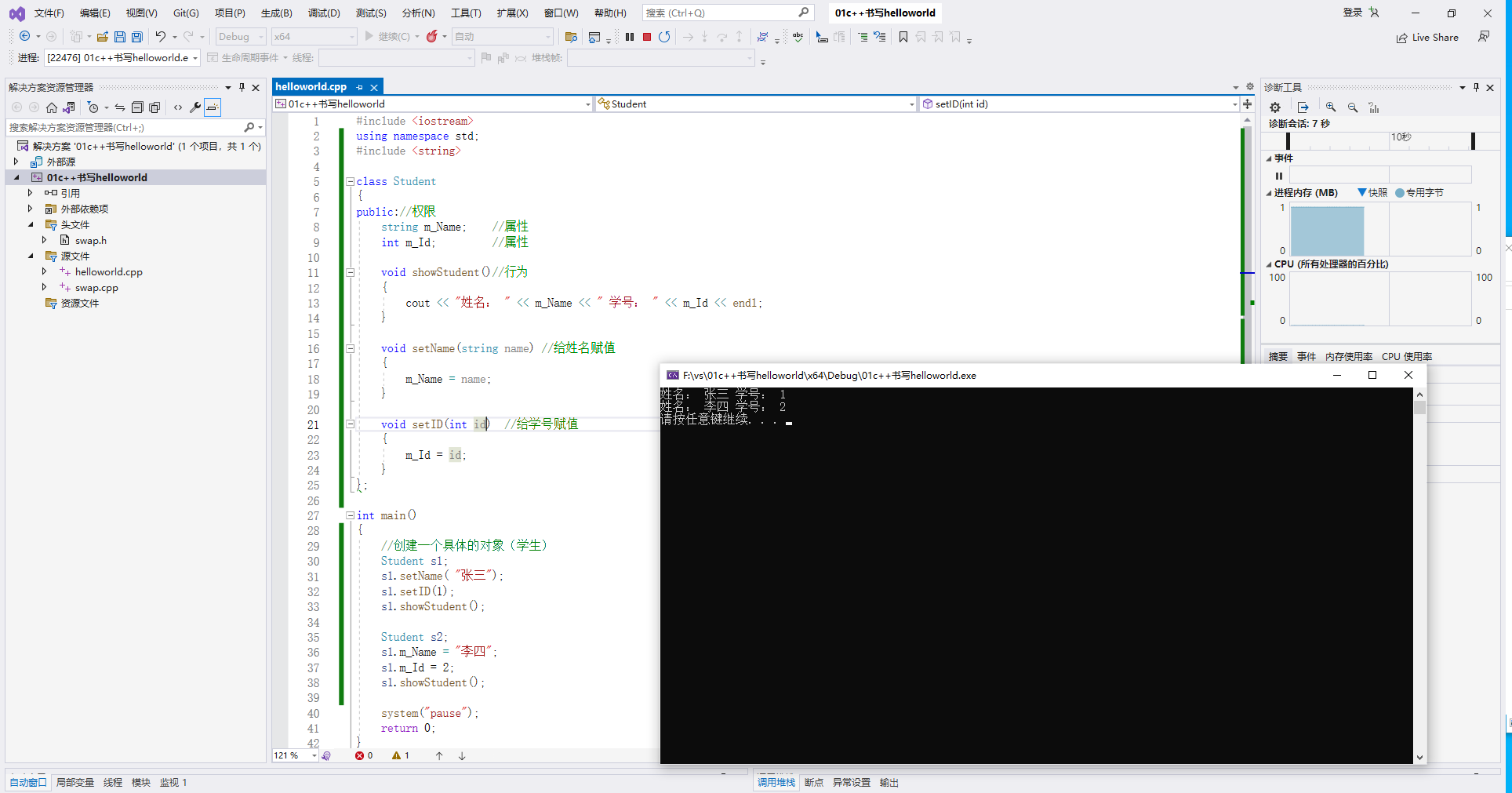
1.1.2struct 和 class 区别
struct 默认权限为公共,class默认访问权限为私有。
#include <iostream>
using namespace std;
#include <string>
class C1
{
int m_A; //默认权限 是私有
};
struct C2
{
int m_A;//默认权限 是公共 public
};
int main()
{
C1 c1;
//cl.m_A = 100; 会报错,在class里默认权限 私有,因此类外不可以访问
C2 c2;
c2.m_A = 100;
system("pause");
return 0;
}
1.1.3成员属性设置为私有
可读可写:
#include <iostream>
using namespace std;
#include <string>
class Person
{
public: //若属性都为私有,会给出Public的接口,对属性进行访问
//设置姓名
void setName(string name)
{
m_Name = name;
}
//获取姓名
string getName()
{
return m_Name;
}
private:
string m_Name; //可读可写
};
int main()
{
Person p;
p.setName ("张三");
cout << "姓名为: " << p.getName() << endl;
system("pause");
return 0;
}
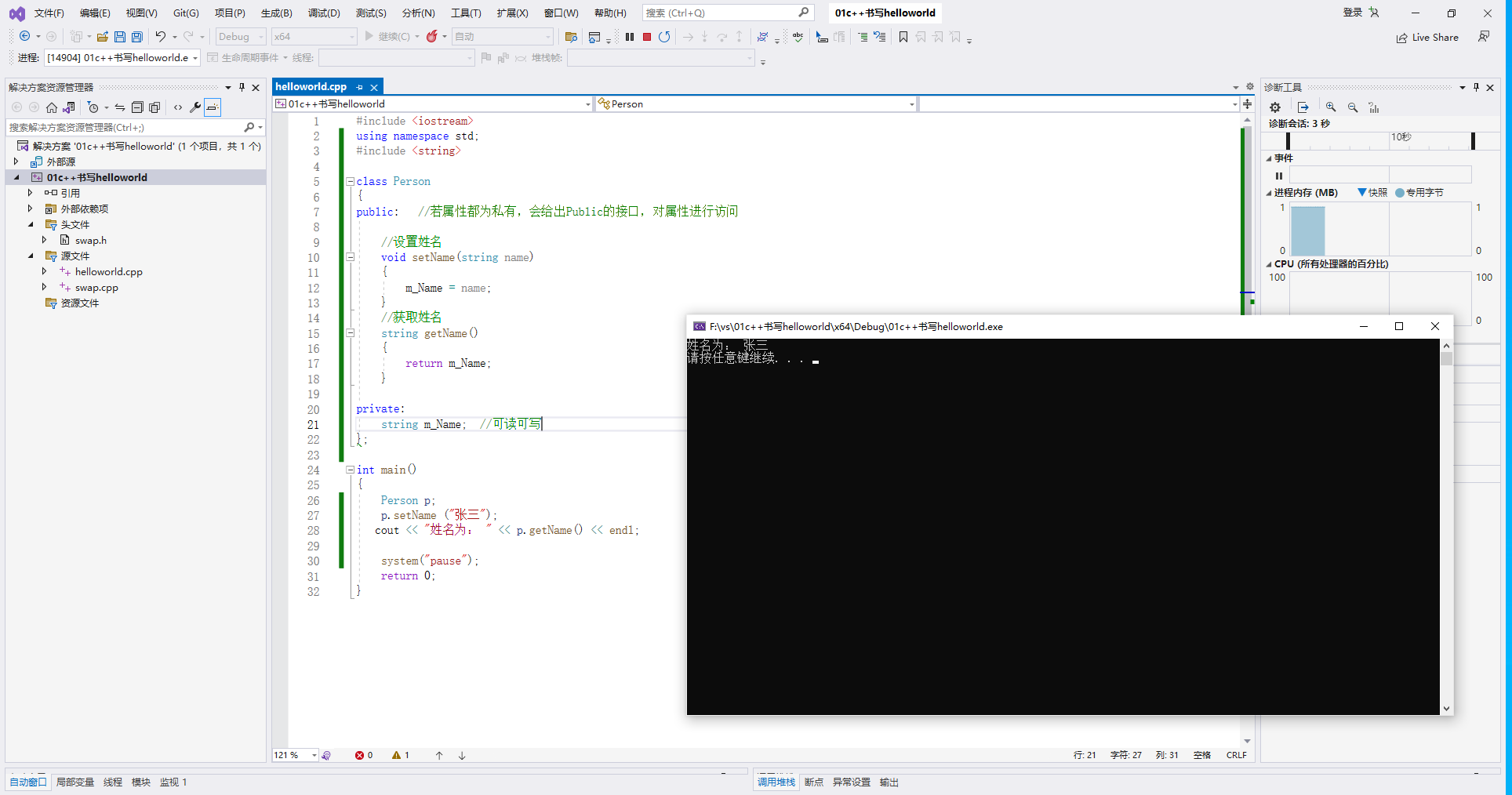
只读 get
#include <iostream>
using namespace std;
#include <string>
class Person
{
public: //若属性都为私有,会给出Public的接口,对属性进行访问
//获取年龄
int getAge()
{
m_Age = 0;
return m_Age;
}
private:
int m_Age; //只读
};
int main()
{
Person p;
cout << "年龄为 " << p.getAge() << endl;
system("pause");
return 0;
}
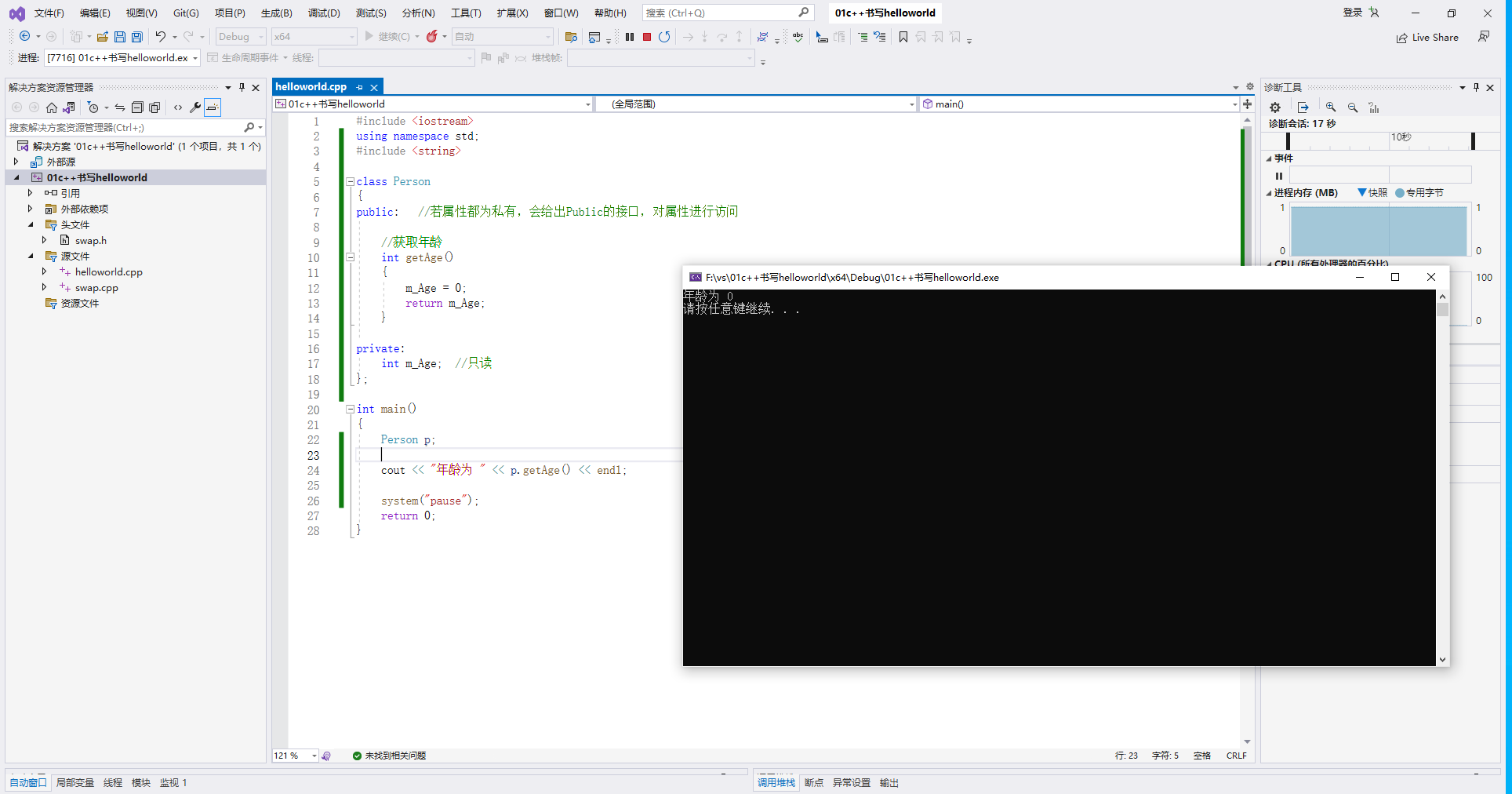
只写 set
#include <iostream>
using namespace std;
#include <string>
class Person
{
public: //若属性都为私有,会给出Public的接口,对属性进行访问
//获取年龄
void setLover(string lover)
{
m_Lover = lover;
}
private:
string m_Lover; //只读
};
int main()
{
Person p;
p.setLover("苍井");
// cout << "情人为" << p.m_lover << endl; 会报错,只能进行写的操作
system("pause");
return 0;
}
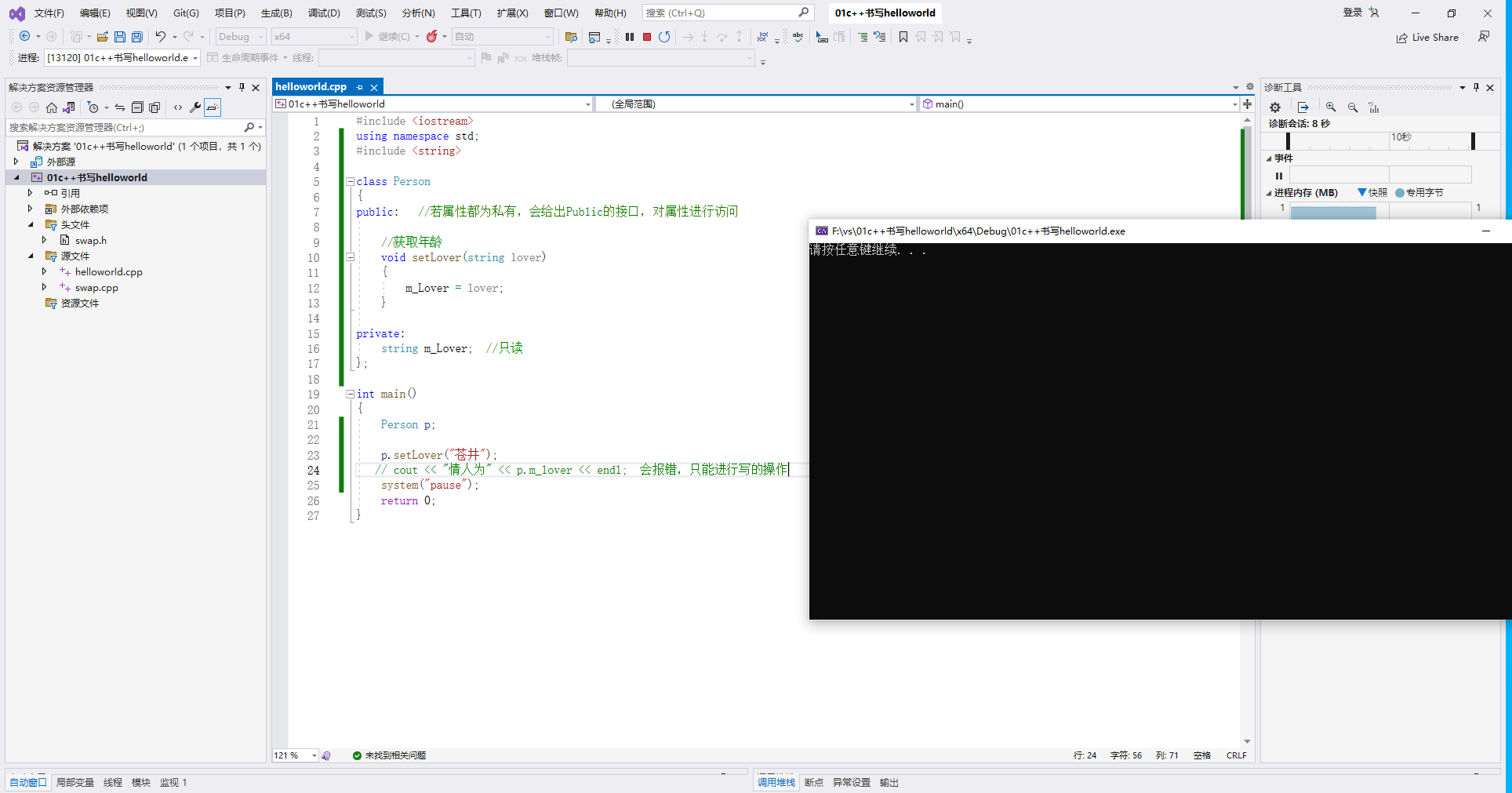
案例:设计立方块体
设计立方块体类(cube),求立方体面积和体积,分别用全局函数和成员函数(写在类内)判断两个立方体是否相等。
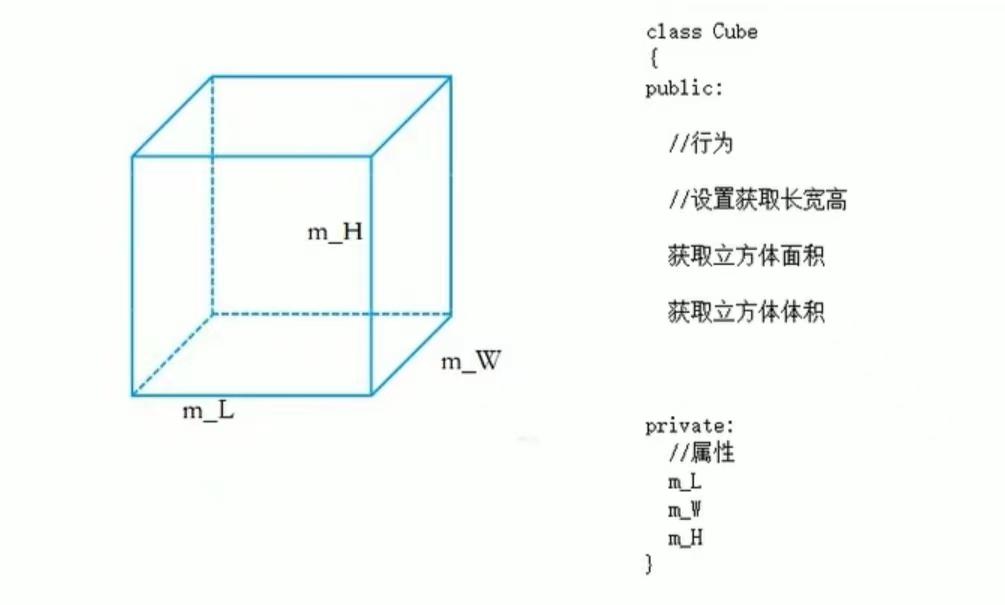
#include <iostream>
using namespace std;
#include <string>
class Cube
{
public:
void setL(int l) //设置长
{
m_L = l;
}
int getL() //获取长
{
return m_L;
}
void setW(int w) //设置宽
{
m_W = w;
}
int getW() //获取宽
{
return m_W;
}
void setH(int h) //设置高
{
m_H = h;
}
int getH() //获取高
{
return m_H;
}
//获取面积
int calculateS()
{
return 2 * m_L * m_W + 2 * m_H * m_W + 2 * m_L * m_H;
}
//获取体积
int calculateV()
{
return m_L * m_W * m_H;
}
private:
int m_L;
int m_W;
int m_H;
};
//利用全局函数判断两个立方体是否相等
bool isSame(Cube &c1, Cube &c2) //不加&是直传递,会拷贝一份数据,加&是引用传递,不会拷贝,用原始数据
{
if (c1.getL() == c2.getL() && c1.getW() == c2.getW() && c1.getH() == c2.getH())
{
return true;
}
return false;
}
int main()
{
Cube c1;
c1.setL(10);
c1.setW(10);
c1.setH(10);
cout << "c1的面积是: " << c1.calculateS() << endl;
cout << "c1的体积是: " << c1.calculateV() << endl;
Cube c2;
c2.setL(11);
c2.setW(10);
c2.setH(10);
bool ret = isSame(c1, c2);
if (ret)
{
cout << "c1和c2是相等的" << endl;
}
else
{
cout << "c1和c2是不相等的" << endl;
}
system("pause");
return 0;
}
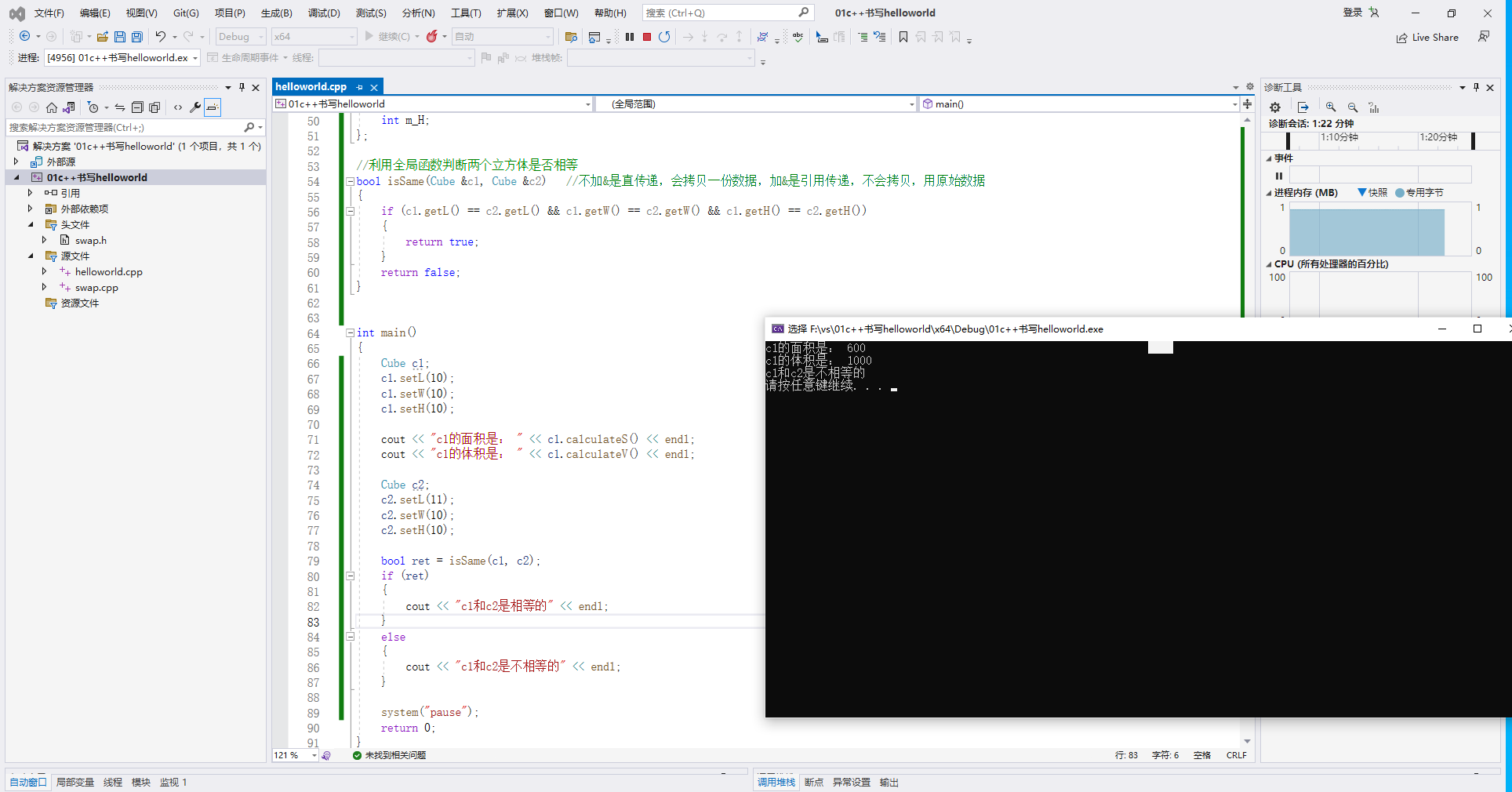
#include <iostream>
using namespace std;
#include <string>
class Cube
{
public:
void setL(int l) //设置长
{
m_L = l;
}
int getL() //获取长
{
return m_L;
}
void setW(int w) //设置宽
{
m_W = w;
}
int getW() //获取宽
{
return m_W;
}
void setH(int h) //设置高
{
m_H = h;
}
int getH() //获取高
{
return m_H;
}
//获取面积
int calculateS()
{
return 2 * m_L * m_W + 2 * m_H * m_W + 2 * m_L * m_H;
}
//获取体积
int calculateV()
{
return m_L * m_W * m_H;
}
//利用成员函数判断两个立方体是否相等
bool isSameByClass(Cube &c) //只需要传一个参数
{
if (m_L == c.getL() && m_W == c.getW() && m_H == c.getH())
{
return true;
}
return false;
}
private:
int m_L;
int m_W;
int m_H;
};
int main()
{
Cube c1;
c1.setL(10);
c1.setW(10);
c1.setH(10);
cout << "c1的面积是: " << c1.calculateS() << endl;
cout << "c1的体积是: " << c1.calculateV() << endl;
Cube c2;
c2.setL(11);
c2.setW(10);
c2.setH(10);
int ret = c1.isSameByClass(c2);
if (ret)
{
cout << "成员函数:c1和c2是相等的" << endl;
}
else
{
cout << "成员函数:c1和c2是不相等的" << endl;
}
system("pause");
return 0;
}
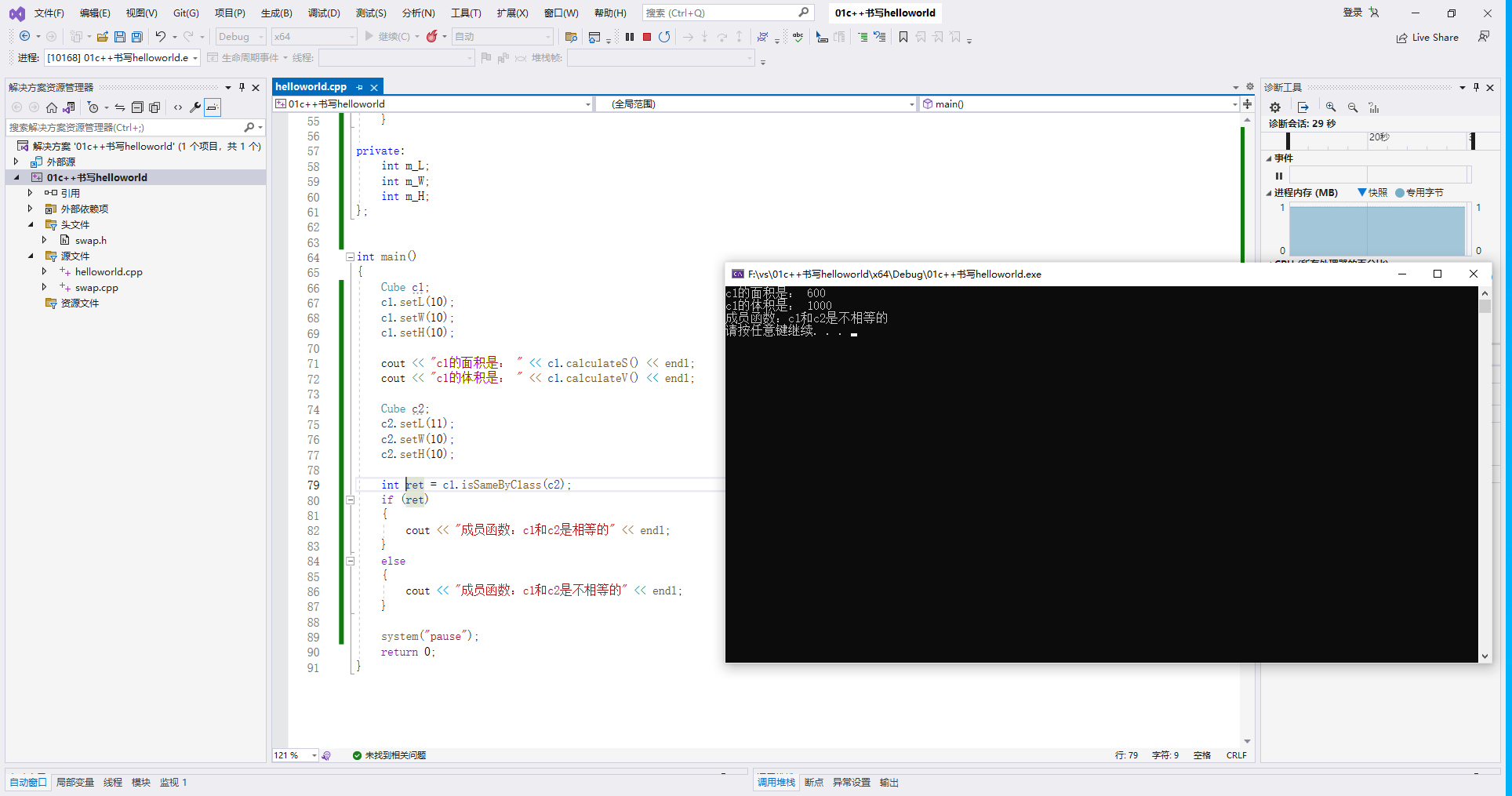