实验名称:实验1:bash脚本编程
- 实验目的
- 1、熟悉Linux系统下bash编程
- 2、利用bash编程完成简易功能
实验内容
- 编写一个脚本文件checkuser,该脚本运行时带一个用户名作为参数,具体要求如下:
- 如果命令行格式不符合要求,应该有错误提示信息。
- 在/etc/passwd 文件中查找是否有该用户,如果有则输出”Foundin the /etc/passwd file”;否则输出“No such user on our system.”。
- 写一个脚本文件printernumber,该脚本运行时带一个数值参数,参数可以包含小数部分。具体要求如下:
- 如果命令行格式不符合要求,应该有错误提示信息。
- 小数点前从个位开始。每三位为一节,节与节之间应该有逗号分开。
实验环境
- VMware
- Ubuntu
实验作业
一、注释与简单命令
一个简单的bash文件想要执行,首先需要被赋予可执行权限,否则无法在系统中运行。 Linux/Unix 的文件调用权限分为三级 : 文件所有者(Owner)、用户组(Group)、其它用户(Other Users),只有文件所有者和超级用户可以修改文件或目录的权限。可以使用绝对模式(八进制数字模式),符号模式指定文件的权限。
语法:chmod [-cfvR] [--help] [--version] mode file...
参数: mode
: 权限设定字串,格式如下 : [ugoa...][[+-=][rwxX]...][,...]
- `u `表示该文件的拥有者,`g` 表示与该文件的拥有者属于同一个群体(group)者,`o`表示其他以外的人,`a` 表示这三者皆是。
- `+` 表示增加权限、`- `表示取消权限、`= `表示唯一设定权限。
- `r `表示可读取,`w` 表示可写入,`x` 表示可执行,X 表示只有当该文件是个子目录或者该文件已经被设定过为可执行。
其他参数说明:
-c : 若该文件权限确实已经更改,才显示其更改动作
-f : 若该文件权限无法被更改也不要显示错误讯息
-v : 显示权限变更的详细资料
-R : 对目前目录下的所有文件与子目录进行相同的权限变更(即以递归的方式逐个变更)
--help : 显示辅助说明
--version : 显示版本
示例代码:
#!/bin/bash
#name:hello
echo 'hello,world'//输出字符串
如果想要运行该脚本程序,需要为他赋予可执行权限:
语句:
$chmod +x hello//控制用户对文件的权限的命令
$./hello //运行生成文件
其中的注释由#
开始,直至行位结束。但是#!/bin/bash
较为特殊,他表示这是一个bash脚本,需要用命令解释器来执行。
运行图:
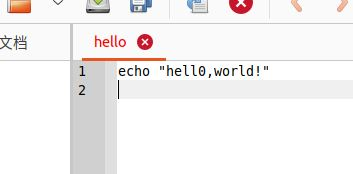

二、环境变量
在bash中定义变量不需要什么数据类型,在使用之前要加上$,使用各种字符的时候要注意空格的使用,否则会对关键字的应用产生影响。
环境变量可以向子进程传递数据:export $msg
此外还有许多的功能,可以联机查看。
测试代码:
msg='hello ,world!'
echo $msg
运行图:
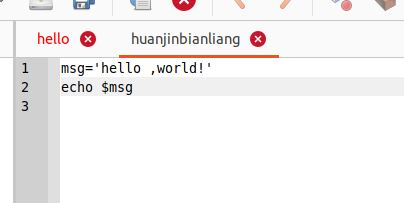

三、控制结构
if语句
语法:
if test-commands
then
Consequent-commands;
else
Other
fi
含义:如果`test-commands`的返回值为0,则执行下一步执行命令,否则进入else。Elif可以有多个,也可以没有。
测试代码:
#!/bin/bash
if [ "${1##*.tar.}"="gz" ];
then
echo It appears to be a tarball zipped by gzip.
else
echo It appears to NOT be a tarball zipped by gzip.
fi
测试图:
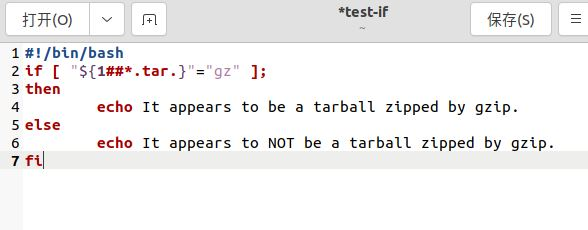

Case语句
语法:
case word in
[[)]pattern[| pattern]...)
Command-list ;;]
.....
esac
含义:寻找与word相匹配的第一个pattern,然后执行对应的命令列表command-list,如果没有匹配任何字符串或模式,则不执行任何代码。
测试代码:
#!/bin/bash
if [ -e "$1" ]
then
case ${1##*.} in
bz2)
tar jxvf $1
;;
gz)
tar zxvf $1
;;
zip)
unzip $1
;;
*)
echo "wrong file type"
;;
esac
else
echo "the file $1 does not exist"
fi
运行图:
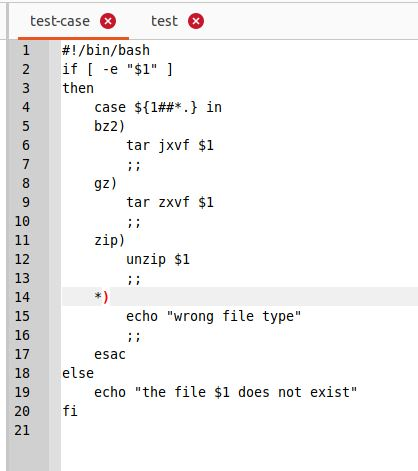

For语句
语法:
for name [in words ...];
do
Commands;
done
含义:对于word中的每一个字符串,都将其赋给变量name,然后执行命令列表sommands
测试代码:
#!/bin/bash
echo $0
echo $#
echo $*
for para in "$@"
do
echo ${para}
done
运行图:
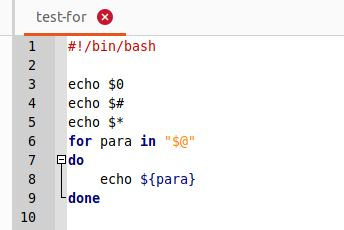
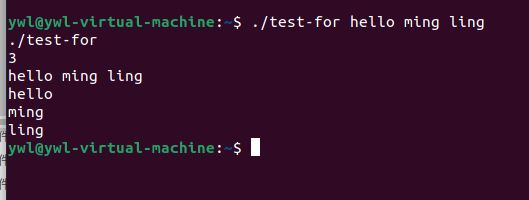
While和until语句
While的语法:
while test-commands;
do
Consequent-commands
done
含义:只要命令列表test-commands的返回结果为0,就执行命令列表,不断重复此过程,直至返回结果为非0;
测试代码:提示用户输入一个字符串,如果输入的是end就结束,否则继续读入
#!/bin/bash
unset var
while [ "$var"!="end" ]
do
echo -n "please input a strin(\"end\" to exit):"
read var
if [ "$var" = "end" ]
then
break
fi
echo "the string you input id $var"
done
运行图:
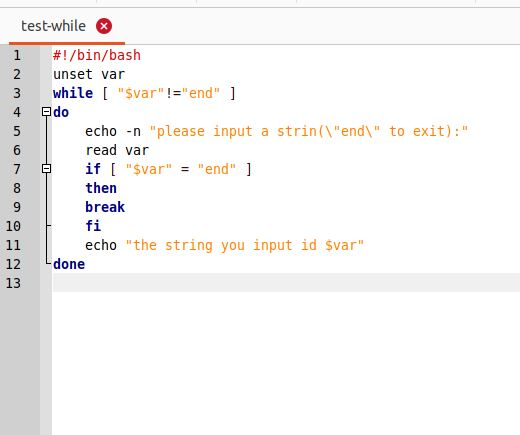
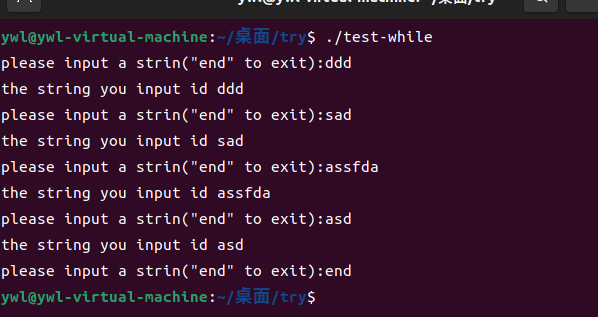
四、函数
在bash脚本中,也可以定义与过程式语言类似的函数,并且bash脚本中的函数也可以接受和处理命令行参数其方式类似于脚本对命令行参数的使用。
运行代码:
#!/bin/bash
myunnzip()
{
if [ -e "$1" ]
then
case ${1##*.tar.} in
bz2)
tar jxvf $1
;;
gz)
tar zxvf $1
;;
*)
echo "wrong file type"
;;
esac
else
echo "the file is not find"
fi
}
myunnzip a.tar.gz
myunnzip input.tar
运行图:
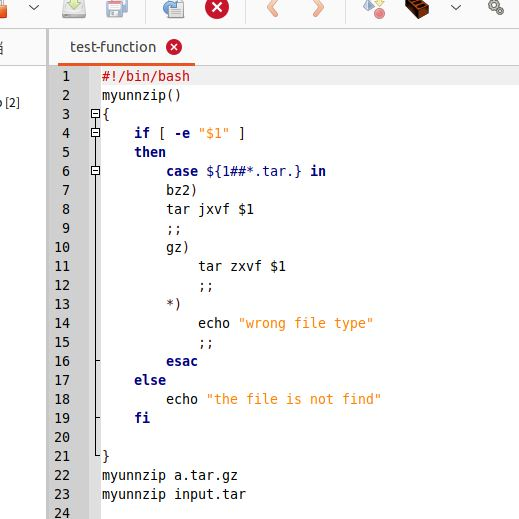

五、实验结果
实验一:checkuser
编写一个脚本文件checkuser,该脚本运行时带一个用户名作为参数,具体要求如下:
- 如果命令行格式不符合要求,应该有错误提示信息。
- 在/etc/passwd 文件中查找是否有该用户,如果有则输出”Foundin the /etc/passwd file”;否则输出“No such user on our system.”。
需求知识点:
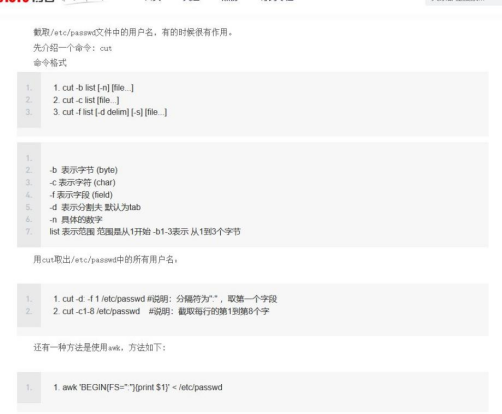
实验代码:
#!/bin/bash
echo input your user name
read username
if [ 'cut /etc/passwd -d: -f1 |grep -c $username' ]
then
echo i can find
else
echo i can not find
fi
运行图:
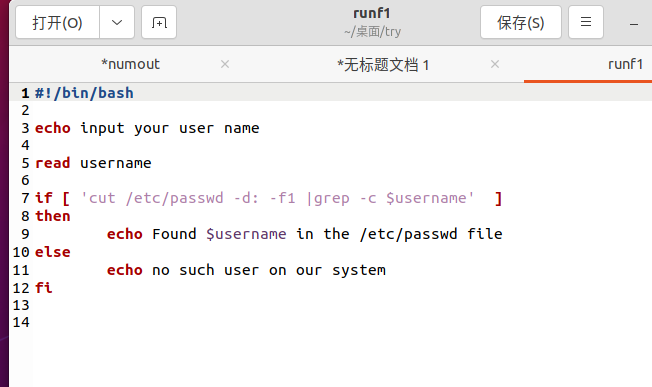
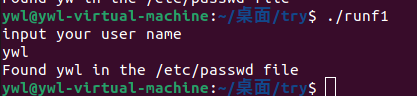
实验二:printnumber
写一个脚本文件printernumber,该脚本运行时带一个数值参数,参数可以包含小数部分。具体要求如下:
- 如果命令行格式不符合要求,应该有错误提示信息。
- 小数点前从个位开始。每三位为一节,节与节之间应该有逗号分开。
运行代码:
#!/bin/bash
if [ $# != 1 ]
then
echo "error"
exit 1
else
printf " '.10g\n" &1
fi
运行图:
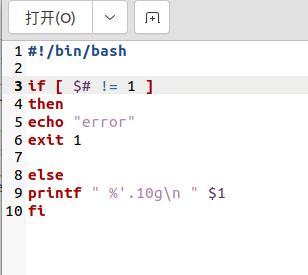

六、实验体会
在本次实验中,我对从课本所学知识进行应用,学到了基础的bash编程,完成了简单的利用。加深了我对bash脚本语言的理解和认识,在实验过程中,更容易记忆和深入理解各种脚本命令以及命令行语言工作还有在bash编程中应该注意的一系列问题。
在本次实验中,揭开了脚本语言神秘的面纱。当然在实验中出现了很多的插曲,查阅书籍和网上咨询才弄懂了他们的来龙去脉,最终完成了练习。开始接触bash语言一头雾水。在看了网上的介绍和讲解后才有所起色,了解到最主要还是命令行的使用以逻辑框架的搭建,才能够自己编写程序。我最深的体会就是对具体的内部细节不熟悉,导致在调试的过程中感觉到很无力。各种命令行的含义不熟悉。在以后要经常将用到的命令的用法温习一下,最后还是能够解决很多的问题的。
本次试验是操作系统实验的lab1。这次的实践对我们的挑战还算不是太大,通过努力,所有的困难被克服掉了。在以后的工作中要的就是我们的实际的动手能力,如果我们在学习期间就是只学了书本上的知识,那样对理论的了解是不够深刻的,只有通过实验才能激发我们的学习兴趣。实验才是检验理论的唯一标准。