uniapp开发小程序,不同角色/已登录未登录,都有不一样的底部导航栏,这些情况下就需要自行定义tabbar,从而实现动态tabbar的实现。
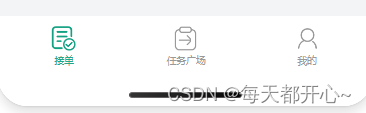
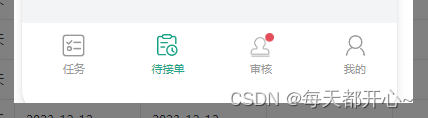
1、如果所有角色加在一起的页面超过5个 那就需要开启custom(自定义) 在pages.json文件中配置 tarBar
// pages.json 文件下
"tabBar": {
"custom": true,
"color": "#999",
"selectedColor": "#21A78A",
"borderStyle": "white",
"backgroundColor": "#ffffff",
"list": [{
"pagePath": "pages/driver/receiveTask"
}, {
"pagePath": "pages/driver/taskPlaza"
}, {
"pagePath": "pages/mine/index"
}, {
"pagePath": "pages/task/company/checkTask"
}, {
"pagePath": "pages/task/company/pending"
}, {
"pagePath": "pages/task/index"
}]
},
2、配置权限路由数据 在 utils文件夹下新建tabBar.js文件
// 货主
const company = [{
"pagePath": "/pages/task/index",
"iconPath": require("@/static/images/tabbar/home.png"),
"selectedIconPath": require("@/static/images/tabbar/home_.png"),
"text": "任务"
}, {
"pagePath": "/pages/task/company/pending",
"iconPath": require("@/static/images/tabbar/pending.png"),
"selectedIconPath": require("@/static/images/tabbar/pending_.png"),
"text": "待接单"
}, {
"pagePath": "/pages/task/company/checkTask",
"iconPath": require("@/static/images/tabbar/checktask.png"),
"selectedIconPath": require("@/static/images/tabbar/checktask_.png"),
"text": "审核"
},{
"pagePath": "/pages/mine/index",
"iconPath": require("@/static/images/tabbar/mine.png"),
"selectedIconPath": require("@/static/images/tabbar/mine_.png"),
"text": "我的"
}
]
// 司机
const driver = [{
"pagePath": "/pages/driver/receiveTask",
"iconPath": require("@/static/images/tabbar/receive.png"),
"selectedIconPath": require("@/static/images/tabbar/receive_.png"),
"text": "接单"
}, {
"pagePath": "/pages/driver/taskPlaza",
"iconPath": require("@/static/images/tabbar/plaza.png"),
"selectedIconPath": require("@/static/images/tabbar/plaza_.png"),
"text": "任务广场"
},{
"pagePath": "/pages/mine/index",
"iconPath": require("@/static/images/tabbar/mine.png"),
"selectedIconPath": require("@/static/images/tabbar/mine_.png"),
"text": "我的"
}
]
// 未注册
const notMember = [{
"pagePath": "/pages/driver/receiveTask",
"iconPath": require("@/static/images/tabbar/receive.png"),
"selectedIconPath": require("@/static/images/tabbar/receive_.png"),
"text": "接单"
}, {
"pagePath": "/pages/driver/taskPlaza",
"iconPath": require("@/static/images/tabbar/plaza.png"),
"selectedIconPath": require("@/static/images/tabbar/plaza_.png"),
"text": "派单广场"
},{
"pagePath": "/pages/mine/index",
"iconPath": require("@/static/images/tabbar/mine.png"),
"selectedIconPath": require("@/static/images/tabbar/mine_.png"),
"text": "我的"
}
]
export default {
company,
driver,
notMember,
}
3、使用VueX 存路由信息
(1)注意在main.js文件中使用store
import App from './App'
import store from './store/index.js'
import uView from "uview-ui";
Vue.use(uView);
Vue.prototype.$store = store
// #ifndef VUE3
import Vue from 'vue'
import './uni.promisify.adaptor'
Vue.config.productionTip = false
App.mpType = 'app'
const app = new Vue({
...App,
store
})
app.$mount()
(2) 新建store文件夹 在文件夹下新建index.js文件
import Vue from 'vue'
import Vuex from 'vuex'
import tarBarUserType from '@/utils/tabBar.js';
Vue.use(Vuex)
const store = new Vuex.Store({
state: {
// 判断是否已登录(member/notMember)
isMemberType: '',
// tabbar列表数据
tabBarList: []
},
mutations: {
setType(state, isMemberType) {
state.isMemberType = isMemberType;
state.tabBarList = tarBarUserType[isMemberType];
}
},
})
export default store
4、自行封装一个tabbar组件
<template>
<view class="tab-bar">
<view class="content">
<view class="one-tab" v-for="(item, index) in tabBarList" :key="index" @click="selectTabBar(item.pagePath)">
<view>
<view class="tab-img">
<image v-if="routePath === item.pagePath" class="img" :src="item.selectedIconPath"></image>
<image v-else class="img" :src="item.iconPath"></image>
</view>
</view>
<view class="tit">
<text v-if="routePath === item.pagePath" style="color: #21A78A;">{{ item.text }}</text>
<text v-else style="color: #999999;">{{ item.text }}</text>
</view>
</view>
</view>
</view>
</template>
<script>
export default {
props: {
// 底部导航栏数据
tabBarList: {
type: Array,
required: true
},
// 当前页面路径
routePath: {
type: String,
required: true
}
},
data() {
return {};
},
mounted() {
// console.log(this.routePath, "this.routePath")
},
methods: {
selectTabBar(path) {
uni.switchTab({
url: path
})
},
}
};
</script>
<style lang="scss">
.tab-bar {
position: fixed;
bottom: 0;
left: 0;
width: 100vw;
padding: 20rpx 10rpx 10rpx;
padding-bottom: calc(5rpx + constant(safe-area-inset-bottom));
padding-bottom: calc(5rpx + env(safe-area-inset-bottom));
background-color: #fff;
z-index: 99999;
.content {
display: flex;
.one-tab {
display: flex;
flex-direction: column;
align-items: center;
width: 50%;
.tab-img {
width: 50rpx;
height: 50rpx;
.img {
width: 100%;
height: 100%;
}
}
.tit {
// font-size: $font-size-base;
transform: scale(0.7);
}
}
}
}
</style>
5、在存在tabbar的页面中都需要引入组件,并传相关数据 (在每个页面都需要在下方额外留出tabbar组件的高度(50px左右))
HTML
<Tabbar :tabBarList="tabBarList" :routePath="routePath"></Tabbar>
JavaScript
import Tabbar from "@/pages/components/tabbar.vue";
data() {
return {
tabBarList: [], // tabbar列表
routePath: "/pages/driver/taskPlaza", // 路由地址 每个有该组件的都需要配置地址
};
},
components: {
Tabbar,
},
created() {
this.tabBarList = this.$store.state.tabBarList; // 获取每个角色对应的tabbarList
},
6、在选择角色时存对应的权限List
import { mapMutations } from 'vuex';
export default {
data() {
return {
};
},
mounted() {
this.beforeEnter()
},
methods: {
...mapMutations(['setType']),
// 选择货主
chooseRolecompany() {
this.setType('company'); // 把路由列表存到vuex中
},
// 选择司机或游客
chooseRoledriver(name) {
// 调用上文vuex
this.setType(name); // 把路由列表存到vuex中
},
},
}
完结~~~~