1.第一个SpringBoot程序
文件目录及组成
在java目录下有Applicaetion为主程序入口
在resources中有application.properties配置文件
在test中有单元测试文件
包的创建和所在目录
新建包必须和Application同级目录,可新建controller,dao,service,pojo
点击application运行即可运行项目(输入localhost:8080可跳转到error页面)
在controller中新建HelloWorld类
package com.wtf.helloworld.controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloControllere {
@RequestMapping("/hello")
public String hello(){
return "hello";
}
}
第一种访问方式(需要借助ideal开发工具)运行,然后在网站中输入localhost:8080/hello即可访问该页面
第二种访问方式(不需要借助ideal开发工具):可以在ideal项目右边的Lifecycle中点击package对项目进行打包,然后在target目录下打包成功,打开cmd窗口使用cd命令进入到该jar包的上一层目录,使用java -jar 包名来运行,然后在网站中输入localhost:8080/hello即可访问该页面
pom.xml依赖文件介绍
parent:继承spring-boot-starter-parent的依赖管理,版本控制和打包
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.6.5</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
spring-boot-starter-web:用于实现HTTP接口(该依赖包含了Spring MVC,使用Tomact作为默认嵌入式容器)
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
build:构建项目配置,使用spring-boot-maven-plugin配合spring-boot-starter-parent来实现项目打包功能
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
springboot项目可以配置热部署(不需要重启服务器)
在application.properties可以进行一些配置
1.修改端口号:server.port=8081
2.修改banner:在resources中新建banner.txt可以修改一些logo
2.自动装配
pom.xml
- spring-boot-depedences :核心依赖在父工程中
- 我们写入一些或者引入一些springboot依赖的时候,不需要指定版本
- 启动器:springboot的启动场景;
比如spring-web-starter-web,会帮助我们自动导入web的所有依赖,要使用什么杨的功能就找到对应的启动器
<dependency
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
主程序
package com.wtf.helloworld;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication //标注这个类时一个springBoot类
public class HelloWorldApplication {
public static void main(String[] args) {
SpringApplication.run(HelloWorldApplication.class, args);
}
}
- 注解
@SpringBootConfiguration :SpringBoot的配置,启动配置类下的所有资源
@configuration:spring的配置
@Component:也是spring的一个组件
@EnableAutoConfiguration:自动配置
@AutoConfigurationPackage:自动配置包
@Import(AutoConfigurationPackage.Registrar.class):自动注册包
@Import({AutoConfigurationImportSelector.class}):自动配置导入选择
@ComponentScan:扫描包
//获取所有的配置
List<String> configurations = this.getCandidateConfigurations(annotationMetadata, attributes);
Spring自动配置的核心文件:在MWTA-INF/spring.factories
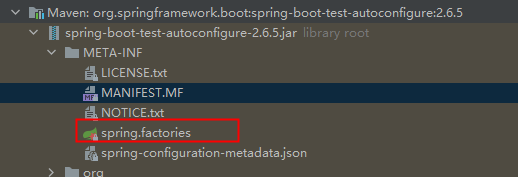
所有的资源会加载到配置类中
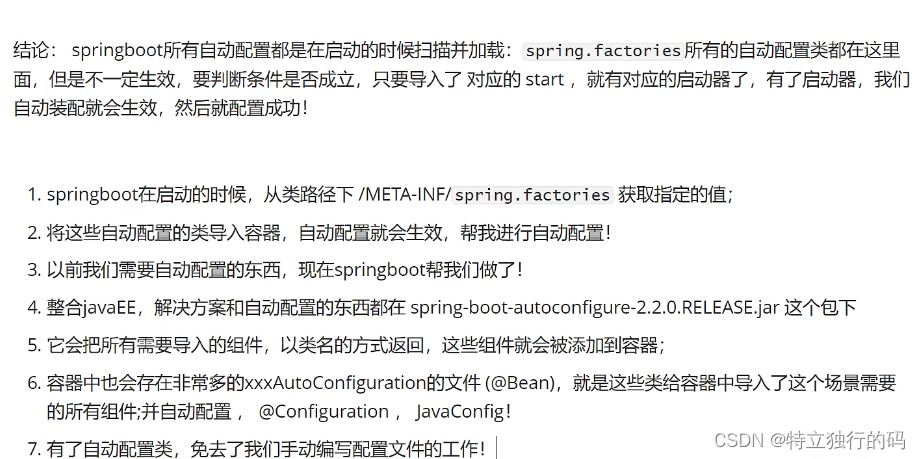
### 3.主启动类怎么运行
```java
//SpringApplication
//run方法
SpringApplication.run(HelloWorldApplication.class, args);
关于SpringBoot,谈谈你的理解A[博客园讲解](spring boot(二):启动原理解析 - 平凡希 - 博客园 (cnblogs.com))
自动装配
run()方法
全面接管SpringMVC的配置
4.SpringBoot的配置(application.yaml)
#对空格要求极为严格
name: wtf
server:
port:8081
#对象
student:
name: wtf
agr: 3
#对象行内写法
student1: {name: wtf,age: 3}
#数组
pets:
- cat
- dog
- pig
pet: [cat,dog,pig]
yaml的作用:
-
可以为对象赋值
-
传统方式:
在包下新建两个实体类,在类名上写入@Componment(加载配置),载属性名上面使用@value(“值”)为对象进行赋值,然后在测试类中使用@Autowired ,private Dog dog 即可加载类
Dog.class
package com.wtf.helloworld.pojo; import org.springframework.beans.factory.annotation.Value; import org.springframework.stereotype.Component; @Component //用组件进行配置,注入到spring中 public class Dog { @Value("小红") //使用Value来进行赋值 private String name; @Value("3") private int age; public Dog() { } public Dog(String name, int age) { this.name = name; this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } @Override public String toString() { return "Dog{" + "name='" + name + '\'' + ", age=" + age + '}'; } }
测试类
@SpringBootTest class HelloWorldApplicationTests { @Autowired //将对象进行自动装配 private Dog dog; @Test void contextLoads() { System.out.printf(dog.toString()); } }
yaml方式赋值
package com.wtf.helloworld.pojo; import org.springframework.boot.context.properties.ConfigurationProperties; import org.springframework.stereotype.Component; import java.util.Date; import java.util.List; import java.util.Map; @ConfigurationProperties(prefix = "Person") @Component public class Person { private String name; private int age; private Boolean happy; private Date birth; private Map<String,Object> maps; private List<Object> lists; private Dog dog; public Person() { } public Person(String name, int age, Boolean happy, Date birth, Map<String, Object> maps, List<Object> lists, Dog dog) { this.name = name; this.age = age; this.happy = happy; this.birth = birth; this.maps = maps; this.lists = lists; this.dog = dog; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public Boolean getHappy() { return happy; } public void setHappy(Boolean happy) { this.happy = happy; } public Date getBirth() { return birth; } public void setBirth(Date birth) { this.birth = birth; } public Map<String, Object> getMaps() { return maps; } public void setMaps(Map<String, Object> maps) { this.maps = maps; } public List<Object> getLists() { return lists; } public void setLists(List<Object> lists) { this.lists = lists; } public Dog getDog() { return dog; } public void setDog(Dog dog) { this.dog = dog; } @Override public String toString() { return "Person{" + "name='" + name + '\'' + ", age=" + age + ", happy=" + happy + ", birth=" + birth + ", maps=" + maps + ", lists=" + lists + ", dog=" + dog + '}'; } }
测试类:
@SpringBootTest class HelloWorldApplicationTests { @Autowired private Dog dog; private Person person; @Test void contextLoads() { System.out.printf(person.toString()); } }
yaml文件
Person: name: wtf age: 22 happy: false birth: 1999/11/01 maps: {ke: v1} lists: - code - music dog: name: 小花 age: 3