JDBC流程
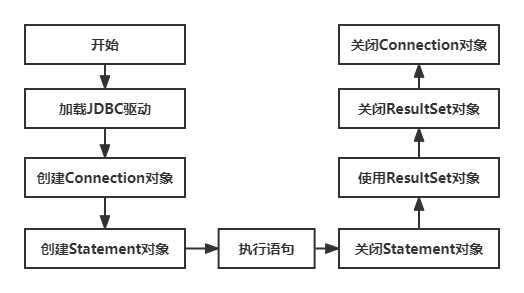
JDBC步骤
编写jdbc.properties文件
driver = oracle.jdbc.driver.OracleDriver
url = jdbc:oracle:thin:@127.0.0.1:1521:orcl
user = epoint
password = Gepoint
driver = com.mysql.jdbc.Driver
url = jdbc:mysql://127.0.0.1:3306/epoint?useUnicode=true&characterEncoding=UTF-8
user = epoint
password = Gepoint
driverClassName=com.mysql.jdbc.Driver
#URL连接数据库的URL,其中travel(以下面例子来说)为连接的数据库,后面的参数可不改但不删
url=jdbc:mysql://127.0.0.1:3306/epoint?useUnicode=true&charaterEncoding=UTF-8
#安装mysql时候设置的用户与密码
username=root
password=Gepoint
#初始化物理连接的个数
initialSize=5
#最大连接池数量
maxActive=10
编写读取文件工具类
public class ConfigUtil {
public static String getValue(String configName) {
if(configName == null || "".equals(configName)) {
return "";
}
String configValue = "";
ResourceBundle bundle = ResourceBundle.getBundle("jdbc");
configValue = bundle.getString(configName);
if(configValue != null) {
configValue = configValue.trim();
}
return configValue;
}
}
编写连接数据库工具类
public class JDBCUtil {
private static String driver = ConfigUtil.getValue("driver");
private static String url = ConfigUtil.getValue("url");
private static String user = ConfigUtil.getValue("user");
private static String password = ConfigUtil.getValue("password");
public static Connection getConnection() {
Connection conn = null;
try {
Class.forName(driver);
conn = DriverManager.getConnection(url, user, password);
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}
return conn;
}
public static void closeConnection(ResultSet rs,Statement stmt,PreparedStatement pstmt, Connection conn) {
try {
if(rs != null)
rs.close();
if(stmt != null)
stmt.close();
if(pstmt != null)
pstmt.close();
if(conn != null)
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
使用Druid数据源连接数据库
private static DataSource datasource;
public DataSource getDataSource() {
if (null == datasource) {
try {
Properties properties = new Properties();
properties.load(JDBCUtils.class.getClassLoader().getResourceAsStream("jdbc.properties"));
datasource = DruidDataSourceFactory.createDataSource(properties);
} catch (Exception e) {
e.printStackTrace();
}
}
return datasource;
}
public static Connection getConnection() {
Connection connection = null;
try {
datasource = new JDBCUtils().getDataSource();
connection = datasource.getConnection();
} catch (SQLException e) {
e.printStackTrace();
}
return connection;
}
public static void closeConn(ResultSet rs, Statement stmt, PreparedStatement pstmt, Connection conn) {
try {
if (null != rs) {
rs.close();
}
if (null != null) {
stmt.close();
}
if (null != pstmt) {
pstmt.close();
}
if (null != conn) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
测试案例
public class Employee {
private String employeeId;
private String name;
private String password;
private int age;
private Double salary;
}
public class EmployeeDao {
public void insertEmp(Employee emp) {
Connection conn = JDBCUtil.getConnection();
PreparedStatement pstmt = null;
String sql = "insert into employee values(?,?,?,?,?)";
try {
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, emp.getEmployeeId());
pstmt.setString(2, emp.getPassword());
pstmt.setString(3, emp.getName());
pstmt.setInt(4, emp.getAge());
pstmt.setDouble(5, emp.getSalary());
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}finally {
JDBCUtil.closeConnection(null, null, pstmt, conn);
}
}
public void updateEmp(Employee emp) {
Connection conn = JDBCUtil.getConnection();
PreparedStatement pstmt = null;
String sql = "update employee set password = ?, name = ?, age = ?, salary = ? where employeeId = ?";
try {
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, emp.getPassword());
pstmt.setString(2, emp.getName());
pstmt.setInt(3, emp.getAge());
pstmt.setDouble(4, emp.getSalary());
pstmt.setString(5, emp.getEmployeeId());
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}finally {
JDBCUtil.closeConnection(null, null, pstmt, conn);
}
}
public void selectEmp(Employee emp) {
Connection conn = JDBCUtil.getConnection();
PreparedStatement pstmt = null;
ResultSet rs = null;
String sql = "select * from employee where employeeId = ?";
try {
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, emp.getEmployeeId());
rs = pstmt.executeQuery();
while(rs.next()) {
Employee emp1 = new Employee();
emp1.setEmployeeId(rs.getString("EmployeeId"));
emp1.setPassword(rs.getString("password"));
emp1.setName(rs.getString("name"));
emp1.setAge(rs.getInt("age"));
emp1.setSalary(rs.getDouble("salary"));
System.out.println(emp1);
}
} catch (SQLException e) {
e.printStackTrace();
}finally {
JDBCUtil.closeConnection(rs, null, pstmt, conn);
}
}
public void selectEmpById(String keyword,int index,int pageSize) {
Connection conn = JDBCUtil.getConnection();
PreparedStatement pstmt = null;
ResultSet rs = null;
String sql = "select * from employee where name like ? limit ?,?";
try {
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, keyword);
pstmt.setInt(2, index);
pstmt.setInt(3,pageSize);
rs = pstmt.executeQuery();
while(rs.next()) {
Employee emp = new Employee();
emp.setEmployeeId(rs.getString("EmployeeId"));
emp.setPassword(rs.getString("password"));
emp.setName(rs.getString("name"));
emp.setAge(rs.getInt("age"));
emp.setSalary(rs.getDouble("salary"));
System.out.println(emp);
}
} catch (SQLException e) {
e.printStackTrace();
}finally {
JDBCUtil.closeConnection(rs, null, pstmt, conn);
}
}
public void deleteEmp(Employee emp) {
Connection conn = JDBCUtil.getConnection();
PreparedStatement pstmt = null;
String sql = "delete from employee where EmployeeId = ?";
try {
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, emp.getEmployeeId());
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}finally {
JDBCUtil.closeConnection(null, null, pstmt, conn);
}
}
public void selectAllEmp() {
Connection conn = JDBCUtil.getConnection();
PreparedStatement pstmt = null;
ResultSet rs = null;
String sql = "select * from Employee";
try {
pstmt = conn.prepareStatement(sql);
rs = pstmt.executeQuery();
while(rs.next()) {
Employee emp = new Employee();
emp.setEmployeeId(rs.getString("EmployeeId"));
emp.setPassword(rs.getString("password"));
emp.setName(rs.getString("name"));
emp.setAge(rs.getInt("age"));
emp.setSalary(rs.getDouble("salary"));
System.out.println(emp);
}
} catch (SQLException e) {
e.printStackTrace();
}finally {
JDBCUtil.closeConnection(rs, null, pstmt, conn);
}
}
使用juint测试
public class JDBCTest {
@Test
public void test() {
Connection conn = JDBCUtil.getConnection();
System.out.println(conn);
}
@Test
public void testInsert() {
EmployeeDao dao = new EmployeeDao();
Employee emp = new Employee("1005", "是四", "1111", 22, 3000.0);
dao.insertEmp(emp);
}
@Test
public void testUpdate() {
EmployeeDao dao = new EmployeeDao();
Employee emp = new Employee("1002","sun","2222",55,8000.0);
dao.updateEmp(emp);
}
@Test
public void testSelect() {
Employee emp = new Employee();
EmployeeDao dao = new EmployeeDao();
emp.setEmployeeId("1002");
dao.selectEmp(emp);
}
@Test
public void testSelectKeyWord() {
EmployeeDao dao = new EmployeeDao();
dao.selectEmpById("%四%", 0, 3);
}
@Test
public void testDelete() {
EmployeeDao dao = new EmployeeDao();
Employee emp = new Employee();
emp.setEmployeeId("1002");
dao.deleteEmp(emp);
}
@Test
public void testSelectAll() {
EmployeeDao dao = new EmployeeDao();
dao.selectAllEmp();
}
}