需求:
某班班要选班长, 采取投票的方式
参选人已经知道了, 有3个, 分别是小强, 小美, 老王
某班共有10个同学, 每个人可以投两票, 不能重复
同学们的票是随机在参选人中选的
最后输出每个同学的票数以及当参选人
实现效果:
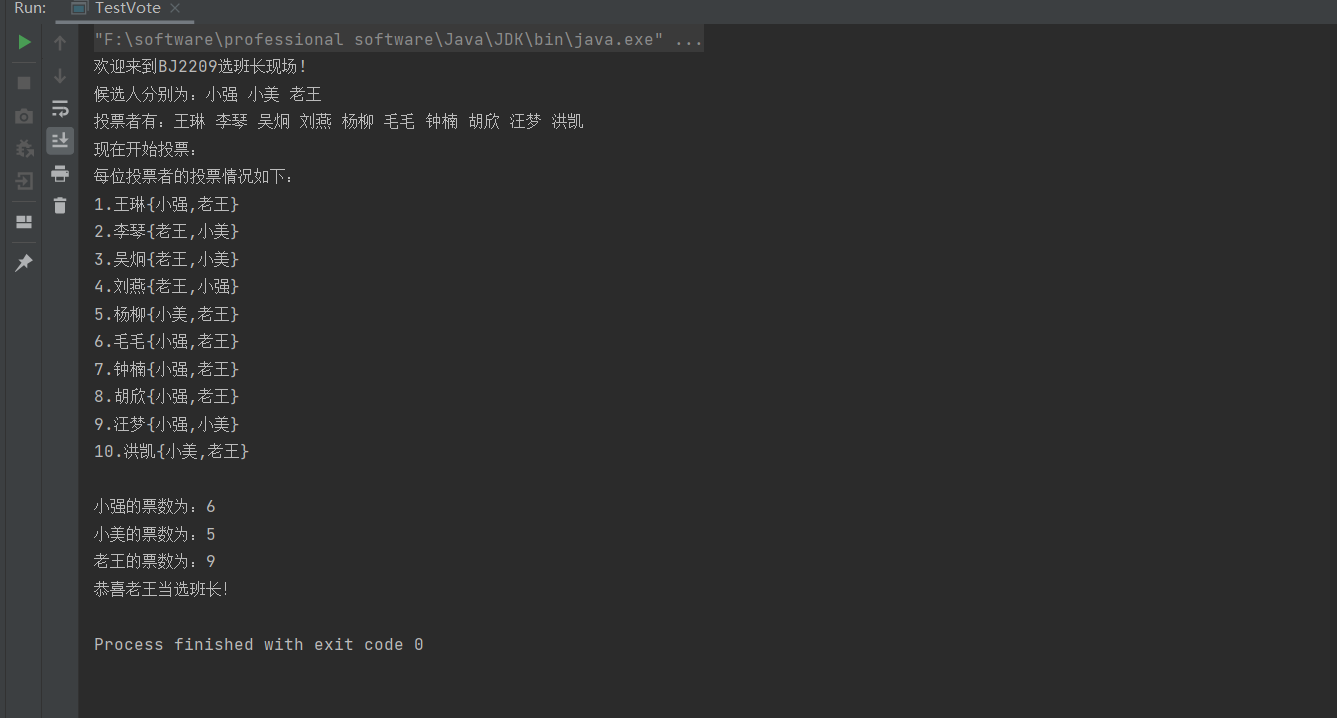
文件结构:
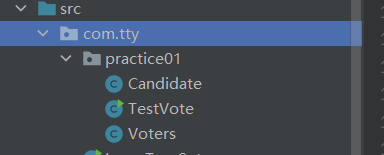
代码实现:
Candidate.java
package com.tty.practice01;
/**
* @ClassName
* @Author mao
* @Description TODO
* @Date 2023/2/21 16:51
*/
public class Candidate {
private int id;
private String name;
private int vote;
public Candidate() {
}
public Candidate(int id, String name, int vote) {
this.id = id;
this.name = name;
this.vote = vote;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getVote() {
return vote;
}
public void setVote(int vote) {
this.vote = vote;
}
@Override
public String toString() {
return "候选人{" +
"姓名='" + name + '\'' +
", 票数=" + vote +
'}';
}
}
Voters.java
package com.tty.practice01;
/**
* @ClassName
* @Author mao
* @Description TODO
* @Date 2023/2/21 17:09
*/
public class Voters {
private String name;
public Voters() {
}
public Voters(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return name;
}
}
TestVote.java
package com.tty.practice01;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
/**
* @ClassName
* @Author mao
* @Description TODO
* @Date 2023/2/21 16:57
*/
public class TestVote {
static ArrayList<Candidate> candidates;
static ArrayList<Voters> voters;
static ArrayList<Integer> ids;
public static void main(String[] args) {
candidates = new ArrayList<>(3);
voters = new ArrayList<>(10);
String[] names = {"王琳", "李琴", "吴炯", "刘燕", "杨柳", "毛毛", "钟楠", "胡欣", "汪梦", "洪凯"};
for (String name : names) {
Voters v = new Voters(name);
voters.add(v);
}
Candidate candidate1 = new Candidate(1, "小强", 0);
Candidate candidate2 = new Candidate(2, "小美", 0);
Candidate candidate3 = new Candidate(3, "老王", 0);
candidates.add(candidate1);
candidates.add(candidate2);
candidates.add(candidate3);
System.out.print("欢迎来到BJ2209选班长现场!\n候选人分别为:" );
for (Candidate candidate : candidates) {
System.out.print(candidate.getName() + " ");
}
System.out.print("\n投票者有:");
for (Voters voter : voters) {
System.out.print(voter.getName() + " ");
}
System.out.println("\n现在开始投票:");
System.out.println("每位投票者的投票情况如下:\n" + vote());
for (int i = 0; i < candidates.size(); i++) {
System.out.println(candidates.get(i).getName() + "的票数为:" + candidates.get(i).getVote());
}
int max = candidates.get(0).getVote() > candidates.get(1).getVote() ? candidates.get(0).getVote() : candidates.get(1).getVote();
max = max > candidates.get(2).getVote() ? max : candidates.get(2).getVote();
for (int i = 0; i < candidates.size(); i++) {
if (max == candidates.get(i).getVote()){
System.out.println("恭喜" + candidates.get(i).getName() + "当选班长!");
}
}
}
//投票
public static String vote(){
String res = "";
int count = 0;
for (int i = 0; i < voters.size(); i++) {
buildList();
res += ++count + "." + voters.get(i).getName() + "{";
switch (ids.get(0)){
case 1:
candidates.get(0).setVote(candidates.get(0).getVote() + 1);
res += candidates.get(0).getName() + ",";
break;
case 2:
candidates.get(1).setVote(candidates.get(1).getVote() + 1);
res += candidates.get(1).getName() + ",";
break;
case 3:
candidates.get(2).setVote(candidates.get(2).getVote() + 1);
res += candidates.get(2).getName() + ",";
break;
}
switch (ids.get(1)){
case 1:
candidates.get(0).setVote(candidates.get(0).getVote() + 1);
res += candidates.get(0).getName() + "}";
break;
case 2:
candidates.get(1).setVote(candidates.get(1).getVote() + 1);
res += candidates.get(1).getName() + "}";
break;
case 3:
candidates.get(2).setVote(candidates.get(2).getVote() + 1);
res += candidates.get(2).getName() + "}";
break;
}
res += "\n";
}
return res;
}
//随机产生不重复长度为2的数组
public static void buildList() {
ids = new ArrayList<>(2);
Random random = new Random();
while (true) {
int num = random.nextInt(3) + 1;
if (!ids.contains(num)) {
ids.add(num);
if (ids.size() == 2) {
break;
}
}
}
}
}