背景图 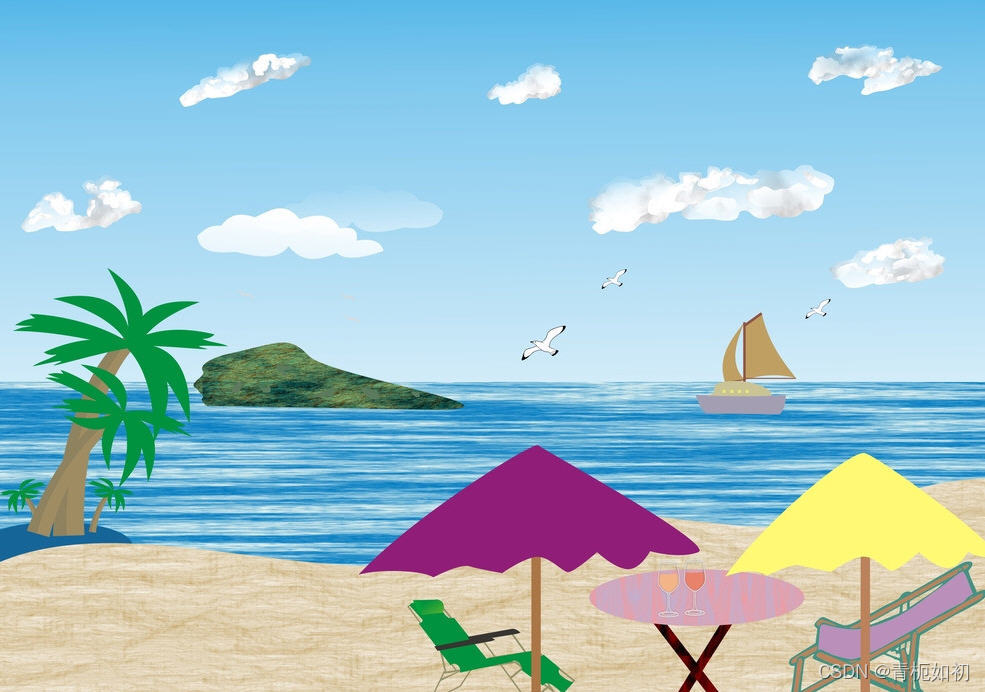
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
display: flex;
justify-content: center;
align-items: center;
width: 100vw;
height: 100vh;
}
#container {
display: flex;
flex-direction: column;
width: 800px;
height: 400px;
border: 1px solid #000;
border-radius: 10px;
box-shadow: 5px 5px 5px #666;
}
#mycanvas {
border: 2px solid #000;
margin-top: 10px;
}
.top {
display: flex;
justify-content: space-between;
align-items: center;
}
.right button {
width: 50px;
}
.active {
background-color: pink;
}
</style>
<body>
<div class="container">
<canvas id="mycanvas" width="800px" height="600px"></canvas>
</div>
</body>
<script>
let canvas = document.querySelector('#mycanvas')
//创建画笔
let ctx = canvas.getContext('2d')
//创建图片元素
let img = new Image()
img.src = './images/clock.jpg'
let req
img.onload = () => {
ctx.strokeStyle = '#00FFFF' //边框颜色
ctx.lineWidth = 5
ctx.arc(300, 300, 200, 0, (Math.PI / 180) * 360, false)//绘制圆弧
ctx.stroke() //调用绘制方法
ctx.clip() //裁剪背景图片
ctx.drawImage(img, 100, 100, 400, 400)//绘图
//时钟
//中心点
Kedu()
//圆心
CircleOrigin()
//时针
Hour()
//分针
Minutes()
//秒针
Seconds()
//绘制时间
Timeing()
req = requestAnimationFrame(img.onload)
}
req = requestAnimationFrame(img.onload)//浏览器在下次重绘之前调用指定的回调函数更新动画
//刻度
function Kedu() {
for (let i = 0; i < 12; i++) {
ctx.save() //保存坐标系
ctx.translate(300, 300)//移动坐标系 改变原点位置
ctx.beginPath() //开始绘制路径
ctx.strokeStyle = '#FFFF00'
ctx.lineWidth = 7
ctx.rotate((30 * i * Math.PI) / 180)
ctx.moveTo(0, -200)
ctx.lineTo(0, -180)
ctx.stroke()
ctx.restore() //恢复到之前保存的坐标系
}
for (let i = 0; i < 60; i++) {
if (i % 5 == 0) continue
ctx.save() //保存坐标系
ctx.translate(300, 300)
ctx.beginPath()
ctx.strokeStyle = '#FFFF00'
ctx.lineWidth = 3
ctx.rotate((6 * i * Math.PI) / 180)
ctx.moveTo(0, -200)
ctx.lineTo(0, -188)
ctx.stroke()
ctx.restore()
}
}
//圆心
function CircleOrigin() {
ctx.save()
ctx.translate(300, 300)//移动坐标系
ctx.beginPath()
ctx.arc(0, 0, 8, 0, Math.PI * 2)
ctx.fillStyle = 'red'
ctx.fill()
ctx.beginPath()
ctx.arc(0, 0, 6, 0, Math.PI * 2)
ctx.fillStyle = '#FFFF00'
ctx.fill()
}
//时针
function Hour() {
ctx.save()
let { hh } = getTime()
let location = getHourLocation(hh)
ctx.rotate((location * Math.PI) / 180)
ctx.beginPath()
ctx.strokeStyle = 'skyblue'
ctx.lineWidth = 6
ctx.moveTo(0, -8)
ctx.lineTo(0, -100)
ctx.stroke()
}
//分针
function Minutes() {
ctx.restore()
let { mm } = getTime()
let location = getMSLocation(mm)
ctx.save()
ctx.rotate((location * Math.PI) / 180)
ctx.beginPath()
ctx.strokeStyle = '#FFFF00'
ctx.lineWidth = 4
ctx.moveTo(0, -8)
ctx.lineTo(0, -135)
ctx.stroke()
}
//秒针
function Seconds() {
ctx.restore()
let { ss } = getTime()
let location = getMSLocation(ss)
ctx.rotate((location * Math.PI) / 180)
ctx.beginPath()
ctx.strokeStyle = 'red'
ctx.lineWidth = 3
ctx.moveTo(0, -8)
ctx.lineTo(0, -160)
ctx.stroke()
//秒针上的圆
ctx.beginPath()
ctx.arc(0, -130, 8, 0, Math.PI * 2)
ctx.fillStyle = 'red'
ctx.fill()
ctx.beginPath()
ctx.arc(0, -130, 6, 0, Math.PI * 2)
ctx.fillStyle = '#FFFF00'
ctx.fill()
ctx.restore()
}
//时间
function Timeing() {
const { hh, mm, ss } = getTime()
//设置样式
ctx.font = '20px 微软雅黑'
ctx.fillStyle = '#fff'
ctx.lineWidth = 5
//获取当前时间
ctx.fillText(`${hh}:${mm}:${ss}`, 260, 420) //实心文字
ctx.lineWidth = 1
ctx.strokeStyle = '#ff0000'
ctx.strokeText('Made in China', 230, 450) //空心文字
}
//得到当前时间
function getTime() {
let nowTime = new Date()
let hh = Zero(parseInt(nowTime.getHours()))
let mm = Zero(parseInt(nowTime.getMinutes()))
let ss = Zero(parseInt(nowTime.getSeconds()))
return { hh, mm, ss }
}
//判断是否加零
function Zero(x) {
return x > 9 ? x : '0' + x
}
//得到当前时针的位置 返回该旋转的角度
function getHourLocation(hh) {
let rotate = (hh % 12) * 30
return rotate
}
//得到当前 分针 或秒针 的位置
function getMSLocation(r) {
let rotate = (r % 60) * 6
return rotate
}
</script>
</html>