案例一:买飞机票
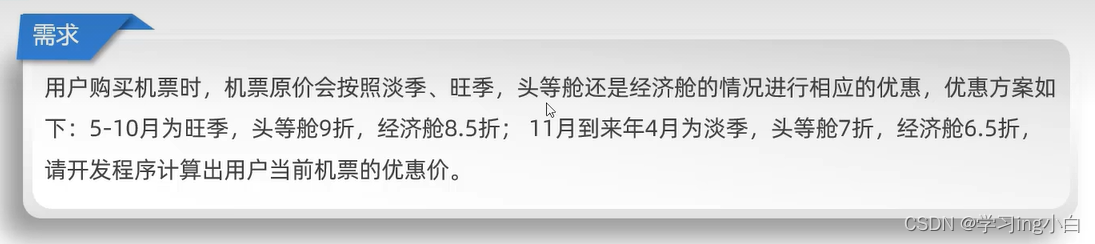
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入机票票价、月份和舱位类型:");
double money = sc.nextDouble();
int month = sc.nextInt();
String level = sc.next();
System.out.println("优惠价:"+price(money,month,level));
}
public static double price(double money,int month,String level){
//if(a)表示a为真时继续执行,if(!a)表示a为假时继续执行
if(month >= 5 && month <= 10){
return level.equals("头等舱") ? money*0.9 : money*0.85;
}else{
return level.equals("头等舱") ? money*0.7 : money*0.65;
}
}
/**
public static double price(double money,int month,String level){
//也可以用switch分支
if(month >= 5 && month <= 10){
switch (level){
case "头等舱":
money = money*0.9;
break;
case "经济舱":
money = money*0.85;
break;
}
}else{
switch (level){
case "头等舱":
money = money*0.7;
break;
case "经济舱":
money = money*0.65;
break;
}
}
return money;
}
*/
案例二:开发验证码

public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入需要的验证码的位数:");
int n = sc.nextInt();
System.out.println("随机验证码:" + checkNum(n));
}
public static String checkNum(int n){
String str = "";
Random r = new Random();
for (int i = 0; i < n; i++) {
//怎么随机生成一个随机字符、可能是数字、大写字母、小写字母?
//思路:随机一个0-1-2,0表示数字、1表示大写字母、2表示小写字母
int type = r.nextInt(3);//可以产生0-1-2的随机数
if(type == 0){
//0表示随机一个数字
str += r.nextInt(10);//0-9
}else if(type == 1){
//1表示随机大写字母 A 65 Z 65+25=90
str += (char)(r.nextInt(26)+65);
}else{
//2表示随机小写字母 a 97 z 97+25=122
str += (char)(r.nextInt(26)+97);
}
}
return str;
}
案例三:评委打分

public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入评委个数(>2):");
int n = sc.nextInt();
int[] arr =new int[n];
for (int i = 0; i < arr.length; i++) {
System.out.println("评委"+(i+1)+"打分:");
arr[i] = sc.nextInt();
}
if(arr.length <= 2){
System.out.println("评委数量不足");
}else{
System.out.println("平均分:"+avg(arr));
}
}
public static double avg(int[] arr){
int max = arr[0];
int min = arr[0];
double sum = arr[0];
for (int i = 1; i < arr.length; i++) {
if(arr[i] > max) {
max = arr[i];
}else if(arr[i] < min){
min = arr[i];
}
sum += arr[i];
}
return (sum-min-max)/(arr.length - 2);
}
案例四:数字加密

public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入需要加密的数字:");
int n = sc.nextInt();
System.out.println("加密结果:"+secret(n));
}
public static int secret(int n){
int x = n; //用x记住原数字
int count = 0;
while(n>0){ //求n是几位数
n=n/10;
count++;
}
int[] arr = new int[count];
int j = 0;
while(x>0){ // 存到数组 + 反转
arr[j] = x%10;
x=x/10;
j++;
}
int sum = 0;
for (int i = 0; i < count; i++) {
arr[i] = (arr[i]+5) % 10;
//Java中没有10^3=1000的乘方运算符,要用pow
sum += arr[i]*pow(10,count-1-i);
}
return sum;
}
案例五:数组拷贝
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int[] arr = {11,22,33,44,55};
int[] newarray = copy(arr);
System.out.print("复制后的结果:[");
for (int i = 0; i < newarray.length; i++) {
System.out.print(i == newarray.length-1 ? arr[i] : arr[i]+",");
}
System.out.print("]");
}
public static int[] copy(int[] arr){
int[] arr_new = new int[arr.length];
for (int i = 0; i < arr.length; i++) {
arr_new[i] = arr[i];
}
return arr_new;
}
案例六:抢红包

public static void main(String[] args) {
int[] arr = {9,666,188,520,99999};
redBag(arr);
}
public static void redBag(int[] arr){
Random r = new Random();
Scanner sc = new Scanner(System.in);
for (int i = 0; i < arr.length; i++) {
System.out.println("请按任意键进行抽奖:");
sc.next(); //等待用户输入内容,按了回车继续往下
//ctrl + alt + T
//用死循环保证该次抽奖一定能抽中,如果随机抽到空红包则继续生成随机数
//这样的程序性能不好
while (true) {
int x = r.nextInt(arr.length);
if(arr[x] != 0){
System.out.println("恭喜您抽中:"+arr[x]);
arr[x] = 0;
break; //结束这次抽奖
}
}
}
System.out.println("活动结束");
}
/**
public static void redBag(int[] arr){
Random r = new Random();
Scanner sc = new Scanner(System.in);
//打乱红包顺序,等下顺序抽取也是随机的
for (int i = 0; i < arr.length; i++) {
int x = r.nextInt(arr.length);
int temp = 0;
temp = arr[x];
arr[x] = arr[i];
arr[i] = temp;
}
for (int i = 0; i < arr.length; i++) {
System.out.println("请按任意键进行抽奖:");
sc.next(); //等待用户输入内容,按了回车继续往下
System.out.println("恭喜您抽中:"+arr[i]);
}
System.out.println("活动结束");
}
*/
案例七:找素数
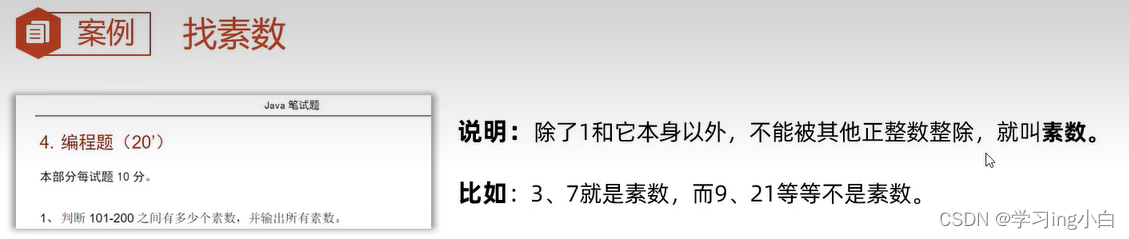
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入您需要寻找素数的范围:");
int a = sc.nextInt();
int b = sc.nextInt();
isSushu(a,b);
}
//方法一
public static void isSushu(int a,int b){
int count = 0;
for (int i = a; i <= b; i++) {
if(i==1){
continue;
}
boolean isSu = true;
for (int j = 2; j <= sqrt(i); j++){
if(i%j==0){
//不是素数
isSu = false;
break;
}
}
if(isSu == true){
count++;
System.out.print(i+" ");
}
}
System.out.println();
System.out.println("总共有:"+count+"个素数");
}
/**
//方法二
public static void isSushu(int a,int b){
int count = 0;
OUT: //为外部循环指定标签
for (int i = a; i <= b; i++) {
if(i==1){
continue;
}
for (int j = 2; j <= sqrt(i); j++){
if(i%j==0){
//不是素数
continue OUT; //直接结束外部循环的当次,进入外部循环的下一次
}
}
count++;
System.out.print(i+" ");
}
System.out.println();
System.out.println("总共有:"+count+"个素数");
}
*/
案例八:打印九九乘法表
public static void main(String[] args) {
for (int i = 1; i <=9 ; i++) {
for (int j = 1; j <= i; j++) {
System.out.print(j+"*"+i+"="+(i*j));
System.out.print(" ");
}
System.out.println();
}
}
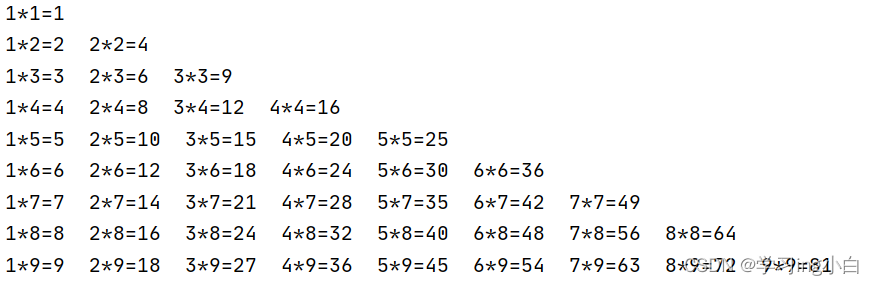
案例九:模拟双色球
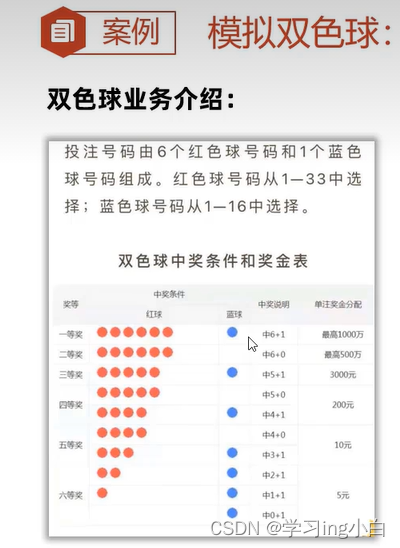
public static void main(String[] args) {
int[] userNumber = userSelect();
int[] luckNumber = luck();
judge(userNumber,luckNumber);
}
public static int[] userSelect(){
int[] arr = new int[7];
Scanner sc = new Scanner(System.in);
for (int i = 0; i < 6; i++) {
while (true) {
System.out.println("请输入您所选择的第"+(i+1)+"个红色球号码(1-33):");
int x = sc.nextInt();
if(x > 33 || x < 1){
System.out.println("您输入的号码不符合规范,请重新输入");
}else{
boolean flag = false;
for (int j = 0; j <= i; j++) {
if(x == arr[j]){
//如果有重复号码
System.out.println("您输入的号码重复,请重新输入");
flag = true;
break;
}
}
if(flag==false){
arr[i] = x;
break;
}
}
}
}
while (true) {
System.out.println("请输入您所选择的蓝色球号码(1-16):");
int y = sc.nextInt();
if(y > 16 || y < 1){
System.out.println("您输入的号码不符合规范,请重新输入");
}else{
arr[6] = y;
break;
}
}
return arr;
}
public static int[] luck(){
int[] arr = new int[7];
Random r = new Random();
for (int i = 0; i < 6; i++) {
while (true) {
int x = r.nextInt(33)+1;
boolean flag = false;
for (int j = 0; j <= i; j++) { //1 2 3 4 0 0
if(x == arr[j]){
//如果有重复号码
System.out.println("您输入的号码重复,请重新输入");
flag = true;
break;
}
}
if(flag==false){
arr[i] = x;
break;
}
}
}
arr[6] = r.nextInt(16)+1;
return arr;
}
public static void judge(int[] userNumber, int[] luckNumber){
printArr(userNumber);
printArr(luckNumber);
int count = 0;
for (int i = 0; i < 6; i++) {
for (int j = 0; j < 6; j++) {
if(userNumber[i] == luckNumber[j]){
count++;
break;
}
}
}
System.out.println("你选中了"+count+"个红球");
boolean flag = false;
if(userNumber[6] == luckNumber[6]){
flag = true;
System.out.println("你选中了蓝色球");
}else{
System.out.println("你没选中了蓝色球");
}
switch (count){
case 0:
if(flag){
System.out.println("中5元");
}else{
System.out.println("没中奖");
}
break;
case 1:
if(flag){
System.out.println("中5元");
}else{
System.out.println("没中奖");
}
break;
case 2:
if(flag){
System.out.println("中10元");
}else{
System.out.println("没中奖");
}
break;
case 3:
if(flag){
System.out.println("中10元");
}else{
System.out.println("没中奖");
}
break;
case 4:
if(flag){
System.out.println("中200元");
}else{
System.out.println("中10元");
}
break;
case 5:
if(flag){
System.out.println("中3000元");
}else{
System.out.println("中200元");
}
break;
case 6:
if(flag){
System.out.println("中1000万元");
}else{
System.out.println("中500万元");
}
break;
default:
System.out.println("没中奖");
break;
}
}
public static void printArr(int[] arr){
System.out.print("[");
for (int i = 0; i < arr.length; i++) {
System.out.print((i==arr.length-1)? arr[i] : arr[i]+" ");
}
System.out.println("]");
}