15.1、环境搭建
结构图:
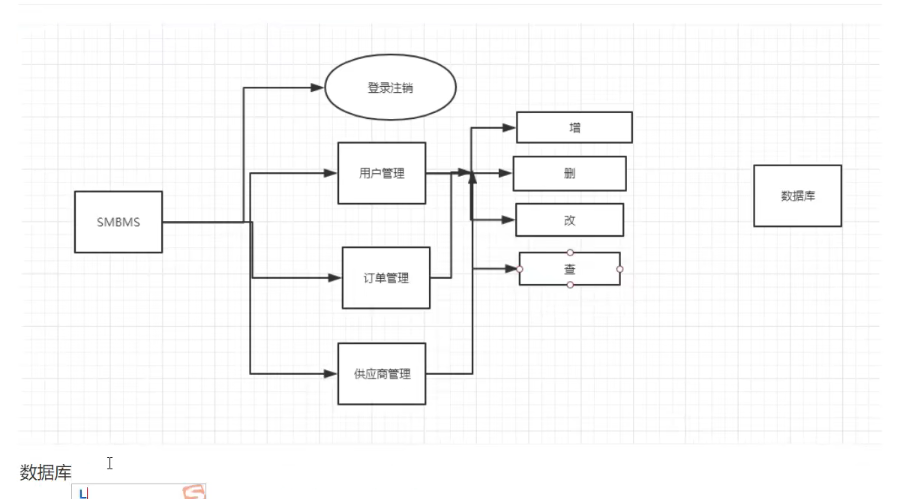
项目如何搭建?
考虑是否使用Maven? 依赖 , jar
项目搭建
1.搭建一个maven项目
2.配置Tomcat
3.测试项目是否能够跑起来
4.导入项目中会遇到的jar
jsp、Servlet、mysql驱动、jstl、stand……
5.创建项目包结构
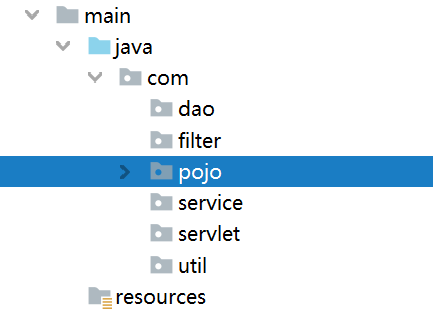
6.编写实体类
ORM映射: 表-类映射
7.编写基础公共类
①数据库配置文件
driver=com.mysql.jdbc.Driver
url=jdbc:mysql://localhost:3306?useUnicode=true&characterEncoding=utf-8
username=root
password=123456
②编写数据库的公共类
package com.dao;
import java.io.InputStream;
import java.sql.*;
import java.util.Properties;
//操作数据库的公共类
public class BaseDao {
private static String driver;
private static String url;
private static String username;
private static String password;
//静态代码块, 类加载的时候就初始化了
static {
Properties properties = new Properties();
//通过类加载器读取对应的资源
InputStream is = BaseDao.class.getClassLoader().getResourceAsStream("db.properties");
try {
properties.load(is);
} catch (Exception e) {
e.printStackTrace();
}
driver = properties.getProperty("driver");
url = properties.getProperty("url");
username = properties.getProperty("username");
password = properties.getProperty("password");
}
//获取数据库的链接
public static Connection getConnection() throws ClassNotFoundException, SQLException {
Class.forName(driver);
Connection connection = DriverManager.getConnection(url, username, password);
return connection;
}
//编写查询公共类
public static ResultSet execute(Connection connection, String sql, Object[] params, ResultSet resultSet, PreparedStatement preparedStatement) throws SQLException {
preparedStatement = connection.prepareStatement(sql);//预解析sql ,后面执行就不需要传入sql语句
for (int i = 0; i < params.length; i++) {
//setObject,占位符从1开始, 但数组是从0开始!
preparedStatement.setObject(i + 1, params[i]);
}
resultSet = preparedStatement.executeQuery();
return resultSet;
}
//编写增删改公共类
public static int execute(Connection connection, String sql, Object[] params, PreparedStatement preparedStatement) throws SQLException {
preparedStatement = connection.prepareStatement(sql);//预解析
for (int i = 0; i < params.length; i++) {
//setObject,占位符从1开始, 但数组是从0开始!
preparedStatement.setObject(i + 1, params[i]);
}
int updateRow = preparedStatement.executeUpdate();
return updateRow;
}
//释放资源
public static boolean closeResource(Connection connection, PreparedStatement preparedStatement, ResultSet resultSet) {
boolean flag = true;
if (resultSet != null) {
try {
resultSet.close();
//GC回收(垃圾回收)
resultSet = null;
} catch (SQLException e) {//如果关闭出现错误就令flag=false表示关闭出现问题
e.printStackTrace();
flag = false;
}
}
if (preparedStatement != null) {
try {
preparedStatement.close();
//GC回收(垃圾回收)
preparedStatement = null;
} catch (SQLException e) {//如果关闭出现错误就令flag=false表示关闭出现问题
e.printStackTrace();
flag = false;
}
}
if (connection != null) {
try {
connection.close();
//GC回收(垃圾回收)
connection = null;
} catch (SQLException e) {//如果关闭出现错误就令flag=false表示关闭出现问题
e.printStackTrace();
flag = false;
}
}
return flag;
}
}
③编写字符编码过滤器
servletRequest.setCharacterEncoding("utf-8");
servletResponse.setCharacterEncoding("utf-8");
filterChain.doFilter(servletRequest, servletResponse);
8.登陆界面
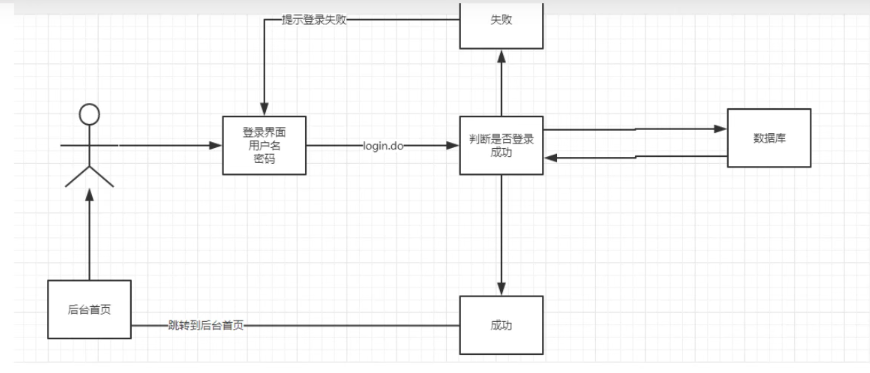
15.2、登陆功能实现
1.编写前端页面
2.设置首页
<!-- 设置欢迎页面-->
<welcome-file-list>
<welcome-file>login.jsp</welcome-file>
</welcome-file-list>
3.编写dao层登陆用户登录的接口
public interface UserDao {
//得到要登录的用户
public User getLoginUser(Connection connection, String userCode) throws SQLException;
}
4.编写实现类
public class UserDaolmpl implements UserDao {
@Override
public User getLoginUser(Connection connection, String userCode) throws SQLException {
PreparedStatement pstm = null;
ResultSet rs = null;
User user = null;
if (connection != null) {
String sql = "select * from smbms.smbms_user where userCode=?";
Object[] params = {userCode};
rs = BaseDao.execute(connection, pstm, rs, sql, params);
if (rs.next()) {
user = new User();
user.setId(rs.getInt("id"));
user.setUserCode(rs.getString("userCode"));
user.setUserName(rs.getString("userName"));
user.setUserPassword(rs.getString("userPassword"));
user.setGender(rs.getInt("userGender"));
user.setBirthday(rs.getDate("userBirthday"));
user.setPhone(rs.getString("phone"));
user.setAddress(rs.getString("address"));
user.setUserRole(rs.getInt("userRole"));
user.setCreateBy(rs.getInt("createBy"));
user.setCreationDate(rs.getDate("creationDate"));
user.setModifyBy(rs.getInt("modifyBy"));
user.setModifyDate(rs.getTimestamp("modifyDate"));
}
BaseDao.closeResource(null, pstm, rs);
}
return user;
}
}
5.业务层接口
public interface UserDao {
//得到要登录的用户
public User getLoginUser(Connection connection, String userCode) throws SQLException;
}
6.业务层实现类
public class UserServiceImpl implements UserService {
//业务层都会调用dao层, 所以我们要引入Dao层;
private UserDao userDao;
public UserServiceImpl() {
userDao = new UserDaoImpl();
}
//
@Override
public User login(String userCode, String password) throws SQLException, ClassNotFoundException {
Connection connection = null;
User user = null;
connection = BaseDao.getConnection();
//通过业务层调用对应的具体的数据库操作
user = userDao.getLoginUser(connection, userCode);
BaseDao.closeResource(connection, null, null);
return user;
}
@Test
public void test() throws SQLException, ClassNotFoundException {
UserServiceImpl userService = new UserServiceImpl();
User admin = userService.login("admin", "741852");
System.out.println(admin.getUserPassword());
}
}
7.编写Servlet
public class LoginServlet extends HttpServlet {
//Servlet: 控制层, 调用业务层代码
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws IOException, ServletException {
System.out.println("LoginServlet--start……");
//获取用户输入的用户名和密码
String userCode = req.getParameter("userCode");
String userPassword = req.getParameter("userPassword");
//和数据库中的密码进行对比, 调用业务层
UserServiceImpl userService = new UserServiceImpl();
User user = null;
try {
user = userService.login(userCode, userPassword);//这里已经将登陆的人查出
} catch (SQLException | ClassNotFoundException e) {
e.printStackTrace();
}
if (user != null) {//查有此人, 可以登陆
//将用户的信息放到Session中;
req.getSession().setAttribute(Constants.USER_SESSION, user);
//跳转到主页
resp.sendRedirect("jsp/frame.jsp");
} else {//查无此人
req.setAttribute("error", "用户名或者密码不正确");
req.getRequestDispatcher("login.jsp").forward(req, resp);
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
}
8.注册Servlet
<!-- LoginServlet-->
<servlet>
<servlet-name>LoginServlet</servlet-name>
<servlet-class>com.servlet.user.LoginServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>LoginServlet</servlet-name>
<url-pattern>/login.do</url-pattern>
</servlet-mapping>
9.测试访问确保以上功能成功
15.3、登陆功能优化
注销功能 :
思路 : 移除Session, 返回登陆页面
①编写过滤器
public class SysFilter implements Filter {
@Override
public void init(FilterConfig filterConfig) throws ServletException {
}
//首页拦截器
@Override
public void doFilter(ServletRequest servletRequest, ServletResponse servletResponse, FilterChain filterChain) throws IOException, ServletException {
HttpServletRequest request = (HttpServletRequest) servletRequest;
HttpServletResponse response = (HttpServletResponse) servletResponse;
//过滤器, 从Session中获取用户
User user = (User) request.getSession().getAttribute(Constants.USER_SESSION);
if (user == null) {//已经被注销或者未登录
response.sendRedirect("/smbms/error.jsp");
}
filterChain.doFilter(servletRequest, servletResponse);
}
@Override
public void destroy() {
}
}
测试、登陆、注销
15.4、密码修改
1.导入前端素材<**li**><**a href="${**pageContext.request.contextPath **}/jsp/pwdmodify"**>密码修改</**a**></**li**>
2.建议从底层往上写
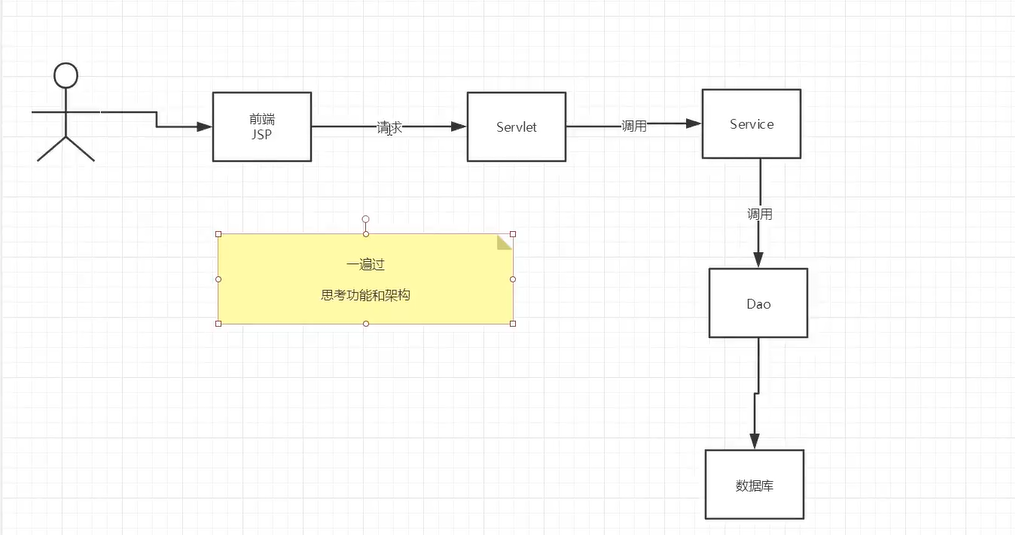
3.编写UserDao接口
//修改当前用户密码
public int updatePwd(Connection connection, int id, int password)throws SQLException;
4.实现UserDaoImpl类
//修改当前用户密码
@Override
public int updatePwd(Connection connection, int id, int password) throws SQLException {
PreparedStatement pstm = null;
int execute = 0;
if (connection != null) {
String sql = "update smbms.smbms_user set userPassword = ? where id = ?";
Object[] params = {password, id};
execute = BaseDao.execute(connection, pstm, sql, params);
BaseDao.closeResource(connection, pstm, null);
}
return execute;//如果>0则修改成功(否则修改失败)
}
5.UsreService接口
//根据用户ID修改密码
public boolean updatePwd(int id, int pwd) throws SQLException, ClassNotFoundException;
6.UsreService实现类
//修改密码的业务层
@Override
public boolean updatePwd(int id, int pwd) throws SQLException, ClassNotFoundException {
Connection connection = null;
boolean flag = false;
//修改密码
connection = BaseDao.getConnection();
if (userDao.updatePwd(connection, id, pwd) > 0) {
flag = true;
BaseDao.closeResource(connection, null, null);
}
return flag;
}
7.编写Servlet(记得实现复用)
//实现复用,就要提取方法
public class UserServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String method = req.getParameter("method");
//修改密码的方法
if (method.equals("savepwd")) {
this.updatePwd(req, resp);
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
public void updatePwd(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//从Session里面拿ID;
Object o = req.getSession().getAttribute(Constants.USER_SESSION);
String newpassword = req.getParameter("newpassword");
boolean flag = false;
if (o != null && newpassword != null && newpassword.length() != 0) {
UserServiceImpl userService = new UserServiceImpl();
try {
flag = userService.updatePwd(((User) o).getId(), newpassword);//调用业务层修改密码
} catch (SQLException | ClassNotFoundException e) {
e.printStackTrace();
}
if (flag) {
req.setAttribute(Constants.MESSAGE, "修改密码成功, 请退出, 使用新密码登录");
//密码修改成功, 移除当前Session, 重新登录
req.getSession().removeAttribute(Constants.USER_SESSION);
req.getRequestDispatcher("login.jsp").forward(req, resp);
} else {
req.setAttribute(Constants.MESSAGE, "修改密码失败");
//修改密码失败
}
} else {
req.setAttribute(Constants.MESSAGE, "新密码有问题");
}
req.getRequestDispatcher("pwdmodify.jsp").forward(req, resp);
}
}
8.注册Servlet
9.优化密码修改使用Ajax
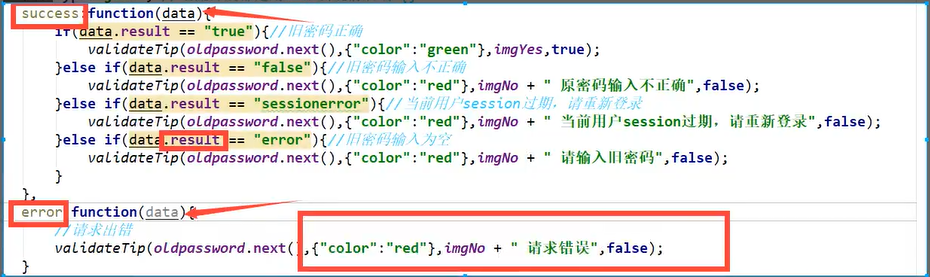
①阿里巴巴的fastjson
<!--阿里巴巴的fastjson-->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.61</version>
</dependency>
②后台代码修改
//记得实现复用,就要提取方法
public class UserServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String method = req.getParameter("method");
//修改密码的方法
if (method.equals("savepwd")) {
this.updatePwd(req, resp);
}else if (method == "pwdmodify") {//验证就密码的方法
this.pwdmodify(req,resp);
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
//修改密码
public void updatePwd(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//从Session里面拿ID;
Object o = req.getSession().getAttribute(Constants.USER_SESSION);
String newpassword = req.getParameter("newpassword");
boolean flag = false;
if (o != null && newpassword != null && newpassword.length() != 0) {
UserServiceImpl userService = new UserServiceImpl();
try {
flag = userService.updatePwd(((User) o).getId(), newpassword);//调用业务层修改密码
} catch (SQLException | ClassNotFoundException e) {
e.printStackTrace();
}
if (flag) {
req.setAttribute(Constants.MESSAGE, "修改密码成功, 请退出, 使用新密码登录");
//密码修改成功, 移除当前Session, 重新登录
req.getSession().removeAttribute(Constants.USER_SESSION);
req.getRequestDispatcher("login.jsp").forward(req, resp);
} else {
req.setAttribute(Constants.MESSAGE, "修改密码失败");
//修改密码失败
}
} else {
req.setAttribute(Constants.MESSAGE, "新密码有问题");
}
req.getRequestDispatcher("pwdmodify.jsp").forward(req, resp);
}
//验证旧密码, 与session中的密码对比即可(用的js中的Ajax实现)
public void pwdmodify(HttpServletRequest req, HttpServletResponse resp) {
//从Session里面拿oldpassword;
Object o = req.getSession().getAttribute(Constants.USER_SESSION);
//获取用户输入的旧密码
String oldpassword = req.getParameter("oldpassword");
//万能的Map: 结果集
HashMap<String, String> resultMap = new HashMap<String, String>();
if (o == null) {//Session失效了, session过期
resultMap.put("result", "sessionerror");
} else if (StringUtils.isNullOrEmpty(oldpassword)) {//输入密码为空
resultMap.put("result", "error");
} else {//可能为真
String userPassword = ((User) o).getUserPassword();//获取Session中的用户密码
if (oldpassword.equals(userPassword)) {//密码正确
resultMap.put("result", "true");
} else {//密码错误
resultMap.put("result", "false");
}
}
try {
resp.setContentType("application/json");
PrintWriter writer = resp.getWriter();
//JSONArray 阿里爸爸的JSON工具类, 转换格式
/*
* resultMap = ["result","sessionerror","result","true"]
* Json格式={key: value}
* */
writer.write(JSONArray.toJSONString(resultMap));
writer.flush();
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
15.5、用户管理实现
思路:
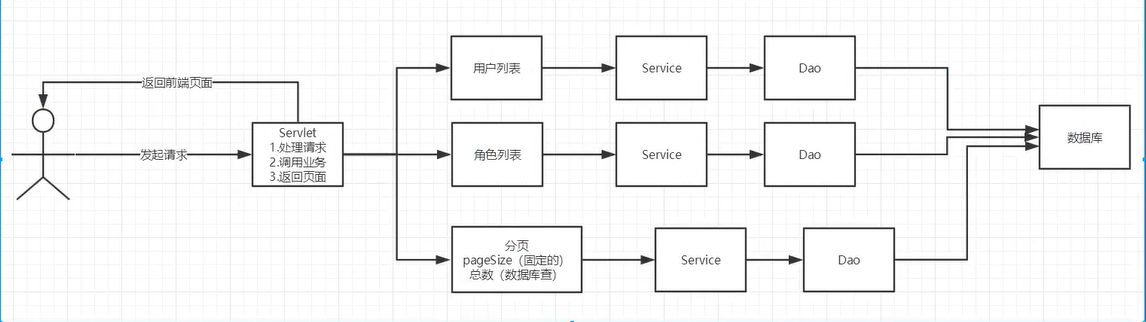
1.导入分页的工具类
PageSupport.java
2.用户列表页面导入
rollpage.jsp
userlist.jsp
获取用户数量
15.5.1、获取用户数量
- UserDao
//根据用户名或者角色 查询用户总数
public int getUserCount(Connection connection, String username, int uerRole) throws SQLException;
- UserDaolmpl
//根据用户名或者角色 查询用户总数(最难的SQL)
@Override
public int getUserCount(Connection connection, String username, int uerRole) throws SQLException {
PreparedStatement pstm = null;
ResultSet re = null;
int count = 0;
if (connection != null) {
StringBuffer sql = new StringBuffer();
ArrayList<Object> list = new ArrayList<Object>();//用来存放参数
sql.append("select count(1) as count from smbms_user as u,smbms_role as r where u.userRole=r.id");//查询所有用户
if (!StringUtils.isNullOrEmpty(username)) {//通过用户名查询
sql.append(" and u.userName like ?");
list.add("%" + username + "%");//模糊查询
}
if (uerRole > 0) {//通过用户角色查询
sql.append(" and u.userRole like ?");
list.add(uerRole);
}
//怎么把List转化为数组
Object[] params = list.toArray();
System.out.println("UserDaoImpl->getUserCount: " + sql.toString());//输出最后完整的SQL语句
re = BaseDao.execute(connection, pstm, re, sql.toString(), params);
if (re.next()) {
count = re.getInt("count");//从结果集中获取最终数量
}
BaseDao.closeResource(null, pstm, re);//数据库还要处理事务,暂时不能关
}
return count;
}
- UserService
//查询记录数
public int getUserCount(String username, int uerRole);
- UserServicelmpl
public int getUserCount(String username, int uerRole) {
Connection connection = null;
int count = 0;
try {
connection = BaseDao.getConnection();
count = userDao.getUserCount(connection, username, uerRole);
} catch (ClassNotFoundException | SQLException e) {
e.printStackTrace();
} finally {
BaseDao.closeResource(connection, null, null);
}
return count;
}
15.5.2、获取用户列表
- UserDao
//根据用户名或者角色 获取用户列表
public List<User> getUserList(Connection connection, String username, int uerRole,int currentPageNo,int pageSize) throws SQLException;
- UserDaolmpl
//根据用户名或者角色 获取用户列表
@Override
public List<User> getUserList(Connection connection, String username, int uerRole, int currentPageNo, int pageSize) throws SQLException {
StringBuffer sql = new StringBuffer();
PreparedStatement pstm = null;
ResultSet re = null;
ArrayList<Object> list = new ArrayList<Object>();//用来存放参数
List<User> userList = new ArrayList<>();//存放用户列表
if (connection != null) {
//sql语句的拼接
sql.append("select u.*,r.roleName as userRoleName from smbms_user as u,smbms_role as r where u.userRole=r.id");//查询所有用户
if (!StringUtils.isNullOrEmpty(username)) {//通过用户名查询
sql.append(" and u.userName like ?");
list.add("%" + username + "%");//模糊查询
}
if (uerRole > 0) {//通过用户角色查询
sql.append(" and u.userRole = ?");
list.add(uerRole);
}
//数据库中,分页使用limit
sql.append(" order by u.creationDate asc limit ?,?");
currentPageNo = (currentPageNo - 1) * pageSize;
list.add(currentPageNo);
list.add(pageSize);
//怎么把List转化为数组
Object[] params = list.toArray();
System.out.println("UserDaoImpl->getUserCount: " + sql.toString());//输出最后完整的SQL语句
re = BaseDao.execute(connection, pstm, re, sql.toString(), params);
while (re.next()) {
User _user = new User();
_user.setId(re.getInt("id"));
_user.setUserCode(re.getString("userCode"));
_user.setUserName(re.getString("userName"));
_user.setGender(re.getInt("userGender"));
_user.setBirthday(re.getDate("userBirthday"));
_user.setPhone(re.getString("phone"));
_user.setAddress(re.getString("address"));
_user.setUserRole(re.getInt("userRole"));
_user.setCreationDate(re.getDate("creationDate"));
_user.setModifyBy(re.getInt("modifyBy"));
_user.setModifyDate(re.getTimestamp("modifyDate"));
_user.setUserRoleName(re.getString("userRoleName"));
userList.add(_user);
}
BaseDao.closeResource(null, pstm, re);//数据库还要处理事务,暂时不能关
}
return userList;
}
- UserService
//根据用户名或者角色 获取用户列表
public List<User> getUserList(String username, int uerRole, int currentPageNo, int pageSize) throws SQLException;
- UserServicelmpl
//根据用户名或者角色 获取用户列表
@Override
public List<User> getUserList(String queryUserName, int queryUserRole, int currentPageNo, int pageSize) throws SQLException {
Connection connection = null;
List<User> userList = null;
System.out.println("queryUserName --> " + queryUserName);
System.out.println("queryUserRole --> " + queryUserRole);
System.out.println("currentPageNo --> " + currentPageNo);
System.out.println("pageSize --> " + pageSize);
try {
connection = BaseDao.getConnection();
userList = userDao.getUserList(connection, queryUserName, queryUserRole, currentPageNo, pageSize);
} catch (ClassNotFoundException e) {
e.printStackTrace();
} finally {
BaseDao.closeResource(connection, null, null);
}
return userList;
}
15.5.3、获取角色操作
为了职责统一, 可以把角色的操作单独放在一个包中, 与POJO类对应
- UserDao
//获取角色列表
public List<Role> getRoleList(Connection connection) throws SQLException;
- UserDaolmpl
//获取角色列表
@Override
public List<Role> getRoleList(Connection connection) throws SQLException {
PreparedStatement pstm = null;
ResultSet resultSet = null;
ArrayList<Role> roleList = new ArrayList<Role>();
if (connection != null) {
String sql = "select * from smbms_role";
pstm = connection.prepareStatement(sql);
Object[] param = {};
resultSet = BaseDao.execute(connection, pstm, resultSet, sql, param);
while (resultSet.next()) {
Role _role = new Role();
_role.setId(resultSet.getInt("id"));
_role.setRoleCode(resultSet.getString("roleCode"));
_role.setRoleName(resultSet.getString("roleName"));
roleList.add(_role);
}
BaseDao.closeResource(null, pstm, resultSet);
}
return roleList;
}
- UserService
//获取角色列表
public List<Role> getRoleList();
- UserServicelmpl
//根据用户名或者角色 获取角色列表
@Override
public List<Role> getRoleList() {
Connection connection = null;
List<Role> roleList = null;
try {
connection = BaseDao.getConnection();
} catch (ClassNotFoundException | SQLException e) {
e.printStackTrace();
} finally {
BaseDao.closeResource(connection, null, null);
}
return roleList;
}
15.5.4、用户显示的Servlet
1.获取用户前端的数据(查询)
2.判断请求是否需要执行, 看参数的值
3.为了实现分页, 需要计算出当前页面和总页面, 页面大小……
4.用户列表展示
5.返回前端
//重点、难点
private void query(HttpServletRequest req, HttpServletResponse resp) throws SQLException, ServletException, IOException {
//查询用户列表
//从前端获取数据
String queryUsername = req.getParameter("queryname");
String temp = req.getParameter("queryUserRole");
String pageIndex = req.getParameter("pageIndex");
//获取用户列表(调用业务层)
UserServiceImpl userService = new UserServiceImpl();
List<User> userList = null;
//第一次走这个请求, 一定是第一页, 页面大小固定
int pageSize = 5;//可以把这个写到配置文件中, 方便后期修改
int currentPageNo = 1;
int queryUserRole = 0;
//第N次走这个请求
if (queryUsername == null) {//如果查询框为空
queryUsername = "";
}
if (temp != null && !temp.equals("")) {//(点击下一页或者上一页)
queryUserRole = Integer.parseInt(temp);//给查询赋值! 0,1,2,3
}
if (pageIndex != null) {//默认值是1, 确定每一页的第一个数据
currentPageNo = Integer.parseInt(pageIndex);
}
//获取用户的总数(分页: 上一页,下一页的情况做准备)
int totalCount = userService.getUserCount(queryUsername, queryUserRole);
//总页数支持
PageSupport pageSupport = new PageSupport();
pageSupport.setCurrentPageNo(currentPageNo);
pageSupport.setPageSize(pageSize);
pageSupport.setTotalCount(totalCount);
int totalPageCount = pageSupport.getTotalPageCount();//一共有几页
//控制首页和尾页
//如果页面小于1,就强制显示第一页
if (currentPageNo < 1) {
currentPageNo = 1;
} else if (currentPageNo > totalPageCount) {//当前页面大于最后一页,强制显示最后一页
currentPageNo = totalPageCount;
}
//获取用户列表展示
userList = userService.getUserList(queryUsername, queryUserRole, currentPageNo, pageSize);
req.setAttribute("userList", userList);//查询出的用户保存在req中即可(不需要用Session)
//获取角色列表
RoleServicelmpl roleServicelmpl = new RoleServicelmpl();
List<Role> roleList = roleServicelmpl.getRoleList();
req.setAttribute("roleList", roleList);//查询出的角色保存在req中即可(不需要用Session)
//存储其他前端需要的信息
req.setAttribute("totalCount", totalCount);
req.setAttribute("currentPageNo", currentPageNo);
req.setAttribute("totalPageCount", totalPageCount);
req.setAttribute("queryUserName", queryUsername);
req.setAttribute("queryUserRole", queryUserRole);
//返回前端
req.getRequestDispatcher("userlist.jsp").forward(req, resp);
}
15.6、增删改用户(必须启用事务)
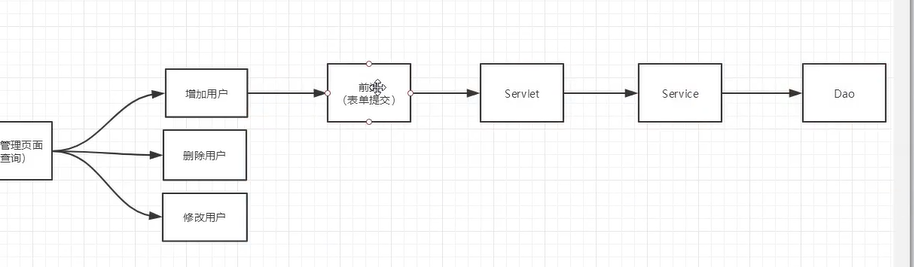
15.7、其余代码全是重复