部分内容参考官网 https://cn.vuejs.org/
vue是一套用于构建用户界面的渐进式JavaScript框架
渐进式——易用、灵活、高效
易用:易于上手
灵活:拥有强大的生态系统
高效:文件大小20kb 加载速度快 DOM操作优化
基于vue实现前后端分离 双向绑定机制(MVVM)
bootstrap是一个css框架 封装css
vue是一个JavaScript框架 简化页面js操作
一、下面通过一个程序简单说明一下vue
<!DOCTYPE html>
<html lang="en">
<head>
<!--js是一门解释型的编程语言 从上到下运行-->
<meta charset="UTF-8">
<title>the first vue page</title>
<script src="js/vue.js"></script>
</head>
<body>
<div id="app">
<h1>The first Vue</h1>
<h1>获取vue中的数据:{{message}}</h1>
{{message}}
<h2>{{count+1}}</h2>
<h3>name的长度为:{{name.length}}</h3>
<h4>name的大写为:{{name.toUpperCase()}}</h4>
</div>
{{message}} <!--如果写在div外面 就不会显示数据-->
</body>
<!--准备script标签书写vue代码-->
<script>
new Vue({
// #id id选择器
el:"#app" , //element 简写 元素 指定当前vue实例的作用范围
data:{ //用于给vue实例绑定数据
message:"hello vue!",
count:19,
name:"zhangqq"
}
});
</script>
</html>
页面展示效果
注意:
1、一张html页面只能定义一个vue实例
2、el属性指定vue实例的作用范围 在vue作用范围内都可以使用vue语法
3、data属性为vue属性绑定一些数据,可以通过{{data中属性的名字}}} 获取对应的属性值
4、在{{}}中可以书写表达式,调用相关方法,以及逻辑运算
5、在绑定vue实例时 支持各种css选择器 最好使用id选择器
二、获取对象类型数据以及数组类型数据
<!DOCTYPE html>
<html lang="en" xmlns:v-bind="http://www.w3.org/1999/xhtml">
<head>
<meta charset="UTF-8">
<title>the second vue page</title>
</head>
<body>
<div id="app">
{{user.id}}------{{user.name}}-------{{user.age}}<br/>
{{names[0]}}------{{names[1]}}-------{{names[2]}}-----{{names[3]}}<br/>
{{users[0]}}<br/>
{{users[1]}}<br/>
{{users[2]}}
</div>
</body>
<script src="js/vue.js"></script>
<script>
new Vue({
el:"#app", //element: 用来指定vue作用范围
data:{
message:"Hello Vue",
//定义对象类型数据
user:{id:"1",name:"xxx",age:18},
//定义数组类型数据
names:["xiaoh","benben","pangp","lanlan"],
users:[
{id:1,name:"小红",age:19},
{id:2,name:"小黄",age:22},
{id:3,name:"小兰",age:24}
]
}
});
</script>
</html>
页面展示效果
三、v-text和v-html的区别
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>the third vue page</title>
</head>
<body>
<div id="app">
<h1>{}{}:{{message}} xx{{message}}xx</h1>
<span v-text="message">{{message}}</span>
<h2 v-html="message"></h2>
<h1 v-text="content"></h1>
<h1 v-html="content"></h1>
</div>
</body>
<script src="js/vue.js"></script>
<script>
new Vue({
el:"#app", //指定vue实例的作用范围
data:{
message:"Hello Vue",
content:"this is text<a href='www.baidu.com'>点我</a>",
}
});
</script>
</html>
页面展示效果
v-text v-html指令 作用:获取vue实例中data属性里的属性 并且放置在指定的标签中
{{}} 和 v-text 指令区别
1、{{}} 取值不会覆盖标签原有的内容(插值表达式) ,v-text取值会覆盖标签原有内容
v-text v-html 取值会覆盖标签原有的内容
2、网络环境较差的情况下 {{}}容易出现插值闪烁 而v-text不会
v-text v-html取值的区别
v-text 等价于 js innerText 获取数据内容时标签也按照文本处理
v-html 等价于 js innerHtml 获取数据内容时会解析html标签
四、事件绑定
<!DOCTYPE html>
<html lang="en" xmlns:v-on="http://www.w3.org/1999/xhtml">
<head>
<meta charset="UTF-8">
<title>the forth vue page</title>
</head>
<body>
<div id="app">
{{message}}<br/>
<button v-on:click="test1()">点我</button>
<button v-on:click="test2()">点我</button>
</div>
</body>
<script src="js/vue.js"></script>
<script>
new Vue({
el:"#app",
data:{
message:"Hello Vue",
},
methods:{
test1:function (){
alert();
},
test2:function (){
alert("666");
}
}
});
</script>
</html>
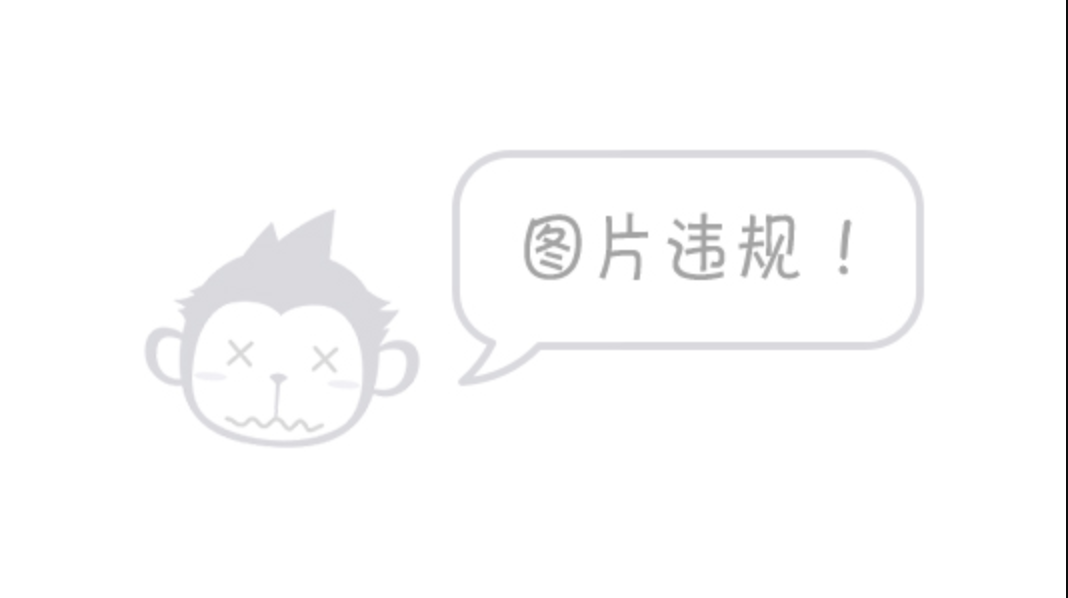
页面展示
事件 js 中的事件编程 3大要素
1、事件源头:发生这个事件的源头标签 html标签
2、事件属性:用户的一些操作 动词(on... onclick、onmouseover....)
3、事件监听:发生事件后处理方案[执行的功能] 函数
vue中也有事件编程 3大要素
绑定事件的语法: v-on:事件属性="监听函数的名字"
函数定义:要定义在vue实例中的method属性中
定义语法: 函数名:function(){
//函数功能
}
五、事件绑定this关键字的使用
<!DOCTYPE html>
<html lang="en" xmlns:v-on="http://www.w3.org/1999/xhtml">
<head>
<meta charset="UTF-8">
<title>the fifth vue page</title>
</head>
<body>
<div id="app">
{{count}}
<br/>
<button v-on:click="test2">点我</button>
</div>
</body>
<script src="js/vue.js"></script>
<script>
new Vue({
el:"#app",
data:{
count:0,
},
methods:{
test2: function () {
/* 将当前data中的count+1 this代表的vue实例 *
* 1、this.data中的属性名 直接获取属性值
* 2、this也可以直接调用当前实例中的其他函数
* */
this.count++;
this.aa();
},
aa:function (){
console.log("冲冲冲~");
}
}
});
</script>
</html>
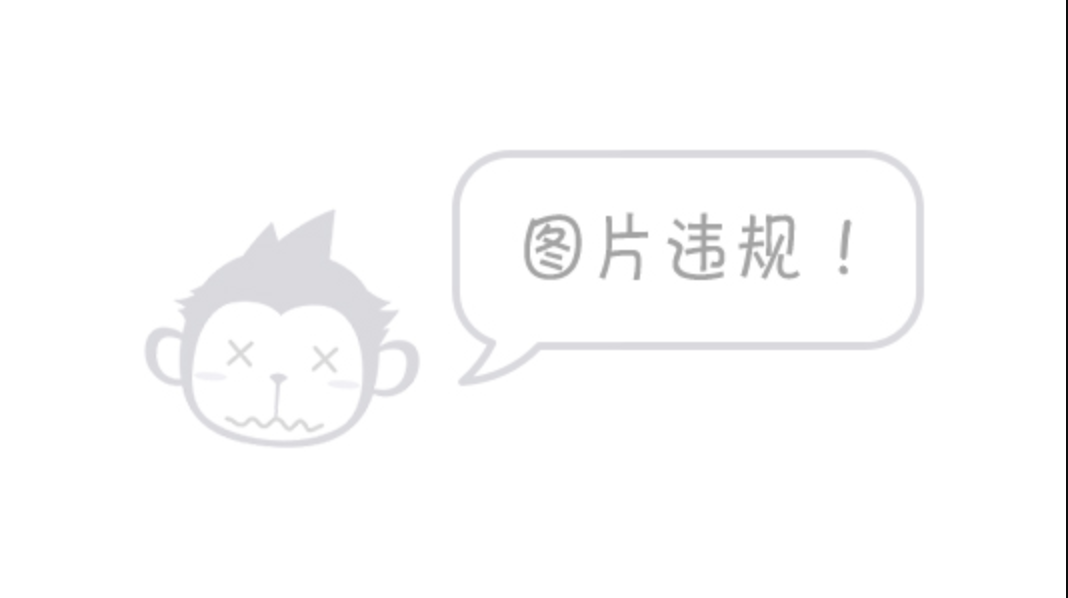
页面展示
六、事件绑定函数中的传递参数
<!DOCTYPE html>
<html lang="en" xmlns:v-on="http://www.w3.org/1999/xhtml">
<head>
<meta charset="UTF-8">
<title>the sixth vue page</title>
</head>
<body>
<div id="app">
<h1>{{count }}</h1>
<h1>{{message}}</h1>
<br/>
<button v-on:click="incr">点击+1</button>
<button v-on:click="addMore(15)">点击+任意</button>
<button v-on:click="addMoreString(50,'xxxx',666,'vue')">点击count+任意,message拼接一个任意字符串</button>
<button v-on:click="addMoreStrings({num:100,value:'xxx',a:true,b:false})">点击count+任意,message拼接一个任意字符串</button>
</div>
</body>
<script src="js/vue.js"></script>
<script>
new Vue({
el:"#app", //指定vue实例的作用范围
data:{ //为实例创建数据
message:"Hello Vue",
count:0,
},
methods:{ //在实例中定义函数
incr:function (){
this.count+=1;
},
addMore:function (num){
this.count+=num;
},
addMoreString:function (num,value,a,b){
this.count+=num;
this.message=this.message+value;
console.log(a);
console.log(b);
},
addMoreStrings:function (obj){
this.count+=obj.num;
this.message=this.message+obj.value;
console.log(obj.a);
console.log(obj.b);
}
}
});
</script>
</html>
展示页面: