1、代码
package LinkList;
public class Linklist {
static class ListNode{
int val;
ListNode next;
public ListNode(int val){
this.val = val;
}
}
ListNode head;
ListNode tail;
int size;
public Linklist(){
head = null;
tail = null;
size = 0;
}
public static void main(String[] args) {
Linklist newList = new Linklist();
newList.appendIntail(5);
newList.appendIntail(7);
newList.appendIntail(4);
newList.appendIntail(2);
newList.printList();
newList.appendInHead(30);
newList.appendInHead(27);
newList.insert(3,9);
newList.printList();
newList.deleteNode(9);
newList.printList();
newList.findInNumber(4);
newList.findInNumber(1);
newList.findInIndex(9);
newList.update(11,2);
newList.printList();
}
public void appendIntail(int number){
ListNode newNode = new ListNode(number);
if(tail == null){
tail = newNode;
head =newNode;
}else {
tail.next = newNode;
tail = newNode;
}
size++;
System.out.println("值为" + number +"的结点成功加入链表!");
}
public void appendInHead(int number){
ListNode newNode = new ListNode(number);
if(tail == null){
head = newNode;
tail = newNode;
System.out.println("头插法成功!");
}else {
newNode.next = head;
head = newNode;
System.out.println("头插法成功!");
}
}
public void insert(int position,int number){
if(position >size){
return;
}
ListNode newNode = new ListNode(number);
if(position == 0){
newNode.next=head;
head = newNode;
if(tail == null){
tail = newNode;
}
size++;
System.out.println("插入成功!");
} else if (position == size){
this.appendIntail(number);
System.out.println("插入成功!");
}else {
ListNode pre = head;
for(int i = 0;i < position-1;i++){
pre = pre.next;
}
newNode.next=pre.next;
pre.next = newNode;
size++;
System.out.println("插入成功!");
}
}
public void printList(){
System.out.print("链表:[");
ListNode cur = head;
while (cur != null){
if(cur.next == null){
System.out.println(cur.val +"]");
cur = cur.next;
return;
}
System.out.print(cur.val + ",");
cur = cur.next;
}
System.out.println(" ");
}
public void deleteNode(int number){
if(head !=null && head.val == number){
head = head.next;
size--;
if(size == 0){
tail = head;
}
System.out.println("删除成功,成功删除值为" + number + "的结点");
}else {
ListNode pre = head;
ListNode cur = head;
while (cur != null){
if(cur.val == number){
if (cur == tail){
pre.next = null;
tail = pre;
size--;
System.out.println("删除成功,成功删除值为" + number + "的结点");
return;
}else {
pre.next = cur.next;
size--;
System.out.println("删除成功,成功删除值为" + number + "的结点");
return;
}
}else {
pre = cur;
cur = cur.next;
}
}
System.out.println("删除失败,没有该结点");
return;
}
}
public void findInNumber(int number){
ListNode p = head;
while (p != null){
if(p.val == number){
System.out.println("查找成功!");
return;
}else {
p = p.next;
}
}
System.out.println("不存在该数,查找失败!");
return;
}
public void findInIndex(int index){
if(index > size){
System.out.println("查找失败,位置非法!");
}else {
ListNode cur = head;
int i = 0;
while (i < index){
cur = cur.next;
i++;
}
System.out.println("查找成功,查找到的数值为:" + cur.val);
}
}
public int update(int oldValue,int newValue){
int i = 1;
ListNode cur = head;
while (cur != null){
if(cur.val == oldValue){
cur.val = newValue;
System.out.println("更新成功!");
return i;
}else {
i++;
cur = cur.next;
}
}
System.out.println("更新失败!不存在值为" + oldValue + "的结点");
return -1;
}
}
2、结果
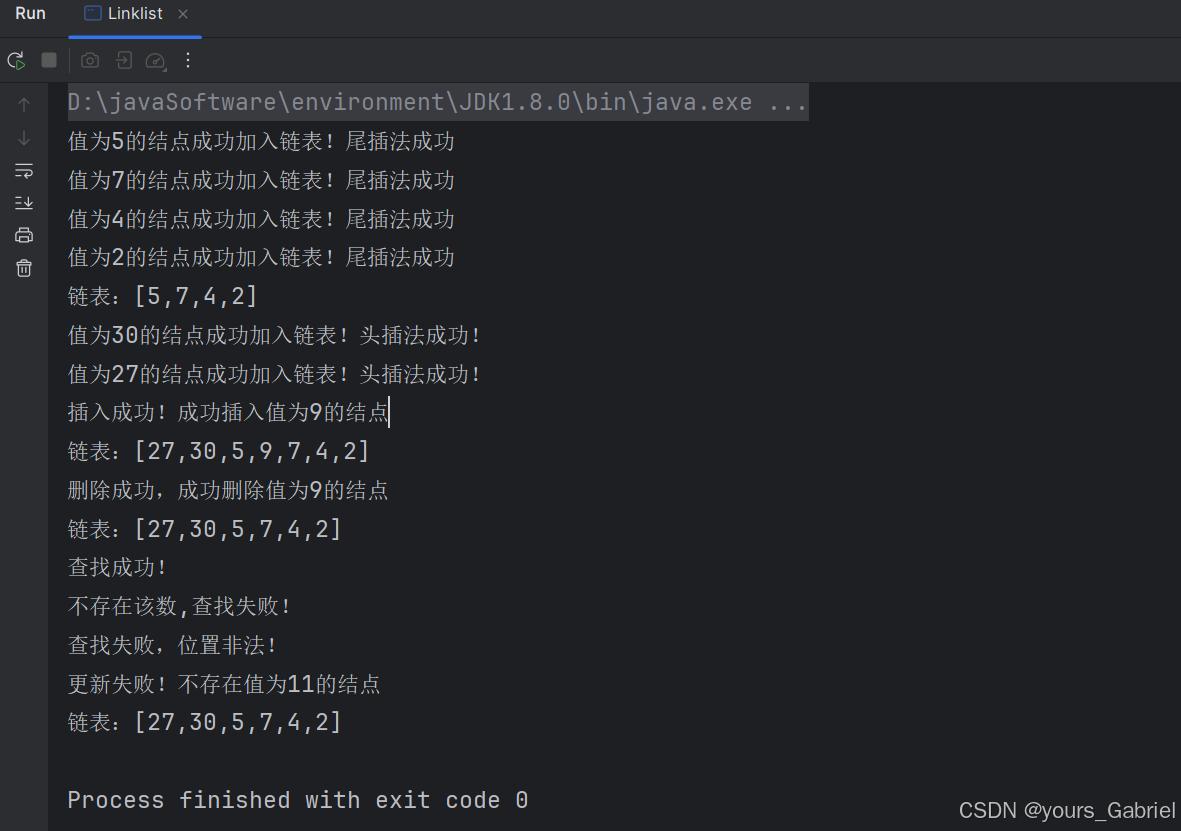