1.创建Game类
import Map from './Map.js';
import Time from './Time.js';
import Wolf from './Wolf.js';
class Game {
constructor(e1, width, height) {
this.e1 = e1;
this.width = width;
this.height = height;
//初始化Canvas
this.initCanvas();
//初始化Map类
this.map = new Map();
//初始化Wolf类
this.wolf = new Wolf();
//初始化Time类
this.time = new Time();
this.wolf.score = 0;
}
initCanvas() {
//在div里创建canvas标签
let canvas = document.createElement('canvas');
//设置canvas的宽高
canvas.width = this.width;
canvas.height = this.height;
this.context = canvas.getContext('2d');
//放在哪个div标签里面,需要传标签id
this.e1.append(canvas);
//给canvas添加点击事件
canvas.onclick = () => {
//1.获取鼠标位置event.offsetX,event.offsetY
let x = event.offsetX;
let y = event.offsetY;
//2.再判断鼠标点击的位置是否在狼的图片上面
this.wolf.canvasClick(x, y);
}
}
start() {
this.time.tid = setInterval(() => {
//清除画布
this.context.clearRect(0, 0, this.width, this.height);
//绘制地图
this.map.draw(this.context, this.width, this.height);
//绘制狼
this.wolf.draw(this.context);
//绘制分数
this.context.font = "30px asa";
this.context.fillStyle = 'red';
this.context.fill();
this.context.fillText(this.wolf.score, 100, 50);
//绘制时间
this.time.draw(this.context);
}, 300)
}
}
export default Game;
2.创建一个背景图片的类在Canvas上绘制出来
class Map {
constructor() {
this.initImage();//初始化图片
}
initImage() {
this.img = new Image();
this.img.src = "./img/game_bg.jpg";
}
draw(context, width, height) {
context.drawImage(this.img, 0, 0, width, height);
}
}
export default Map;
3.创建灰太狼类,绘制出灰太狼在洞里的坐标,实现打击效果
class Wolf {
constructor() {
this.initData();//初始化数据
this.initIamge();//初始化图片
}
initData() {
// 狼的位置坐标
this.positionArr = [
{ x: 170, y: 245 },
{ x: 50, y: 320 },
{ x: 45, y: 420 },
{ x: 70, y: 540 },
{ x: 200, y: 506 },
{ x: 330, y: 545 },
{ x: 320, y: 405 },
{ x: 305, y: 290 },
{ x: 200, y: 510 }
];
//狼图片下标
this.index = 0;
//产生一个随机数(随机洞位置的下标)
this.posIndext = Math.floor(Math.random() * this.positionArr.length);
//产生一个随机数(狼的类型 0:大灰太狼,1:小灰太狼)
this.type = Math.round(Math.random());
this.state = 0;//状态是否打击到了狼 0没打击到,1打击到了
}
initIamge() {
//大灰太狼图片
let bigWolfUrl = [
//展示图片
'./img/wolf/h0.png',
'./img/wolf/h1.png',
'./img/wolf/h2.png',
'./img/wolf/h3.png',
'./img/wolf/h4.png',
'./img/wolf/h5.png',
//打击图片
'./img/wolf/h6.png',
'./img/wolf/h7.png',
'./img/wolf/h8.png',
'./img/wolf/h9.png'
];
this.bigImage = [];
bigWolfUrl.forEach(item => {
let img = new Image();
img.src = item;
this.bigImage.push(img);
})
//小灰太狼图片
let smallWolfUrl = [
//展示图片
'./img/wolf/x0.png',
'./img/wolf/x1.png',
'./img/wolf/x2.png',
'./img/wolf/x3.png',
'./img/wolf/x4.png',
'./img/wolf/x5.png',
//打击图片
'./img/wolf/x6.png',
'./img/wolf/x7.png',
'./img/wolf/x8.png',
'./img/wolf/x9.png'
];
this.smallImage = [];
smallWolfUrl.forEach(item => {
let img = new Image();
img.src = item;
this.smallImage.push(img);
});
this.allImage = [this.bigImage, this.smallImage];
}
draw(context) {
context.drawImage(this.allImage[this.type][this.index], this.positionArr[this.posIndext].x, this.positionArr[this.posIndext].y);
switch (this.state) {
case 0://没打击到
//判断狼的图片展示是否完成
if (++this.index == 6) {
this.index = 0;
this.posIndext = Math.floor(Math.random() * this.positionArr.length); //狼动态展示完后,重新产生一个随机洞位置的下标)
this.type = Math.round(Math.random());//重新产生一个狼类型的随机数 0大灰太狼,1小灰太狼)
}
break;
case 1://打击到
if (++this.index == 10) {
this.index = 0;
this.state = 0;
this.posIndext = Math.floor(Math.random() * this.positionArr.length); //狼动态展示完后,重新产生一个随机洞位置的下标)
this.type = Math.round(Math.random());//重新产生一个狼类型的随机数 0大灰太狼,1小灰太狼)
}
break;
}
}
canvasClick(x, y) {
// 判断鼠标点击的位置是否在狼的图片上面
if (x > this.positionArr[this.posIndext].x &&
x < this.positionArr[this.posIndext].x + 108 &&
y > this.positionArr[this.posIndext].y &&
y < this.positionArr[this.posIndext].y + 101) {
//打击到了,播放6~9的图片
console.log("打击到了");
this.index = 6;
this.state = 1;
if (this.type == 0) {
this.score += 10;
}
else {
this.score -= 10;
}
}
}
}
export default Wolf;
4.创建一个进度条类,显示游戏还有多久结束
class Time {
constructor() {
this.initImage();
this.timeimgw = 270;
}
initImage() {
this.img = new Image();
this.img.src = "./img/progress.png";
}
draw(context) {
context.drawImage(this.img, 95, 110, this.timeimgw, 28);
this.timeimgw -= 7;
if (this.timeimgw < -7) {
context.clearRect(0, 0, 480, 800);
context.font = "30px asa";
context.fillStyle = 'red';
context.fill();
context.fillText("GAME OVER!", 100, 50);
//清除定时器
clearInterval(this.tid);
}
}
}
export default Time;
5.主体部分
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
</style>
</head>
<body>
<button>开始游戏</button>
<div id="game">
</div>
<script type="module">
import Game from './js/Game.js';
let game = new Game(document.querySelector('#game'), 480, 800);
document.querySelector('button').onclick = function () {
game.start();
}
</script>
</body>
</html>
运行效果
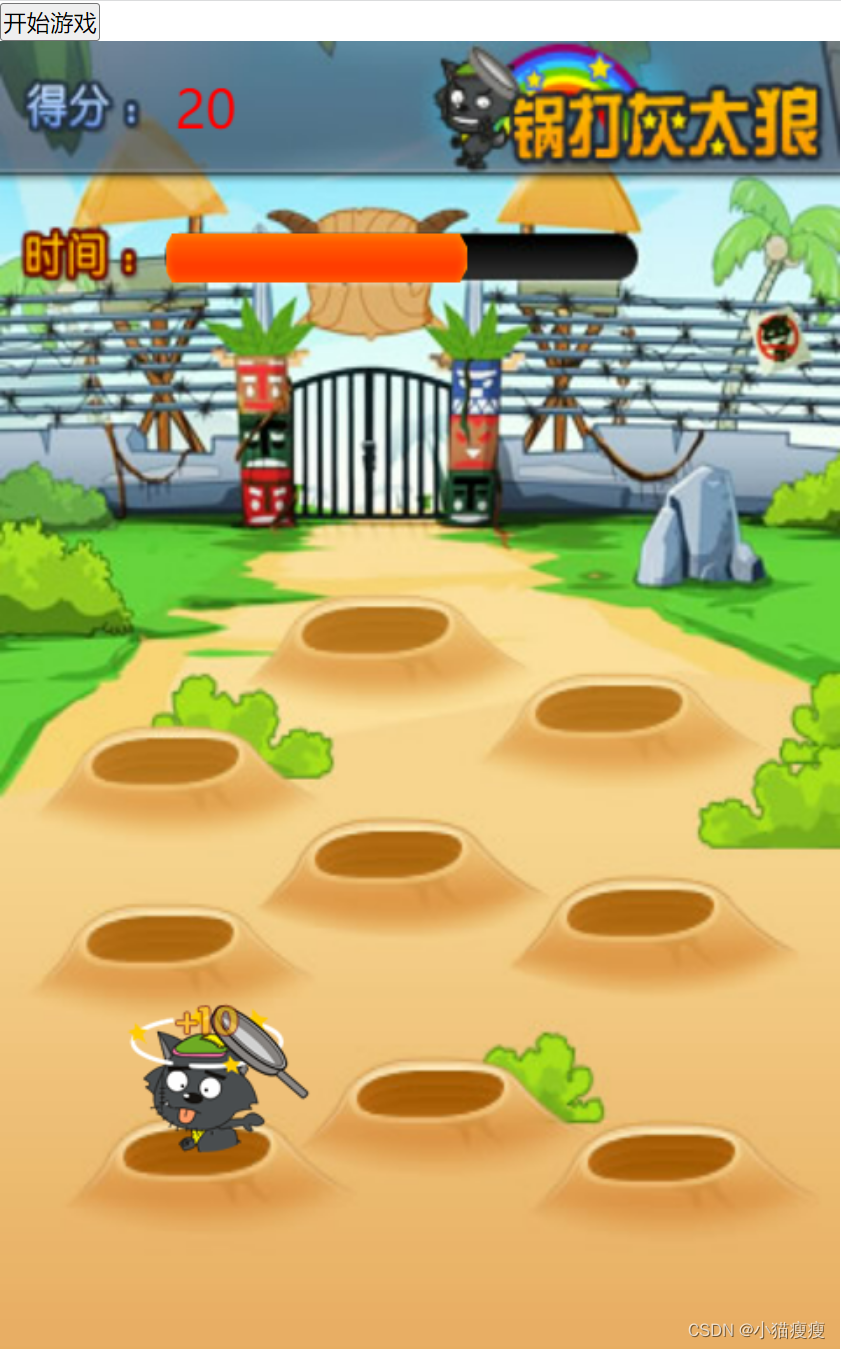
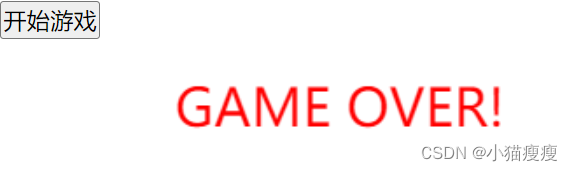