思路:采用层次遍历,设置一个变量last记录当前层最右结点的下标,每次循环与last比较,相等,则层数+1,并且让last指向下一层最右结点下标,直到退出循环,level即为高度
样例:
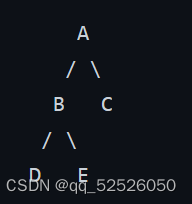
一、非递归方法
//二叉树结构体
typedef struct bitree {
char data;
struct bitree* lchild, * rchild;
}bitree;
//队列
typedef struct {
bitree* data[50];
int front, rear;
}sequeue;
//二叉树的创建
bitree* create(bitree* t) {
char ch;
scanf("%c", &ch);
if (ch =='#') {
return NULL;
}
else {
t = (bitree*)malloc(sizeof(bitree));
t->data = ch;
t->lchild = create(t->lchild);
t->rchild = create(t->rchild);
return t;
}
}
//计算二叉树的深度
int Btdepth(bitree* t) {
if (t == NULL) {
return 0;
}
int last = 0, level = 0;//last 指向最右层的结点,level记录高度
sequeue s;
s.front = s.rear = 0; //队列初始化
bitree* p = t;
s.data[(s.rear)++] = p; //根节点入队
while (s.front != s.rear) {
p = s.data[(s.front)++];
if (p->lchild != NULL) {
s.data[(s.rear)++] = p->lchild;
}
if (p->rchild != NULL) {
s.data[(s.rear)++] = p->rchild;
}
if (last == s.front - 1) { //这里减一是因为,front是在赋值结束之后会才会自增,所以-1
level++;
last = s.rear - 1; //last记录下一层的最后一个结点的下标
}
}
return level;
}
int main() {
bitree* t = NULL;
t = create(t);
int level = Btdepth(t);
printf("该二叉树的高度:%d", level);
return 0;
}
一、递归方法
#define _CRT_SECURE_NO_WARNINGS
#include<stdio.h>
#include<string.h>
#include <stdlib.h>
typedef struct bitree {
char data;
struct bitree* lchild, * rchild;
}bitree;
bitree* create(bitree* t) {
char ch;
scanf("%c", &ch);
if (ch == '#') {
return NULL;
}
else {
t = (bitree*)malloc(sizeof(bitree));
t->data = ch;
t->lchild = create(t->lchild);
t->rchild = create(t->rchild);
return t;
}
}
int Btdepth(bitree* t) {
if (t == NULL) {
return 0;
}
int ldep = Btdepth(t->lchild);
int rdep = Btdepth(t->rchild);
if (ldep > rdep)
return ldep + 1;
else
return rdep + 1;
}
int main() {
bitree* t = NULL;
t = create(t);
int level = Btdepth(t);
printf("二叉树的高度为:%d", level);
return 0;
}
结果: