MybtaisPlus概述
学习MyBatis-Plus之前要先学MyBatis–>Spring—>SpringMVC
为什么要学它?MyBatisPlus可以节省我们大量的时间,所有CRUD代码都可以自动完成
JPA、 tk-mapper 、MyBatisPlus
偷懒用的!
简介
是什么?Mybatis就是为了简化JDBC操作的!
官网:https://baomidou.com/ MybtisPlus, 简化Mybatis!
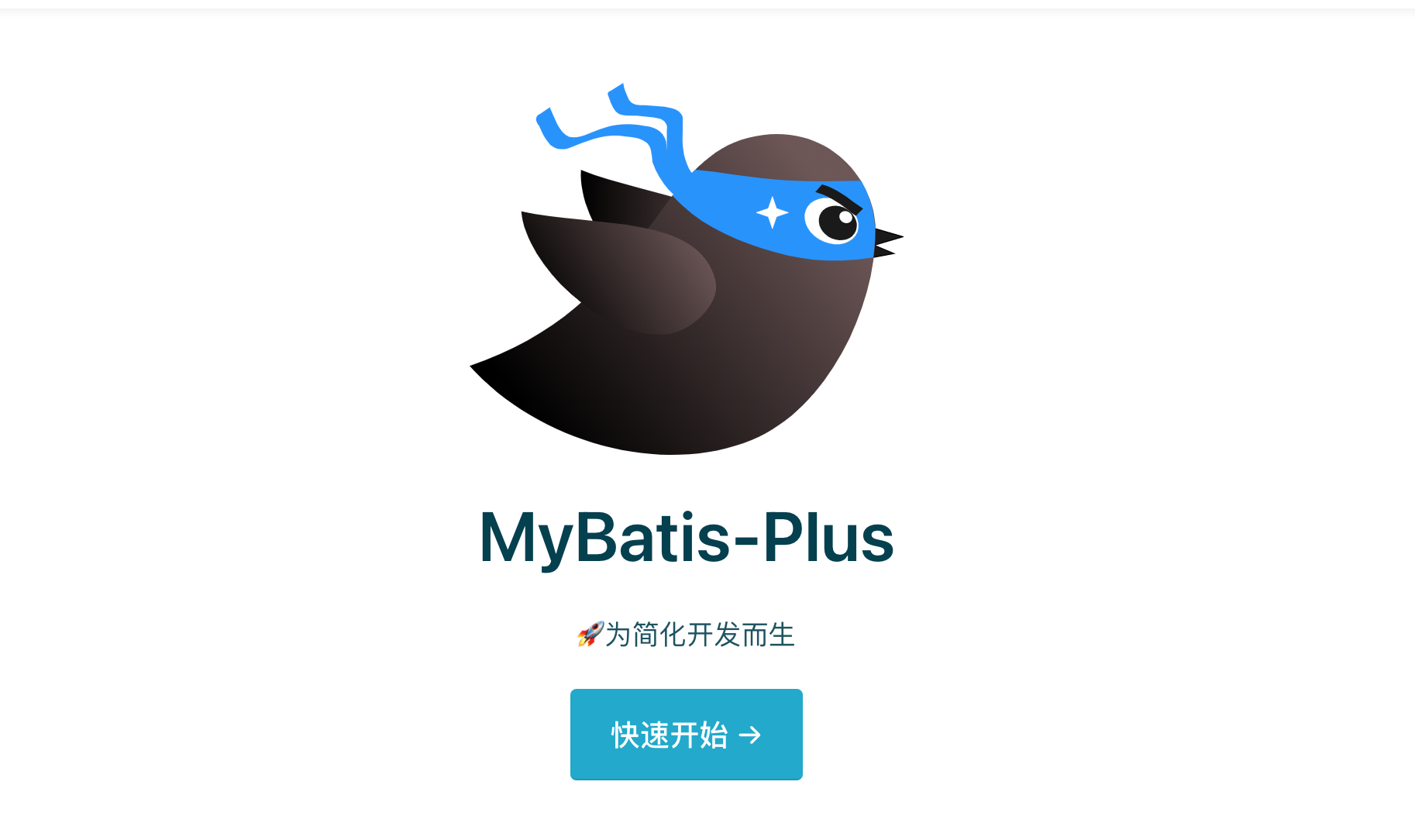
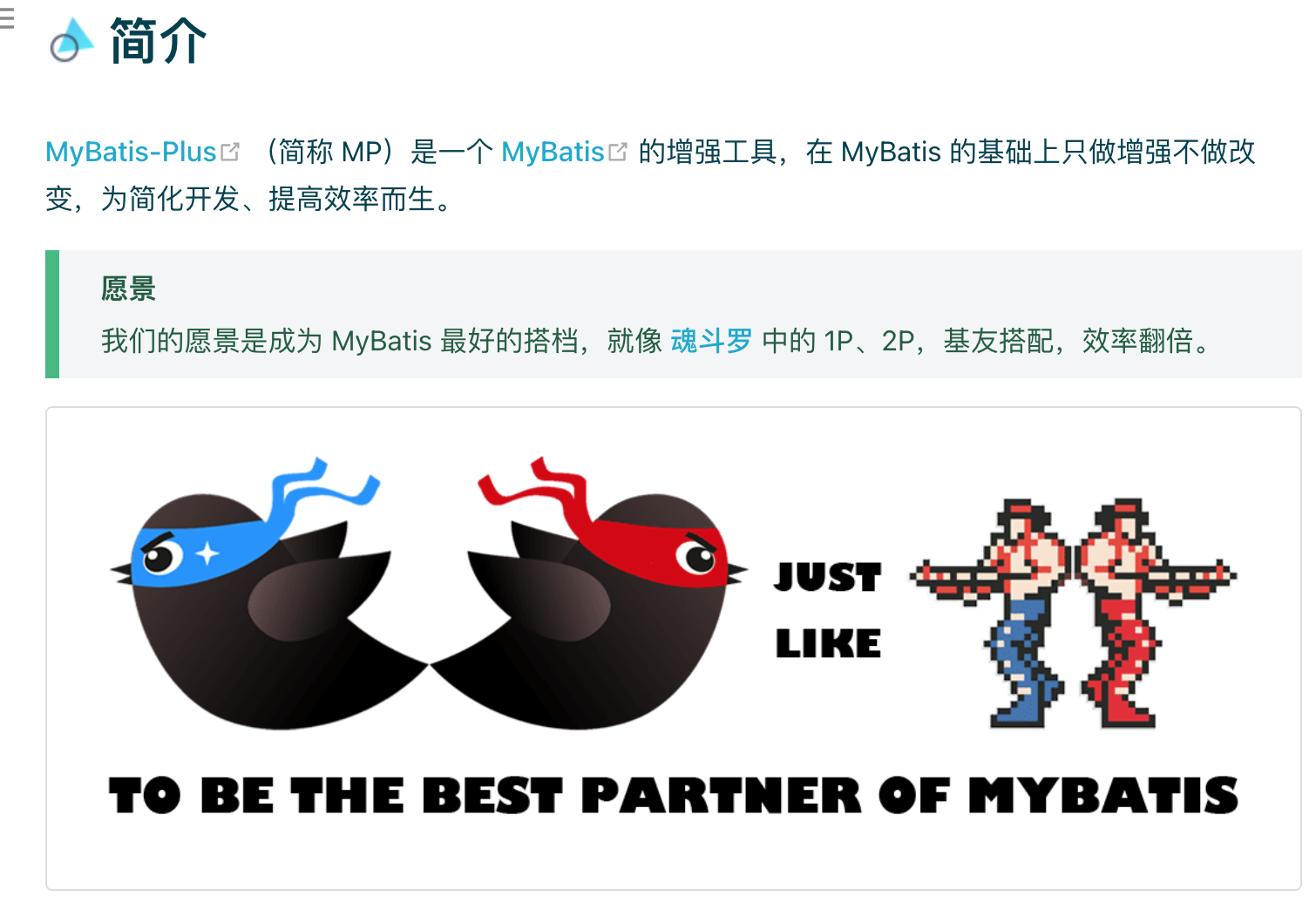
特性:
-
无侵入:只做增强不做改变,引入它不会对现有工程产生影响,如丝般顺滑
-
损耗小:启动即会自动注入基本 CURD,性能基本无损耗,直接面向对象操作,BASEMapper!
-
强大的 CRUD 操作:内置通用 Mapper、通用 Service,仅仅通过少量配置即可实现单表大部分 CRUD 操作,更有强大的条件构造器,满足各类使用需求,以后简单的CRUD操作,他不用自己编写了 !
-
支持 Lambda 形式调用:通过 Lambda 表达式,方便的编写各类查询条件,无需再担心字段写错
-
支持主键自动生成:支持多达 4 种主键策略(内含分布式唯一 ID 生成器 - Sequence),可自由配置,完美解决主键问题
-
支持 ActiveRecord 模式:支持 ActiveRecord 形式调用,实体类只需继承 Model 类即可进行强大的 CRUD 操作
-
支持自定义全局通用操作:支持全局通用方法注入( Write once, use anywhere )
-
内置代码生成器:采用代码或者 Maven 插件可快速生成 Mapper 、 Model 、 Service 、 Controller 层代码,支持模板引擎,更有超多自定义配置等您来使用
-
内置分页插件:基于 MyBatis 物理分页,开发者无需关心具体操作,配置好插件之后,写分页等同于普通 List 查询
-
分页插件支持多种数据库:支持 MySQL、MariaDB、Oracle、DB2、H2、HSQL、SQLite、Postgre、SQLServer 等多种数据库
-
内置性能分析插件:可输出 SQL 语句以及其执行时间,建议开发测试时启用该功能,能快速揪出慢查询
-
内置全局拦截插件:提供全表 delete 、 update 操作智能分析阻断,也可自定义拦截规则,预防误操作
1、快速入门
地址:https://baomidou.com/pages/226c21/#%E5%88%9D%E5%A7%8B%E5%8C%96%E5%B7%A5%E7%A8%8B
步骤
1、创建数据库mybatis_plus
2、创建user表
DROP TABLE IF EXISTS user;
CREATE TABLE user
(
id BIGINT(20) NOT NULL COMMENT '主键ID',
name VARCHAR(30) NULL DEFAULT NULL COMMENT '姓名',
age INT(11) NULL DEFAULT NULL COMMENT '年龄',
email VARCHAR(50) NULL DEFAULT NULL COMMENT '邮箱',
PRIMARY KEY (id)
);
-- 真实开发中,version(乐观锁)、deleted(逻辑删除)、gmt_create、gmt_modified
插入数据
DELETE FROM user;
INSERT INTO user (id, name, age, email) VALUES
(1, 'Jone', 18, 'test1@baomidou.com'),
(2, 'Jack', 20, 'test2@baomidou.com'),
(3, 'Tom', 28, 'test3@baomidou.com'),
(4, 'Sandy', 21, 'test4@baomidou.com'),
(5, 'Billie', 24, 'test5@baomidou.com');
3、编写项目,初始化项目!使用springboot初始化!
4、导入依赖
<!--数据库驱动-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<!--lombok-->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
<!--mybatis-plus 是自己开发的,并非官方的!-->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.0.5</version>
</dependency>
说明:我们使用mybatis-plus 可以节省我们大量的代码,尽量不要同时导入mybatis和mybatis-plus因为版本有差异!
5、连接数据库!这一步和mybatis相同!
# mysql 5 驱动不同
# mysql 8 驱动不同com.mysql.cj.jdbc.Driver 需要增加时区的配置 serverTimezone=GMT%2B8 UTC
#spring.datasource.url=jdbc:mysql://localhost:3306/mybatis_plus?useSSL=false&useUnicode=true&characterEncoding=utf-8&serverTimezone=GMT%2B8
spring.datasource.username=root
spring.datasource.password=123456
spring.datasource.url=jdbc:mysql://localhost:3306/mybaits_plus?useSSL=false&useUnicode=true&characterEncoding=utf-8&serverTimezone=GMT%2B8
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
6、传统方式pojo-dao(连接mybatis,配置mapper.xml)-service-controller
6、使用了mybatis-plus之后
-
pojo
@Data
@AllArgsConstructor
@NoArgsConstructor
public class User {
private Long id;
private String name;
private Integer age;
private String email;
}
-
mapper接口
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.kuang.pojo.User;
import org.springframework.stereotype.Repository;
@Repository //代表持久层
//在对应的mapper上面实现继承我们的BaseMapper
public interface UserMapper extends BaseMapper<User> {
//所有的CRUD操作已将编写完成了
//你不需要像以前一样的配置一大堆文件了!
}
注意点:需要在启动类MybatisPlusApplication
上扫描包下的所有接口
//扫描我们的mapper文件夹
@MapperScan("com.kuang.mapper")
-
测试类中使用
@SpringBootTest
class MybatisPlusApplicationTests {
//继承了BaseMapper,所有的方法都来自自己的父类,我们也可以编写自己的扩展方法!
@Autowired
private UserMapper userMapper;
@Test
void contextLoads() {
//参数是一个Wrapper ,条件构造器,这里我们先不使用 null
//查询全部用户
List<User> users = userMapper.selectList(null);
users.forEach(System.out::println);
}
}
-
查询结果:
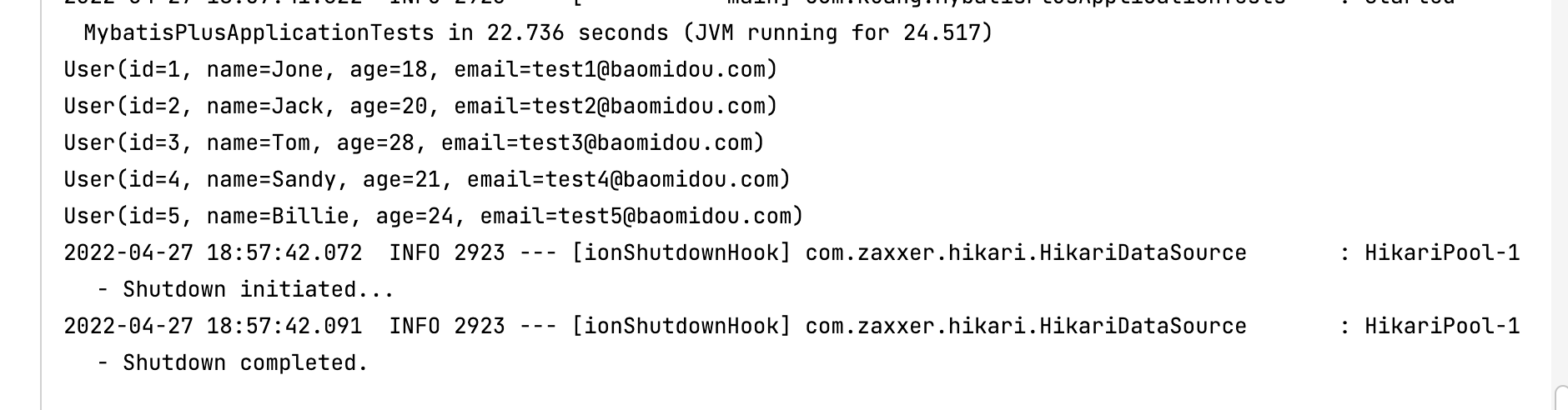
思考问题:
-
sql是谁帮我们写的? —mybatis_plus
-
方法是谁帮我们写的? —mybatis_plus
2、配置日志
我们所以的sql现在是不可见的,我们希望知道他是如何执行的,所以我们必须看日志!
# 配置日志
mybatis-plus.configuration.log-impl=org.apache.ibatis.logging.stdout.StdOutImpl
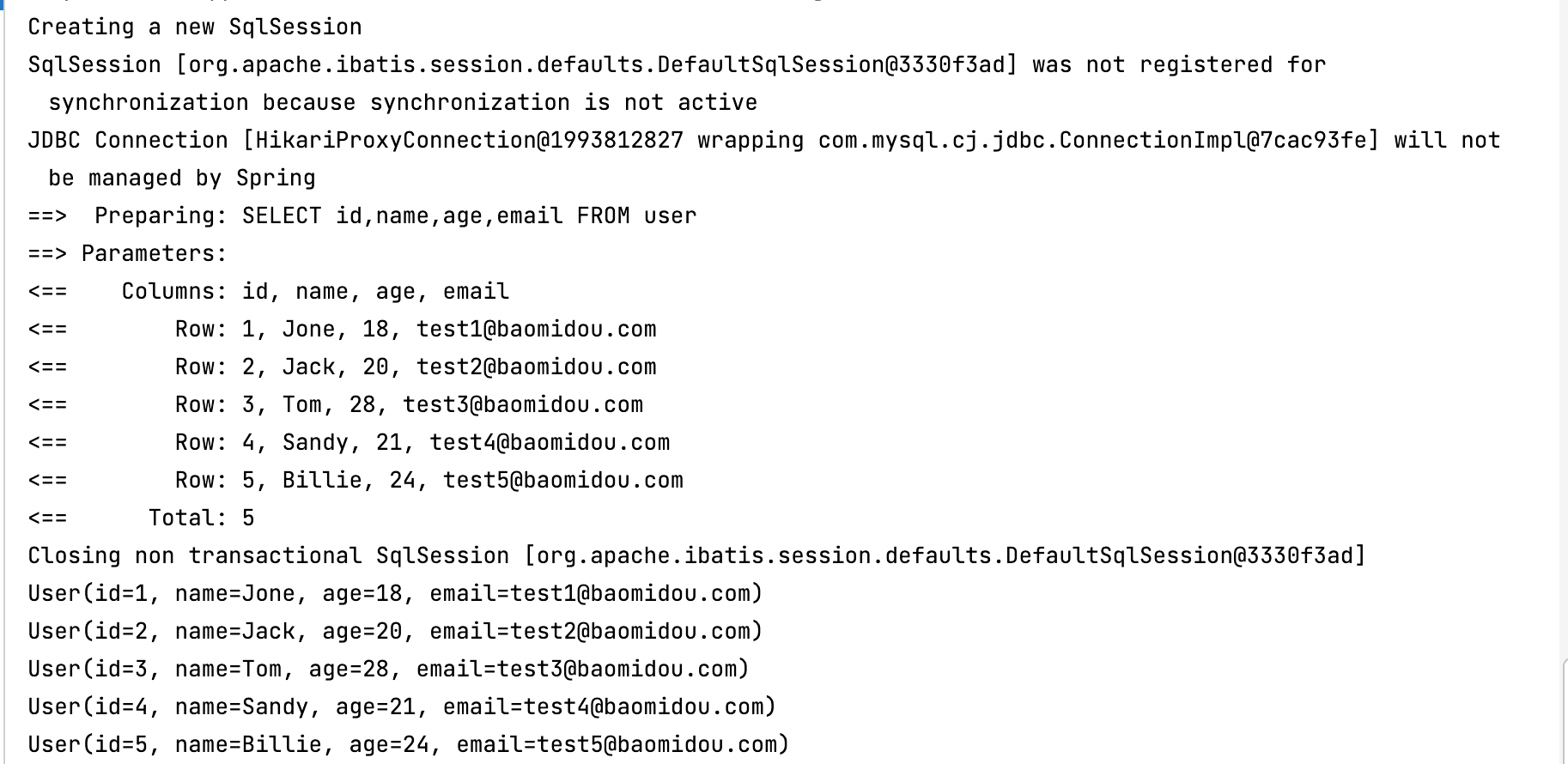
配置完日志之后,后面的学习就需要注意这个自动生成的SQL,你们会喜欢上Mybatis-Plus!
3、CRUD拓展
1、插入数据
Insert 插入
//测试插入
@Test
void testInsert(){
User user = new User();
user.setName("Jacker");
user.setAge(3);
user.setEmail("2724964601@qq.com");
int result = userMapper.insert(user);//帮我们自动生成id
System.out.println(result);//发现受影响的行数
System.out.println(user);// 发现,id 会自动回填
}

注意点:数据库插入的id默认值为:全局的唯一id
2、主键生成策略
默认 ID_WORKER 全局唯一id
对应数据库中的主键(uuid.自增id.雪花算法.redis.zookeeper)
分布式系统唯一id生成:https://www.cnblogs.com/haoxinyue/p/5208136.html
雪花算法😦Twitter的snowflake算法)
snowflake是Twitter开源的分布式ID生成算法,结果是一个long型的ID。其核心思想是:使用41bit作为毫秒数,10bit作为机器的ID(5个bit是数据中心,5个bit的机器ID),12bit作为毫秒内的流水号(意味着每个节点在每毫秒可以产生 4096 个 ID),最后还有一个符号位,永远是0.可以保证几乎全球唯一!
主键自增
我们需要配置主键自增:
-
实体类字段上
@TableId(type = IdType.ID_WORKER)
-
数据库字段一定是自增!
其他源码解释
public enum IdType {
/**
* 数据库ID自增
*/
AUTO(0),
/**
* 该类型为未设置主键类型
*/
NONE(1),
/**
* 用户输入ID
* 该类型可以通过自己注册自动填充插件进行填充
*/
INPUT(2),
/* 以下3种类型、只有当插入对象ID 为空,才自动填充。 */
/**
* 全局唯一ID (idWorker)
*/
ID_WORKER(3),
/**
* 全局唯一ID (UUID)
*/
UUID(4),
/**
* 字符串全局唯一ID (idWorker 的字符串表示)
*/
ID_WORKER_STR(5);
}
3、更新数据
动态SQL
注意:updateById()参数是 一个对象!
@Test
void testUpdate(){
User user = new User();
//通过条件自动拼接动态SQL
user.setId(5L);
user.setName("关注我:Jacker!!");
user.setAge(18);
//注意: updateById ,但是参数是一个对象!
int i = userMapper.updateById(user);
System.out.println(i);
}


所有的sql 都是自动帮你进行配置的!
4、动态填充
创建事件、修改事件!这些个操作一般都是自动化完成的,我们不希望手动更新!
阿里巴巴开发手册:所有的数据库表:gmt_create、gmt_modified几乎所有的表都要配置上!而且需要自动化!
方式一:数据库级别(工作中不允许修改数据库的!)
1、在表中新增字段:create_time、update_time!
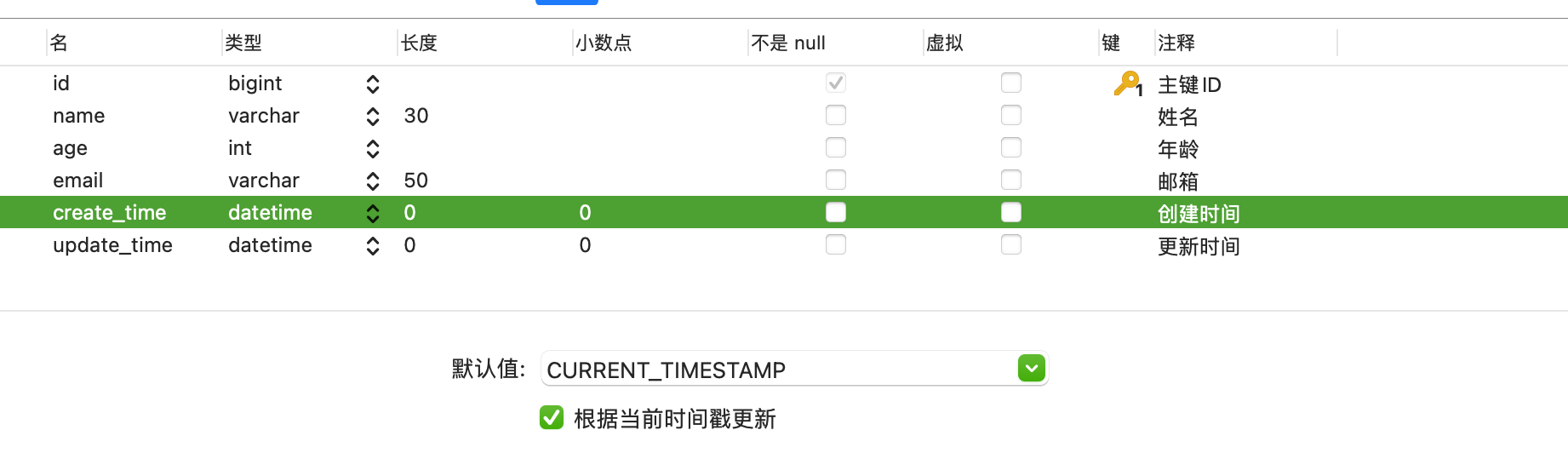
2、再次测试插入方法,我们需要把实体类同步!
private Date createTime;
private Date updateTime;
再次更新查看结构即可!
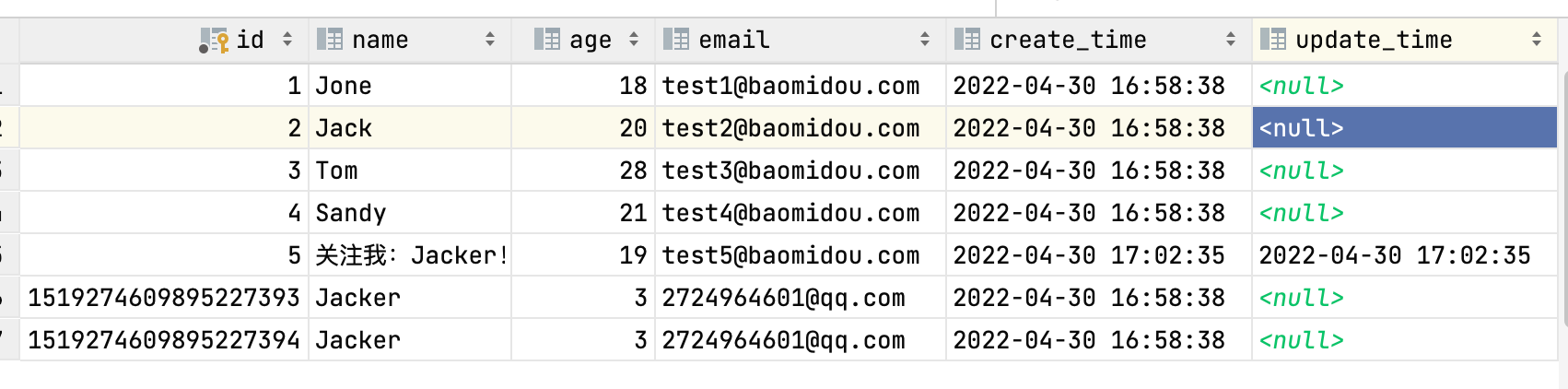
方式二:代码级别
1、删除数据库默认值,更新操作!
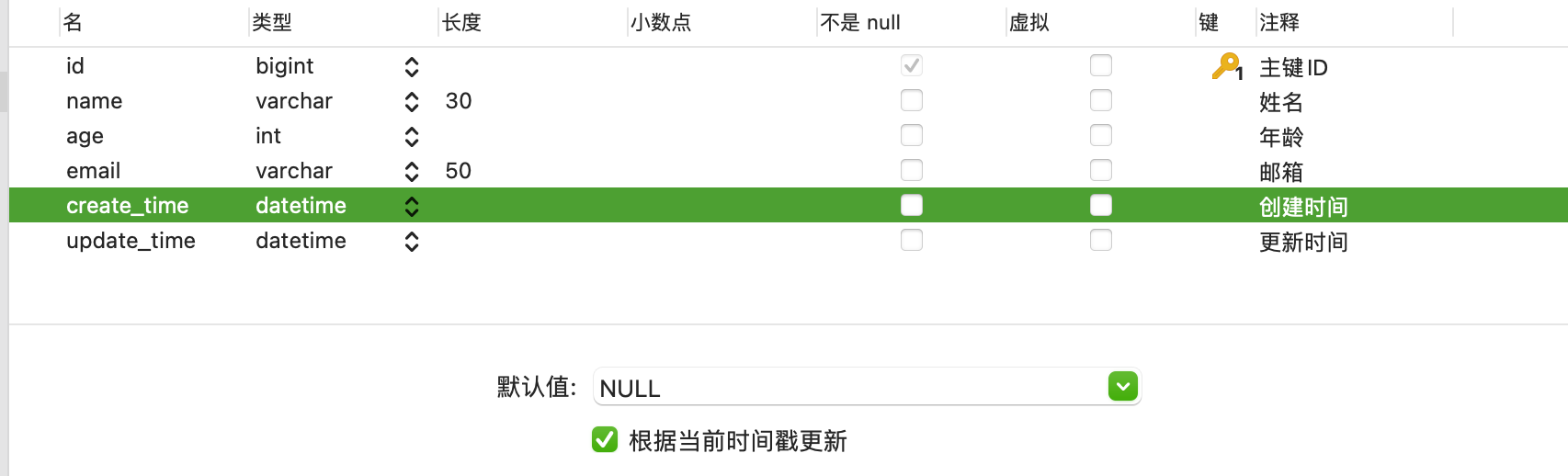
这里create_time与update_time默认值为:CURRENT_TIMESTAMP、只有update_time需要根据当前时间戳更新!
2、实体类字段属性上需要添加注解!
//字段添加填充内容!
@TableField(fill = FieldFill.INSERT)
private Date createTime;
@TableField(fill = FieldFill.INSERT_UPDATE)
private Date updateTime;
3、编写处理器来处理这个注解!
package com.kuang.handler;
import com.baomidou.mybatisplus.core.handlers.MetaObjectHandler;
import lombok.extern.slf4j.Slf4j;
import org.apache.ibatis.reflection.MetaObject;
import org.springframework.stereotype.Component;
import java.util.Date;
@Slf4j
@Component //把处理器加到IOC容器中!
public class MyMetaObjectHandler implements MetaObjectHandler {
//插入时的填充策略
@Override
public void insertFill(MetaObject metaObject) {
log.info("start insert fill...");
//default MetaObjectHandler setFieldValByName(String fieldName, Object fieldVal, MetaObject metaObject)
this.setFieldValByName("createTime",new Date(),metaObject);
this.setFieldValByName("updateTime",new Date(),metaObject);
}
//更新时的填充策略
@Override
public void updateFill(MetaObject metaObject) {
this.setFieldValByName("updateTime",new Date(),metaObject);
}
}
4、测试插入!
5、测试更新,观察事件即可!
5、乐观锁&悲观锁
乐观锁:顾名思义十分乐观,他总是认为不会出现问题,无论干什么都不去上锁!如果出现了问题,再次更新值测试!
悲观锁:顾名思义十分悲观,他总是认为出现问题,无论干什么都去上锁!再去操作!
这里我们主要讲解 乐观锁机制!
乐观锁实现方式:
-
取出记录时,获得当前version
-
更新时,带上这个version
-
执行更新时,set version = new Version() where version = oldVersion
-
如果version不对,就更新失败!
乐观锁:1、先查询,获得版本号 version = 1
-- A
update user set name = "kuangshen",version = version + 1
where id = 2 and version = 1
-- B 线程抢先完成,这个时候 version = 2,会导致 A 修改失败!
update user set name = "kuangshen",version = version + 1
where id = 2 and version = 1
测试一下MP的乐观锁插件
1、给数据库中增加version字段!
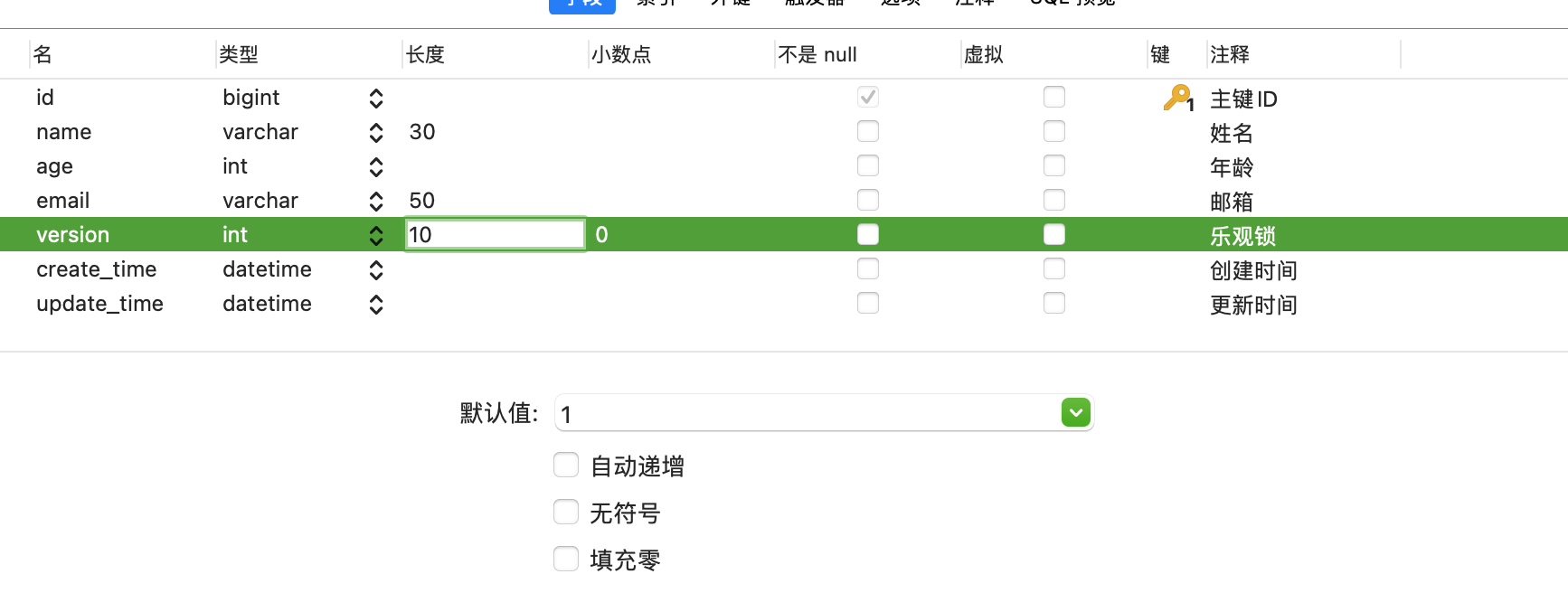
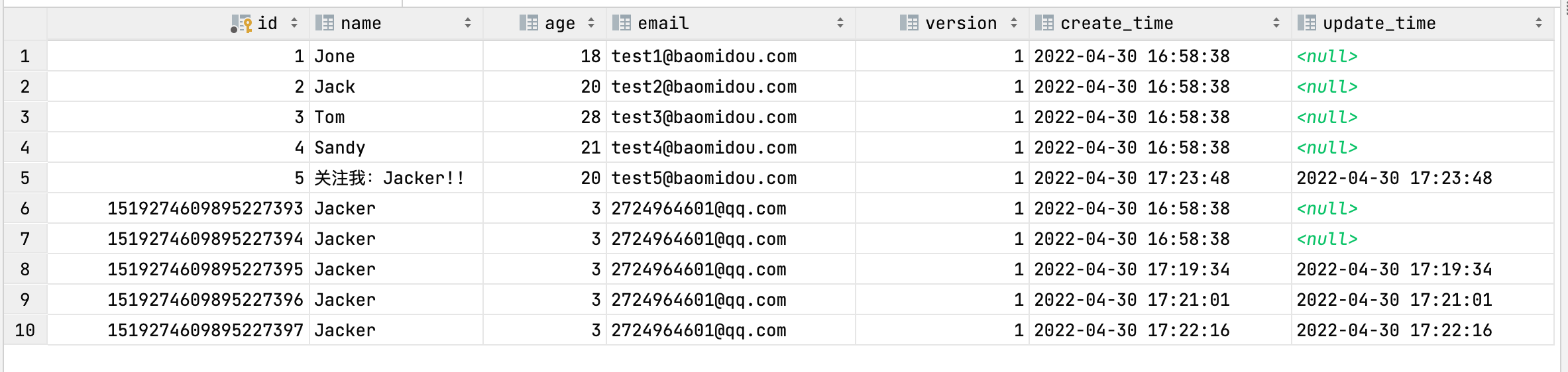
2、实体类增加对应的字段!
@Version //乐观锁version注解
private Integer version;
3、注册组件!
package com.kuang.config;
import com.baomidou.mybatisplus.extension.plugins.OptimisticLockerInterceptor;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.transaction.annotation.EnableTransactionManagement;
//扫描我们的mapper文件夹
@MapperScan("com.kuang.mapper")
@EnableTransactionManagement
@Configuration //配置类
public class MybatisPlusConfig {
//注册乐观锁插件
@Bean
public OptimisticLockerInterceptor optimisticLockerInterceptor() {
return new OptimisticLockerInterceptor();
}
}
4、测试一下!
//测试乐观锁成功!
@Test
void testOptimisticLocker(){
//1、查询用户信息
User user = userMapper.selectById(1L);
//2、修改用户信息
user.setName("Jackerzcr");
user.setEmail("2724964601@qq.com");
//3.执行更新操作
userMapper.updateById(user);
}

//测试乐观锁失败! 多线程下
@Test
void testOptimisticLocker2(){
//线程1
User user = userMapper.selectById(1L);
user.setName("Jackerzcr111");
user.setEmail("2724964601@qq.com");
//模拟另一个线程执行了插队操作!
User user2 = userMapper.selectById(1L);
user2.setName("Jackerzcr222");
user2.setEmail("2724964601@qq.com");
userMapper.updateById(user2);
// 自旋锁来多次尝试提交!
userMapper.updateById(user);//如果没有乐观锁就会覆盖插队线程的值!
}
测试结果!
Creating a new SqlSession
SqlSession [org.apache.ibatis.session.defaults.DefaultSqlSession@6680f714] was not registered for synchronization because synchronization is not active
JDBC Connection [HikariProxyConnection@397639322 wrapping com.mysql.cj.jdbc.ConnectionImpl@12b5736c] will not be managed by Spring
==> Preparing: UPDATE user SET name=?, age=?, email=?, version=?, create_time=?, update_time=? WHERE id=? AND version=?
==> Parameters: Jackerzcr222(String), 18(Integer), 2724964601@qq.com(String), 3(Integer), 2022-04-30 16:58:38.0(Timestamp), 2022-05-01 14:38:27.65(Timestamp), 1(Long), 2(Integer)
<== Updates: 1
Closing non transactional SqlSession [org.apache.ibatis.session.defaults.DefaultSqlSession@6680f714]
Creating a new SqlSession
SqlSession [org.apache.ibatis.session.defaults.DefaultSqlSession@250a9031] was not registered for synchronization because synchronization is not active
JDBC Connection [HikariProxyConnection@375781972 wrapping com.mysql.cj.jdbc.ConnectionImpl@12b5736c] will not be managed by Spring
==> Preparing: UPDATE user SET name=?, age=?, email=?, version=?, create_time=?, update_time=? WHERE id=? AND version=?
==> Parameters: Jackerzcr111(String), 18(Integer), 2724964601@qq.com(String), 3(Integer), 2022-04-30 16:58:38.0(Timestamp), 2022-05-01 14:38:27.665(Timestamp), 1(Long), 2(Integer)
<== Updates: 0
6、查询操作
// 测试查询!
@Test
void testSelectById(){
User user = userMapper.selectById(1l);
System.out.println(user);
}
// 测试批量查询!
@Test
void testSelectByBatchId(){
List<User> userList = userMapper.selectBatchIds(Arrays.asList(1, 2, 3));
userList.forEach(System.out::println);
}
// 按条件查询之一使用 map操作!
@Test
void testSelectByBatchIds(){
HashMap<String, Object> map = new HashMap<>();
//自定义查询条件!
map.put("name","Jacker");
map.put("age",3);
List<User> users = userMapper.selectByMap(map);
users.forEach(System.out::println);
}
7、分页查询
一、第一种方式:
分页在网站使用的十分之多!
-
原始的 limit 进行分页
-
pageHelper 第三方插件
-
MP 其实也内置了分页插件!
如何使用!
1、配置拦截器组件即可!
// 分页插件
@Bean
public PaginationInterceptor paginationInterceptor(){
return new PaginationInterceptor();
}
2、直接使用Page对象即可!
//测试分页插件
@Test
void testPage(){
// 参数一: 当前页
// 参数二: 页面大小
// 使用了分页插件之后,所有的分页操作也变得十分简单!
Page<User> page = new Page<>(2,5);
userMapper.selectPage(page,null);
page.getRecords().forEach(System.out::println);
System.out.println(page.getTotal());
}
二、第二种方式:(新版)
<!-- https://mvnrepository.com/artifact/com.baomidou/mybatis-plus-boot-starter -->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.5.1</version>
</dependency>
import com.baomidou.mybatisplus.annotation.DbType;
import com.baomidou.mybatisplus.extension.plugins.MybatisPlusInterceptor;
import com.baomidou.mybatisplus.extension.plugins.inner.PaginationInnerInterceptor;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
@MapperScan("com.example.mapper")
public class MybatisPlusConfig {
/**
* 新的分页插件,一缓和二缓遵循mybatis的规则,需要设置 MybatisConfiguration#useDeprecatedExecutor = false 避免缓存出现问题(该属性会在旧插件移除后一同移除)
*/
@Bean
public MybatisPlusInterceptor mybatisPlusInterceptor() {
MybatisPlusInterceptor interceptor = new MybatisPlusInterceptor();
interceptor.addInnerInterceptor(new PaginationInnerInterceptor(DbType.MYSQL));
return interceptor;
}
@Bean
public ConfigurationCustomizer configurationCustomizer() {
return configuration -> configuration.setUseDeprecatedExecutor(false);
}
}
对于mybatis-plus分页插件可能遇到的一些问题:如果我们已经定义了@mapper注解但是没有生效的话,可以尝试一下使用@MapperScan注解扫描包!
8、删除操作
基本的删除操作!
// 测试删除!
@Test
void testDeleteById(){
userMapper.deleteById(1519274609895227397l);
}
// 测试批量删除!
@Test
void testDeleteBatchId(){
userMapper.deleteBatchIds(Arrays.asList(1519274609895227395l,1519274609895227396l));
}
// 通过map删除
@Test
void testDeleteMap(){
HashMap<String, Object> map = new HashMap<>();
map.put("name","Jacker");
userMapper.deleteByMap(map);
}
我们在工作中会遇到一些问题:逻辑删除!
9、逻辑删除
物理删除:从数据库中直接移除!
逻辑删除:在数据库中没有被移除,而是通过一个变量来让他失效!deleted = 2 => deleted = 1
管理员可以查看被删除的记录!防止数据的丢失,类似于回收站!
测试一下:
1、在数据库中增加一个 deleted 字段
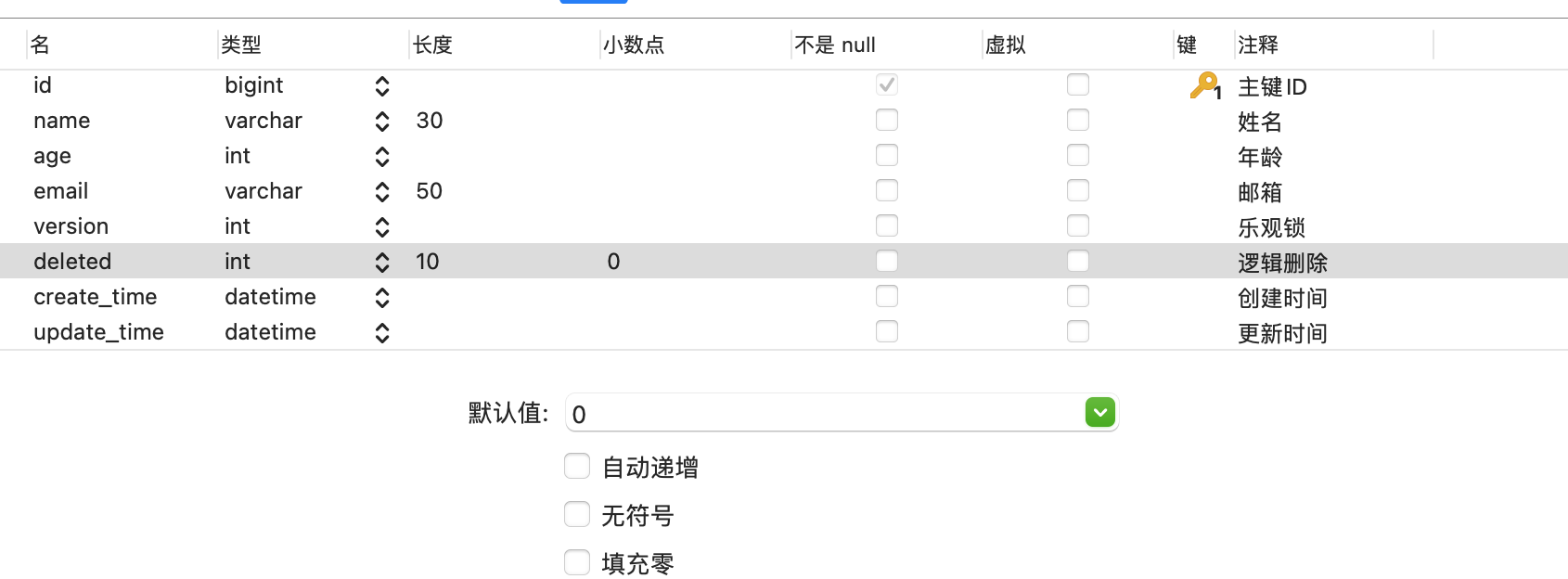
2、实体类中增加属性
@TableLogic //逻辑删除
private Integer deleted;
3、配置!
//注册逻辑删除
@Bean
public ISqlInjector sqlInjector(){
return new LogicSqlInjector();
}
# 配置逻辑删除
mybatis-plus.global-config.db-config.logic-delete-value=1
mybatis-plus.global-config.db-config.logic-not-delete-value=0
在application.yml中的配置:
mybatis-plus:
global-config:
db-config:
logic-delete-field: flag # 全局逻辑删除的实体字段名(since 3.3.0,配置后可以忽略不配置步骤2)
logic-delete-value: 1 # 逻辑已删除值(默认为 1)
logic-not-delete-value: 0 # 逻辑未删除值(默认为 0)
删除接口自动填充功能失效
- 使用
deleteById
方法(推荐)- 使用
update
方法并:UpdateWrapper.set(column, value)
(推荐)- 使用
update
方法并:UpdateWrapper.setSql("column=value")
- 使用 Sql 注入器 注入
com.baomidou.mybatisplus.extension.injector.methods.LogicDeleteByIdWithFill
并使用(3.5.0版本已废弃,推荐使用deleteById)
4、测试一下删除!
@Test
void testDeleteById(){
userMapper.deleteById(1l);
}
记录依旧在数据库,但是值却已经变化了!
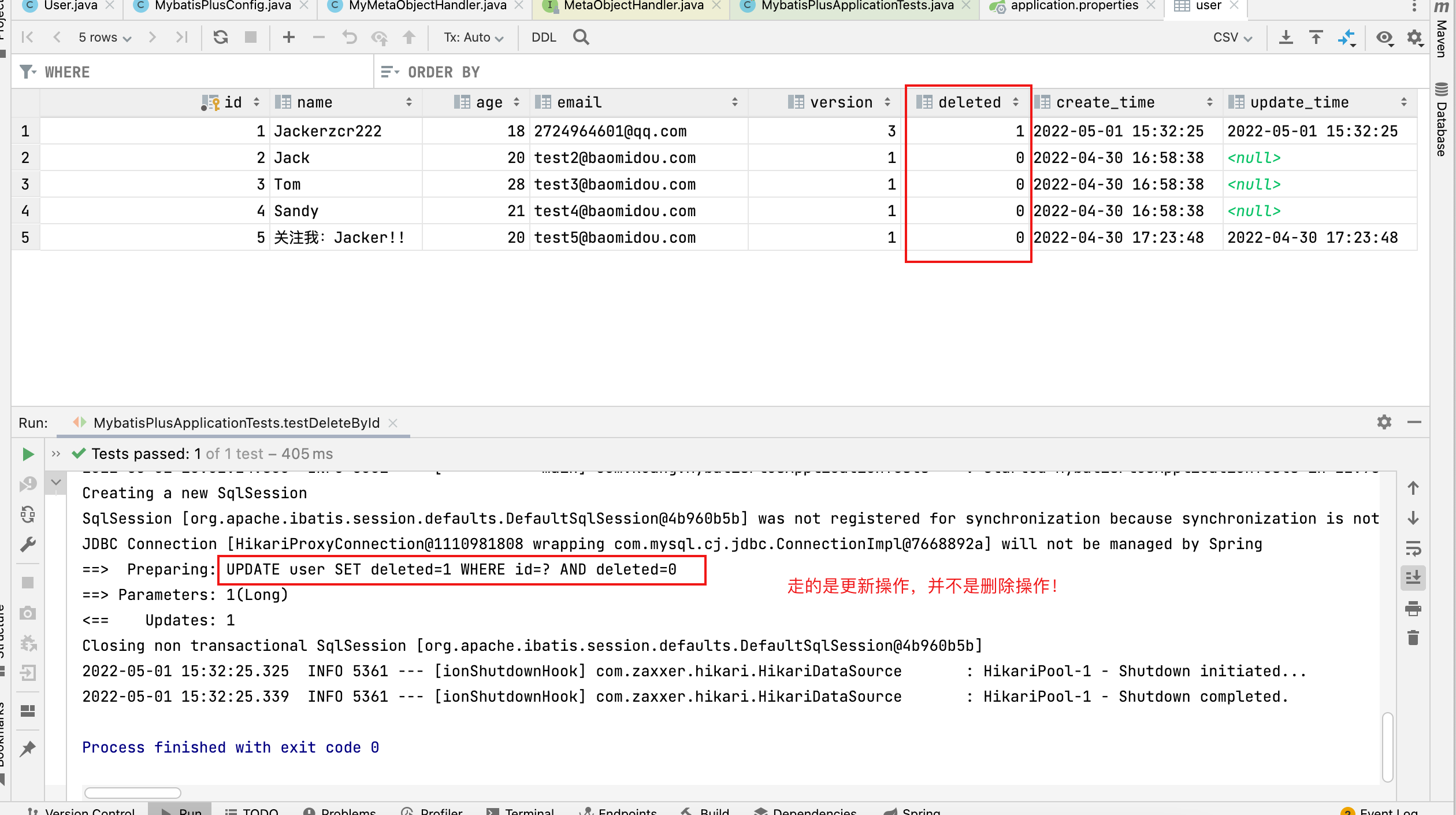
@Test
void testSelectById(){
User user = userMapper.selectById(1l);
System.out.println(user);
}
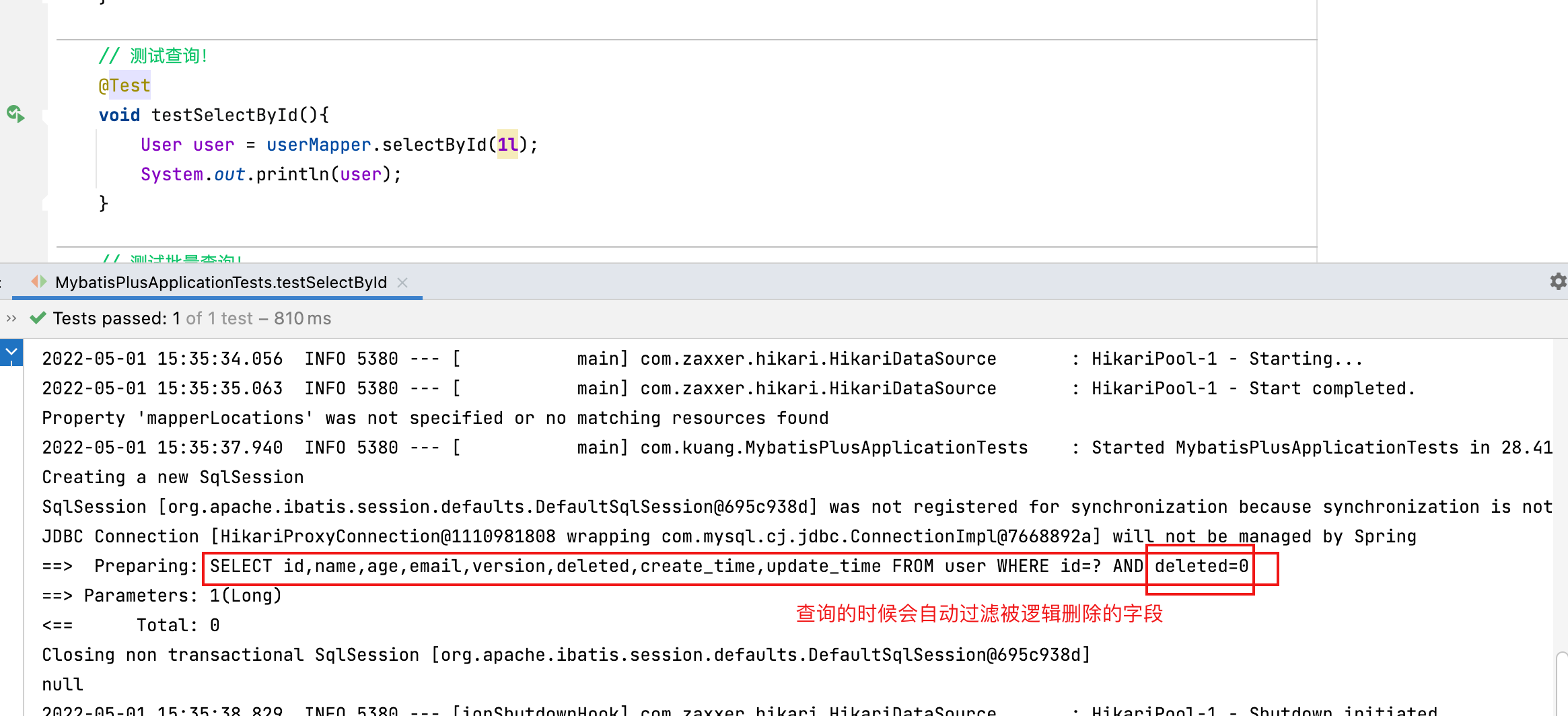
以上的所有CRUD操作及其扩展操作,我们必须精通掌握!会大大挺好你的工作和写项目的效率!
10、性能分析插件
我们在平时的开发中,会遇到一些慢sql。测试!druid…!
作用:性能分析拦截器,用于输出每条SQL语句及其执行时间 !
MP也提供了性能分析插件,如果超过了这个时间就停止运行!
1、导入插件
/*性能分析插件*/
@Bean
@Profile(value = {"dev","test"}) // 设置 test环境下 会开启SQL性能分析
public PerformanceInterceptor performanceInterceptor(){
PerformanceInterceptor performanceInterceptor = new PerformanceInterceptor();
performanceInterceptor.setMaxTime(1); // ms 设置SQL执行的最大时间,如果超过了则不执行!
performanceInterceptor.setFormat(true); // 是否格式化代码!
return performanceInterceptor;
}
记住,要在springboot中配置环境为dev 或者 test 环境!
# 设置环境
spring.profiles.active=dev
2、测试使用!
@Test
void contextLoads() {
//参数是一个Wrapper ,条件构造器,这里我们先不使用 null
//查询全部用户
List<User> users = userMapper.selectList(null);
users.forEach(System.out::println);
}
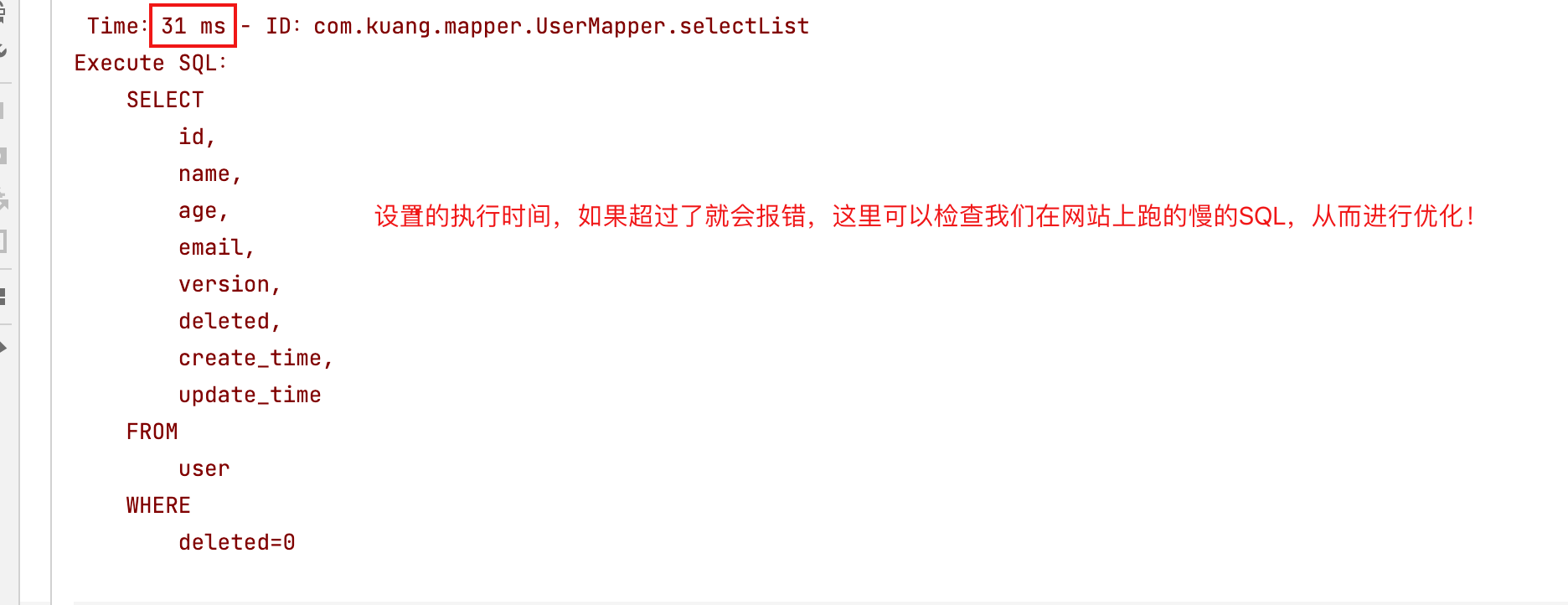
使用性能分析插件,可以帮助我们提高效率!
11、条件构造器
十分重要:Wrapper
Wrapper : 条件构造抽象类,最顶端父类,抽象类中提供4个方法西面贴源码展示
AbstractWrapper : 用于查询条件封装,生成 sql 的 where 条件
AbstractLambdaWrapper : Lambda 语法使用 Wrapper统一处理解析 lambda 获取 column。
LambdaQueryWrapper :看名称也能明白就是用于Lambda语法使用的查询Wrapper
LambdaUpdateWrapper : Lambda 更新封装Wrapper
QueryWrapper : Entity 对象封装操作类,不是用lambda语法
UpdateWrapper : Update 条件封装,用于Entity对象更新操作
我们写一些复杂的sql就可以使用他来代替!
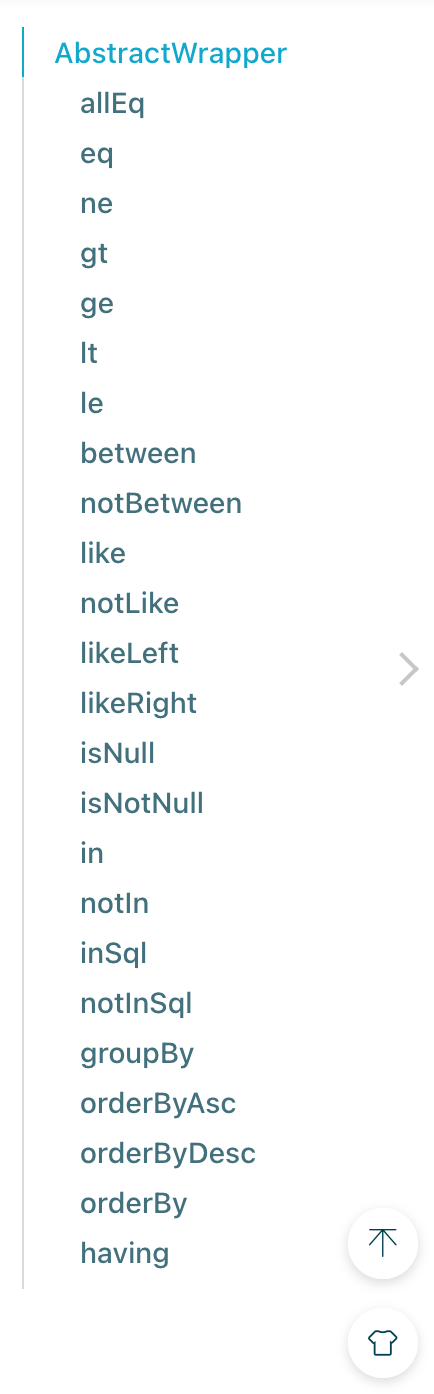
1、测试一,记住查看输出的sql记性分析:
@Test
void contextLoads() {
// 查询name不为空的用户,并且邮箱不为空的用户,年龄大于等于12
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper
.isNotNull("name")
.isNotNull("email")
.ge("age",12);
userMapper.selectList(wrapper).forEach(System.out::println);// 和我们刚才的map进行对比学习!
}
2、测试二,记住查看输出的sql记性分析:
@Test
void test2(){
// 查询名字为Jack
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.eq("name","Jack");
User user = userMapper.selectOne(wrapper);// 查询一个数据,多线多个结果使用List 或者 Map!
System.out.println(user);
}
3、测试三,记住查看输出的sql记性分析:
@Test
void test3(){
// 查询年龄在 20~30 岁之间的用户
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.between("age",20,30);// 区间
Integer count = userMapper.selectCount(wrapper);// 查询结果数
System.out.println(count);
}
4、测试四,记住查看输出的sql记性分析:
// 模糊查询
@Test
void test4(){
QueryWrapper<User> wrapper = new QueryWrapper<>();
//左和右 t%
wrapper
.notLike("name","e")
.likeRight("email","t");
List<Map<String, Object>> maps = userMapper.selectMaps(wrapper);
maps.forEach(System.out::println);
}
5、测试五():
@Test
void test5(){
QueryWrapper<User> wrapper = new QueryWrapper<>();
// id在子查询中查出来
wrapper.inSql("id","select id from user where id < 3");
List<Object> objects = userMapper.selectObjs(wrapper);
objects.forEach(System.out::println);
}
输出结果:
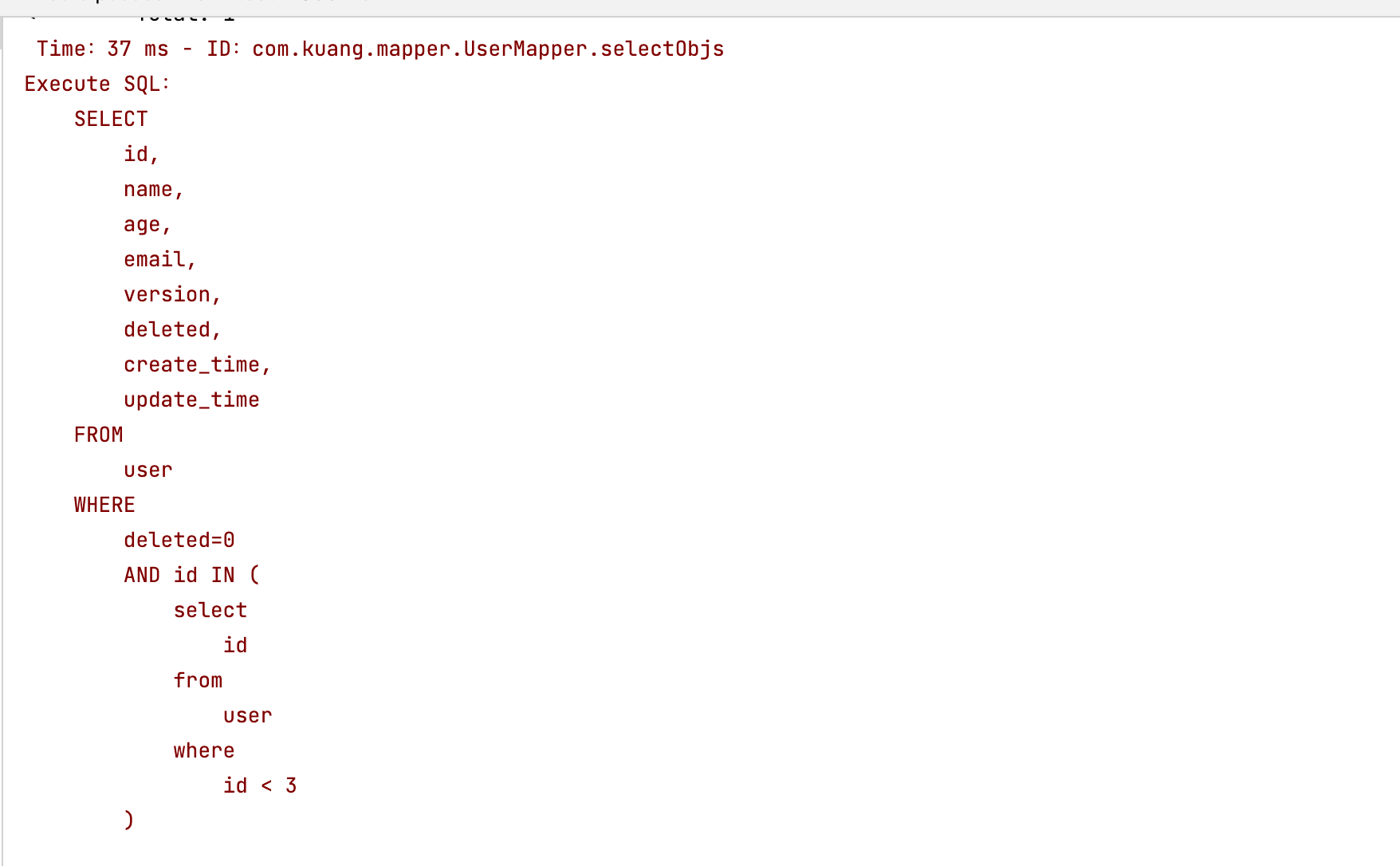
6、测试六:
@Test
void test6(){
QueryWrapper<User> wrapper = new QueryWrapper<>();
// 通过id降序排列
wrapper.orderByDesc("id");
//wrapper.orderByAsc("id"); 升序
List<User> users = userMapper.selectList(wrapper);
users.forEach(System.out::println);
}
12、代码自动生成器
使用首先导入依赖:
<!--mybatis-plus-->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.2.0</version>
</dependency>
<!-- framework: mybatis-plus代码生成需要一个模板引擎 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-freemarker</artifactId>
</dependency>
<!--mp代码生成器-->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-generator</artifactId>
<version>3.2.0</version>
</dependency>
dao、pojo、service、controller都给我自己去编写完成!
AutoGenerator 是 MyBatis-Plus 的代码生成器,通过 AutoGenerator 可以快速生成 Entity、Mapper、Mapper XML、Service、Controller 等各个模块的代码,极大的提升了开发效率。
测试:
package com.kuang;
import com.baomidou.mybatisplus.annotation.DbType;
import com.baomidou.mybatisplus.annotation.FieldFill;
import com.baomidou.mybatisplus.annotation.IdType;
import com.baomidou.mybatisplus.generator.AutoGenerator;
import com.baomidou.mybatisplus.generator.config.DataSourceConfig;
import com.baomidou.mybatisplus.generator.config.GlobalConfig;
import com.baomidou.mybatisplus.generator.config.PackageConfig;
import com.baomidou.mybatisplus.generator.config.StrategyConfig;
import com.baomidou.mybatisplus.generator.config.po.TableFill;
import com.baomidou.mybatisplus.generator.config.rules.DateType;
import com.baomidou.mybatisplus.generator.config.rules.NamingStrategy;
import java.util.ArrayList;
// 代码自动生成器
public class AutoGeneratorCode {
public static void main(String[] args) {
//需要构建一个 代码自动生成器 对象
AutoGenerator mpg = new AutoGenerator();
//配置策略
//1、全局配置
GlobalConfig gc = new GlobalConfig();
String projectPath = System.getProperty("user.dir");
gc.setOutputDir(projectPath+"/src/main/java");
gc.setAuthor("狂神说");
gc.setOpen(false); // 是否打开资源管理器
gc.setFileOverride(false); // 是否覆盖
// 去Service的I前缀
gc.setServiceName("%sService");
gc.setIdType(IdType.ID_WORKER);
gc.setDateType(DateType.ONLY_DATE);
gc.setSwagger2(true);
mpg.setGlobalConfig(gc);
//2、设置数据源
DataSourceConfig dsc = new DataSourceConfig();
dsc.setUrl("jdbc:mysql://localhost:3306/kuang_community? useSSL=false&useUnicode=true&characterEncoding=utf-8&serverTimezone=GMT%2B8");
dsc.setDriverName("com.mysql.cj.jdbc.Driver");
dsc.setUsername("root");
dsc.setPassword("123456");
dsc.setDbType(DbType.MYSQL);
mpg.setDataSource(dsc);
//3、包的配置
PackageConfig pc = new PackageConfig();
//只需要改实体类名字 和包名 还有 数据库配置即可
pc.setModuleName("blog");
pc.setParent("com.kuang");
pc.setEntity("entity");
pc.setMapper("mapper");
pc.setService("service");
pc.setController("controller");
mpg.setPackageInfo(pc);
//4、策略配置
StrategyConfig strategy = new StrategyConfig();
// 设置要映射的表名
strategy.setInclude("blog_tags","course","links","sys_settings","user_record"," user_say");
strategy.setNaming(NamingStrategy.underline_to_camel);
strategy.setColumnNaming(NamingStrategy.underline_to_camel);
// 自动lombok;
strategy.setEntityLombokModel(true);
strategy.setLogicDeleteFieldName("deleted");
// 自动填充配置
TableFill gmtCreate = new TableFill("gmt_create", FieldFill.INSERT);
TableFill gmtModified = new TableFill("gmt_modified", FieldFill.INSERT_UPDATE);
ArrayList<TableFill> tableFills = new ArrayList<>();
tableFills.add(gmtCreate);
tableFills.add(gmtModified);
strategy.setTableFillList(tableFills);
// 乐观锁
strategy.setVersionFieldName("version");
strategy.setRestControllerStyle(true);
// localhost:8080/hello_id_2
strategy.setControllerMappingHyphenStyle(true);
mpg.setStrategy(strategy);
mpg.execute(); //执行
}
}
这个工具类大家可以放心使用,可以大大简化我们的开发效率!
新版本的AutoGenerator代码生成器
1、pom.xml
<!--mybatis-plus代码生成器!-->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-generator</artifactId>
<version>3.5.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.velocity/velocity-engine-core -->
<dependency>
<groupId>org.apache.velocity</groupId>
<artifactId>velocity-engine-core</artifactId>
<version>2.3</version>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.5.1</version>
</dependency>
2、设置代码自动生成的utils:
package com.example.utils;
import com.baomidou.mybatisplus.generator.FastAutoGenerator;
import com.baomidou.mybatisplus.generator.config.OutputFile;
import java.util.Collections;
public class AutoGenerator {
public static void main(String[] args) {
generate();
}
private static void generate(){
FastAutoGenerator.create("jdbc:mysql://localhost:3306/back?serverTimezone=UTC", "root", "123456")
.globalConfig(builder -> {
builder.author("yykk") // 设置作者
.enableSwagger() // 开启 swagger 模式
.fileOverride() // 覆盖已生成文件
.outputDir("/Users/mac/Documents/项目/后台管理系统/springboot/src/main/java/"); // 指定输出目录
})
.packageConfig(builder -> {
builder.parent("com.example") // 设置父包名
.moduleName("") // 设置父包模块名 如果路径出现了// 那么请设置为null
.pathInfo(Collections.singletonMap(OutputFile.mapperXml, "/Users/mac/Documents/项目/后台管理系统/springboot/src/main/resources/mapper/")); // 设置mapperXml生成路径
})
.strategyConfig(builder -> {
builder.entityBuilder().enableLombok();
//builder.mapperBuilder().enableMapperAnnotation().build(); //添加mapper注解,可以添加mapperscan就不需要了
builder.controllerBuilder().enableHyphenStyle() // 开启驼峰转连字符
.enableRestStyle(); // 开启生成@RestController 控制器
builder.addInclude("sys_user") // 设置需要生成的表名
.addTablePrefix("t_", "sys_"); // 设置过滤表前缀
})
//.templateEngine(new FreemarkerTemplateEngine()) // 使用Freemarker引擎模板,默认的是Velocity引擎模板
.execute();
}
}
3、自定义模板生成controller:
package ${package.Controller};
import org.springframework.web.bind.annotation.*;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import javax.annotation.Resource;
import java.util.List;
import ${package.Service}.${table.serviceName};
import ${package.Entity}.${entity};
#if(${restControllerStyle})
import org.springframework.web.bind.annotation.RestController;
#else
import org.springframework.stereotype.Controller;
#end
#if(${superControllerClassPackage})
import ${superControllerClassPackage};
#end
/**
* <p>
* $!{table.comment} 前端控制器
* </p>
*
* @author ${yykk}
* @since ${date}
*/
#if(${restControllerStyle})
@RestController
#else
@Controller
#end
@RequestMapping("#if(${package.ModuleName})/${package.ModuleName}#end/#if(${controllerMappingHyphenStyle})${controllerMappingHyphen}#else${table.entityPath}#end")
#if(${kotlin})
class ${table.controllerName}#if(${superControllerClass}) : ${superControllerClass}()#end
#else
#if(${superControllerClass})
public class ${table.controllerName} extends ${superControllerClass} {
#else
public class ${table.controllerName} {
#end
@Resource
private ${table.serviceName} ${table.entityPath}Service;
@PostMapping
public Boolean save(@RequestBody ${entity} ${table.entityPath}) {
return ${table.entityPath}Service.saveOrUpdate(${table.entityPath});
}
@DeleteMapping("/{id}")
public Boolean delete(@PathVariable Integer id) {
return ${table.entityPath}Service.removeById(id);
}
@GetMapping
public List<${entity}> findAll() {
return ${table.entityPath}Service.list();
}
@GetMapping("/{id}")
public ${entity} findOne(@PathVariable Integer id) {
return ${table.entityPath}Service.getById(id);
}
@GetMapping("/page")
public Page<${entity}> findPage(@RequestParam Integer pageNum,
@RequestParam Integer pageSize) {
QueryWrapper<${entity}> queryWrapper = new QueryWrapper<>();
return ${table.entityPath}Service.page(new Page<>(pageNum, pageSize),queryWrapper);
}
}
#end
---------------------------------
mybatis-plus自定义生成模板的一些参考博客:
- https://blog.csdn.net/zhilonng/article/details/109132292
- https://blog.csdn.net/lh155136/article/details/123423762
- https://blog.csdn.net/qq_45246098/article/details/123023742
- https://www.cnblogs.com/liyh321/p/14172930.html
- https://blog.csdn.net/xqnode?type=blog