小组成员分工
zengweihan:搭建环境配置
luoyichun:index提示helloworld
liyanru:网站登录功能
masihuan :网站收藏功能
luoxinwei:网站购买功能
chenjie:网站查看旅游景点功能
项目介绍
主要功能:旅游网站,有登录、收藏、购买、查看旅游景点的功能
package com.cn.panda.entity;
public class User {
private int id = 0;
private String username;
private String password;
private String realname = "";
private String phone = "";
private String idcard = "";
private int gender = 0;
private int role = 0;
public User(){
}
public User(int id, String username, String password, String realname, String phone, String idcard, int gender, int role) {
this.id = id;
this.username = username;
this.password = password;
this.realname = realname;
this.phone = phone;
this.idcard = idcard;
this.gender = gender;
this.role = role;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getRealname() {
return realname;
}
public void setRealname(String realname) {
this.realname = realname;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getIdcard() {
return idcard;
}
public void setIdcard(String idcard) {
this.idcard = idcard;
}
public int getGender() {
return gender;
}
public void setGender(int gender) {
this.gender = gender;
}
public int getRole() {
return role;
}
public void setRole(int role) {
this.role = role;
}
@Override
public String toString() {
return "User{" +
"id=" + id +
", username='" + username + '\'' +
", password='" + password + '\'' +
", realname='" + realname + '\'' +
", phone='" + phone + '\'' +
", idcard='" + idcard + '\'' +
", gender=" + gender +
", role=" + role +
'}';
}
}
package com.cn.panda.controller;
import com.cn.panda.entity.User;
import com.cn.panda.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
@Controller
@RequestMapping("user")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("getUser")
public String getUser(int id) {
User user = userService.queryUser(id);
System.out.println(user);
return "front/user";
}
@PostMapping("login")//注意此处与表单action的对应
public String getUser(User user, HttpServletRequest request, HttpSession session) {
System.out.println ("=============");
User u = userService.getUserByUsernameAndPassword(user);
System.out.println("-------- u="+u);
if (u != null) {
System.out.println ("------登录OK");
//保存用户至session
session.setAttribute("user", u);
//重定向至景点
String path = "redirect:/attraction/list";
return path;
} else {
System.out.println ("------登录Error");
//跳转至登录
String path = "front/login";
return path;
}
}
}
package com.cn.panda.mapper;
import com.cn.panda.entity.User;
import org.springframework.stereotype.Repository;
@Repository
public interface UserMapper {
// 自动拆箱和装箱
User findUserById(int id);
//依据用户名和密码查询用户
User getUserByUsernameAndPassword(User user);
}
package com.cn.panda.service.impl;
import com.cn.panda.entity.User;
import com.cn.panda.mapper.UserMapper;
import com.cn.panda.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
public User queryUser(int id) {
User user = userMapper.findUserById(id);
return user;
}
public User getUserByUsernameAndPassword(User user){
return userMapper.getUserByUsernameAndPassword(user);
}
}
```java
package com.cn.panda.service;
import com.cn.panda.entity.User;
public interface UserService {
User queryUser(int id);
User getUserByUsernameAndPassword(User user);
}
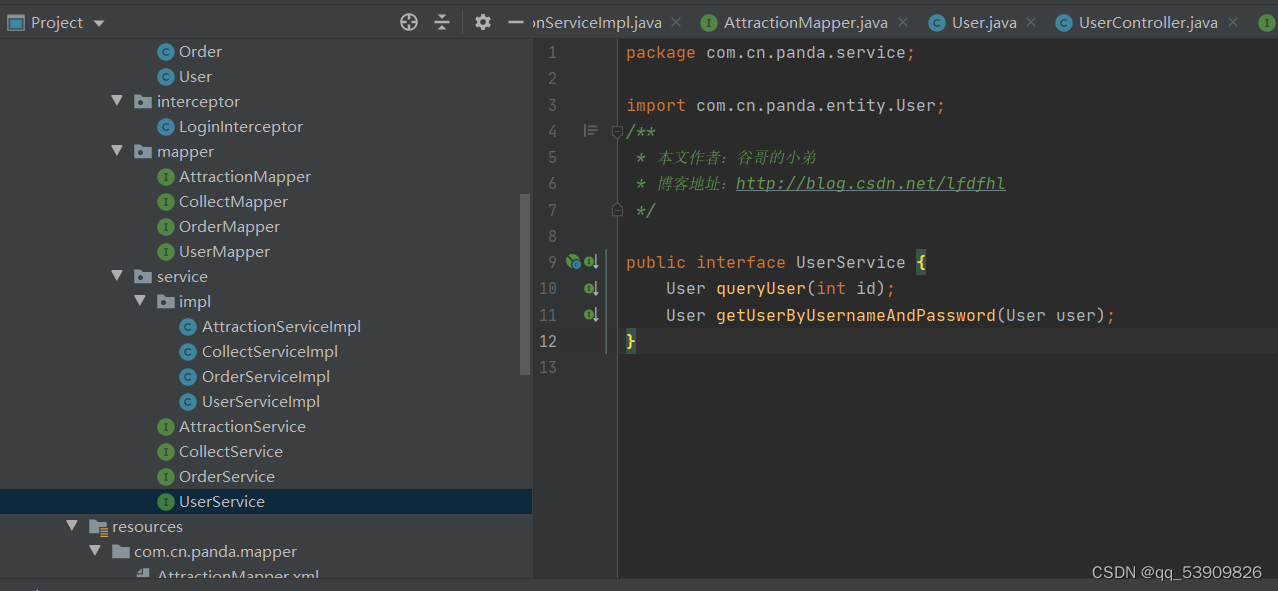
```java
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.cn.panda.mapper.UserMapper">
<select id="findUserById" parameterType="int" resultType="user">
select * from user where id = #{id}
</select>
<select id="getUserByUsernameAndPassword" parameterType="user" resultType="user">
SELECT * FROM user WHERE username = #{username} AND password = #{password};
</select>
</mapper>
package com.cn.panda.controller;
import com.cn.panda.entity.Collect;
import com.cn.panda.service.CollectService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import javax.servlet.http.HttpSession;
@Controller
@RequestMapping("/collect")
public class CollectController {
@Autowired
public CollectService collectService;
@PostMapping("/on")
public String on(Collect collect,@RequestParam(name = "from",required = true)String from, HttpSession session){
boolean on = collectService.saveCollect(collect);
if(on){
session.setAttribute("message","收藏成功");
} else{
session.setAttribute("message", "收藏失败");
}
return "redirect:" + from;
}
@PostMapping("/off")
public String off(Collect collect,@RequestParam(name = "from",required = true)String from,HttpSession session){
boolean off = collectService.deleteCollect(collect);
if(off){
session.setAttribute("message","取消收藏成功");
}else{
session.setAttribute("message","取消收藏成功");
}
return "redirect:" + from;
}
}
package com.cn.panda.entity;
import java.util.Date;
public class Collect{
private int id;
private int uid;
private int type;
private int oid;
private Date collectTime = new Date();
public Collect(int id, int uid, int type, int oid, Date collectTime) {
this.id = id;
this.uid = uid;
this.type = type;
this.oid = oid;
this.collectTime = collectTime;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public int getUid() {
return uid;
}
public void setUid(int uid) {
this.uid = uid;
}
public int getType() {
return type;
}
public void setType(int type) {
this.type = type;
}
public int getOid() {
return oid;
}
public void setOid(int oid) {
this.oid = oid;
}
public Date getCollectTime() {
return collectTime;
}
public void setCollectTime(Date collectTime) {
this.collectTime = collectTime;
}
@Override
public String toString() {
return "Collect{" +
"id=" + id +
", uid=" + uid +
", type=" + type +
", oid=" + oid +
", collectTime=" + collectTime +
'}';
}
}
package com.cn.panda.mapper;
import com.cn.panda.entity.Collect;
import org.apache.ibatis.annotations.Param;
import org.springframework.stereotype.Repository;
@Repository
public interface CollectMapper{
Collect isCollect(@Param("uid")int uid, @Param("type")int type, @Param("oid")int oid);
long saveCollect(Collect collect);
long deleteCollect(Collect collect);
}
package com.cn.panda.service.impl;
import com.cn.panda.entity.Collect;
import com.cn.panda.mapper.CollectMapper;
import com.cn.panda.service.CollectService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class CollectServiceImpl implements CollectService{
@Autowired
private CollectMapper collectMapper;
@Override
public boolean isCollect(int uid, int type, int oid) {
return collectMapper.isCollect (uid,type,oid)!= null;
}
@Override
public boolean saveCollect(Collect collect) {
return collectMapper.saveCollect(collect)>0;
}
@Override
public boolean deleteCollect(Collect collect) {
return collectMapper.deleteCollect(collect)>0;
}
}
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.cn.panda.mapper.CollectMapper">
<select id="isCollect" resultType="collect">
SELECT *
FROM collect
WHERE uid = #{uid}
AND type = #{type}
AND oid = #{oid}
</select>
<insert id="saveCollect" parameterType="collect">
INSERT INTO collect(uid,type,oid,collectTime)
VALUES (#{uid},#{type},#{oid},#{collectTime})
</insert>
<delete id="deleteCollect" parameterType="collect">
DELETE FROM collect
WHERE uid = #{uid}
AND type = #{type}
AND oid = #{oid}
</delete>
</mapper>
package com.cn.panda.controller;
import com.cn.panda.entity.Collect;
import com.cn.panda.service.CollectService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import javax.servlet.http.HttpSession;
@Controller
@RequestMapping("/collect")
public class CollectController {
@Autowired
public CollectService collectService;
@PostMapping("/on")
public String on(Collect collect,@RequestParam(name = "from",required = true)String from, HttpSession session){
boolean on = collectService.saveCollect(collect);
if(on){
session.setAttribute("message","收藏成功");
} else{
session.setAttribute("message", "收藏失败");
}
return "redirect:" + from;
}
@PostMapping("/off")
public String off(Collect collect,@RequestParam(name = "from",required = true)String from,HttpSession session){
boolean off = collectService.deleteCollect(collect);
if(off){
session.setAttribute("message","取消收藏成功");
}else{
session.setAttribute("message","取消收藏成功");
}
return "redirect:" + from;
}
}
package com.cn.panda.entity;
import java.util.Date;
public class Order {
private int id;
private int uid;//用户id
private int type;//类型
private int oid;//对象id
private Date orderTime = new Date();
private int price;//价钱
private int state = 1;//状态
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public int getUid() {
return uid;
}
public void setUid(int uid) {
this.uid = uid;
}
public int getType() {
return type;
}
public void setType(int type) {
this.type = type;
}
public int getOid() {
return oid;
}
public void setOid(int oid) {
this.oid = oid;
}
public Date getOrderTime() {
return orderTime;
}
public void setOrderTime(Date orderTime) {
this.orderTime = orderTime;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
public int getState() {
return state;
}
public void setState(int state) {
this.state = state;
}
@Override
public String toString() {
return "Order{" +
"id=" + id +
", uid=" + uid +
", type=" + type +
", oid=" + oid +
", orderTime=" + orderTime +
", price=" + price +
", state=" + state +
'}';
}
public Order(int id, int uid, int type, int oid, Date orderTime, int price, int state) {
this.id = id;
this.uid = uid;
this.type = type;
this.oid = oid;
this.orderTime = orderTime;
this.price = price;
this.state = state;
}
}
package com.cn.panda.mapper;
import com.cn.panda.entity.Order;
import org.apache.ibatis.annotations.Param;
import org.springframework.stereotype.Repository;
@Repository
public interface OrderMapper {
Order getOrderByTypeAndIDs(@Param("uid") int uid,@Param("type")int type,@Param("oid")int oid);
long saveOrder(Order newOrder);
long updateOrderStatus();
}
package com.cn.panda.mapper;
import com.cn.panda.entity.Order;
import org.apache.ibatis.annotations.Param;
import org.springframework.stereotype.Repository;
@Repository
public interface OrderMapper {
Order getOrderByTypeAndIDs(@Param("uid") int uid,@Param("type")int type,@Param("oid")int oid);
long saveOrder(Order newOrder);
long updateOrderStatus();
}
package com.cn.panda.service.impl;
import com.cn.panda.entity.Order;
import com.cn.panda.mapper.OrderMapper;
import com.cn.panda.service.OrderService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.text.SimpleDateFormat;
@Service
public class OrderServiceImpl implements OrderService {
private OrderMapper orderMapper;
@Override
public Order getOrderByTypeAndIDs(int uid,int type,int oid){
return orderMapper.getOrderByTypeAndIDs(uid,type,oid);
}
/**
* 同一个商品每天只能购买一次;即:当天不可重复购买同一个商品
*/
@Override
public boolean saveOrder(Order newOrder){
int uid = newOrder.getUid();
int type = newOrder.getType();
int oid = newOrder.getOid();
//获取以往的最新的一个订单
Order oldOrder = orderMapper.getOrderByTypeAndIDs(uid,type,oid);
if(oldOrder==null){
//未购买
long result = orderMapper.saveOrder(newOrder);
if(result>0){
return true;
}else{
return false;
}
}else{
//曾经购买过
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
String newTime = sdf.format(newOrder.getOrderTime());
String oldTime = sdf.format(oldOrder.getOrderTime());
//比较时间判断是不是今天购买的
if (newTime.equals(oldTime)){
//以往的订单是今天购买
return false;
}else{
//以往的订单不是今天购买
long result = orderMapper.saveOrder(newOrder);
if (result>0){
return true;
}else{
return false;
}
}
}
}
@Override
public boolean updateOrderStatus() {
return orderMapper.updateOrderStatus()>0;
}
}
package com.cn.panda.service;
import com.cn.panda.entity.Order;
public interface OrderService {
Order getOrderByTypeAndIDs(int uid,int type,int oid);
boolean saveOrder(Order newOrder);
boolean updateOrderStatus();
}
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.cn.panda.mapper.OrderMapper">
<select id="getOrderByTypeAndIDs" resultType="order">
SELECT *
FROM userOrder
WHERE uid =#{uid}
AND type = #{type}
AND oid = #{oid}
ORDER BY orderTime DESC
LIMIT 0,1
</select>
<insert id="saveOrder" parameterType="order">
INSERT INTO userOrder(uid,type,oid,price,orderTime)
VALUES(#{uid},#{type},#{oid},#{price},#{orderTime})
</insert>
<update id="updateOrderStatus">
UPDATE userOrder
SET state = 0
WHERE TO_DAYS(NOW())-TO_DAYS(orderTime) > 0
</update>
</mapper>
package com.cn.panda.controller;
import com.cn.panda.entity.Attraction;
import com.cn.panda.entity.Order;
import com.cn.panda.entity.User;
import com.cn.panda.service.AttractionService;
import com.cn.panda.service.CollectService;
import com.cn.panda.service.OrderService;
import com.github.pagehelper.PageHelper;
import com.github.pagehelper.PageInfo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import javax.servlet.http.HttpServletRequest;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.List;
import static com.cn.panda.config.Type.*;
@Controller
@RequestMapping("/attraction")
public class AttractionController {
@Autowired
private AttractionService attractionService;
@Autowired
private CollectService collectService;
@Autowired
private OrderService orderService;
@RequestMapping("/list")
public String getAttraction(HttpServletRequest request,@RequestParam(required = true,name = "page",defaultValue = "1")Integer page){
//page:表示查询在第几页;8:表示每页显示的数量
PageHelper.startPage(page,8);
List<Attraction> attractions = attractionService.getAttractions();
//将attractions封装至PageInfo;5表示分页导航的最大页码数
PageInfo<Attraction> pageInfo = new PageInfo<>(attractions,5);
request.setAttribute("pageInfo",pageInfo);
//---------------以下为验证打印信息------------------
System.out.println("当前页:" + pageInfo.getPageNum());
System.out.println("总条数:" + pageInfo.getTotal());
System.out.println("每页显示的条数:" + pageInfo.getPageSize());
List<Attraction> list = pageInfo.getList();
for (int i=0;i<list.size();i++){
Attraction attraction = list.get(i);
System.out.println(attraction);
}
//---------------以下为验证打印信息------------------
return "front/attraction";
}
@RequestMapping("/{id}")
public String getAttractionById(@PathVariable(name = "id") int id,HttpServletRequest request){
//获取景点
Attraction attraction = attractionService.getAttractionById(id);
request.setAttribute("attraction",attraction);
//获取用户
User user = (User) request.getSession().getAttribute("user");
if (user != null){
int userID = user.getId();
//判断该用户是否已收藏此景点
boolean isCollect = collectService.isCollect(userID, ATTRACTION,id);
request.setAttribute("isCollect",isCollect);
//判断该用户是否已购买此景点
Order order = orderService.getOrderByTypeAndIDs(userID, ATTRACTION,id);
if (order!=null){
//比较购买时间(年月日)与今天(年月日)是否相等
String pattern = "yyyy-MM-dd";
SimpleDateFormat simpleDateFormat = new SimpleDateFormat(pattern);
Date createTimeDate = order.getOrderTime();
String createTimeString = simpleDateFormat.format(createTimeDate);
Date todayTimeDate = new Date();
String todayTimeString = simpleDateFormat.format(todayTimeDate);
if(createTimeString.equals(todayTimeString)){
request.setAttribute("isOrder",true);
}else{
request.setAttribute("isOrder",false);
}
}else{
request.setAttribute("isOrder",false);
}
}else{
request.setAttribute("isOrder",false);
}
return "front/attraction_detail";
}
}
package com.cn.panda.entity;
public class Attraction {
private int id;//编号
private String name;//名字
private String abridge;//缩略词
private String address;//地址
private int sales;//月销量
private int price;//门票费
private int threshold;//最低消费
private String header;//头部图片
private String month = "全年";//开放时间段
private String hour = "08:00-21:00";//具体时间
private String promise;//服务承诺
private String recommend;//推荐语
private String nickname;//网友昵称
private String feature;//景点特色
private String introduce;//景点介绍
public Attraction(int id, String name, String abridge, String address, int sales, int price, int threshold, String header, String month, String hour, String promise, String recommend, String nickname, String feature, String introduce) {
this.id = id;
this.name = name;
this.abridge = abridge;
this.address = address;
this.sales = sales;
this.price = price;
this.threshold = threshold;
this.header = header;
this.month = month;
this.hour = hour;
this.promise = promise;
this.recommend = recommend;
this.nickname = nickname;
this.feature = feature;
this.introduce = introduce;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAbridge() {
return abridge;
}
public void setAbridge(String abridge) {
this.abridge = abridge;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public int getSales() {
return sales;
}
public void setSales(int sales) {
this.sales = sales;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
public int getThreshold() {
return threshold;
}
public void setThreshold(int threshold) {
this.threshold = threshold;
}
public String getHeader() {
return header;
}
public void setHeader(String header) {
this.header = header;
}
public String getMonth() {
return month;
}
public void setMonth(String month) {
this.month = month;
}
public String getHour() {
return hour;
}
public void setHour(String hour) {
this.hour = hour;
}
public String getPromise() {
return promise;
}
public void setPromise(String promise) {
this.promise = promise;
}
public String getRecommend() {
return recommend;
}
public void setRecommend(String recommend) {
this.recommend = recommend;
}
public String getNickname() {
return nickname;
}
public void setNickname(String nickname) {
this.nickname = nickname;
}
public String getFeature() {
return feature;
}
public void setFeature(String feature) {
this.feature = feature;
}
public String getIntroduce() {
return introduce;
}
public void setIntroduce(String introduce) {
this.introduce = introduce;
}
@Override
public String toString() {
return "Attraction{" +
"id=" + id +
", name='" + name + '\'' +
", abridge='" + abridge + '\'' +
", address='" + address + '\'' +
", sales=" + sales +
", price=" + price +
", threshold=" + threshold +
", header='" + header + '\'' +
", month='" + month + '\'' +
", hour='" + hour + '\'' +
", promise='" + promise + '\'' +
", recommend='" + recommend + '\'' +
", nickname='" + nickname + '\'' +
", feature='" + f`在这里插入代码片`eature + '\'' +
", introduce='" + introduce + '\'' +
'}';
}
}
package com.cn.panda.mapper;
import com.cn.panda.entity.Attraction;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public interface AttractionMapper {
List<Attraction> getAttractions();
Attraction getAttractionById(int id);
long updateAttractionSales(Attraction attraction);
}
package com.cn.panda.service.impl;
import com.cn.panda.entity.Attraction;
import com.cn.panda.mapper.AttractionMapper;
import com.cn.panda.service.AttractionService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class AttractionServiceImpl implements AttractionService {
@Autowired
private AttractionMapper attractionMapper;
@Override
public List<Attraction> getAttractions() {
return attractionMapper.getAttractions ();
}
public Attraction getAttractionById(int id){
return attractionMapper.getAttractionById (id);
}
public boolean updateAttractionSales(Attraction attraction,int i) {
attraction.setSales (attraction.getSales() + i);
return attract`在这里插入代码片`ionMapper.updateAttractionSales(attraction) > 0;
}
}
package com.cn.panda.service;
import com.cn.panda.entity.Attraction;
import java.util.List;
public interface AttractionService {
List<Attraction> getAttractions();
Attraction getAttractionById(int id);
boolean updateAttractionSales(Attraction attraction,int i);
}
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.cn.panda.mapper.AttractionMapper">
<select id="getAttractions" resultType="attraction">
SELECT *
FROM attraction
</select>
<select id="getAttractionById" parameterType="int" resultType="attraction">
SELECT *
FROM attraction
WHERE id = #{id}
</select>
<update id="updateAttractionSale" parameterType="attraction">
UPDATE attraction
SET sales = #{sales}
WHERE id = #{id}
</update>
</mapper>
学习总结
首次接触Tocmat、Idea、maven、阿里云oss、java语言,完成一个结合css、mysql搭建出基本框架拥有基本登录、收藏、下单、浏览功能的旅游网站。