Future和Callable接口
- Future接口定义了操作异步任务执行一些方法,如获取异步任务的执行结果、取消任务的执行、判断任务是否被取消、判断任务执行是否完毕等。
- Callable接口中定义了需要有返回的任务需要实现的方法
- join和get对比,get会抛出异常,join不需要
- 示例代码如下
public class FutureTaskTest {
public static void main(String[] args) {
FutureTask<String> task = new FutureTask<>(new MyThread());
Thread t1 = new Thread(task, "t1");
t1.start();
try {
String res = task.get();
System.out.println(res);
} catch (InterruptedException e) {
e.printStackTrace();
} catch (ExecutionException e) {
e.printStackTrace();
}
}
}
class MyThread implements Callable<String>{
@Override
public String call() throws Exception {
System.out.println("come in call()...");
return "hello callable";
}
}
CompletableFuture类

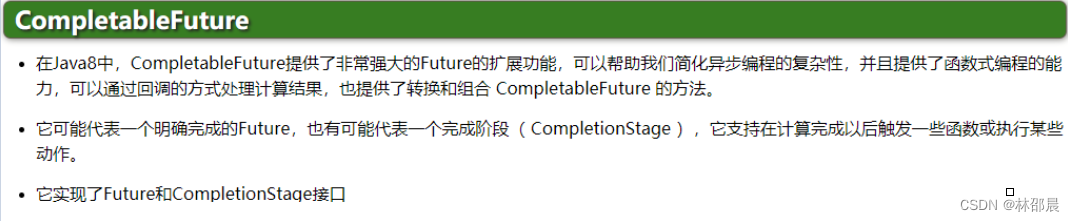

核心的四个静态方法
public static CompletableFuture<Void> runAsync(Runnable runnable)
public static CompletableFuture<Void> runAsync(Runnable runnable,Executor executor)
public static <U> CompletableFuture<U> supplyAsync(Supplier<U> supplier)
public static <U> CompletableFuture<U> supplyAsync(Supplier<U> supplier,Executor executor)
runAsync无返回值
public class CompletableFutureTest01 {
public static void main(String[] args) {
ExecutorService threadPool = Executors.newFixedThreadPool(1);
CompletableFuture<Void> completableFuture = CompletableFuture.runAsync(() -> {
System.out.println("run task...");
System.out.println(Thread.currentThread().getName());
try {
TimeUnit.SECONDS.sleep(3);
} catch (InterruptedException e) {
e.printStackTrace();
}
},threadPool);
try {
System.out.println(completableFuture.get());
} catch (InterruptedException e) {
e.printStackTrace();
} catch (ExecutionException e) {
e.printStackTrace();
}
threadPool.shutdown();
}
}
supplyAsync有返回值
public class CompletableFutureTest02 {
public static void main(String[] args) {
ExecutorService threadPool = Executors.newFixedThreadPool(1);
CompletableFuture<String> completableFuture = CompletableFuture.supplyAsync(() -> {
System.out.println("run task...");
System.out.println(Thread.currentThread().getName());
try {
TimeUnit.SECONDS.sleep(3);
} catch (InterruptedException e) {
e.printStackTrace();
}
return "hello supplyAsync";
},threadPool);
try {
System.out.println(completableFuture.get());
} catch (InterruptedException e) {
e.printStackTrace();
} catch (ExecutionException e) {
e.printStackTrace();
}
threadPool.shutdown();
}
}
CompletableFuture常用方法
supplyAsync有返回值,回调函数 & 异常处理
public class CompletableFutureTest03 {
public static void main(String[] args) {
ExecutorService threadPool = Executors.newFixedThreadPool(1);
try {
CompletableFuture<Integer> completableFuture = CompletableFuture.supplyAsync(() -> {
System.out.println("run task...");
System.out.println(Thread.currentThread().getName());
try {
TimeUnit.SECONDS.sleep(3);
} catch (InterruptedException e) {
e.printStackTrace();
}
int res = ThreadLocalRandom.current().nextInt(10);
return res;
},threadPool).whenComplete((v,e)->{
if (e==null){
System.out.println("任务执行完毕,没有出错!res="+v);
}
}).exceptionally(e->{
System.err.println("出现的异常:=>>"+e.getCause());
return null;
});
System.out.println(completableFuture.get());
} catch (Exception e) {
e.printStackTrace();
} finally {
threadPool.shutdown();
}
}
}
supplyAsync有返回值,返回结果处理
- thenApply(无异常处理) & handle(有异常处理)
public class CompletableFutureTest04 {
public static void main(String[] args) {
ExecutorService threadPool = Executors.newFixedThreadPool(1);
try {
CompletableFuture<Integer> completableFuture = CompletableFuture.supplyAsync(() -> {
System.out.println("run task...");
System.out.println(Thread.currentThread().getName());
try {
TimeUnit.SECONDS.sleep(3);
} catch (InterruptedException e) {
e.printStackTrace();
}
int res = 1;
return res;
},threadPool).handle((v,e)->{
if (e==null){
return v+2;
}
System.err.println(e.getCause());
return null;
}).whenComplete((v, e)->{
if (e==null){
System.out.println("任务执行完毕,没有出错!res="+v);
}
}).exceptionally(e->{
System.err.println("出现的异常:=>>"+e.getCause());
return null;
});
System.out.println(completableFuture.get());
} catch (Exception e) {
e.printStackTrace();
} finally {
threadPool.shutdown();
}
}
}
supplyAsync有返回值,返回结果消费 (消费完 无返回值)
public class CompletableFutureTest05 {
public static void main(String[] args) {
System.out.println(CompletableFuture.supplyAsync(()->"A").thenRun(()->{}).join());
System.out.println(CompletableFuture.supplyAsync(()->"A").thenAccept(r->{
System.out.println(r);
}).join());
}
}
supplyAsync有返回值,比较两个任务计算速度 (谁快)
public class CompletableFutureTest06 {
public static void main(String[] args) {
CompletableFuture<String> res1 = CompletableFuture.supplyAsync(() -> {
try {
TimeUnit.SECONDS.sleep(3);
} catch (InterruptedException e) {
e.printStackTrace();
}
return "1号";
});
CompletableFuture<String> res2 =CompletableFuture.supplyAsync(()->{
try {
TimeUnit.SECONDS.sleep(2);
} catch (InterruptedException e) {
e.printStackTrace();
}
return "2号";
});
CompletableFuture<String> ans = res1.applyToEither(res2, f -> {
return f + "is win";
});
System.out.println(ans.join());
}
}
supplyAsync有返回值,合并两个任务计算结果
public class CompletableFutureTest07 {
public static void main(String[] args) {
CompletableFuture<Integer> res1 = CompletableFuture.supplyAsync(() -> {
try {
TimeUnit.SECONDS.sleep(3);
} catch (InterruptedException e) {
e.printStackTrace();
}
return 10;
});
CompletableFuture<Integer> res2 =CompletableFuture.supplyAsync(()->{
try {
TimeUnit.SECONDS.sleep(2);
} catch (InterruptedException e) {
e.printStackTrace();
}
return 20;
});
CompletableFuture<Integer> ans = res1.thenCombine(res2,(x,y)->{
return x+y;
});
System.out.println(ans.join());
}
}