*零基础搭建spring boot项目---查看*
导入依赖包
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.0</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.47</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
<!--<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.2.4</version>
</dependency>-->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid-spring-boot-starter</artifactId>
<version>1.2.4</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<version>2.2.1.RELEASE</version>
<executions>
<execution>
<goals>
<!--可以把依赖的包都打包到生成的Jar包中-->
<goal>repackage</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
架构图
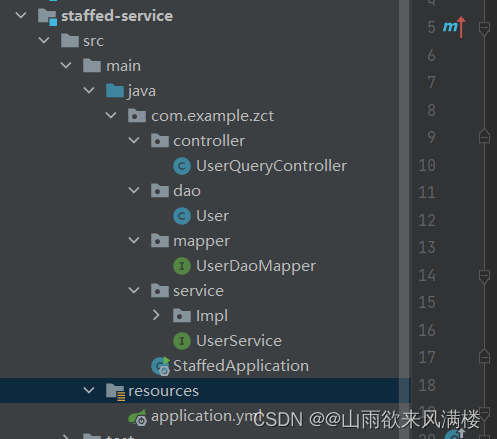
dao包中User类
package com.example.zct.dao;
import lombok.Data;
@Data
public class User {
private Integer id;
private String name;
private Integer age;
}
mapper包中UserDaoMapper接口
package com.example.zct.mapper;
import com.example.zct.dao.User;
import org.apache.ibatis.annotations.Select;
import java.util.List;
//@Mapper
public interface UserDaoMapper {
/**
* 查询所有
*
* @param
* @return List
*/
@Select("select *from user ")
List<User> QueryAll();
/**
* 查询单个
*
* @param id
* @return
*/
@Select("select * from user where id=#{id}")
User getById(Integer id);
}
service包中的UserService接口
package com.example.zct.service;
import com.example.zct.dao.User;
import java.util.List;
public interface UserService {
User getById(Integer id);
List<User> QueryAll();
}
创建一个UserServiceImpl实现UserService接口
package com.example.zct.service.Impl;
import com.example.zct.dao.User;
import com.example.zct.mapper.UserDaoMapper;
import com.example.zct.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserDaoMapper userDaoMapper;
@Override
public User getById(Integer id) {
return userDaoMapper.getById(id);
}
@Override
public List<User> QueryAll() {
return userDaoMapper.QueryAll();
}
}
controller包创建一个UserQueryController类
package com.example.zct.controller;
import com.example.zct.dao.User;
import com.example.zct.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
@RequestMapping("user")
public class UserQueryController {
@Autowired
UserService userService;
/**
* 查询所有
*
* @return
*/
@GetMapping("all")
public List<User> QueryAll() {
return userService.QueryAll();
}
/**
* 查询单个
*
* @param id
* @return
*/
@GetMapping("{id}")
public User getById(@PathVariable Integer id) {
return userService.getById(id);
}
}
启动类
package com.example.zct;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@MapperScan("com.example.zct.mapper")
public class StaffedApplication {
public static void main(String[] args) {
SpringApplication.run(StaffedApplication.class, args);
}
}
``
## rusouces下的application.yml文件
server:
port: 8089
#druid连接池配置的起步依赖方式
spring:
datasource:
druid:
url: jdbc:mysql:///boot_db?useSSL=false
username: root
password: 123456
mybatis:
configuration:
# 开启驼峰映射
map-underscore-to-camel-case: true
# 标准的日志输出
log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
数据库表
在values中根据创建表的字段进行添加名字即可
