0、结果
说明:先来看看串口调试助手显示的结果,第一个值是温度值,第二个值是大气压强,单位是百帕,第三个值是当前的海拔高度,如果是你想要的,可以接着往下看。
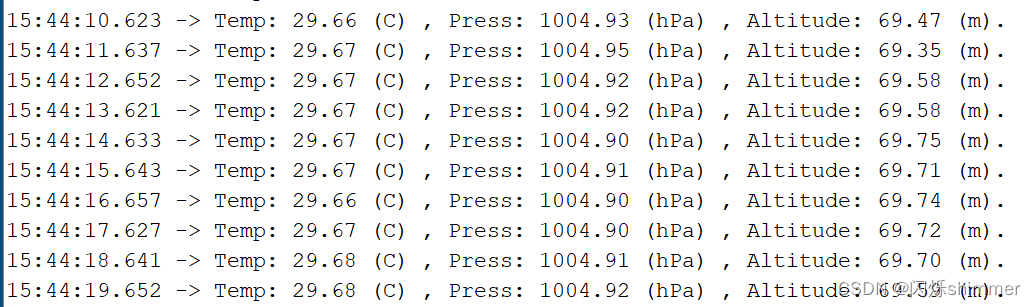
1、外观
说明:虽然BMP280大气压强传感器形态各异,但是原理和代码都是适用的。
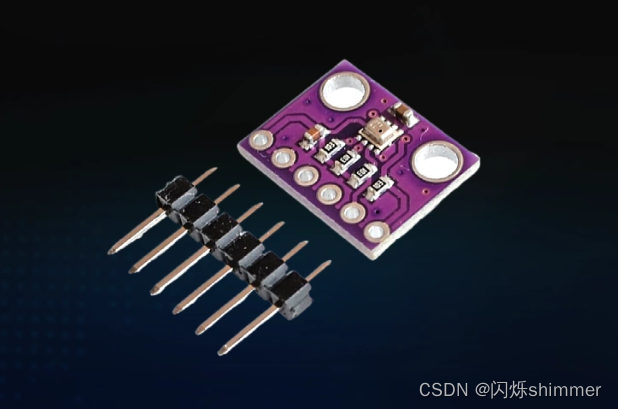
2、连线
说明:只需要连接四根线。
- uno————BMP280大气压强传感器
- 3.3V--------------VCC
- GND--------------GND
- SCL--------------SCL
- SDA--------------SDA
3、源程序
说明:采用非阻塞方式编写,一定时间检测一次温度、大气压强和海拔信息,并将对应功能进行函数化,方便移植。
/****************************************bmp280 part****************************************/
/*
wiring:
3.3V------VCC
GND------GND
SCL------SCL
SDA------SDA
*/
#include <Adafruit_BMP280.h> //include library
Adafruit_BMP280 bme; //Instantiate object
#define bmp280TimeInterval 1000 //Detect the time interval of a trip
unsigned long bmp280Times = 0; //Record the device running time
float bmp280Temp, bmp280Press, bmp280Altitude; //They are temperature, pressure and altitude
/****************************************set up and loop part*********************************/
void setup() {
Serial.begin(9600); //Example Set the baud rate of the serial port to 9600
bme.begin(0x76); //Example Initialize the IIC address of BMP280
Serial.println("Go online!");
}
void loop() {
getBmp280Data(); //Example Obtain data about BMP280
}
/****************************************bmp280 part****************************************/
/*Example Obtain data about BMP280*/
void getBmp280Data() {
if (millis() - bmp280Times >= bmp280TimeInterval) { //This command is executed once in a while
bmp280Times = millis();
bmp280Temp = bme.readTemperature(); //Acquired temperature
bmp280Press = bme.readPressure() / 100; //Get the air pressure, hpa
bmp280Altitude = bme.readAltitude(1013.25); //Elevation acquisition this should be adjusted to your local forcase
Serial.print("Temp: "); // The serial port displays the corresponding value
Serial.print(bmp280Temp); // The serial port displays the corresponding value
Serial.print(" (C) , "); // The serial port displays the corresponding value
Serial.print("Press: "); // The serial port displays the corresponding value
Serial.print(bmp280Press); // The serial port displays the corresponding value
Serial.print(" (hPa) , "); // The serial port displays the corresponding value
Serial.print("Altitude: "); // The serial port displays the corresponding value
Serial.print(bmp280Altitude); // The serial port displays the corresponding value
Serial.println(" (m)."); // The serial port displays the corresponding value
}
}
4、注意事项
说明:需要下载对应的库文件才不会编译报错。大气压强单位我转化成了百帕。如果求海拔高度不是很准,需要更改bme.readAltitude(1013.25)中的数字,根据当地的压强来改就行了,但实际也不用改,大差不差。导电物体例如手,最好不要碰到传感器,不然会造成短路,损坏传感器。
5、基本原理
热电效应:BMP280传感器内部具有一种PTAT(Proportional To Absolute Temperature)温度传感器和一种CTAT(Complementary To Absolute Temperature)温度传感器。当传感器发生温度变化时,PTAT和CTAT产生的热电效应就会引起输出电压的变化,从而实现温度测量。
压阻效应:BMP280传感器内部还安装了一个高精度的压阻元件,用来测量大气压强。当外界气压变化时,压阻元件的电阻值也相应改变,从而通过外部电路转化为电压信号输出。
信号处理:BMP280传感器内部的芯片会对PTAT、CTAT和压阻元件产生的电压信号进行采样和处理,以获得更加高精度和稳定的温度和气压值。
数据输出:BMP280传感器可以通过I2C或SPI接口与Arduino等控制器进行通信,将测量到的温度和气压值以数字信号的形式输出给控制器,从而实现对大气压强的测量和监控。