Java常用API
java.lang 包下的API不需要导包
1 包装类
将基本数据类型封装成对象的好处在于可以在对象中定义更多的功能方法操作该数据
最常用到的操作之一:用于基本数据类型与字符串之间的转换
基本类型对应的包装类:
基本数据类型 | 包装类 |
---|---|
byte | Byte |
short | Short |
int | Integer |
long | Long |
float | Float |
double | Double |
char | Character |
boolean | Boolean |
Integer
包装一个对象中的原始类型 int 的值
Integer可以不用初始化
Integer i = null;
Integer的构造方法
方法名 | 说明 |
---|---|
public Integer(int value) | 根据 int 值创建 Integer 对象(过时) |
public Integer(String s) | 根据 String 值创建 Integer 对象(过时) |
public static Integer valueOf(int i) | 返回表示指定的 int 值的 Integer 对象 |
public static Integer valueOf(String s) | 返回一个保存指定String值的 Integer 对象 ;如果传入的String不是数字,形如"abc",会报NumberFormatException的错误 |
int和String类型的相互转换
-
int转换为String
-
转换方式
- 方式一:直接在数字后加一个空字符串
- 方式二:通过String类静态方法valueOf()
-
示例代码
//int --- String int number = 100; //方式1 String s1 = number + ""; System.out.println(s1); //方式2 //public static String valueOf(int i) String s2 = String.valueOf(number); System.out.println(s2); System.out.println("--------");
-
-
String转换为int
-
转换方式
- 方式一:先将字符串数字转成Integer,再调用valueOf()方法
- 方式二:通过Integer静态方法parseInt()进行转换
-
示例代码
//String --- int String s = "100"; //方式1:String --- Integer --- int Integer i = Integer.valueOf(s); //public int intValue() int x = i.intValue(); System.out.println(x); //方式2 //public static int parseInt(String s) int y = Integer.parseInt(s); System.out.println(y);
-
自动拆箱与自动装箱
-
自动装箱
把基本数据类型转换为对应的包装类类型
-
自动拆箱
把包装类类型转换为对应的基本数据类型
-
示例代码
Integer i = 100; // 自动装箱 i += 200; // i = i + 200; i + 200 自动拆箱;i = i + 200; 是自动装箱
2 日期与时间
2.1 Date类
-
Date类概述
Date 代表了一个特定的时间,精确到毫秒
-
Date类构造方法
方法名 说明 public Date() 分配一个 Date对象,并初始化,以便它代表它被分配的时间,精确到毫秒 public Date(long date) 分配一个 Date对象,并将其初始化为表示从标准基准时间起指定的毫秒数 标准基准时间:
Thu Jan 01 08:00:00 CST 1970
-
示例代码
public class DateDemo01 { public static void main(String[] args) { //public Date():分配一个 Date对象,并初始化,以便它代表它被分配的时间,精确到毫秒 Date d1 = new Date(); System.out.println(d1); //输出Sun Nov 26 15:08:06 CST 2023 //public Date(long date):分配一个 Date对象,并将其初始化为表示从标准基准时间起指定的毫秒数 long date = 1000*60*60; Date d2 = new Date(date); System.out.println(d2); //输出Thu Jan 01 09:00:00 CST 1970 } }
-
常用方法
方法名 说明 public long getTime() 获取的是日期对象从 Sun Nov 26 15:12:58 CST 2023
public void setTime(long time) 设置时间,给的是毫秒值 //设置一个时间,这个时间距离 Sun Nov 26 15:12:58 CST 2023
有传入的参数那么多毫秒System.currentTimeMillis();
返回自1970年1月1日 00:00:00 GMT
以来当前时间的毫秒数;静态类Date类需要创建一个对象才可以调用
getTime()
函数 -
示例代码
public class DateDemo02 { public static void main(String[] args) { //创建日期对象 Date d = new Date(); //public long getTime():获取的是日期对象从1970年1月1日 00:00:00到现在的毫秒值 //System.out.println(d.getTime()); //System.out.println(d.getTime() * 1.0 / 1000 / 60 / 60 / 24 / 365 + "年"); //public void setTime(long time):设置时间,给的是毫秒值 //long time = 1000*60*60; long time = System.currentTimeMillis(); d.setTime(time); System.out.println(d); //输出现在的时间 } }
2.2 SimpleDateFormat类
-
SimpleDateFormat类概述
SimpleDateFormat
是一个具体的类(是DateFormat
这个抽象类的子类),用于以区域设置敏感的方式格式化和解析日期。 主要用于:日期格式化和解析
-
SimpleDateFormat类构造方法
方法名 说明 public SimpleDateFormat()
构造一个SimpleDateFormat,使用默认模式和日期格式 public SimpleDateFormat(String pattern)
构造一个SimpleDateFormat使用给定的模式和默认的日期格式 -
SimpleDateFormat类的常用方法
- 格式化(从Date到String)
public final String format(Date date)
:将日期格式化成日期/时间字符串
- 解析(从String到Date)
public Date parse(String source)
:从给定字符串的开始解析文本以生成日期
- 格式化(从Date到String)
-
示例代码
//格式化:从 Date 到 String Date d = new Date(); //SimpleDateFormat sdf = new SimpleDateFormat(); SimpleDateFormat sdf = new SimpleDateFormat("yyyy年MM月dd日 HH:mm:ss"); String s = sdf.format(d); System.out.println(s); System.out.println("--------"); //从 String 到 Date String ss = "2048-08-09 11:11:11"; //ParseException SimpleDateFormat sdf2 = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); Date dd = sdf2.parse(ss); System.out.println(dd);
2.3 Calendar类
Calendar为抽象类,Calendar在创建的时候并非直接创建,而是通过静态方法创建,返回子类对象,然后子类通过父类接受
其日历字段已使用当前日期和时间初始化:
Calendar rightNow = Calendar.getInstance();
- Calendar类常用方法
方法名 | 说明 |
---|---|
public int get(int field) | 返回给定日历字段的值;传入枚举值,分别获得年月日 |
public abstract void add(int field, int amount) | 根据日历的规则,将指定的时间量添加或减去给定的日历字段 |
public final void set(int year,int month,int date) | 设置当前日历的年月日 |
//获取日历类对象
Calendar c = Calendar.getInstance();
//public int get(int field):返回给定日历字段的值
int year = c.get(Calendar.YEAR);
int month = c.get(Calendar.MONTH) + 1;
int date = c.get(Calendar.DATE);
System.out.println(year + "年" + month + "月" + date + "日");
//三年前
c.add(Calendar.YEAR,-3);
3 Random类
作用:产生随机数
Random random = new Random();
random.nextInt(10); //产生0-9的数字 //e.g. 6
// 创建一个 Random 对象
Random random = new Random();
// 生成一个 0.0 到 1.0 之间的随机双精度浮点数
double randomValue = random.nextDouble(); //e.g. 0.8485394329946438
4 String类
4.1 String的构造方法
方法名 | 说明 |
---|---|
public String() | 空白字符串对象,不含有任何内容 |
public String(char[] chs) | 字符数组来创建字符串对象 |
public String(byte[] bys) | 字节数组来创建字符串对象 |
String s = “abc”; | 直接赋值的方式创建字符串对象,内容就是abc |
根据字节数组来创建字符串对象:
byte[] bys = {97, 98, 99};
String s3 = new String(bys);
System.out.println("s3:" + s3); //输出:s3:abc
4.2 String类的特点
-
Java 程序中所有的双引号字符串,都是 String 类的对象
-
字符串不可变,它们的值在创建后不能被更改
-
虽然 String 的值是不可变的,但是它们可以被共享
-
字符串效果上相当于字符数组( char[] ),但是底层原理是字节数组( byte[] ) JDK8以前是字符数组,JDK9及以后是字节数组
4.3 两种创建字符串对象的区别
-
通过构造方法创建
通过 new 创建的字符串对象,每一次 new 都会申请一个内存空间,虽然内容相同,但是地址值不同
-
直接赋值方式创建
以“”方式给出的字符串,只要字符序列相同(顺序和大小写),无论在程序代码中出现几次,JVM 都只会建立一个 String 对象,并在字符串池中维护
4.4 字符串的比较
4.4.1 ==号的作用
- 比较基本数据类型:比较的是具体的值
- 比较引用数据类型:比较的是对象地址值
4.4.2 equals方法的作用
-
方法介绍
public boolean equals(String s) 比较两个字符串内容是否相同、区分大小写
4.5 字符串常用方法
- 获取字符串信息:
length()
: 返回字符串的长度charAt(int index)
: 返回指定索引位置的字符
- 提取子字符串:
substring(int beginIndex)
: 返回从指定索引开始到字符串末尾的子字符串substring(int beginIndex, int endIndex)
: 返回从 beginIndex 到 endIndex - 1 的子字符串
- 字符串比较:
equals(Object another)
: 比较两个字符串的内容是否相等equalsIgnoreCase(String another)
: 比较两个字符串的内容是否相等,忽略大小写
- 查找字符串:
indexOf(String str)
: 返回指定子字符串在当前字符串中第一次出现的索引
- 替换字符:
replace(char oldChar, char newChar)
: 替换字符串中的字符toCharArray()
:将此字符串转换为新的字符串数组getBytes()
:该String转化为新的字节数组
- 大小写转换:
toLowerCase()
: 将字符串中的所有字符转换为小写toUpperCase()
: 将字符串中的所有字符转换为大写
5 StringBuilder类
StringBuilder 是一个可变的字符串类,我们可以把它看成是一个容器,这里的可变指的是 StringBuilder 对象中的内容是可变的
5.1 StringBuilder类和String类的区别
- String类:内容是不可变的
- StringBuilder类:内容是可变的
为什么String为什么不可变?StringBuffer/StringBuilder是可变的?
String类中有一个char数组,并且这个char数组是被final修饰的。因为数组一旦创建长度不可变。并且被final修饰的引用一旦指向某个对象之后,不可在指向其它对象,所以String是不可变的!
我看过源代码,StringBuffer/StringBuilder内部实际上是一个char[ ]数组,这个char[ ]数组没有被final修饰,StringBuffer和StringBulider的初始化容量应该为16,当存满之后会进行扩容,底层调用了数组拷贝的方法:System.arraycopy()…扩容的,所以StringBuffer/StringBuilder适用于字符串的频繁拼接操作;并且StringBuffer是线程安全的,StringBuilder是非线程安全的。
5.2 StringBuilder类的构造方法
-
常用的构造方法
方法名 说明 public StringBuilder() 创建一个空白可变字符串对象,不含有任何内容 public StringBuilder(String str) 根据字符串的内容,来创建可变字符串对象
5.3 StringBuilder类添加和反转方法
-
添加和反转方法
方法名 说明 public StringBuilder append(任意类型) 添加数据,并返回对象本身 public StringBuilder reverse() 返回相反的字符序列
5.4 StringBuilder和String相互转换
-
StringBuilder转换为String
public String toString():通过 toString() 就可以实现把 StringBuilder 转换为 String
-
String转换为StringBuilder
public StringBuilder(String s):通过构造方法就可以实现把 String 转换为 StringBuilder
6 StringBuffer类
6.1 构造方法
public StringBuffer()
:构造一个其中不带字符的字符串缓冲区,其初始容量为 16 个字符。
public StringBuffer(int capacity)
:指定容量的字符串缓冲区对象
public StringBuffer(String str)
: 指定字符串内容的字符串缓冲区对象
6.2 常用方法
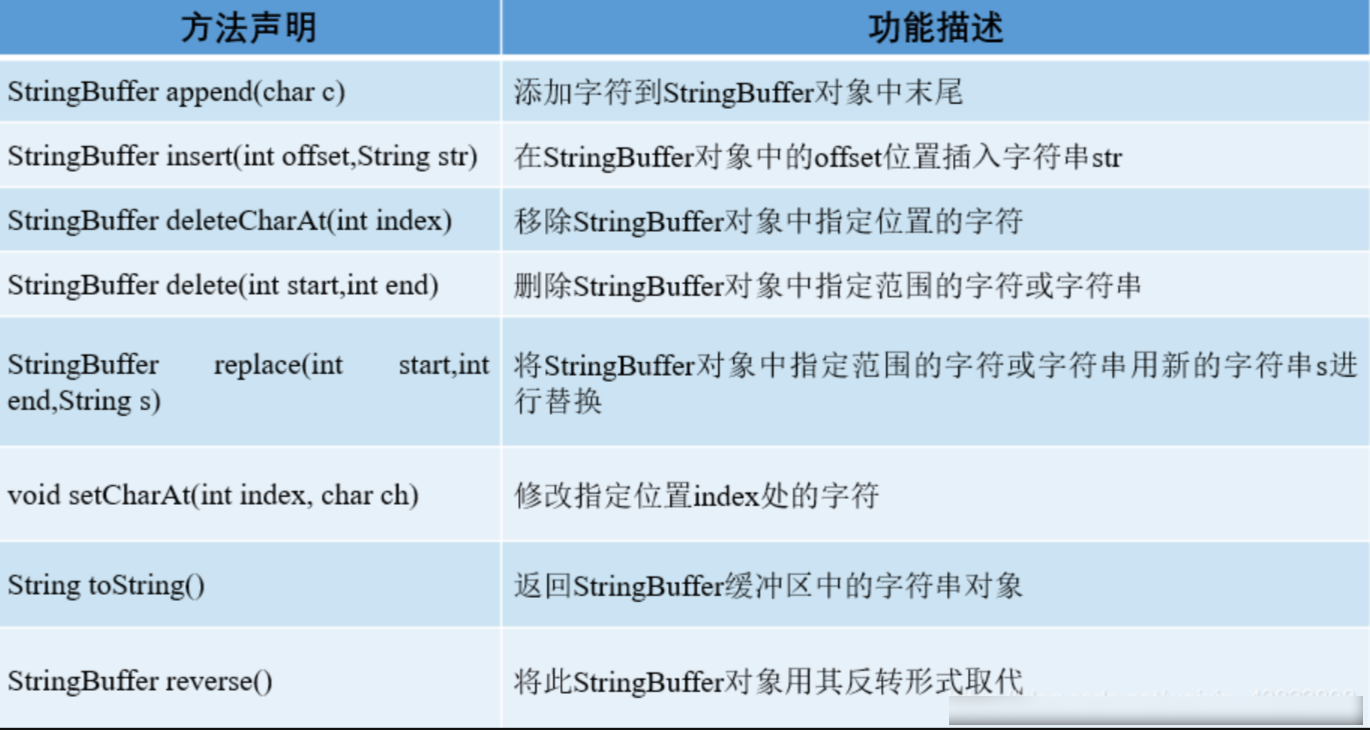
5 Math类
public static double abs (double num)
:返回绝对值
public static double ceil (double num)
:向上取整
public static double floor(double num)
:向下取整
public static long round(double num)
: 返回Math.floor(x+0.5),即“四舍五入”值
6 集合类
集合体系结构
-
集合类的特点
提供一种存储空间可变的存储模型,存储的数据容量可以随时发生改变
-
集合类的体系图
6.1 Collection集合
-
Collection集合概述
-
是单例集合的顶层接口,它表示一组对象,这些对象也称为Collection的元素
-
JDK 不提供此接口的任何直接实现,它提供更具体的子接口(如Set和List)实现
-
-
Collection集合基本使用
//创建Collection集合的对象 Collection<String> c = new ArrayList<String>(); //添加元素:boolean add(E e) c.add("hello"); c.add("world"); c.add("java"); //输出集合对象 System.out.println(c);
6.1.1 Collection集合的常用方法
方法名 | 说明 |
---|---|
boolean add(E e) | 添加元素 |
boolean remove(Object o) | 从集合中移除指定的元素 |
void clear() | 清空集合中的元素 |
boolean contains(Object o) | 判断集合中是否存在指定的元素 |
boolean isEmpty() | 判断集合是否为空 |
int size() | 集合的长度,也就是集合中元素的个数 |
6.1.2 Collection集合的遍历
- 迭代器的介绍
- 迭代器,集合的专用遍历方式
- Iterator iterator():返回此集合中元素的迭代器,通过集合的iterator()方法得到
- 迭代器是通过集合的iterator()方法得到的,所以我们说它是依赖于集合而存在的
- Collection集合的遍历
//创建集合对象
Collection<String> c = new ArrayList<>();
//添加元素
c.add("hello");
c.add("world");
c.add("java");
c.add("javaee");
//Iterator<E> iterator():返回此集合中元素的迭代器,通过集合的iterator()方法得到
Iterator<String> it = c.iterator();
//用while循环改进元素的判断和获取
while (it.hasNext()) {
String s = it.next();
System.out.println(s);
}
6.1.3 Collection集合数据结构
6.2 List集合
6.2.1 List集合概述和特点
- List集合概述
- 有序集合(也称为序列),用户可以精确控制列表中每个元素的插入位置。用户可以通过整数索引访问元素,并搜索列表中的元素
- 与Set集合不同,列表通常允许重复的元素
- List集合特点
- 有索引
- 可以存储重复元素
- 元素存取有序
6.2.2 List集合子类的特点
-
ArrayList集合
底层是数组结构实现,查询快、增删慢
-
LinkedList集合
底层是链表结构实现,查询慢、增删快
LinkedList集合的特有功能:
方法名 说明 public void addFirst(E e) 在该列表开头插入指定的元素 public void addLast(E e) 将指定的元素追加到此列表的末尾 public E getFirst() 返回此列表中的第一个元素 public E getLast() 返回此列表中的最后一个元素 public E removeFirst() 从此列表中删除并返回第一个元素 public E removeLast() 从此列表中删除并返回最后一个元素
6.2.3 List集合的特有方法(特有方法!)
方法名 | 描述 |
---|---|
void add(int index,E element) | 在此集合中的指定位置插入指定的元素 |
E remove(int index) | 删除指定索引处的元素,返回被删除的元素 |
E set(int index,E element) | 修改指定索引处的元素,返回被修改的元素 |
E get(int index) | 返回指定索引处的元素 |
6.2.4 并发修改异常 重点
-
出现的原因
迭代器遍历的过程中,通过集合对象修改了集合中的元素,造成了迭代器获取元素中判断预期修改值和实际修改值不一致,则会出现:
ConcurrentModificationException
-
解决的方案
用for循环遍历,然后用集合对象做对应的操作即可;或使用列表迭代器
-
示例代码
public class ListDemo { public static void main(String[] args) { //创建集合对象 List<String> list = new ArrayList<String>(); //添加元素 list.add("hello"); list.add("world"); list.add("java"); //遍历集合,得到每一个元素,看有没有"world"这个元素,如果有,我就添加一个"javaee"元素,请写代码实现 // Iterator<String> it = list.iterator(); // while (it.hasNext()) { // String s = it.next(); // if(s.equals("world")) { // list.add("javaee"); // } // } for(int i=0; i<list.size(); i++) { String s = list.get(i); if(s.equals("world")) { list.add("javaee"); } } //输出集合对象 System.out.println(list); } }
6.2.5 列表迭代器
-
ListIterator介绍
- 通过List集合的listIterator()方法得到,所以说它是List集合特有的迭代器
- 用于允许程序员沿任一方向遍历的列表迭代器,在迭代期间修改列表,并获取列表中迭代器的当前位置
-
示例代码
public class ListIteratorDemo { public static void main(String[] args) { //创建集合对象 List<String> list = new ArrayList<String>(); //添加元素 list.add("hello"); list.add("world"); list.add("java"); //获取列表迭代器 ListIterator<String> lit = list.listIterator(); while (lit.hasNext()) { String s = lit.next(); if(s.equals("world")) { lit.add("javaee"); } } System.out.println(list); } }
6.2.6增强for循环
-
定义格式
for(元素数据类型 变量名 : 数组/集合对象名) { 循环体; }
-
示例代码
int[] arr = {1,2,3,4,5}; for(int i : arr) { System.out.println(i); }
String[] strArray = {"hello","world","java"}; for(String s : strArray) { System.out.println(s); }
List<String> list = new ArrayList<String>(); list.add("hello"); list.add("world"); list.add("java"); for(String s : list) { System.out.println(s); }
//内部原理是一个Iterator迭代器 /* for(String s : list) { if(s.equals("world")) { list.add("javaee"); //ConcurrentModificationException } } */
for(元素数据类型 变量名 : 数组/集合对象名) {
循环体;
} -
示例代码
int[] arr = {1,2,3,4,5}; for(int i : arr) { System.out.println(i); }
String[] strArray = {"hello","world","java"}; for(String s : strArray) { System.out.println(s); }
List<String> list = new ArrayList<String>(); list.add("hello"); list.add("world"); list.add("java"); for(String s : list) { System.out.println(s); }
//内部原理是一个Iterator迭代器 /* for(String s : list) { if(s.equals("world")) { list.add("javaee"); //ConcurrentModificationException } } */
7 工具包
7.1 Commons-io
介绍:
Commons是apache开源基金组织提供的工具包,里面有很多帮助我们提高开发效率的API
比如:
-
StringUtils 字符串工具类
-
NumberUtils 数字工具类
-
ArrayUtils 数组工具类
-
RandomUtils 随机数工具类
-
DateUtils 日期工具类
-
StopWatch 秒表工具类
-
ClassUtils 反射工具类
-
SystemUtils 系统工具类
-
MapUtils 集合工具类
-
Beanutils bean工具类
-
Commons-io io的工具类
等等…
其中:Commons-io是apache开源基金组织提供的一组有关IO操作的开源工具包。
作用:提高IO流的开发效率。
使用方式:
1,新建lib文件夹
2,把第三方jar包粘贴到文件夹中
3,右键点击add as a library
代码示例:
public class CommonsIODemo1 {
public static void main(String[] args) throws IOException {
/*
FileUtils类
static void copyFile(File srcFile, File destFile) 复制文件
static void copyDirectory(File srcDir, File destDir) 复制文件夹
static void copyDirectoryToDirectory(File srcDir, File destDir) 复制文件夹
static void deleteDirectory(File directory) 删除文件夹
static void cleanDirectory(File directory) 清空文件夹
static String readFileToString(File file, Charset encoding) 读取文件中的数据变成成字符串
static void write(File file, CharSequence data, String encoding) 写出数据
IOUtils类
public static int copy(InputStream input, OutputStream output) 复制文件
public static int copyLarge(Reader input, Writer output) 复制大文件
public static String readLines(Reader input) 读取数据
public static void write(String data, OutputStream output) 写出数据
*/
/* File src = new File("myio\\a.txt");
File dest = new File("myio\\copy.txt");
FileUtils.copyFile(src,dest);*/
/*File src = new File("D:\\aaa");
File dest = new File("D:\\bbb");
FileUtils.copyDirectoryToDirectory(src,dest);*/
/*File src = new File("D:\\bbb");
FileUtils.cleanDirectory(src);*/
}
}
7.2 hutool
介绍:
Commons是国人开发的开源工具包,里面有很多帮助我们提高开发效率的API
比如:
-
DateUtil 日期时间工具类
-
TimeInterval 计时器工具类
-
StrUtil 字符串工具类
-
HexUtil 16进制工具类
-
HashUtil Hash算法类
-
ObjectUtil 对象工具类
-
ReflectUtil 反射工具类
-
TypeUtil 泛型类型工具类
-
PageUtil 分页工具类
-
NumberUtil 数字工具类
使用方式:
1,新建lib文件夹
2,把第三方jar包粘贴到文件夹中
3,右键点击add as a library
代码示例:
public class Test1 {
public static void main(String[] args) {
/*
FileUtil类:
file:根据参数创建一个file对象
touch:根据参数创建文件
writeLines:把集合中的数据写出到文件中,覆盖模式。
appendLines:把集合中的数据写出到文件中,续写模式。
readLines:指定字符编码,把文件中的数据,读到集合中。
readUtf8Lines:按照UTF-8的形式,把文件中的数据,读到集合中
copy:拷贝文件或者文件夹
*/
/* File file1 = FileUtil.file("D:\\", "aaa", "bbb", "a.txt");
System.out.println(file1);//D:\aaa\bbb\a.txt
File touch = FileUtil.touch(file1);
System.out.println(touch);
ArrayList<String> list = new ArrayList<>();
list.add("aaa");
list.add("aaa");
list.add("aaa");
File file2 = FileUtil.writeLines(list, "D:\\a.txt", "UTF-8");
System.out.println(file2);*/
/* ArrayList<String> list = new ArrayList<>();
list.add("aaa");
list.add("aaa");
list.add("aaa");
File file3 = FileUtil.appendLines(list, "D:\\a.txt", "UTF-8");
System.out.println(file3);*/
List<String> list = FileUtil.readLines("D:\\a.txt", "UTF-8");
System.out.println(list);
}
}