使用google的开发工具包ZXing生成二维码
一、导入jar包
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>core</artifactId>
<version>3.3.3</version>
</dependency>
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>javase</artifactId>
<version>3.3.3</version>
</dependency>
二、加入自定义二维码工具类
public class QRCodeUtil {
private static final Logger log= LoggerFactory.getLogger(QRCodeUtil.class);
private static final int CODE_WIDTH = 400;
private static final int CODE_HEIGHT = 400;
private static final int FRONT_COLOR = 0x000000;
private static final int BACKGROUND_COLOR = 0xFFFFFF;
public static void createCodeToFile(String content, File codeImgFileSaveDir, String fileName) {
try {
if (StringUtils.isEmpty(content) || StringUtils.isEmpty(fileName)) {
return;
}
content = content.trim();
if (codeImgFileSaveDir==null || codeImgFileSaveDir.isFile()) {
codeImgFileSaveDir = FileSystemView.getFileSystemView().getHomeDirectory();
}
if (!codeImgFileSaveDir.exists()) {
codeImgFileSaveDir.mkdirs();
}
BufferedImage bufferedImage = getBufferedImage(content);
File codeImgFile = new File(codeImgFileSaveDir, fileName);
ImageIO.write(bufferedImage, "png", codeImgFile);
log.info("二维码图片生成成功:" + codeImgFile.getPath());
} catch (Exception e) {
log.error("生成二维码失败:",e);
}
}
public static void createCodeToOutputStream(String content, HttpServletResponse response) {
try(PrintWriter out = response.getWriter()) {
if (StringUtils.isEmpty(content)) {
return;
}
response.setHeader("Content-type", "text/html;charset=UTF-8");
response.setContentType("text/html;charset=UTF-8");
response.setCharacterEncoding("UTF-8");
content = content.trim();
BufferedImage bufferedImage = getBufferedImage(content);
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
ImageIO.write(bufferedImage, "png", outputStream);
BASE64Encoder base64 = new BASE64Encoder();
String result = base64.encode(outputStream.toByteArray());
out.write(result);
out.flush();
log.info("二维码图片生成到输出流成功...");
} catch (Exception e) {
log.error("生成二维码失败:",e);
}
}
private static BufferedImage getBufferedImage(String content) throws WriterException {
Map<EncodeHintType, Object> hints = new HashMap();
hints.put(EncodeHintType.CHARACTER_SET, "UTF-8");
hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.M);
hints.put(EncodeHintType.MARGIN, 1);
MultiFormatWriter multiFormatWriter = new MultiFormatWriter();
BitMatrix bitMatrix = multiFormatWriter.encode(content, BarcodeFormat.QR_CODE, CODE_WIDTH, CODE_HEIGHT, hints);
BufferedImage bufferedImage = new BufferedImage(CODE_WIDTH, CODE_HEIGHT, BufferedImage.TYPE_INT_BGR);
for (int x = 0; x < CODE_WIDTH; x++) {
for (int y = 0; y < CODE_HEIGHT; y++) {
bufferedImage.setRGB(x, y, bitMatrix.get(x, y) ? FRONT_COLOR : BACKGROUND_COLOR);
}
}
return bufferedImage;
}
}
三、编写Controller类
@RestController
@Slf4j
public class TestController {
@PostMapping("/localTest")
public String localTest(String url,String path,String fileName){
try {
fileName = System.currentTimeMillis() + fileName + ".png";
QRCodeUtil.createCodeToFile(url,new File(path),fileName);
return "成功";
}catch (Exception e){
log.info("localTest 失败原因");
}
return "失败";
}
@PostMapping("/test")
public void test(String url,HttpServletResponse response){
try {
if(!StringUtils.isEmpty(url)){
QRCodeUtil.createCodeToOutputStream(url,response);
}
} catch (Exception e) {
log.info("test 失败原因");
}
}
}
测试:
一、本地:
链接:
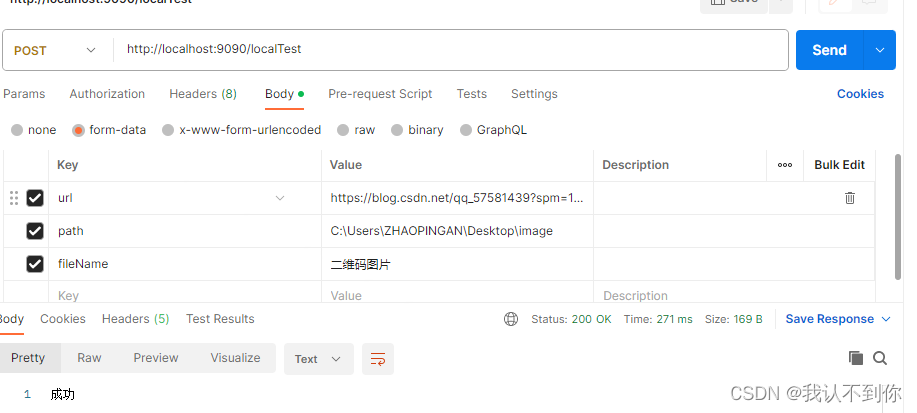
结果:
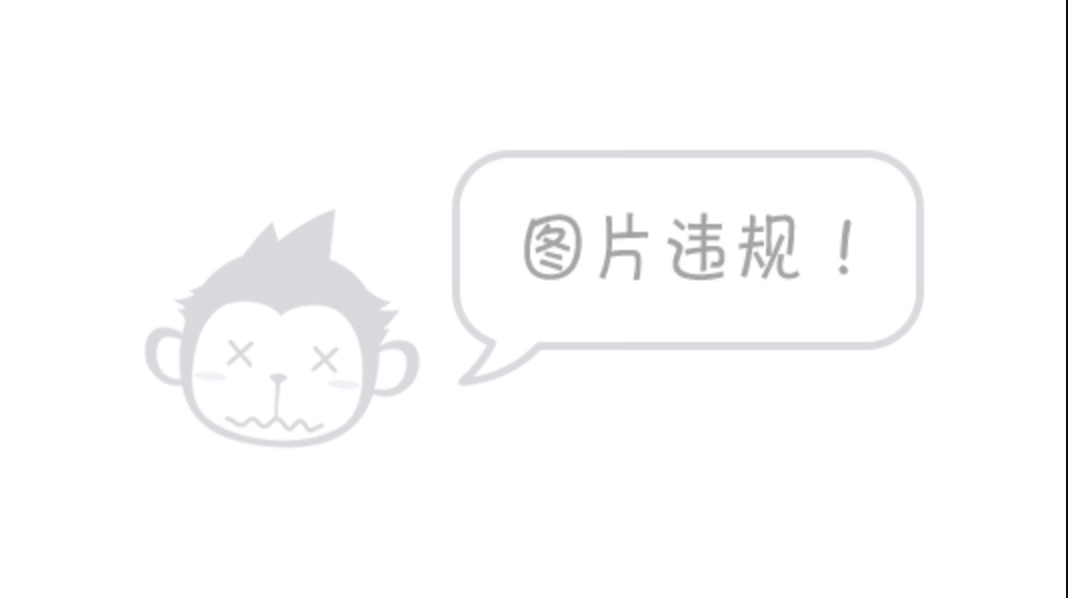
二、文件流
链接:
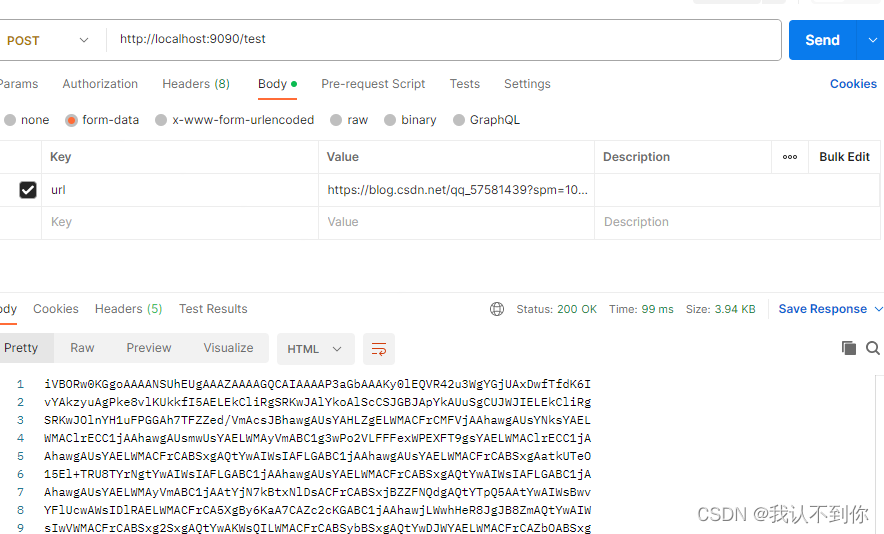
写入html中的img标签中:
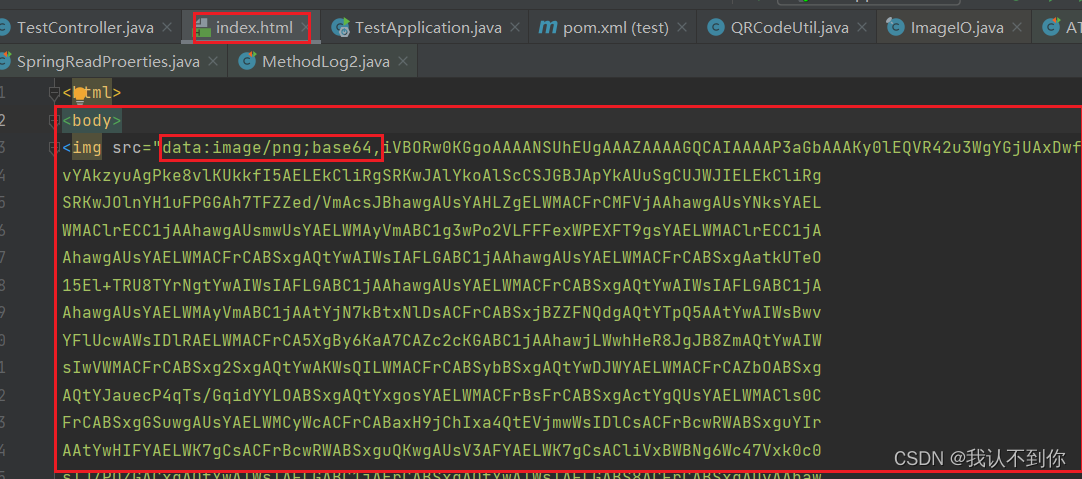
结果:
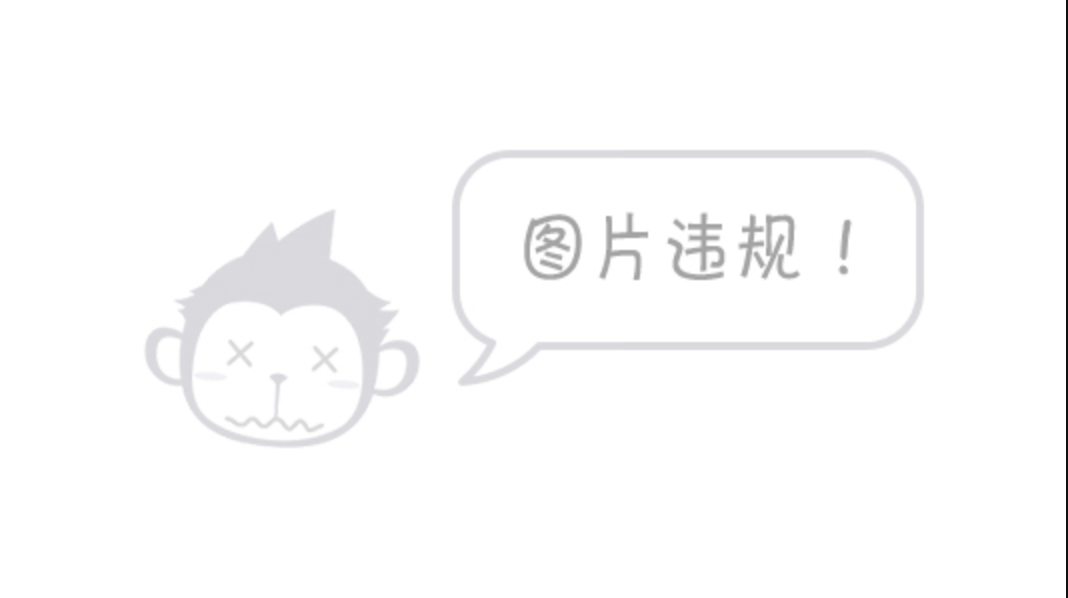