本文介绍单链表的简单使用:
单链表的创建
单链表的按位置插入
单链表的按位置删除
单链表的翻转
其他如按值删除等操作方法都是相似的,这里和代码均不没有说明和实现。
主要介绍下单链表的反转(图片来源于网络):
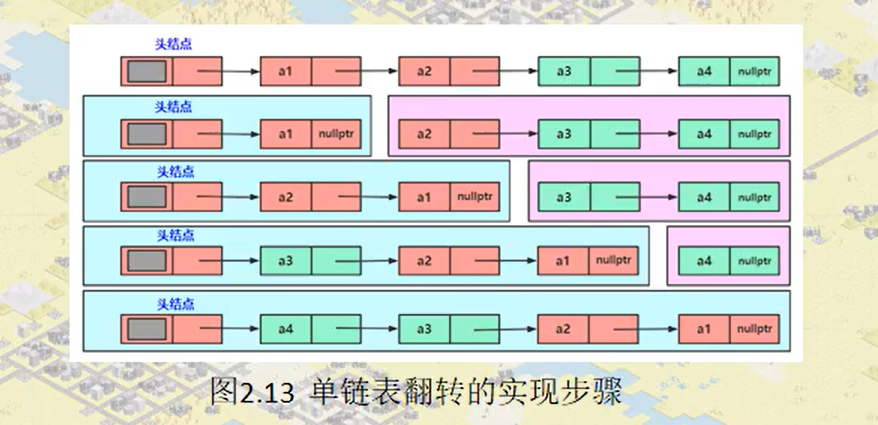
此处用的方法核心思想:将单链表分为两个部分,头结点和首节点为一组,后面的节点为一组。后一组的各个节点一个个往前一组移动。具体实现见代码。
当然,翻转单链表的实现方法有很多种,具体可参考下面链接:
http://c.biancheng.net/view/8105.html
linklist.h
#pragma one
#include <iostream>
using namespace std;
#define OK 1
#define FLASE -1
typedef int ElemType;
struct Node {
ElemType data;
Node *next;
};
typedef Node* LinkList;
void ListCreate(LinkList *L);
// insert
int ListInsert(LinkList *L, int sp, int data);
int ListLength(LinkList *L);
int ListDelete(LinkList *L, int sp);
int ListDisplay(LinkList *L);
void ListInvert(LinkList *L);
linklist.cpp
#include "linklist.h"
void ListCreate(LinkList *L)
{
Node *head = new Node;
head->next = NULL;
*L = head;
}
int ListInsert(LinkList *L, int sp, int data)
{
Node * temp = *L;
int length = ListLength(L);
if (sp < 1 || sp > length + 1) {
cout << "space error" << endl;
return FLASE;
}
Node *m_node = *L;
for (int i = 1 ; i < sp; i++) {
m_node = m_node->next;
}
Node *node = new Node;
node->data = data;
node->next = m_node->next;
m_node->next = node;
return OK;
}
int ListLength(LinkList *L)
{
Node *node = *L;
int length = 0;
while(node->next != NULL)
{
length++;
node = node->next;
}
return length;
}
int ListDisplay(LinkList *L)
{
Node *node = *L;
while (node->next != NULL) {
cout << node->next->data << " ";
node = node->next;
}
cout << endl;
return OK;
}
int ListDelete(LinkList *L,int sp)
{
Node *node = *L;
for (int i = 0; i < sp -1 ; i++)
{
node = node->next;
}
node ->next = node->next->next;
// delete node->next;
return OK;
}
void ListInvert(LinkList *L)
{
if (ListLength(L) < 2) {
cout << "no need invert" << endl;
}
Node *p_start = (*L)->next->next;
(*L)->next->next = NULL;
Node *node;
while (p_start != NULL) {
node = p_start;
p_start = p_start->next;
node ->next = (*L)->next;
(*L)->next = node;
// p_start = p_start->next;
}
}
int main()
{
int TAG = 0;
LinkList lethe = NULL;
ListCreate(&lethe);
TAG = ListInsert(&lethe, 1, 50);
TAG = ListInsert(&lethe, 2, 100);
TAG = ListInsert(&lethe, 3, 150);
TAG = ListInsert(&lethe, 4, 200);
TAG = ListInsert(&lethe, 4, 250);
TAG = ListInsert(&lethe, 4, 300);
int length = ListLength(&lethe);
cout << "ListLength = " << length << endl;
cout << "ListDisplay : ";
TAG = ListDisplay(&lethe);
ListDelete(&lethe, 2);
ListDelete(&lethe, 2);
length = ListLength(&lethe);
cout << "ListLength = " << length << endl;
cout << "ListDisplay : ";
TAG = ListDisplay(&lethe);
ListInvert(&lethe);
cout << "ListDisplay : ";
TAG = ListDisplay(&lethe);
return 0;
}
执行上述程序结果如下:
ListLength = 6
ListDisplay : 50 100 150 300 250 200
ListLength = 4
ListDisplay : 50 300 250 200
ListDisplay : 200 250 300 50