效果展示
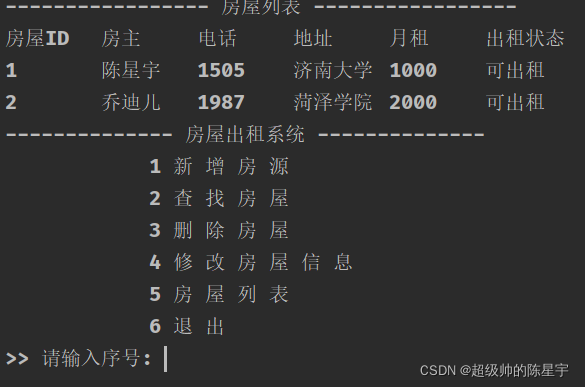
主类
package RentHouseSys;
import RentHouseSys.HouseView.MainView;
public class AppEntrance {
public static void main(String[] args) {
new MainView().view();
}
}
界面类
package RentHouseSys.HouseView;
import RentHouseSys.HouseServices.Services;
import java.util.Scanner;
public class MainView {
boolean signal = true;
Scanner scan = new Scanner(System.in);
Services service = new Services(10);
public void view() {
while (signal) {
MainMenu();
System.out.print(">> 请输入序号: ");
int answer = scan.nextInt();
switch (answer) {
case 1:
service.addHouse();
break;
case 2:
service.findHouse();
break;
case 3:
service.delHouse();
break;
case 4:
service.alterInfo();
break;
case 5:
service.showList();
break;
case 6:
signal = false;
System.out.println("退出系统!");
}
}
}
public void MainMenu() {
System.out.println("-------------- 房屋出租系统 --------------");
System.out.println("\t\t\t" + "1 新 增 房 源");
System.out.println("\t\t\t" + "2 查 找 房 屋");
System.out.println("\t\t\t" + "3 删 除 房 屋");
System.out.println("\t\t\t" + "4 修 改 房 屋 信 息");
System.out.println("\t\t\t" + "5 房 屋 列 表");
System.out.println("\t\t\t" + "6 退 出 ");
}
}
房屋类
package RentHouseSys.House;
public class House {
private int id;
private String owner;
private String telephone;
private String address;
private int monthlyRent;
private boolean rentStatus = true;
public House(int id, String owner, String telephone, String address, int monthlyRent) {
this.owner = owner;
this.telephone = telephone;
this.monthlyRent = monthlyRent;
this.address = address;
this.id = id;
}
public String transStatus() {
if (rentStatus) {
return "可出租";
}
return "已出租";
}
@Override
public String toString() {
return id + "\t\t" + owner + "\t" + telephone + "\t" + address + "\t" + monthlyRent + "\t" + transStatus();
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getOwner() {
return owner;
}
public void setOwner(String owner) {
this.owner = owner;
}
public String getTelephone() {
return telephone;
}
public void setTelephone(String telephone) {
this.telephone = telephone;
}
public int getMonthlyRent() {
return monthlyRent;
}
public void setMonthlyRent(int monthlyRent) {
this.monthlyRent = monthlyRent;
}
public boolean isRentStatus() {
return rentStatus;
}
public void setRentStatus(boolean rentStatus) {
this.rentStatus = rentStatus;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
}
服务类
package RentHouseSys.HouseServices;
import RentHouseSys.House.House;
import java.util.Scanner;
public class Services {
House[] houseList;
int len = 0;
Scanner scan = new Scanner(System.in);
public Services(int listCapacity) {
houseList = new House[listCapacity];
houseList[0] = new House(++len,"陈星宇", "1505", "济南大学", 1000);
houseList[1] = new House(++len, "乔迪儿", "1987", "菏泽学院", 2000);
}
public void addHouse() {
System.out.print("输入房主: ");
String owner = scan.next();
System.out.print("输入电话: ");
String telephone = scan.next();
System.out.print("输入地址: ");
String address = scan.next();
System.out.print("输入月租: ");
int monthlyRent = scan.nextInt();
House addedHouse = new House(++len, owner, telephone, address, monthlyRent);
houseList[len - 1] = addedHouse;
}
public void findHouse() {
System.out.print(">> 输入要查找的房屋ID: ");
int id = scan.nextInt();
for (int i = 0; i < len; i++) {
if (houseList[i].getId() == id) {
System.out.println("ID号为:" + houseList[i].getId());
System.out.println("房主为:" + houseList[i].getOwner());
System.out.println("电话为:" + houseList[i].getTelephone());
System.out.println("地址为:" + houseList[i].getAddress());
System.out.println("月租为:" + houseList[i].getMonthlyRent());
System.out.println("出租状态为:" + houseList[i].transStatus());
return;
}
}
System.out.println("未找到房屋信息!");
}
public void delHouse() {
System.out.print(">> 输入要删除的房屋ID: ");
int id = scan.nextInt();
for (int i = 0; i < len; i++) {
if (houseList[i].getId() == id) {
System.out.println("找到了该房屋信息并进行了删除!");
for (int j = i; j < len; j++) {
houseList[j] = houseList[j + 1];
}
len--;
return;
}
}
System.out.println("找不到房屋信息!");
}
public void alterInfo() {
System.out.print(">> 输入要修改信息的房屋ID: ");
int id = scan.nextInt();
for (int i = 0; i < len; i++) {
if (houseList[i].getId() == id) {
System.out.print("输入房主: ");
String owner = scan.next();
houseList[i].setOwner(owner);
System.out.print("输入电话: ");
String telephone = scan.next();
houseList[i].setTelephone(telephone);
System.out.print("输入地址: ");
String address = scan.next();
houseList[i].setAddress(address);
System.out.print("输入月租: ");
String monthlyRent = scan.next();
houseList[i].setAddress(monthlyRent);
System.out.println("修改成功!");
}
}
}
public void showList() {
System.out.println("----------------- 房屋列表 -----------------");
System.out.println("房屋ID" + "\t" + "房主" + "\t\t" + "电话" + "\t\t" + "地址" + "\t\t" + "月租" + "\t\t" + "出租状态");
for (int i = 0; i < len; i++) {
System.out.println(houseList[i].toString());
}
}
public void exit() {
return;
}
}