这是第二章,0基础可以看我第一章的Stream流
Stream基础
映射Map和flatMap
map映射 接收一个Lambda,将元素转换为其他形式 接收一个函数或者参数 该函数会被应用到每个元素上
先是Map映射
List<String> list = Arrays.asList("aaa,", "bbb,", "ccc", "ddd");
//将每个元素转换成大写 并且遍历
list.stream().map((str) -> str.toUpperCase())
.forEach(System.out::println);
将每一个元素提取出来 映射到Map上
然后问题来了 怎么把上边数组里边的元素 怎么一个个单独的提出来 再放到集合中
public static Stream<Character> filterCharacter(String str){
List<Character> list=new ArrayList<>();
for (Character ch : str.toCharArray()) {
list.add(ch);
}
return list.stream();
}
然后遍历集合
Stream<Stream<Character>> stream = list.stream().map(Stream02_::filterCharacter);
stream.forEach((str)->{
str.forEach(System.out::println);
});
flatMap接收一个函数作为参数 将流中每个值都换成另一种流 然后把所有的流连接成流
Stream<Character> stream1 = list.stream().flatMap(Stream02_::filterCharacter);
stream1.forEach(System.out::println);
- 总结 map和 flatMap区别
map是把{ {“a”,“a”,“a”,“b”,“b”,“b”} }aaabbb流整成一个大流 把{aaa}放入map中
flatMap是把{“a”,“a”,“a”,“b”,“b”,“b”}整合成了一个流 是把{aaa}中的a的元素放到flatMap
还记得集合中由add和addall方法么
List<String> list = Arrays.asList("aaa,", "bbb,", "ccc", "ddd");
ArrayList<Object> list1 = new ArrayList<>();
list1.add(11);
list1.add(22);
//list1.addAll(list);
list1.add(list);
System.out.println(list1);
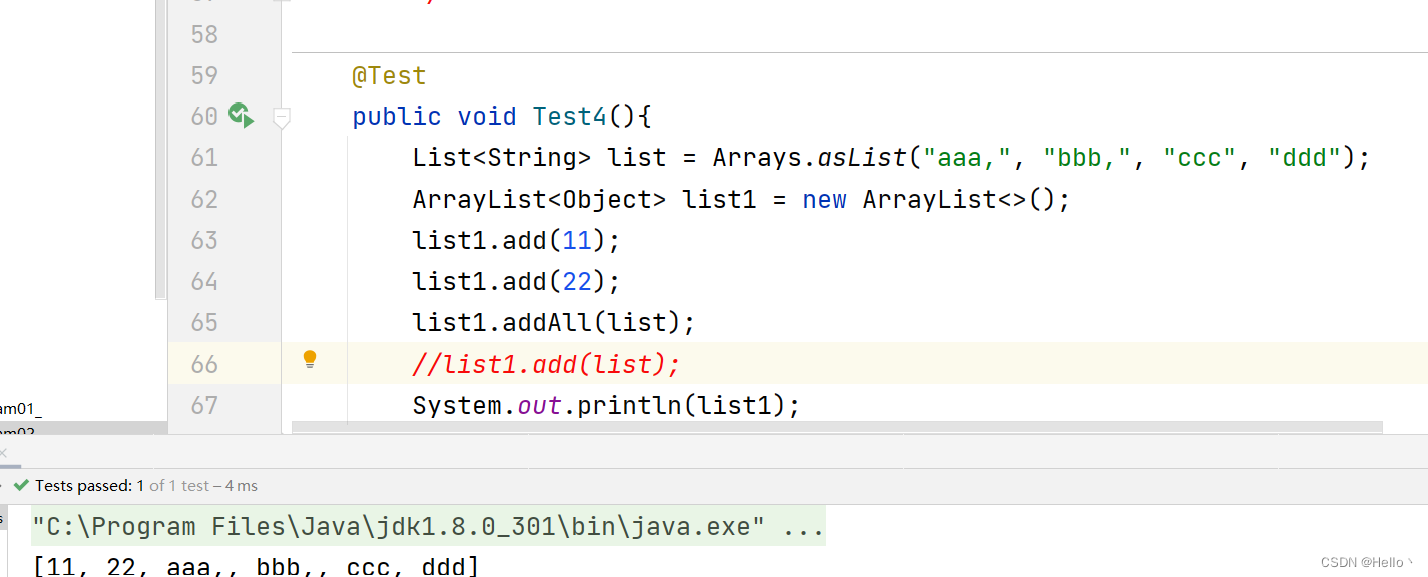
排序
sorted()排序 自然排序Comparable 因为String实现再自然排序 Double Integer等等
List<String> list = Arrays.asList("aaa","bbb","ccc","ddd","eee");
list.stream().sorted().forEach(System.out::println);
sorted(Comparator)排序 定制排序
emp.stream().sorted((e1,e2)->{
if (e1.getAge()==e2.getAge()){
return e1.getName().compareTo(e2.getName());
}else {
return -e1.getAge().compareTo(e2.getAge());
}
}).forEach(System.out::println);
按照年龄进行排序 如果年龄相同就按照名字
/*
allMatch-检查是否匹配所有元素
anyMatch-检查是否匹配至少一个元素
noneMatch-检查是否没有匹配的所有元素
findFirst-查找第一个元素
findAny-查找当前流中的任意元素
count-返回流的总数
max-返回流的最大值
min-返回流的最小值
*/
@Test
public void Test1() {
//allMatch-检查是否匹配所有元素
boolean b1 = employee.stream()
.allMatch((e) -> e.getStatus().equals(Employee.Status.BUSY));
System.out.println(b1);
System.out.println("===================================");
//anyMatch-检查是否匹配至少一个元素
boolean b2= employee.stream()
.anyMatch((e)->e.getStatus().equals(Employee.Status.BUSY));
System.out.println(b2);
System.out.println("===================================");
//noneMatch-检查是否没有匹配的所有元素
boolean b3=employee.stream()
.noneMatch((e)->e.getStatus().equals(Employee.Status.FREE));
System.out.println(b3);
System.out.println("===================================");
//findFirst-查找第一个元素 默认从低到高
Optional<Employee> first = employee.stream().sorted((e1, e2) ->
Double.compare(e1.getSalary(), e2.getSalary())
).findFirst();
System.out.println("first = " + first);
System.out.println("===================================");
//findAny-查找当前流中的任意元素
Optional<Employee> any = employee.stream().filter((e) -> e.getStatus().equals(Employee.Status.VOCATION)).findAny();
System.out.println(any.get());
System.out.println("===================================");
//count-返回流的总数
long count = employee.stream().filter((e) -> e.getStatus().equals(Employee.Status.VOCATION)).count();
System.out.println(count);
// max-返回流的最大值
System.out.println("===================================");
Optional<Employee> max = employee.stream().max((e1, e2) -> Double.compare(e1.getSalary(), e2.getSalary()));
System.out.println(max.get());
System.out.println("===================================");
employee.stream().map(Employee::getSalary).min(Double::compare);
}
归约T reduce(T identity, BinaryOperator accumulator) / reduce(BinaryOperator accumulator)
可以将流中的元素反复结合起来 得到一个值
先把100赋值给x y=1 会得到101 在去加2 得到103 以此类推 加到9
List<Integer>list=Arrays.asList(1,2,3,4,5,6,7,8,9);
Integer sum= list.stream().reduce(100,(x,y)->x+y);
System.out.println(sum);
System.out.println("=========================");
//需求工资的总和 类::静态方法名
Optional<Double> reduce = employee.stream().map(Employee::getSalary).reduce(Double::sum);
System.out.println(reduce.get());
collect
List<String> list= employee.stream()
.distinct()
.map(Employee::getName)
.collect(Collectors.toList());
把employee转换成list集合
Map<String, Double> collect = employee.stream().collect(Collectors.toMap(Employee::getName, Employee::getSalary));
System.out.println(collect);
把 employee转换成map集合 根据Name获取Salary