C++运算符重载
1.时间+重载
#include<iostream>
using namespace std;
class mytime {
private:
int m_hour;
int m_minutes;
public:
mytime(int m_hour, int m_minutes) :m_hour(m_hour), m_minutes(m_minutes) {};
mytime() :m_hour(0), m_minutes(0) {};
mytime operator+(const mytime& t) const;
int show_min();
int show_hour();
};
mytime mytime::operator + (const mytime& t)const
{
mytime sum;
sum.m_minutes = m_minutes + t.m_minutes;
sum.m_hour = t.m_hour + m_hour + sum.m_minutes / 60;
sum.m_minutes %= 60;
return sum;
}
int mytime::show_min()
{
return m_minutes;
}
int mytime::show_hour()
{
return m_hour;
}
int main(void)
{
mytime t1(20,35);
mytime t2(18, 27);
mytime t3 = t1 + t2;
printf("%d,%d",t3.show_hour(),t3.show_min());
return 1;
}
运行结果1:
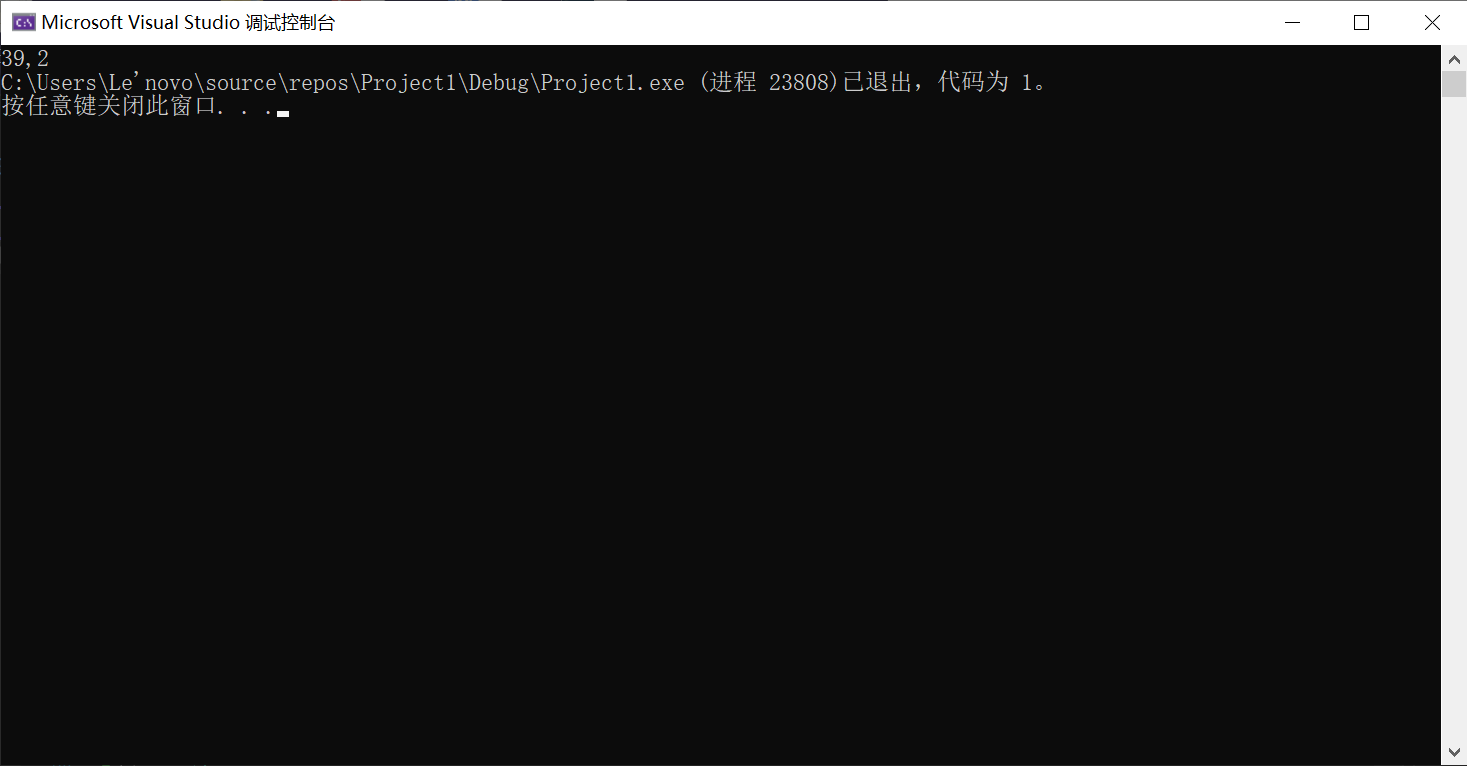
2.字符串比较>操作
#include<iostream>
using namespace std;
class string_new
{
private:
const char *string_n;
public:
string_new(const char* string_n):string_n(string_n){};
bool operator>(const string_new&)const;
};
bool string_new::operator>(const string_new& s)const
{
if (strcmp(s.string_n, string_n) > 0)return false;
else return true;
}
int main(void)
{
string_new s1("abc"), s2("abd");
printf("%d\n", s1 > s2);
printf("%d\n", s2 > s1);
return 0;
}
运行结果2:
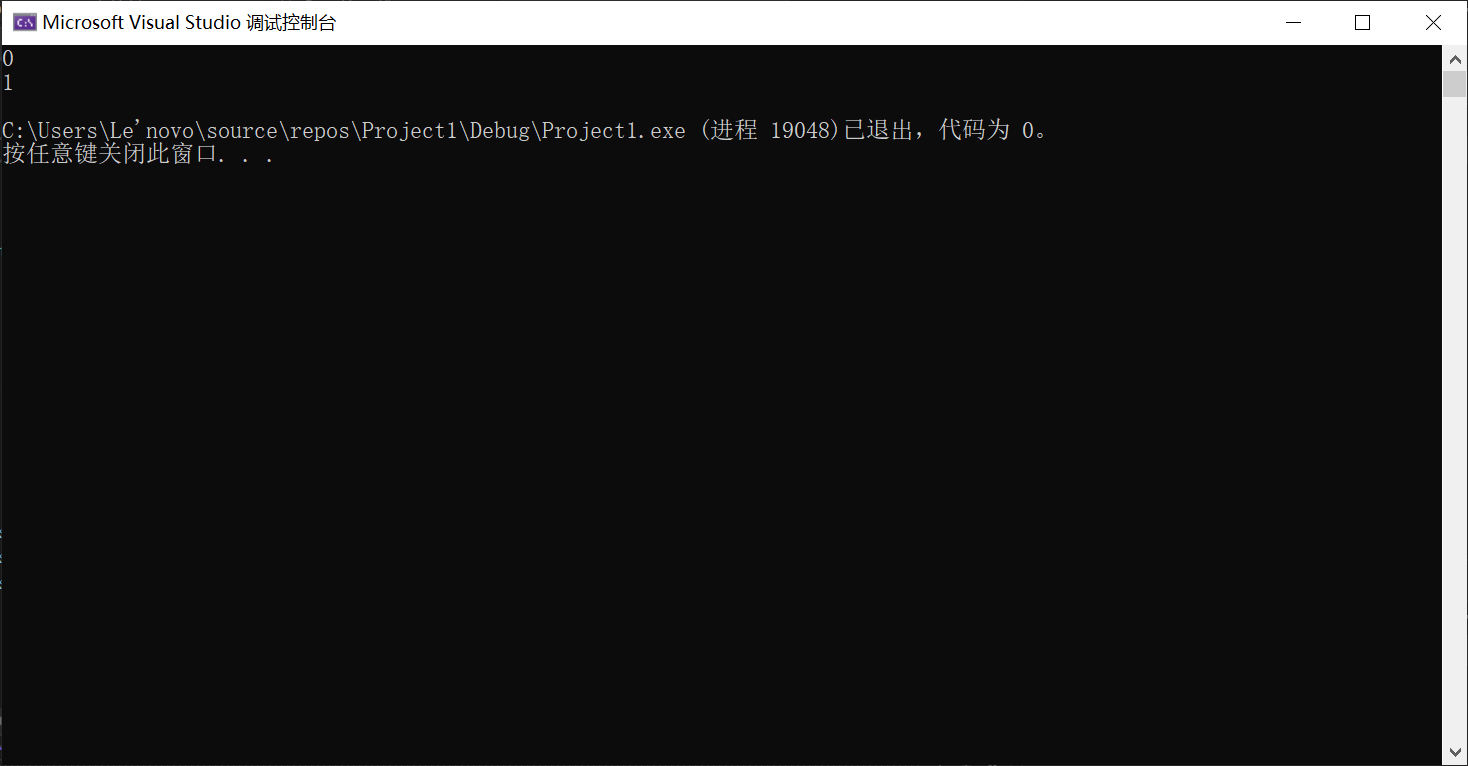
3.前++后++的重载
#include<iostream>
using namespace std;
class CMtime {
private:
int m_minutes;
int m_hour;
public:
CMtime(int hour, int min) :m_minutes(min), m_hour(hour) {};
CMtime() :m_minutes(0), m_hour(0) {};
CMtime operator ++();
CMtime operator ++(int);
int get_minutes(void);
int get_hour(void);
};
int CMtime::get_hour(void) {
return m_hour;
}
int CMtime::get_minutes(void) {
return m_minutes;
}
CMtime CMtime::operator++() //前++的重载,单目运算符,括号内不需要填入任何参数
{
m_minutes++;
if (m_minutes >= 60) {
m_minutes -= 60;
m_hour++;
}
return *this;
}
CMtime CMtime::operator++(int) //后++的重载,int是用来标记重载的是后++运算符
{
CMtime temp(*this); //拷贝*this对象
m_minutes++;
if (m_minutes >= 60) {
m_minutes -= 60;
m_hour++;
}
return temp;
}
int main(void)
{
CMtime t1(14, 59), t2(16, 21);
CMtime t3 = t2++;
CMtime t4 = ++t1;
printf("t3: %d : %d\n", t3.get_hour(), t3.get_minutes());
printf("t4: %d : %d\n", t4.get_hour(), t4.get_minutes());
return 0;
}
补充:this指针的含义与用法
# this指针为隐含传入的参数,指向调用该函数的对象,因此用*this可以取得该对象
# 在类函数后方加上const保留字指示对象的属性不可以在函数中被改变
* 在上例中,前++中return *this(先实现++,再返回被++后的Complex对象)
* 后++先创建*this的拷贝,再进行++操作
运行结果3:
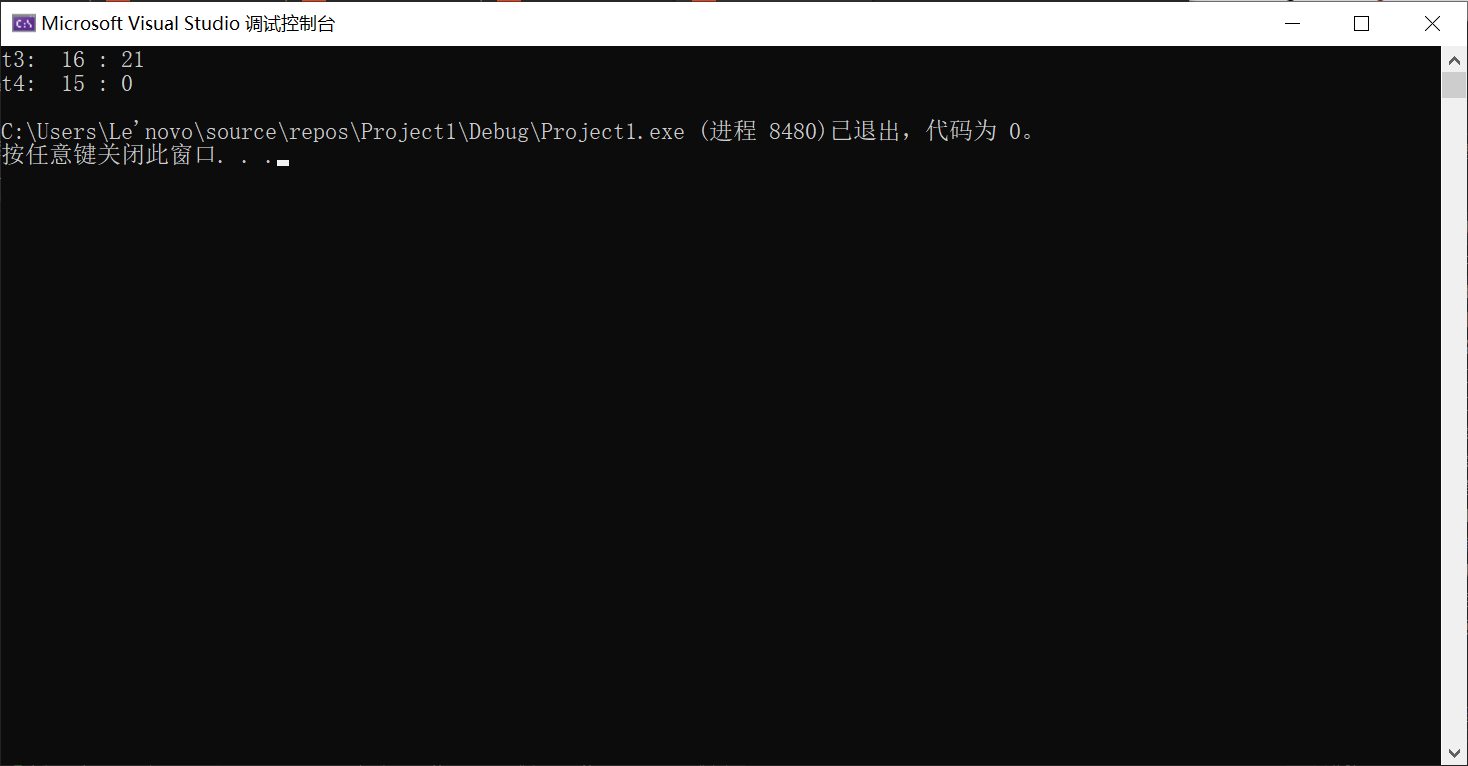
4.输出<<输出>>流重载
#include<iostream>
using namespace std;
class Complex {
private:
double real;
double image;
public:
Complex(double r, double i) :real(r), image(i) {};
Complex() :real(0), image(0) {};
friend Complex operator+(Complex&,Complex&);
friend ostream& operator<<(ostream&, Complex&); //必须定义成友元函数/普通函数,不能定义成成员函数
friend istream& operator>>(istream&, Complex&);
};
ostream& operator << (ostream &output, Complex &c1) {
output << c1.real << " + " << c1.image << "j";
return output;
}
istream& operator >> (istream& input, Complex& c2) {
input >> c2.real >> c2.image;
return input;
}
Complex operator+(Complex& c1, Complex& c2) {
Complex c3;
c3.real = c1.real + c2.real;
c3.image = c1.image + c2.image;
return c3;
}
int main(void) {
Complex c1(2.35, 2.73), c2, c3;
cin >> c2;
c3 = c1 + c2;
cout << c3 << endl;
return 0;
}
运行结果4:
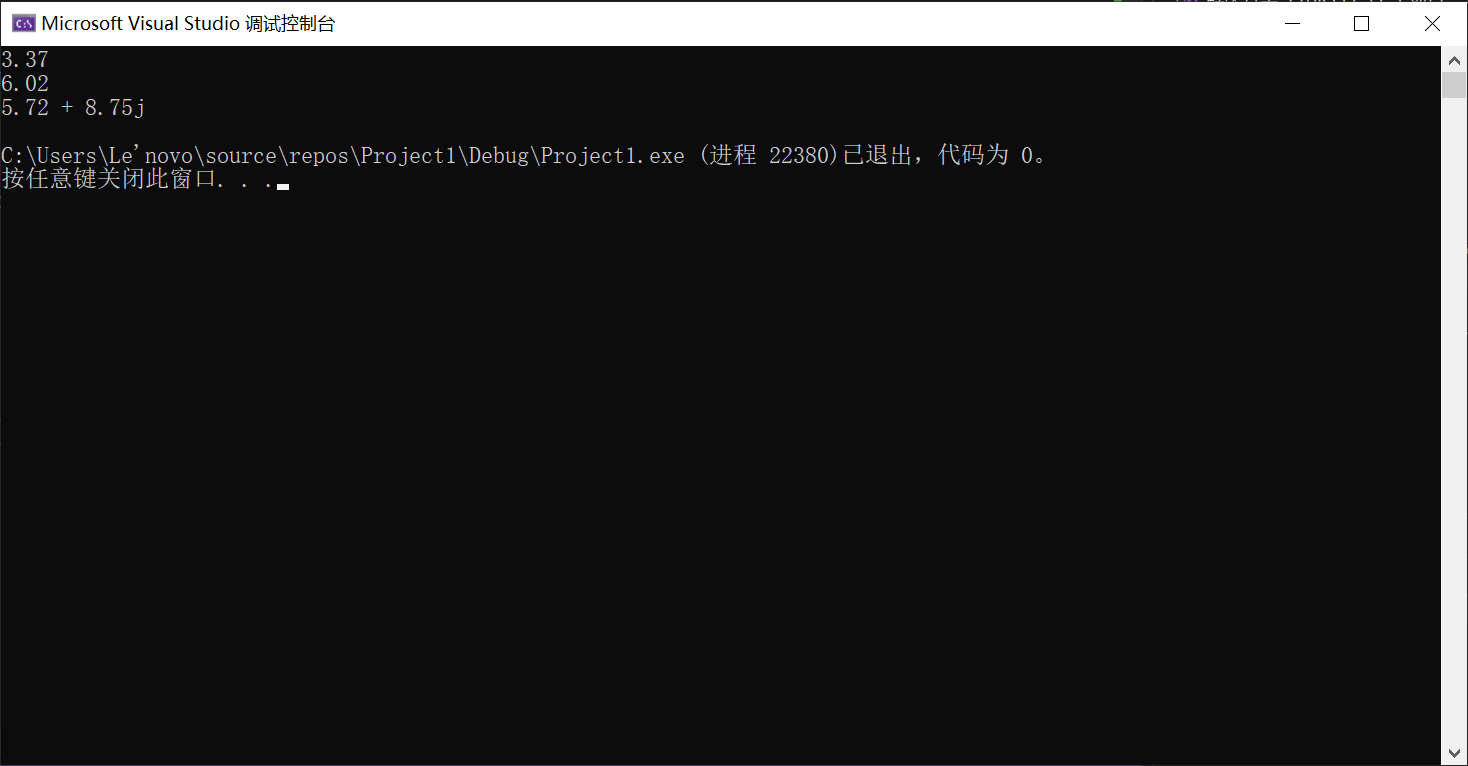
5.强制类型转化函数
#include<iostream>
using namespace std;
class Double {
private:
double D;
public:
Double() :D(0) {};
Double(double D) :D(D) {};
friend ostream& operator << (ostream& , Double &);
};
ostream& operator << (ostream& output, Double& d) {
output << d.D;
return output;
}
class Complex {
private:
double real;
double image;
public:
Complex(double r, double i) :real(r), image(i) {};
Complex() :real(0), image(0) {};
operator Double();
};
Complex::operator Double() {
cout << "Warning:强制类型转化可能使得信息损失!" << endl;
return real;
}
int main(void) {
Double d1;
Complex c1(3.27, 6.24);
d1 = c1;
cout << d1 << endl;
return 0;
}
注:强制类型转化函数只能作为成员函数被定义在类中
运行结果5:
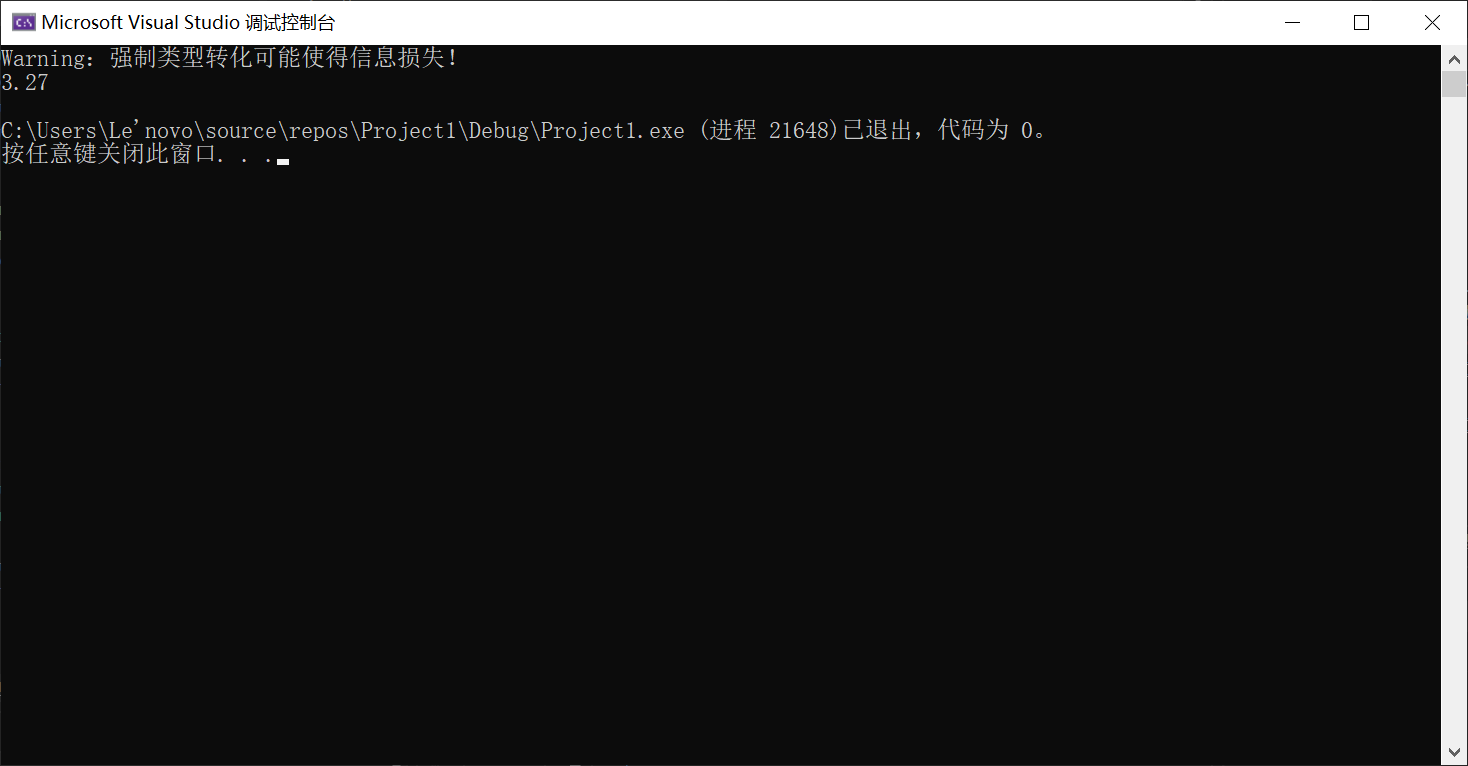
注:强制类型转化函数只能作为成员函数被定义在类中
运行结果5:
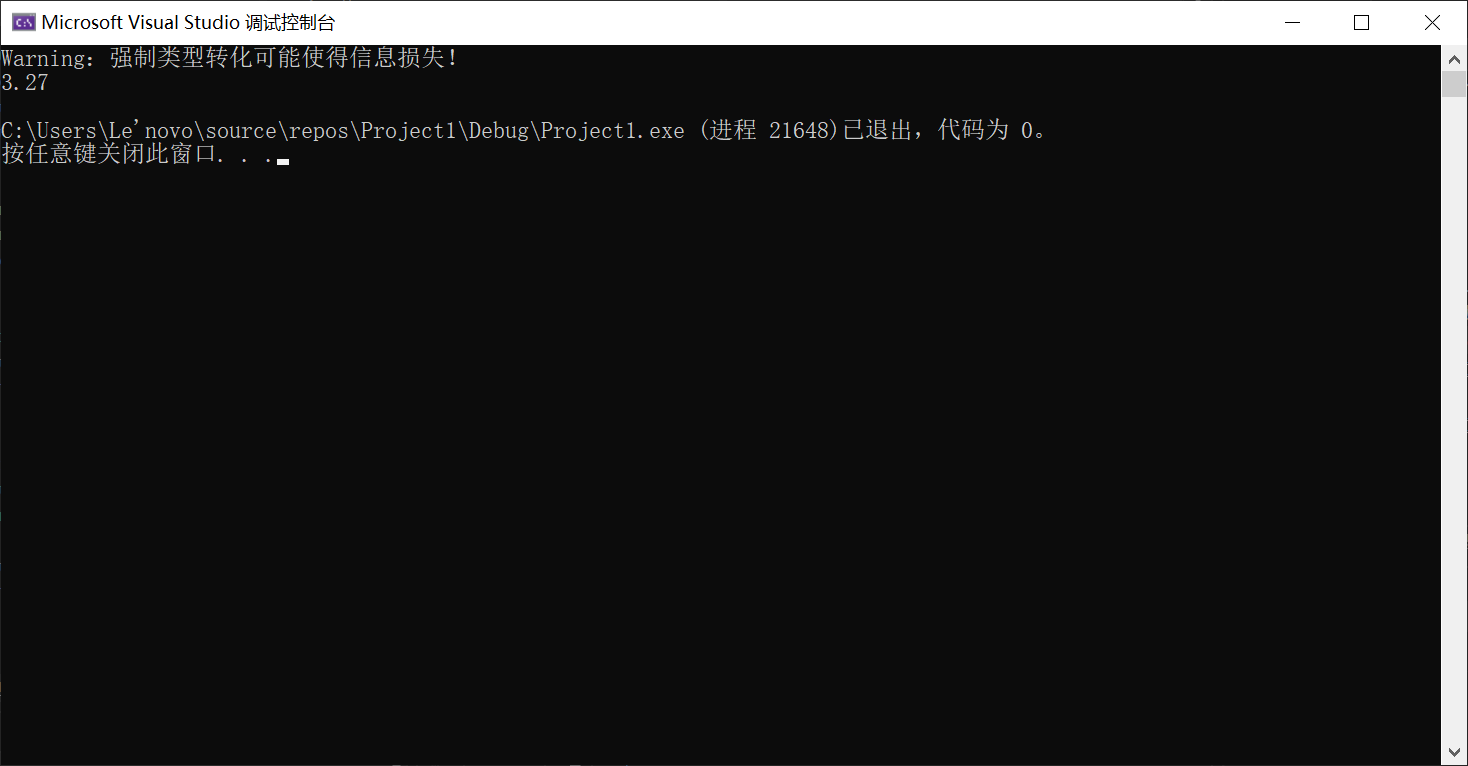