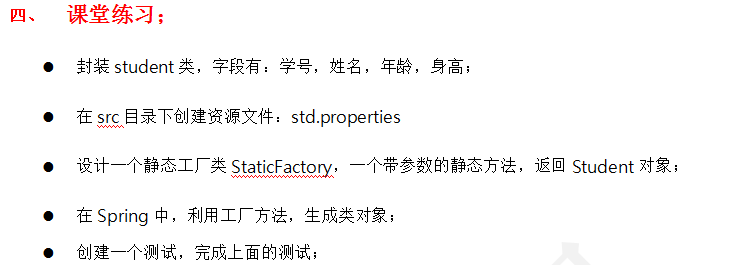
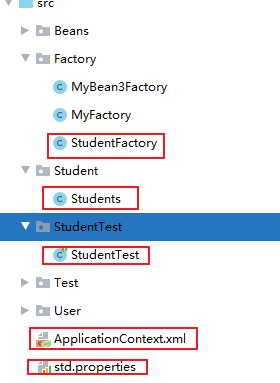
包:Student
Students.java
package Student;
public class Students {
private int ID;
private String name;
private int age;
private double height;
public Students setID(int ID) {
this.ID = ID;
return this;
}
public Students setName(String name) {
this.name = name;
return this;
}
public Students setAge(int age) {
this.age = age;
return this;
}
public Students setHeight(double height) {
this.height = height;
return this;
}
@Override
public String toString() {
return "Student{" +
"ID=" + ID +
", name='" + name + '\'' +
", age=" + age +
", height=" + height +
'}';
}
}
包:StudentTest
StudentTest.java
package StudentTest;
import Student.Students;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class StudentTest {
ClassPathXmlApplicationContext ctx = null;
@Before
public void before(){
ctx = new ClassPathXmlApplicationContext("ApplicationContext.xml");
System.out.println();
}
@After
public void after(){
ctx.close();
}
@Test
public void testStudent(){
var student = ctx.getBean("student", Students.class);
System.out.println("Students"+student);
}
}
包:Factory
StudentFactory.java
package Factory;
import Student.Students;
public class StudentFactory {
public static Students createStudent(int ID, String name, int age, double height){
System.out.println("--------参数和返回值都为复杂类型");
return new Students().setID(ID).setName(name).setAge(age).setHeight(height);
}
}
src下:std.properties
std.id=2023
std.name=WuWeiXian
std.age=20
std.height=185
src下:ApplicationContest.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:contest="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd">
<contest:property-placeholder location="classpath:std.properties"/>
<bean id="student" class="Factory.StudentFactory" lazy-init="true" factory-method="createStudent">
<constructor-arg name = "ID" value="${std.id}"/>
<constructor-arg name ="name" value="${std.name}"/>
<constructor-arg name = "age" value="${std.age}"/>
<constructor-arg name = "height" value ="${std.height}"/>
</bean>
</beans>
运行图:
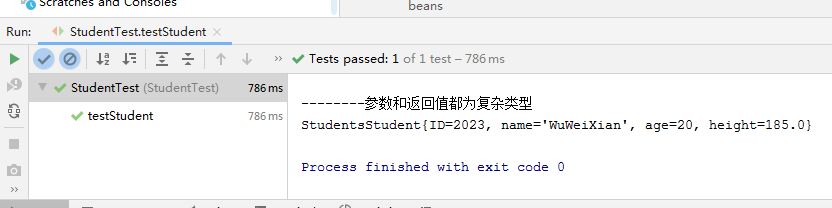