将dict.txt存储到数据库中
#include<myhead.h>
#define ERR(msg) do{\
fprintf(stderr, "__%d__:", __LINE__);\
perror(msg);\
}while(0);
int main(int argc, const char *argv[])
{
FILE* fp;
fp = fopen("./dict.txt", "r");
if(fp == NULL)
{
ERR("fopen");
return -1;
}
sqlite3 *db;
if(sqlite3_open("./dict.db", &db) != SQLITE_OK)
{
fprintf(stderr, "sqlite3_open: %s %d __%d__\n", \
sqlite3_errmsg(db), sqlite3_errcode(db), __LINE__);
return -1;
}
printf("sqlite3_open success\n");
char sql[128] = "create table if not exists dict (word char, explain char);";
char* errmsg = NULL;
if(sqlite3_exec(db, sql, NULL, NULL, &errmsg) != SQLITE_OK)
{
fprintf(stderr, "sqlite3_exec: %s __%d__\n", errmsg, __LINE__);
return -1;
}
printf("create table dict success\n");
char word[128] = "";
char explain[128] = "";
char temp[128] = "";
while(1)
{
bzero(sql, sizeof(sql));
bzero(word, sizeof(word));
bzero(temp, sizeof(temp));
bzero(explain, sizeof(explain));
int i = 0;
fgets(temp, 128, fp);
temp[strlen(temp)-1] = 0;
for(i=0; *(temp+i)!=' '; i++)
*(word+i) = *(temp+i);
for(; *(temp+i)==' '; i++);
//以下两种方法皆可
//strcpy(explain, &temp[i]);
for(int j=0; *(temp+i)!=0; i++,j++)
*(explain+j) = *(temp+i);
sprintf(sql, "insert into dict values(\"%s\", \"%s\");", word, explain);
printf("%s\n", sql);
if(sqlite3_exec(db, sql, NULL, NULL, &errmsg) != SQLITE_OK)
{
fprintf(stderr, "sqlite3_exec: %s __%d__\n", errmsg, __LINE__);
return -1;
}
//判断结尾
if(fgetc(fp) == EOF)
{
break;
}
fseek(fp, -1, SEEK_CUR);
}
if(sqlite3_close(db) != SQLITE_OK)
{
fprintf(stderr, "sqlite3_close: %s %d __%d__\n", sqlite3_errmsg(db), sqlite3_errcode(db), __LINE__);
return -1;
}
printf("sqlite3_close success\n");
fclose(fp);
return 0;
}
效果图
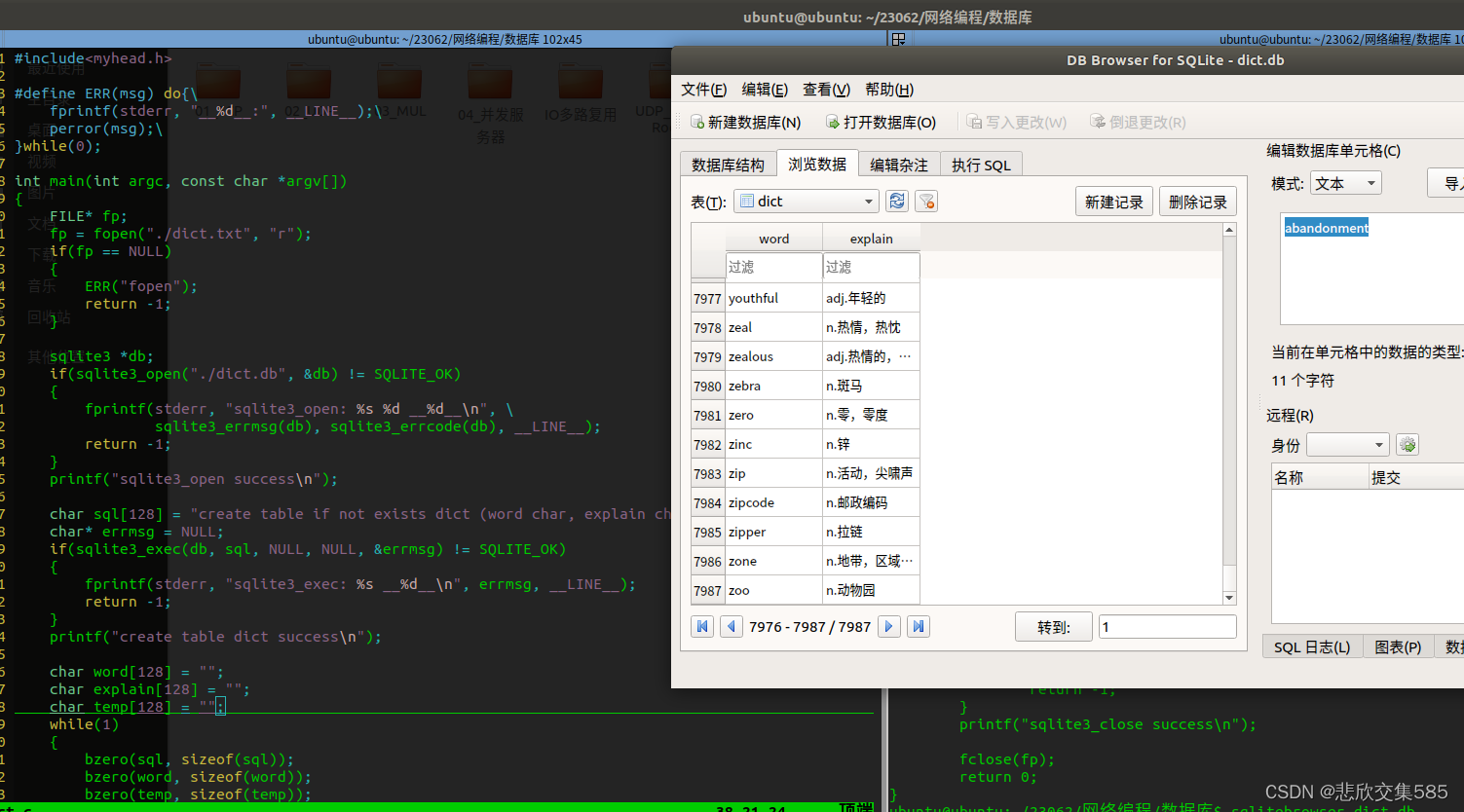