有头循环链表
无头循环链表
约瑟夫环
有头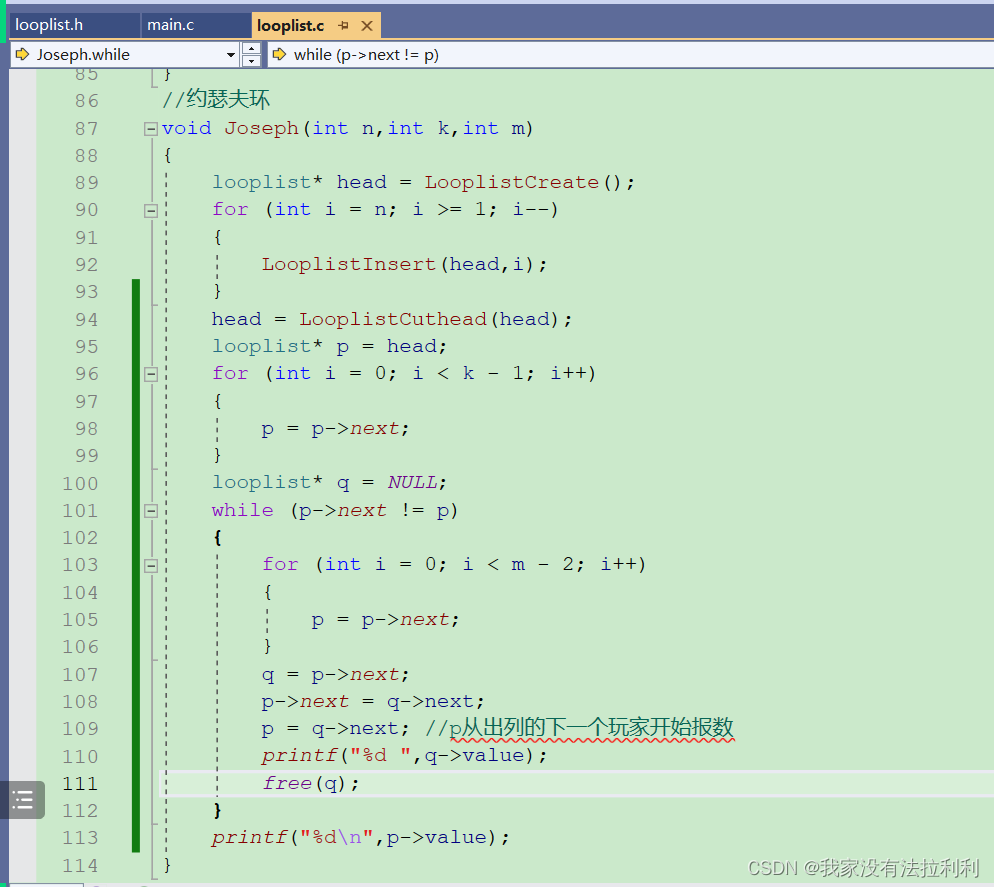
无头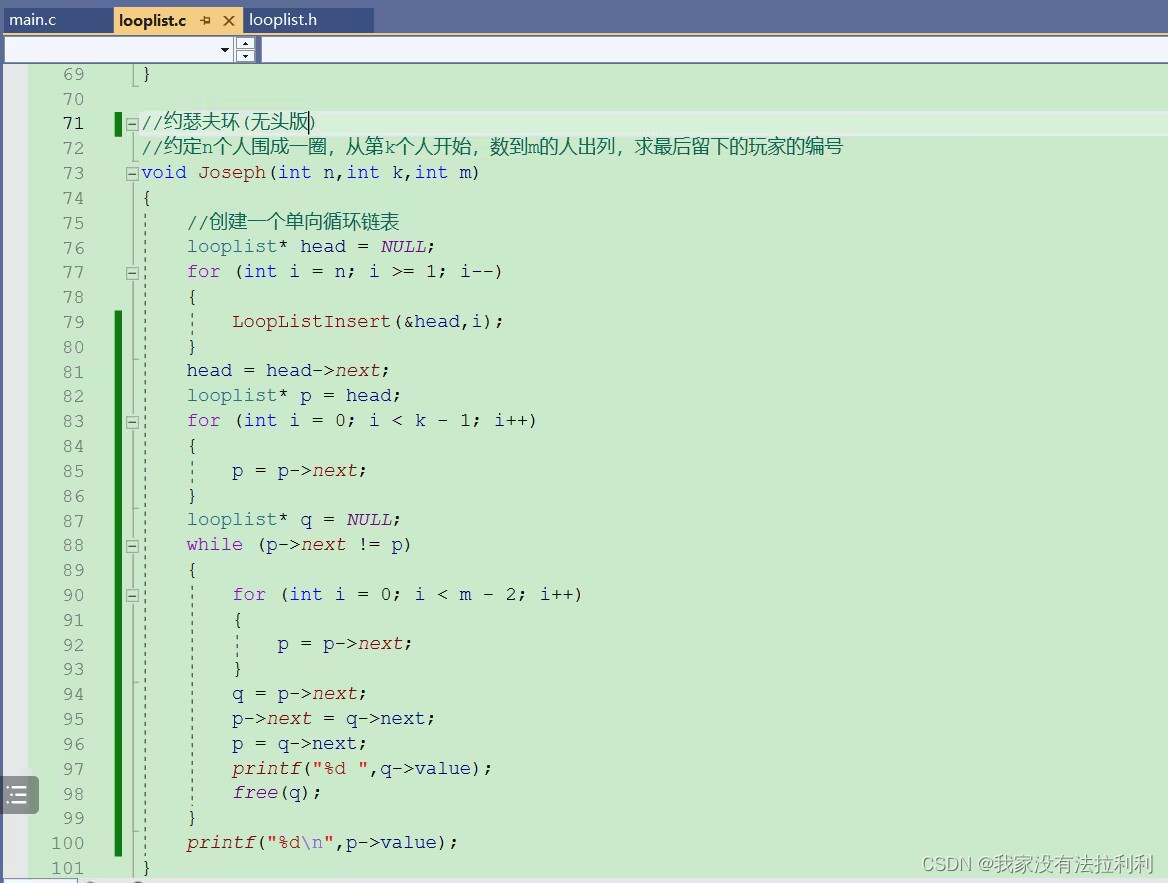
查找最小的五个元素
#include <stdio.h>
#include <stdlib.h>
/*
输入n个数,找出最小的五个数并打印,并且也要打印这五个数输入的位置(就是第几次输入的)
要求:不能使用排序
建议:使用单链表直接插入排序
*/
typedef struct node
{
int data; //输入的数据
int times; //保存第几次输入
struct node* next; //指针域
}node;
typedef struct linklist //无头单链表信息
{
node* headnode; //保存头结点的地址
int length; //保存无头单链表的长度
}linklist;
//创建一个空的单链表
linklist* LinklistCreate()
{
linklist* Info = (linklist*)malloc(sizeof(linklist));
if (Info == NULL)
{
printf("malloc failure!\n");
return NULL;
}
//赋初值
Info->headnode = NULL;
Info->length = 0;
return Info;
}
//直接插入排序
void Push_front_Sort(linklist *Info,int data)
{
if (Info == NULL)
{
printf("单链表初始化失败!\n");
return;
}
//申请一个新的结点
node* temp = (node*)malloc(sizeof(node));
if (temp == NULL)
{
printf("malloc failure!\n");
return;
}
//赋初值
static int times = 0;
temp->data = data;
temp->times = ++times;
temp->next = NULL;
node* p = Info->headnode;
//插入结点
if (p == NULL) //第一次插入结点
{
Info->headnode = temp;
Info->length++;
return;
}
//非第一次插入结点
if (p->data > data) //将结点插入到第一个结点的前面
{
temp->next = Info->headnode;
Info->headnode = temp;
return;
}
while ((p->data < data) && (p->next != NULL) && (p->next->data < data))
{
p = p->next;
}
temp->next = p->next;
p->next = temp;
Info->length++;
return;
}
void Print(linklist* Info)
{
if (Info == NULL)
{
printf("单链表初始化失败!\n");
return;
}
node* p = Info->headnode;
while (p != NULL)
{
printf("%d ", p->data);
p = p->next;
}
putchar(10);
}
void Print5(linklist *Info)
{
if (Info == NULL)
{
printf("单链表初始化失败!\n");
return;
}
node* p = Info->headnode;
for (int i = 0; i < 5; i++)
{
if(p != NULL)
{
printf("%d 是第%d次输入\n",p->data,p->times);
}
p = p->next;
}
}
int main(void)
{
linklist* Info = LinklistCreate();
srand(time(NULL));
int data;
for (int i = 0; i < 15; i++)
{
data = rand() % 100;
printf("%d ",data);
Push_front_Sort(Info, data);
}
putchar(10);
Print5(Info);
return 0;
}