简单记事本
实验目的:
-
掌握菜单的编写以及其事件处理;
-
掌握文本区的使用,换行,添加滚动条;
-
掌握对话框的编写以及和父窗体间传递数据;
-
掌握文件的读写操作。
实验内容:
-
仿照Windows中的记事本,编写一个Java程序记事本。
-
要求:有打开和保存文本文件,设置字体功能,其他功能根据自身能力添加。
效果图: 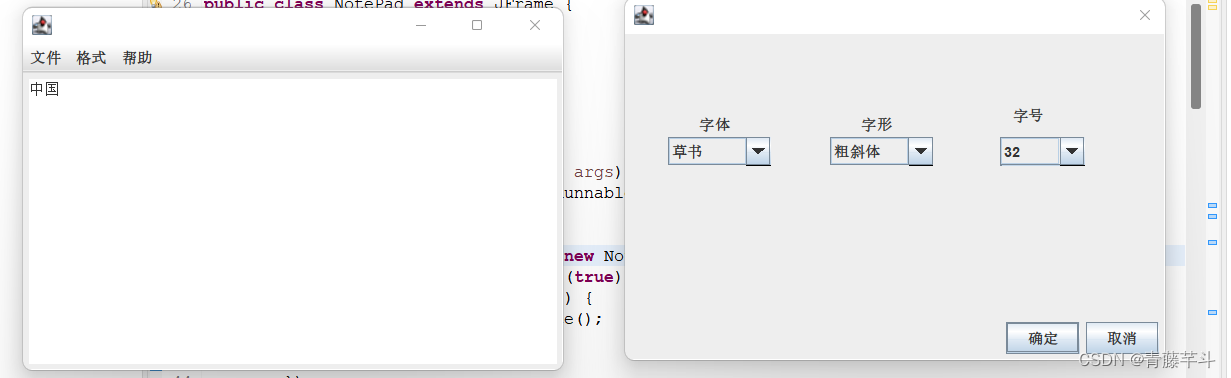
源码:
package notepad;
import java.awt.BorderLayout;
import java.awt.EventQueue;
import java.awt.Font;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import javax.swing.JTextArea;
import javax.swing.JMenuBar;
import javax.swing.JMenu;
import javax.swing.JMenuItem;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.awt.event.ActionEvent;
import javax.swing.JRadioButtonMenuItem;
import javax.swing.JScrollPane;
public class NotePad extends JFrame {
private JPanel contentPane;
JTextArea textArea;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
NotePad frame = new NotePad();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public NotePad() {
setAlwaysOnTop(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 450, 300);
JMenuBar menuBar = new JMenuBar();
setJMenuBar(menuBar);
JMenu mnNewMenu = new JMenu("文件");
menuBar.add(mnNewMenu);
JMenuItem mntmNewMenuItem = new JMenuItem("打开");
mntmNewMenuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
JFileChooser chooser = new JFileChooser();
chooser.showOpenDialog(NotePad.this);
File selectedFile = chooser.getSelectedFile();
textArea.setText("");
FileReader fReader = null;
BufferedReader bReader = null;
try {
fReader = new FileReader(selectedFile);
bReader = new BufferedReader(fReader);
String str ;
while((str = bReader.readLine())!=null) {
textArea.append(str + "\r\n");
}
} catch (FileNotFoundException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
} catch (IOException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}finally {
try {
if(bReader != null)bReader.close();
if(fReader != null)fReader.close();
} catch (IOException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}
}
});
mnNewMenu.add(mntmNewMenuItem);
JMenuItem mntmNewMenuItem_1 = new JMenuItem("保存");
mntmNewMenuItem_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
JFileChooser chooser = new JFileChooser();
chooser.showSaveDialog(NotePad.this);
File selectedFile = chooser.getSelectedFile();
try(FileWriter fWriter = new FileWriter(selectedFile);) {
fWriter.write(textArea.getText());
fWriter.flush();
} catch (IOException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}
});
mnNewMenu.add(mntmNewMenuItem_1);
JMenuItem mntmNewMenuItem_2 = new JMenuItem("关闭");
mntmNewMenuItem_2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
dispose();
}
});
mnNewMenu.add(mntmNewMenuItem_2);
JMenu mnNewMenu_1 = new JMenu("格式");
menuBar.add(mnNewMenu_1);
JRadioButtonMenuItem rdbtnmntmNewRadioItem = new JRadioButtonMenuItem("自动换行");
rdbtnmntmNewRadioItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
Boolean selected = rdbtnmntmNewRadioItem.isSelected();
if(selected) {
textArea.setLineWrap(true);
}else {
textArea.setLineWrap(false);
}
}
});
mnNewMenu_1.add(rdbtnmntmNewRadioItem);
JMenuItem mntmNewMenuItem_4 = new JMenuItem("字体");
mntmNewMenuItem_4.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
JFontChooser chooser = new JFontChooser();
int n = chooser.showFontDialog();
if(n== JFontChooser.APPROVE_OPTION) {
Font font = chooser.getSelectedFont();
textArea.setFont(font);
}
}
});
mnNewMenu_1.add(mntmNewMenuItem_4);
JMenu mnNewMenu_2 = new JMenu("帮助");
menuBar.add(mnNewMenu_2);
JMenuItem mntmNewMenuItem_5 = new JMenuItem("查看帮助");
mnNewMenu_2.add(mntmNewMenuItem_5);
JMenuItem mntmNewMenuItem_6 = new JMenuItem("关于");
mnNewMenu_2.add(mntmNewMenuItem_6);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(new BorderLayout(0, 0));
textArea = new JTextArea();
contentPane.add(textArea, BorderLayout.CENTER);
//contentPane.add(new JScrollPane(textArea), BorderLayout.CENTER);//设置滚动条
}
}
package notepad;
import java.awt.BorderLayout;
import java.awt.FlowLayout;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JDialog;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import javax.swing.JComboBox;
import javax.swing.DefaultComboBoxModel;
import javax.swing.JLabel;
public class JFontChooser extends JDialog {
//点击取消按钮
public static final int CANCEL_OPTION = 1;
//点击确定按钮
public static final int APPROVE_OPTION = 0;
private final JPanel contentPanel = new JPanel();
JComboBox fontComboBox;
JComboBox styleComboBox;
JComboBox sizeComboBox;
private Font font;
private int returnValue = CANCEL_OPTION ;
/**
* Launch the application.
*/
public static void main(String[] args) {
try {
JFontChooser dialog = new JFontChooser();
dialog.setDefaultCloseOperation(JDialog.DISPOSE_ON_CLOSE);
dialog.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
/**
* Create the dialog.
*/
public JFontChooser() {
setModal(true);//设置对话框为模态;只有按了按钮或关闭才会返回父窗体
setBounds(100, 100, 450, 300);
getContentPane().setLayout(new BorderLayout());
contentPanel.setBorder(new EmptyBorder(5, 5, 5, 5));
getContentPane().add(contentPanel, BorderLayout.CENTER);
contentPanel.setLayout(null);
fontComboBox = new JComboBox();
fontComboBox.setModel(new DefaultComboBoxModel(new String[] {"宋体", "楷体", "小篆", "草书"}));
fontComboBox.setBounds(35, 83, 83, 23);
contentPanel.add(fontComboBox);
styleComboBox = new JComboBox();
styleComboBox.setModel(new DefaultComboBoxModel(new String[] {"常规", "斜体", "粗体", "粗斜体"}));
styleComboBox.setBounds(166, 83, 83, 23);
contentPanel.add(styleComboBox);
sizeComboBox = new JComboBox();
sizeComboBox.setModel(new DefaultComboBoxModel(new String[] {"8", "9", "10", "11", "12", "14", "16", "18", "20", "24", "28", "32", "30"}));
sizeComboBox.setBounds(303, 83, 68, 23);
contentPanel.add(sizeComboBox);
JLabel lblNewLabel = new JLabel("字体");
lblNewLabel.setBounds(60, 65, 58, 15);
contentPanel.add(lblNewLabel);
JLabel lblNewLabel_1 = new JLabel("字形");
lblNewLabel_1.setBounds(191, 65, 58, 15);
contentPanel.add(lblNewLabel_1);
JLabel lblNewLabel_2 = new JLabel("字号");
lblNewLabel_2.setBounds(313, 58, 58, 15);
contentPanel.add(lblNewLabel_2);
{
JPanel buttonPane = new JPanel();
buttonPane.setLayout(new FlowLayout(FlowLayout.RIGHT));
getContentPane().add(buttonPane, BorderLayout.SOUTH);
{
JButton okButton = new JButton("确定");
okButton.addActionListener(new ActionListener() {
//@Override
public void actionPerformed(ActionEvent e) {
// TODO Auto-generated method stub
String selectedFont = (String) fontComboBox.getSelectedItem();
int selectedStyle = styleComboBox.getSelectedIndex();
int selectedSize = Integer.parseInt( (String) sizeComboBox.getSelectedItem());
font = new Font(selectedFont, selectedStyle, selectedSize);
returnValue = APPROVE_OPTION;
dispose();
}
});
okButton.setActionCommand("OK");
buttonPane.add(okButton);
getRootPane().setDefaultButton(okButton);
}
{
JButton cancelButton = new JButton("取消");
cancelButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
dispose();
}
});
cancelButton.setActionCommand("Cancel");
buttonPane.add(cancelButton);
}
}
}
public Font getSelectedFont() {
return font;
}
public int showFontDialog() {
setVisible(true);
return returnValue;
}
}