#include<iostream>
using namespace std;
//创建链表类
template<typename T>
class My_list {
using element = T;
struct node{
element ele;
node* next;
node* pre;
node() {
next = nullptr;
pre = nullptr;
}
};
public:
My_list():lenth(0) //初始化链表
{
head = tail = new node;
}
node* begin() {
return head->next;
}
node* end() {
return tail->next;
}
bool empty() {
return lenth==0;
}
void push_Back(element e) {
node* tmp = new node;
tmp->ele = e;
tmp->pre = tail;
tail->next = tmp;
tail = tmp;
lenth++;
}
void pop_Back() {
if (empty()) {
return;
}
else {
node* tmp=tail->pre;
delete tail;
tail = tmp;
lenth--;
}
}
void push_Front(element e) {
node* tmp = new node;
tmp->ele = e;
node* s = head->next;
tmp->next = head->next;
s->pre = tmp;
tmp->pre = head;
head->next = tmp;
}
void pop_Front() {
if (empty()) {
return;
}
node* tmp = head->next;
head->next = tmp->next;
if (tmp->next == nullptr)
{
tail = head;
}
else {
tmp->next->pre = head;
}
delete tmp;
lenth--;
}
void print_List() {
if (head->next == nullptr) {}
else {
node* ptr = new node;
ptr = head->next;
while (ptr!=nullptr) {
cout << ptr->ele << " ";
ptr = ptr->next;
}
}
}
My_list(const My_list& list) {
head = tail = new node;
node* ptr = list.head->next;
while (ptr != nullptr) {
push_Back(ptr->ele);
ptr = ptr->next;
}
}
void operator=(My_list list) {
My_list(list);
}
//定义链表指针
class iterator {
public:
node* it;
iterator(node* x) {//指针初始化
it = x;
}
element& operator*() {
return it->ele;
}
iterator& operator++() {
it = it->next;
return *this;
}
iterator& operator++(int) {
iterator tmp = *this;
it = it->next;
return tmp;
}
bool operator != (const iterator& it) const { // 重载运算符
return this->it != it.it;
}
};
public:
int lenth;//存放链表长度
node* head;
node* tail;
};
int main() {
}
手写list(c++)
最新推荐文章于 2024-04-23 17:37:57 发布
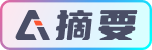