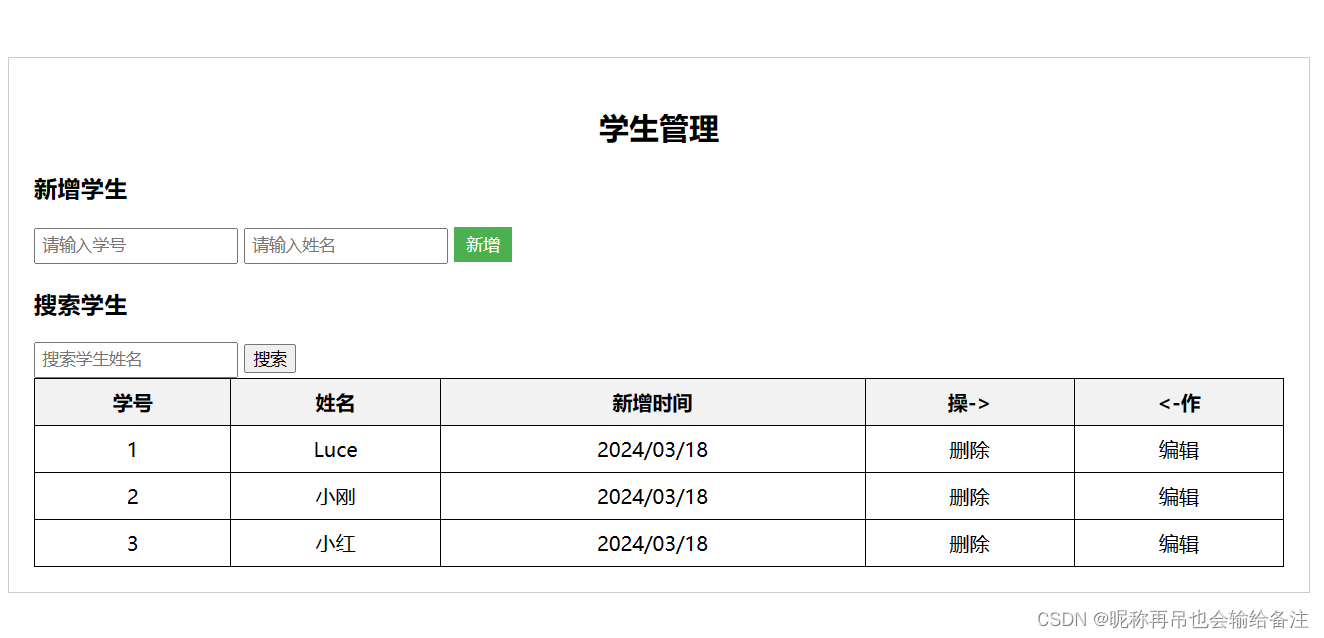
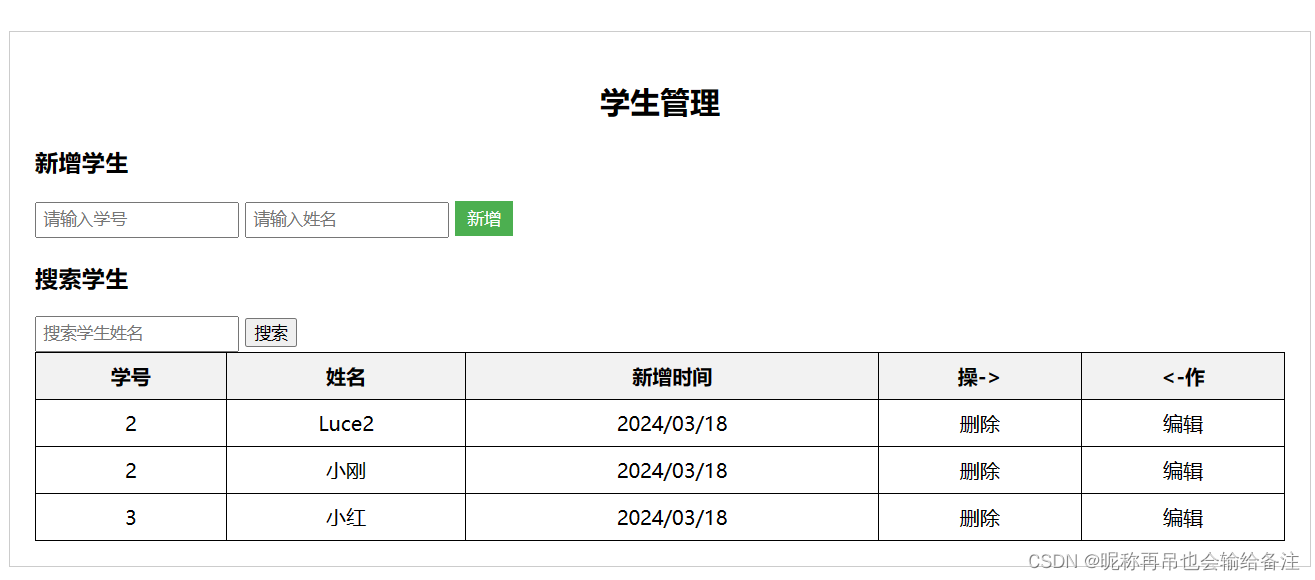
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>学生管理系统</title>
<script src="vue.js"></script>
</head>
<style>
body {
display: flex;
justify-content: center; /* 水平居中 */
align-items: center; /* 垂直居中 */
height: 100vh;
margin: 0;
}
#app {
width: 100%;
max-width: 1000px;
padding: 20px;
border: 1px solid #ccc;
}
table {
border-collapse: collapse;
width: 100%;
}
table thead tr th:nth-child(4){
}
th, td {
border: 1px solid black;
padding: 8px;
text-align: center;
}
th {
background-color: #f2f2f2;
}
input[type="text"] {
width: 150px;
padding: 5px;
}
input[type="button"] {
padding: 5px 10px;
background-color: #4CAF50;
color: white;
border: none;
cursor: pointer;
}
h2 {
display: flex;
justify-content: center; /* 水平居中 */
align-items: center; /* 垂直居中 */
}
.modal {
display: none;
position: fixed;
z-index: 1;
left: 0;
top: 0;
width: 100%;
height: 100%;
overflow: auto;
background-color: rgba(0,0,0,0.4);
}
.modal-content {
background-color: #fefefe;
margin: 15% auto;
padding: 20px;
border: 1px solid #888;
width: 80%;
}
.close {
color: #aaa;
float: right;
font-size: 28px;
font-weight: bold;
}
.close:hover,
.close:focus {
color: black;
text-decoration: none;
cursor: pointer;
}
</style>
<body>
<div id="app" v-cloak>
<h2>学生管理</h2>
<div>
<h3>新增学生</h3>
<input type="text" placeholder="请输入学号" v-model="newId">
<input type="text" placeholder="请输入姓名" v-model="newName">
<input type="button" value="新增" @click="addStudent">
</div>
<div>
<h3>搜索学生</h3>
<input type="text" placeholder="搜索学生姓名" v-model="searchKeyword">
<button @click="searchStudents">搜索</button>
</div>
<table>
<thead>
<tr>
<th>学号</th>
<th>姓名</th>
<th>新增时间</th>
<th>操-><th><-作</th></th>
</tr>
</thead>
<tbody>
<tr v-for="(student, index) in students" :key="index">
<td>{{ student.id }}</td>
<td>{{ student.name }}</td>
<td>{{ formatDate(student.time) }}</td>
<td><a @click.prevent="deleteStudent(index)">删除</a></td>
<!-- <td><a @click.prevent="editStudent(index)">编辑</a></td> -->
<td><a @click.prevent="openModal(index)">编辑</a></td>
</tr>
<!-- <div v-if="editIndex !== -1">
<input type="button" value="保存" @click="saveEdit">
</div> -->
</tbody>
</table>
<div id="myModal" class="modal">
<div class="modal-content">
<span class="close" @click="closeModal">×</span>
<h3>编辑学生信息</h3>
<input type="text" placeholder="请输入学号" v-model="newId">
<input type="text" placeholder="请输入姓名" v-model="newName">
<input type="button" value="保存" @click="saveEdit">
</div>
</div>
</div>
<script>
var vm = new Vue({
el: '#app',
data: {
newId: '',
newName: '',
searchKeyword: '',
editIndex: -1,
students: [
{ id: 1, name: "Luce", time: new Date() },
{ id: 2, name: "小刚", time: new Date() },
{ id: 3, name: "小红", time: new Date() }
]
},
methods: {
//添加功能
addStudent() {
var newStudent = {
id: this.newId,
name: this.newName,
time: new Date()
};
this.students.push(newStudent);
this.newId = this.newName = '';
},
//删除功能
deleteStudent(index) {
this.students.splice(index, 1);
},
// searchStudents() {
// let keyword = this.searchKeyword.toLowerCase();
// this.filteredStudents = this.students.filter(student => student.name.toLowerCase().includes(keyword));
// }
//搜索功能
searchStudents() {
let keyword = this.searchKeyword.toLowerCase();
if (keyword) {
this.students = this.students.filter(student => student.name.toLowerCase().includes(keyword));
} else {
// 如果搜索关键词为空,恢复显示所有学生
this.students = [
{ id: 1, name: "Luce", time: new Date() },
{ id: 2, name: "小刚", time: new Date() },
{ id: 3, name: "小红", time: new Date() }
];
}
},
// 格式化时间
formatDate(date) {
const d = new Date(date);
const year = d.getFullYear();
const month = ('0' + (d.getMonth() + 1)).slice(-2);
const day = ('0' + d.getDate()).slice(-2);
return `${year}/${month}/${day}`;
},
// 编辑功能
// editStudent(index) {
// this.editIndex = index;
// this.newId = this.students[index].id;
// this.newName = this.students[index].name;
// },
// 保存修改
// saveEdit() {
// this.students[this.editIndex].id = this.newId;
// this.students[this.editIndex].name = this.newName;
// this.editIndex = -1; // 重置编辑索引
// this.newId = this.newName = ''; // 清空输入框
// this.closeModal
// },
saveEdit() {
this.students[this.editIndex].id = this.newId;
this.students[this.editIndex].name = this.newName;
this.editIndex = -1; // 重置编辑索引
this.newId = this.newName = ''; // 清空输入框
this.closeModal(); // 关闭模态框
},
// 关闭模态框
closeModal() {
var modal = document.getElementById("myModal");
modal.style.display = "none";
},
// 打开模态框并加载学生信息
openModal(index) {
this.editIndex = index;
this.newId = this.students[index].id;
this.newName = this.students[index].name;
var modal = document.getElementById("myModal");
modal.style.display = "block";
},
// 关闭模态框
closeModal() {
var modal = document.getElementById("myModal");
modal.style.display = "none";
this.editIndex = -1; // 重置编辑索引
this.newId = this.newName = ''; // 清空输入框
},
},
})
</script>
</body>
</html>