gcc 版本 12.2.0
vscode 需要修改脚本 添加"-mwindows"编译指令
"-mwindows"
tasks.json
"args": [
"-fdiagnostics-color=always",
"-g",
"${file}",
"-o",
"${fileDirname}\\${fileBasenameNoExtension}.exe",
"-mwindows"
]
如果出现乱码修改系统设置
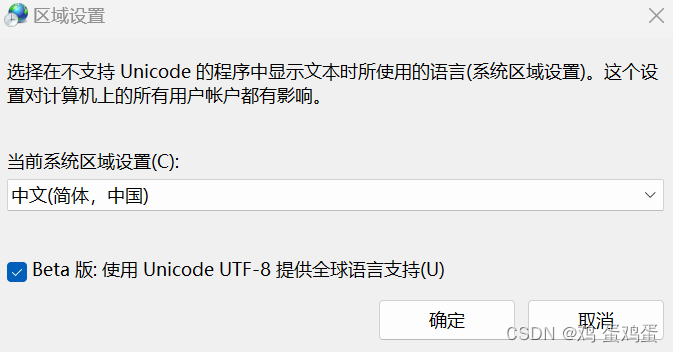
src
#include <windows.h>
#include <iostream>
#include <sstream>
HANDLE get_put=0;
#define MY_ONE_A WM_USER+1020
void print_painting(HWND hWnd);
void create_A(HWND hWnd);
void print_size_A(HWND hWnd,LPARAM lParam);
void print_def_message(HWND hWnd,WPARAM wParam,LPARAM lParam);
void keydown_key(HWND hWnd,WPARAM wParam);
void keyup_key(HWND hWnd,WPARAM wParam);
void distinguish_key_char(HWND hWnd,WPARAM wParam);
void lllbutton_down(HWND hWnd,WPARAM wParam,LPARAM lParam);
void lllbutton_up(HWND hWnd,WPARAM wParam,LPARAM lParam);
void rrrbutton_down(HWND hWnd,WPARAM wParam,LPARAM lParam);
void rrrbutton_up(HWND hWnd,WPARAM wParam,LPARAM lParam);
void mousemove(HWND hWnd,WPARAM wParam,LPARAM lParam);
WPARAM timer_bomb(HWND hWnd,WPARAM wParam);
std::string wm_rl(std::string xxx,WPARAM wParam,LPARAM lParam);
std::string wm_rl(std::string xxx,WPARAM wParam);
std::string wm_rl(std::string xxx,std::string xxx_2,std::string xxx_3,std::string xxx_4,WPARAM wParam,LPARAM lParam);
LRESULT CALLBACK WndProc(HWND hWnd,UINT msgld,WPARAM wParam,LPARAM lParam);
LRESULT CALLBACK WndProc(HWND hWnd,UINT msgld,WPARAM wParam,LPARAM lParam)
{
switch (msgld)
{
case WM_TIMER:
timer_bomb(hWnd,wParam);
break;
case WM_LBUTTONDOWN:
lllbutton_down(hWnd,wParam,lParam);
break;
case WM_LBUTTONUP:
lllbutton_up(hWnd,wParam,lParam);
break;
case WM_RBUTTONDOWN:
rrrbutton_down(hWnd,wParam,lParam);
break;
case WM_RBUTTONUP:
rrrbutton_up(hWnd,wParam,lParam);
break;
case WM_MOUSEMOVE:
mousemove(hWnd,wParam,lParam);
case WM_CHAR:
distinguish_key_char(hWnd,wParam);
break;
case WM_KEYDOWN:
keydown_key(hWnd,wParam);
break;
case WM_KEYUP:
keyup_key(hWnd,wParam);
break;
case MY_ONE_A:
print_def_message(hWnd,wParam,lParam);
break;
case WM_SIZE:
print_size_A(hWnd,lParam);
break;
case WM_CREATE:
SetTimer(hWnd,1,1000,NULL);
SetTimer(hWnd,2,1000,NULL);
create_A(hWnd);
break;
case WM_SYSCOMMAND:
if (wParam==SC_CLOSE)
{
int off_xxx=MessageBox(hWnd,"是否退出","?",MB_YESNO);
if (off_xxx==IDYES){}
else return 0;
}
break;
case WM_COMMAND:
if (LOWORD(wParam)==1)
{
HDC hdc=GetDC(hWnd);
RECT rect={45,45,60,60};
DrawText(hdc,"a",1,&rect,DT_LEFT);
ReleaseDC(hWnd,hdc);
}
break;
case WM_DESTROY:
PostMessage(hWnd,WM_QUIT,0,0);
break;
}
return DefWindowProc(hWnd,msgld,wParam,lParam);
}
WPARAM timer_bomb(HWND hWnd,WPARAM wParam)
{
WriteConsole(get_put,wm_rl("定时器:",wParam).c_str(),strlen(wm_rl("定时器:",wParam).c_str()),NULL,NULL);
return wParam;
}
void distinguish_key_char(HWND hWnd,WPARAM wParam)
{
WriteConsole(get_put,wm_rl("WM_CHAR:",wParam).c_str(),strlen(wm_rl("WM_CHAR:",wParam).c_str()),NULL,NULL);
}
void keyup_key(HWND hWnd,WPARAM wParam)
{
WriteConsole(get_put,wm_rl("WM_KEYUP:",wParam).c_str(),strlen(wm_rl("WM_KEYUP:",wParam).c_str()),NULL,NULL);
}
void keydown_key(HWND hWnd,WPARAM wParam)
{
WriteConsole(get_put,wm_rl("WM_KEYDOWN:",wParam).c_str(),strlen(wm_rl("WM_KEYDOWN:",wParam).c_str()),NULL,NULL);
}
void print_size_A(HWND hWnd,LPARAM lParam)
{
short _width=LOWORD(lParam);
short _height=HIWORD(lParam);
std::stringstream ss;
ss<<"W:"<<_width<<"|H:"<<_height<<std::endl;
std::string text=ss.str();
const char *_text_a=text.c_str();
WriteConsoleA(get_put,_text_a,strlen(_text_a),NULL,NULL);
}
void print_def_message(HWND hWnd,WPARAM wParam,LPARAM lParam)
{
std::stringstream de;
de<<"自定义消息"<<wParam<<"|"<<lParam;
std::string texts=de.str();
MessageBox(hWnd,texts.c_str(),"",MB_OK);
}
void create_A(HWND hWnd)
{
PostMessage(hWnd,MY_ONE_A,1,1);
MessageBox(NULL,"create","a",MB_OK);
CreateWindowEx
(
0,
"EDIT",
"aaaaa",
WS_CHILD|WS_VISIBLE|WS_BORDER,
0,
0,
200,
200,
hWnd,
NULL,
0,
NULL
);
}
void print_painting(HWND hWnd)
{
PAINTSTRUCT ps={0};
HDC hdc=BeginPaint(hWnd,&ps);
HBRUSH hBrush=CreateSolidBrush(RGB(255,0,0));
HGDIOBJ def_f = SelectObject(hdc,hBrush);
Ellipse(hdc,0,0,100,100);
EndPaint(hWnd,&ps);
SelectObject(hdc,def_f);
DeleteObject(hBrush);
}
std::string wm_rl(std::string xxx,WPARAM wParam)
{
std::stringstream key_char;
key_char<<std::endl<<xxx<<" "<<wParam;
std::string text_key=key_char.str();
return text_key;
}
std::string wm_rl(std::string xxx,WPARAM wParam,LPARAM lParam)
{
std::stringstream key_char;
key_char<<std::endl<<xxx<<" | 摁键状态 | "<<wParam<<" | "<<"X | "<<LOWORD(lParam)<<"Y |"<<HIWORD(lParam);
std::string text_key=key_char.str();
return text_key;
}
std::string wm_rl(std::string xxx,std::string xxx_2,std::string xxx_3,std::string xxx_4,WPARAM wParam,LPARAM lParam)
{
std::stringstream key_char;
key_char<<std::endl<<xxx<<" | "<<xxx_2<<" | "<<wParam<<" | "<<xxx_3<<" | "<<LOWORD(lParam)<<xxx_4<<" |"<<HIWORD(lParam);
std::string text_key=key_char.str();
return text_key;
}
void lllbutton_down(HWND hWnd,WPARAM wParam,LPARAM lParam)
{
WriteConsole(get_put,wm_rl("WM_LBUTTONDOWN",wParam,lParam).c_str(),strlen(wm_rl("WM_LBUTTONDOWN",wParam,lParam).c_str()),NULL,NULL);
}
void lllbutton_up(HWND hWnd,WPARAM wParam,LPARAM lParam)
{
WriteConsole(get_put,wm_rl("WM_LBUTTONUP",wParam,lParam).c_str(),strlen(wm_rl("WM_LBUTTONUP",wParam,lParam).c_str()),NULL,NULL);
}
void rrrbutton_down(HWND hWnd,WPARAM wParam,LPARAM lParam)
{
WriteConsole(get_put,wm_rl("WM_RBUTTONDOWN",wParam,lParam).c_str(),strlen(wm_rl("WM_RBUTTONDOWN",wParam,lParam).c_str()),NULL,NULL);
}
void rrrbutton_up(HWND hWnd,WPARAM wParam,LPARAM lParam)
{
WriteConsole(get_put,wm_rl("WM_RBUTTONUP",wParam,lParam).c_str(),strlen(wm_rl("WM_RBUTTONUP",wParam,lParam).c_str()),NULL,NULL);
}
void mousemove(HWND hWnd,WPARAM wParam,LPARAM lParam)
{
WriteConsole(get_put,wm_rl("鼠标移动消息","鼠标移动消息状态","x","y",wParam,lParam).c_str(),strlen(wm_rl("鼠标移动消息","鼠标移动消息状态","x","y",wParam,lParam).c_str()),NULL,NULL);
}
int WINAPI WinMain(_In_ HINSTANCE hInstance,_In_opt_ HINSTANCE hPrevInstance,_In_ LPSTR lpCmdLine,_In_ int nShowCmd)
{
AllocConsole();
get_put=GetStdHandle(STD_OUTPUT_HANDLE);
WNDCLASS windows_x={0};
windows_x.cbClsExtra=0;
windows_x.cbWndExtra=0;
windows_x.hbrBackground=(HBRUSH)GetStockObject(WHITE_BRUSH);
windows_x.hCursor=NULL;
windows_x.hIcon=NULL;
windows_x.hInstance=hInstance;
windows_x.lpfnWndProc=WndProc;
windows_x.lpszClassName=TEXT("mainss");
windows_x.lpszMenuName=NULL;
windows_x.style=CS_HREDRAW|CS_VREDRAW|CS_DBLCLKS;
RegisterClass(&windows_x);
HWND hWnd=CreateWindow
(
TEXT("mainss"),
TEXT("mainss_windows"),
WS_OVERLAPPEDWINDOW,
0,
0,
1024,
768,
NULL,
NULL,
hInstance,
NULL
);
CreateWindow
(
"BUTTON",
"Press Me",
WS_CHILD|WS_VISIBLE|BS_PUSHBUTTON,
150,100,100,50,
hWnd,
(HMENU)1,
NULL,
NULL
);
ShowWindow(hWnd,SW_SHOW);
UpdateWindow(hWnd);
MSG msg={0};
while (1)
{
if (PeekMessage(&msg,NULL,0,0,PM_NOREMOVE))
{
if(GetMessage(&msg,NULL,0,0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}else return 0;
}
else
{
}
}
return 0;
}